Table of content:
- Understanding count() Function In Python
- How To Use count() Function In Python?
- Using count() Function In Python With Lists
- Using count() Function In Python With Strings
- Using count() Function In Python With Tuples
- Practical Applications Of count() Function In Python
- Conclusion
- Frequently Asked Questions
Python count() Function | Explained In Detail With Code Examples
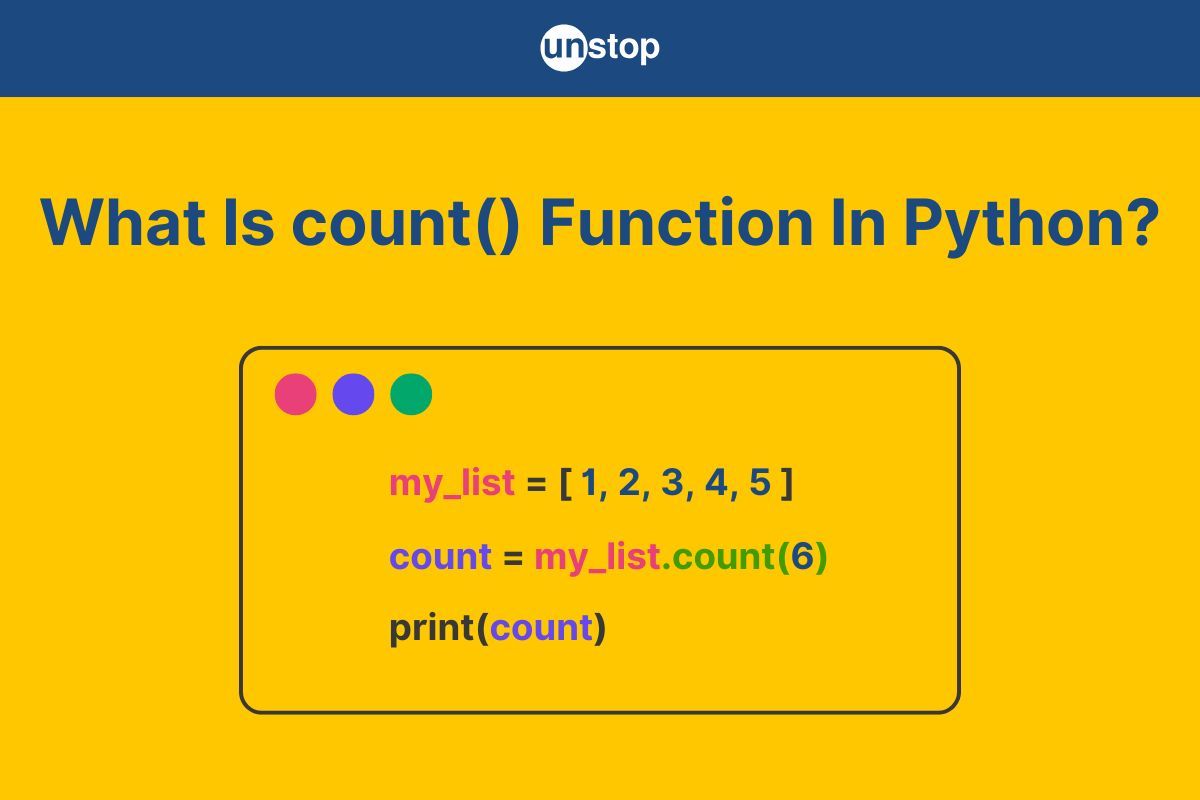
In Python, data manipulation and analysis are fundamental skills that developers must master. One of the most useful built-in methods for this purpose is the count() function, which provides a straightforward way to determine the frequency of specific elements or substrings within various data structures.
Whether you are working with strings to analyze text data, lists to manage collections of items, or tuples for immutable sequences, the count() function can significantly streamline your coding process. In this article, we will understand the functionality of the Python count() function, exploring its syntax, application across different data types, limitations, and practical examples to enhance your understanding and usage of this essential tool in Python.
Understanding count() Function In Python
The count function in Python programming is used to count the number of times a specified element (in lists) or substring (in strings) appears. It's particularly useful for tasks like analyzing data, tracking patterns, or searching text. For example, if you have a list of names, you can count how many times "Alia" appears.
Syntax Of count() Function In Python
In Python, you can use the count() method with both lists and strings-
For List:
list.count(element)
Here:
- list: The list you want to search in.
- element: The value you want to count in the list.
For String:
string.count(substring, start, end)
Here:
- string: The string in which to search.
- substring: The substring to count.
- start (optional): The position where the search begins (default is the start of the string).
- end (optional): The position where the search ends (default is the end of the string).
Return Value Of count() Function In Python
The return value of the count() function in Python is an integer that represents the number of times a specified element (for lists) or substring (for strings) appears in the given list or string.
- If the element or substring is found one or more times, the function returns the count as an integer.
- If the element or substring is not found, the function returns 0.
How To Use count() Function In Python?
The working mechanism of the count() function in Python is as follows:
- The count() function is a method available for various sequence types, including strings, lists, and tuples, primarily used to count the number of occurrences of a specified value within that sequence.
- When you invoke count(), Python interprets the sequence and the specified value, preparing to iterate through the entire sequence to identify how many times the value appears.
- The function performs a loop through the sequence; for strings, it looks for the substring within the entire string, while for lists and tuples, it checks each element for a match against the specified value.
- During each iteration, the function compares the current element (or substring) with the specified value, incrementing a counter each time a match is found.
- The function is case-sensitive when counting occurrences in strings, meaning "Hello" and "hello" would be counted separately; for instance, calling "Hello".count("hello") returns 0, while "Hello".count("Hello") returns 1.
- After completing the iteration, the function returns the total count as an integer, yielding the number of occurrences if found, or 0 if the value is not present in the sequence.
- The count() function iterates through the entire sequence, which can lead to performance issues with very large datasets, running in O(n) time complexity, where n is the length of the sequence.
- The count() function can only count one specified value at a time, requiring multiple calls or alternative methods for counting multiple values, and it cannot be used on non-iterable data types.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Using count() Function In Python With Lists
The list count method is a built-in function in Python. It counts how many times an item appears in a list. This method is straightforward to use. You call it on a list and pass the item you want to count as an argument. For example, my_list.count(item) returns the number of occurrences of that item.
Python's list count can handle various data types, including numbers and strings. It works by checking each element in the list against the specified item. The function then tallies up all matches. This makes it useful for tasks like item counting or determining duplicates in a dataset.
Let's say we have a list of numbers, and we want to count how many times the number 5 appears in the list.
Code Example:
IyBMaXN0IG9mIG51bWJlcnMKbnVtYmVycyA9IFsxLCA1LCAzLCA1LCA3LCA5LCA1LCAyLCA1XQoKIyBVc2luZyBjb3VudCgpIHRvIGNvdW50IG9jY3VycmVuY2VzIG9mIHRoZSBudW1iZXIgNQpjb3VudF9vZl81ID0gbnVtYmVycy5jb3VudCg1KQoKIyBPdXRwdXQgdGhlIHJlc3VsdApwcmludChmIlRoZSBudW1iZXIgNSBhcHBlYXJzIHtjb3VudF9vZl81fSB0aW1lcyBpbiB0aGUgbGlzdC4iKQo=
Output:
The number 5 appears 4 times in the list.
Explanation:
In the above code example-
- We start by defining a list of numbers as [1, 5, 3, 5, 7, 9, 5, 2, 5].
- Next, we use the count() method to count how many times the number 5 appears in the list.
- The result of this count is stored in the list variable count_of_5.
- We then print the result using an f-string. The output will tell us how many times the number 5 is present in the list.
Using count() Function In Python With Strings
The count() function in Python is specifically designed for strings. This method counts how many times a substring appears within a string. For example, if you have a string like "hello world", calling "hello world".count("o") returns 2. This shows that the letter "o" appears twice in the string.
Let’s say we have a sentence, and we want to count how many times the word "Python" appears in it.
Code Example:
IyBTdHJpbmcgY29udGFpbmluZyB0aGUgd29yZCAiUHl0aG9uIiBtdWx0aXBsZSB0aW1lcwpzZW50ZW5jZSA9ICJQeXRob24gaXMgZnVuLiBQeXRob24gaXMgZWFzeSB0byBsZWFybi4gSSBsb3ZlIFB5dGhvbiEiCgojIFVzaW5nIGNvdW50KCkgdG8gY291bnQgb2NjdXJyZW5jZXMgb2YgdGhlIHN1YnN0cmluZyAiUHl0aG9uIgpjb3VudF9weXRob24gPSBzZW50ZW5jZS5jb3VudCgiUHl0aG9uIikKCiMgT3V0cHV0IHRoZSByZXN1bHQKcHJpbnQoZiJUaGUgd29yZCAnUHl0aG9uJyBhcHBlYXJzIHtjb3VudF9weXRob259IHRpbWVzIGluIHRoZSBzZW50ZW5jZS4iKQo=
Output:
The word 'Python' appears 3 times in the sentence.
Explanation:
In the above code example-
- We start by defining a string sentence that contains the word "Python" multiple times.
- Next, we use the count() method to count how many times the substring "Python" appears in the sentence.
- The result of this count is stored in the variable count_python.
- We then print the result using an f-string, which outputs the message to the console.
- The output will tell us how many times the word "Python" appears in the sentence, which in this case is 3.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Using count() Function In Python With Tuples
Counting elements in tuples works similarly to strings. Tuples are immutable sequences in Python. They can hold multiple items. The count() method tallies how many times an element appears.
For example, if you have a tuple like (1, 2, 2, 3), using tuple.count(2) returns 2. This means the number 2 occurs twice. Tuples can store different data types. You can count integers, strings, or even other tuples. This flexibility makes tuples useful for various applications.
Syntax:
tuple.count(element)
Here:
- tuple: The tuple in which you want to count occurrences.
- element: The element you want to count in the tuple.
Let’s say we have a tuple of numbers, and we want to count how many times the number 10 appears in the tuple.
Code Example:
IyBUdXBsZSBvZiBudW1iZXJzCm51bWJlcnNfdHVwbGUgPSAoMTAsIDIwLCAxMCwgMzAsIDEwLCA0MCwgNTApCgojIFVzaW5nIGNvdW50KCkgdG8gY291bnQgb2NjdXJyZW5jZXMgb2YgdGhlIG51bWJlciAxMApjb3VudF9vZl8xMCA9IG51bWJlcnNfdHVwbGUuY291bnQoMTApCgojIE91dHB1dCB0aGUgcmVzdWx0CnByaW50KGYiVGhlIG51bWJlciAxMCBhcHBlYXJzIHtjb3VudF9vZl8xMH0gdGltZXMgaW4gdGhlIHR1cGxlLiIpCg==
Output:
The number 10 appears 3 times in the tuple.
Explanation:
In the above Python program-
- We define a tuple of numbers called numbers_tuple, which contains the values (10, 20, 10, 30, 10, 40, 50).
- Next, we use the count() method to count how many times the number 10 appears in the tuple.
- The result of this count is stored in the variable count_of_10.
- We then print the result using an f-string, which outputs the message to the console.
- The output will indicate how many times the number 10 appears in the tuple, which in this case is 3.
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Practical Applications Of count() Function In Python
The Python count function has many practical applications across different contexts, especially when working with lists or strings. Here are some common use cases:
-
Counting Occurrences in a Dataset: When working with a dataset, you can use count() function in Python to find how many times a certain value (or element) appears. For instance, in a list of customer orders or transactions, you can count how often a certain product was purchased. For Example-
orders = ['apple', 'banana', 'apple', 'orange', 'apple', 'banana']
apple_count = orders.count('apple')
print(apple_count) # Output: 3
-
Text Analysis and Word Frequency: The count() function in Python is useful in text processing, such as counting the number of occurrences of a particular word or character in a piece of text, making it valuable for tasks like word frequency analysis, sentiment analysis, or simple spell checking. For Example-
text = "Python is great. Python is easy to learn."
python_count = text.count("Python")
print(python_count) # Output: 2
-
Finding Duplicates in Lists: In data validation, you might want to identify duplicate entries in a list by counting how often certain elements appear. This can help identify issues such as repeated data entries in a list or array. For Example-
numbers = [1, 2, 3, 2, 4, 5, 3, 2]
duplicate = [n for n in numbers if numbers.count(n) > 1]
print(set(duplicate)) # Output: {2, 3}
-
Validating User Input: You can also use the count() function in Python to check whether a specific character, word, or phrase exists in a string. This process can be useful in form validation (e.g., checking if a password contains a certain number of special characters). For Example-
password = "myp@ssword123"
special_chars = "!@#$%^&*()"
count_special = sum(password.count(char) for char in special_chars)
print(count_special) # Output: 1
-
DNA Sequence Analysis: In bioinformatics, the count() function in Python can be used to count the occurrences of nucleotides (A, T, G, C) in a DNA sequence, which is important for understanding genetic patterns and mutations. For Example-
dna_sequence = "ATGCGATGACCTG"
adenine_count = dna_sequence.count('A')
print(adenine_count) # Output: 3
-
Log or Error Monitoring: When analyzing log files or system output, the count() function in Python can help identify how many times certain keywords (such as "ERROR" or "WARNING") appear, which is useful for system health monitoring and debugging. For Example-
logs = "INFO: Server started. ERROR: Failed to connect. INFO: Retry. ERROR: Connection failed."
error_count = logs.count('ERROR')
print(error_count) # Output: 2
-
Counting Delimiters in CSV Parsing: When manually parsing CSV (comma-separated values) files or similar data formats, you might want to count how many delimiters (commas, semicolons, etc.) are present in a string, ensuring that the correct number of fields are processed. For Example-
csv_line = "name,age,city,country"
comma_count = csv_line.count(',')
print(comma_count) # Output: 3
-
Pattern Matching in Strings: You can use the count() function in Python to detect simple patterns in strings, such as counting how many times a specific substring (e.g., a date format or recurring symbols) appears in a larger text or document. For Example-
log = "2023-05-01: login successful; 2023-05-02: login failed; 2023-05-03: login successful"
date_count = log.count("2023-05")
print(date_count) # Output: 3
-
Counting Characters or Words for Formatting: When working with text formatting or preprocessing, such as preparing documents or HTML/XML, the count() function in Python can help count how many specific tags or line breaks are present, assisting with formatting or cleaning data. For Example-
html_content = "<html><body><h1>Title</h1><p>Paragraph</p></body></html>"
tag_count = html_content.count('<')
print(tag_count) # Output: 4
-
Voting or Survey Result Analysis: For survey results or voting systems, the count() function in Python can be used to count the number of votes or responses for specific options, helping to tally up results. For Example-
votes = ['Yes', 'No', 'Yes', 'No', 'Yes']
yes_count = votes.count('Yes')
print(yes_count) # Output: 3
Conclusion
The count() function in Python language is a powerful and versatile tool for analyzing sequences such as strings, lists, and tuples. Its simplicity allows for quick and efficient counting of occurrences of specific elements or substrings, making it invaluable for data manipulation and analysis. While it offers straightforward functionality, it’s essential to be aware of its case sensitivity and performance considerations with large datasets.
Additionally, understanding its limitations, such as its inability to count multiple elements at once or work seamlessly with complex data types, can help you use it effectively. By utilizing the count() function alongside other Python features and libraries, developers can enhance their data processing capabilities, enabling more sophisticated analyses and insights.
Frequently Asked Questions
Q. What types of sequences can the count() function be used on?
The count() function in Python can be used on several types of sequences, including:
-
Strings: You can use count() function in Python to find the number of occurrences of a substring within a string.
text = "Hello, world! Hello again!"
count_hello = text.count("Hello") # Returns 2
-
Lists: The count() method can be used to count the occurrences of a specific element in a list.
numbers = [1, 2, 3, 1, 4, 1]
count_ones = numbers.count(1) # Returns 3
-
Tuples: Similar to list operations, you can use count() to find the number of times a specific element appears in a tuple.
my_tuple = (1, 2, 3, 1, 4, 1)
count_ones_in_tuple = my_tuple.count(1) # Returns 3
-
Bytes and Bytearrays: The count() method can also be used with bytes and bytearrays to count the occurrences of a byte or sequence of bytes.
byte_seq = b"Hello, Hello!"
count_hello_bytes = byte_seq.count(b"Hello") # Returns 2
-
Other Iterable Sequences: While the built-in count() method is specifically tied to these types, you can implement similar counting behavior in custom iterable classes by defining your own count() method.
Overall, count() function is versatile and can be applied to any sequence type that supports this method, making it a useful tool for data analysis and manipulation in Python.
Q. Is the count() function in Python case-sensitive when counting substrings in strings?
Yes, the count() function in Python is case-sensitive when counting substrings in strings. This means that it treats uppercase and lowercase letters as distinct characters. For example:
text = "Hello World"
count_lower = text.count("hello") # This will return 0
count_upper = text.count("Hello") # This will return 1
In the above example, text.count("hello") returns 0 because "hello" (all lowercase) does not match "Hello" (with an uppercase 'H'). Conversely, text.count("Hello") returns 1 because it matches exactly with the substring in the string.
If you need a case-insensitive count, you can convert both the original string and the substring to the same case (either all lower or all upper) before counting:
count_case_insensitive = text.lower().count("hello") # This will return 1
This way, you can achieve case-insensitive counting.
Q. What does the count() function return if the specified element or substring is not found?
If the specified element or substring is not found, the count() function in Python returns 0.
For example:
- In a list, if you call list.count(element) and element is not present in the list, it will return 0.
- In a string, if you use string.count(substring) and substring does not exist within the string, it will also return 0.
This behavior makes the count() function in Python useful for checking the presence of elements or substrings without raising an error, as it simply indicates the absence with a return value of 0.
Q. Can I use the count() function with a list of mixed data types?
Yes, the count() function can be used with lists that contain mixed data types. It will count occurrences based on the exact type and value. For example, in the list [1, 'apple', 1, 'banana'], calling list.count(1) will return 2, while list.count('1') will return 0, since the types are different.
Q. Are there any limitations to the count() function in Python?
Yes, there are some limitations to the count() function in Python, depending on the context in which it is used:
- Data Type Dependency: The count() method behaves differently based on the data type. For instance, for strings, it counts the occurrences of a substring, while for lists, it counts the occurrences of a specific element. It may not handle complex data types (like objects) intuitively without custom logic.
- Performance with Large Data: Using count() function on large datasets, especially with lists or strings, can be inefficient because it iterates through the entire collection. In large datasets, this can lead to performance issues.
- Limited to Exact Matches: The count() method only counts exact matches. For example, it won't count variations in case or whitespace in strings, unless you normalize the data beforehand.
- Single Value Counting: The count() function in Python typically counts only one specific value at a time. If you need to count multiple values or conditions, you may need to use additional logic, such as loops or comprehensions.
- Not Suitable for Multidimensional Data: When dealing with multidimensional data (like lists of lists or arrays), the built-in count() method may not be directly applicable, requiring more complex implementations or the use of libraries like NumPy or Pandas.
- No Aggregation: The count() function in Python does not provide aggregation features like grouping, which are available in libraries like Pandas.
Q. Can I combine count() function in Python with other functions?
Yes, you can combine the count() function in Python with other functions and methods to perform more complex data analysis.
- For instance, if you're working with lists or strings, you can use count in combination with list comprehensions or the filter function to count specific items based on certain conditions.
- In libraries like Pandas, you can use the count method on DataFrame objects along with aggregation functions like groupby, sum, or mean to analyze data. For example, you can count the number of occurrences of each unique value in a column and then calculate the average of another column for those grouped values.
- You can also use Python count in conjunction with other statistical functions, such as apply, to perform operations across rows or columns, allowing for sophisticated data manipulation and analysis. This makes Python a powerful tool for data science and analytics.
This compiles our discussion on the count() function in Python. Here are a few other topics that you might be interested in reading:
- Difference Between Java And Python Decoded
- Difference Between C and Python | C or Python - Which One Is Better?
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment