Variables In C | Types, Rules & Related Concepts (+Code Examples)
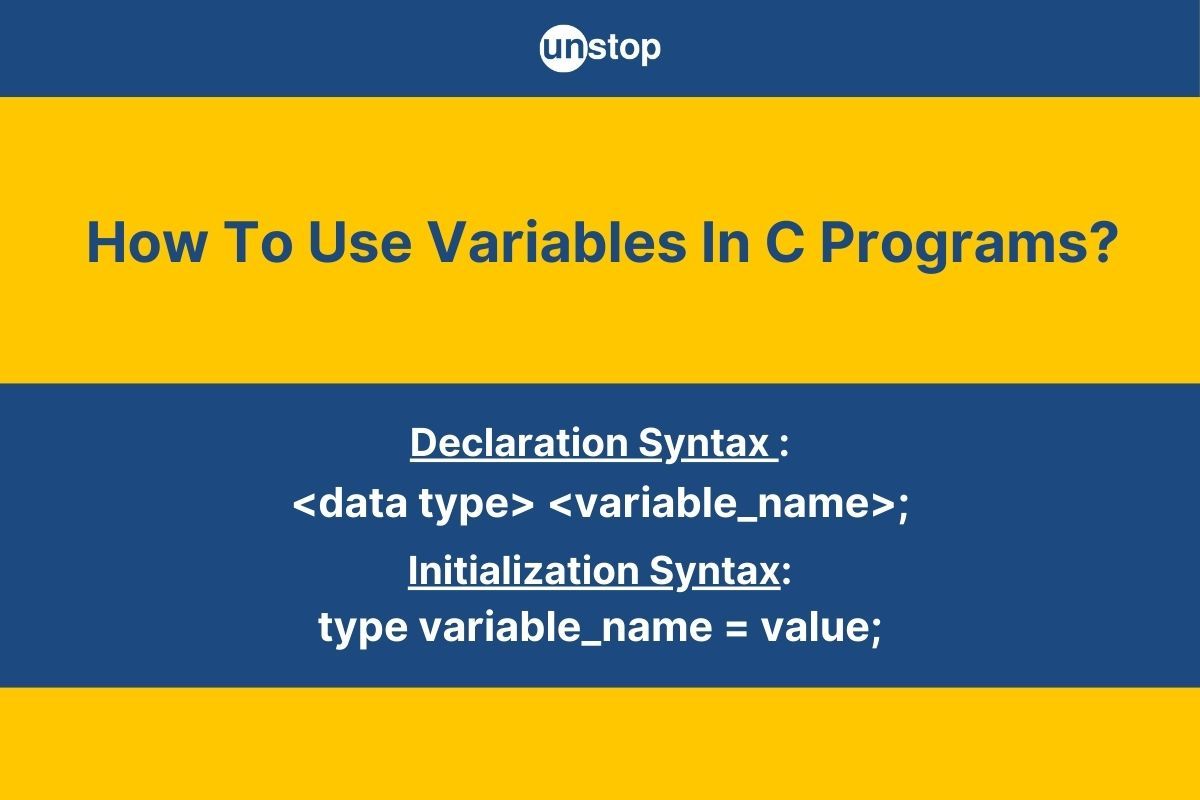
Variables are named memory locations that store data or information and can be accessed, manipulated, or changed. They are usually declared with specific data types so that the compiler knows how much memory it needs to allocate for them.
In this article, you will find everything you need to know about these variables in C programming, including how to define/ declare them, initialize values, retrieve the data and the various types, with detailed examples.
What Is A Variable In C Programming?
In simple terms, a variable in C programs refers to the names given to a memory location where we store data. We can create variables to store different types of data, which also determines the amount of space the variable takes.
- This means that variables in C language can hold values ranging from various primitive data types (such as integers, characters, strings, floating-point numbers, etc.) to user-defined types like structures and unions.
- These variables must be explicitly declared by using keywords for specific data types, like int (for integer type), float (for floating-point values), char (for characters), etc.
- Also, each variable has a unique name/identifier, which helps us retrieve the values stored in that location without memorising the actual address in memory.
Syntax For Declaring Variables In C Language
data_type variable_name;
Here,
- The data_type indicates the type of value/ data that you can store in the variable. These are usually keywords like int (for integer type), char (for character type), etc.
- The term variable_name refers to the identifier/ name you give the respective variable.
This is the basic syntax for declaring a variable. We will explore all the other aspects of declaring, defining, and initializing variables in the sections below. Here are some example snippets of how to declare variables in C language:
int age; //This is an integer type variable with the name age
char letter; // This character type variable can store any letters
float pi; // Stores floating-point values with accurate decimal places like 3.141592
Process Of Creating A Variable In C Programs
Creating a variable in C and readying it for use can be divided into three steps:
- Declaring Variables In C
- Defining Variables In C
- Initializing Variables In C
Let's discuss what each of these steps entails.
Declaration & Definition Of Variables In C
We've already seen the syntax for declaring a variable in C language. The main purpose of declaring a variable is to inform the compiler about the existence of a variable where we will store data.
- In this phase, indicating the variable's name and the data type is enough for the compiler to proceed with the compilation process (without details).
- As soon as the compiler gets the signal, it creates an entry for the variable in the symbol table.
Note: Until C99 and C11, ANSI C standard, stipulated that all variables must be declared at the beginning of the program. While these stipulations have been relaxed in newer standards, the practice has been carried on and most prefer to declare variables at the start.
We can provide additional information at the time of declaration of variables in C (in addition to data type and name) by using qualifiers. In such a case, the syntax for the declaration of variables in C will be:
qualifier data_type variable_name;
Here, the data_type and variable_name components are the same as above. The expression qualifiers refer to other modifiers that provide additional information about the variable and its nature.
- For example, the const keyword can be added to indicate constant variables, thus modifying how it behaves at runtime. It indicates that the value assigned will remain constant/ unchanged throughout the program.
- Similarly, storage class keywords like auto and register can be used to declare auto variables (whose data type is determined automatically depending on the type of value) and register variables to specify where the data will be stored in memory.
What Does Definition Of Variables In C Programs Mean?
Variable definition is a sort of mid step (or behind-the-scenes step) fulfilled by the compiler. It entails reserving/ allocating memory space for the variable for when any program execution happens. The size of this memory space is determined with the help of the data type.
In other words, in the variable definition phase, the compiler connects the variable name to a memory address of sufficient size. Note that, at this point, the variable has not yet been initialized and will contain some random garbage value. (The value will be set in variable initialization, which we will discuss in the next section).
The variable definition step only has relevance during the compilation process, as the compileruses this info for linking the program. Ideally, the declaration and definition of the variables in C is s single step.
Initialization Of Variables In C
In C programming, initialization refers to the process of assigning an initial explicit value to the variable. This value replaces the garbage value assigned to the variable by the compiler during definition.
There are two ways to initialize or assign a value to the variable: at the time of the declaration or after the declaration. The former can be called inline initialization.
Syntax For Initialization Of Variables In C
data_type variable_name = value;
Here,
- The variable_name and data_type refer to the name of the variable and the type of value stored in it, respectively.
- The terms value refers to the dedicated data value you want to assign to the variables. This literal value must be of the data type specified.
int myNumber = 10;
Here, we define an int type variable with the name myNumber and assign it an integer value of 10. The alternate way to initialize a variable in C is to assign a value in a separate line after the declaration. For example:
int number1 = 23; // This is single line declaration & initialization
int number2;
number2 = 11; //Initialization after declaration
This alternate method is also used to modify the value after initialization, and is hence often referred to as assignment of value to variables in C programs.
The Need To Initialize A Variable
While allocating memory for that specific variable, the compiler does not change its value. Initializing variables in C programs is hence essential to ensure that the variables have known values when used in expressions and other computations later within your code instead of uninitialized garbage values. This helps avoid bugs caused by incorrect/ unexpected results from undefined behavior.
Look at the simple C program example below to understand how to declare variables, initialize and use them.
Code Example:
Output:
myNumber: 20
MyCharacter: s
MyFloat: 4.500000
Explanation:
In the simple C code example, we begin by including the header file <stdio.h> for input/output operations.
- We then initiate the main() function, which is the entry point for program execution.
- In main(), we declare three variables of different data types and initialize them at the same time, i.e., in a single line.
- The first variable is of integer type with the name myNumber and is initialized with the integer value 20.
- Then, we have the character type variable called myChar, which we initialize with the single character 's'.
- Lastly, we have a floating point variable called myFloat, whose initial value is 4.5.
- After that, we use three printf() statements to display the values of these variables inside a formatted string.
- In the formatted strings, we use %d, %c, and %f format specifiers to indicate the data type of the value that will be inserted in the place. Also, the newline escape sequence (\n) shifts the cursor to the next line after every output.
In the C code example above, we explored how to declare and initialize variables of different data types in a single line. Let's look at another example where we use the alternate approach to declare and initialize variables separately.
Code Example:
Output:
The value stored in variable var is: 89
Explanation:
In the C program example,
- Inside the main() function, we declare an integer variable called var without any initial value.
- In the next line, we assign the value 89 to the var variable using the assignment operator.
- Then, we use the printf() function to display the value of the variable to the console.
- Lastly, the main() function completes successful execution with a return 0 statement.
Check out this amazing course to become the best version of the C programmer you can be.
Rules To Name Variables In C
When declaring or naming the variables in a C program, one must take note of the following stipulations:
- Variable names should begin with a letter or underscore(_).
- No special characters other than an underscore are allowed (e.g., #, @, $, etc.) in C variable names.
- Whitespaces and hyphens (-) are not allowed while declaring a variable in C language.
- Keywords like int, char, and float cannot be used as variable names since they have special meanings in the programming language syntax and are reserved by the compiler to perform specific tasks only.
- The declaration of variables inside the block of functions are automatic variables by default.
When naming variables in C, it's important to follow certain conventions and restrictions:
-
Variable names must begin with a letter (a-z, A-Z) or an underscore (_). Variables cannot start with a digit.
int _validName; // Correct
int validName; // Correct
int 9invalid; // Incorrect: Cannot start with a number
-
No special characters, other than an underscore (_), are allowed. Avoid using characters like #, @, or $.
int valid_name; // Correct
int invalid#name; // Incorrect: Special character # is not allowed
-
Variable names cannot contain spaces or hyphens (-): For multi-word variable names, use underscores (e.g., total_count) or follow camelCase (e.g., totalCount).
int validName; // Correct
int invalid name; // Incorrect: Whitespace is not allowed
int invalid-name; // Incorrect: Hyphen is not allowed
-
C keywords cannot be used as variable names: Keywords like int, float, or return are reserved for specific functionalities within the language.
int validVar; // Correct
int int; // Incorrect: int is a reserved keyword
-
Case sensitivity: C is case-sensitive, meaning var, Var, and VAR would all be considered distinct variable names.
-
Variable names should be meaningful: Descriptive names make code easier to read and understand, especially in larger programs (e.g., totalStudents is preferable over x).
-
Automatic variables: Variables declared inside function blocks are automatic variables by default, meaning their storage duration is automatically managed by the program.
void myFunction() {
int autoVar; // Correct: Automatically an auto variable
}
Types Of Variables In C
There are two commonly known types of variables: local and global variables in C. In this section, we’ll dive into these types, along with automatic, external, and static variables in C language.
Local Variables In C
In C, a local variable is one that’s accessible only within the scope where it's declared. This means the same-named variables declared in different functions or blocks are treated as separate entities. Local variables in C code can store data while running a specific set of instructions without affecting other parts of your program.
Syntax For Local Variables In C
datatype variableName; // Declare local variable
The syntax is same as normal variable, the difference is in the place where the variable is declared. Look at the example C program below for a better understanding of the concept.
Code Example:
Output:
The value a is: 25
Explanation
In the example C code,
- We declare a local variable a and initialise it with the value 25 inside the main() function.
- The scope of this variable is only within the main function, and it cannot be used in any other functions or blocks outside of main().
- Next, we initiate an if-statement to check if the value of a is equal to 25, using the relational equality operator.
- If the condition is true, the if-block executed printing the value of a with a descriptive string message, to the console.
- This shows that the local variable a is accessible from anywhere in the main() function.
- Inside the statement we also declare another variable c without initialization. This variable is local to the if-statement and its scope restricted to this statement only.
- Outside the statement, we have a comment where we use printf() to display the value of variable c.
- If we uncomment this line, it will raise an error message (error: ‘c’ was not declared in this scope) since c can only be accessed from inside the if-statement.
Global Variables In C
A global variable is declared outside any function and can be used in any function within the same program. These variables persist throughout the program’s execution and are accessible from all functions. Use of global variables in C programs makes debugging easier as their values persist if there’s an internal breakpoint set for debugging purposes.
Syntax For Global Variables In C
datatype variableName; // Declare global variable
Here, just like local variables in C, the syntax for variable declaration is same but the difference lies in the place where it is declared in the program. The sample C program below illustrates this concept.
Code Example:
Output:
15 25
Explanation:
In the sample C code,
- We first declare an integer variable named x and assign value 15 to it, outside any function or code block. This variable has a global scope, i.e., it is accessible to all parts of the program.
- Then, we define a function fun() with void return type, meaning it does not return a specific type of data. Inside:
- We declare another variable y of int type and initialize it with the value 15. This is local to that function only, so it is accessible only to the function fun().
- Then, we use a printf() function to display the values of these variables separated by a white space.
- In the main() function, we call the fun() function without any arguments.
- It prints the values to the console, verifying that the global variable is accessible from anywhere in the program, including other code blocks.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Automatic Variables In C
In C programming language, automatic variables are created when a function or block of code is executed. They automatically go out-of-scope and get destroyed once the function or block exits. These variables have limited visibility and are only accessible within the block where they are declared. If the block is executed again, a new instance of the variable is created.
This means that every time we enter into the same scope /function, an auto keyword variable with the same name but a different value can exist there. Hence one should not rely upon their old state while using it again inside that particular scope/function because its content may change at any time due to a new declaration with the same name in the current execution context.
Syntax For Automatic Variables In C
datatype variableName; // Declare automatic variable (default behavior)
The C program sample below showcases the creation and use of automatic variables.
Code Example:
Output:
Value of x: 0
Value of x: 1
Value of x: 2
Value of x: 3
Explanation
In the C code sample,
- In the main() function, we declare a variable x of type int without initialization. This is an automatic variable, which is the default behaviour for local variables.
- Then we use a for loop with x as its counter variable initially set to 0. Inside:
- The loop begins iteration from x=0 and continues until the loop condition x<=3 remains true. This means the loop will run for 4 iterations.
- In every iteration, the loop prints the current value of the x with a descriptive string message and then the value of x is incremented by 1 (x++).
- The loop terminates when the value of x exceeds 3 (loop condition becomes false), and the main() function finishes execution with a return 0.
- After main() completes execution, all these variables created in it go out-of-scope & destroyed automatically.
External Variables In C
External variables are declared outside any function using the extern keyword. They can be accessed by all functions within the same file or even across multiple C files when declared using extern (within the other file).
- When you declare an external variable, it tells the compiler that this variable has been defined elsewhere in the program.
- The actual memory allocation for the variable happens where it is defined, not where it's declared.
- External variables in C programs have a global scope, meaning they can be accessed by any function that includes their declaration.
- They are typically used when multiple functions or files need to share the same variable.
Syntax For External Variables In C
extern datatype variableName;
Here, the extern keyword is the qualifier that indicates the variable is defined elsewhere. Typically, you would define the external variable in one .c file, and other files can access it using extern. Below is a basic C program example to help you understand these variables.
Code Example:
File 1: extern_var.c
This file gives the definition of the external variable:
// Definition of the external variable
int c; // This allocates storage for the variable
File 2: main.c
In this file, we declare and use the external variable in C language.
#include <stdio.h>
// Declaration of external variable
extern int c;void func() {
printf("In func(): c = %d\n", c);
}int main() {
c = 90; // Assign a value to the external variable
printf("In main(): c = %d\n", c);func(); // Call the function that also uses the external variable
return 0;
}
Output:
In main(): c = 90
In func(): c = 90
Explanation:
In the C example,
- We define the variable c inside the extern_var.c file, where the memory is allocated for it. This variable is a global variable in the actual sense.
- Then, in the main.c file, we use the extern keyword to declare the c variable, signalling to the compile that the variable exists in some other file (i.e., extern_var.c)
- Next, we define the func() function, which displays the value of the variable c, using a printf() statement.
- Then, inside the main() function, we initialize the c variable with the value 90 and print the same using the printf() function.
- After that, we call the func() function, which also prints the value of the c variable with a descriptive message.
- As seen in the output, the external variable c is shared between the main() function and func(). Both functions can access and modify the value of c.
This is how multiple .c files can access and share a single variable. When working with an online compiler, you can combine the two files above and run it as a single code, just like you do for global variables.
Static Variables In C
Static variables are declared using the static keyword, and they retain their value across function calls. Static variables in C can be local (accessible only within the function where it is declared) or global (accessible across the program). However, unlike regular variables, their values persist between function calls.
Syntax For Static Variables In C
static datatype var_name;
Here, the static keyword is the qualifier, indicating that the variable will retain its value across function calls. The basic C code example below illustrates the use of this variable type.
Code Example:
Output:
Result of increment operation is 1
Result of decrement operation is 0
Explanation:
In the example,
- We declare a static variable a of type int and assign the value 0 to it.
- Then, we define two void functions, increment() and decrement(), which increase and decrease the value of a by 1, respectively.
- They also print the updated values using the printf() function.
- In the main() function, we call the increment() function and then the decrement() function.
- As shown in the output, the static variable a retains its value across calls to increment() and decrement().
In short, even though a is local to the file, it keeps its value between function calls, making it ideal when you need to preserve a value across multiple function executions.
Data Type Of Variables In C
The type of a variable in C depends on its contents. The most commonly used data types include integers, floats, characters, and strings. Integer values are stored as whole numbers, while float values can store decimal places. Characters represent individual letters, symbols, or special characters, and strings are composed of multiple characters.
Data Type Of Variables Is Immutable
In C, the data type of a variable must be specified when it is declared. Once assigned a specific data type, you cannot change it directly, as this will result in an error. This immutability is crucial because the compiler needs to know the correct size and format for storing each value. Allowing dynamic type changes could lead to memory allocation chaos and complex debugging issues. Therefore, C requires that types are defined at compile time to efficiently manage storage without unnecessary overhead.
Uses Of Variables In C
Some important uses of variables in C code include:
- Data Storage: Variables hold data used throughout program execution, which can be outputted when required.
- Program Manipulation: By manipulating variables (like arithmetic operations or logical comparison operations, etc.), programs can be easily modified to produce different outputs or solve problems without major code changes.
- Memory Management: Variables are automatically allocated memory locations in RAM, facilitating efficient resource management.
- Increased Code Readability: Variables provide a logical structure to programs, making them easier to read and debug.
- Improved Performance: Reusing variables during execution can reduce overhead associated with frequent memory allocation.
Declaring Multiple Variables In C
You can declare more than one variable in C programs (of the same data type) in a single statement by using the comma separator/ operator. For example:
int x, y;
char c;
float f;
Here, x and y are declared as integer types, c as a character, and f as a float. However, this might not work with all user-defined variables like arrays or structures (struct) in C programs.
Syntax For Declaring Mulitple Variables In C
data_type variable1, variable2, ....;
The C code example below illustrates the concept of declaring multiple variables in a single line.
Code Example:
Output:
x = 5, y = 10, c = A, f = 3.14
Explanation:
In the example,
- Inside the main() function, we declare two integer variables, x and y, and initialize them with values 5 and 10 (respectively) in a single line.
- Then, we declare a character variable c and initialize it with 'A', and a floating-point variable f and initialize it with 3.14.
- We then have a commented line showing an invalid declaration of multiple variables since they have different data types.
- In the following line, we have declared one char and one float variable in a single line using the comma operator.
- If we uncomment this line and run the C program, it will generate an error like
- error: expected identifier or ‘(’ before ‘float’
5 | char c = 'A', float f = 3.14; // Declaring a character variable
| ^~~~~
main.c:7:59: error: ‘f’ undeclared (first use in this function)
7 | printf("x = %d, y = %d, c = %c, f = %.2f\n", x, y, c, f);
| ^
- After that, we call the standard library function printf() to display the values of all the variables, using respective format specifiers and the newline escape sequence.
- Lastly, the main() function closes with a return 0, indicating successful execution.
Format Specifiers & Variables In C
The format specifiers are special characters used to specify the type of data being printed to the output screen. They begin with a per cent (%) sign followed by the corresponding data type character. For example, %d is used for integers, %f for floats, and %c for characters. These specifiers determine how arguments given in the printf() function are displayed. We have used them in examples in all the sections above.
Rvalues And Lvalues In C
Lvalues are objects (variables) occupying identifiable locations in memory, allowing data storage for future use. They refer to the left-hand operand of assignment statements, capable of being specified at compile time, and their contents may change during execution.
Rvalues are temporary values that cannot be assigned or manipulated, typically appearing on the right side of an assignment statement. They do not refer to memory locations and cannot be values.
For example, in variable declarations, the variable name appears on the left-hand side, and the value you want to assign is on the right side.
- The variable name has a memory location associated with it, and its contents can be assigned at compile time and they can be changed during execution. So, in this case, the variable name is the Lvalue.
- On the other hand, the value you want to assign to the variable does not have a memory location explicitly associated with it. Also, it cannot be assigned a value of its own since it is a value to be assigned.
Look at the example of Lvalues and Rvalues in C below to better understand this concept.
Code Example:
Output:
No output. The code only assigns values to variables and does not print anything out, so the program runs successfully with no compile-time errors and output.
Explanation:
In the example,
- We declare a variable RollNo in the main() function and assign the value 4 to it. Here:
- RollNo is the lvalue because it represents a memory location (where an integer is stored).
- The value 4 is the rvalue because it is a literal value and does not represent a memory location. It can only appear on the right-hand side of an assignment, not on the left.
- Then, we assign the value 5 to RollNo variable, where the variable name is again the lvalue, and 5 is the literal/ rvalue.
- Next, we have two commented lines illustrating invalid lvalue and rvalue. Here, we are trying to assign the value 8 to value 4. If uncommented, this will generate a compile-time error because:
- Both 4 and 8 are rvalues (they are literals and have no memory address). You cannot assign a value to an rvalue because rvalues cannot exist on the left side of an assignment.
- Essentially, you're trying to change the value of 4 (which is not allowed) because 4 is a literal and does not have a memory location that can be altered.
Change Values Of Variables In C
Changing the values assigned to variables in C programs simply involves assigning a new value to an existing variable, which replaces its previous contents. This can be done using assignment operators, direct assignment, or through functions like scanf().
Note that this differs from initialization even though we use the same operator,i.e., equality assignment (=), in both because the variable has no or garbage values before the initial value is set.
Syntax:
variableName = newValue;
Here, the variableName refers to the variable whose value you want to modify by assigning it a value different from its previous one, given by newValue.
Code Example:
Output:
Old value of x = 25
New value of x = 45
Explanation:
In the example above,
- We declare and initialize an integer variable x with 25 in the main() function.
- Then, we print this value using the printf() function to showcase the initial value.
- After that, we assign the value 45 to x using the assignment operator and then print the modified value.
This program demonstrates how to change the value of variables in C programming language.
Difference Between Global And Local Variables In C
The main differences between global and local variables can be summarized as follows:
Global Variable | Local Variable |
---|---|
Declared outside the main() function or any code blocks. | Declared inside specific blocks like loops, conditions, or functions. |
It has a global scope, meaning it can be accessed anywhere in the program. | It has local scope, accessible only within the block it was declared. |
Exists for the duration of the program execution. | Exists only for the lifetime of the block or function. |
Visible to all functions in the same source file. | Visible only within the function or block where it was declared. |
Looking for mentors? You might find a match made in heaven, just for you, here.
Conclusion
Variables are essential for programming in the C language. They store data that can be reused and manipulated throughout execution.
- The types of variables must be declared with specific keywords like int for integer variables, float for floating-point and so on. This is needed to let the compiler know how much memory space it needs to allocate for these values during runtime.
- There are various types of variables in C, such as local, global, automatic, and static keywords. Each has its own scope limitations depending on its visibility within certain parts of the code.
- Understanding the difference between local and global variables is crucial, as it directly impacts the program's scope and accessibility.
- You can modify a variable's value, by assigning new data to it using assignment operators.
- Finally, format specifiers allow control over how data is presented during output, enhancing the clarity and readability of program results.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is the variable name in C?
The name of a variable in C refers to an identifier used to label a storage location in memory that holds data. It consists of alphanumeric characters (A-Za-z0-9_) and must follow certain rules:
- It must begin with a letter or underscore (_).
- It can contain letters, digits, and underscores but no special characters like # or &.
- The maximum length is 31 characters (including the null terminator \0).
- Variable names are case-sensitive (e.g., myVar and MyVar are distinct).
Q. What is a variable and its type?
Variables in C are named locations that we use to store data, which can be changed during the program's execution. The type of a variable indicates the kind of data it can store, such as integers, floats, or characters. In C, variables are classified into:
- Local Variables: Local variables are the ones whose scope is limited to inside the block of code where they are declared. That is, they cannot be used outside that particular block/scope.
- Static Variables: Static variables retain their values between function calls, meaning static variable can’t be changed after it is initialized once in a program life-cycle and remains the same till the end of the program execution duration.
- Instance Variables: The instance variable belongs to an instance (object). They hold info related to class members associated with a particular instance.
- Global Variables: Global variables are defined at the module level means these exist throughout the program's lifetime and are accessible from any part of it.
Q. What symbol represents a variable in programming?
In programming and mathematics, variables are represented by symbols like x, y, and z or more descriptive names such as temperature or age. In equations or formulas, they represent unknown values. For example, in the equation 2x + 7 = 15, the variable x represents a value that can be solved (here, x = 3).
Q. What is variable type in C++?
In C++, a variable's type defines what kind of data it can store. Common types include:
- int: Stores whole numbers.
- float: Stores decimal (floating-point) numbers.
- char: Stores single characters.
- string: Stores sequences of characters (strings).
- bool: Stores boolean values (true or false).
The type ensures that the compiler allocates the correct amount of memory and enforces type-specific operations.
Q. What is a variable in programming?
A variable in programming is a named storage location in a computer’s memory that holds data. Unlike constants, whose values remain fixed, the value of a variable can change during the execution of a program.
- Variables are crucial for storing dynamic values such as user input, results from calculations, and other data.
- One of the key benefits of using variables is the ability to reuse and manipulate data without hardcoding values, thus making code more efficient and maintainable.
By now, must know everything about variables in C programming and the role they play in your code. Here are a few other articles you must explore:
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Ternary (Conditional) Operator In C Explained With Code Examples
- Recursion In C | Components, Working, Types & More (+Examples)
- Control Statements In C | The Beginner's Guide (With Examples)
- Type Casting In C | Cast Functions, Types & More (+Code Examples)
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment