Library Functions In C | Header Files, Uses & More (+ Examples)
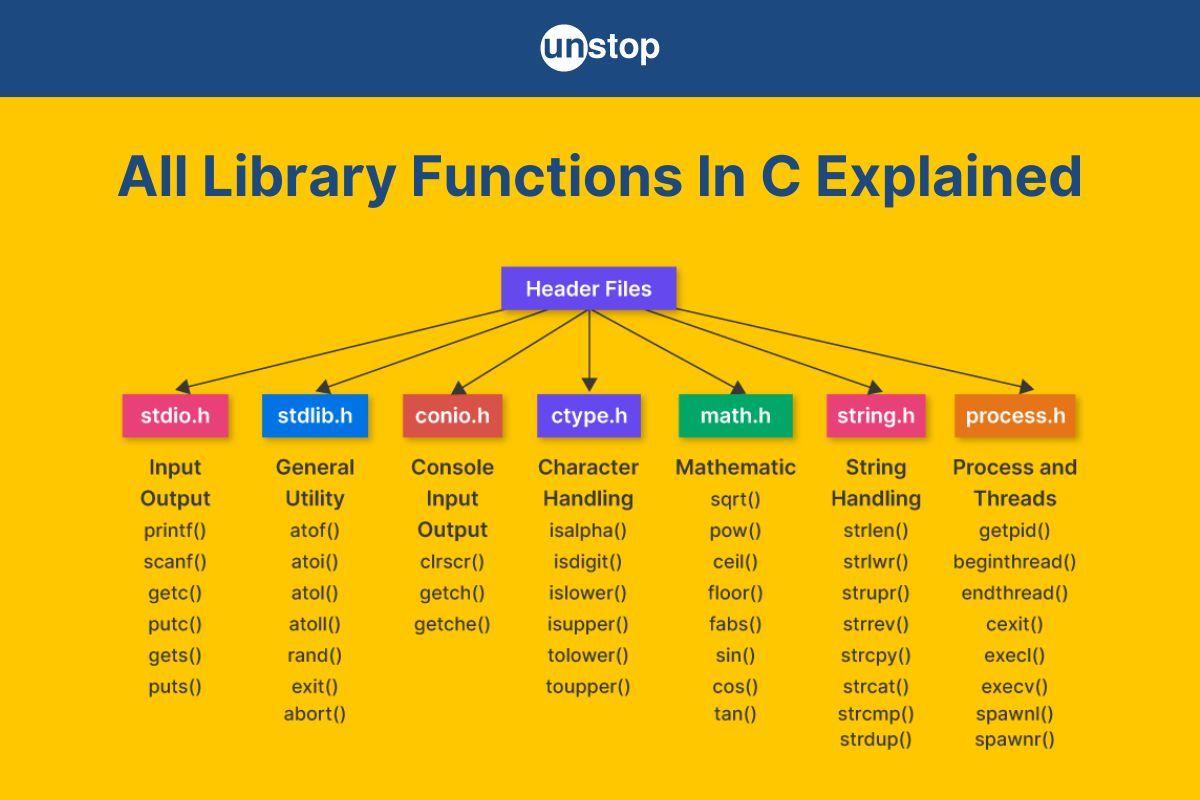
Library functions in C programming language are pre-defined functions(or inbuilt functions) that are provided by the C Standard Library. These functions are stored in header files and offer a convenient way to implement common functionalities without rewriting code from scratch. From mathematical computations to string manipulation, library functions make programming more efficient and error-free.
In this article, we will explore the significance of library functions in C, their various types, and how they enhance productivity. We'll also discuss how to use these functions, the importance of including the correct header files, and some commonly used standard libraries.
What Are User-Defined Functions In C?
User-defined functions in C programming are functions created by the programmer to perform specific tasks or calculations within a program.
- These functions are not part of the language's standard library but are custom-written to modularize and organize code and promote code reusability.
- A user-defined function has the following elements: a name, a list of parameters, a return type (which can be void for functions that don't return a value), and a function body.
- By using user-defined functions, we can break down complex problems into smaller, manageable pieces, making the code more readable and maintainable.
These are in contrast to the library functions, which are the focus of this article.
What Are Library Functions In C?
The C Standard Library is a collection of header files and routines that provide a set of common functions and macros used in C programming. It is designed to provide a consistent and portable interface for performing various tasks, such as input/output operations, string manipulation, memory management, and mathematical calculations.
- The functions and macros in the standard library are defined by the C standard and are implemented by all conforming compilers in C, making them universally available across different platforms.
- Library functions in C are pre-written functions provided by this standard library or other libraries that we can use in our programs.
These functions are designed to perform common tasks, making programming easier and more efficient. Instead of writing these functions from scratch, we can simply include the appropriate library and call the functions as needed in our programs.
Here are some of the most commonly used standard library functions in C:
Category | Functions | Example |
---|---|---|
Input/Output Functions | printf(), scanf() |
#include <stdio.h> |
String Handling Functions | strlen() |
#include <string.h> |
Memory Management Functions | malloc(), free() |
#include <stdio.h> |
Header Files For Library Functions In C Programming
Header files in C programming are files that contain definition of functions, macros, and types, as well as definitions of constants. They serve as an interface between the code written by the programmer and the library functions provided by the C Standard Library or other external libraries.
By including the appropriate header files in a C program, we inform the compiler about the existence and details of the functions and other entities that will be used in the code.
Why Do We Use Header Files?
Header files are essential for several reasons, including:
- Function Declaration: They provide the implementation of library functions, allowing the compiler to check for correct usage, such as the number and types of arguments and return types.
- Encapsulation:Header files encapsulate the interface of a module or library. They provide a clear separation between what a module offers (the interface) and how it is implemented (the source code).
- Maintainability: When you need to make changes to a module's interface, you only need to update the header file, not every source file that uses the module. This reduces the risk of introducing errors during code changes and simplifies maintenance.
- Code Reusability: They allow for the separation of interface and implementation, making it possible to include common declarations in multiple source files without duplication.
- Error Checking: Header files help catch errors in your code early in the development process. When a header file is included in a source file, the compiler ensures that the functions and data structures are used correctly based on the declarations provided in the header. This can prevent common programming mistakes.
- Modularity: By using header files, we can organize code into separate modules, making it easier to manage and maintain.
- Readability: Header files improve code readability by providing a concise summary of the functions and data structures available in a module. Seasoned programmers can quickly reference the header file to understand how to use the module's features without delving into the source code.
- Consistency: They help ensure consistency in the declarations and definitions used across different parts of a program.
List Of Commonly Used Header Files In C
Here is a list of commonly used header files in C along with the purpose:
Header File | Purpose/Use | Where to Declare |
---|---|---|
<stdio.h> | Input and output functions (e.g., printf, scanf) | In source files where input/output operations are needed. |
<stdlib.h> | General utility functions, including memory management (e.g., malloc, free), program control, and conversions | In source files where dynamic memory allocation and other utility functions are required. |
<string.h> | String manipulation functions (e.g., strlen, strcpy) | In source files where string operations are performed. |
<math.h> | Mathematical functions (e.g., sqrt, sin, cos) | In source files where mathematical calculations are used. |
<time.h> | Date and time functions (e.g., time, ctime) | In source files where date and time-related operations are performed. |
<ctype.h> | Character classification and conversion functions (e.g., isalpha, toupper) | In source files where character manipulation is needed. |
<stdbool.h> | Defines bool, true, and false for boolean operations | In source files using boolean values. |
<assert.h> | Macros for debugging and runtime assertion testing | In source files to include assertions for debugging purposes. |
<stddef.h> | Defines several standard types and macros (e.g., NULL, size_t, ptrdiff_t) | In source files where these types and macros are used. |
<limits.h> | Defines implementation-specific limits for different data types | In source files requiring knowledge of data type limits. |
<float.h> | Defines implementation-specific limits for floating-point types | In source files requiring knowledge of floating-point type limits. |
<errno.h> | Defines error codes and provides error handling mechanisms | In source files where error handling is necessary. |
<complex.h> | Complex number support (e.g., complex, cabs, cexp) | Typically at the top of source files where complex number operations are performed. |
<setjmp.h> | Non-local jump functions using setjmp() and longjmp(). | At the beginning of source files where non-local jumps are used. |
<locale.h> | Localization support for internationalization (e.g., setlocale, localeconv) | Typically at the top of source files where localization is implemented. |
<stdarg.h> | Handling of variable-length argument lists (variadic functions) | Inside a function that uses variadic arguments or at the top of the file if used multiple times. |
<wchar.h> | Wide character and string handling (e.g., wprintf, wcslen) | Typically at the top of source files where wide characters are used. |
<graphics.h> | Graphics and drawing (Non-standard header, specific to certain compilers/libraries) | At the top of source files for using graphics functions, depending on the specific graphics library used. |
In practice, we include a header file using the #include preprocessor directive at the beginning of a C source file, like this:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
This inclusion ensures that the compiler is aware of the library functions we intend to use, providing necessary declarations and definitions.
Implementation Of Library Functions In C
In this section, we’ll explore the implementation of several commonly used library functions in C, along with code examples to enhance our understanding.
The <stdio.h> Header File & Its Library Functions In C
The <stdio.h> header file provides functionalities for input and output operations in C. It includes functions for reading from and writing to standard input and output, file handling, and formatted input and output. This header is essential for performing basic and advanced I/O operations.
Rules and Regulations:
- Include this header when performing any form of input or output operations in C.
- Standard I/O functions typically interact with files or streams. The standard streams stdin, stdout, and stderr represent standard input, output, and error, respectively.
- When working with files, always check if the file operations are successful and close files using fclose() to avoid resource leaks.
Commonly Used Library Functions In C:
Function | Description |
---|---|
printf() |
Print formatted data to the console. |
scanf() |
Read formatted data from the console input. |
fprintf() |
Print formatted data to a file. |
fscanf() |
Read formatted data from a file. |
fopen() |
Open a file for reading or writing. |
fclose() |
Close a file. |
fread() |
Read data from a file. |
fwrite() |
Write data to a file. |
remove() |
Delete a file. |
rename() |
Rename a file. |
The printf() Library Function In C Example
This function is used to print formatted output to the standard output stream (stdout).
Syntax:
printf(format_string, arg1, arg2, ...);
Here,
- printf(): The function name, used for formatted output to the standard output stream.
- format_string: A string that specifies how subsequent arguments (arg1, arg2, etc.) should be formatted. It includes format specifiers (e.g., %d, %s) that define the type and format of the arguments.
- arg1, arg2, ...: The arguments to be formatted and printed according to the format_string.
Code:
Output:
Unstop
Explanation:
In the above code example-
- We start by including the <stdio.h> header file to use the printf() library function in C.
- In the main() function, we print "Unstop" to the console using printf() and then return 0 to indicate that the program executed successfully.
The <math.h> Header File & Its Library Functions In C
The <math.h> header file provides a set of mathematical functions for performing common arithematic operations such as trigonometric calculations, exponential and logarithmic functions, power and square root operations, and other mathematical utilities. These functions operate on float, double, and long double types.
Rules and Regulations:
- Include this header when performing mathematical computations that require functions beyond basic arithmetic operations.
- The functions in <math.h> are available in the standard library, and they generally operate on floating-point types.
- Be mindful of the domain and range of these functions to avoid invalid results or domain errors (e.g., passing a negative number to sqrt).
Commonly Used Library Functions In C:
Function |
Description |
---|---|
sin(x) |
Sine function |
cos(x) |
Cosine function |
tan(x) |
Tangent function |
log(x) |
Natural logarithm |
exp(x) |
Exponential function |
sqrt(x) |
Square root |
pow(x, y) |
Power (x raised to the power of y) |
The sqrt() Library Function In C Example
This function is primarily used to calculate the square root of a given number.
Syntax:
double sqrt(double x);
Here,
- double: The return type, indicating that the function returns a double-precision floating-point number.
- sqrt: The function name, which calculates the square root of a number.
- double x: The parameter, where x is the number for which the square root is to be calculated.
Code:
Output:
Square root of 16.00 is 4.00
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <math.h> for the sqrt() library functions in C.
- We then calculate the square root of 16.0 using the sqrt() function, store it in the result, and print the result with a formatted message using the printf() function.
The <stdlib.h> Header File & Its Library Functions In C
The <stdlib.h> header file provides functions for performing various utility operations such as memory allocation, process control, conversions, and random number generation. It includes C library functions for managing dynamic memory, converting strings to numeric values, and handling program termination.
Rules and Regulations:
- Include this header when performing tasks related to dynamic memory management, process control, or conversions between strings and numeric values.
- Be cautious with memory allocation library functions in C to avoid memory leaks and ensure proper deallocation of allocated memory.
- Functions that allocate memory (e.g., malloc, calloc) should be paired with corresponding functions to free memory (e.g., free).
Commonly Used Library Functions In C:
Function/Constant | Description |
---|---|
malloc(size_t size) |
Allocate dynamic memory |
free(void* ptr) |
Deallocate dynamically allocated memory |
exit(int status) |
Terminate the program |
atoi(const char* str) |
Convert a string to an integer |
rand() |
Generate a random integer |
srand(unsigned int seed) |
Seed the random number generator |
system(const char* command) |
Execute a shell command |
The atoi() Library Function In C Example
This function is used to convert a string representing an integer into an actual integer value.
Syntax:
int atoi(const char* str);
Here,
- int: The return type, indicating that the function returns an integer.
- atoi: The function name, which converts a string to an integer.
- const char str*: The pointer to the string that will be converted to an integer. The string should represent a number in decimal format.
Code:
Output:
Converted integer: 42
Explanation:
In the above code example-
- We include <stdio.h> for printf() and <stdlib.h> for atoi() library functions in C repsectively.
- We then convert the string "42" to an integer using atoi() function, store it in the integer variable num, and print the converted integer using the printf() function.
The <string.h> Header File & Its Library Functions In C
The <string.h> header file provides functions for manipulating strings and arrays of characters. It includes functions for copying, concatenating, comparing, and searching within strings. These functions operate on null-terminated strings, which are arrays of characters terminated by a null character ('\0').
Rules and Regulations:
- Include this header when performing operations on strings or character arrays.
- Functions in <string.h> expect null-terminated strings, so proper null termination is crucial for correct operation.
- Be cautious with buffer sizes and memory allocation to avoid buffer overflows and ensure that functions do not read or write outside the bounds of allocated memory.
Commonly Used Library Functions In C:
Function |
Description |
---|---|
strcpy(char* dest, const char* src) |
Copy one string to another |
strcat(char* dest, const char* src) |
Concatenate two strings |
strlen(const char* str) |
Get the length of a string |
strcmp(const char* str1, const char* str2) |
Compare two strings |
strncpy(char* dest, const char* src, size_t n) |
Copy part of a string to another |
strncat(char* dest, const char* src, size_t n) |
Concatenate part of a string to another |
The strcpy() Library Function In C Example
The strcpy() function is used to copy the contents of one string to another.
Syntax:
char* strcpy(char* destination, const char* source);
Here,
- char*: The return type, which is a pointer to a character.
- strcpy: The function name, used to copy a string from one location to another.
- char destination*: The pointer to the destination buffer where the source string will be copied.
- const char source*: The pointer to the source string that will be copied to the destination buffer.
Code:
Output:
Source: Hello, World!
Destination: Hello, World!
Explanation:
In the above code example-
- We include <stdio.h> and <string.h> for printf() and strcpy() library functions in C repsecitvely.
- We have a source string "Hello, World!" and a destination character array destination.
- The strcpy() function is used to copy the content of the source string to the destination array.
- After the copy, both source and destination strings are printed using the printf() and format specifier %s, demonstrating that the content has been successfully copied from source to destination.
The <float.h> Header File & Its Library Functions In C
The <float.h> header file defines macros for the properties of floating-point types (float, double, and long double). It provides information about the limits and precision of these types, such as their minimum and maximum values, precision, and the smallest positive number.
Rules and Regulations:
- Include this header when you need to obtain information about the properties and limits of floating-point types.
- The constants defined in <float.h> are useful for writing portable and precise floating-point code.
- Use these constants to ensure that floating-point operations do not exceed the range or precision limits of the data types.
Commonly Used Library Functions In C:
Constant/Macro |
Description |
---|---|
FLT_DIG |
Decimal digits of precision for float type |
DBL_DIG |
Decimal digits of precision for double type |
LDBL_DIG |
Decimal digits of precision for long double type |
FLT_MAX |
Maximum finite positive value for float |
DBL_MAX |
Maximum finite positive value for double |
LDBL_MAX |
Maximum finite positive value for long double |
FLT_MIN |
Minimum positive normalized value for float |
DBL_MIN |
Minimum positive normalized value for double |
LDBL_MIN |
Minimum positive normalized value for long double |
The FLT_MAX Constant In C Example
This constant is used to represent the maximum finite positive value that can be represented using the float data type.
Syntax: No specific syntax is required, as it is a constant.
Code:
Output:
Maximum finite positive value for float: 3.40282e+38
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <float.h> for the FLT_MAX constant.
- We then print the maximum finite positive value that a float can hold using printf() and FLT_MAX constant.
The <time.h> Header File & Its Library Functions In C
The <time.h> header file provides functions and types for manipulating and measuring time. It includes functions for getting the current time, measuring intervals, and formatting time values. This header is useful for time-related operations, such as timestamping events or implementing delays.
Rules and Regulations:
- Include this header when you need to work with time and date functions in C programs.
- Functions and types in <time.h> operate on time values and often use the time_t type, which is typically an alias for an integer type that represents the number of seconds since the Epoch (January 1, 1970).
- Functions for time formatting and manipulation require careful handling to ensure correct date and time representation.
Commonly Used Library Functions In C:
Function/Data Type |
Description |
---|---|
time_t |
Data type for representing time in seconds since the epoch (usually January 1, 1970) |
struct tm |
Data structure for representing broken-down time (year, month, day, etc.) |
time() |
Get the current time as a time_t value |
ctime() |
Convert a time_t value to a string in a human-readable format |
gmtime() |
Convert a time_t value to a struct tm in UTC (Coordinated Universal Time) |
localtime() |
Convert a time_t value to a struct tm in the local time zone |
strftime() |
Format a struct tm as a string based on a format string |
difftime() |
Calculate the difference between two time_t values in seconds |
The time() Library Function In C Example
This function is used to retrieve the current time as a time_t value representing the number of seconds elapsed since the epoch.
Syntax:
time_t time(time_t* timer);
Here,
- time_t: The return type, which is a data type representing time (usually as the number of seconds since the Unix epoch, January 1, 1970).
- time: The function name, which returns the current calendar time.
- time_t timer*: An optional pointer to a time_t object where the function can store the current time. If this pointer is NULL, the function does not store the time.
Code:
Output:
Current time: 1632284235 seconds since the epoch
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <time.h> for handling time() library functions in C.
- We then use the time() function to get the current time in seconds since the epoch (January 1, 1970) and print the obtained value using the printf() function.
The <assert.h> Header File & Its Library Functions In C
The <assert.h> header file defines the assert macro, which is used to perform runtime checks of conditions in C programs. If the condition evaluated by assert is false, the program prints an error message and terminates. This is useful for debugging and validating assumptions made by the code.
Rules and Regulations:
- Include this header when you want to use assertions to enforce assumptions and invariants in your program.
- Assertions are primarily used for debugging and should not replace proper error handling in production code.
- If the macro NDEBUG is defined before including <assert.h>, the assert macro is disabled, and no code is generated for it.
- Use assertions to catch programming errors, not to handle run-time errors that can be anticipated and managed.
Commonly Used Library Functions In C:
Macro |
Description |
---|---|
assert(expr) |
Evaluates expr. If it's false, it generates a diagnostic message and terminates the program. Typically used for debugging purposes. |
The assert() Library Function In C Example
This macro is used to check if a condition is true during program execution. If the condition is false, it triggers a runtime error.
Syntax:
assert(expression);
Here,
- assert: The macro name used for debugging purposes.
- expression: The condition to be tested, which should be an expression that evaluates to true or false.
Code:
Output:
Result: 2
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <assert.h> for the assert() library function in C.
- Next, we check that y is not zero using assert() before performing the division to prevent a divide-by-zero error.
- We then compute the result of x divided by y and print it using the printf() function.
The <complex.h> Header File & Its Library Functions In C
The <complex.h> header file provides functions and macros for complex number arithmetic. It defines a complex number type and a set of functions for performing standard mathematical operations on complex numbers, such as addition, subtraction, multiplication, division, and various complex-specific operations like obtaining the magnitude or phase.
Rules and Regulations:
- Include this header when working with complex numbers in C programs.
- The complex numbers are represented using the complex keyword, and there are several macros and functions available for operations on these numbers.
- Complex numbers are stored as pairs of double values representing the real and imaginary parts.
- Functions in <complex.h> typically return results of type double complex.
Commonly Used Library Functions In C:
Function/Macro |
Description |
---|---|
cabs() |
Calculate the absolute value of a complex number. |
cimag() |
Get the imaginary part of a complex number. |
creal() |
Get the real part of a complex number. |
conj() |
Calculate the complex conjugate of a complex number. |
cexp() |
Calculate the exponential of a complex number. |
cpow() |
Raise a complex number to a power. |
csin(), ccos() |
Calculate the sine and cosine of a complex number. |
ctan() |
Calculate the tangent of a complex number. |
The cabs() Library Function In C Example
This function is used to calculate the magnitude (absolute value) of a complex number.
Syntax:
double cabs(double complex z);
Here,
- double: The return type, indicating that the function returns a double-precision floating-point number.
- cabs: The function name, which computes the absolute value (or magnitude) of a complex number.
- double complex z: The parameter, where z is a complex number of type double complex.
Code:
Output:
Absolute value of z = 5.000000
Explanation:
In the above code example-
- We start by including <complex.h> for complex number function like cabs() and <stdio.h> for printf() library function in C.
- Next we create a complex number z with real part as 3.0 and imaginary part as 4.0.
- We then compute the absolute value of z using cabs() function and print the result to the console.
The <setjmp.h> Header File & Its Library Functions In C
The <setjmp.h> header file is used to work with non-local jumps within a program. It provides functions and macros that enable you to perform longjmp and setjmp operations, and non-local control transfers.
Rules and Regulations:
- Include this header when using library functions in C related to non-local jumps such as setjmp and longjmp.
- The setjmp should be called before longjmp to save the program's state.
- Ensure that longjmp is used cautiously to avoid undefined behavior, such as jumping over variable declarations or into code where stack frames may be altered.
Commonly Used Library Functions In C:
Function/Macro |
Description |
---|---|
setjmp() |
Saves the environment for a non-local jump. |
longjmp() |
Jumps back to the point saved by setjmp. |
The setjmp() Library Function In C Example
This library function saves the current environment for later use by longjmp and returns zero initially.
Syntax:
int setjmp(jmp_buf env);
Here,
- int: The return type, indicating that the function returns an integer.
- setjmp: The function name, used to save the current execution context.
- jmp_buf env: A buffer where the function saves the current context, allowing longjmp to later restore this context.
Code:
Output:
Calling function
In function
Returned from longjmp
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <setjmp.h> for setjmp() and longjmp() library function in C.
- Next, we use setjmp() function to save the current execution state in env.
- When longjmp() is called in function, it jumps back to the setjmp point, returning a non-zero value and causing the program to print "Returned from longjmp."
- If longjmp is not executed, the program prints "Calling function" and then calls function.
The <locale.h> Header File & Its Library Functions In C
The <locale.h> header file is used for handling localization and internationalization in programs. It provides functions and macros for dealing with various aspects of local settings, such as character encoding, date and time formats, and message translations.
Rules and Regulations:
- Include this header when using locale-related library functions such as setlocale, localeconv, and lc_collate.
- Locale settings affect various aspects of program behavior, including string comparison and numeric formatting.
- Ensure to use setlocale to set the desired locale before performing locale-specific operations.
Commonly Used Library Functions In C:
Function/Macro |
Description |
---|---|
setlocale() |
Sets or queries the program's locale. |
localeconv() |
Returns information about numeric formatting. |
iconv() |
Converts characters between different encodings. |
nl_langinfo() |
Retrieves specific information about the locale. |
newlocale() |
Creates a new locale object. |
freelocale() |
Frees resources associated with a locale object. |
uselocale() |
Sets the locale for thread-local storage. |
The setlocale() Library Function In C Example
This library function is used to set or query the program's locale. It allows you to configure how various locale categories behave, such as character encoding or date and time formatting.
Syntax:
char *setlocale(int category, const char *locale);
Here,
- char *: The return type, which is a pointer to a character string.
- setlocale: The function name, used to set or query the program's locale.
- int category: The category of locale settings to be changed or queried, such as LC_ALL, LC_TIME, etc.
- const char *locale: A string representing the locale name. If set to NULL(null pointer), the current locale setting for the specified category is returned.
Code:
Output:
Current locale for LC_TIME: C
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <locale.h> for setlocale() library functions in C.
- Next, we set the locale for date and time formatting to U.S. English using setlocale() function.
- We then query and print the current locale setting for LC_TIME using printf() to confirm the change.
The <stdarg.h> Header File & Its Library Functions In C
The <stdarg.h> header file is used for working with functions that accept a variable number of arguments. It provides a mechanism for functions to receive a variable number of arguments, making it possible to write more flexible and versatile functions.
Rules and Regulations:
- Include this header when you need to create functions that accept a variable number of arguments.
- Use the va_start, va_arg, and va_end macros to handle variable arguments safely and correctly.
- Ensure that the va_end macro is called before the function returns to clean up the argument list.
Commonly Used Library Functions In C:
Macro/Function |
Description |
---|---|
va_list |
Type for declaring a variable argument list. |
va_start |
Initializes the va_list for variable argument access. |
va_arg |
Retrieves the next argument from the va_list. |
va_copy |
Makes a copy of a va_list. |
va_end |
Cleans up and finalizes the va_list. |
The va_arg() Library Function In C Example
This macro retrieves the next argument from the va_list. It requires two arguments: the va_list and the type of the argument to retrieve.
Syntax:
type va_arg(va_list ap, type);
Here,
- type: It specifies the type of the next argument to be retrieved from the variable argument list.
- va_arg: The macro used to access the next argument in a variable argument list.
- va_list ap: A variable of type va_list that holds the information needed to retrieve additional arguments. This is typically initialized using va_start.
Code:
Output:
Sum = 100
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <stdarg.h> for handling variable arguments like va_arg, va_list etc.
- Next, we define a sum() function that calculates the sum of a variable number of integers.
- Using va_start, we initialize va_list to process the arguments, then iterate through them with va_arg to add each integer to total.
- After processing, we use va_end to clean up. In main() function, we call sum() with four integers, print the result using printf(), and return 0 to indicate successful execution.
The <errno.h> Header File & Its Library Functions In C
The <errno.h> header file is used for error handling and provides a standard mechanism to access and manage error codes in C programs. It defines the errno variable, which stores error codes for various library and system functions.
Rules and Regulations:
- Include this header when handling errors from system or library functions that set the errno variable.
- The errno variable is an external integer variable that is set to a specific error code when a function fails.
- Always check errno after a function fails and ensure it is not used before setting it to a valid value.
- After handling an error, you can clear errno by setting it to 0.
Commonly Used Library Functions In C:
Macro/Function |
Description |
---|---|
errno |
A global variable that stores the error code. |
perror() |
Prints an error message corresponding to errno. |
strerror() |
Returns a string describing the error code. |
errno.h Constants |
Constants representing common error codes. |
The perror() Library Function In C Example
This library function is used to print an error message corresponding to the value of errno. It helps in diagnosing errors by providing a human-readable description of the error.
Syntax:
void perror(const char *s);
Here,
- void: The return type, indicating that the function does not return a value.
- perror(): The function name, which prints a description of the last error that occurred.
- const char *s: A string that is printed before the error message. It is a constant pointer to a character string.
Code:
Output:
Error opening file: No such file or directory
Error code: 2
Explanation:
In the above code example-
- We start by including <stdio.h> for file operations, <errno.h> for perror() library function, and <string.h> for error message handling.
- We then attempt to open a file named "nonexistent.txt" in read mode.
- The fopen() function returns a file pointer (file), or NULL if the file can't be opened.
- If the file cannot be opened (file == NULL), we use perror() function to print an error message and display the error code stored in errno.
The <graphics.h> Library Header File & Its Library Functions In C
The <graphics.h> header file provides functions for graphical operations, particularly for creating graphics-based applications in a DOS environment. It includes library functions for drawing shapes, setting colors, and managing graphics modes.
Rules and Regulations:
- Include this header when using graphics-related library functions in C programs.
- The <graphics.h> library is typically available in Turbo C/C++ compilers and is not part of the standard C library.
- It is primarily used in older, DOS-based systems and is not supported in modern compilers.
- To use <graphics.h>, the initgraph function must be called to initialize the graphics mode.
Commonly Used Library Functions In C:
Function |
Description |
---|---|
initgraph() |
Initializes the graphics system. |
closegraph() |
Closes the graphics system. |
line() |
Draws a line between two points. |
circle() |
Draws a circle with a specified radius and center. |
rectangle() |
Draws a rectangle defined by its top-left and bottom-right corners. |
putpixel() |
Sets the color of a single pixel at a given position. |
outtext() |
Displays text at a specified position. |
getch() |
Waits for a key press and returns the ASCII code of the key. |
mousex(), mousey() |
Retrieves the current mouse cursor position. |
The line() Library Function In C Example
This library function is used to draw a straight line between two specified points on the graphics screen.
Syntax:
line(int x1, int y1, int x2, int y2);
Here,
- line(): The function name, which is used to draw a line on a graphical screen.
- int x1, int y1: The coordinates of the starting point of the line.
- int x2, int y2: The coordinates of the ending point of the line.
Code:
Output:
A graphics window will open, displaying a line from (100, 100) to (300, 200).
Explanation:
In the above code example-
- We start by including <graphics.h> for graphics functions like line().
- Next, we initialize the graphics system using initgraph() with gd and gm to detect and set the graphics mode.
- We then draw a line from coordinates (100, 100) to (300, 200) using the line() function.
- We next wait for a key press from the user with getch() function and close the graphics system with closegraph() function.
The <wchar.h> Library Header File & Its Library Functions In C
The <wchar.h> header file provides functions and types for handling wide characters and wide character strings. Wide characters (wchar_t) are used to represent extended character sets that cannot be accommodated by regular character types, such as international character sets.
Rules and Regulations:
- Include this header when working with wide characters or wide character strings in C programs.
- Functions in <wchar.h> are analogous to those in <string.h>, but they operate on wide character types.
- Wide characters are typically used for internationalization, allowing for the representation of a broader range of characters.
- Properly manage memory when working with wide character strings, similar to regular strings.
Commonly Used Library Functions In C:
Macro/Function |
Description |
---|---|
wprintf() |
Print wide character data to the console. |
wscanf() |
Read wide character data from the console. |
wcslen() |
Get the length of a wide character string. |
wcscpy() |
Copy one wide character string to another. |
wcsncpy() |
Copy a specified number of characters from one wide character string to another. |
wcscat() |
Concatenate two wide character strings. |
wcstok() |
Tokenize a wide character string. |
wcschr(), wcsrchr() |
Locate a wide character in a string. |
The wprintf() Library Function In C Example
This library function prints wide character data to the console or a stream. It is similar to printf(), but it deals with wide character strings and formats.
Syntax:
int wprintf(const wchar_t *format, ...);
Here,
- int: The return type, indicating that the function returns an integer.
- wprintf(): The function name, which is used for formatted wide-character output.
- const wchar_t *format: The format string, specifying how to format the output. It is a constant pointer to a wide character string.
Code:
Output:
My name is Jai, and I am 30 years old.
Explanation:
In the above code example-
- We start by including <stdio.h> for standard I/O functions and <wchar.h> for wide character support like wprint() library function in C.
- Next , we define a wide character array name with the value "Jai" and an integer age with the value 30.
- We then use wprintf() function to print the name and age in a wide-character string format.
The <ctype.h > Header File & Its Library Functions In C
The <ctype.h> header file provides functions for testing and mapping characters. It includes functions to classify and transform individual characters, such as checking if a character is a letter, digit, or whitespace, and converting characters to uppercase or lowercase.
Rules and Regulations:
- Include this header when working with character classification or conversion in C programs.
- The functions typically take and return int values, which represent unsigned char values or EOF.
- Properly handle input values to avoid undefined behavior, especially with signed characters that may have negative values.
Commonly Used Library Functions In C:
Function/Macro |
Description |
---|---|
isalpha() |
Check if a character is alphabetic. |
isdigit() |
Check if a character is a digit. |
islower() |
Check if a character is a lowercase letter. |
isupper() |
Check if a character is an uppercase letter. |
isalnum() |
Check if a character is alphanumeric. |
isspace() |
Check if a character is a whitespace character. |
tolower() |
Convert a character to lowercase. |
toupper() |
Convert a character to uppercase. |
The isalpha() Library Function In C Example
This library function is used to check if a character is an alphabetic character (either uppercase or lowercase).
Syntax:
int isalpha(int c);
Here,
- int: It is the return type, indicating that the function returns an integer.
- isalpha(): It represents the function name, which checks if the given character is an alphabetic letter.
- int c: The parameter, where c is the character (as an integer value) to be checked.
Code:
Output:
A is an alphabetic character.
Explanation:
In the above code example-
- We start by including <stdio.h> for printf() and <ctype.h> for isalpha() library functions in C.
- Next, we define a character with the value 'A'.
- We then use isalpha() function to check if character is an alphabetic character. If it is, we print that it is an alphabetic character using printf(); otherwise, we print that it is not.
Advantages Of Using Library Functions In C
The C standard library provides a set of built-in functions and utilities that can significantly simplify and enhance the development of C programs. Here are some advantages of using these library functions in C:
- Portability: Library functions in C are part of the C standard, which means they are available on virtually all C compilers and platforms. This ensures that code using these functions can be easily ported and run on different systems without modification.
- Code Reusability: Library functions save time and effort by providing pre-written, well-tested code for common tasks. Developers can reuse these functions instead of writing the same code from scratch.
#include <math.h>
int main() {
double x = 4.0;
double result = sqrt(x);
return 0;
}
- Efficiency: Library functions in C are typically highly optimized and written in C or assembly language, making them efficient and fast. Using library functions often leads to more efficient code than custom implementations.
#include <stdlib.h>
int main() {
int* arr = (int*)malloc(10 * sizeof(int));
// Memory allocation done efficiently by malloc.
free(arr); // Efficient memory deallocation.
return 0;
}
- Standardization: C library functions adhere to the C standard, which ensures consistency and compatibility across different systems and compilers. This standardization reduces the likelihood of subtle bugs caused by platform-specific behavior.
- Safety and Reliability: Many C library functions perform boundary checks and error handling, making them safer to use compared to custom implementations. This reduces the risk of memory leaks, buffer overflows, and other common programming errors.
#include <stdio.h>
int main() {
FILE* file = fopen("example.txt", "r");
if (file != NULL) {
// Read from the file safely with error handling.
fclose(file); // Properly close the file.
}
return 0;
}
- Consistency: Using library functions in C programs ensures that code follows established conventions and coding styles. This makes the codebase more consistent and easier to maintain, especially in collaborative projects.
- Documentation: C library functions come with comprehensive documentation, making it easier for developers to understand how to use them. Standardized documentation helps in quickly learning and using these functions effectively.
#include <stdlib.h>
int main() {
// Refer to official documentation for details on functions like malloc and free.
return 0;
}
List Of Library Functions In C For All Header Files
In this section, we have created comprehensive lists of library functions in C, grouped as per the respective header files. The prototype and short description provided along will help you quickly grasp the meaning and purpose of these functions.
The <stdio.h> Library Functions In C
Function |
Prototype |
Description |
---|---|---|
printf |
int printf(const char *format, ...); |
Writes formatted output to the standard output (usually the console). |
scanf |
int scanf(const char *format, ...); |
Reads formatted input from the standard input (usually the keyboard). |
fprintf |
int fprintf(FILE *stream, const char *format, ...); |
Writes formatted output to the specified file stream. |
fscanf |
int fscanf(FILE *stream, const char *format, ...); |
Reads formatted input from the specified file stream. |
sprintf |
int sprintf(char *str, const char *format, ...); |
Writes formatted output to a character array. |
snprintf |
int snprintf(char *str, size_t size, const char *format, ...); |
Writes formatted output to a character array with a specified size limit. |
fopen |
FILE *fopen(const char *filename, const char *mode); |
Opens a file with the specified mode (e.g., "r" for read, "w" for write). |
fclose |
int fclose(FILE *stream); |
Closes the specified file stream. |
fread |
size_t fread(void *ptr, size_t size, size_t count, FILE *stream); |
Reads binary data from a file stream into a buffer. |
fwrite |
size_t fwrite(const void *ptr, size_t size, size_t count, FILE *stream); |
Writes binary data from a buffer to a file stream. |
fseek |
int fseek(FILE *stream, long int offset, int whence); |
Sets the file position indicator for the specified file stream. |
ftell |
long int ftell(FILE *stream); |
Returns the current position of file indicator for the specified file stream. |
fflush |
int fflush(FILE *stream); |
Flushes the output buffer of the specified file stream. |
getchar |
int getchar(void); |
Reads a character from the standard input. |
putchar |
int putchar(int character); |
Writes a character to the standard output. |
fgets |
char *fgets(char *str, int size, FILE *stream); |
Reads a line from a file stream into a string. |
fputs |
int fputs(const char *str, FILE *stream); |
Writes a string to a file stream. |
remove |
int remove(const char *filename); |
Deletes a file with the specified name. |
rename |
int rename(const char *oldname, const char *newname); |
Renames a file from oldname to newname. |
perror |
void perror(const char *str); |
Prints an error message to the standard error stream with a user-supplied prefix. |
tmpfile |
FILE *tmpfile(void); |
Creates a temporary binary file. |
tmpnam |
char *tmpnam(char *str); |
Generates a unique temporary filename. |
setbuf |
void setbuf(FILE *stream, char *buffer); |
Sets the buffer for a file stream (used for stream buffering). |
setvbuf |
int setvbuf(FILE *stream, char *buffer, int mode, size_t size); |
Sets the buffer and buffer mode for a file stream. |
The <math.h> Library Functions In C
Function |
Prototype |
Description |
---|---|---|
sqrt |
double sqrt(double x); |
Calculates the square root of the argument x. |
fabs |
double fabs(double x); |
Returns the absolute value (magnitude) of the argument x. |
ceil |
double ceil(double x); |
Rounds up the argument x to the smallest integer not less than x. |
floor |
double floor(double x); |
Rounds down the argument x to the largest integer not greater than x. |
round |
double round(double x); |
Rounds the argument x to the nearest integer, rounding halfway cases to the nearest even number. |
trunc |
double trunc(double x); |
Truncates (removes the fractional part) of the argument x. |
pow |
double pow(double base, double exponent); |
Calculates base raised to the power of exponent. |
exp |
double exp(double x); |
Calculates the exponential value e^x, where e is the base of natural logarithms. |
log |
double log(double x); |
Calculates the natural logarithm of the argument x. |
log10 |
double log10(double x); |
Calculates the base-10 logarithm of the argument x. |
sin |
double sin(double x); |
Calculates the sine of the angle x (in radians). |
cos |
double cos(double x); |
Calculates the cosine of the angle x (in radians). |
tan |
double tan(double x); |
Calculates the tangent of the angle x (in radians). |
asin |
double asin(double x); |
Calculates the arcsine of x, returning a result in radians in the range [-π/2, π/2]. |
acos |
double acos(double x); |
Calculates the arccosine of x, returning a result in radians in the range [0, π]. |
atan |
double atan(double x); |
Calculates the arctangent of x, returning a result in radians in the range [-π/2, π/2]. |
atan2 |
double atan2(double y, double x); |
Calculates the arctangent of the ratio y/x, returning a result in radians in the range [-π, π]. |
sinh |
double sinh(double x); |
Calculates the hyperbolic sine of the argument x. |
cosh |
double cosh(double x); |
Calculates the hyperbolic cosine of the argument x. |
tanh |
double tanh(double x); |
Calculates the hyperbolic tangent of the argument x. |
fmod |
double fmod(double x, double y); |
Calculates the remainder of x divided by y. |
remainder |
double remainder(double x, double y); |
Calculates the remainder of x divided by y. |
fmax |
double fmax(double x, double y); |
Returns the larger of two values, x and y. |
fmin |
double fmin(double x, double y); |
Returns the smaller of two values, x and y. |
isnan |
int isnan(double x); |
Checks if the argument x is NaN (not-a-number). |
isinf |
int isinf(double x); |
Checks if the argument x is infinity. |
The <stdlib.h> Library Functions In C
Function |
Prototype |
Description |
---|---|---|
malloc |
void *malloc(size_t size); |
Allocates a block of memory of the specified size and returns a pointer to the first byte of the block. |
calloc |
void *calloc(size_t num_elements, size_t element_size); |
Allocates a block of memory for an array of num_elements each of element_size bytes and returns a pointer to the first byte of the block. |
realloc |
void *realloc(void *ptr, size_t new_size); |
Changes the size of the memory block pointed to by ptr to new_size bytes. |
free |
void free(void *ptr); |
Deallocates the memory previously allocated by malloc, calloc, or realloc. |
exit |
void exit(int status); |
Terminates the program and returns the status code status to the calling environment (usually the operating system). |
getenv |
char *getenv(const char *name); |
Retrieves the value of the environment variable specified by name. |
system |
int system(const char *command); |
Executes the shell command specified by command and returns the status code of the command. |
rand |
int rand(void); |
Generates a pseudo-random integer in the range [0, RAND_MAX]. You can use srand to seed the random number generator. |
srand |
void srand(unsigned int seed); |
Seeds the random number generator used by rand with the specified seed. |
abs |
int abs(int x); |
Returns the absolute value of the integer x. |
labs |
long int labs(long int x); |
Returns the absolute value of the long integer x. |
atof |
double atof(const char *str); |
Converts a string str to a double-precision floating-point number. |
atoi |
int atoi(const char *str); |
Converts a string str to an integer. |
atol |
long int atol(const char *str); |
Converts a string str to a long integer. |
strtod |
double strtod(const char *str, char **endptr); |
Converts a string str to a double-precision floating-point number and stores a pointer to the first invalid character in endptr. |
strtol |
long int strtol(const char *str, char **endptr, int base); |
Converts a string str to a long integer in the specified base and stores a pointer to the first invalid character in endptr. |
strtoul |
unsigned long int strtoul(const char *str, char **endptr, int base); |
Converts a string str to an unsigned long integer in the specified base and stores a pointer to the first invalid character in endptr. |
The <time.h> Library Functions In C
Function |
Prototype |
Description |
---|---|---|
time |
time_t time(time_t *tloc); |
Returns the current calendar time as a time_t value. If tloc is not NULL, it also stores the current time in the object pointed to by tloc. |
clock |
clock_t clock(void); |
Returns the processor time used by the program since the program started or since the last call to clock. The value is implementation-dependent and may not represent real-time. |
difftime |
double difftime(time_t time1, time_t time2); |
Computes the difference in seconds between time1 and time2. This function is often used to measure the time duration between two time points. |
mktime |
time_t mktime(struct tm *timeptr); |
Converts a tm structure representing a calendar time into a time_t value. This is useful for creating a time_t value from a specific date and time specified in a tm structure. |
asctime |
char *asctime(const struct tm *timeptr); |
Converts a tm structure to a string in the format "Day Month Date HH:MM:SS Year\n" (e.g., "Wed Sep 1 12:00:00 2023\n"). |
ctime |
char *ctime(const time_t *timep); |
Converts a time_t value into a string representation of the local time, in a format similar to that of asctime. |
gmtime |
struct tm *gmtime(const time_t *timep); |
Converts a time_t value into a tm structure representing the UTC (Coordinated Universal Time) time. |
localtime |
struct tm *localtime(const time_t *timep); |
Converts a time_t value into a tm structure representing the local time for the current time zone. |
strftime |
size_t strftime(char *str, size_t maxsize, const char *format, const struct tm *timeptr); |
Formats the information from a tm structure according to the format string and stores it in the character array str. The format string can include various format specifiers for different time elements (e.g., %Y for year, %m for month, %d for day). |
The <string.h> Library Functions In C
Function |
Prototype |
Description |
---|---|---|
strlen |
size_t strlen(const char *str); |
Returns the length of the null-terminated string str, excluding the null character. |
strcpy |
char *strcpy(char *dest, const char *src); |
Copies the null-terminated string src to dest. The destination string must have enough space to accommodate the source string. Returns a pointer to dest. |
strncpy |
char *strncpy(char *dest, const char *src, size_t n); |
Copies at most n characters from src to dest, adding null characters if necessary. The destination string must have enough space to accommodate the specified number of characters. Returns a pointer to dest. |
strcat |
char *strcat(char *dest, const char *src); |
Appends the null-terminated string src to the end of the null-terminated string dest. The destination string must have enough space to accommodate both strings. Returns a pointer to dest. |
strncat |
char *strncat(char *dest, const char *src, size_t n); |
Appends at most n characters from src to the end of dest, adding null characters if necessary. The destination string must have enough space to accommodate the specified number of characters. Returns a pointer to dest. |
strcmp |
int strcmp(const char *str1, const char *str2); |
Compares two null-terminated strings str1 and str2. Returns an integer less than, equal to, or greater than zero depending on whether str1 is less than, equal to, or greater than str2. |
strncmp |
int strncmp(const char *str1, const char *str2, size_t n); |
Compares at most n characters of str1 and str2. Returns an integer less than, equal to, or greater than zero depending on whether str1 is less than, equal to, or greater than str2. |
strchr |
char *strchr(const char *str, int character); |
Searches for the first occurrence of character in the null-terminated string str. Returns a pointer to the first occurrence of the character or NULL if not found. |
strrchr |
char *strrchr(const char *str, int character); |
Searches for the last occurrence of character in the null-terminated string str. Returns a pointer to the last occurrence of the character or NULL if not found. |
strstr |
char *strstr(const char *haystack, const char *needle); |
Searches for the first occurrence of the null-terminated string needle in the null-terminated string haystack. Returns a pointer to the first occurrence of needle in haystack or NULL if not found. |
strtok |
char *strtok(char *str, const char *delimiters); |
Splits the null-terminated string str into tokens based on the characters in delimiters. Returns a pointer to the next token in the string, or NULL if there are no more tokens. |
memset |
void *memset(void *ptr, int value, size_t num); |
Sets the first num bytes of the memory pointed to by ptr to the specified value. Returns a pointer to ptr. |
memcpy |
void *memcpy(void *dest, const void *src, size_t num); |
Copies num bytes from the memory location src to the common location dest. Returns a pointer to dest. |
memcmp |
int memcmp(const void *ptr1, const void *ptr2, size_t num); |
Compares the first num bytes of memory pointed to by ptr1 and ptr2. Returns an integer less than, equal to, or greater than zero depending on whether the memory content is less than, equal to, or greater than the other. |
memmove |
void *memmove(void *dest, const void *src, size_t num); |
Moves num bytes from the memory location src to the memory location dest, even if the memory regions overlap. Returns a pointer to dest. |
The <wchar.h> Library Functions In C
Function | Prototype | Description |
---|---|---|
btowc | wint_t btowc(int c); | Converts a single-byte character c to its wide character representation. Returns WEOF if the argument is not a valid single-byte character or if the value is outside the representable range of wchar_t. |
wctob | int wctob(wint_t c); | Converts a wide character c to its single-byte character representation. If c represents a valid single-byte character, the function returns that character's integer value. Otherwise, it returns EOF. |
mbrlen | size_t mbrlen(const char *s, size_t n, mbstate_t *ps); | Determines the number of bytes needed to complete the next multibyte character in the sequence pointed to by s. The parameter n specifies the maximum number of bytes to examine. Returns the number of bytes needed, or (size_t)-1 if an encoding error occurs. |
mbrtowc | size_t mbrtowc(wchar_t *pwc, const char *s, size_t n, mbstate_t *ps); | Converts the multibyte character sequence pointed to by s into a corresponding wide character and stores it in pwc. The parameter n specifies the maximum number of bytes to examine. Returns the number of bytes used, (size_t)-1 for an encoding error, or (size_t)-2 for an incomplete multibyte character. |
wcrtomb | size_t wcrtomb(char *s, wchar_t wc, mbstate_t *ps); | Converts a wide character wc into a multibyte character sequence and stores it in the character array s. Returns the number of bytes written, or (size_t)-1 for an encoding error. |
mbsinit | int mbsinit(const mbstate_t *ps); | Checks if the conversion state object ps represents the initial state. Returns a nonzero value if it does, or zero if it does not. |
fgetwc | wint_t fgetwc(FILE *stream); | Reads a wide character from the specified file stream stream. Returns the wide character read, or WEOF on end-of-file or error. |
fputwc | wint_t fputwc(wchar_t wc, FILE *stream); | Writes a wide character wc to the specified file stream stream. Returns the wide character written, or WEOF on error. |
getwc | wint_t getwc(FILE *stream); | Reads a wide character from the standard input (usually the keyboard). Equivalent to fgetwc(stdin). Returns the wide character read, or WEOF on end-of-file or error. |
getwchar | wint_t getwchar(void); | Reads a wide character from the standard input (usually the keyboard). Equivalent to fgetwc(stdin). Returns the wide character read, or WEOF on end-of-file or error. |
putwc | wint_t putwc(wchar_t wc, FILE *stream); | Writes a wide character wc to the specified file stream stream. Equivalent to fputwc(wc, stream). Returns the wide character written, or WEOF on error. |
putwchar | wint_t putwchar(wchar_t wc); | Writes a wide character wc to the standard output (usually the console). Equivalent to fputwc(wc, stdout). Returns the wide character written, or WEOF on error. |
ungetwc | wint_t ungetwc(wint_t wc, FILE *stream); | Pushes the wide character wc back into the specified file stream stream for future reading. Returns wc if successful, or WEOF on error. |
The <complex.h> Library Functions In C
Function | Prototype | Description |
---|---|---|
cabs() |
double cabs(double complex z); |
Calculate the magnitude (absolute value) of a complex number. |
cacos() |
double complex cacos(double complex z); |
Calculate the arc cosine of a complex number. |
cacosh() |
double complex cacosh(double complex z); |
Calculate the inverse hyperbolic cosine of a complex number. |
carg() |
double carg(double complex z); |
Calculate the phase angle (argument) of a complex number. |
casin() |
double complex casin(double complex z); |
Calculate the arc sine of a complex number. |
casinh() |
double complex casinh(double complex z); |
Calculate the inverse hyperbolic sine of a complex number. |
catan() |
double complex catan(double complex z); |
Calculate the arc tangent of a complex number. |
catanh() |
double complex catanh(double complex z); |
Calculate the inverse hyperbolic tangent of a complex number. |
ccos() |
double complex ccos(double complex z); |
Calculate the cosine of a complex number. |
ccosh() |
double complex ccosh(double complex z); |
Calculate the hyperbolic cosine of a complex number. |
cexp() |
double complex cexp(double complex z); |
Calculate the exponential of a complex number. |
cimag() |
double cimag(double complex z); |
Get the imaginary part of a complex number. |
clog() |
double complex clog(double complex z); |
Calculate the natural logarithm of a complex number. |
conj() |
double complex conj(double complex z); |
Calculate the complex conjugate of a complex number. |
cproj() |
double complex cproj(double complex z); |
Calculate the projection of a complex number onto the Riemann sphere. |
creal() |
double creal(double complex z); |
Get the real part of a complex number. |
csin() |
double complex csin(double complex z); |
Calculate the sine of a complex number. |
csinh() |
double complex csinh(double complex z); |
Calculate the hyperbolic sine of a complex number. |
csqrt() |
double complex csqrt(double complex z); |
Calculate the square root of a complex number. |
ctan() |
double complex ctan(double complex z); |
Calculate the tangent of a complex number. |
ctanh() |
double complex ctanh(double complex z); |
Calculate the hyperbolic tangent of a complex number. |
The <setjmp.h> Library Functions In C
Function/Macro | Prototype | Description |
---|---|---|
setjmp() |
int setjmp(jmp_buf env); |
Saves the current execution context in env and returns 0. If longjmp is called with env, setjmp returns a non-zero value. |
longjmp() |
void longjmp(jmp_buf env, int val); |
Restores the execution context saved in env and jumps to that context. The value val is returned by setjmp, where val can be any integer (including 0). |
The <locale.h> Library Function In C
Function/Macro | Prototype | Description |
---|---|---|
setlocale() |
char *setlocale(int category, const char *locale); |
Sets the program's locale to the specified locale and returns a pointer to a string describing the new locale setting. If locale is NULL, it returns the current locale setting. |
localeconv() |
struct lconv *localeconv(void); |
Returns a pointer to a structure containing locale-specific information for formatting numbers, such as decimal points, thousands separators, and currency symbols. |
LC_ALL |
- |
A macro representing all locale categories. Used with setlocale() to set the locale for all categories. |
LC_COLLATE |
- |
A macro representing the collation (string comparison) category. Used with setlocale() to set the collation locale. |
LC_CTYPE |
- |
A macro representing the character classification category. Used with setlocale() to set the character classification locale. |
LC_MONETARY |
- |
A macro representing the monetary formatting category. Used with setlocale() to set the monetary locale. |
LC_NUMERIC |
- |
A macro representing the numeric formatting category. Used with setlocale() to set the numeric locale. |
LC_TIME |
- |
A macro representing the date and time formatting category. Used with setlocale() to set the date and time locale. |
struct lconv |
- |
A structure type containing information about numeric and monetary formatting for a locale. Used with localeconv(). |
The <errno.h> Library Functions In C
Macro/Function | Prototype | Description |
---|---|---|
errno |
- |
A global integer variable that stores the error code for the most recent error condition. |
perror() |
void perror(const char *s); |
Prints an error message corresponding to the current value of errno. |
strerror() |
char *strerror(int errnum); |
Returns a string describing the error code specified by errnum. It provides a textual description of the error code passed to it. |
errno.h Constants |
- |
Constants representing common error codes (e.g., EIO, ENOMEM, etc.). These constants are used to check or specify error conditions in various system calls and library functions. |
The <ctype.h> Library Functions In C
Function/Macro | Prototype | Description |
---|---|---|
isalnum() |
int isalnum(int c); |
Check if the character c is alphanumeric (a letter or a digit). |
isalpha() |
int isalpha(int c); |
Check if the character c is an alphabetic character (a letter). |
iscntrl() |
int iscntrl(int c); |
Checks if the character c is a control character. |
isdigit() |
int isdigit(int c); |
Check if the character c is a digit. |
isgraph() |
int isgraph(int c); |
Check if the character c is a printable character excluding space. |
islower() |
int islower(int c); |
Check if the character c is a lowercase letter. |
isprint() |
int isprint(int c); |
Check if the character c is a printable character including space. |
ispunct() |
int ispunct(int c); |
Check if the character c is a punctuation character. |
isspace() |
int isspace(int c); |
Checks if the character c is a whitespace character. |
isupper() |
int isupper(int c); |
Check if the character c is an uppercase letter. |
isxdigit() |
int isxdigit(int c); |
Check if the character c is a hexadecimal digit. |
tolower() |
int tolower(int c); |
Converts the character c to lowercase if it is an uppercase letter; otherwise, it returns c unchanged. |
toupper() |
int toupper(int c); |
Converts the single character c to uppercase if it is a lowercase letter; otherwise, it returns c unchanged. |
Conclusion
In this article, we’ve explored the core library functions in C through a detailed examination of key header files such as <stdio.h>, <stdlib.h>, <math.h>, <string.h>, and others. Each header file serves a distinct purpose, providing essential functions that simplify programming tasks ranging from basic input and output to complex mathematical operations and memory management.
Understanding these library functions and their proper usage is crucial for writing efficient and effective C programs. The collection of functions provided by these respective header files not only enhances productivity by offering pre-written, reliable routines but also contributes to writing portable and maintainable code. From handling strings and performing mathematical calculations to managing files and dynamic memory, these library functions in C form the backbone of this programming language.
Frequently Asked Questions
Q. What are the different categories of library functions in C language?
Functions in the C language library can be categorized into several types based on their functionality and purpose.
- Input and Output Functions: These library functions are used for reading input from the user and writing output to the screen or files. Examples include printf(), scanf(), and file-related functions like fopen() and fclose.
- String Handling Functions: String functions are used for tasks such as copying, concatenating, comparing, and searching within strings. Functions like strlen(), strcpy(), and strtok() fall into this category.
- Memory Management Functions: These C library functions handle dynamic memory allocation and deallocation, such as malloc(), free(), and memory manipulation functions like memcpy().
- Mathematical Functions: Mathematical operations and calculations are performed using functions like sqrt, sin, pow, and others in this category.
- Date and Time Functions: Date and time-related operations are facilitated by functions like time, mktime(), and strftime().
- Character Handling Functions: These C library functions handle character-based operations, including checks for alphanumeric characters, case conversions, and more. Examples include isalpha(), tolower(), and isdigit().
- File Handling Functions: File input and output operations are supported by C library functions like fread(), fwrite(), fseek(), and file manipulation functions like remove and rename.
- Error Handling Functions: Functions like perror() and errno() are used for handling errors and generating error messages.
- Locale and Multibyte Functions: These functions deal with internationalization and multibyte character encodings, including setlocale() and conversion functions like mbstowcs().
- Miscellaneous Functions: This category encompasses a wide range of utility functions, including abort, exit, and random number generation functions.
Q. How to create a library function in C?
Creating a library function in C involves writing a function or set of functions in one or more source files and then compiling them into a library file that can be linked with other C programs. Here are the steps to create a library function in C:
- Write Your C Code: First, write the C code for the functions you want to include in your library. You can create a .c source file (e.g., mylib.c) and define your functions in it.
- Create a Header File: Create a header file (e.g., mylib.h) that declares the function prototypes you want to include in your library. This output header file should be included in both your library source file and any other C files that will use the library.
- Compile the Library: Compile the source file(s) into a library file using a C compiler. You can create a static library (.a file) or a dynamic/shared library (.so or .dll file) depending on your needs.
- Use the Library: To use your library functions in another C program, include the library header file and link your program with the library file during the compilation process.
- Run the Program: Now you can run your program, which uses the functions from your library.
Q. What is the syntax of function in C?
The basic syntax of a function in C is as follows:
return_type function_name(parameter_list) {
// Function body (statements and logic)
// ...
return return_value; // Optional return statement
}
Here:
- return_type: The data type of the value that the function returns. If the function does not return a value, this is void.
- function_name: The name of the function, used to call it in the code.
- parameter_list: A list of parameters (if any) enclosed in parentheses. Each parameter is specified by its data type and name, separated by commas. Parameters provide input to the function.
- *{ / Function body */ return return_value; }**: The function body enclosed in curly braces. It contains statements and logic to perform the function's task. The return statement (if present) provides the value to be returned to the caller.
Q. What is the purpose of including header files in C?
Header files, such as <stdio.h>, <stdlib.h>, and others, contain definitions of functions, macros, and types that are essential for various programming tasks. Including these headers allows a program to use the C library functions and constants defined in them, ensuring that the compiler knows about the functions' signatures and how to link them correctly during compilation.
Q. What are the common pitfalls to avoid when using library functions in C?
The common pitfalls that we need to avoid when using library functions in C are as follows:
- Buffer Overflows: Ensure that you do not write more data than the buffer can hold.
- Memory Leaks: Always free dynamically allocated memory using the free() function.
- Invalid Input: Validate inputs to functions like scanf() to prevent undefined behavior.
- Error Handling: Check for errors after performing file operations or memory allocations using malloc(), calloc(), etc.
You might also be interested in reading the following:
- Compilation In C | Detail Explanation Using Diagrams & Examples
- The Sizeof() Operator In C | A Detailed Explanation (+Examples)
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Comma Operator In C | Code Examples For Both Separator & Operator
- Function Pointers In C | A Comprehensive Guide With Code Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment