Table of content:
- What Is String Concatenation In Python?
- How To Concatenate Two Strings In Python?
- Python String Concatenation With Addition/ Concatenation Operator
- Repetition Operator For Python String Concatenation
- Modulus Operator For Python String Concatenation
- Assignment Operator For Python String Concatenation
- Comma Operator For Python String Concatenation
- The Format() Method For Python String Concatenation
- The Join() Method For Python String Concatenation
- The Join() And Str() Methods To Join List Of Numbers Into A String
- The Join() Method Concatenate List Of Strings In Python To A String
- Python String Concatenation With StringIO Class
- F-Strings For Python String Concatenation
- Conclusion
- Frequently Asked Questions
Python String Concatenation In 10 Easy Ways Explained (+Examples)
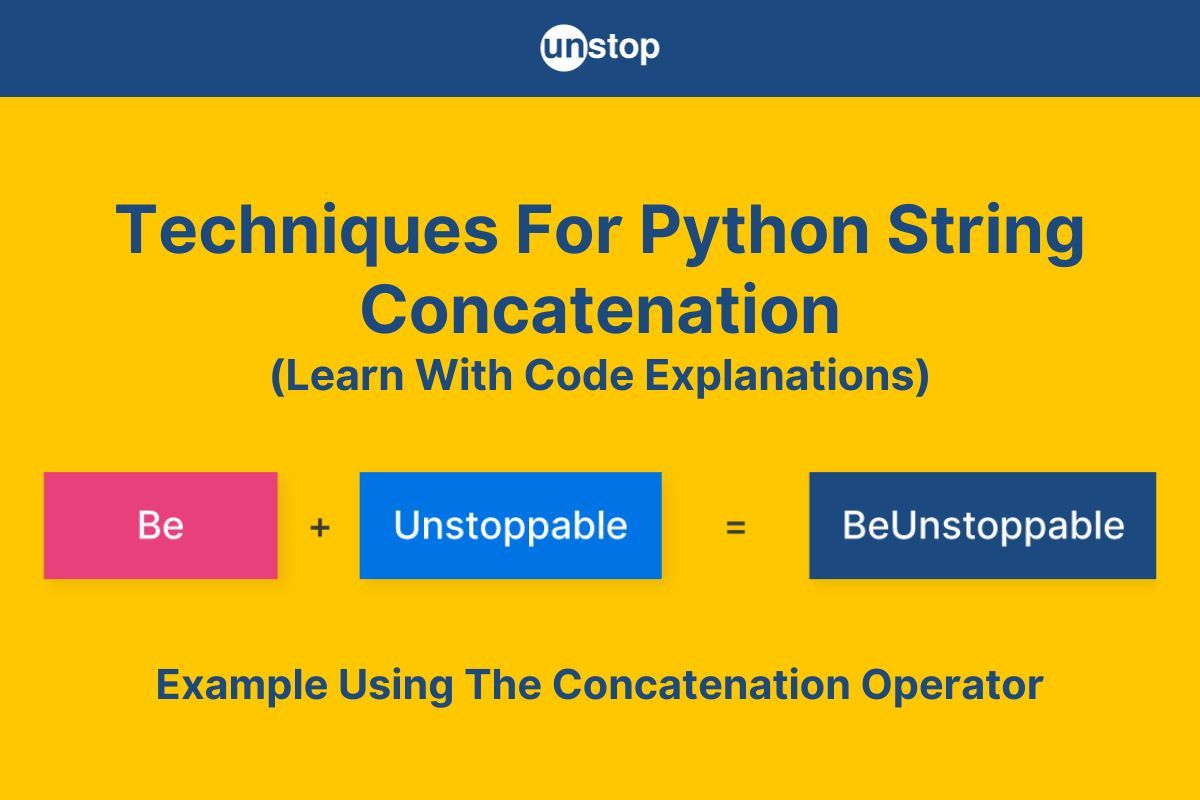
Python, a versatile and powerful programming language, offers a multitude of features that make it a favorite among developers. One of the fundamental operations in programming is string manipulation, and Python programming provides a straightforward and efficient way to concatenate strings. In this article, we'll delve into the various methods Python offers for concatenating strings and explore some best practices.
What Is String Concatenation In Python?
A string in Python is a sequence of characters that can be created in three ways, i.e., using single quotes, double quotes, or even triple quotes. These data types help us work with textual data and manipulate them in our Python programs.
Amongst various operations/ manipulations we can conduct on these variables, Python string concatenation is one of the most commonly used operations. It is defined as the method of joining two or more strings to create a new string.
- The simplest way to concatenate strings in Python is by using the concatenation operator (+) or the addition arithmetic operator for strings.
- A Python string is immutable (i.e., it cannot be edited once created), which is why a a new variable is assigned the value of the new string on concatenation.
- It may also be sometimes used to add spaces, punctuations, etc., between separate strings.
Let us consider a practical example where we have two separate initial strings, “Un” and “stop”. When we concatenate these two strings, we obtain a new string, “Unstop”.
“Un” + “Stop” = “Unstop”
How Is Python String Concatenation Different From String Formatting?
Python string concatenation is the process of joining two or more strings into a new string. It works only with strings. In contrast, string formatting allows inserting variables (other non-string objects) into strings. Formatting may also be sometimes used to control their alignment, formatting, etc.
- String formatting is slower than the string concatenation process. This is because formatting has to parse the string before inserting variables into it.
- However, formatting holds higher flexibility than string concatenation. For example, we can add other non-string objects into strings with simple formatting.
- String formatting is comparatively more readable and maintainable due to its syntax and the ease of changing the values assigned to variables instead of the entire code in concatenation.
Example Of Python String Concatenation Vs. String Formatting Function
Let's take a look at an example that showcases how we can use the concatenation operators, as well as the formatting technique to join two strings. The result for both operations will be the same, but the approach differs.
Sample Code:
# Initial string variables
founder = "Ankit Aggarwal"
year = 2019
# String concatenation
print(founder + " started Unstop in the year " + str(year) + ".")
# String formatting
print("{founder} started Unstop in the year {year}.".format(founder=founder, year=year))
Output:
Ankit Aggarwal started Unstop in the year 2019.
Ankit Aggarwal started Unstop in the year 2019.
Explanation:
In the sample Python program above-
- We begin by defining a variable of string data type, called founder and initialize it with the value Ankit Aggarwal using the double quotes method.
- Another interger type variable year is initialized with the value 2019.
- Next, we use a print() statement to display the result of a quick string concatenation operation. Inside the statement-
- We use the concatenation operator (+) to join the string variable founder with a new string- _started Unstop in the year.
- Then, we use the str() method to convert the value of the year variable to a string and then concatenate it with the previous string.
- Lastly, we add a single character (full stop) in string form to complete the new string. The print() statement then prints the new string to the console.
- We define a second print() statement that displays the result of string formatting.
- Here, we begin by inserting the placeholders, founder, and year in curly braces {}.
- After that, we use the string formatting function. i.e., .format() to substitute the placeholders with the values of founder and year.
- Both statements output information about the founder starting Unstop in the year 2019.
How To Concatenate Two Strings In Python?
In Python, there are several techniques for string concatenation. String concatenation refers to the process of combining multiple strings into a single string. These methods include-
- Concatenate Operator
- Repetition Operator
- Modulus Operator
- Format Function for String Concatenation
- Python F-String Method
- Join() Method
- Assignment Operator
- Comma Operator
We will explore all of these methods along with examples in the sections ahead.
Python String Concatenation With Addition/ Concatenation Operator
The simplest method to concatenate strings in Python is by using the plus operator (+), also known as the concatenation operator. However, it is important to note that this string concatenation process/ operator does not add blank spaces between the concatenated strings by default. Hence, spaces or other separators need to be added within quotes.
- This method has a time complexity of O(n^2), where n refers to the length of the longer string.
- This explains the quadratic growth of the concatenation time of two strings with their increasing length.
NOTE: The time complexity of an algorithm is defined as the measure of time it takes to run. Also referred to as the runtime, it is a function of the input size and is commonly expressed using the big O notation.
Concatenating Two Or More Strings
We can use the concatenation operator to join two or more strings in Python. Take a look at the example below to understand the practical implementation of the same.
Sample Code:
# Initializing two strings
str1 = 'String Concatenation'
str2 = 'in Python'
# Using the + operator for string concatenation
result = str1 + " " + str2
# Print the result
print(result)
Output:
String Concatenation in Python
Explanation:
In the example Python program-
- We begin by initializing two variables, str1 and str2, with the values (String Concatenation) and (in Python), respectively.
- Then, we use the concatenation operator (+) to concatenate the two strings along with a space (as a separator string) in between.
- The outcome of this operation is stored in the result variable.
- Lastly, we use the print() statement to display the content of the result variable to the console.
Concatenating Strings In Python With Numbers
We have already seen how to concatenate two or more strings in Python. We can also use the concatenation operator to join strings with numbers, but for that, we must convert the number values into a string. This is done with the help of the built-in string method (str()) in Python.
Let's compare two examples showing what happens when we concatenate string in Python with numbers without converting them, and then the correct way to concatenate strings and numbers.
Sample Code:
a = 'Welcome'
b = 2
c = 'Unstop!'
print(a + " " + b + " " + c)
Output:
TypeError: can only concatenate str (not “int”) to str
Explanation:
In the lines of code above, we observe that concatenating strings with numbers in Python using the + operator throws a TypeError stating that it can only concatenate strings and not the integer data types. This is because we are trying to concatenate a string data type with an integer data type.
Therefore, the number needs to be first typecasted to a string and then be used in concatenation operation. The correct code structure is given below.
Correct Code:
# Initializing three variables
a = 'Welcome'
b = 2
c = 'Unstop!'
# Using the + operator for string concatenation with str()
result = a + " " + str(b) + " " + c
# Print the result
print(result)
Output:
Welcome 2 Unstop!
Explanation:
- The example is the same as the one above, where we have three variables, i.e., a (with string value Welcome), b (with integer value 1), and c (with string value Unstop).
- We then use the concatenation operator (+) to join the strings and the integer variable. The only difference is that we use the str() function to convert the integer variable to a string.
- The outcome of the Python string concatenation is stored in the result variable.
- Lastly, we use the print() function to display the value of the result variable.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Repetition Operator For Python String Concatenation
The repetition operator is represented by the asterisk symbol (*) and is used to concatenate the same string to itself. It forms multiple copies of the input/ initial string, combines them, and gives the concatenated string as output.
Code Example:
# Defining a string variable
my_str = 'Python is a programming language.'
# Using the * operator for string repetition
result = my_str * 3
# Print the result
print(result)
Output:
Python is a programming language.Python is a programming language.Python is a programming language.
Explanation:
In this code example-
- We initialize a string variable, my_str, with the value- Python is a programming language.
- Next, we use the repetition operator (*) for string repetition, and the string is repeated three times.
- The outcome of this operator is stored in the variable result.
- Finally, we employ the print() function to display the new string to the console.
Time Complexity: The time complexity for this method in Python is O(n^2), where n refers to the number of strings being concatenated. This is due to the creation of a new string for each concatenation, with the time taken being proportional to its length.
Modulus Operator For Python String Concatenation
The modulo operator (%) is generally used for string formatting. However, it may also be sometimes used for concatenation. The operator is a part of the format specifier. When two string types are written side by side using this specifier, the operator will lead to the concatenation of the strings. Take a look at the example below, which shows the implementation of this operator for Python string concatenation.
Code Example:
# Defining strings
str1 = 'Python String'
str2 = 'Concatenation'
# Using string formatting with % operator
result = '%s %s' % (str1, str2)
# Print the result
print(result)
Output:
Python String Concatenation
Explanation:
- We begin the Python example by initializing two string variables, i.e., str1 with the value Python String and str2 with the value Concatenation.
- Next, we use the modulo operator (%) to combine the two strings with a space in between.
- Here, we initialize a new string with %s%s, which are the placeholders for str1 and str2.
- We specify that the placeholders must be replaced with the values of the string.
- The outcome is stored in the variable result.
- Finally, using the print() statement, we display the new string to the console.
Time Complexity: The time complexity for this method is also O(n^2), where n is the number of strings being concatenated. This is due to the creation of a new string for each concatenation, with the time taken being proportional to its length.
Assignment Operator For Python String Concatenation
We can use the compound assignment operator, i.e., addition assignment (+=), to concatenate strings in Python. This method is simply an extension of using the concatenation operator (+), as it automatically assigns the updated string value to the given variable name. It is used to add a certain value to a given variable, but note that it does not add any spaces between strings by default.
Code Example:
# Initializing strings
str1 = "Hello"
str2 = "World"
# Using the += operator for string concatenation in place
str1 += str2
# Print the result
print(str1)
Output:
HelloWorld
Explanation:
In this example code, we showcase Hello-World string concatenation by using the compound assignment operator.
- We define two string variables, str1 and str2, and initialize them with values Hello and World, respectively.
- Next, we use the assignment addition operator (+=) for in-place string concatenation. It appends the content of str2 to str1.
- The outcome of this operation is updated back to variable str1, which we then display on the console using the print() function.
Time Complexity: This method in Python has a time complexity of O(n^2), where n is the number of strings being concatenated. This is due to the creation of a new string for each concatenation, with the time taken being proportional to the length of the string.
Comma Operator For Python String Concatenation
String concatenation using a comma is a simple string method of combining two or more strings together, which adds a space between strings by default. The comma operator is often used as a separator between strings. But here, we use it as a separator between two variables inside the print() statement, and the outcome is the two (or more) strings being printed to the console as one, separated by whitespace.
Code Example:
# Initializing strings
s1 = "Hello"
s2 = "World"
# Using the print statement to display both strings
print(s1, s2)
Output:
Hello World
Explanation:
- We declare two string variables, s1 with the value Hello and s2 with the value World, at the beginning of the code example.
- Then, we use the comma operator in the print() statement as a separator between the two arguments (s1 and s2).
- When you use a comma between items in a print statement in Python, it automatically adds a space between the items in the output.
Time Complexity: The time complexity for this method is O(n^2) as the comma operator is evaluated from left to right, and the previous string is completely evaluated for each string being concatenated.
The Print() Function For Python String Concatenation
Apart from its most prominent use in displaying the output on the window, the print() function can also be used to join strings and then display them. That is while printing, it automatically concatenates the string with the other values passed to it.
This method simplifies the efficient code by reducing the need to declare variables for storing the strings and the typecasting of numbers to strings.
Code Example:
# Print statement with multiple arguments
print("Welcome", 2, "Unstop!")
Output:
Welcome 2 Unstop!
Explanation:
- This example uses a simple print() statement with the intention to display the text Welcome, followed by the number 2, and then the string Unstop!.
- Multiple object arguments are separated by commas within the print function. It then displayed the concatenated string to the console.
Time Complexity: The time complexity for this automatic string concatenation method is O(n^2), where n is the number of strings being concatenated, as this function creates a new string before concatenating each string.
The Format() Method For Python String Concatenation
The format() method is generally used for individual string formatting. However, it may also be used for concatenation as it allows inserting variables into strings. This method is slower than other methods of string concatenation. However, it is more readable and maintainable due to its syntax and the ease of changing the values of variables instead of changing the entire code as in Python string concatenation.
Code Example:
# Initializing two variables
name = "Unstop"
year = 2019
# Using the format method to create a formatted string
output_string = "{0} was founded in the year {1}.".format(name, year)
# Print the result
print(output_string)
Output:
Unstop was founded in the year 2019.
Explanation:
- In this code example, we initialize one string variable, name, with the value Unstop and one integer variable, year, with the value 2019.
- Next, we use the format() method to create a formatted string. Inside the string-
- We have two placeholders inside the curly braces, i.e., {0} and {1}.
- These curly brackets are replaced with the values of name and year (respectively), which are specified using the format() method at the end of the formatted string.
- The outcome of this string formatting and concatenation is saved in the output_string variable.
- Lastly, we display this new string to the console using the print() function.
Time Complexity: The time complexity for this method is O(n), where n is the length of the output string. This is because the format() function first parses the format string before constructing it by appending the formatted values into output.
The Join() Method For Python String Concatenation
Traditionally, the join() method is used to join lists into strings. However, it can also be used for the concatenation of a tuple of strings in Python. It is used to concatenate individual strings but with a delimiter between strings. In other words, the function takes strings as inputs and concatenates them while separating them with the pre-defined delimiter.
This delimiter string can be a single space or any other entity. For this, you must specify the delimiter in a string format before you apply the join() method to the strings.
Code Example:
# Creating three strings
s1 = "Welcome"
s2 = "to"
s3 = "Unstop!"
# Join the strings with a space as a separator
result = " ".join([s1, s2, s3])
# Print the result
print(result)
Output:
Welcome to Unstop!
Explanation:
- We begin the code example by declaring and initializing three variables, i.e., s1, s2, and s3, with the values Welcome, to, and Unstop!, respectively.
- Next, we use the join() method to concatenate the three strings into one, with a whitespace (" ") as the separator.
- The outcome of this join() method is stored in the variable result, which is then displayed to the console using a print() statement.
Time Complexity: The time complexity for this method is O(n), where n is the total length of strings being concatenated. This is due to the looping of the join() function over each string in an iterable and the insertion of a separator at the end of each string.
Also read- How To Reverse A String In Python? 10 Easy Ways With Examples!
The Join() And Str() Methods To Join List Of Numbers Into A String
The join() method is generally used to convert a list of characters to a string, and the str() method is used to convert non-string objects to strings. We can combine these when we have a list of numbers as input, and we require the output as a string separated by some delimiter. Take a look at the example below, for a better understanding of the same.
Code Example:
# Creating a list of numbers
num = [1, 2, 3, 4, 5]
# Convert numbers to strings using list comprehension
strings_list = [str(number) for number in num]
# Join the strings using "," as a separator
string = ','.join(strings_list)
# Print the result
print(string)
Output:
1,2,3,4,5
Explanation:
- We begin by initializing a list variable called num with the values- [1, 2, 3, 4, 5].
- Next, using a list comprehension with the str() method, we convert each number in the list to a string.
- We use list comprehension to traverse each element of the lists.
- The str() function then converts them to the string data type.
- The result is stored in the list variable strings_list.
- After that, we concatenate the individual strings to a common string using the join() method.
- We also use a comma as a separator/ delimiter, and the outcome is stored in the variable string.
- Then, we display the new string representation on the output window using the print() statement.
The Join() Method Concatenate List Of Strings In Python To A String
Just like we can use the join() method to convert a list of characters to a string, we can also use this concatenation technique to join a given list of strings into a single string. And just like in other cases where we use the join() method, we can use a specific delimiter to separate the strings.
Code Example:
# Creatig a list of strings
strings_list = ["This", "is", "a", "tutorial", "on", "Unstop"]
# Join the strings using "." as a separator
new_string = ".".join(strings_list)
# Print the result
print(new_string)
Output:
This.is.a.tutorial.on.Unstop
Explanation:
- In this example, we initialize a list of strings called strings_list with the values- [This, is, a, tutorial, on, Unstop].
- Then, we use the join() method on the strings_list variable to concatenate the strings with the full stop/ dot (.) as the separator/ delimiter.
- The outcome string is stored in the variable new_string., which we then print with the use of the print() function.
Python String Concatenation With StringIO Class
The StringIO class provides an alternative to string concatenation in Python without creating intermediate strings. This results in more efficient code by improving the performance while performing the task for large or unknown numbers of strings. It consists of a few functions which help concatenate strings in Python.
We can use the write() method from the StringIO class to store the strings in a buffer memory and then print them to the console as a single concatenated string.
Code Example:
from io import StringIO
# Create a StringIO object
sio = StringIO()
# Write "Hello" to the StringIO object
sio.write("Hello ")
# Write "World" to the StringIO object
sio.write("World")
# Print the combined content of the StringIO object
print(sio.getvalue())
Output:
Hello World
Explanation:
- We begin the code by importing the StringIO class from the io module.
- Next, we create an object of the StringIO class called sio. This object serves as an in-memory buffer.
- Then, using the write() method, we add the string- Hello (with a whitespace after it) to the StringIO buffer.
- Again, using the write() operation appends the string- World to the existing content in the StringIO buffer.
- Finally, we use the getvalue() method, which retrieves the combined content of the StringIO object inside a print() statement to display the concatenated string (Hello World) to the console.
Time Complexity: The time complexity for this method is O(n^2), where n is the length of strings being concatenated. This is because the StringIO class uses a resizable buffer to store strings on every concatenation.
F-Strings For Python String Concatenation
F-strings can be used for concatenation and formatting in a more concise way for Python 3.6 or above versions. It is a cleaner and easier method than the format() function. In this method, we use the notation (f" ") to mark the string we are defining. Inside the string, we can add placeholders in curly braces, which will be replaced by their values on execution.
Code Example:
#Declaring and initializing two variables
name = "Ankit Aggarwal"
year = 2019
#Unsing formatted string for concatenation
concatstr = f"Unstop was founded by {name} in the year {year}."
print(concatstr)
Output:
Unstop was founded by Ankit Aggarwal in the year 2019.
Explanation:
In the code above-
- We first declare and initialize two variables, i.e., name (type string) with the value- Ankit Aggarwal and year (type integer) with the value 2019.
- Then, we define an f-string named concatstr (this is the Python string concat), incorporating the values of name and year into a descriptive message.
- After that is done, we display its value using the print() statement in the last step.
Time Complexity: This method has a time complexity of O(n) as the compiler directly concatenates the strings without copying them into a new string object, n being the length of the string.
How Are Python F-Strings Different From String Literals?
F-strings, introduced in Python 3.6, are a specific type of string literals that allow for easy string interpolation. While both f-strings and traditional string literals are used to represent textual data in Python, the key difference lies in their ability to include expressions and variables within the string itself.
Here are the main differences between f-strings and traditional string literals-
Feature | Traditional String Literals | F-Strings |
---|---|---|
Expression Evaluation | Use % formatting or str.format() | Directly embed regular expressions within curly braces {} |
Readability and Conciseness | Can be less readable, especially with multiple variables. | More concise and readable syntax. |
Ease of Use | Involves additional formatting syntax. | Intuitive and straightforward, with no additional syntax needed. |
Performance | Can be less efficient, especially in terms of concatenation. | Generally more efficient, especially in concatenation operations. |
Conclusion
Python string concatenation refers to the process of joining two or more strings into a new string. There are multiple methods of string concatenation, including common operations like the concatenation operator (+), assignment addition operator (+=), comma operator (,), and modulo operator (%). And some advanced/ alternative string concatenation approaches like the join() method, str(), stringIO class, and many more. We can also use some string formatting methods like the F-strings and the format() method for concatenation. It is important to find the most optimal method to cater to your specific requirements.
Frequently Asked Questions
Q. How do you concatenate a list in Python?
Lists in Python can be concatenated by several popular methods, the easiest of them being the concatenation operator (+) and the extend() function similar to the join() function in strings.
Using the + Operator: You can use the + operator to concatenate two or more lists.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
concatenated_list = list1 + list2
The concatenated_list will be [1, 2, 3, 4, 5, 6].
Using the extend() Method: The extend() method is used to append the elements of one list to another.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list1.extend(list2)
After execution, the list1 will be [1, 2, 3, 4, 5, 6].
Q. What's the difference between using + and += for Python string concatenation?
The table below highlights the difference between the concatenation operator and the compound assignment addition operator for Python string concatenation.
Operation | Explanation |
---|---|
Concatenation Operator (+) |
The concatenation operator (+) creates a new string by concatenating the values on either side. It does not modify the original strings but instead produces a new string as a result of concatenation. For example: |
Assignment Addition Operator (+=) |
The addition assignment operator (+=) performs in-place concatenation, modifying the existing string on the left side of the operator. It appends the content on the right side to the original string. For example: |
Q. How do you concatenate strings in Python without spaces?
To concatenate strings without spaces in Python, you can use the concatenation operator (+) or other techniques for string concatenation while omitting spaces between the strings. Here's an example using the + operator for string formatting-
str1 = "Hello"
str2 = "World"
concatenated_string = str1 + str2
In this example, concatenated_string will be "HelloWorld" with no spaces between the two strings.
Q. How do you capitalize the first character of a string in Python?
In Python, you can capitalize the first character of a string using the capitalize() method or by using string slicing along with the upper() method. Below are examples of both the common methods-
Using capitalize() method: The capitalize() method capitalizes the first character of a string.
text = "python is awesome"
capitalized_text = text.capitalize()
After execution, capitalized_text will be- Python is awesome.
Using string slicing and upper() method: You can use string slicing to extract the first character and then apply the upper() method to capitalize it.
text = "python is cool"
modified_text = text[0].upper() + text[1:]
After execution, modified_text will be- Python is cool.
Q. How do I handle concatenating strings with different data types, such as a string and an integer?
When concatenating strings with different data types, such as a string and an integer, you need to convert the non-string data type to a string before concatenation. You can achieve this by using the str() function, which converts the non-string value to its string representation. Here's an example:
Code Example:
# Example: Concatenating a string and an integer
string_part = "The value is: "
integer_part = 42
# Convert the integer to a string before concatenation
result = string_part + str(integer_part)
# Print the result
print(result)
Output:
The value is: 42
This compiles our discussion on Python string concatenation. Here are a few other amazing articles you must read:
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment