Constant In C | How To Define, Rules & Types (+Detailed Examples)
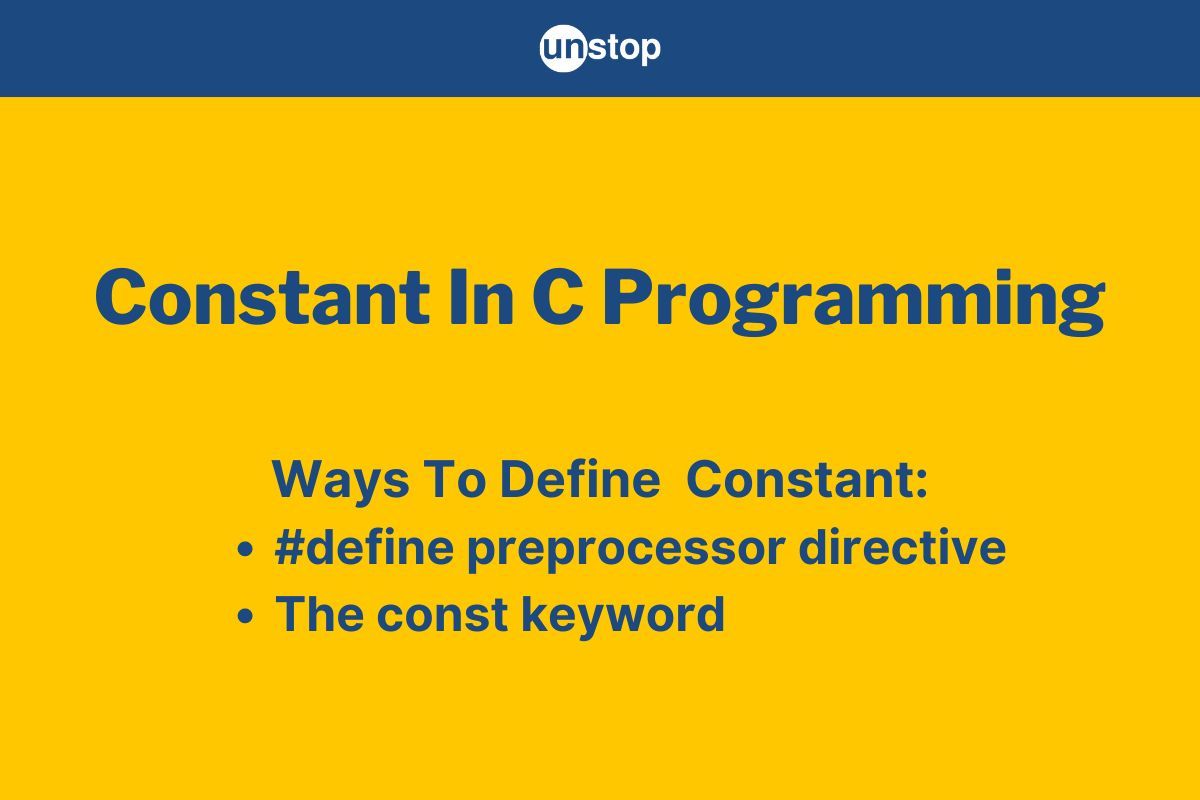
A constant in C language is a value that remains constant throughout the program and cannot be changed during program execution. They can be of many types, including integer constants, character constants, floating point constants, arithmetic constants, and array constants. Simply put values defined as constants in C programs (as the name suggests) do not change during the entire program execution.
For example, if you're working on a program that requires a Pi value, you can define ‘Pi’ as a constant so its value doesn't change while the C program is running.
Constants in C are usually defined at the beginning of the program, and the name given a is used throughout the program to represent that respective value. This helps make the program easier to read and manage. In this article, we will discuss everything related to constants in C in detail and give proper examples to help clarify the concepts.
Rules For Defining A Constant In C
Constants are declared using the const keyword, followed by an appropriate state and a constant value. However, there is a set of rules and regulations that one must follow when using constants. The rules for defining constants in C language are as follows:
- At the time of declaration of a constant value, you must use the const keyword followed by the data type of the respective variable/ value and the variable name. That is, constants are declared using appropriate literals such as const int, const float, etc.
const data_type var_name;
- For example, if you want to define a constant integer value with the name number and initial value 10. Here is what the line of code will look like-
const int number = 10
- An alternate way of defining constants in C programs is by using the #define pre-processor directive. For example, if you want to use the constant value of Pi across a program., you can define it using the directive as follows-
#define PI 3.1415
- Note that we can define constants globally to be accessible from any part of the program. We can also define them locally, in which case we can access them only by deriving from the number block they are defined in. (We will discuss this in later sections.)
- Note that you cannot make any modifications to values declared as constant. Attempts to update the constant value will result in an error.
Look at the simple C program example below, which illustrates these rules. In the sections ahead, we will discuss the methods of constant variable definition in detail.
Code Example:
Output:
Max value: 100
Pi: 3.14
Min value: 0
Explanation:
In the simple C code example, we first include the header file <stdio.h> for input/output operation.
- We then declare and initialize two constant variables, MAX_VALUE (of integer type)and PI (of float type), with the values 100 and 3.14, respectively.
- Then, inside the main() function, we declare another integer type constant, MIN_VALUE, with the initial value 0.
- Next, we use a set of printf() statements to access and display all the values to the console.
- As shown in the output, constant variables defined outside of the main() part are still accessible globally (global access).
- Also, the numeric constant defined inside the main() part can be accessed from within it (local access).
How To Define Constant In C?
As discussed above, there are two ways of defining constants in C programming. That is, either by using the #define pre-processor directive or the const keyword. Let's discuss these methods in detail.
Defining Constant In C Using The #define Preprocessor Directive
The #define directive is a preprocessor command used to define macros or constant values. It replaces a sequence of characters in the source code with another sequence during the compilation of the C program.
Syntax For Defining Constant In C Using #Define Directive:
#define CONSTANT_NAME constant_value
Here, the CONSTANT_NAME is the name/ identifier of the variable with the value set to constant_value. By convention, macro names are written in all caps. This helps simplify code and improve code readability when defining constants. Here are some examples:
Defining a fixed value for a macro with preprocessor directive #define:
#define BUFFER_SIZE 1024
The syntax example for PI is as follows:
#define PI 3.14159
After this definition, every time you use the term PI in the code, it will be replaced by 3.14159. The #define preprocessor directive is particularly useful for defining constants in C programs or macros substitution that apply throughout the program and can handle any basic data type.
Look at the C program example below for the implementation of this method of defining a constant.
Code Example:
Output:
3.14159
Explanation:
In the C code example-
- We include the required header file and then define a constant variable called PI with the value 3.14159 using the preprocessor directive.
- Inside the main() function, we use the printf() function with a formatted string to display the value of PI.
- Here, we use the %f format specifier as a placeholder for the floating point number/value.
Defining Constant In C Using The const Keyword
The const keyword, is also referred to as the const qualifier. It is another way of defining constants in C programs. It declares variables that cannot be changed after initialization, providing a safer and more readable alternative to #define.
When using this technique to define constant values, we mention the const keyword at the beginning of the line of code. This is followed by the data type of the variable and its name.
Syntax For Defining Constant In C:
const data_type constant_name = value;
Here,
- We use the const keyword to define the constant variable whose name is given by constant_name.
- The data_type indicates the type of value stored in the variable, which can be
For Example:
const int MAX_VALUE = 100;
This creates a constant variable MAX_VALUE with the value 100, and any attempt to modify it later in the program will result in a compilation error. Look at the example C program below for a better understanding of how this method is implemented.
Code Example:
Output:
The maximum value is: 100
Explanation:
In the example C code-
- We initiate the main() function, which is the entry point for the program's execution.
- Inside main(), we define a constant integer variable MAX_VAL (using const keyword) and assign the value 100 to it.
- Next, as mentioned in the code comments, we use the printf() library function to display the value of the variable with a descriptive string message.
- Here, we use the %d format specifier for integers and the newline escape sequence (\n), which shifts the cursor to the next line.
- Finally, the main() function completes with a return 0 statement, indicating successful execution.
Advantages Of Using The const Keyword
- Prevents accidental changes to values: When you attempt to modify a const variable, the compiler detects this violation and throws an error (compile time error) before the program can even run.
- Improves code readability: Using a constant in C programs to replace hard-coded values with descriptive names makes the code more understandable and maintainable.
Other Examples Of Const Qualifier/ Keyword
Here are some other ways in which we can use a constant in C programs:
- Fixed Size Array Definition
const int ARRAY_SIZE = 10;
int myArray[ARRAY_SIZE];
Here, we first define a constant variable to hold the size of an array. Then, when defining the array, we can use this const value to fix the size.
- Mathematical Constant Definition:
const double PI = 3.14159265359;
We have used the PI example before, but here it represents any mathematical values which must remain constant by definition.
- Boolean Flag Creation:
const int TRUE = 1;
const int FALSE = 0;
Boolean values are used in many control statements and comparative operations. It is important for these values to remain constant throughout. We can use the const qualifier to define boolean flags if needed.
Check out this amazing course to become the best version of the C programmer you can be.
Types Of Constants In C
There are two primary classifications/ types of constants in C language−
- Primary Constants: These include character constants, real constants, and integer constants.
- Secondary Constants: These include constants like enumerations (enum), arrays, pointers, unions, and structures in C programing.
Table Of Primary Constant In C (With Examples)
Type Of Constants |
Data Type |
Examples |
Integer Constants (int) |
unsigned int |
200u, 500U |
long int, long long int |
10, -5, 0 |
|
Floating-point or Real Constants |
float, double |
10.584634, 3.14 |
Character Constants |
char |
'X', 'Y', 'Z' |
The table provides a snapshot view of the different types of constants in C. Let's discuss these constants in detail.
Primary Constants In C
In C programming language, primary constants are those that are represented directly by a value, and there is no possibility of direct operations or substitutions on them. In other words, primary constants in C are those that are of the standard data type. For example, integer constants are declared using the int data type, while floating-point constants are declared using the float or double data type.
Example For Primary Constants In C:
- Integer: 3, 10
- Floating-point: 2.33, 3.14
- Character: "Hello", 'h'
As mentioned, primary constants in C can be divided into standard types, i.e., integer, floating-point, and character constants. An explanation for all is given ahead.
Integer Constant In C
Numeric constants that represent whole numbers are referred to as integer constants in C. They can be written in many formats, including decimal, binary, octal, and hexadecimal. Here are some examples:
- Decimal Format: 42, 100
- Octal Format: 052 (equivalent to decimal 42)
- Hexadecimal Format: 0xFF (equivalent to decimal 255)
- Binary format (C99 and above): 0b101010 (equivalent to 42 decimal numbers)
Integer constants can also be suffixed with letters to indicate their type. For example, integer constants ending in U represent unsigned integers and constants ending in L represent long integers. Some examples of integer constants in C with suffixes are:
- Unsigned Integer: 42U
- Long Integer: 123456L
- Unsigned Long Integer: 0xFFUL
Floating-Point Constant In C (Real Constants)
Numeric constants that represent real numbers are known as floating point constants in C. They can be written in two forms: decimal and exponential. Some examples of floating point in C are:
- Decimal Format: 3.14, 0.123, 1000.
- Exponential Notation/ Format: 3.14e2 (equal to 314), 1.23e-3 (equal to 0.00123)
In exponential form, the letters e or E indicate that the value is multiplied by the power of 10. For example, 3.14e2 means that 3.14 is raised to the power (10*2), which equals 314.
Character Constants In C
A constant character refers to a variable that represents a single character. These characters are enclosed in single quotes (' ') and can represent alphanumeric and special characters. Some examples of character constants in C are:
- 'A' (represents the character 'A')
- 'a' (represents the character 'a')
- '3' (represents the character '3')
- ' ' (represents a whitespace character/ space)
- '\n' (represents a newline character/ escape sequence)
- ''' (represents a single quote character)
- '\' (represents a backslash symbol/ character)
String Constants In C
The string constant is a sequence of characters enclosed in double quotes (" "). It can represent text, including alphanumeric characters and other special characters. Some examples of string constants in C are:
- "Hello, world!" (represents the string "Hello, world!")
- "12345" (represents the string "12345")
- " " (represents a string with a single whitespace character)
- "A\nB" (represents the string "A" followed by a newline character and then "B")
In other words, string constants in C can be thought of as strings of characters that end in a null character ('\0'). This character is automatically appended to the end of the string when defined and is used to indicate the end of the string. For example, "Hello" will be {'H', 'e', 'l', 'l', 'o', '\0'}.
Backslash Character Constants In C
The backslash (\) is used to start an escape that represents a special character that cannot be typed directly into a string. For example, characters like single and double quotes have predefined meanings in programming language as they are used in character and string creation. Hence, we use the backlash character followed by these special characters to insert them into strings. Here are some common backslash characters in C:
Meaning of Character |
Backslash Character |
Backspace |
\b |
New line |
\n |
Form feed |
\f |
Horizontal tab |
\t |
Carriage return |
\r |
Single quote |
\’ |
Double quote |
\” |
Vertical tab |
\v |
Backslash |
\\ |
Question mark |
\? |
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Conclusion
Constants in C Language are used to define values that remain unchanged during execution of a program. There are two ways of creating constants: using the const qualifier/ keyword or using the #define preprocessor directive. They can be created globally for access throughout the program or locally in which case they can be accessed only within the block they are defined in.
They enhance code readability, prevent accidental changes to important values, and are crucial for defining fixed values, such as array dimensions, flags, macros, and mathematical constants. Additionally, constants in C programs can also be used to define and pass parameters to functions and to create and initialize variables. Constants play a significant role in structuring robust and error-free code, helping maintain program integrity and making the code more readable.
Also Read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Is there any difference between floating-point constants and real constants?
Floating point constants represent real numbers with fractional parts. They are stored in computer memory using a format that allows for a certain degree of perfection and variety (precision). Floating point constants are frequently used in applications requiring exact/ precise calculations, such as scientific or engineering computations.
Real constants represent real numbers, often stored as floating-point values in many programming languages. However, different languages may store them in various formats (including but not limited to floating-point value format), depending on their required precision or range.
Q. Shall I use uppercase letters or lowercase to write the hexadecimal constant?
In computer programming, we typically use capital/ uppercase letters to write hexadecimal integer values/ constants. This makes them distinguishable from other types of constants, such as numeric or binary, and improves the overall readability of code.
However, some programming languages or coding conventions may specify the use of lowercase letters or a combination of upper and lowercase letters for hexadecimal constants. So, it is best to check your language's coding style guide for specific instructions on how to format hexadecimal constants.
Q. What are the types of constants in C?
In C, constants are values that remain unchanged during the execution of the program. In other words, a constant in C programs is used for values that should remain fixed throughout the program's execution. There are several types of constants in C based on the data types, including:
- Integer constants
- Floating-point constants
- Character constants
- String constants
- Boolean constants
- Enumeration constants
- Hexadecimal constants
Q. What are the variables and constants in C?
A variable refers to a named memory location that can hold the value of a particular data type. The value stored in the variable can change each execution of the program. When declaring a variable you must specify the data type of the value it can hold and the name of the variable which you will use to identify it across the program. The syntax for this is:
data_type var_name;
For example, if you want to declare a variable called age to hold the integer type value referring to a person's age, the line of code will be:
int age;
In comparison, a constant in C is a value that cannot be changed throughout the program's execution. The most common way to create a constant is by using the const keyword followed by the value's data type and the name of the constant variable. The syntax is:
const data_type var_name;
For example, you want to define a constant called PI to hold the double type value 3.14159265359 up to 11 decimal integer places. Then, the line of code will be:
const double PI = 3.14159265359;
Q. What is the use of the const keyword?
The const keyword in C is used to declare a variable as a constant. This helps ensure that once initialized, these values cannot be modified/ changed. This is useful when you want to ensure that the value of a variable remains constant throughout the program and cannot be accidentally modified.
Example: const int MAX_VALUE = 100;
Q. Is there any way to change the value of a constant variable in C?
The whole purpose of defining variable constants in C programs is to avoid accidental or any changes to the value once initialized. There is one way to alter these values, but it is not recommended that you do so. To change the value of a constant variable, you can use a pointer to directly modify the memory address where the constant is stored. This bypasses the protection offered by the const keyword.
const int MAX_VALUE = 100;
int *ptr = (int *)&MAX_VALUE; // Use a pointer to change the value
*ptr = 200;printf("%d", MAX_VALUE); // Output: 200
In the code snippet above, we have a const int MAX_VALUE whose address we assign to the pointer variable ptr. Here, we typecast the pointer type from const int to int. After that, we change the value pointed to by ptr to 200, thus modifying the value stored in the MAX_VALUE variable.
Note that this approach is considered bad practice and should be avoided, as it undermines the intention behind declaring a variable as const.
Q. What is a literal?
A literal is a value written directly into the program's code rather than calculated or referenced from variables. Examples include:
- 42 (integer literal)
- 3.14 (floating-point literal)
- 'c' (character literal)
- "hello" (string literal)
This compiles our discussion on constant in C. Go ahead and explore the following topics:
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
- Bitwise Operators In C Programming Explained With Code Examples
- Understanding Looping Statements In C [With Examples]
- Conditional/ If-Else Statements In C Explained With Code Examples
- Tokens In C | A Guide For All Types & Their Uses (With Examples)
- Difference Between Call By Value And Call By Reference (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment