Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
Mutable & Immutable In Python | Difference, Uses & More (+Examples)
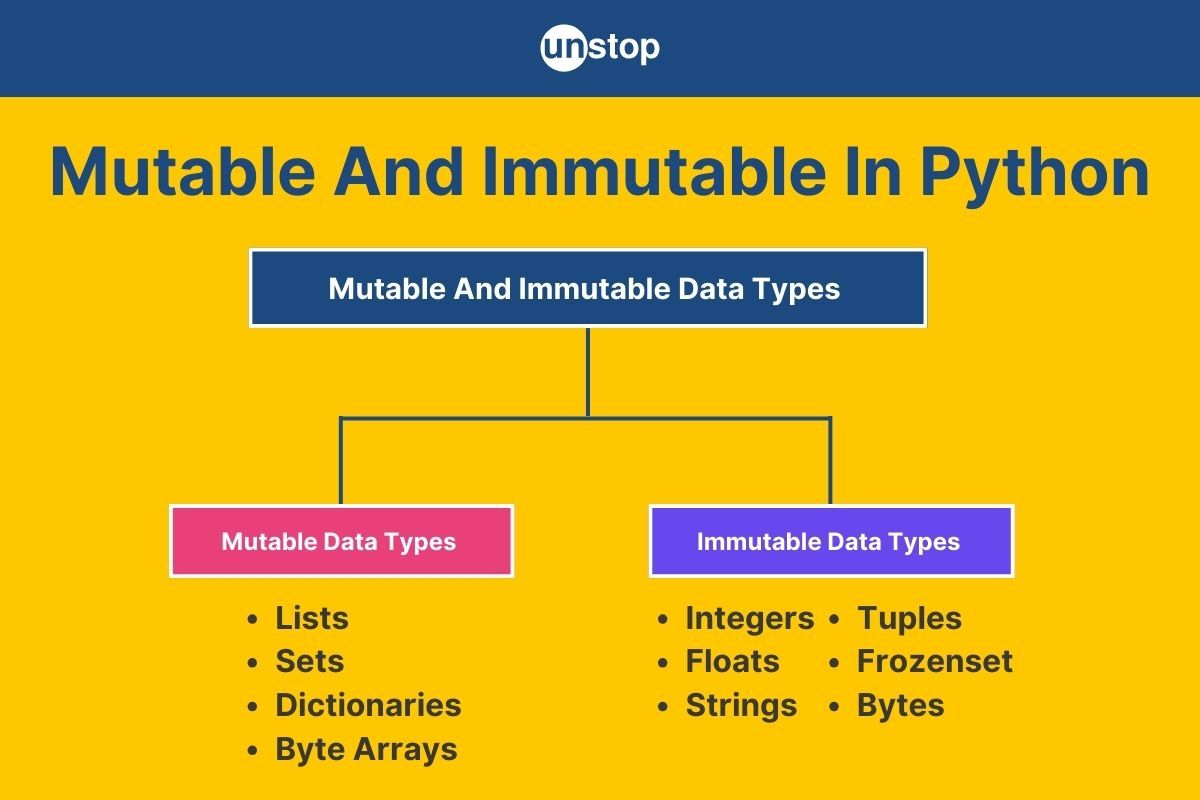
Python is one of the most widely used programming languages, renowned for its simplicity and powerful capabilities. One of the key features of Python is its dynamic typing system, where the data type of a variable is determined automatically based on the value it holds, unlike programming languages such as Java, C, or C++, which require explicit declaration of data types. In Python, data types are classified into two broad categories:
- Mutable Data Types – These are data types whose values can be modified after they are created. Examples: List, Dictionary, Set
- Immutable Data Types – These are data types whose values cannot be altered once they are set. Examples: String, Tuple
In this article, we will explore the differences between mutable and immutable types in Python, examine their practical uses, and understand how they affect the behaviour of our programs. By the end, you'll have a clear understanding of when to use each type and how they influence performance and code integrity.
Difference Between Mutable And Immutable Data Types in Python
Given below are the key differences between the mutable and immutable data types in Python programming:
Aspect |
Mutable Objects |
Immutable Objects |
Definition |
Objects whose state or value can be changed after they are created. |
Objects whose state or value cannot be changed once they are created. |
Examples |
Lists, Dictionaries, Sets |
Integers, Floats, Strings, Tuples, Frozensets |
Memory Allocation |
Can modify in place without creating a new object. |
Creating a new object is required when attempting to modify. |
Behavior with Assignment |
Assigning a new reference does not affect other references. |
Assigning a new reference creates a new object, leaving the original unchanged. |
Efficiency |
More memory efficient as they allow changes without allocating new memory. |
Less memory efficient as new objects are created for any modification. |
Impact on Functions |
Functions can alter the original object, affecting other references to it. |
Functions cannot alter the original object, ensuring it remains unchanged. |
Use Cases |
Ideal for situations where changes to the object are frequent, e.g., dynamic data storage. |
Ideal when the object should remain constant throughout the program, e.g., keys in dictionaries, constants. |
Hashability |
Not hashable, so they cannot be used as keys in dictionaries or elements in sets. |
Hashable, making them suitable as dictionary keys or set elements. |
Example of Mutation |
list1 = [1, 2, 3] list1[0] = 10 modifies the object. |
string1 = "hello" string1[0] = "H" results in an error. |
Thread Safety |
Not thread-safe, as changes to mutable objects can lead to inconsistent state in multi-threaded programs. |
Thread-safe, as immutable objects cannot be altered after creation, ensuring consistency. |
What Is Mutable Data Type In Python?
In Python, a mutable data type refers to a data type whose value or state can be changed after it is created. This means that once an object of a mutable type is initialized, you can modify its content or structure without creating a new object. The ability to change the contents of a mutable object directly can be particularly useful for scenarios where you need to perform frequent modifications to data without creating copies of the object each time.
Mutable types are commonly used in cases where performance is important, especially when dealing with large datasets, as modifying the object in place is more memory-efficient than creating new copies.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Types Of Mutable Data Types In Python
Here are the most common mutable data types in Python:
Lists
Lists are ordered collections of elements that are changeable, meaning their content can be modified after they are created. Lists allow duplicate elements and maintain the order of insertion.
Code Example:
bXlfbGlzdCA9IFsxLCAyLCAzXQoKcHJpbnQoIk9yaWdpbmFsIGxpc3Q6IiwgbXlfbGlzdCkKCiMgTW9kaWZ5aW5nIGFuIGVsZW1lbnQKbXlfbGlzdFswXSA9IDEwCnByaW50KCJNb2RpZmllZCBsaXN0IChhZnRlciBjaGFuZ2luZyBmaXJzdCBlbGVtZW50KToiLCBteV9saXN0KQoKIyBBZGRpbmcgYSBuZXcgZWxlbWVudApteV9saXN0LmFwcGVuZCg0KQpwcmludCgiTW9kaWZpZWQgbGlzdCAoYWZ0ZXIgYXBwZW5kaW5nIDQpOiIsIG15X2xpc3QpCgojIFJlbW92aW5nIGFuIGVsZW1lbnQKbXlfbGlzdC5yZW1vdmUoMikKcHJpbnQoIk1vZGlmaWVkIGxpc3QgKGFmdGVyIHJlbW92aW5nIDIpOiIsIG15X2xpc3QpCg==
Output:
Original list: [1, 2, 3]
Modified list (after changing first element): [10, 2, 3]
Modified list (after appending 4): [10, 2, 3, 4]
Modified list (after removing 2): [10, 3, 4]
Explanation:
In the above code example-
- We start with defining a list called my_list containing the elements [1, 2, 3] and print the original list.
- Next, we modify the first element of the list by changing my_list[0] to 10. This alters the list to [10, 2, 3], which we then print.
- We then add a new element 4 to the end of the list using the append() method. After this, the list becomes [10, 2, 3, 4], and we print the updated list.
- Finally, we remove the element 2 from the list using the remove() method. This changes the list to [10, 3, 4], and we print the final modified list.
Dictionaries
Dictionaries are unordered collections of key-value pairs. Each key is unique, and values can be modified. You can also add new key-value pairs or remove existing ones.
Code Example:
bXlfZGljdCA9IHsiYSI6IDEsICJiIjogMn0KCnByaW50KCJPcmlnaW5hbCBkaWN0aW9uYXJ5OiIsIG15X2RpY3QpCgojIE1vZGlmeWluZyB0aGUgdmFsdWUgYXNzb2NpYXRlZCB3aXRoIGEga2V5Cm15X2RpY3RbImEiXSA9IDEwCnByaW50KCJNb2RpZmllZCBkaWN0aW9uYXJ5IChhZnRlciBjaGFuZ2luZyB2YWx1ZSBvZiAnYScpOiIsIG15X2RpY3QpCgojIEFkZGluZyBhIG5ldyBrZXktdmFsdWUgcGFpcgpteV9kaWN0WyJjIl0gPSAzCnByaW50KCJNb2RpZmllZCBkaWN0aW9uYXJ5IChhZnRlciBhZGRpbmcgbmV3IGtleSAnYycpOiIsIG15X2RpY3QpCgojIFJlbW92aW5nIGEga2V5LXZhbHVlIHBhaXIKZGVsIG15X2RpY3RbImIiXQpwcmludCgiTW9kaWZpZWQgZGljdGlvbmFyeSAoYWZ0ZXIgcmVtb3Zpbmcga2V5ICdiJyk6IiwgbXlfZGljdCkK
Output:
Original dictionary: {'a': 1, 'b': 2}
Modified dictionary (after changing value of 'a'): {'a': 10, 'b': 2}
Modified dictionary (after adding new key 'c'): {'a': 10, 'b': 2, 'c': 3}
Modified dictionary (after removing key 'b'): {'a': 10, 'c': 3}
Explanation:
In the above code example-
- We start with a dictionary my_dict containing two key-value pairs: {"a": 1, "b": 2}, and we print the original dictionary.
- Next, we modify the value associated with the key "a" by setting my_dict["a"] = 10. This changes the value of "a" to 10, resulting in the updated dictionary {"a": 10, "b": 2}, which we then print.
- We then add a new key-value pair "c": 3 to the dictionary. After adding this, the dictionary becomes {"a": 10, "b": 2, "c": 3}, and we print the updated dictionary.
- Finally, we remove the key-value pair with the key "b" using the del statement. This results in the dictionary {"a": 10, "c": 3}, and we print the final modified dictionary.
Sets
Sets are unordered collections of unique elements. You can add or remove elements from a set, but duplicate elements are automatically discarded.
Code Example:
bXlfc2V0ID0gezEsIDIsIDN9CgpwcmludCgiT3JpZ2luYWwgc2V0OiIsIG15X3NldCkKCiMgQWRkaW5nIGEgbmV3IGVsZW1lbnQKbXlfc2V0LmFkZCg0KQpwcmludCgiTW9kaWZpZWQgc2V0IChhZnRlciBhZGRpbmcgNCk6IiwgbXlfc2V0KQoKIyBSZW1vdmluZyBhbiBlbGVtZW50Cm15X3NldC5yZW1vdmUoMikKcHJpbnQoIk1vZGlmaWVkIHNldCAoYWZ0ZXIgcmVtb3ZpbmcgMik6IiwgbXlfc2V0KQoKIyBBdHRlbXB0aW5nIHRvIGFkZCBhIGR1cGxpY2F0ZSBlbGVtZW50ICh3b24ndCBjaGFuZ2UgdGhlIHNldCkKbXlfc2V0LmFkZCgzKQpwcmludCgiTW9kaWZpZWQgc2V0IChhZnRlciBhZGRpbmcgZHVwbGljYXRlIDMpOiIsIG15X3NldCkK
Output:
Original set: {1, 2, 3}
Modified set (after adding 4): {1, 2, 3, 4}
Modified set (after removing 2): {1, 3, 4}
Modified set (after adding duplicate 3): {1, 3, 4}
Explanation:
In the above code example-
- We begin with a set called my_set containing the elements {1, 2, 3}, and we print the original set.
- Next, we add a new element 4 to the set using the add() method. After adding it, the set becomes {1, 2, 3, 4}, and we print the updated set.
- We then remove the element 2 from the set using the remove() method. This changes the set to {1, 3, 4}, and we print the modified set.
- Finally, we attempt to add the element 3 again, which is a duplicate. Since sets do not allow duplicate values, the set remains unchanged as {1, 3, 4}, and we print the set after this operation.
Byte Arrays
Byte arrays are mutable sequences of bytes. Unlike strings (which are immutable), byte arrays can be modified directly. They are typically used for binary data and manipulation.
Code Example:
bXlfYnl0ZV9hcnJheSA9IGJ5dGVhcnJheShiImhlbGxvIikKCnByaW50KCJPcmlnaW5hbCBieXRlIGFycmF5OiIsIG15X2J5dGVfYXJyYXkpCgojIE1vZGlmeWluZyBhIGJ5dGUKbXlfYnl0ZV9hcnJheVswXSA9IDcyICAjIEFTQ0lJIHZhbHVlIG9mICdIJwpwcmludCgiTW9kaWZpZWQgYnl0ZSBhcnJheSAoYWZ0ZXIgY2hhbmdpbmcgZmlyc3QgYnl0ZSk6IiwgbXlfYnl0ZV9hcnJheSkKCiMgTW9kaWZ5aW5nIGFub3RoZXIgYnl0ZQpteV9ieXRlX2FycmF5WzFdID0gMTAxICAjIEFTQ0lJIHZhbHVlIG9mICdlJwpwcmludCgiTW9kaWZpZWQgYnl0ZSBhcnJheSAoYWZ0ZXIgY2hhbmdpbmcgc2Vjb25kIGJ5dGUpOiIsIG15X2J5dGVfYXJyYXkpCg==
Output:
Original byte array: bytearray(b'hello')
Modified byte array (after changing first byte): bytearray(b'Hello')
Modified byte array (after changing second byte): bytearray(b'Hello')
Explanation:
In the above code example-
- We start with a bytearray called my_byte_array initialized with the bytes of the string "hello", and we print the original byte array.
- Next, we modify the first byte of the array by setting my_byte_array[0] = 72, which corresponds to the ASCII value of the letter 'H'. After this change, the byte array becomes bytearray(b"Hello"), and we print the modified array.
- We then modify the second byte of the array by setting my_byte_array[1] = 101, which corresponds to the ASCII value of the letter 'e'. This updates the byte array to bytearray(b"Hello"), and we print the final modified array.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
What Are Immutable Data Types In Python?
In Python, immutable data types are data types whose values cannot be changed once they are created. This means that once an object of an immutable type is assigned a value, it cannot be modified directly. Any attempt to alter the contents of an immutable object results in the creation of a new object.
Immutable types offer several benefits, including easier debugging, better safety in concurrent programs, and more predictable behavior since their state cannot change unexpectedly. They are particularly useful when data should not be altered once it is initialized, ensuring that the integrity of the data remains intact throughout the program.
Types Of Immutable Data Types In Python
Here are the most common immutable data types in Python:
Integers
Integers are whole numbers, and in Python, they are immutable. Once an integer is created, its value cannot be changed. Any operation that appears to modify an integer actually creates a new integer object.
Code Example:
bXlfaW50ID0gMTAKCnByaW50KCJPcmlnaW5hbCBpbnRlZ2VyOiIsIG15X2ludCkKCiMgVHJ5aW5nIHRvIG1vZGlmeSB0aGUgdmFsdWUgKGFjdHVhbGx5IGNyZWF0ZXMgYSBuZXcgaW50ZWdlciBvYmplY3QpCm15X2ludCA9IG15X2ludCArIDUKCnByaW50KCJNb2RpZmllZCBpbnRlZ2VyOiIsIG15X2ludCkK
Output:
Original integer: 10
Modified integer: 15
Explanation:
In the above code example-
- We start with an integer my_int assigned the value 10, and we print the original integer.
- Next, we attempt to modify the value of my_int by adding 5 to it. However, in Python, integers are immutable, so this actually creates a new integer object with the value 15.
- The variable my_int is now pointing to this new integer, and we print the modified value of my_int.
Floats
Floating-point numbers, like integers, are immutable in Python. Once a float object is created, its value cannot be changed. Any modification results in the creation of a new float object.
Code Example:
bXlfZmxvYXQgPSAzLjE0CgpwcmludCgiT3JpZ2luYWwgZmxvYXQ6IiwgbXlfZmxvYXQpCgojIE1vZGlmeWluZyB0aGUgdmFsdWUgKGNyZWF0ZXMgYSBuZXcgZmxvYXQgb2JqZWN0KQpteV9mbG9hdCA9IG15X2Zsb2F0ICogMgoKcHJpbnQoIk1vZGlmaWVkIGZsb2F0OiIsIG15X2Zsb2F0KQo=
Output:
Original float: 3.14
Modified float: 6.28
Explanation:
In the above code example-
- We start with a float variable my_float assigned the value 3.14, and we print the original float.
- Next, we attempt to modify the value of my_float by multiplying it by 2. Since floats are immutable in Python, this operation creates a new float object with the value 6.28.
- The variable my_float now points to this new float, and we print the modified value of my_float.
Strings
Strings are sequences of characters, and they are immutable. Once a string is created, you cannot modify its individual characters. Any operation that changes the string results in a new string object.
Code Example:
bXlfc3RyaW5nID0gImhlbGxvIgoKcHJpbnQoIk9yaWdpbmFsIHN0cmluZzoiLCBteV9zdHJpbmcpCgojIFRyeWluZyB0byBtb2RpZnkgdGhlIHN0cmluZyAoYWN0dWFsbHkgY3JlYXRlcyBhIG5ldyBzdHJpbmcgb2JqZWN0KQpteV9zdHJpbmcgPSBteV9zdHJpbmcgKyAiIHdvcmxkIgoKcHJpbnQoIk1vZGlmaWVkIHN0cmluZzoiLCBteV9zdHJpbmcpCg==
Output:
Original string: hello
Modified string: hello world
Explanation:
In the above code example-
- We start with a string variable my_string assigned the value "hello", and we print the original string.
- Next, we attempt to modify the string by concatenating " world" to it. Since strings are immutable in Python, this operation creates a new string object with the value "hello world".
- The variable my_string now points to this new string, and we print the modified value of my_string.
Tuples
Tuples are ordered, immutable collections of elements. Unlike lists, the elements of a tuple cannot be modified once the tuple is created. However, if a tuple contains mutable elements (e.g., lists), those elements can be modified, but the tuple itself remains immutable.
Code Example:
bXlfdHVwbGUgPSAoMSwgMiwgMykKCnByaW50KCJPcmlnaW5hbCB0dXBsZToiLCBteV90dXBsZSkKCiMgVHJ5aW5nIHRvIG1vZGlmeSB0aGUgdHVwbGUgKHRoaXMgd2lsbCByYWlzZSBhbiBlcnJvcikKIyBteV90dXBsZVswXSA9IDEwICAjIFVuY29tbWVudGluZyB0aGlzIGxpbmUgd2lsbCByYWlzZSBhIFR5cGVFcnJvcgoKIyBNb2RpZnlpbmcgdGhlIHR1cGxlIGJ5IGNyZWF0aW5nIGEgbmV3IG9uZQpteV90dXBsZSA9IG15X3R1cGxlICsgKDQsKQoKcHJpbnQoIk1vZGlmaWVkIHR1cGxlOiIsIG15X3R1cGxlKQo=
Output:
Original tuple: (1, 2, 3)
Modified tuple: (1, 2, 3, 4)
Explanation:
In the above code example-
- We start with a tuple called my_tuple containing the elements (1, 2, 3), and we print the original tuple.
- Next, we attempt to modify the first element of the tuple by setting my_tuple[0] = 10. However, since tuples are immutable in Python, this operation will raise a TypeError, indicating that you cannot modify a tuple after it is created.
- Instead, we modify the tuple by creating a new one.
- We concatenate the tuple (4,) to my_tuple, resulting in the new tuple (1, 2, 3, 4). We then print the modified tuple.
Frozensets
Frozensets are similar to sets, but unlike regular sets, frozensets are immutable. They cannot be modified after creation, which means you cannot add or remove elements from a frozenset.
Code Example:
bXlfZnJvemVuc2V0ID0gZnJvemVuc2V0KFsxLCAyLCAzXSkKCnByaW50KCJPcmlnaW5hbCBmcm96ZW5zZXQ6IiwgbXlfZnJvemVuc2V0KQoKIyBUcnlpbmcgdG8gbW9kaWZ5IHRoZSBmcm96ZW5zZXQgKHRoaXMgd2lsbCByYWlzZSBhbiBlcnJvcikKIyBteV9mcm96ZW5zZXQuYWRkKDQpICAjIFVuY29tbWVudGluZyB0aGlzIGxpbmUgd2lsbCByYWlzZSBhbiBBdHRyaWJ1dGVFcnJvcgo=
Output:
Original frozenset: frozenset({1, 2, 3})
Explanation:
In the above code example-
- We start with a frozenset called my_frozenset, which contains the elements {1, 2, 3}, and we print the original frozenset.
- Next, we attempt to modify the frozenset by adding an element 4 using the add() method.
- However, since frozensets are immutable in Python, this operation will raise an AttributeError, indicating that you cannot modify a frozenset after it is created.
Bytes
Bytes are immutable sequences of bytes. Once a bytes object is created, its value cannot be changed. Any operation that modifies bytes will create a new bytes object.
Code Example:
bXlfYnl0ZXMgPSBiImhlbGxvIgoKcHJpbnQoIk9yaWdpbmFsIGJ5dGVzOiIsIG15X2J5dGVzKQoKIyBNb2RpZnlpbmcgdGhlIGJ5dGVzIChjcmVhdGVzIGEgbmV3IGJ5dGVzIG9iamVjdCkKbXlfYnl0ZXMgPSBteV9ieXRlcyArIGIiIHdvcmxkIgoKcHJpbnQoIk1vZGlmaWVkIGJ5dGVzOiIsIG15X2J5dGVzKQo=
Output:
Original bytes: b'hello'
Modified bytes: b'hello world'
Explanation:
In the above code example-
- We start with a bytes object my_bytes initialized with the value b"hello", and we print the original bytes.
- Next, we attempt to modify the bytes by concatenating b" world" to my_bytes. Since bytes objects are immutable in Python, this operation creates a new bytes object with the value b"hello world".
- The variable my_bytes now points to this new bytes object, and we print the modified bytes.
Key Similarities Between Mutable And Immutable Data Types In Python
Despite the fundamental differences in how mutable and immutable data types behave, there are several key similarities between them:
- Both are Objects: In Python, all data types (whether mutable or immutable) are objects. Both mutable and immutable data types are instances of their respective classes, and they follow the same object model.
- Can Be Assigned to Variables: Both mutable and immutable data types can be assigned to variables. This means that you can use variables to store references to any object in Python, regardless of whether the object is mutable or immutable.
- Can Be Passed to Functions: Both types can be passed as arguments to functions. The behavior within the function will differ based on whether the data type is mutable or immutable, but both can be used as function parameters.
- Support Operators and Methods: Both mutable and immutable data types support various operators (like addition, multiplication, etc.) and built-in methods (like .append(), .remove(), .len(), etc.), depending on their respective nature. For example:
- Immutable types like strings and tuples support methods such as .count(), .find(), etc.
- Mutable types like lists and dictionaries support methods such as .append(), .update(), etc.
- Have Built-in Types: Both mutable and immutable data types are built into Python. For instance, lists, dictionaries, and sets are mutable, whereas integers, floats, strings, and tuples are immutable. They all belong to Python's standard data types.
- Can Be Iterated: Both types can be iterated using loops. For example, you can loop through a list (mutable) or a string (immutable) and access each element. Example-
my_list = [1, 2, 3]
for item in my_list:
print(item) # Works for mutable data types
my_string = "abc"
for char in my_string:
print(char) # Works for immutable data types
- Can Be Nested: Both mutable and immutable data types can be nested inside each other. For example, you can have a list containing tuples (immutable) or a dictionary containing lists (mutable). Example-
my_list = [("a", 1), ("b", 2)] # List of tuples (immutable inside mutable)
my_dict = {"key1": [1, 2, 3]} # Dictionary with a list as a value (mutable inside immutable)
- Are Referenced by Memory Addresses: All objects, whether mutable or immutable, are referenced by memory addresses in Python. When you assign one variable to another, both refer to the same memory location (until modifications are made, in the case of mutable types).
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
When To Use Mutable Vs Immutable In Python?
Choosing between mutable and immutable data types in Python depends on the specific requirements of your program. Here are some guidelines on when to use each:
When To Use Mutable Data Types:
- When You Need to Modify the Object: If you need to change the data after it’s created, such as adding, removing, or updating elements, mutable data types like lists, dictionaries, and sets are ideal. For example, if you're building a collection of items that will be frequently updated, such as in a shopping cart or a task list, mutable types are the right choice.
- When Performance is a Concern with Frequent Changes: Mutable objects are more efficient when you need to make frequent modifications, as they don’t require the creation of new objects every time an update is needed. For example, appending items to a list or updating dictionary keys is more efficient with mutable types.
- When You Need to Share the Object Between Different Parts of the Program: If you want to allow multiple functions or parts of your program to modify and share a common object, mutable data types are suitable. However, be cautious of unintended side effects if shared across different parts of the code.
- When Working with Large Data Structures: Mutable data types like dictionaries and sets are beneficial when you need to store and modify large collections of data, as their size can change dynamically without needing to create new objects.
When To Use Immutable Data Types:
- When You Don’t Want to Modify the Object: If your data should remain unchanged, such as constant values, using immutable data types (like strings, tuples, or frozensets) is ideal. They ensure the integrity of the data, preventing accidental changes.
- When You Need Data Integrity: Immutable objects are excellent when you need to ensure that your data remains consistent across the program, especially when passed to different functions. For example, using strings or tuples can help prevent accidental modifications, which is particularly useful in large, complex programs.
- When Using the Data as Dictionary Keys or Set Elements: Immutable types are hashable and can be used as keys in dictionaries or elements in sets, unlike mutable types. If you need to store data in a dictionary or a set and rely on the object’s value remaining constant, tuples or frozensets are ideal choices.
- When Working in Multi-threaded Environments: Immutable data types provide inherent thread-safety since their values cannot change. This makes them a good choice when dealing with shared data across multiple threads, reducing the need for complex locking mechanisms.
- When You Want to Avoid Side Effects: In functional programming or when working with pure functions, using immutable data types encourages the avoidance of side effects, as you can be sure that the object won’t change after it’s created. This makes the code easier to reason about and test.
In Summary:
- Use mutable types (lists, dictionaries, sets) when you need to modify data, share it between parts of your program, or manage large collections.
- Use immutable types (strings, tuples, frozensets) when you need data integrity, thread safety, or to ensure that the data will not change unexpectedly. They are also crucial when working with hash-based collections like dictionaries and sets.
Conclusion
In Python, understanding the distinction between mutable and immutable data types is crucial for writing efficient and reliable code. While mutable data types allow for modifications after they are created, immutable data types offer data integrity by preventing alterations. Both types have their unique advantages and are suited to different programming scenarios.
- Mutable data types such as lists, dictionaries, and sets provide flexibility, allowing us to modify the contents directly, making them ideal for cases where data needs to change over time.
- Immutable data types like integers, strings, and tuples are best suited for situations where data integrity is essential, as their values cannot be modified once created. They help prevent unintended side effects and provide safety in multi-threaded environments.
Both categories of data types share common features, such as being objects, supporting various operators, and being iterable. However, their key difference lies in how they handle modifications, which influences their use cases and performance.
By understanding when to use mutable or immutable data types, we can make better design decisions and write more efficient and maintainable Python code.
Frequently Asked Questions
Q. What is the main difference between mutable and immutable data types in Python?
The main difference lies in whether the object's state can be changed after it is created. Mutable data types (like lists, dictionaries, and sets) can be changed, whereas immutable data types (like integers, strings, and tuples) cannot be altered once assigned.
Q. Can we change the value of an immutable object in Python?
No, we cannot change the value of an immutable object in Python. Immutable objects, such as strings, tuples, and integers, are designed in such a way that their state cannot be modified once they are created. Any attempt to change the value of an immutable object will result in the creation of a new object rather than modifying the existing one.
For example, if you try to modify an element in a tuple or alter a character in a string, Python will not allow it and will instead generate a new object with the updated value. This behavior ensures data integrity and safety, particularly in situations where objects need to remain constant throughout the program.
Q. What are the advantages of using immutable data types?
The advantages of using immutable data types in Python are significant, especially in scenarios where data integrity, predictability, and performance are crucial:
- Data Integrity: Since immutable objects cannot be changed once created, they ensure that their state remains consistent throughout the program. This is particularly useful in preventing accidental modifications, which can lead to bugs and unpredictable behavior.
- Thread Safety: In multi-threaded environments, immutable data types provide thread safety, as their values cannot be altered by multiple threads. This eliminates the need for complex locking mechanisms to protect shared data, reducing the potential for race conditions.
- Hashability: Immutable objects can be used as keys in dictionaries and elements in sets because their hash value remains constant throughout their lifetime. This allows them to function efficiently in hashed collections.
- Efficiency in Memory Usage: Immutable objects can be optimized by Python’s memory management system. Since their values do not change, Python can reuse the same object in multiple places, saving memory. This is particularly beneficial when working with large datasets.
- Predictable Behavior: With immutable data types, you can be confident that the object’s value will not change unexpectedly, leading to more predictable and reliable code. This is especially important when debugging or working with functions that rely on consistent input values.
- Facilitates Functional Programming: Immutable data types are a cornerstone of functional programming paradigms, where the goal is to avoid side effects. Their use encourages a more declarative style of programming and makes it easier to reason about code.
Q. Can mutable data types be used as dictionary keys?
No, only immutable data types (such as strings, tuples, and frozensets) can be used as dictionary keys. Mutable types like lists and dictionaries cannot be used as dictionary keys because they can change, and the hash value may no longer remain consistent.
Q. Are strings in Python mutable or immutable?
Strings in Python are immutable. Once a string is created, its content cannot be changed directly. Any operation that modifies a string will create a new string object.
Q. What happens when a mutable object is passed to a function in Python?
When a mutable object is passed to a function, any modifications made to the object within the function will affect the original object outside the function. This is because the function operates on the same object reference. However, for immutable objects, since they cannot be modified, any change results in a new object being created inside the function.
With this, we conclude our discussion on the mutable and immutable data types in Python. Here are a few other topics that you might be interested in reading:
- Fibonacci Series In Python & Nth Term | Generate & Print (+Codes)
- Python Program To Find LCM Of Two Numbers | 5 Methods With Examples
- Convert Int To String In Python | Learn 6 Methods With Examples
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Flask vs Django: Understand Which Python Framework You Should Choose
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment