- What Is Data Structure?
- Key Features Of Data Structures
- Basic Terminologies Related To Data Structures
- Types Of Data Structures
- What Are Linear Data Structures & Its Types?
- What Are Non-Linear Data Structures & Its Types?
- Importance Of Data Structure In Programming
- Basic Operations On Data Structures
- Applications Of Data Structures
- Real-Life Applications Of Data Structures
- Linear Vs. Non-linear Data Structures
- What Are Algorithms? The Difference Between Data Structures & Algorithms
- Conclusion
- Frequently Asked Questions
- What Is Asymptotic Notation?
- How Asymptotic Notation Helps In Analyzing Performance
- Types Of Asymptotic Notation
- Big-O Notation (O)
- Omega Notation (Ω)
- Theta Notation (Θ)
- Little-O Notation (o)
- Little-Omega Notation (ω)
- Summary Of Asymptotic Notations
- Real-World Applications Of Asymptotic Notation
- Conclusion
- Frequently Asked Questions
- Understanding Big O Notation
- Types Of Time Complexity
- Space Complexity In Big O Notation
- How To Determine Big O Complexity
- Best, Worst, And Average Case Complexity
- Applications Of Big O Notation
- Conclusion
- Frequently Asked Questions
- What Is Time Complexity?
- Why Do You Need To Calculate Time Complexity?
- The Time Complexity Algorithm Cases
- Time Complexity: Different Types Of Asymptotic Notations
- How To Calculate Time Complexity?
- Time Complexity Of Sorting Algorithms
- Time Complexity Of Searching Algorithms
- Writing And optimizing An algorithm
- What Is Space Complexity And Its Significance?
- Relation Between Time And Space Complexity
- Conclusion
- What Is Linear Data Structure?
- Key Characteristics Of Linear Data Structures
- What Are The Types Of Linear Data Structures?
- What Is An Array Linear Data Structure?
- What Are Linked Lists Linear Data Structure?
- What Is A Stack Linear Data Structure?
- What Is A Queue Linear Data Structure?
- Most Common Operations Performed in Linear Data Structures
- Advantages Of Linear Data Structures
- What Is Nonlinear Data Structure?
- Difference Between Linear & Non-Linear Data Structures
- Who Uses Linear Data Structures?
- Conclusion
- Frequently Asked Questions
- What is a linear data structure?
- What is a non-linear data structure?
- Difference between linear data structure and non-linear data structure
- FAQs about linear and non-linear data structures
- What Is Search?
- What Is Linear Search In Data Structure?
- What Is Linear Search Algorithm?
- Working Of Linear Search Algorithm
- Complexity Of Linear Search Algorithm In Data Structures
- Implementations Of Linear Search Algorithm In Different Programming Languages
- Real-World Applications Of Linear Search In Data Structure
- Advantages & Disadvantages Of Linear Search
- Best Practices For Using Linear Search Algorithm
- Conclusion
- Frequently Asked Questions
- What Is The Binary Search Algorithm?
- Conditions For Using Binary Search
- Steps For Implementing Binary Search
- Iterative Method For Binary Search With Implementation Examples
- Recursive Method For Binary Search
- Complexity Analysis Of Binary Search Algorithm
- Iterative Vs. Recursive Implementation Of Binary Search
- Advantages & Disadvantages Of Binary Search
- Practical Applications & Real-World Examples Of Binary Search
- Conclusion
- Frequently Asked Questions
- Understanding The Jump Search Algorithm
- How Jump Search Works?
- Code Implementation Of Jump Search Algorithm
- Time And Space Complexity Analysis
- Advantages Of Jump Search
- Disadvantages Of Jump Search
- Applications Of Jump Search
- Conclusion
- Frequently Asked Questions
- What Is Sorting In Data Structures?
- What Is Bubble Sort?
- What Is Selection Sort?
- What Is Insertion Sort?
- What Is Merge Sort?
- What Is Quick Sort?
- What Is Heap Sort?
- What Is Radix Sort?
- What Is Bucket Sort?
- What Is Counting Sort?
- What Is Shell Sort?
- Choosing The Right Sorting Algorithm
- Applications Of Sorting
- Conclusion
- Frequently Asked Questions
- Understanding Bubble Sort Algorithm
- Bubble Sort Algorithm
- Implementation Of Bubble Sort In C++
- Time And Space Complexity Analysis Of Bubble Sort Algorithm
- Advantages Of Bubble Sort Algorithm
- Disadvantages Of Bubble Sort Algorithm
- Applications of Bubble Sort Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding The Merge Sort Algorithm
- Algorithm For Merge Sort
- Implementation Of Merge Sort In C++
- Time And Space Complexity Analysis Of Merge Sort
- Advantages And Disadvantages Of Merge Sort
- Applications Of Merge Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Selection Sort Algorithm
- Algorithmic Approach To Selection Sort
- Implementation Of Selection Sort Algorithm
- Complexity Analysis Of Selection Sort
- Comparison Of Selection Sort With Other Sorting Algorithms
- Advantages And Disadvantages Of Selection Sort
- Application Of Selection Sort
- Conclusion
- Frequently Asked Questions
- What Is Insertion Sort Algorithm?
- How Does Insertion Sort Work? (Step-by-Step Explanation)
- Implementation of Insertion Sort in C++
- Time And Space Complexity Of Insertion Sort
- Applications Of Insertion Sort Algorithm
- Comparison With Other Sorting Algorithms
- Conclusion
- Frequently Asked Questions
- Understanding Quick Sort Algorithm
- Step-By-Step Working Of Quick Sort
- Implementation Of Quick Sort Algorithm
- Time Complexity Analysis Of Quick Sort
- Advantages Of Quick Sort Algorithm
- Disadvantages Of Quick Sort Algorithm
- Applications Of Quick Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding The Heap Data Structure
- Working Of Heap Sort Algorithm
- Implementation Of Heap Sort Algorithm
- Advantages Of Heap Sort
- Disadvantages Of Heap Sort
- Real-World Applications Of Heap Sort
- Conclusion
- Frequently Asked Questions
- Understanding The Counting Sort Algorithm
- Conditions For Using Counting Sort Algorithm
- How Counting Sort Algorithm Works?
- Implementation Of Counting Sort Algorithm
- Time And Space Complexity Analysis Of Counting Sort
- Comparison Of Counting Sort With Other Sorting Algorithms
- Advantages Of Counting Sort Algorithm
- Disadvantages Of Counting Sort Algorithm
- Applications Of Counting Sort Algorithm
- Conclusion
- Frequently Asked Questions
- Understanding Shell Sort Algorithm
- Working Of Shell Sort Algorithm
- Implementation Of Shell Sort Algorithm
- Time Complexity Analysis Of Shell Sort Algorithm
- Advantages Of Shell Sort Algorithm
- Disadvantages Of Shell Sort Algorithm
- Applications Of Shell Sort Algorithm
- Conclusion
- Frequently Asked Questions
- What Is Linked List In Data Structures?
- Types Of Linked Lists In Data Structures
- Linked List Operations In Data Structures
- Advantages And Disadvantages Of Linked Lists In Data Structures
- Comparison Of Linked Lists And Arrays
- Applications Of Linked Lists
- Conclusion
- Frequently Asked Questions
- What Is A Singly Linked List In Data Structure?
- Insertion Operations On Singly Linked Lists
- Deletion Operation On Singly Linked List
- Searching For Elements In Single Linked List
- Calculating Length Of Single Linked List
- Practical Applications Of Singly Linked Lists In Data Structure
- Common Problems With Singly Linked Lists
- Conclusion
- Frequently Asked Questions (FAQ)
- What Is A Linked List?
- Reverse A Linked List
- How To Reverse A Linked List? (Approaches)
- Recursive Approach To Reverse A Linked List
- Iterative Approach To Reverse A Linked List
- Using Stack To Reverse A Linked List
- Complexity Analysis Of Different Approaches To Reverse A Linked List
- Conclusion
- Frequently Asked Questions
- What Is A Stack In Data Structure?
- Understanding Stack Operations
- Stack Implementation In Data Structures
- Stack Implementation Using Arrays
- Stack Implementation Using Linked Lists
- Comparison: Array vs. Linked List Implementation
- Applications Of Stack In Data Structures
- Advantages And Disadvantages Of Stack Data Structure
- Conclusion
- Frequently Asked Questions
- What Is A Graph Data Structure?
- Importance Of Graph Data Structures
- Types Of Graphs In Data Structure
- Types Of Graph Algorithms
- Application Of Graphs In Data Structures
- Challenges And Complexities In Graphs
- Conclusion
- Frequently Asked Questions
- What Is Tree Data Structure?
- Terminologies Of Tree Data Structure:
- Different Types Of Tree Data Structures
- Basic Operations On Tree Data Structure
- Applications Of Tree Data Structures
- Comparison Of Trees, Graphs, And Linear Data Structures
- Advantages Of Tree Data Structure
- Disadvantages Of Tree Data Structure
- Conclusion
- Frequently Asked Questions
- What Is Dynamic Programming?
- Real-Life Example: The Jigsaw Puzzle Analogy
- How To Solve A Problem Using Dynamic Programming?
- Dynamic Programming Algorithm Techniques
- Advantages Of Dynamic Programming
- Disadvantages Of Dynamic Programming
- Applications Of Dynamic Programming
- Conclusion
- Frequently Asked Questions
- Understanding The Sliding Window Algorithm
- How Does The Sliding Window Algorithm Works?
- How To Identify Sliding Window Problems?
- Fixed-Size Sliding Window Example: Maximum Sum Subarray Of Size k
- Variable-Size Sliding Window Example: Smallest Subarray With A Given Sum
- Advantages Of Sliding Window Technique
- Disadvantages Of Sliding Window Technique
- Conclusion
- Frequently Asked Questions
- Introduction To Data Structures
- Data Structures Interview Questions: Basics
- Data Structures Interview Questions: Intermediate
- Data Structures Interview Questions: Advanced
- Conclusion
What Is Data Structure? Definition, Types, Applications & More
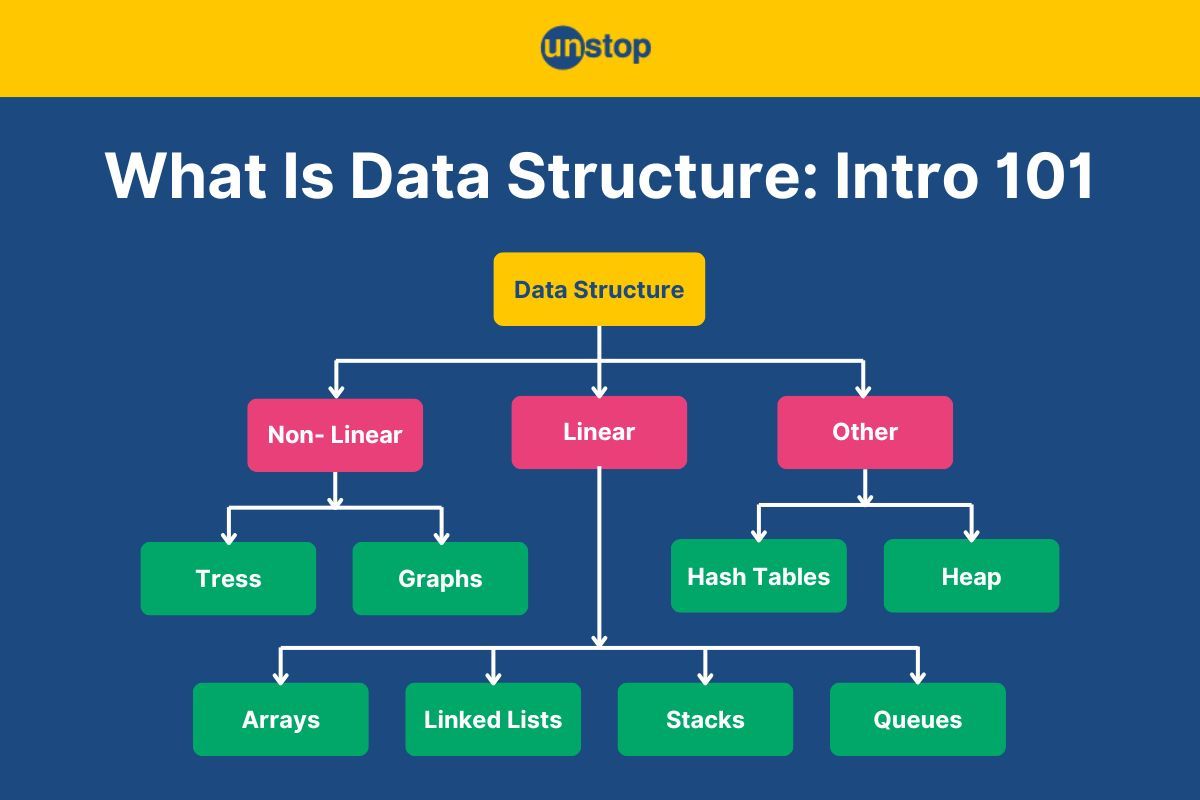
You must agree that in the world of programming and computation, data is king. However, it will go to waste if you don't know how to organize and manage that data. But how? Well, this is where data structures come into play. Data structures are fundamental to organizing, managing, and manipulating data. And that too efficiently, irrespective of whether you're developing software, analyzing data, or optimizing systems.
In this article, we will discuss what is data structure, its types, various operations we can perform on (and with) them, their applications and more.
What Is Data Structure?
A data structure is a specialized format for organizing, processing, storing, and retrieving data. It defines the relationship between data elements and the operations that can be performed on them (for different programming languages).
Think of it this way: Imagine a library with books as data elements and the library shelves as the data structures. It is easier to browse the vast number of books when they are organized/ categorised into different sections (fiction, non-fiction, etc.). Similarly, data structures provide a systematic way to store and access data based on its type and intended use.
- Data structures are fundamental to computer science and programming because they provide a means to manage large amounts of data efficiently.
- In simple terms, data structure definition is- it is a collection of data values and the relation between them, along with the functions or operations that can be applied to the data.
They play a critical role in various applications, from databases and operating systems to artificial intelligence and machine learning. However, you must choose the appropriate data structure based on the programming needs and programming languages to write efficient and optimized code.
Key Features Of Data Structures
Here are the key features of data structures:
-
Data Storage: Data structures provide a way to store data in an organized manner, making it easier to manage and access the data efficiently.
-
Data Organization: They organize data in a structured format, such as linear (arrays, linked lists) or non-linear (trees, graphs), facilitating easier data manipulation.
-
Data Processing: They enable efficient data manipulation, including operations like insertion, deletion, and traversal, which are optimized for performance based on the data structure used.
- Memory Management: They manage memory usage effectively, with some data structures like linked lists and trees dynamically allocating memory as needed.
-
Homogeneous Data: Many data structures store homogeneous data elements, meaning all elements are of the same type, ensuring consistency and predictability.
-
Indexing: Certain data structures, such as arrays, allow for efficient indexing, providing direct access to elements based on their index positions.
-
Sequential Access: Linear data structures like arrays and linked lists allow for sequential access to elements, facilitating ordered data processing.
-
Dynamic Size: Some data structures, like linked lists and dynamic arrays, can adjust their size dynamically to accommodate varying amounts of data.
-
Hierarchy Representation: Non-linear data structures, such as trees and graphs, represent hierarchical relationships between elements, useful for modeling complex systems.
-
Support for Multiple Operations: Data structures support a variety of operations, including searching, sorting, merging, and splitting, making them versatile tools for data manipulation.
-
Ease of Implementation: Many data structures are supported by standard libraries in programming languages, providing easy-to-use implementations for common data structures.
Basic Terminologies Related To Data Structures
Understanding data structures involves familiarity with several key terms and concepts. Here's a list of basic terminologies commonly used in the context of data structures:
Term | Explanation |
Element | An individual item of data within a data structure. |
Node | A basic unit of a data structure, such as linked lists and trees, contains data and pointers. |
Pointer | A variable that holds the memory address of another variable and is often used in linked structures. |
Index | The position of an element within an array, typically starting from 0. |
Hash Table | A data structure that maps keys to values for efficient data retrieval. |
Traversal | The process of visiting all the nodes or elements in a data structure. |
Insertion | Adding a new element to a data structure. |
Deletion | Removing an element from a data structure. |
Searching | Finding the location of an element within a data structure. |
Sorting | Arranging the elements of a data structure in a specific order. |
Dynamic Allocation | Allocating memory at runtime, often used in structures like linked lists. |
Static Allocation | Allocating memory at compile-time, often used in arrays. |
Root Node | The topmost node in a tree structure. |
Child Node | A node that descends from another node in a tree structure. |
Parent Node | A node that has one or more child nodes in a tree structure. |
Sibling Nodes | Nodes that share the same parent in a tree structure. |
Leaf Node | A node with no children in a tree structure. |
Edge | A connection between two nodes in a graph. |
Vertex | A node in a graph. |
Degree | The number of edges connected to a vertex in a graph. |
Adjacency List | A collection of lists used to represent a graph, where each list corresponds to a vertex and contains a list of adjacent vertices. |
Adjacency Matrix | A 2D array used to represent a graph, where each element indicates whether there is an edge between a pair of vertices. |
Self-Referential Structure | A data structure that includes pointers to instances of the same data structure, such as a node in a linked list pointing to the next node. |
Types Of Data Structures
Data structures can be categorized into several types based on organization, storage, and access methods. The four most commonly used types of data structures are:
1. Linear Data Structures
Linear data structures organize data elements sequentially, where each element is connected to its previous and next adjacent elements.
In other words, the data is represented in a sequential or linear order, where elements are arranged one after another, and access typically happens in a specific order (e.g., by index). Its subtypes include- Arrays, Linked Lists, Stacks, and Queues.
2. Non-Linear Data Structures
Non-linear data structures do not follow a sequential order, allowing for more complex relationships between data elements. They are crucial for representing hierarchical or interconnected data. Access can be based on keys, positions, or relationships. Its subtypes/ examples include graphs and tree data structures.
3. Hashed Data Structures
Hashed data structures use hash functions to compute an index (hash code) into an array of buckets or slots, where data elements are stored. This indexing allows for fast access and retrieval operations. For example:
- Hash Tables: A data structure that stores key-value pairs, with keys mapped to unique indices using a hash function.
- Hash Maps: Implementation of associative arrays that map keys to values.
- Hash Sets: Collection of unique elements where each element is hashed to a unique bucket.
4. Heap Data Structures
Heap data structures are specialized binary trees that satisfy the heap property, ensuring the highest (or lowest) priority element is always at the root. They are primarily used to implement priority queues. For example:
- Binary Heaps: Complete binary tree where every parent node has a value less/greater than or equal to its child nodes.
- Priority Queues: Abstract data type where elements are retrieved in order of priority.
Hashed and heaped types are specialized data structures that don't neatly fit into linear or non-linear classifications. They often serve specific purposes and optimize for particular access patterns.
Static Vs. Dynamic Data Structures
A lot of people also classify data structures as static and dynamic. However, it is important to note that this classification isn't strictly a data structure type but rather a property.
- Some data structures, like arrays, have a fixed size at allocation and are hence known as static data structures.
- Others, like linked lists, are dynamic and can grow or shrink as needed and are hence known as dynamic data structures.
- So, static and dynamic data structures differ in managing memory allocation and must be chosen for efficient storage given the requirements.
-
Static Data Structures: Have fixed sizes allocated at compile-time, and their size cannot change during runtime. Examples include arrays and static linked lists.
-
Dynamic Data Structures: Allocate memory as needed during runtime, allowing them to grow or shrink dynamically. Examples include dynamic arrays (ArrayLists in Java), dynamic linked lists, and trees that dynamically adjust their size based on insertions and deletions.
What Are Linear Data Structures & Its Types?
Linear data structures represent data in sequential or linear order, similar to how items are arranged in a line. Elements are connected logically, and access typically happens in a specific order, often by index or position. This organization makes them efficient for certain operations, like iterating through all elements or accessing elements at specific positions.
What Are Types Of Linear Data Structures?
There are several important types of linear data structures, each with its own strengths and weaknesses:
-
Arrays: A collection of elements of the same data type stored at contiguous memory locations. Access is fast using an index (position) within the array. Useful for storing fixed-size collections where random access is needed. There are three primary types of arrays: one-dimensional arrays, two-dimensional arrays and multi-dimensional arrays.
- Linked List Data Structure: A linear data structure where elements (nodes) are not stored contiguously in memory. However, each node contains data and a reference (link) to the next/ subsequent node in the sequence. This allows for dynamic resizing (growing or shrinking the list) and efficient insertions/deletions from specific points within the list.
-
Stack Data Structure: It is a linear data structure that follows the LIFO (Last In, First Out) principle, i.e., elements are added (pushed) and removed (popped) from the top of the stack. Think of a stack of plates - you can only add or remove plates from the top.
Useful for function calls (keeping track of nested function calls), implementing undo/redo functionality, and expression evaluation (processing operators in the correct order). -
Queue Data Structures: This linear data structure follows the FIFO (First In, First Out) principle, i.e., elements are added (enqueued) at the back and removed (dequeued) from the front. Imagine a queue at a bus stop - the first person in line gets served first. Useful for scheduling tasks (processing tasks in the order they are received), managing waiting lists, and processing data in the order it arrives.
For more, read: What Is Linear Data Structure? Types, Uses & More (+ Examples)
What Are Non-Linear Data Structures & Its Types?
A non-linear data structure, as the name suggests, does not follow the sequential format of storing data. Instead, elements can be linked in hierarchical and more complex ways, making them more complex data structures in comparison to their linear counterparts.
They provide more flexibility by allowing for data representation with inherent non-linearity, like networks, family trees, or geographical maps. However, accessing elements in non-linear data structures often involves following these relationships or using keys to locate specific elements.
What Are The Types Of Non-Linear Data Structures?
Here are some key types of non-linear data structures:
-
Tree Data Structures: They are hierarchical structures with a root node, child nodes, central node, structural nodes, and potentially sub-tress.
- The nodes can have a parent-child relationship and store data.
- Its types include Binary Trees, Binary Search Trees, AVL Trees, and B-trees.
- For example, a binary tree is where each parent/ head node has almost two children. The number of children per node can lead to other classifications of the tree data structure.
- They are useful for representing hierarchical relationships (like organizational structures), implementing sorting algorithms efficiently, and performing search operations.
-
Graph Data Structures: These data structures are also a collection of nodes (vertices) and edges that connect pairs of nodes. In other words, the nodes (vertices) are connected by edges, which is why they are also referred to as connected graphs.
- Edges can be directed (one-way connection) or undirected (two-way connection) and may have weights associated with them (representing distances or costs).
- Its types include Directed and Undirected Graphs, Weighted Graphs, and Directed Acyclic Graphs (DAGs).
- These data structure types are useful in representing networks (social networks, computer networks, circuit networks, telephone networks, etc.), modelling transportation systems, and solving problems involving finding paths between nodes.
-
Hash Tables: The data structure types use a hash function to map unique keys (identifiers) to their corresponding values. This allows for fast average-case access time for retrieving data based on the key. Useful for implementing dictionaries, symbol tables (storing variable names and values), and key-value pair collections where fast lookups are crucial.
-
Heaps: They are specialized tree-based structures where the value of a node is either greater than (max-heap) or less than (min-heap) the values of its children. Useful for implementing priority queues (processing elements based on priority) and efficient heap sort algorithms.
Remember, choosing the right non-linear data structure depends on the specific relationships between your data elements and the operations you need to perform.
Importance Of Data Structure In Programming
Data structures are the backbone of efficient and well-organized programs. They are not merely theoretical concepts but practical tools that significantly impact how you write and execute code. Here's why data structures are crucial for programmers:
-
Efficient Data Management: Data structures allow for efficient data storage, retrieval, and manipulation. They provide ways to organize data such that it optimizes various operations, such as insertion, deletion, searching, and updating. This translates to quicker execution times and a smoother user experience.
-
Enhanced Performance: The choice of an appropriate data structure can significantly affect the performance of a program. For example, using a hash table for fast data lookup, a binary search tree for sorted data access, or a queue for managing tasks in a specific order can lead to more efficient and faster programs.
-
Memory Utilization: Data structures help in optimal memory utilization. By using the right data structure, you can minimize memory wastage and manage memory allocation efficiently, ensuring that the program runs smoothly without unnecessary consumption of resources.
-
Simplification of Complex Problems: Data structures break down complex problems into manageable parts. They provide a way to model real-world problems in a structured manner, making it easier to implement and understand algorithms that operate on these structures.
-
Reusability and Scalability: Well-designed data structures can be reused across different programs and applications. This saves development time and promotes consistency in your codebase. They also provide a scalable way to handle increasing amounts of data, ensuring that the program remains efficient and manageable as the data grows.
- Data Integrity and Security: Proper data structures help maintain data integrity and security. Structures like linked lists and trees ensure that data is linked logically, preventing accidental corruption or loss. Additionally, structures like cryptographic hash tables enhance data security.
-
Improved Problem-Solving Skills: Understanding and implementing data structures enhance a programmer's problem-solving skills. It provides a deeper understanding of how data is organized and manipulated, leading to better algorithm design and more effective coding practices.
-
Foundation for Advanced Topics: Data structures form the foundation for many advanced computer science topics, such as databases, operating systems, networking, and artificial intelligence. A solid grasp of data structures is essential for understanding and excelling in these areas.
In summary, data structures are indispensable tools in programming. They provide the backbone for efficient data management, improve program performance, and enable the implementation of complex algorithms.
Basic Operations On Data Structures
Data structures are not just passive containers for data; otherwise, they wouldn't be so important in the world of programming and computation. Their importance comes from the fact that we can perform various common operations on the data they hold. These can vary from basic arithmetic operations to complex operations.
Listed below are some primary operations that can be performed on data structures to maintain and manipulate the data:
-
Insertion: This operation refers to adding a new element to the data structure. For example, you can add a new item to the list of elements you want to buy on a shopping cart (linked list).
-
Deletion Operation: This entails removing an existing element from the data structure. For example, you can remove an item you no longer need from the shopping cart (linked list).
-
Traversal: This refers to traversing/ browsing through or visiting and accessing all elements in the data structure, often in a specific order. For example, when you print a list of the grocery items you want to buy or iterate through all nodes in a linked list or tree in a pre-order, in-order, or post-order fashion.
-
Access: The process of retrieving the value of an element based on its position (index) or key is referred to as access. For example, accessing a specific item's price in a shopping list (array) based on its index (say, the middle element).
- Search: The operation refers to searching for and finding a specific element based on a search criterion (e.g., value, key). Like accessing the elements and browsing for something specific. For example, you might search the inventory (array or a hash table) of an online store for a specific product by its name.
-
Sorting: This process entails arranging the elements of a data structure in a specific order (ascending, descending, based on custom criteria). For example, sorting a list of student names alphabetically (array or linked list) or sorting the products in an online store on the basis of their prices from lowest to highest.
-
Merging: It refers to combining two or more sorted data structures into a single sorted data structure. For example, combining two different shopping lists to make one (linked list or array).
-
Reversing: This process entails reversing the order of elements in the data structure. For example, re-arranging or reversing a list of student names that has been sorted from A to Z.
These are just some core operations; specific implementations can vary depending on the data structure. It is important to understand these common operations to pick the right one for your specific needs.
Applications Of Data Structures
Data structures have wide-ranging applications across various domains and are essential for developing efficient algorithms. Here are some key applications of data structures:
-
Database Management Systems (DBMS): Data structures like B-trees and hash tables are crucial for indexing and organizing database data. They optimize data retrieval and ensure efficient storage.
-
Compiler Design: Compilers use data structures such as symbol tables and abstract syntax trees (ASTs) to analyze and manage source code during compilation. These structures aid in parsing, semantic analysis, and code optimization.
-
Operating Systems: Operating systems utilize data structures like queues, stacks, and scheduling algorithms to manage system resources, process scheduling, and memory allocation efficiently.
-
Networking: Data structures such as graphs and adjacency matrices are used to model and analyze network topologies, routing algorithms, and connectivity in computer networks.
-
Artificial Intelligence and Machine Learning: In AI and ML applications, data structures like decision trees, graphs, and hash tables are used for efficient data representation, searching, and processing large datasets.
-
Web Development: Data structures like arrays, linked lists, and hash maps are essential for managing dynamic content, handling sessions, caching, and optimizing web applications for performance.
- Spatial and Geographic Information Systems (GIS): GIS applications use data structures like spatial indexes and grids to efficiently store and query spatial data, manage geographical information, and perform spatial analysis.
-
Cryptocurrency and Blockchain: Blockchain technology relies on data structures such as Merkle trees for secure and efficient transaction verification and validation across distributed networks.
-
Bioinformatics: Data structures like sequence alignment algorithms, suffix trees, and graphs are used to analyze biological data, DNA sequences, and protein structures in bioinformatics research.
Real-Life Applications Of Data Structures
You might think that data structures are not related to your day-to-day lives. Think again! Data structures are, in fact, invisible workhorses behind many of the programs and applications we use daily. Here are some real-world examples:
-
Social Media Feeds: When you scroll through your social media feed, the posts are likely organized using a combination of data structures. For example, heaps or priority queues might be used to prioritize posts based on factors like engagement or relevance.
-
Online Shopping: Adding items to your shopping cart, searching for products by category or price, and filtering search results rely on data structures. For example, arrays or hash tables might efficiently store product information.
-
Navigation Apps: Finding the quickest route on a map involves traversing a graph data structure. Nodes represent locations, and edges represent the roads or connections between them.
-
Music Streaming Services: When you create a playlist or shuffle your music library, data structures (besides the music) are at play. That is, linked lists might be used to represent the order of songs in a playlist.
-
Computer Games: Efficiently rendering complex game worlds, managing character inventories, and handling user input also involve data structures. For example, trees or graphs could represent the game world's layout and connections between different areas.
-
Web Browsers: Keeping track of browsing history, managing open tabs, and implementing back and forward buttons rely on data structures. The stack data structure is the perfect way to fulfil these functionalities with its LIFO (Last In, First Out) principle.
These are just a few examples, and the applications of data structures are vast and diverse.
Linear Vs. Non-linear Data Structures
Understanding these core differences between linear and non-linear data structures is essential to make informed decisions about which data structure best suits your programming needs. Here are some key differences between the two-
Basis | Linear Data Structure | Non-Linear Data Structure |
Organization | Elements are organized sequentially or linearly, where each element has a predecessor and a successor, except for the first and last elements. | Elements are not organized sequentially; instead, each element can connect to multiple other elements more complexly, such as trees and graphs. |
Traversal | Traversal is straightforward and typically involves iterating from one end to another (e.g., arrays, linked lists). | Traversal can be more complex, often requiring algorithms like depth-first search (DFS) or breadth-first search (BFS) to explore nodes (e.g., trees, graphs). |
Memory Allocation | Memory allocation is usually contiguous, and elements are stored in a continuous block of memory. | Memory allocation can be more scattered or hierarchical, depending on the structure (e.g., trees may use pointers for node connections). |
Example | Arrays, Linked Lists, Stacks, Queues | Tress, Graphs, Hash Tables |
What Are Algorithms? The Difference Between Data Structures & Algorithms
An algorithm is a well-defined set of instructions (step-by-step procedure) on how to take an input, process it and perform a specific task or solve a particular problem.
- They can be simple, like sorting a list of numbers, or complex, like finding the shortest path in a network.
- Algorithms are evaluated based on their efficiency and performance, typically considering factors such as time complexity (how fast an algorithm runs) and space complexity (how much memory an algorithm uses).
- These evaluations help determine the best algorithm to use for a given problem.
Imagine trying to follow a complex recipe - that's essentially what an algorithm is like for computers.
- Think of data structures as the ingredients and containers used in a recipe (the data and how it's organized).
- Algorithms are the actual instructions (the recipe itself) that tell you how to combine and process those ingredients to achieve a desired outcome (the result of the recipe).
The Difference Between Data Structures & Algorithms
The table below highlights the primary differences between the two concepts:
Basis/Parameter | Data Structures | Algorithms |
---|---|---|
Purpose | Organize and store data as per specific formats | Provide step-by-step instructions for solving problems/ performing tasks |
Focus | Data organization and storage | Data processing and manipulation |
Examples | Include arrays, linked lists, stacks, queues, trees, graphs | Include sorting, searching, optimization, and graph algorithms |
Efficiency Measurement | Evaluated based on memory usage and access time | Evaluated based on time complexity and space complexity |
Interrelation | Provide the foundation for algorithm implementation | Operate on data structures to perform tasks |
Understanding both data structures and algorithms is essential for effective problem-solving and optimization in computer science. Data structures provide the necessary means to store and organize data, while algorithms offer the methods to process this data efficiently. Together, they form the backbone of efficient software design and implementation.
Conclusion
In this article, we explored the basics of data structures, including their key features, various types, and basic operations. Data structures are the cornerstone of efficient and well-organized programs. They provide us with different formats for storing and organizing data. We can then perform various operations like insertion, deletion, access, reveal, traversal, etc., on the data stored in them.
They provide the framework for organizing and managing data efficiently, essential for implementing robust algorithms and solving complex problems. All in all, they are powerful tools that influence how you write and execute code.
Frequently Asked Questions
Q. What is the difference between structured and unstructured data?
Structured data is when information is organized in a predefined format, often following a schema or table-like structure. This makes it easily searchable and processable by computers. Examples include data in spreadsheets or databases, where each record has specific fields with defined data types (numbers, text, etc.).
Unstructured data, on the other hand, is when information organization does not follow a pre-defined format. It can be text documents, images, audio, video, social media posts, sensor readings, etc. While this data can be valuable, it requires additional processing and advanced techniques (like natural language processing or machine learning) to extract or analyse meaning effectively.
Q. How does data structure vary from data types?
Data types define the specific kind of data that can be stored in variables or even data structures. Basic data types include integers, floats, characters, and booleans.
Data structures are ways/ formats of organizing and storing data to enable efficient access and modification. Examples include arrays, linked lists, stacks, and trees.
- Data structures determine the relationships among data elements and the operations that can be performed on them.
- Data types are the building blocks used to define variables, while data structures are the constructs that manage collections of these variables.
- Data types define the properties of individual elements, while data structures define how those elements are organized and interrelated within a program.
Think of data types as the bricks and data structures as the architectural styles used to build with those bricks.
Q. Explain the concept of recursion in data structures.
Recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. The recursive function call is built inside the function definition itself.
- It can be a powerful tool for working with certain data structures, particularly those with hierarchical relationships.
- In data structures, recursion is commonly used with structures like trees and linked lists, where problems can naturally be divided into similar subproblems.
For example, in a binary tree, a recursive function can traverse the tree, process nodes, or search for a value. Recursion simplifies code by reducing complex problems into manageable subproblems. However, recursion requires careful design to avoid infinite loops, ensure efficient execution, and avoid issues like infinite recursion or excessive memory usage.
Q. How do data structures help improve program performance?
Data structures improve program performance by organizing data in a way that optimizes access and modification operations. However, you must choose the right data structure to enhance the efficiency of algorithms. For example:
- Faster access and retrieval: Certain data structures like arrays offer quick random access by index, while balanced trees enable efficient searching through sorted data.
- Optimized insertions and deletions: Linked lists excel at adding or removing elements from specific points within the structure, while hash tables provide fast insertion and retrieval based on keys.
- Reduced memory usage: Choosing a data structure that aligns with your data access patterns can minimize wasted memory allocation and improve overall program efficiency.
Efficient data structures reduce the time complexity of operations, minimize memory usage, and ensure that programs run faster and handle larger datasets more effectively.
Q. Explain the relation between an abstract data type (ADT) and data structures.
An Abstract Data Type (ADT) is a blueprint or definition that specifies the data model and the set of operations (insertion, deletion, search, etc.) that can be performed on it. However, it does not define the underlying implementation details. In other words, it focuses on what operations are performed, not how they are executed, thus allowing for flexibility and code reusability.
Data structures, on the other hand, are concrete implementations of these ADTs. They provide the actual means of storing and managing data according to the specifications of the ADT.
- Multiple data structures can implement the same ADT, but they might use different methods to store and organize the data internally.
- For instance, both arrays and linked lists can implement the ADT of a linear list, but they differ in their memory allocation and access methods.
All in all, the relation between ADTs and data structures is that ADTs provide the conceptual framework and interface, while data structures offer the physical realization and efficiency of those concepts.
This concludes our discussion on what is data structure, and by now, you must be able to choose the right one for your programming needs. Here are a few other topics you must explore:
- Difference Between Hashing And Encryption Decoded
- 53 Frequently Asked Linked List Interview Questions With Answers 2024
- Data Structure Interview Questions For 2024 [With Detailed Answers]
- Tree Topology | Advantages & Disadvantages In Computer Network
- Decoding Data Redundancy In DBMS| Causes, Advantages, Solutions
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Abinaya Palani 1 week ago
Divyanshu Kumar 1 month ago
George Nwachukwu 1 month ago
Sithala Sai Vamshi 1 month ago
Gopi Lakke 1 month ago
Jahnavika Gopal 1 month ago