Python Program To Convert Celsius To Fahrenheit (+ Code Examples)
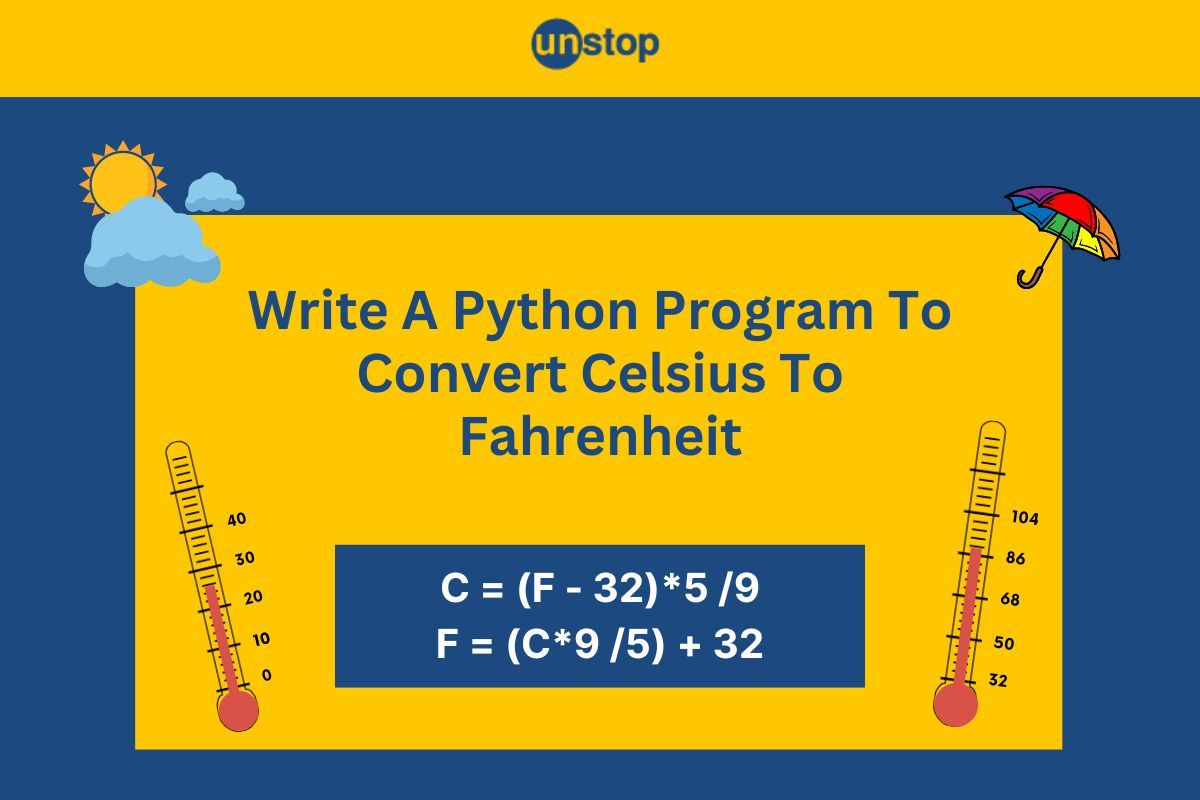
Temperature conversion is a fundamental concept in everyday life, especially in scientific and engineering applications. The Python programming language offers multiple solutions for performing and understanding temperature conversions effortlessly. Celsius(℃) and Fahrenheit(℉) are the temperature scales that are commonly used units of temperature measurement. These two scales are commonly used to express temperatures, but they originate from different reference points and have distinct mathematical formulas for conversion.
In this article, we will explore how to write a Python program to convert Celsius to Fahrenheit. We will cover both directions of conversions, i.e., from Fahrenheit to Celsius and from Celsius to Fahrenheit, with concise code examples. The Python programs will utilize these conversion formulas, allowing users to input temperatures on one scale and obtain their corresponding values on the other scale.
Celsius To Fahrenheit And Fahrenheit To Celsius Conversion Formulas
Before writing a Python program to convert Celsius to Fahrenheit, we must understand the conversion formulas essential for accurate temperature conversion. Let's explore the conversion formulas for both Celsius to Fahrenheit and Fahrenheit to Celsius.
Celsius To Fahrenheit Conversion
To convert a temperature from Celsius (C) to Fahrenheit (F), you can use the following formula:
Fahrenheit(°F) = (Celsius(°C) × 9/5) + 32
In the above formula, we multiply the Celsius temperature by 9/5 and then add it to 32. Look at the mathematical example below.
Example: Let’s convert 25°C to Fahrenheit using the formula: Fahrenheit(°F) = (Celsius(°C) × 9/5) + 32
Fahrenheit(°F) = (25°C × 9/5) + 32
Fahrenheit(°F) = (45) + 32
Fahrenheit(°F) = 77°F
Fahrenheit To Celsius Conversion
Converting from Fahrenheit to Celsius involves a slightly different formula. The formula for Fahrenheit to Celsius conversion is:
Celsius(°C) = (Fahrenheit(°F) - 32) × 5/9
In the above formula, we first subtract 32 from the Fahrenheit temperature and then multiply by 5/9. For example, let’s convert 98.6°F to Celsius using the formula provided above.
Celsius(°C) = (Fahrenheit(°F) - 32) × 5/9
Celsius(°C) = (98.6°F - 32) × 5/9
Celsius(°C) = (66.6) × 5/9
Celsius(°C) = 37°C.
Approach To Write A Python Program To Convert Celsius To Fahrenheit
Named after the Swedish astronomer Anders Celsius, the degree Celsius (also known as Centigrade) serves as the SI unit of measurement for temperature. The algorithm below provides a clear sequence of steps to follow when writing a Python program to convert Celsius to Fahrenheit.
Algorithm/ Approach to Write Python Program to Convert Celsius to Fahrenheit:
Step 1- Input: Firstly, prompt the user to enter the temperature in Celsius. This is in case we require user-generated input.
Step 2- Conversion: Apply the conversion formula, i.e., Fahrenheit(°F) = (Celsius(°C) × 9/5) + 32 to convert Celsius to Fahrenheit.
Step 3- Output: Print the converted temperature in Fahrenheit.
The approach is pretty straightforward and simple. We will explore various Python programs to make these calculations, but first, let's look at a basic Python example.
Code Example:
Output:
Enter the temperature in Celsius: 25.
25.00 degrees Celsius is equal to 77.00 degrees Fahrenheit.
Code Explanation:
In the above source code,
- We begin by prompting the user to input a temperature value in Celsius using the input() function, which is then converted to a floating-point number using float() and stored in the variable celsius.
- Next, we apply the conversion formula (celsius * 9/5) + 32 to convert the Celsius temperature to Fahrenheit, storing the result in the variable fahrenheit.
- Finally, we display the original Celsius temperature and its equivalent Fahrenheit temperature using the print() function and formatted string.
- Inside the formatted string, the interpolation {} is the placeholder for value insertion, and the .2f format specifier displays the values up to 2 decimal places.
- The output message provides a clear indication of the original and converted temperatures in both Celsius and Fahrenheit units.
Python Program To Convert Celsius To Fahrenheit With Predefined Value
In this approach to writing a Python program to convert Celsius to Fahrhite temperature unit, we make use of a predefined value. And we employ the simple conversion formula to convert that into Fahrenheit. That is, the program directly computes and displays the corresponding Fahrenheit temperature for the given Celsius value. This approach allows for quick and automated conversions without user input.
Let's look at an example Python program that provides a clear sequence of steps to achieve accurate Celsius to Fahrenheit conversions using this simple approach.
Code Example:
Output:
Original Celsius temperature: 37°C
Equivalent Fahrenheit temperature: 98.60°F
Code Explanation:
In the example Python code-
- We begin by defining a variable celsius and assigning the value 37 to it. This is the predefined Celsius temperature, which will convert to Fahrenheit.
- Next, we use the conversion formula (celsius * 9/5) + 32 to convert Celsius to Fahrenheit and store the outcome in the variable fahrenheit.
- After that, we use the print() function twice to display the original Celsius temperature and the equivalent Fahrenheit temperature using formatted string interpolation ({}) to display them.
- The original Celsius temperature is printed without any decimal places.
- The equivalent Fahrenheit temperature is printed with the .2f format specifier to display it up to 2 decimal places.
Python Program To Convert Celsius To Fahrenheit With User-Defined Input
Just like we discussed in this basic approach to write a Python program to convert Celsius to Fahrenheit, we take input from the user in Celsius temperature. Then, the conversion formula is applied to get its equivalent in Fahrenheit. In the example Python program below, we display both the entered Celsius value and its Fahrenheit equivalent, allowing interactive temperature conversion.
Code Example:
Output:
Enter temperature in Celsius: 37
Original Celsius temperature: 37.00°C
Equivalent Fahrenheit temperature: 98.60°F
Code Explanation:
In the sample Python code-
- We prompt the user to input a temperature value in Celsius using the input() function and convert that input to floating-point using the float() function. This is stored in the variable called celsius.
- Next, we convert the inputted Celsius temperature to Fahrenheit using the conversion formula (celsius * 9/5) + 32, as mentioned in the code comments.
- The converted temperature in Fahrenheit is stored in the variable fahrenheit.
- We print out both the original Celsius temperature and the equivalent Fahrenheit temperature using formatted string interpolation ({}) and the .2f format specifier to display them up to 2 decimal places inside the print() function.
Python Program To Convert Celsius To Fahrenheit Using NumPy Arrays
The Python NumPy library's primary functionality is its arrays, which offer a flexible and effective approach to working with uniform data arrays. You may use them to manipulate data, execute element-wise operations, and perform mathematical calculations on large arrays without using explicit loops.
- Compared to standard Python lists, NumPy arrays are faster and easier to read in code.
- NumPy arrays are helpful in the context of converting Celsius to Fahrenheit using Python by enabling simplified and vectorized calculations.
- Instead of performing separate computations inside of loops, you can simply apply the Fahrenheit conversion algorithm to a NumPy array of Celsius temperatures.
- This method simplifies the code, boosts productivity, and makes the conversion process clear and readable.
Below is a Python program example to convert Celsius to Fahrenheit by using NumPy arrays.
Code Example:
Output:
25.00°C is equal to 77.00°F
30.50°C is equal to 86.90°F
10.20°C is equal to 50.36°F
15.70°C is equal to 60.26°F
28.30°C is equal to 82.94°F
Code Explanation:
In the Python code example-
- We begin by importing the NumPy library as np to utilize its functionalities.
- Then, we create a NumPy array celsius_array containing several Celsius temperature values.
- Using NumPy's array broadcasting, we perform element-wise conversion of Celsius to Fahrenheit.
- For this we use the formula (celsius_array * 9/5) + 32, resulting in a new NumPy array fahrenheit_array containing the converted temperatures.
- After that, we use a for loop to iterate through the indices of celsius_array with the range(len(celsius_array)) construct.
- Within each iteration, we display each Celsius temperature from celsius_array and its corresponding Fahrenheit temperature from fahrenheit_array using print() function. The output values are formatted to display two decimal places.
Python Program To Convert Celsius To Fahrenheit Using Function
In this approach to writing a Python program to convert Celsius to Fahrenheit, we create a Python function (say, for example, celsius_to_fahrenheit()) that accepts a Celsius temperature as an input. It uses the conversion formula Fahrenheit (°F) = (Celsius (°C) *9/5)+ 32 and outputs the corresponding Fahrenheit temperature.
Below is a sample Python program where we employ a user-defined function to convert Celcius to Fahrenheit.
Code Example:
Output:
Enter temperature in Celsius: 25
25.00°C is equal to 77.00°F
Code Explanation:
In the Python code sample-
- We first define a function named celsius_to_fahrenheit, which takes a temperature value in Celsius as an argument. Inside the function-
- The Celsius temperature is converted to Fahrenheit using the formula (celsius * 9/5) + 32.
- The result, the temperature in Fahrenheit, is returned from the function.
- Next, we prompt the user to input a temperature value in Celsius using the input() function, which is then converted to a float.
- We then call the celsius_to_fahrenheit() function with the inputted Celsius temperature as an argument to perform the conversion.
- The converted temperature in Fahrenheit is stored in fahrenheit_output variable.
- Finally, we use the print() function to display both the original Celsius temperature and the converted Fahrenheit temperature with formatted string interpolation to display them up to 2 decimal places.
Python Program To Convert Celsius To Fahrenheit Using Class
A class in Python is an example of creating objects with specific features and characteristics. In order to promote modularity, reusability, and abstraction, the conversion formula and associated methods for Celsius to Fahrenheit conversion can be defined in a class.
- By designing a class, you provide a structured method for converting temperatures, which organizes the code and makes it simpler to maintain.
- This enables you to use the class's methods to effectively handle the conversion process by creating instances of the class, each of which represents a conversion situation.
In the Python example code below, we will write a Python program to convert temperatures in Celsius and Fahrenheit scales using class.
Code Example:
Output:
Enter temperature in Celsius: 33
33.00°C is equal to 91.40°F
Code Explanation:
In the provided Python code-
- We define a class named TemperatureConverter to encapsulate temperature conversion functionalities.
- Within the class, there's a method celsius_to_fahrenheit(), that accepts a temperature value in Celsius as an argument.
- Inside the method, the conversion formula (celsius * 9/5) + 32 is applied to convert Celsius to Fahrenheit.
- The result, the temperature in Fahrenheit, is returned from the method.
- Moving to the main part of the program, we instantiate an object of the TemperatureConverter class named converter.
- Then, we prompt the user to input a temperature value in Celsius using the input() function, which is converted to a float.
- Following this, we call the celsius_to_fahrenheit() method of the converter object with the inputted Celsius temperature as an argument to perform the conversion.
- The converted temperature in Fahrenheit is stored in fahrenheit_output variable.
- Finally, we use print() to display the original Celsius temperature and the converted Fahrenheit temperature with formatted string interpolation to display them up to 2 decimal places.
Python Program To Convert Fahrenheit To Celsius
The Fahrenheit unit of temperature is named after the Polish-born German physicist Daniel Gabriel Fahrenheit. As mentioned before, we can convert Fahrenheit to Celsius in Python by using the conversion formula given below:
Celsius(°C) = (Fahrenheit(°F) - 32) × 5/9.
This formula provides an accurate way to convert temperatures between the Fahrenheit and Celsius scales within your Python programs. Below is an example of a Python program that can carry out this conversion between the units of temperature.
Code Example:
Output:
Enter temperature in Fahrenheit: 77
Original Fahrenheit temperature: 77.00°F
Equivalent Celsius temperature: 25.00°C
Code Explanation:
In the Python code-
- We prompt the user to input a temperature in Fahrenheit using the input() function.
- Then, we convert the inputted temperature from Fahrenheit to Celsius using the formula (fahrenheit - 32) * 5/9.
- The converted temperature in Celsius is stored in the variable celsius.
- We then print out the original Fahrenheit temperature using formatted string interpolation ({}) and the .2f format specifier to display the temperature up to 2 decimal places.
- Similarly, we print out the equivalent Celsius temperature using the same formatting technique and print() function.
This code snippet efficiently takes user input in Fahrenheit, converts it to Celsius using the formula, and then presents both temperatures to the user.
Conclusion
In conclusion, Celsius, Fahrenheit, and Kelvin are the most commonly used units for temperature measurement. Understanding the fundamentals of these units and the methods to convert one to another has a wide range of applications in the mathematical as well as programming world. We have discussed the various ways we can write a Python program to convert Celsius to Fahrenhite in this article.
Python provides various methods for carrying out these conversions, including utilizing libraries like NumPy for efficient calculations, working with arrays to handle multiple temperature values at once, using functions to encapsulate the conversion logic, and even creating a dedicated class for temperature conversion operations. These different approaches offer flexibility and allow programmers to choose the most suitable method based on their specific requirements and coding style.
Frequently Asked Question
Q. How do you convert Celsius to Kelvin in Python?
Kelvin is an absolute temperature scale where 0 K represents absolute zero, equivalent to -273.15°C. Converting Celsius to Kelvin in Python involves adding 273.15 to the Celsius temperature. The straightforward formula (\( Kelvin (K) = Celsius (°C) + 273.15 \)) allows for direct conversion between the two scales. Below is an example Python program where we convert temperature in Celsius to Kelvin.
Code Example:
Output:
Enter temperature in Celsius: 37
37.00°C is equal to 310.15 K
Code Explanation:
In the conversion Python example-
- We first prompt the user to input a temperature value in Celsius degrees. Then, using the input() function and the float() function, we take the input value and convert it into a floating-point number, which is ultimately stored in the celsius variable.
- Following this, we apply the conversion formula for Celsius to Kelvin. We add 273.15 to the Celsius temperature to get the equivalent Kelvin temperature. This is stored in the kelvin variable.
- Then, we use the print() function to display the original Celsius temperature (celsius variable) and its equivalent Kelvin temperature (kelvin variable) to the console.
Q. What is the formula for temperature scales?
The formula to convert temperature from Celsius to Fahrenheit is:
Fahrenheit (°F) = (Celsius (°C) *9/5) + 32
The formula to convert temperature from Fahrenheit to Celsius is:
Celsius (°C) = (Fahrenheit (°F) - 32) *9/5
Q. How can I ensure consistent precision in the converted temperatures?
To maintain consistent precision, you can use string formatting techniques like specifying the number of decimal places in the formatted output, such as {:.2f} for two decimal places. Let's have a look at the example below to see how this can be done.
Code Example:
Output:
Original Celsius temperature: 25.00°C
Equivalent Fahrenheit temperature: 77.00°F
Code Explanation:
- In the first step, the code initializes a variable celsius with the value 25.0, representing a temperature in Celsius.
- Next, the conversion formula is applied to the celsius value to calculate the equivalent temperature in Fahrenheit. The result is assigned to the variable fahrenheit.
- At last, the original Celsius temperature and its equivalent Fahrenheit temperature are displayed to the user using formatted string literals (f-strings) inside the print() function.
- The .2f format specifier inside the curly braces specifies that both temperatures should be displayed with two decimal places.
Q. What is the purpose of converting temperatures using NumPy arrays?
Most temperature conversions are obtained from the efficient element-wise operations made possible by NumPy arrays. They make the conversion process by allowing you to apply a formula to a wide range of temperatures in a single step.
Additionally, using NumPy arrays for temperature conversions enhances code readability and maintainability, as it eliminates the need for explicit loops and simplifies the overall implementation. This approach also takes advantage of NumPy's optimized computations, resulting in faster and more efficient temperature conversion calculations than traditional iterative methods to write a Python program to convert Celsius to Fahrenheit.
You must also read the following:
- Relational Operators In Python | 6 Types, Usage, Examples & More!
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment