Swapping Of Two Numbers In C | 5 Easy Ways Explained (+Examples)
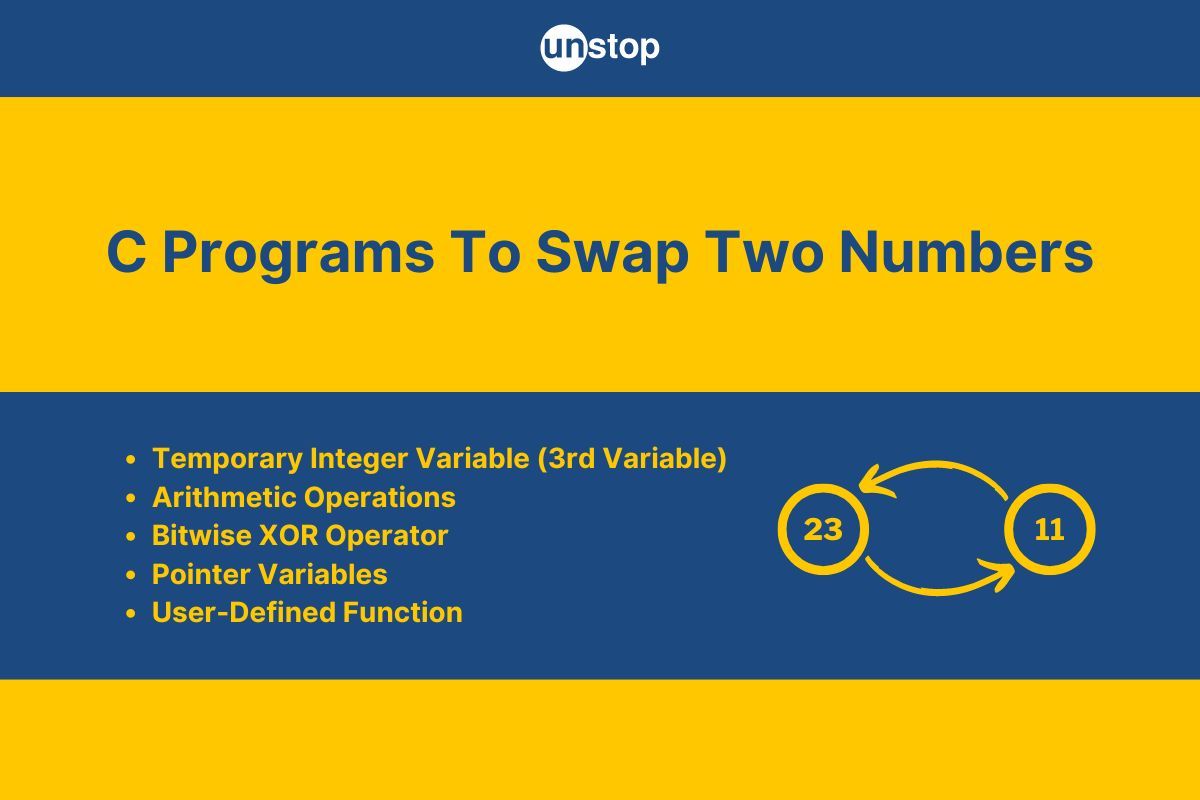
In this article, we will discuss the ways to carry out the swapping of two numbers in C programming language with the help of code examples and explanations. Swapping two numbers is a common operation wherein we exchange the values of two variables.
Say you have two variables, var1 and var2; swapping entails assigning the value of the 1st variable to the 2nd variable, and vice versa. Understanding how to perform such manipulations is important for developing the right programming skills. There are five common ways of swapping two numbers in C programs, including:
- Temporary Integer Variable (3rd Variable)
- Arithmetic Operations
- Bitwise XOR Operator
- Pointer Variables and Custom Swap Function
- The memcpy() Function (<string.h> Library)
We will discuss all these methods in proper detail with examples in the section above.
Swapping Of Two Numbers In C Using Temporary Variable
This is the most straightforward and commonly used approach to swap two numbers in C language. The temporary variable method is also referred to as swapping two numbers with a third variable. Here are the general steps to write and run a C program to swap two numbers using this approach:
- Declare variables: We declare three variables, i.e., the 1st variable and the 2nd variable, to hold values for variables to be swapped and the 3rd variable to act as the temporary variable.
- User Input: If you are not taking given values, then prompt the user to provide input for the 1st and 2nd variables and store them accordingly.
- Initialize Temporary & Swap: The next step is to assign the value of 1st variable to the temporary variable.
- Then, assign the value of the 2nd variable to the 1st variable. Here, you have effectively stored the value of the 2nd variable in the 1st.
- Next, assign the value stored in the temp variable (which originally had the value of 1st variable) to 2nd variable.
In this way, you can easily swap two numbers in C programming. Below is a simple C program example illustrating this.
Code Example:
Output:
Before swapping:
num1 = 10 num2 = 20
After swapping:
num1 = 20 num2 = 10
Explanation:
In the simple C code example, we first include the <stdio.h> header file for input/ output operations and then initiate the main() function, which is the program's entry point for execution.
- Inside the main() function, we declare two integer variables, num1 and num2, and assign them values 10 and 20, respectively.
- Then, we use a set of printf() statements to display these values to the console with a string message.
- In the string message, the %d format specifier indicates integer values, and the newline escape sequence shifts the cursor to the next line.
- As mentioned in the code comment, we then declare an integer data type variable temp to act as the temporary variable.
- Next, we assign the value of num1 to the temp variable, followed by assigning the value of the num2 variable to num1. At this point, num1 has the value of the 2nd variable.
- After that, we assign the value stored in the temp variable to num2, effectively swapping the values of num1 with num2.
- Lastly, we again use a set of printf() statements to display the values of the number after swapping.
- Finally, the main() function terminates with a return 0 statement indicating successful execution without any error.
Time Complexity: O(1) — The program runs in constant time regardless of input. The entire program involves simple assignments and print statements, each taking constant time.
Space Complexity: O(1) — The program uses a constant amount of memory for variables regardless of input.
Swapping Program In C Using Arithmetic Operators (Without Using Third Variable)
Arithmetic operators help us perform arithmetic operations on two or more operands. They consist of addition (+), subtraction (-), multiplication (*), modulus (%), etc. operators. The working mechanism of the C program to swap two numbers using arithmetic operators is given below.
This approach involves swapping two variables in C without using a third/ temp variable. Instead, we use the subtraction and addition arithmetic operators to swap the values of two numbers.
- We declare two variables, say, num1 and num2, to store the initial values that will be swapped.
- Then, use the addition operator to add num1 and num2 and subsequently store the sum in num1.
- Next, subtract num2 from the updated num1 and store the result in num2.
- And finally, subtract the updated num2 from the updated num1 and store the result in num1. This effectively swaps the values of the two numbers.
Below is a C program example that illustrates this approach to swapping of two numbers in C language, where the values are taken from the user.
Code Example:
Output:
Enter the first number: 23
Enter the second number: 44
Before swapping: num1 = 23, num2 = 44
After swapping: num1 = 44, num2 = 23
Explanation:
In this C code example-
- We declare two integer variables, num1 and num2, inside the main() function, then use the printf() function to prompt the user to provide input for these variables.
- Then, we use the scanf() function to read the user input and store them in respective variables using the address-of operator (&).
- Next, we perform a set of arithmetic operations on these values to swap the two.
- First, we add the values of num1 and num2 and assign the outcome to variable num1.
- Then, we subtract the value of num2 from the updated value of num1 and assign the outcome to num2. At this point, num2 stores the original value of the num1 variable.
- Again, we subtract num2 from num1, which will generate the initial value of num2, which we then store in the num1 variable.
- After effectively swapping the two numbers, we use a printf() statement to display the updated values. We use the comma operator/separator in between the values.
Time Complexity: O(1) — The program runs in constant time, with no loops or recursive calls, and all operations are simple arithmetic or input/output operations that take O(1) time.
Space Complexity: O(1) — The program uses a fixed amount of memory, for variables independent of input size.
Check out this amazing course to become the best version of the C programmer you can be.
Swapping Of Two Numbers In C Using Bitwise XOR Operator (^)
The bitwise operators work at the bit level of a value by comparing bits of two values and returning boolean values 0 and 1. The XOR operator compares the bits and returns 1 if either of the bits is 1 and 0 otherwise. We can use the bitwise XOR operator for swapping of two numbers in C programming, however it works only for unsigned integer values. Below is a C program sample that illustrates this approach.
Code Example:
Output:
Enter the first number: 54
Enter the second number: 11
Before swapping:
num1 = 54 num2 = 11
After swapping:
num1 = 11 num2 = 54
Explanation:
In this C code sample-
- We declare two integer variables, num1 and num2, inside the main() function and then store user-generated input in them.
- Next, we use a set of printf() statements to display the values before swapping them with a string message.
- Then, we use the bitwise XOR operator thrice to swap the two variable values. In that-
- We first apply the XOR operation on num1 and num2 and store the outcome in the num1 variable.
- Then, we again perform the XOR operation on num1 and num2 and store the outcome in the num2 variable. At this point, num2 will hold the initial value of the num1 variable.
- Again, we perform the XOR operation and store the outcome in the num1 variable, effectively swapping the two values.
- After swapping, we use a set of printf() statements to display the updated values.
Time Complexity: O(1) — The program runs in constant time, regardless of the values of num1 and num2.
Space Complexity: O(1) — The program uses a constant amount of memory, independent of input size.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Swapping Two Numbers In C With Pointer Variables & Function
Pointer variables are special variables that point to other variables or store the memory address/ location of another variable instead of the data/ values. They can be used to indirectly access and manipulate the values stored in the variables, including swapping of two variables in C programming.
We use a third/ temporary variable to carry out swapping, just like in the temporary variable approach. The general process remains the same, with the primary difference being that we use the pointer to the variables instead of the variable names/ identifiers to swap. Also, it is best to enclose the process inside a function. Look at the sample C program below to better understand this concept.
Code Example:
Output:
Original numbers: num1 = 20, num2 = 30
Swapped numbers: num1 = 30, num2 = 20
Explanation:
In this sample C code-
- We define a function called swap() that takes two integer pointers (int* a and int* b) as parameters. Inside this function with pointers-
- We declare an integer variable temp to store the temporary value during the swapping process.
- Then, using the dereference operator (*), we assign the value stored in variable a to the temp variable.
- Next, we assign the value stored in pointer variable b to pointer variable a, effectively initializing a with the initial value of b.
- After that, we assign the initial value of pointer variable a, stored in the temp variable, to the pointer variable b.
- At this point, the values stored in the pointer variables will be swapped.
- In the main() function, we initialize two integer variables, num1 and num2, with the values 23 and 30, respectively.
- We then print these initial values to the console with a string message using the printf() function.
- Next, we call the swap() function, passing references to num1 and num2 as arguments. This passes the memory addresses of the two variables to the swap function, allowing it to modify their values.
- After the function returns the swapped values, we print them to the console once again using the printf() function.
Time Complexity: O(1) — The program executes in constant time, as all operations take O(1) time.
Space Complexity: O(1) — The program uses a constant amount of memory.
The memcpy() & Custome Function To Swap Two Numbers In C
The memcpy() function is a standard library function from the string header file. It copies the data from one memory location to another. We can use this function to swap two numbers in C programs, just like in the temporary variable approach.
Here, we define a variable swapping function that uses memcpy(). The example C program below illustrates this approach.
Code Example:
Output:
Before swap: a = 16, b = 24
After swap: a = 24, b = 16
Explanation:
In the example C code, we first include the <stdio.h> and <string.h> header files for input/ output and memcpy() function, respectively.
- We then define a function called swap() that takes two integer pointers, a and b, as parameters. Inside the function-
- We declare an array of integers, called temp, with the size determined by the sizeof() operator. This will act as the temporary variable for swapping.
- Then, we use the memcpy() function to copy the memory contents of the variable pointed to by a to the temp variable. This copies the data at the memory location rather than the value of the variable itself.
- Next, we use memcpy() again to copy the memory contents from b to a. At this point, the variable a will hold the original value of b.
- Finally, we use memcpy() to copy the memory contents stored in temp (which holds the original value of a) to b, effectively swapping the two values.
- In the main() function, we initialize two integer variables, a and b, with the values 16 and 24, respectively.
- We print these values in the output window before swapping, using the printf() function.
- Next, we call the swap() function, passing the addresses of variables a and b as arguments.
- The function swaps the values, which we then print to the console, as shown above.
Time Complexity: O(1)
Space Complexity: O(1)
Note: The complexity analysis above shows that using the memcpy() function for swapping two integers is efficient in terms of both time and space complexity, with both complexities being constant. However, it's worth noting that in practice, other methods, like using a temporary variable or bitwise XOR, might be more commonly used for swapping integers due to their simplicity and efficiency.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
Swapping of two numbers in C is a common operation that can be performed using many approaches. These include the temporary variable, arithmetic and bitwise operators, pointer variables, and the memcpy() function from the string library. You can choose any of these techniques depending on the resources and specific requirements.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is the swapping of two numbers in C?
Swapping of two numbers in C refers to the process of exchanging the values of two variables, typically integers so that their values are interchanged.
Q. Why do we need to swap two numbers in a C program?
Swapping two numbers is a common programming task often required in various applications, such as sorting algorithms, mathematical operations, and data manipulation, where the values of two variables must be interchanged.
Q. What are the different methods to swap two numbers in C?
There are multiple methods to swap two numbers in C, including using a third variable, using arithmetic operations, and using bitwise XOR operations.
Q. How can we swap two numbers in C without using a third variable?
One common method to swap two numbers in C without using a third variable is by using arithmetic operations, such as addition and subtraction. Another method is using a bitwise XOR operation.
Q. What is the advantage of swapping two numbers without using a third variable?
The advantage of swapping two numbers without using a third variable is that it saves memory space as it avoids the need for an additional variable, which can be useful in situations where memory space is a concern.
Q. Is swapping two numbers in C platform-dependent?
No, swapping two numbers in C using standard C language constructs is platform-independent and can be used on any system that supports the C programming language.
Q. Can swapping two numbers in C result in loss of data or precision?
No, swapping two numbers in C using standard swapping methods, such as using a third variable or arithmetic operations, does not result in any loss of data or precision, as the values are merely exchanged between variables.
Q. Can I use the swapping of two numbers code in my own C programs without any copyright issues?
Yes, the swapping of two numbers in C code is a basic programming logic that is widely used and not subject to copyright issues. However, it's always good practice to acknowledge the source of the code if you refer to any specific implementation from external sources.
This compiles our discussion on the swapping of two numbers in C language. Here are a few other topics you must read:
- 6 Relational Operators In C & Precedence Explained (+Examples)
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
- Tokens In C | A Guide For All Types & Their Uses (With Examples)
- Control Statements In C | The Beginner's Guide (With Examples)
- Nested Loop In C | For, While, Do-While, Break & Continue (+Codes)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment