Python Program To Convert Decimal To Binary, Octal And Hexadecimal
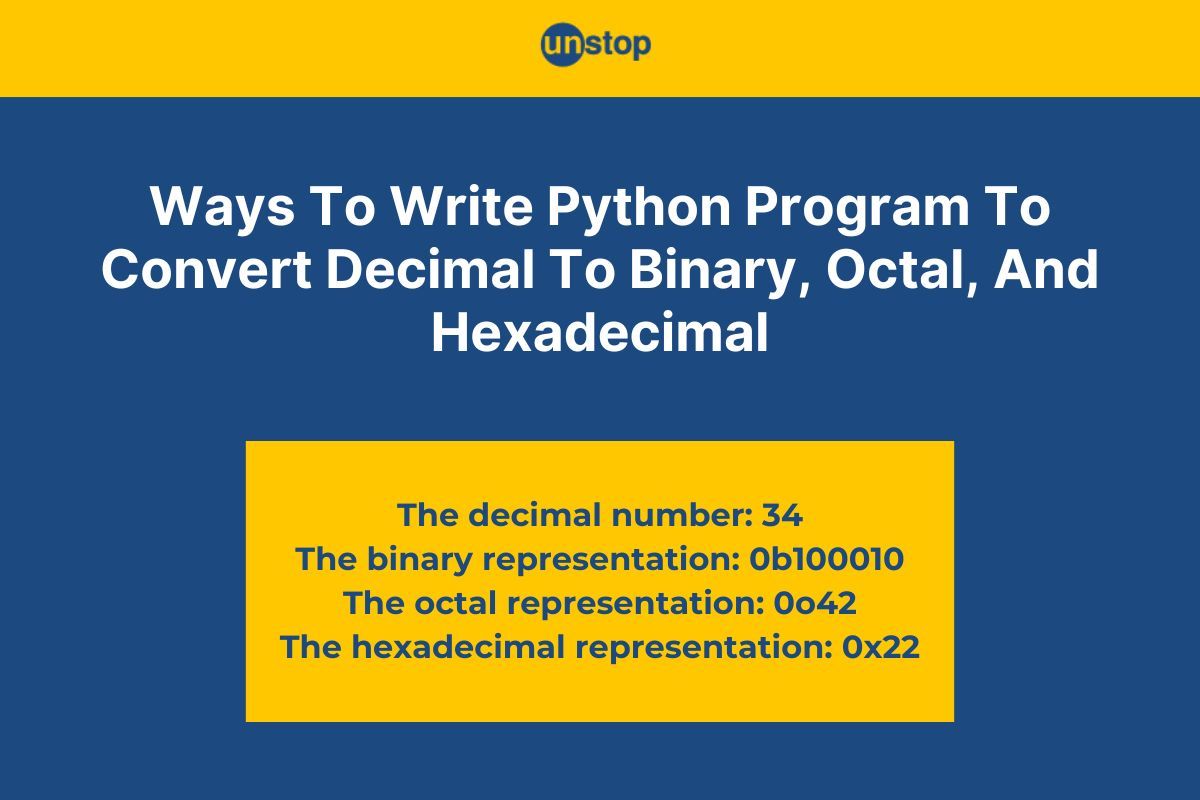
Decimal, binary, octal, and hexadecimal are different ways to represent numbers, making them an integral part of the number system/ digital system. They are used in computing to represent numeric values. While decimal is the base-10 numeral system we're most familiar with (0-9), binary is base-2 (0-1), octal is base-8 (0-7), and hexadecimal is base-16 (0-9, A-F). In this article, we'll explore various techniques for writing a Python program to convert decimal to binary, octal, and hexadecimal. But first, let's look at a few basics.
What Is Decimal Number System?
The decimal number system has a base of 10, and it contains 10 digits from 0 to 9. That is, these digits are used to represent numbers in this number system. Each digit in a decimal number has a value according to its position. Every subsequent digit is 10 times greater than the previous digit while moving from right to left. For example, (257)10 is represented as:
(257)10 = 102 .2 + 101 .5 + 100 .7 = 200 + 50 + 7 = 257
What Is Binary Number System?
The binary number system has a base 2, and it contains only two digits, i.e., 0 and 1. These two digits are known as bits. The group of 4 bits is called a nibble, and the group of 8 bits is called a byte. For example, (1011)2 is represented in decimal as:
(1011)2 = 23 .1 + 22 .0 + 21 .1 + 20 .1 = 8 + 0 + 2 + 1 = (11)10
The process to convert decimal to binary is as follows (example 8):
Divide the decimal number by 2 |
0 |
Use the quotient obtained in the previous step as a dividend and divide by 2 |
0 |
Repeat the second step until the quotient becomes 0 |
0 |
1/2 = 0 |
1 |
Write the remainder in reverse chronological order (bottom to top) |
|
What Is Octal Number System?
The octal number system has a base 8, and it contains 8 digits from 0 to 7. In the octal number system, each place is a power of 8. For example, (713)8 is represented in decimal as:
(713)8 = 82 .7 + 81 .1 + 80 .3 = 448 + 8 + 3 = (459)10
The steps to convert decimal to octal are as follows (example 356):
Divide the decimal number by 8 |
4 |
Use the quotient obtained in the previous step as a dividend and repeat |
4 |
Repeat the second step until the quotient becomes 0 |
5 |
Write the remainder in reverse chronological order (bottom to top) |
|
What Is Hexadecimal Number System?
The hexadecimal is an alphanumeric number system that has base 16, and it contains 10 digits from 0 to 9 and 6 alphabets from A to F. Here, the alphabets A to F represent the values from 10 to 15, where A represents 10, B represents 11 and so on. For example, (13B)16 is represented in decimal as:
(13B)16 = 162 .1 + 161 .3 + 160 .B = 144 + 48 + 11 = (203)10
The steps to convert decimal to hexadecimal numbers are given below (example 974).
Divide the decimal number by 16 |
14 (=E) (Remainder) |
Use the quotient obtained in the previous step as a dividend and continue dividing by 16 until the quotient becomes 0. |
6 |
Write remainders in reverse chronological order (bottom to top) |
|
Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
We can create a Python program to convert decimal to binary, octal, and hexadecimal by using built-in functions provided in Python. The built-in functions used for this purpose are (where n is the decimal number we want to convert):
- bin(): This function converts a decimal number to its equivalent binary number.
- oct(): This function converts a decimal number to its equivalent octal number.
- hex(): This function converts a decimal number to its equivalent hexadecimal number.
The approach for this conversion is as follows:
- First of all, we start by taking a decimal number as input from the user and storing it in a variable number.
- Then, we convert num into its equivalent binary number by passing num as a parameter of the built-in function bin(). We print the obtained binary number as output.
- After that, we pass num as a parameter in the built-in function oct() to convert num into an octal number and print it as output.
- Again, we pass num as a parameter in the built-in function hex() to obtain a hexadecimal representation of num and print it on the screen.
In this way, we can convert decimal to other number formats using built-in functions. Below is a simple Python program to convert decimal to binary, octal, and hexadecimal.
Code Example:
Output:
Enter a number: 46
The decimal number: 46
The binary representation: 0b101110
The octal representation: 0o56
The hexadecimal representation: 0x2e
Explanation:
In the Python code example-
- We create a variable x and prompt the user to input a decimal number using the input() function, which we then convert to integer data type using int() function.
- Next, we utilize built-in Python functions bin(), oct(), and hex() to convert the decimal number x into its binary, octal, and hexadecimal representations, respectively.
- The binary representation of x is stored in the variable binary_representation.
- The octal representation of x is stored in the variable octal_representation.
- The hexadecimal representation of x is stored in the variable hexadecimal_representation.
- Finally, we print the original decimal number x, along with its binary, octal, and hexadecimal representations, using a set of print() statements.
Time Complexity: O(1)
Space Complexity: O(1)
Python Program To Convert Decimal To Binary Using Recursion
A function that repeatedly calls itself is called recursion or recursive function. In recursion, the function breaks the original problem into smaller subproblems and solves those subproblems until the base case is reached. Then, the recursion returns its result without further calling itself.
A decimal number can be converted to a binary number by using recursion. The steps for this approach are as follows:
- Firstly, define a function conversion(), which takes a decimal number n as an argument.
- Then, define the condition that if n is greater than 1, then apply recursion. Within this, call the conversion() function and pass the floor division of n by 2, which provides an integer quotient as an argument.
- After the if condition is executed, print the remainder of the division of n by 2. By this, the binary digits will be printed in reverse order due to the recursive call.
Syntax:
def conversion(n):
if n > 1:
conversion(n//2)
print(n % 2,end = '')
Here,
- conversion(): Function to convert decimal to binary
- n: Decimal number to be converted to binary
- print(): Function to print the binary digits
Look at the Python program example to convert decimal to binary given below, for a better understanding of how to use this approach.
Code Example:
Output:
Enter a number: 13
1101
Explanation:
In the sample Python code-
- We begin by defining a function convertToBinary() that takes an integer n as input.
- Inside the function, there's a recursive call to convertToBinary(n // 2) to convert the quotient of n divided by 2 recursively to binary.
- We use an if-statement for the base case to check if n is less than or equal to 1. If the condition is true, then the recursion stops.
- If the condition is false, we move forward with recursion, during which the remainder of the divisions are printed in reverse order to get the binary representation. (We use the modulo arithmetic operator here)
- The end='' parameter in print() ensures that the output is printed without a newline character.
- Outside the function, we prompt the user to input a decimal number using input(), convert it to an integer and store it in the variable x.
- Next, we call the convertToBinary() function to convert the decimal number x to binary.
- Finally, we print a newline character to ensure subsequent output appears on a new line.
Time Complexity: O(log n)
Space Complexity: O(log n)
Python Program To Convert Decimal To Octal Using Recursion
The decimal number can be converted to an octal number with the help of recursion. To achieve this, the decimal number is divided by 8, and the remainder obtained is stored temporarily. This step is followed until the number becomes 0. After that, the remainders obtained throughout the process are combined in reverse order to get the desired octal number.
Syntax:
def conversion(n):
if n>0:
conversion(n//8)
print(n%8, end='')
Here,
- conversion(): Function to convert decimal to octal
- n: Decimal number to be converted to octal
- print(): Function to print the octal digits
Look at the sample Python program to convert decimal to octal below, to understand how we can use recursion.
Code Example:
Output:
Enter a decimal number: 34
042
Explanation:
In the example Python code-
- We define a function convertToOct(), which takes a decimal number and converts it to its octal representation.
- Inside the function, there's a recursive call to convertToOct(n // 8), which recursively divides n by 8 to convert the quotient to octal.
- The base case is when n becomes less than or equal to 0, terminating the recursion.
- During the recursion, the remainders of the divisions by 8 are printed in reverse order to obtain the octal representation.
- The end='' parameter in print() ensures that the output is printed without a newline character.
- Outside the function, we prompt the user to input a decimal number using input(), and convert it and store it in variable x.
- After that, we call the convertToOct() function by passing variable x to it as an argument. The function converts x to an octal representation and prints the same.
- Finally, we print a newline character to ensure subsequent output appears on a new line.
Time Complexity: O(log n)
Space Complexity: O(log n)
Python Program To Convert Decimal To Hexadecimal Using Recursion
To convert a decimal number to hexadecimal using the recursive approach, we must divide the input decimal value by 16 and temporarily store the remainder obtained. This step is followed until the number becomes 0. After that, the remainders obtained throughout the process are combined in reverse order to get the desired hexadecimal number.
Syntax:
conversion_table = {0: '0', 1: '1', 2: '2', 3: '3',
4: '4', 5: '5', 6: '6', 7: '7',
8: '8', 9: '9', 10: 'A', 11: 'B',
12: 'C', 13: 'D', 14: 'E', 15: 'F'}def conversion(n):
if(n <= 0):
return ''
r = n % 16
return conversion(n//16) + conversion_table[r]
Here,
- conversion_table: Dictionary that maps decimal values to hexadecimal values
- conversion(): Function to convert decimal to hexadecimal
- n: Decimal number to be converted to hexadecimal
Look at the example Python program to convert decimal to hexadecimal using the recursive approach.
Code Example:
Output:
Enter a number: 48
Hexadecimal representation: 30
Explanation:
In the Python code sample-
- We define a dictionary conversion_table, which maps decimal values to their corresponding hexadecimal digits.
- Next, the convertToHex() function is defined to convert a decimal number to its hexadecimal representation.
- Inside the function, there's a recursive call to convertToHex(n // 16), which recursively divides n by 16 to convert the quotient to hexadecimal.
- The base case is when n becomes less than or equal to 0, terminating the recursion. In this case, an empty string is returned.
- During the recursion, the remainder of n divided by 16 is calculated, and the corresponding hexadecimal digit is retrieved from the conversion_table.
- The result of the recursive call concatenated with the hexadecimal digit is returned.
- Outside the function, we prompt the user to input a decimal number using input() and convert it to an integer variable x.
- We call the convertToHex() function to convert the decimal number x to hexadecimal.
Finally, we print the hexadecimal representation obtained from the function call.
Time Complexity: O(log n)
Space Complexity: O(log n)
Python Program To Convert Decimal To Binary Using While Loop
The decimal number can be converted into its equivalent binary number using a while loop. In this, we divide the decimal number by 2 until the number becomes zero. Then, we combine the remainder of these divisions in a bottom-up manner to get the resultant binary number.
The procedure to use a while loop in a Python program to convert decimal to binary is as follows:
- Take a decimal number as input n and initialize a variable binary, in which the resultant binary number will be stored as 0.
- Initialize variable i to 1 and variable r, which is used to store the current remainder, as 0.
- Then, apply a while loop with the condition that n does not equal 0. This loop will run till n is not equal to 0.
- After that, we divide n by 2, and store the remainder in r and then update n to the integer quotient of n/2.
- Update the variable binary by multiplying r with i and then add this result to the binary.
- After that, update i by multiplying it with 10. Here, i will be working as a variable to ensure the correct position of the digits.
- At the end, when the while loop terminates, print the resultant binary number.
Code Example:
Output:
Enter a number: 67
Binary representation: 1000011
Explanation:
In the Python code-
- We define the function convertBinary(n) to convert a decimal number to its binary representation.
- Inside the function, we initialize a variable binary to 0, which will store the binary representation, and variable i to 1, which is used as a multiplier to place binary digits correctly.
- We then enter a while loop that continues until n becomes 0. Inside the loop:
- We calculate the remainder r when n is divided by 2, representing the current binary digit.
- We update n to the integer quotient of n divided by 2, effectively shifting the binary digits to the right.
- We add the current binary digit multiplied by the multiplier i to binary, effectively adding the binary digit to the binary representation.
- We multiply i by 10 to move to the next position in the binary representation.
- Once the loop terminates, the function returns the binary representation stored in the variable binary.
- Outside the function, we prompt the user to input a decimal number using input() and convert it to an integer variable x.
- We then pass this variable to call the convertBinary() function to convert the decimal number x to binary.
- Finally, we print the binary representation obtained from the function call using a print() statement with a string message.
Time Complexity: O(log n)
Space Complexity: O(1)
Python Program To Convert Decimal To Octal Using While Loop
The decimal number can be converted into its equivalent octal number using a while loop. In this, we divide the decimal number by 8 until the number becomes zero. Then, we combine the remainder of these divisions in a bottom-up manner to get the resultant octal number. The Python program sample below provides an implementation of the same.
Code Example:
Output:
Enter a number: 67
Octal representation: 103
Explanation:
In the code-
- We define the function convertOctal(n) to convert a decimal number to its octal representation.
- Inside the function, we initialize variable octal to 0, which will store the octal representation, and variable i to 1, which is used as a multiplier to place octal digits correctly.
- We enter a while loop that continues until n becomes 0. Inside the loop:
- We calculate the remainder r when n is divided by 8, representing the current octal digit.
- We update n to the integer quotient of n divided by 8, effectively shifting the octal digits to the right.
- We add the current octal digit multiplied by the multiplier i to octal, effectively adding the octal digit to the octal representation.
- We multiply i by 10 to move to the next position in the octal representation.
- Once the loop terminates, we return the octal representation stored in the variable octal.
- Outside the function, we prompt the user to input a decimal number using input() and convert it to an integer variable x.
- We call the convertOctal(x) function to convert the decimal number x to octal.
- Finally, we print the octal representation obtained from the function call using the print() function.
Time Complexity: O(log n)
Space Complexity: O(1)
Python Program To Convert Decimal To Hexadecimal Using While Loop
The decimal number can be converted into its equivalent hexadecimal number using a while loop. In this, we divide the decimal number by 16 until the number becomes zero. Then, we combine the remainder of these divisions in a bottom-up manner to get the resultant hexadecimal number.
Code Example:
Output:
Enter a number: 58
Hexadecimal representation: 3A
Explanation:
In the above code,
- We define the function convertHexadecimal() to convert a decimal number to its hexadecimal representation.
- Inside the function, we initialize a variable hexadecimal to an empty string, which will store the hexadecimal representation.
- After that, we define a string variable hex_digits containing all hexadecimal digits for later reference and initialize variable r to 0, which will store the remainder when n is divided by 16.
- Next, we enter a while loop that continues until n becomes 0. Inside the loop:
- We calculate the remainder r when n is divided by 16, representing the current hexadecimal digit.
- Then, we update n to the integer quotient of n divided by 16, effectively shifting the hexadecimal digits to the right.
- Next, we concatenate the hexadecimal digit corresponding to r position from hex_digits to hexadecimal.
- Once the loop terminates, we reverse the hexadecimal representation using [::-1] and return it.
- Outside the function, we prompt the user to input a decimal number using input() and convert it to an integer variable x.
- We call the convertHexadecimal(x) function to convert the decimal number x to hexadecimal.
- Finally, we print the hexadecimal representation obtained from the function call.
Time Complexity: O(log n)
Space Complexity: O(1)
Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
The decimal number can be converted into other bases like binary, octal, and hexadecimal using string formatting. It can be accomplished using format() method, str.format() method, and f-strings. We will discuss each of these methods of writing a Python program to convert decimal to binary, octal, and hexadecimal in detail below.
Python Program Convert Decimal To Binary, Octal, And Hexadecimal Using Format() Method
The format() function is used to perform string formatting in order to convert decimals to other bases, and different format specifiers are used for different bases. The approach for this procedure can be described as:
- Take a decimal number n as user input.
- Then, we apply the format() function by passing n and the format specifier as argument.
- Store the result of the function in a variable and then print the value.
Syntax:
new_num = format(decimal, 'f')
Here,
- new_num: Resultant new number after string formatting.
- format(): Function to perform string formatting.
- decimal: Decimal number you want to convert.
- f: Format specifier for the desired base like b for binary, o for octal and x for hexadecimal.
Code Example:
Output:
Enter a number: 562
562 in binary: 1000110010
562 in octal: 1062
562 in hexadecimal: 232
Explanation:
In the Python code-
- We define the function convertToBases(), which takes an input decimal number n and converts it to its binary, octal, and hexadecimal representations simultaneously.
- Inside the function, we use string formatting with the format() method (format(n, 'b'), format(n, 'o'), format(n, 'x')) to convert the decimal number n into its binary, octal, and hexadecimal representations respectively.
- In the format() function, the second argument indicates the number system/ format we want to convert to, i.e., b stands for binary, o for octal, and x for hexadecimal.
- We then use a series of print() statements to display the original decimal number n along with its binary, octal, and hexadecimal representations.
- Outside the function, we declare a variable x, prompt the user to input a decimal number using input() and convert it to an integer to store in x using the int() function.
- After that, we call the convertToBases() function by passing x as an argument. It converts the input number from decimal to binary, octal, and hexadecimal and prints the respective value to the console.
Time Complexity: O(1)
Space Complexity: O(1)
Python Program Convert Decimal To Binary, Octal, And Hexadecimal Using Str.format() Method
The str.format() function is another method to perform string formatting in order to convert numbers from decimal to other bases. We use different format specifiers for different bases inside the strings. The approach for this procedure can be described as:
- Take a decimal number n as user input.
- Then, apply the str.format() function by passing n as an argument and specify the format specifier for the desired base in the string placeholder.
- Store the result of the function in a variable and then print the value.
Syntax:
new_num = "{0:f}".format(decimal)
Here,
- new_num: Resultant new number after string formatting.
- "{0:f}": It is the string placeholder where 0 is the positional argument and f is the format specifier for the desired base. For example, b is for binary, o is for octal, and x is for hexadecimal.
- format(): Function to perform string formatting.
- decimal: Decimal number you want to convert.
Code Example:
Output:
Enter a number: 83
83 in binary: 1010011
83 in octal: 123
83 in hexadecimal: 53
Explanation:
In the above code-
- We define the convertToBases() function, which takes a decimal input and converts it to binary, octal, and hexadecimal representations simultaneously.
- Inside the function, we use string formatting with the .format() method ("{0:b}".format(n), "{0:o}".format(n), "{0:x}".format(n)) to convert the decimal number n into its binary, octal, and hexadecimal representations respectively.
- In the format strings, {0:b}, {0:o}, and {0:x} indicate that n should be formatted as binary, octal, and hexadecimal, respectively.
- Then, we use the print() function to display the original decimal number n along with its binary, octal, and hexadecimal representations.
- Outside the function, we prompt the user to input a decimal number using input() and convert it to an integer x.
- We call the convertToBases(x) function to convert the decimal number x to binary, octal, and hexadecimal.
- Finally, the function calculates and prints the representations obtained from the string formatting.
Time Complexity: O(1)
Space Complexity: O(1)
Python Program Convert Decimal To Binary, Octal, And Hexadecimal Using F-strings
The f-strings are also used to perform string formatting in order to convert numbers from decimal to other bases. The approach for this procedure can be described as:
- Take a decimal number n as user input.
- Then, create f-string by passing n and the format specifier in the string placeholder.
- Store the resultant string in a variable and then print the value.
Syntax:
new_num = f"{decimal:format_specifier}"
Here,
- new_num: Resultant new number after string formatting.
- f: Representation of f-string.
- "{decimal:format_specifier}": String placeholder where the decimal is the number you want to convert and format_specifier is the format specifier for the targeted base like b for binary, o for octal and x for hexadecimal.
Code Example:
Output:
Enter a number: 35
35 in binary: 100011
35 in octal: 43
35 in hexadecimal: 23
Explanation:
In this example Python code-
- We define the function convertToBases() to convert a decimal number to its binary, octal, and hexadecimal representations simultaneously.
- Inside the function, we use f-strings (f"{n:b}", f"{n:o}", f"{n:x}") to directly convert the decimal number n into its binary, octal, and hexadecimal representations respectively.
- These f-strings format the integer n into a string representation using the specified base (binary, octal, hexadecimal).
- We print the original decimal number n along with its binary, octal, and hexadecimal representations using print() function.
- Outside the function, we prompt the user to input a decimal number using input() and convert it to an integer x.
- We call the convertToBases(x) function to convert the decimal number x to binary, octal, and hexadecimal.
- The invoked function converts and prints the representations obtained from the f-strings.
Time Complexity: O(1)
Space Complexity: O(1)
Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
Now, we will discuss how to convert a given binary, octal, or hexadecimal string to a number. The function used for this purpose is the int() function. It is a built-in function that takes a string and a base as an argument to convert the string into an integer.
The default value of base is 10, i.e., decimal. If the base is set to 0, then the base is decided according to the prefix of the string. For example, the 0b prefix is for binary, 0o for octal and 0x for hexadecimal. We can also use capital letters in prefixes.
Syntax:
int('0pXX', 0)
OR
int('XX', b)
Here,
- int(): Function to convert a binary, octal and hexadecimal string to a number.
- 0p: Prefix to identify the base of the number.
- XX: Numerical value in the string.
- b: Base of numerical string.
Code Example:
Output:
Binary to Decimal: 10
Octal to Decimal: 29
Hexadecimal to Decimal: 92
Explanation:
In the above code,
- We begin by assigning string values to three variables, i.e., binary_str, octal_str, and hexadeciaml_str.
- We then use the int() function to convert these values to decimal representations. Within the function the second argument represents the base of the number represented by the string.
- Here, int(binary_str, 2) function, where binary_str is the binary string and 2 indicates the base of the number system (binary). It converts the binary string to its equivalent decimal value.
- Similarly, we employ the int(octal_str, 8) function, where octal_str is the octal string, and 8 denotes the base of the number system (octal). This converts the octal string to its equivalent decimal value.
- The int(hexadecimal_str, 16) function, where hexadecimal_str is the hexadecimal string, and 16 signifies the base of the number system (hexadecimal). It converts the hexadecimal string to its equivalent decimal value.
- Finally, we print the converted decimal values using print() function, along with appropriate labels for clarity.
Time Complexity: O(n)
Space Complexity: O(1)
Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
The comparison table provided below comprises the time and space complexities of all the methods used to convert decimals to other bases.
Method Name |
Time Complexity |
Space Complexity |
Using in-built function |
O(1) |
O(1) |
Using recursion |
O(log n) |
O(log n) |
Using while loop |
O(log n) |
O(1) |
Using string formatting |
O(1) |
O(1) |
Conclusion
The ability to convert numbers from decimal and other number systems/ bases is a valuable skill in your programming toolkit. In this article, we have explored various methods to write a Python program to convert decimal to binary, octal, and hexadecimal. These methods include using inbuilt functions (bin(), oct(), hex()), recursion, string formatting, and manual calculation with while loops.
Python's built-in functions provide simplicity and efficiency, while manual calculation offers insight into number systems. Mastering these conversion methods equips programmers with versatile skills for working with different data formats, enhancing their proficiency in Python programming and computer science applications.
Frequently Asked Questions
Q. Why do we need to convert decimal numbers to binary, octal, or hexadecimal?
Converting decimal numbers to other numeral systems is essential for various computing tasks, including low-level programming, bitwise operations, data representation, and cryptography. Different numeral systems offer advantages in different contexts, and understanding how to perform conversions provides flexibility in programming.
Q. What is the hexadecimal number system?
The hexadecimal number system is a number system with a base of 16. It contains 10 digits from 0 to 9 and 6 alphabets from A to F. Here, the alphabets A to F represent the values from 10 to 15, where A represents 10, B represents 11 and so on. Therefore, this number system is also called an alphanumeric number system.
For example, (13A)16 is represented in decimal as:
(13A)16 = 162 .1 + 161 .3 + 160 .A = 144 + 48 + 10 = (202)10
Q. What are the built-in functions available in Python for converting decimal numbers?
Python provides built-in functions bin(), oct(), and hex() for converting decimal numbers to binary, octal, and hexadecimal representations, respectively. These functions simplify the conversion process and are efficient for everyday programming tasks.
Q. Are there any limitations to using built-in functions for conversion?
While built-in functions like bin(), oct(), and hex() offer simplicity and efficiency, they may have limitations when dealing with large numbers or requiring specific formatting. In such cases, you must use manual conversion methods like using loops or custom formatting when writing a Python program to convert decimal to binary, octal, and hexadecimal.
Q. How to convert octal string to decimal in Python?
You can use the in-built int() function is used to convert from octal to decimal in Python. It takes a string and a base as an argument to convert the string into an integer. The default value of base is 10, i.e., decimal and is optional. However, since we are converting a string that represents an octal number, we must specify the base 8 as an argument and convert it to a corresponding decimal number. If the base is set to 0, then the base is decided according to the prefix of the string.
Code Example:
Output:
55
Explanation:
- In this code, a variable x is assigned octal string 67.
- Then, the int() function is invoked with x as an argument and base as 8. This function converts x into a decimal number, and the result is stored in y.
- At last, y is printed as output.
Q. How can I handle errors or invalid inputs during conversion?
When converting decimal numbers to other bases, it's essential to handle errors or invalid inputs gracefully. You can use exception-handling techniques to catch and handle errors, such as ValueError for invalid inputs or OverflowError for large numbers. Additionally, validating inputs before the conversion can prevent unexpected behaviour and improve the robustness of your program.
We have discussed various ways you can write a Python program to convert decimal to binary, octal and hexadecimal basses. Here are a few other topics you must know:
- Python For Loop | Syntax & Application (With Multiple Examples)
- Convert Int To String In Python | Learn 6 Methods With Examples
- How To Reverse A String In Python? 10 Easy Ways With Examples
- Swap Two Variables In Python- Different Ways | Codes + Explanation
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment