Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
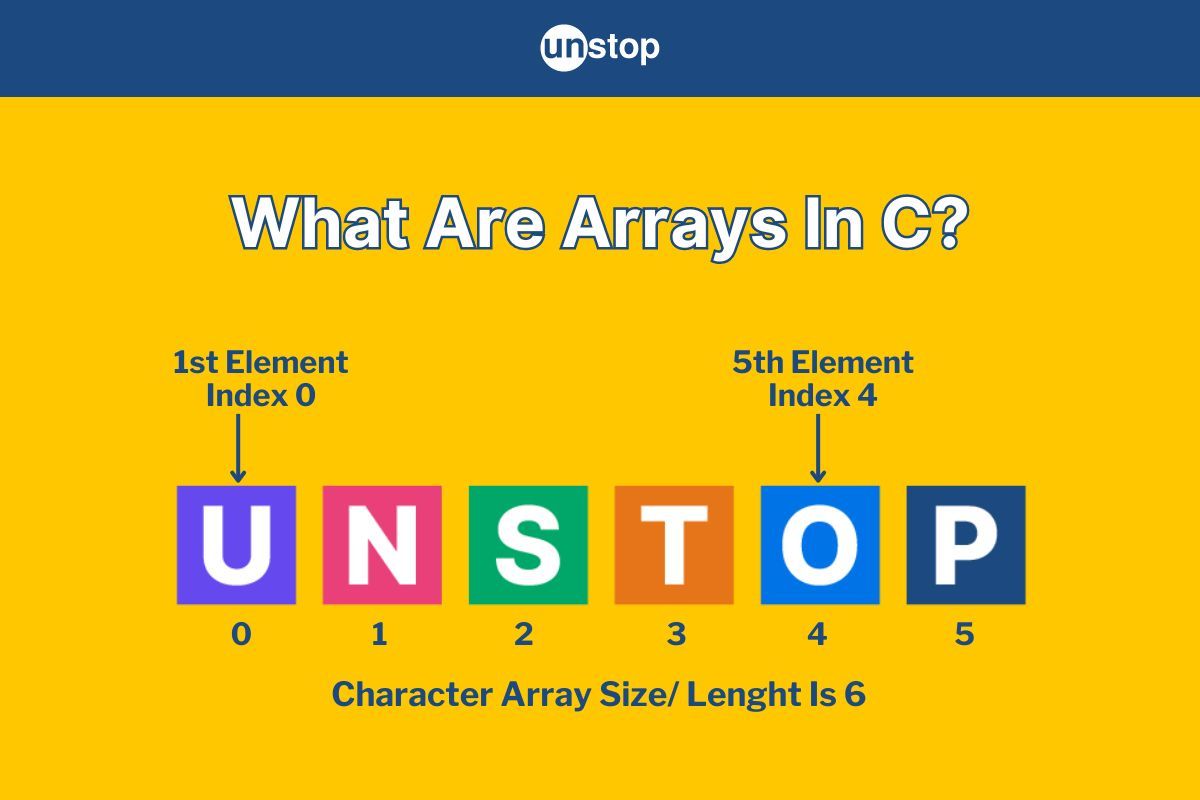
Arrays play a fundamental role in programming languages, providing a powerful and efficient way to store and manipulate data. An array, in its essence, is a collection of elements, each sharing the same data type, residing in contiguous memory locations. The power of arrays lies in their ability to group related pieces of data together, forming cohesive structures that facilitate efficient data management. Arrays in C programming are not just a feature but a fundamental construct that allows developers to navigate through the intricacies of handling diverse sets of information.
In this article, we will delve into the concept of arrays in C, explore their features, and demonstrate how to leverage them effectively in C programs.
Why Do We Need Arrays In C?
Arrays in C are essential data structures that allow you to store and manipulate a collection of elements of the same data type under a single identifier. Here are some reasons why arrays are crucial in C programming language:
- Grouping of Elements: Arrays provide a way to group elements of the same type together under a single name. This is useful for organizing and managing related data.
- Random Access: Elements in an array are stored in contiguous memory locations, allowing for constant-time access to any element using an index. This enables efficient random access to individual elements.
- Memory Efficiency: Arrays are memory-efficient, as they allocate a contiguous block of memory. This eliminates the need to declare separate variables for each element, making it more efficient in terms of memory usage.
- Iterative Processing: Arrays in C facilitate iterative processing through loops. You can easily iterate over the elements of an array using loops, making it convenient for tasks like calculations, comparisons, and modifications.
- Passing to Functions: Arrays can be easily passed to functions. When you pass an array to a function, you're essentially passing a reference to the array, allowing the function to operate on the original data.
- Sorting and Searching: Arrays in C provide a convenient structure for implementing sorting and searching algorithms. Sorting and searching operations can be efficiently performed on arrays due to their random access nature.
- Implementation of Data Structures: Many advanced data structures, such as stacks, queues, matrices, and strings, are implemented using arrays or structures involving arrays. Arrays provide a foundational building block for creating more complex data structures.
- Multi-dimensional Arrays: C supports multi-dimensional arrays, allowing you to create matrices and other multi-dimensional structures. This is particularly useful in applications like image processing, scientific simulations, and matrix manipulations.
How To Declare An Array In C?
Declaring an array in C involves specifying the data type of its elements and providing a name for the array. The syntax for declaring an array is as follows:
datatype arrayName[arraySize];
Here,
- The term datatype refers to the type of data elements stored in the array whose name is given by arrayName.
- The term arraySize refers to the number of elements that are stored inside the array.
For example, to declare an array of integers named numbers with a size of 5, the declaration would look like this:
int numbers[5];
Arrays in C offer a convenient way to store and manage collections of elements of the same data type, providing a foundation for various programming tasks and algorithms. Additionally, C allows for the declaration and initialization of arrays in a single line, providing initial values for the elements at the time of declaration. There are three different methods to declare an array in C.
Array Declaration By Specifying Size
This method involves specifying the name, type, and size of the array, where the size is given as a constant integer expression.
The size of the array is crucial because it determines how many elements the array can hold. We provide the size as a constant integer expression, which means it's a fixed number and doesn't change while the program is running. This helps us keep track of and work with multiple pieces of related information efficiently.
The syntax for declaring an array using this approach is as follows:
type array_name[size];
Here,
- type refers to the data type of elements contained in the array.
- array_name refers to the name of the array being created.
- size refers to the number of elements in the array.
Let's take a look at an example showcasing how we can use this method to declare arrays in C.
Code Example:
Output:
10
20
30
40
50
Explanation:
We start the simple C program above by including the <stdio.h> header file, which offers functions for input and output tasks.
- Inside the main() function, we declare an integer array named numbers with a capacity of 5. This signifies that the array can store five integer values.
- Next, we assign specific values to each element of the array using index notation.
- It's important to note that array indices begin from 0. Hence, numbers[0], i.e., the first element receives the value 10, numbers [1], element 3 holds 20, and so forth.
- We then use a series of printf() statements to display each element of the array on separate lines of code using the newline escape sequence.
- Finally, the program returns the value 0, indicating successful execution.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Array Declaration By Initializing Elements
This method involves specifying the name, type, and initial values of the array. The number of provided values automatically determines the size of the array here.
The syntax for declaring an array using this approach is:
type array_name[] = {value1, value2, ..., valueN};
Here,
- type: Represents the data type of the elements stored in the array.
- array_name: Refers to the distinct identifier for the new array.
- value1, value2, ..., valueN: These are the initial values that you want to assign to the array elements. The size of the array is automatically determined based on the number of provided values.
Code Example:
Output:
11 22 33 44 55
Explanation:
In the C code example above, we first include the header file, which provides input and output functions.
- Inside the main() function, we declare and initialize an integer array called numbers without specifying the size.
- But the values are assigned to the elements and the size of the array is automatically determined by the number of values provided.
- Next, we initialize an integer variable, i, to use as a control variable inside a loop.
- We then create a for loop to iterate through the elements of the array. The loop starts with i = 0 and continues as long as i is less than or equal to 4 (since array indices range from 0 to 4 in this case).
- During each iteration, the printf() function is used to display the value of the single element at the specified index. The output of the program displays the values of each array element.
- After the loop concludes, the program returns the value 0 to indicate successful execution without errors.
Array Declaration With Size Specification & Element Initialization
This method involves specifying the name, type, predefined size, and initial values of the elements of the array during declaration. Note that the size of the array must be equal to or greater than the number of values we provide.
The syntax for this approach is:
type array_name[size] = {value1, value2, ..., valueN};
Here,
- type: Represents the data type of the elements contained in the single array.
- array_name: Refers to the name that you want to assign to the array.
- size: Specifies the number of elements in the array. It must be equal to or greater than the number of values provided in the initialization.
- value1, value2, ..., valueN: These are the initial values you want to assign to the array elements. The number of values provided should match the specified size of the array.
Code Example:
Output:
10 20 30 40 50
Explanation:
We include the stdio.h header file to access input and output functions.
- In the main() function, we declare and initialize an integer array called numbers with the values {10, 20, 30, 40, 50}. The size of the array is explicitly specified as 5 inside the square braces.
- We then declare another integer variable, i, without initialization, for use as the control variable in the loop.
- Next, we create a for loop to iterate through the array elements. The loop starts with i = 0 and continues as long as i is less than or equal to 4 (matching the array's indices).
- At each iteration, the printf() function inside the loop displays the values of array elements at the current index. The function has a formatted string where the %d format specifier indicates that an integer value of the numbers array will be filled.
- After the loop completes, the program returns the value 0, indicating successful execution.
Initialization Of Arrays In C
Array initialization in C refers to the process of assigning initial values to the elements of an array. It allows you to set the initial state of the array, providing meaningful values that the array will hold during the execution of the program. Initialization can be done at compile time using known values or at runtime using user input or dynamically computed values. Additionally, array initialization using a loop provides a way to calculate or generate initial values of the initializer list based on specific conditions.
Compile-Time Array Initialization In C
Compile time array initialization refers to initializing the array using known values at the time of compilation. This method allows you to explicitly provide the initial values for the array elements, which are embedded directly into the executable code.
It is the same as the declaration method we discussed in the section just above. Here, we declare an array by specifying its name, size, and data type of elements and assign the values at the same time using the simple assignment operator.
Syntax:
datatype arrayName[size] = {value1, value2, ..., valueN};
Here,
- datatype: Indicates the data type of the elements within the array.
- arrayName: Represents the chosen name for the array.
- size: Specifies the number of elements in the array.
- value1, value2, ..., valueN: These are the specific values assigned to the array's elements. They are known at compile time and are directly included in the compiled code.
Runtime Array Initialization In C
Runtime array initialization refers to initializing the array during the execution of the program, allowing you to assign values to the array elements based on user input, file input, or dynamically computed values.
Syntax:
datatype arrayName[size];
// Assigning values at runtime
arrayName[index] = value;
Here,
- datatype: Specifies the data type of the elements within the array.
- arrayName: Denotes the chosen name for the array.
- size: Indicates the number of elements in the array.
- index: Represents the position within the array where you want to assign a value.
- value: The specific value you want to assign to the array element at the given index. This assignment takes place during runtime, allowing for dynamic or user-defined inputs.
Code Example:
Output:
Type the value for the element 0: 1
Type the value for the element 1: 2
Type the value for the element 2: 3
Type the value for the element 3: 4
Type the value for the element 4: 5
1 2 3 4 5
Explanation:
In the sample C code, we first include the standard input-output library (<stdio.h>) for utilizing input and output functions in C.
- Inside the main() function, we declare an integer array named numbers of size 5. This array will store values input by the user at runtime.
- As mentioned in the code comments, we use a for loop to iterate through the array elements. Inside the loop-
- We prompt the user to input values for each element using the printf() function.
- After the user inputs a value, we use the scanf() function to read it and then store it in the array using the reference operator (i.e., &numbers[i]).
- This process continues till we have all the elements of the array.
- Then, we use another for loop to access and print the values of array elements. The loop iterates from the first element to the last, and each element is printed using printf.
- The main function concludes with a return 0 statement, indicating successful execution.
Array Initialization Using A Loop
Array initialization in C using a loop allows you to calculate or generate initial values for the array elements based on specific conditions or computations. It provides a way to set the initial state of the array dynamically. The loop begins with an initial value and then iteratively adds subsequent elements to the array.
Syntax:
datatype arrayName[size];
int i;
for (i = 0; i < size; i++){
arrayName[i] = computation or condition;}
Here,
- datatype: Specifies the data type of the elements within the array.
- arrayName: Represents the designated name for the array.
- size: Denotes the total number of elements in the array.
- i: Serves as an iteration variable or control variable for the loop, responsible for traversing the array indices.
- computation or condition: Refers to the calculation or condition that determines the value to be assigned to each array element. This computation or condition can be based on specific logic or calculations, offering dynamic initialization.
Code Example:
Output:
10 20 30 40 50
Explanation:
In this C code example, we create an integer array called numbers with a size of five. However, the array is not initialized with any specific values initially.
- We then use a for loop to iterate over the indices of the array, starting from 0 and ending at 4.
- At each iteration, the values of elements of the array are calculated based on an expression.
- This expression adds 1 to the value of the control variable (i) and then multiplies the result by 10, using arithmetic operators, i.e., (i + 1) * 10.
- The value so calculated is assigned to each element of the array, given the index number.
- After that, we create another for loop to access and print each element of the numbers array to the console.
- The loop iterates through the array, and each element is printed with a space in between, as given in the formatted string.
- The main function concludes with a return 0 statement, indicating successful execution.
In summary, this code initializes an integer array with a size of five, assigns computed values to its elements using a for loop, and then prints the array elements to the console using another for loop.
Properties Of Arrays In C
Arrays are a fundamental data structure in programming that allows us to store multiple values of the same data type in a contiguous block of memory. Some of the key properties and characteristics of arrays in C are:
- Fixed Size: Arrays in C have a fixed size, meaning that once you declare an array with a specific size, you cannot change its size during runtime. The size of an array is determined at compile time based on the number of elements you specify.
Example:
int numbers[5]; // An array of integers with size 5 - Contiguous Memory Allocation: Array elements are stored in contiguous memory locations. This means that each element occupies a fixed amount of memory, and the elements are stored one after another in memory.
Example:
Address Element
---------------------------
1000 10
1004 20
1008 30.... and so on. - Indexed Access: Array elements are accessed using an index, which represents the position of the element in the array. The index of an array begins at 0 and increases by 1 for each additional element.
Example:
int numbers[5] = {15, 20, 25, 30,35};
int firstElement = numbers[1]; // Accessing the second element (20)
int thirdElement = numbers[4]; // Accessing the last element (35) - Homogeneous Data Type: Arrays in C store elements of the same data type. This ensures that each element in the array occupies the same amount of memory and can be accessed and manipulated uniformly.
Example:
int numbers[3] = {10, 20, 30}; // Array of integers
char characters[4] = {'a', 'b', 'c', 'd'}; // Array of characters - Zero-based Indexing: In most programming languages, including C, arrays use zero-based indexing. This means that the index of the first element is 0, the index of the second element is 1, and so on.
Example:
int numbers[3] = {10, 20, 30};
int firstElement = numbers[0]; // Accessing the first element (10)
int secondElement = numbers[1]; // Accessing the second element (20) - Initialization: Arrays in C can be initialized during declaration by providing a comma-separated list of values enclosed in curly braces {}. The number of values should match the size of the array if specified, or the compiler will automatically determine the size based on the number of values initialized.
Example:
int numbers[3] = {10, 20, 30}; // Initializing an array with values
int moreNumbers[] = {1, 2, 3}; // Compiler determines size based on the number of Values - Memory Efficiency: Arrays offer efficient memory usage since elements are stored in contiguous memory locations, allowing for quick and direct access using the index. Arrays are more memory-efficient compared to other data structures that require additional memory for pointers or metadata.
Example:
int numbers[1000000]; // Array of one million integers - Uninitialized Elements: If an array is declared but not initialized explicitly, the elements will have an indeterminate value. It is recommended to initialize all elements before using them to avoid unpredictable behavior.
Example:
int numbers[5]; // Array of integers with uninitialized elements.
In this example, an integer array number of size 5 is declared. Since the elements are not initialized explicitly, their values are indeterminate and may contain any garbage values present in the memory. - Size Calculation: In C, an operator called sizeof() can be used to determine the size of an array in bytes. It returns the total number of bytes occupied by the array, including all its elements. The size of an array is determined during the compilation process.
Example:
int numbers[5];
int size = sizeof(numbers); // Size will be 20 bytes (assuming int is 4 bytes) - String Arrays: In C, strings are represented as character arrays. A string is a sequence of characters terminated by a null character (\0), which marks the end of the string. Arrays in C can be used to store and manipulate strings.
Example:
char greeting[10] = "Hello"; // String array to store "Hello"
In this example, a character array greeting of size 10 is declared and initialized with the string- Hello. The array can hold up to 9 characters (including the null character \0) since one element is reserved for the null character automatically added by the compiler. - Multidimensional Arrays: Arrays in C can have multiple dimensions, allowing the creation of matrices or tables. A multidimensional array can be thought of as an array of arrays, where each element of the outer array is itself an inner array.
Example:
int matrix[3][3] = {{11, 22, 33},{44, 55, 66},{77, 88, 99}}; - Array Decay: When an array is passed to a function in C, it decays into a pointer to its first element. This means that the array is effectively treated as a pointer, and the size of the array is lost. To operate on the array within the function, the size needs to be passed explicitly or determined using a sentinel value (like the null character for strings).
Code Example:
Output:
100 200 300 400 500
Explanation:
We include the standard input-output library header file (<stdio.h>) to enable the use of input and output functions in C.
- We define a function named printArray, which takes two parameters: a pointer to an integer array (int *arr) and the size of the array (int size).
- Inside the function, we use a for loop to iterate through the array elements and the printf() function to display the value of each element followed by a space.
- After printing an element, the newline escape sequence in the next printf() statement shifts the cursor to the next line.
- We then declare and initialize an integer array called numbers with the values {100, 200, 300, 400, 500} inside the main() function.
- Then, we calculate the normal variable size using the sizeof() operators. The formula sizeof(numbers) / sizeof(numbers[0]) determines the number of elements in the array.
- Next, we call the printArray function with the array numbers and their size as arguments.
- The printArray function prints the elements of the numbers array using the printf() statements within the function.
- The main function ends with a return 0 statement, indicating successful execution.
How To Access The Elements Of An Array In C?
Accessing array elements refers to retrieving the values stored in specific positions within an array. Array elements are accessed using their indices, which represent their positions within the array. These indices are put inside the square braces [], also known as the array subscript operator.
In the C programming language, it's important to note that array indices commence from 0, denoting the first element, and progress sequentially up to the size of the array minus 1, which signifies the last element in the array. This indexing concept serves as a fundamental principle for effectively interacting with arrays in C and other programming languages. By specifying the correct index, you can access the specific values held in different positions within the array.
Code Example:
Output:
Element at index 0: 101
Element at index 1: 202
Element at index 2: 303
Element at index 3: 404
Element at index 4: 505
Explanation:
We start the C program example by including the necessary <stdio.h> header file for input and output operations.
- Inside main() function, we declare an integer array called numbers with a size of 5 and initialize it with values 101, 202, 303, 404, and 505.
- To access and display the elements of the array, we use the printf() function. Each printf() statement is used to print an element at a specific index in the array, ranging from 0 to 4.
- By using the format specifier %d within the printf() statements, we can display the integer values stored in the array.
- The code will execute sequentially, printing the elements of the numbers array along with their corresponding indices.
- Ultimately, the program returns 0, indicating a successful execution.
In summary, this code demonstrates the process of accessing and printing array elements by utilizing indexing. It showcases the concept of storing and retrieving values within an array using their respective positions.
What Happens When You Try To Access Elements Beyond The Bounds Of An Array In C?
When attempting to access elements beyond the bounds of an array in C, it can lead to various consequences due to undefined behavior. These consequences are unpredictable and may vary depending on the compiler, platform, and runtime environment. Here are some potential outcomes:
- Memory Corruption: Accessing elements outside the valid range of an array can result in memory corruption. It can overwrite or interfere with adjacent memory locations, potentially corrupting data or causing unexpected behavior in other parts of the program.
- Segmentation Fault: In certain scenarios, accessing elements beyond an array's bounds can trigger a segmentation fault. A segmentation fault occurs when a program attempts to access some memory that it is not allowed to access, leading to a program crash.
- Garbage Values or Unpredictable Results: Accessing out-of-bound elements may result in retrieving random data from uninitialized array memory locations or obtaining unpredictable values. This can lead to erroneous calculations, incorrect program output, or unexpected behavior.
Accessing elements beyond the array's bounds is considered a programming mistake and should be avoided to maintain program correctness and stability. To prevent accessing elements outside the array's bounds, it is crucial to carefully validate and verify the array indices before accessing elements. By ensuring that the indices are within the valid range (0 to the size of the array minus one), you can avoid the issues associated with out-of-bounds access.
Loop Through An Array In C
Iterating through an array in C involves using loop constructs. The most popular ones are the for loop or the while loop. These loops allow sequential accessing and processing of each element of the array without the need for repetitive code. By utilizing a loop, you can perform operations on every element of the array in an organized and efficient manner. The essence of iteration lies in its ability to repeatedly perform the same set of actions on different array elements, thereby avoiding the need for redundant code and manual handling.
Steps to loop through arrays in C:
The following are the steps to loop through an array using a for-loop:
- Initialize a loop variable (usually an index variable/ control variable) to represent the current position within the array. You typically start with an index of 0 if you want to iterate through the entire array from the beginning.
- Use a for loop to iterate through the array. The loop should run from the initial index (usually 0) to the last index (size of the array minus one).
- Access the elements of the array using the loop variable/ index. You can retrieve the element at the current index using square brackets, e.g., array[index].
- Perform any desired operations on the array elements within the loop. Including reading, modifying, or processing the elements based on your specific requirements.
- Continue looping until you reach the end of the array, which is determined by the loop condition.
- After processing all elements in the array, exit the for loop, and you've successfully looped through the entire array.
So now, let's look at an example that showcases how we iterate through an array in C and access its elements using a for-loop.
Code Example:
Output:
15 25 35 45 55
Explanation:
- In this example, we declare an integer array called numbers with five elements, and initialize it with values {10, 20, 30, 40, 50}, inside the main() function.
- We then create a for loop, with the control/ loop variable i initialized to 0. The loop continues as long as i is less than the size of the array (5 in this case).
- In each iteration, the loop body is executed, which performs an operation on the current element numbers[i].
- In this example, we add 5 to each element by using the compound addition assignment operator (+=).
- After the loop finishes execution, we have modified the elements of the array.
- To verify the changes, we use another for loop to print the updated array elements. Each element is accessed using the index i, and its value is printed to the console using the printf() function.
- The output shows the updated array elements where each element has been increased by 5 due to the operation performed inside the loop.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Changing Elements In An Array In C
Changing elements in an array refers to modifying the values stored at specific positions within the array. In C, you can update the contents of an array by assigning new values to individual array elements using their indices.
Code Example:
Output:
Original Array: 110 210 310 410 510
Modified Array: 110 210 90 410 510
Explanation:
- In this C example, we have an integer array called numbers with five elements. The array is initially initialized with values {110, 210, 310, 410, 510}.
- We then employ a for loop to print the original array elements, where it loops through the array from indices 0 to 4, and uses a printf() statement to display them to the console.
- To change an element in the array, we use the array name followed by the index within square brackets to access the specific element we want to modify.
- In the code example, we change the value of the element at index 2 by assigning a new value of 90, i.e., numbers [2] = 90.
- After modifying the array element, we again iterate over the array using a for loop and print both the modified array elements to the console.
The output demonstrates how the value of the array element at index 2 is changed from 30 to 90. The rest of the array elements remain unaffected. By assigning new values to specific indices, you can effectively change the contents of individual elements within an array.
How To Sort Arrays In C?
Sorting an array involves arranging the elements of the array in a specific order, like ascending or descending. Sorting allows the organization of the elements to make searching, manipulation, and other operations more efficient. There are various sorting algorithms available, but for simplicity, let's focus on the bubble sort algorithm.
Syntax to implement bubble sort algorithm:
void bubbleSort(data_type array [], size_t length);
Here,
- void: Specifies the return type of the function. In this case, Bubble Sort typically does not return any value (hence void).
- bubbleSort: Represents the name of the Bubble Sort function. This is the identifier you will use to call the sorting algorithm.
- data_type: Specifies the data type of the elements in the array that you want to sort (e.g., int, float, char, etc.). This is the type of data that the array is holding.
- array []: This is the name of the array that you want to sort. The square braces [] indicates that the variable is an array.
- size_t: This is a data type used to represent the size or length of the array. It's used here to specify the number of elements in the array.
- length: This is a parameter representing the number of elements in the array that you want to sort. It's of type size_t and should be replaced with the actual length of your array when calling the function.
Code Example:
Output:
Array before sorting: 5 2 8 12 1
Array after sorting: 1 2 5 8 12
Explanation:
- We begin the C code example by including the essential header files and then define the bubbleSort() function. which implements the bubble sort algorithm to sort an array in ascending order
- The function takes an integer array and its size as parameters. Inside the function-
- There are two for loops where the outer loop iterates size-1 times, and at each iteration, it moves the largest element to the correct position.
- The inner loop compares two adjacent elements using an if-statement, and if the first is greater than the second, it swaps the elements using a temporary variable.
- Inside the main() function, we first declare an integer array called numbers with five elements and initialize it with unsorted array values {5, 2, 8, 12, 1}.
- We then use a for loop to print the original array elements before sorting.
- Next, we call the bubbleSort() function by passing the numbers array and its size as arguments. The function modifies the array in place.
- Finally, we print the sorted array using a for loop with the printf() function to verify the sorting result.
- The output demonstrates the array before sorting and after sorting using the bubble sort algorithm. The array elements are rearranged in ascending order, as indicated by the sorted output.
It's important to note that the bubble sort method is a simple sorting algorithm often used for educational purposes. In practice, more efficient sorting algorithms like quicksort or mergesort are commonly used for larger arrays to achieve better performance.
Input And Output Elements In Arrays In C
Input and output of array elements in C refer to the process of reading values from the user or a data source and storing those values in an array, as well as displaying the values of the array elements to the user or outputting them to a desired destination. This allows for interaction with the array data and facilitates data processing or presentation.
Example:
Output:
Enter 5 integers:
3 45 6 7 92
The array elements are:
3 45 6 7 92
Explanation:
- In this example, we begin by including the standard input/ output library and defining a macro global value SIZE.
- Then, inside the main() function, we declare an integer array called numbers of size SIZE.
- We then use the printf() function to prompt the user to enter 5 integers, which are then stored in the array using a for loop and the scanf() function.
- The entered values are then outputted by iterating over the array using another for loop and the printf function. Each element is printed to the console, separated by a space.
- The program demonstrates how to input values into an array by sequentially reading user input and storing it in the array elements. It also illustrates how to output the array elements to the user, providing a visual representation of the array data.
- By combining input and output operations, you can interact with arrays in C, allowing users to provide input that is stored in the array and display the array's contents in a meaningful way.
How To Search For An Element In Arrays In C?
Searching for an element in an array involves finding the position or index of a specific value within the array. This process allows you to determine whether a particular value exists in the array and locate its position if it does.
Example:
Output:
Enter the value to search for: 87
Element not found in the array
Explanation:
- In the given code example, we have initialized an array called numbers containing five integers: {10, 20, 30, 40, 50}.
- We declare two more integer variables, searchValue without initialization and position initialized with -1.
- Next, we prompt the user to input a value to search for using printf(), which we store in the variable searchValue using scanf() and address operator (&).
- We then use a for loop (starting with the for keyword) to iterate through each element of the array.
- Within the loop, we check if the current element matches the searchValue using the equality relational operator (==).
- If a match is found, the program records the index of the element in the single variable position and terminates the loop using a break statement.
- Following the loop run, the program examines the value of the position variable using an if-else statement.
- If it is not -1, it signifies that the element was found. In such a case, the program displays the index at which it was found.
- Else, if the position variable remains -1, it indicates that the element was not found, and the program displays a corresponding message.
- The main function ends with a return statement, indicating successful execution.
- This code demonstrates a simple linear search algorithm in C.
Types Of Arrays In C
In this section, we will discuss the primary types of arrays in C programming.
One-Dimensional Arrays In C
A one-dimensional or 1-D array in C is a collection of elements of the same data type arranged in a linear sequence. It is the simplest and most commonly used type of array. Each element in a one-dimensional array is accessed using a single index. The size of a one-dimensional array is determined at the time of declaration and remains fixed throughout the program execution.
The syntax for 1-D Array Initialization in C:
data_type array_name[size];
Here,
- data_type: Specifies the data type of the elements in the array (e.g., int, float, char, etc.).
- array_name: Represents the name of the array.
- size: Indicates the number of elements the array can hold.
Example:
Output:
1 2 3 4 5
Explanation:
- In this C code, we declare an integer array named numbers with five elements, initialized with values {1, 2, 3, 4, 5}.
- We then use a for loop to iterate through the array elements, allowing us to access and print each element individually using the printf() function.
- The loop is structured to traverse the array systematically, starting from the first element (index 0) and ending at the last element (index 4).
- The main function ends with a return statement, indicating successful execution.
This code illustrates the concept of a one-dimensional array and demonstrates how to access and print its elements using a for loop.
A Characters Array In C (Strings)
In the C programming language, a string is stored as an array of characters. It is essentially a one-dimensional character array terminated by a null character, i.e., \0. Strings are frequently used to hold and manipulate text data.
Syntax to Declare String/ Character Arrays in C:
char string_name[size];
Here,
- char: Specifies the data type of the elements in the string (characters).
- string_name: Represents the chosen identifier for the string array.
- size: Specifies the maximum size of the character array. This includes the characters of the string and the null character (\0), which marks the end of the string.
Code Example:
Output:
Example: Unstoppable content
Explanation:
- In this C code, we declare a character array named name with a size of 20 characters, initialized with the string value- Unstoppable content.
- We then use the printf() function to print the content of the character array, with the format specifier %s indicating that the argument is a string.
- The string in the name array is then printed as part of the output.
- The main function ends with a return statement, indicating successful execution.
This code illustrates the concept of a character array (string) in C and demonstrates how to initialize and print its content using the 'printf' function.
Multidimensional Arrays In C
In C programming language, a multidimensional array is an array that has more than one level or dimension. It is used to represent tabular or matrix-like data structures. This could be a square matrix or a rectangle matrix.
Multidimensional arrays in C can be two-dimensional, three-dimensional, or even higher-dimensional. We will discuss these types with the help of examples in this section.
Two-Dimensional Arrays In C
In programming, a two-dimensional array is a data structure that provides a way to store and manipulate values in a grid-like fashion, consisting of rows and columns.
- It is commonly used to represent matrices or grids.
- Accessing elements in a two-dimensional array requires two indices- one for the row and one for the column.
- Unlike a one-dimensional array, which is a linear collection of elements, a two-dimensional array adds a level of complexity by introducing rows and columns.
To declare a two-dimensional array in C, you need to specify the dimensions (number of rows and columns) along with the data type of the elements it will hold.
Syntax to Declare Two-Dimensional Arrays in C:
data_type array_name[row_size][column_size];
Here.
- data_type: Indicates the type of data elements that will be stored in the array.
- array_name: This is the chosen name for the two-dimensional array.
- row_size: Specifies the number of rows the array should have.
- column_size: Specifies the number of columns the array should have.
Code Example:
Output:
1 2 3
4 5 6
7 8 9
Explanation:
- In this C code, we declare a two-dimensional integer array named matrix with dimensions 3x3 inside the main() function.
- The dimensions represent a 3x3 matrix with elements initialized in row-major order.
- We then use a set of nested for loops to iterate through each row and column of the array, allowing us to access and print each element.
- The control variable of the outer loop runs/ traverses the row beginning from the first to the last.
- The inner loop (and control variable) iterates through the columns.
- We use the printf() function to display the element at that position. The loops are structured to traverse the matrix systematically.
- After the inner loop is done with iterations of the columns in that specific row, the control moves back to the outer loop, which moves to the next row.
- When the loops are done, the main function ends with a return statement, indicating successful execution.
This code illustrates the concept of a two-dimensional array and demonstrates how to access and print its elements using nested loops.
Three-Dimensional Arrays In C
In the context of programming, a three-dimensional array is a data structure that allows you to store and manipulate values in a three-dimensional grid. It can be thought of as a collection of two-dimensional arrays stacked on top of each other.
- A three-dimensional array in C expands on the idea of a two-dimensional array by introducing another dimension.
- This enables data organization in a grid-like manner with depth, rows, and columns.
- This structure is valuable for handling volumetric data, like 3D graphics, medical images, and simulations.
- It is used to represent data structures that have three levels of organization.
- Similar to two-dimensional arrays, accessing elements in a three-dimensional array requires three indices.
To declare a 3D array in C, you need to specify the dimensions along with the data type of the elements it will hold.
Syntax to Declare Three-Dimensional Arrays in C:
data_type array_name[size1][size2][size3];
- data_type: Specifies the data type of the elements in the array (e.g., `int`, `float`, `char`, etc.).
- array_name: Represents the name of the array.
- size1, size2, size3: Indicate the sizes of the three dimensions of the array.
Code Example:
Output:
1 2 3 4
5 6 7 8
9 10 11 1213 14 15 16
17 18 19 20
21 22 23 24
Explanation:
- In this C code, we declare a three-dimensional integer array named cube with dimensions 2x3x4.
- It consists of two 2D arrays, each with three rows and four columns, forming a structure similar to a cube.
- We use nested for loops to iterate through each dimension of the array, accessing and printing each element. The single loops are structured to traverse the array systematically.
- The printf() statements inside the loops print the value of the element at the position given by indices i, j, and k.
- The main function ends with a return statement, indicating successful execution.
This code illustrates the concept of a three-dimensional array and demonstrates how to access and print its elements using nested loops.
Functions And Arrays In C
Arrays in C can be used in close association with functions. They can be passed as parameters to a function and can also be returned from a function. We will discuss both these concepts in this section.
Passing An Array To A Function In C
When an array in C is passed to a function as its argument, it is actually passed by reference. This means that any modifications made to the array within the function will affect the original array in the calling code. This allows functions to operate on arrays and modify their contents.
The Syntax for Passing an Array to a Function in C:
To pass an array to a function, you need to declare the function parameter as an array. Since arrays in C decay into pointers when passed to functions, you can either declare the parameter as a pointer or as an array of fixed size. The basic syntax for both cases is given below.
1. Declaring the parameter as a pointer:
return_type function_name(data_type *array_name, size_t array_size);
2. Declaring the parameter as an array of fixed size:
return_type function_name(data_type array_name[size]);
Here;
- return_type: Specifies the data type of the value returned by the function.
- function_name: Represents the function name.
- data_type: Specifies the data type of the elements in the array.
- array_name: Represents the name of the array parameter.
- size: Indicates the size of the array or can be left empty for a dynamically sized array.
Example:
Output:
Array elements: 1 2 3 4 5
Explanation:
- In this sample program, we include the standard input-output library header file and define a function printArray.
- The function takes a pointer to an integer array and the size of the array as parameters, printing each element using a for loop.
- This for loop begins with the control variable set to 0 (i=0), and the printf() statement displays the value of the element at ith position.
- After every iteration, the control variable is incremented by 1, and the loop runs till i<size.
- In the main() function, we declare and initialize an integer array named numbers with values {1, 2, 3, 4, 5}.
- We then print a message indicating array elements will follow and call the printArray function with the array and its size as arguments.
- The program ends with a return statement in the main function, indicating successful execution.
Note: This code demonstrates our use of a separate function for printing array elements, enhancing modularity and reusability. By utilizing the printArray() function, the code becomes more organized, and any changes made to the array within the function are automatically reflected in the main code. This eliminates the need for explicitly returning modified arrays.
Returning An Array From A Function In C
In C programming language, it is not possible to directly return an entire array from a function. However, a similar result can be achieved by returning a pointer to the first element of the array in C. By returning a pointer, you can access the elements of the array in the calling code.
Syntax to Return an Array from a Function in C:
To return an array from a function in C, you need to declare the return type of the function as a pointer to the element type of the array. The syntax is as follows:
data_type* function_name(arguments){
// Function body
// ...
return pointer_to_array;}
Here.
- data_type: Specifies the data type of the elements in the array.
- function_name: Represents the name of the function.
- arguments: Any arguments required by the function.
Inside the function, you can create an array, allocate memory dynamically, or use a static array. Then, you can return the array by returning the pointer to its first element.
Note that when you return an array from a function, you need to be cautious about the lifetime of the array. If you allocate memory dynamically, the responsibility lies with the caller to free that memory when it's no longer needed.
Code Example:
Output:
Array elements: 1000 2000 3000 4000 5000
Explanation:
- In this C code, we include the standard input-output library header file and define a function named generateArray.
- In this function, we declare a static integer array named arr and initialize it with values {1000, 2000, 3000, 4000, 5000}. We then return a pointer to the first element of the array.
- In the main() function, we declare a pointer to an integer named result and assign it the return value of the generateArray function. This ensures that result points to the beginning of the array.
- Next, we print a message indicating that array elements will follow using a printf() statement.
- We then use a for loop to iterate through the array elements, printing each element using the pointer result.
- The expression result[i] dereferences the pointer and accesses each element in the array as we iterate.
- The main function ends with a return statement, indicating successful execution.
This code demonstrates the concept of returning a pointer to an array from a function and using it in the main program to access and print array elements.
Relationship Between Pointers And Arrays In C
Pointers are data types that are capable of storing the addresses of other array variables within the code. As the name suggests, they point towards the address (highest address or lowest address) of memory locations or collection of variables.
In the C programming language, there exists a close relationship between arrays and pointers. In fact, arrays can be considered as a form of pointers. Understanding this relationship is crucial for effectively working with arrays.
- In C, the name of an array can be used as a pointer to the first element of the array.
- This means that the array name itself represents the memory address of the first element in the array.
- When an array is declared, for example, int numbers [5], it represents a contiguous block of memory capable of holding 5 integer elements. The name numbers can be treated as a pointer that points to the memory location of the first element, which is numbers [0].
By leveraging this array-pointer relationship, we can perform operations on arrays using pointer arithmetic and other pointer-related operations. It allows us to access and manipulate array elements by dereferencing the pointer or using pointer arithmetic to navigate through the array.
Syntax to Access Array Elements Using Pointers:
*(array_name + index)
Here,
- array_name: The name of the array
- index: Represents the position of the element we want to access.
- dereference operator (*) is used to get the value at the memory location pointed to by the pointer.
Code Example:
Output:
10 15 20 25 30
Explanation:
- In this C program, we include the standard input-output library header file.
- Inside the main() function, we then declare an integer array named numbers, initializing it with values {10, 15, 20, 25, 30}.
- We then declare a pointer to an integer named ptr and assign it the address of the first element of the numbers array. This allows ptr to point to the beginning of the array.
- Using a for loop, we then access and print array elements using pointers. The expression *(ptr + i) is used to dereference the pointer and access each element of the array as we iterate through it.
- The main function ends with a return statement, indicating successful execution.
This code demonstrates the concept of using pointers to access and manipulate array elements in C.
Advantages Of Arrays In C
Some of the most common advantages of using arrays in C are as follows:
- Efficient Access: Arrays allow for efficient access to elements using their indices. Since arrays store elements in adjacent memory locations, accessing elements by an index is a constant time operation. This makes arrays in C suitable for situations where fast and direct element access is needed.
- Memory Efficiency: Arrays have a fixed, determined size during declaration, allowing for efficient memory allocation. The memory for the entire array is allocated in a single block, resulting in better memory management compared to dynamically allocated structures.
- Sequential Storage: Array elements are stored one after the other in memory, making it the simplest form to go through the elements by using loops. This pattern of storing is helpful when doing tasks such as searching, sorting, or changing the array elements
- Efficient for Numerical Computations: Arrays in C are often used for numerical calculations and handling substantial amounts of data. Their quick access and sequential storage make them perfect for tasks that involve math operations, data analysis, and scientific computing
- Compatible with Pointers: Array data structures in C have a close relationship with pointers. They can be easily manipulated using constant pointer variable arithmetic and can be passed as arguments of arrays to functions. This compatibility with pointers allows for more flexibility in working with arrays in C and implementing advanced algorithms.
Disadvantages Of Arrays In C
- Fixed Size: Arrays in C have a fixed size determined at compile time. Once the size is defined, it cannot be changed dynamically during runtime. This limitation restricts the flexibility of array after creation, making them less suitable for scenarios where the size of the data needs to grow or shrink dynamically.
- No Bounds Checking: C does not perform automatic bounds checking on array accesses. If an index goes out of bounds, it can lead to memory block access errors or undefined behavior and unexpected output. It is the programmer's responsibility to ensure proper bounds checking when working with arrays in C to avoid such issues.
- Inefficient Insertion and Deletion: Insertion or deletion of elements can require shifting or rearranging the existing elements, which can be inefficient for large arrays in C. The time complexity for such operations is proportional to the size of the array, making them less efficient compared to other dynamic data structures like linked lists.
- Wasted Memory: Array data type allocates memory for the maximum possible size, even if the actual number of elements stored is less. This can result in wasted memory if the array does not reach its full capacity.
- Lack of Dynamic Resizing: Arrays cannot be easily resized dynamically in C. If the size requirements change, the programmer needs to manually reallocate memory and copy the elements to a new array of the desired size. This process can be complex and error-prone, such as compile time error.
Understanding these advantages and disadvantages of arrays in C allows programmers to make informed decisions when choosing the appropriate data structure for their specific needs.
Unique Features Of Arrays In C
Here are some unique aspects and considerations specific to arrays in C that showcase the versatility of arrays in C programming, demonstrating how they can be applied to various scenarios and challenges:
- Memory Alignment: The memory alignment of array elements is determined by the size of the data type. This consideration becomes crucial in applications where memory access performance is critical.
- Arrays and Standard Library Functions: Arrays are extensively used in combination with standard library functions such as those in <string.h> and <stdlib.h>. Understanding how these functions interact with arrays is essential for efficient coding.
- VLA (Variable Length Arrays): C99 introduced the concept of Variable Length Arrays, allowing the size of an array to be determined at runtime. However, VLA usage is not as common, and dynamic memory allocation is often preferred for flexibility.
- Sparse Arrays: While not directly supported, sparse arrays (arrays with a large number of zero or null values) can be optimized by using techniques such as sparse matrix representations.
- Jagged Arrays: Although not a native concept in C, jagged arrays can be simulated using arrays of pointers. This enables the creation of arrays where each element can have a different size.
- Array Arithmetic: Pointer arithmetic can be applied to arrays in C, allowing for advanced manipulation and navigation through array elements.
Application Of Arrays In C
Arrays in C find application in various programming scenarios due to their versatility and efficiency. Here are some common applications of arrays in C:
- Storing and Processing Lists of Data: Arrays are widely used to store collections of similar user-defined data types, such as a list of integers, floating-point numbers, or characters. For example, you might use an array to store the scores of students in a class, temperatures over a period, or the elements of a vector.
- Matrices and Multi-dimensional Arrays: Arrays in C are employed to represent matrices and multi-dimensional data structures. Matrices are commonly used in mathematical and scientific computations, graphics processing, and image manipulation.
- String Manipulation: Strings in C are essentially arrays of characters. Arrays provide an efficient way to work with strings, allowing for tasks like concatenation, comparison, and manipulation of characters in a string.
- Implementation of Data Structures: Arrays in C are fundamental for implementing various data structures, such as stacks, queues, hash tables, and graphs. For example, an array might be used to implement a stack where elements are pushed and popped from the end of the array.
- Sorting and Searching Algorithms: Sorting and searching algorithms, like bubble sort, quicksort, and binary search, often utilize arrays. Arrays facilitate efficient access to elements, making them suitable for implementing these algorithms.
- Dynamic Memory Allocation: Arrays in C are used in conjunction with dynamic memory allocation functions like malloc() and free() to create resizable data structures. Dynamic arrays can be resized during runtime based on the program's needs.
- Image Processing: In image processing applications, pixel values are often stored in arrays. Operations like blurring, sharpening, or applying filters involve manipulating arrays of pixel values.
- Simulation and Scientific Computing: Arrays in C are crucial for simulations and scientific computing, where large sets of numerical data need to be processed. Applications include simulations of physical systems, numerical analysis, and solving mathematical equations.
- Handling Multiple Values in Functions: Functions in C can return arrays, allowing the handling of multiple values as a single unit. This is useful when a function needs to return more than one value.
- Database Management Systems: Arrays in C are used to represent tables and columns in memory in database management systems. Operations like sorting, filtering, and aggregations often involve manipulating data stored in arrays.
Conclusion
Arrays in C are indispensable tools for programmers, offering a structured approach to handle collections of elements. Their simplicity, efficiency, and versatility make them vital in numerous programming scenarios, from basic data storage to complex algorithmic implementations. Understanding arrays is crucial for mastering C programming and lays the groundwork for advanced data manipulation and algorithm design. As you delve deeper into C programming, honing your skills with arrays will empower you to tackle a wide array of computational challenges.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. What are arrays in C with example?
In C, an array is a collection of elements of the same data type stored in contiguous memory locations. Each element in the array is identified by its index or subscript. Below is an example of arrays in C.
Code Snippet:
Output:
Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
Explanation:
In this C program, we declare and initialize a positive integer array of numbers with values {10, 20, 30, 40, 50}. We then iterate through the array using a for loop, printing each element's value along with its index using the printf function. The output displays the elements and their respective indices in the array.
Q. How to write an array of arrays in C?
An array of arrays in C is often referred to as a two-dimensional array (2-D Array). It's essentially an array in which each element is itself an array. Below is an example showcasing how you can declare and work with a two-dimensional array
Code Snippet:
Output:
1 2 3 4
5 6 7 8
9 10 11 12
Explanation:
In this example, We create a column order matrix is a 2D array with three rows and four columns. We initialize its elements using nested curly braces. The outer set of braces represents the rows, and the inner set represents the values in each row.
You can access individual elements using the row and column indices. In this example, we use a nested loop, where the terms 2-D matrix [i][j] give you the element at the ith row and jth column. The loops are used to iterate through all elements of the 2D array.
Q. Is an array in C a collection of values?
Yes, an array is a collection of values in programming. In the context of programming languages like C, an array is a data structure that allows you to group together a fixed-size sequence of elements, all of the same data type, under a single identifier. These elements are stored in contiguous memory locations, and each element in the array is identified by its index or position within the collection.
Arrays in C programming are commonly used to store and organize related pieces of data. For example, you might use an array type to represent a list of temperatures over time, scores of students in a class, or the pixel values of an image. The ability to access elements using an array by indices makes it efficient to perform operations on the entire collection, such as iterating through elements, sorting, searching, and applying mathematical operations.
Q. What is the difference between collection and arrays in C?
Aspect |
Array |
Collection |
Definition |
A fixed-size sequence of elements |
General term for various data structures |
Data Type |
Elements must be of the same type |
Elements can be of different types |
Contiguity |
Stored in contiguous locations in memory |
May or may not be stored in contiguous locations |
Access |
Direct access using numerical indices |
Access methods vary based on data structure |
Resizing |
Fixed-size, which cannot be dynamically resized |
Can dynamically resize based on user-specified size requirements |
insertion |
Limited or complex insertion operations |
Can support efficient insertion operations |
Deletion |
Limited or complex deletion operations |
Can support efficient deletion operations |
Searching |
Linear search or other custom algorithms |
Can offer efficient search algorithms |
Flexibility |
Limited flexibility due to fixed size |
More flexibility in terms of size and usage |
Memory Usage |
May have unused memory if the size is large |
Memory-efficient data structures possible |
Q: What are the different types of arrays in C?
In programming, arrays come in various forms to accommodate different yet simplest data structures and use cases. Here are some common types of arrays:
- One-Dimensional Arrays: Just like having a row of things, and you use numbers to pick which one you want.
- Multi-Dimensional Arrays: Think of a table, like a spreadsheet, where you can organize stuff in rows and columns.
- Fixed-Size Arrays: Imagine having boxes of different sizes where you neatly arrange your things.
- Dynamic Arrays: These are like magic boxes that can expand or shrink to hold your things as you need.
- Sparse Arrays: Imagine having boxes that only store important things and leave spaces empty for the rest.
- Jagged Arrays: It's like a box that holds smaller boxes, and each of those might have even smaller boxes inside.
- Pointer Arrays: These boxes don't store things themselves; they just point you to where the things are.
- Character Arrays (Strings): Think of boxes that hold words and sentences, like tiny notes or letters.
- Homogeneous Arrays: These boxes are only like one kind of thing, just numbers or only words.
- Heterogeneous Arrays: These boxes are friendly and let you put different stuff in them, like numbers and words together.
- Static Arrays: Boxes with fixed sizes that don't change, just like a puzzle with a set number of pieces.
- Dynamic Arrays: Imagine boxes that stretch or shrink, like a balloon, so they always fit what you need to store.
These were the different types of arrays in C and other programming languages.
You might also be interested in reading the following:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment