Type Casting In C | Cast Functions, Types & More (+Code Examples)
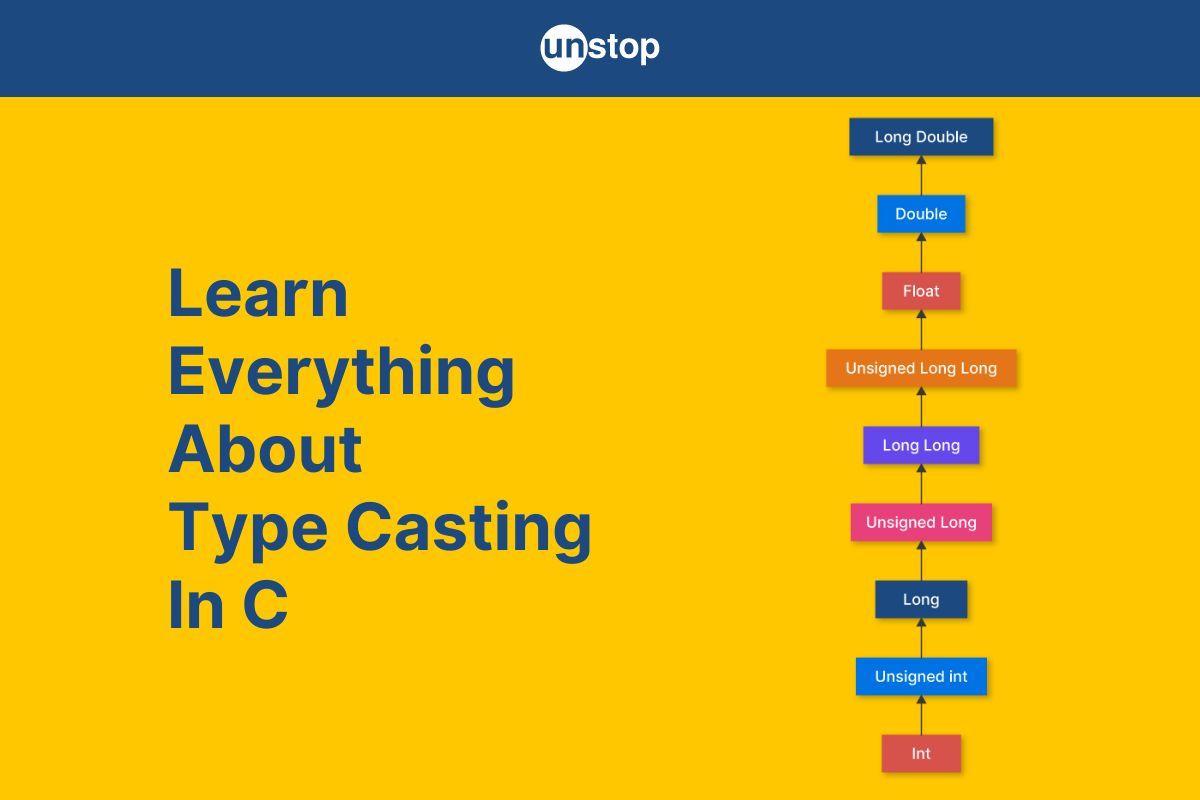
In programming, a data type serves as a crucial descriptor that instructs a computer on how to comprehend a value. It determines the nature of data stored in a variable. The world of C programming encompasses multiple data types like integer (int), character (char), floating-point (float type), and double-precision floating-point (double), among others. When working with code, you need to have precision in handling data types. Type casting in C is a fundamental concept when it comes to doing just that.
Type casting allows developers to convert one data type into another, enabling seamless operations and flexible variable assignments. In this article, we will discuss the intricacies of type casting in C, its significance, types, and the crucial role it plays in ensuring accurate and efficient programming.
What Is Type Casting In C Language?
Type casting is a fundamental operation in C that enables the modification of a variable's data type to a different one. In other words, it provides programmers with the ability to explicitly convert variables from one data type to another, tailoring them to meet specific program requirements. Through type casting, the compiler automatically adjusts the data type, guaranteeing compatibility and facilitating the desired behaviour of the program.
Also, by utilizing type casting in C language, programmers gain precise control over data interpretation and manipulation, facilitating smooth integration of different data types and empowering the accomplishment of targeted computational objectives.
The syntax for the type casting in C:
( data_type ) statement
Here,
- data_type: This is the data type in which we want to convert the statement.
- statement: It represents the value or variable that needs to be type cast.
Example of type casting in C:
Output:
(typecasting the expression) 25/3= 8.3333
Explanation:
We begin the simple C program by including the standard input-output library <stdio.h>, which is essential for input and output operations.
- We then begin the main() function, which is the entry point of the program, i.e., where the execution starts.
- Inside main(), we declare and initialize two integer variables, a and b, with the values 25 and 3, respectively.
- We also declare another variable c as a floating-point number. This variable will store the result of the division of a by b after typecasting a to a float.
- Next, we perform the division of a by b, and store the result in variable c, i.e., c = (float)a/b.
- Since both a and b are integers, (float)a is used for typecasting to ensure that the division result is stored as a floating-point number in c.
- We then use the printf() statement to display the result of the division. The format specifier %.4f is the placeholder for the floating-point result with four decimal places.
- The message within the printf statement provides additional information, indicating that we are typecasting the expression 25/3 to a floating-point number.
- The program concludes with a return 0 statement, indicating a successful execution of our program. The value 0 is conventionally used to signify that we terminated the program without errors.
Why Do We Need Type Casting In C?
As mentioned before, type casting is an integral concept in programming. Here are a few prominent reasons why we might need to use type casting in C programming:
- Type casting allows for the conversion of data between different types, ensuring compatibility between variables and expressions.
- It enables data of one type to be used in operations or assignments that expect a different type.
- Type casting in C gives us control over how data is interpreted and manipulated. It ensures that calculations and conversions are performed with the desired level of precision and accuracy.
- It can help us expand the range of values that a variable can hold.
- Type casting in C allows us to pass the correct data types when we are calling functions that require specific data types as arguments.
- It enables us to convert the entire structure, union, or specific members to different data types as needed.
- Type casting in C provides flexibility and control over how data is handled in C programs. It ensures that the program behaves as intended by allowing us to convert data between types.
What Is Cast Operator In C?
In C, the cast operator is a unary operator that is used to temporarily change the interpretation or representation of a value, variable, or expression to a specific data type.
The syntax of the cast operator in C:
( data_type ) statement
Here,
- The parentheses () can be referred to as the casting operator.
- The data_type represents the desired data type to which the expression is being cast.
- The statement is the value or variable to be cast.
Uses Of Cast Operator In C
- The main purpose of the cast operator is to explicitly convert values from one data type to another.
- The cast operator in C allows you to extend the range of values that a variable can hold. Suppose you have an integer variable that needs to store larger values beyond its default range. In such cases, you can utilize the cast operator to convert the variable to a larger data type, such as long or double. This type casting operation enables the variable to accommodate a broader range of values without losing information.
- The cast operator helps ensure compatibility when calling functions that expect specific data types as arguments.
- The cast operator in C is also useful for converting between different types of pointers. It allows you to change the interpretation of a pointer to a specific data type.
- When dealing with composite data types such as structures and unions, the cast operator becomes crucial. It provides the ability to cast the entire structure or union, or specific members within them, to different data types as required.
Types Of Type Casting In C
In C, type casting refers to the explicit conversion of a variable from one data type to another. There are two main conversion types in type casting, i.e., explicit and implicit. Both implicit and explicit type casting serve important roles in C programming. Implicit casting is automatic and is based on predefined rules, while explicit casting gives the programmer more control over the conversion process. We will explore both these type casting methods in C, in the sections ahead.
Implicit Type Casting In C
Implicit type casting in C, also called automatic type conversion, is when a programming language automatically converts one data type to another compatible type without the programmer needing to give explicit instructions.
- It happens when an operation involves operands with different data types, and the programming language automatically adjusts one operand to match the other's type.
- Implicit type casting in C aims to ensure compatibility between data types in an operation, enabling seamless execution without losing any data or precision.
Syntax Of Implicit Type Casting In C:
The syntax of implicit typecasting in C depends on the programming language you are using. However, in most languages, implicit typecasting occurs automatically based on certain rules defined by the language. In general, you don't need to explicitly write code for implicit typecasting. It happens implicitly when you perform operations or assignments involving variables of different data types.
Let's take a look at an example of implicit type casting in C, where we convert the character data type to an integer.
Code Example:
Output:
Character: A
Integer: 65
Explanation:
We start by including the <stdio.h> header file and initiate the main function, which is the entry point for the program's execution.
- Inside the main() function, we declare a character variable myChar and assign it the value of character A.
- We also declare an integer variable, myInt, without initializing it at this stage.
- Then, we conduct implicit type casting on the myInt variable (an integer) and assign it the value of myChar (a character), i.e., myInt = myChar. As a result, the ASCII value of the character A is stored in myInt.
- Next, we use a set of printf() statements to display the values of myChar and myInt.
- In the formatted string inside printf(), the %c format specifier is used for characters, %d for integers, and the newline escape sequence shifts the cursor to the next line.
- The first printf statement displays the character stored in myChar, which is 'A'.
- The second printf statement displays the integer value stored in myInt, which is the ASCII value of 'A'.
- The program concludes with a return of 0, indicating a successful execution without any errors.
Important Points About Implicit Conversions
- Implicit type conversion, also known as standard type conversion, is a type of conversion that occurs automatically without the need for any keywords or special statements.
- Converting from a smaller data type to a larger data type is referred to as type promotion.
- Implicit type conversion always takes place between compatible data types, ensuring a smooth and seamless conversion process.
- When performing a conversion between two different data types in a program, the automatic implicit type casting will convert the lower data type to the higher data type.
- When we perform type casting between different data types like float and int, the resulting value will be in the float data type.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Usual Arithmetic Conversion & Type Casting In C
The usual arithmetic conversions in C refer to the set of rules that define how operands with different data types are converted during arithmetic operations. These conversions ensure that the operands have a common type before the operation is performed. The goal is to avoid loss of precision and to maintain consistency in the resulting type. The usual arithmetic conversions are applied in the following scenarios:
- Binary Operators: When two operands of different types are used with a binary operator (e.g., addition, subtraction, multiplication), the usual arithmetic conversions come into play.
- Assignment Operators: The usual arithmetic conversions are also applied during assignment operations when the right-hand side and left-hand side have different types.
Steps involved in the arithmetic conversion process:
- If the operands involve integral types, they are first promoted to a common integral type. This usually means converting char, short, or enum types to int or unsigned int.
- If one of the operands is a floating-point number (float, double, or long double), the other operand is promoted to the same floating-point type.
- During the conversion, the goal is to preserve the precision and range of the original values as much as possible.
- If the operands have different signedness (signed and unsigned), the signedness is generally retained in the promoted type.
- The resulting operands are of the same type, and the arithmetic operation can be performed using that common type.
Arithmetic Conversion Hierarchy
Arithmetic conversions in C follow a hierarchy or a set of rules to determine the resulting type when operands of different types are involved in an arithmetic operation. The hierarchy helps maintain consistency and avoid loss of precision during these conversions. The general hierarchy, from lower to higher precision, is as follows:
- Long double
- Double
- Float
- Signed and unsigned long
- Signed and unsigned int
- Signed and unsigned short
The hierarchy is designed to ensure that the result of an arithmetic operation has a type that can represent both operands without loss of precision. For example, if you add an int and a double, the int is usually promoted to a double before the addition.
Explicit Type Casting In C
The second form of type casting in C referred to as explicit type conversion, is defined by the user. With explicit conversion, the user has the ability to specify the desired type to which the expression should be converted. This conversion can involve either a larger or smaller data type, depending on the requirements. Prior to the conversion, a runtime check is performed to ensure that the destination type can accommodate the value from the source.
In C, explicit type conversion can be accomplished by utilizing the cast operator. By employing the cast operator, the programmer can explicitly indicate the desired data type for the expression, facilitating the conversion process.
The syntax of explicit type casting in C:
(target-type)statement;
Here;
- The target-type is the desired data type to which the expression is being converted.
- The statement is either a variable, a constant, or an expression that must be converted/ cast.
Now, let's take a look at a code example of explicit type conversion in C for a better understanding of the same.
Code Example:
Output:
The value of x is 2.300000
The value of y is 4
Explanation:
In the C code example above-
- Inside the main() function, we declare a float variable x and initialize it with the value 2.3.
- We also declare an integer variable, y, and initialize it with the typecasted value of x + 2.
- As mentioned in code comments, the expression (int)x represents the typecasting of the float value x to an integer. This truncates the decimal part of x, leaving only the integer part.
- Next, we use two printf() statements to display the values of x and y. The %f format specifier is used for floats, and %d is used for integers.
- The first printf statement displays the float value stored in x, which is 2.3.
- The second printf statement displays the integer value stored in y, which results from explicit typecasting (int)x + 2. The float value x is truncated to 2 when typecasted, and then 2 is added to it.
- The program concludes successfully with a return of 0.
Rules For Type Explicit Casting In C
To gain a better understanding of the rules governing explicit type casting in a C program, consider the following points:
- The target type must be compatible with the expression being converted. For example, you cannot cast a string to an integer directly.
- When you convert a larger type of data to a smaller type, you should be careful because there is a chance that some data may be lost.
- That is, if the value being converted is too big to fit into the smaller type, it will be cut off or rounded, which means you won't have the exact same value anymore. It's important to remember this to ensure you get the expected results when performing such conversions.
- Casting from a data type with higher precision (e.g., float) to a lower precision data type (e.g., int) may result in a loss of information. Be aware of this potential loss and consider whether it is acceptable for your specific use case.
- Explicit type casting is checked during compilation for syntax correctness, but the programmer must ensure the validity and safety of the conversion at runtime.
- In some cases, runtime checks are performed to verify whether the value being converted can fit into the target type. A runtime check ensures that the value is within the range of the target type.
Integer Promotion & Explicit Type Casting In C
Integer promotion can be employed to convert integer values of smaller types, such as short or char, to their respective counterparts, unsigned int or int. By utilizing integer promotion, we can seamlessly convert these smaller integer types to the larger unsigned int or int values, ensuring compatibility and consistency throughout the program.
Code Example :
Output:
a: 50
b: 50
Explanation:
In this C example-
- Inside the main() function, we declare a character variable a and initialize it with the value 50.
- We also declare an integer variable b and assign the value of character a to it.
- This step involves implicit type casting, where the character variable a is automatically converted to an integer.
- Next, we use two printf() statements to display the values of a and b.
- The first printf statement displays the integer value stored in a, which is 50. The character variable a is implicitly converted to its ASCII value when printed as an integer.
- The second printf statement displays the integer value stored in b, which is also 50. This is the result of implicit type casting from a character to an integer.
- The program concludes with a return of 0, indicating a successful execution.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Built-In Type Cast Functions In C Language
Several inbuilt typecast functions in C language allow you to explicitly convert values from one data type to another. These typecast functions provide a way to override the default type conversions performed by the compiler. They are commonly used when you want to convert a value to a different data type temporarily or when you need to ensure a specific type of conversion.
These include function include atof(), atoi(), atol(), itoa(), etc. Let's take a look at a few of these functions and how they work to get type casting done in C programs.
1. The atof() Function For Type Casting In C
The atof() function converts a string data type to a float data type. It is defined in the <stdlib.h> header file. This function takes a string as input and returns the equivalent floating-point value.
The syntax for atof() function is:
double atof (const char* string);
Here,
- The term double means, returning a double value which represents the converted floating-point number.
- The atof() function takes a C-style string (const char *str) as its input parameter. That is, a pointer to the null-terminated string that represents the floating-point number.
Code Example:
Output:
Value of b = 1.120000
Explanation:
We begin by including two standard libraries, <stdio.h> for input-output operations and <stdlib.h> for functions involving memory allocation and conversions.
- Inside the main() function, we declare a character array a of size 10 and initialize it with the string- "1.12". Note that the array is declared as a character array, as it will be used to represent a string.
- Then, we declare a float variable b and use the atof() function from the stdlib.h library to convert the string in the character array a to a float.
- The atof() function stands for ASCII to float and is used for converting string representations of numbers to float values.
- The line float b = atof(a); takes the string "1.12" stored in the character array a and converts it to the floating-point value b.
- We use the printf() statement to display the value of b. The %f format specifier is used for floats.
- The output of the program will be the float value represented by the string "1.12".
- The program concludes with a return of 0, indicating a successful execution of our program.
2. The atoi() Function For Type Casting In C
The atoi() function in C is a standard library function used for converting ASCII strings representing integers to actual integer values. The name atoi stands for ASCII to Integer. The function is declared in the <stdlib.h> header file.
The atoi() function scans the input string until it encounters the first non-whitespace character (whitespace includes spaces, tabs, and newline characters). It then reads characters from that point until a non-digit character or the null terminator is encountered, converting the digits to an integer value.
The syntax of atoi() function is:
int atoi(const char *str);
Here,
- The atoi() function takes a C-style string (const char *str) as its input parameter.
- The term int means that the function will return an int value, which represents the converted integer number.
Code Example:
Output:
Value of b = 70
Explanation:
In this sample C code-
- Inside the main() function, we declare a character array arr of size 10 and initialize it with the string value 70 using double quotes. Note that the array is declared as a character array, as it will be used to represent a string.
- We then declare an integer variable b and use the atoi() function from the stdlib.h library to convert the string in the character array arr to an integer.
- It takes the string value- 70, stored in character array arr and converts it to the integer value b, i.e. int b = atoi(arr).
- Next, we use the printf() statement to display the value of b. The %d format specifier is used for integers.
3. The atol() Function For Type Casting In C
The atol() function is a part of the C standard library <stdlib.h>, and it is short for ASCII to Long. As evident by the full form, it is used to convert a string representing a long integer (in decimal notation) into a long integer value.
The syntax of atol() function is:
long int atol(const char * str);
Here,
- The atol() function takes a C-style string (const char *str) as its input parameter.
- The term long means that the function will return a long int value which represents the converted long-integer number.
Code Example:
Output:
Value of b = 1234567890
Explanation:
We begin the sample C program above by including essential libraries, i.e., <stdio.h> and <stdlib.h>.
- Inside the main() function, we declare a character array a with the size set to 30 and initialize it with the string value- 1234567890, using double quotes. Note that the array is declared as a character array, as it will be used to represent a string.
- We then declare a long integer variable b and use the atol() function to convert the string in the character array a to a long integer.
- The function takes the string value 1234567890 stored in character array a and converts it to the long integer value b, i.e., long b = atol(a).
- Then, we print the value of the variable b using a printf() statement. As shown above, the output is the long integer value represented by the string, i.e., 1234567890.
4. The itoa() Function For Type Casting In C
The itoa() function is not a standard function defined by the C language itself, but it is commonly found in many C libraries as a part of the standard library. It stands for Integer to ASCII and is used to convert an integer value to its equivalent ASCII string representation. The itoa() function typically takes three parameters, i.e., the integer value that we want to convert, the character array to store the resulting string, and a base (radix) of output representation.
The syntax of itoa() function is:
char *itoa(int value, char *str, int base);
Here,
- The value parameter represents the integer value that you want to convert to a string.
- The str parameter is a character array where the resulting string will be stored. A pointer to the character array (buffer) where the resulting ASCII string will be stored, i.e., char *str
- The base parameter specifies the numeric base or radix of the output representation (e.g., 10 for decimal, 16 for hexadecimal).
It is important to ensure that the array has enough space to accommodate the converted string.
5. The ltoa() Function For Type Casting In C
The ltoa() function is used to convert a long integer (type long) to a string. This function is not a standard C library function, which means that it may not be available on all compilers. However, it is found in some implementations, such as Microsoft's Visual Studio.
The syntax for Itoa() function:
char *ltoa(long value, char *str, int base);
Here,
- The parameter value refers to the long integer value that you want to convert to a string.
- The term char *str represents the pointer to a character array where the resulting string will be stored. It is important to ensure that the array has enough space to accommodate the converted string.
- And base specifies the numeric base or radix of the output representation. It can be any integer from 2 to 36. Common bases include 10 (decimal), 16 (hexadecimal), and 8 (octal).
Note: The itoa and ltoa functions may not recognized by all the compilers. This is because these functions are not standard C library functions, and their availability is not guaranteed across all compilers. You might use other functions like sprintf() or snprintf() for similar purposes.
Data Loss In Type Conversion
Data loss in type conversion occurs when a value is converted from one data type to another, and the target data type cannot fully represent the original value. This loss of precision or information can lead to unintended consequences in the program. Here are some common scenarios where data loss can occur:
-
Floating-point to Integer Conversion: Converting a floating-point value to an integer results in the truncation of the decimal part. For example, converting 3.14 to an integer will result in 3, and the fractional part (0.14) is lost. For example:
float floatValue = 3.14;
int intValue = (int)floatValue; // Data loss: intValue will be 3
-
Overflow in Integer Conversion: When converting a large integer to a smaller integer type, there might be an overflow, leading to data loss. If the value is outside the representable range of the target type, it wraps around or produces undefined behaviour. For example:
long longValue = 2147483648; // Beyond the range of int
int intValue = (int)longValue; // Data loss: intValue may not be as expected
-
Conversion between Signed and Unsigned Types: When converting between signed and unsigned types, the sign information may be lost. For instance, converting a negative signed integer to an unsigned integer may result in a large positive value. For example:
int negativeValue = -5;
unsigned int unsignedValue = (unsigned int)negativeValue; // Data loss: unsignedValue will be a large positive value
-
Character to Integer Conversion: Converting a character to an integer might lead to data loss if the character represents a value outside the representable range of the integer type. For example, converting a character 'A' to an integer may result in the ASCII value. For example:
char charValue = 'A';
int intValue = (int)charValue; // Data loss: intValue will be the ASCII value of 'A'
Developers must be aware of potential data loss during type conversion and handle such scenarios carefully. Consider using appropriate type conversions or ensuring that the target type has sufficient range to represent the converted value without loss of information.
Difference Between Type Conversion & Type Casting In C
Typecasting and type conversion are often used interchangeably, but there are subtle differences in their meanings. This table highlights the distinctions between the two concepts, emphasizing that typecasting is a specific form of type conversion that involves explicit instructions from the programmer to interpret a value as a different type.
Feature | Type Conversion | Type Casting |
---|---|---|
Definition | It is the process of converting a value from one data type to another. | It is a specific form of type conversion where the programmer explicitly instructs the compiler to interpret a value as a different type. |
Scope | Broad term covering various ways of changing data types, including implicit and explicit conversions. | A subset of type conversion, specifically referring to explicit operations where the programmer provides instructions to interpret a value as a different type. |
Implicit Conversion | It occurs automatically by the compiler when it can safely convert one data type to another without loss of information or precision. | It may cause a loss of information or precision, as the programmer explicitly specifies the desired type of conversion using casting operators or conversion functions. |
Examples |
|
|
Usage | Covers both implicit and explicit conversions, depending on the programming language and context. | Specifically refers to explicit operations where the programmer needs control over the conversion process. |
Language Examples | Implicit conversions may happen in languages like Python, JavaScript, etc. | Explicit typecasting is common in languages like C, C++, and related languages using casting operators or conversion functions. |
Advantages & Disadvantages Of Type Casting In C
There are a series of advantages and disadvantages that come with using type casting in C, which we will discuss in this section.
Advantages Of Type Casting In C
- Flexibility in Operations: Type casting in C allows for flexibility in performing operations involving different data types. It enables the programmer to explicitly convert data from one type to another, facilitating mixed-type arithmetic and other operations.
- Compatibility: Type casting helps in ensuring compatibility between different data types. It allows the programmer to convert data to a compatible type before using it in a specific context, preventing data mismatch errors.
- Precision Control: In situations where precision control is crucial, type casting in C allows the programmer to explicitly specify the desired precision by converting between data types, especially in numeric operations.
- Explicitness: Type casting makes the code more explicit by indicating the programmer's intention to change the data type. This can enhance code readability and reduce confusion regarding the type of data being manipulated.
Disadvantages Of Type Casting In C
- Loss of Precision: One of the major disadvantages of type casting in C is the potential loss of precision. For example, when converting a floating-point number to an integer, the fractional part is truncated, leading to a loss of information.
- Runtime Overhead: Explicit type casting in C often incurs runtime overhead as the conversion needs to be performed during program execution. This additional processing can impact performance, especially in situations where type casting is frequent.
- Compiler Warnings and Errors: Incorrect or unsafe type casting in C can lead to compiler warnings or errors. For example, attempting to cast between incompatible types or using invalid casting syntax may result in issues that can be challenging to debug.
- Potential for Undefined Behavior: In some cases, type casting can lead to undefined behaviour, especially when converting between incompatible types or when the value being cast is out of the range of the target type. This can introduce unpredictability in the program.
- Code Maintenance Challenges: Code that heavily relies on explicit type casting may become more challenging to maintain and understand, especially when performed in complex or nested expressions. It may lead to increased code complexity and reduced readability.
- Portability Concerns: Code that relies heavily on explicit type casting may be less portable across different platforms or compilers. The behaviour of type casting in C can vary, and assumptions about specific data type sizes may not hold true in all environments.
Conclusion
In conclusion, type casting in C is a fundamental tool for managing the complexities associated with different data types. Whether it's about preserving precision in mathematical operations or ensuring compatibility between variables, type casting empowers developers to exercise control over the interpretation of data.
Understanding the nuances of both implicit and explicit type casting in C is essential for writing robust and error-free C code. As developers navigate the intricacies of data manipulation, the judicious use of type casting becomes a skill that contributes to the efficiency and reliability of their programs.
Frequently Asked Questions
Q. What is type casting and its types?
Type casting in C, also known as type conversion, is the process of converting a value from one data type to another in a programming language. It allows you to interpret a given value as a different data type, which can be useful in various scenarios.
There are two major types of type casting in C:
- Implicit Type Casting: This type of type casting is performed by the compiler automatically when it can safely convert one data type to another without any loss of data. For example, it is converting an integer to a floating-point number.
- Explicit Type Casting: Also known as type casting operator or type coercion, this type of type casting in C is done explicitly by the programmer. It involves using the cast operator (parentheses with a type inside) to convert a value from one type to another.
Q. What are type casting structures in C?
In C, you cannot directly type-cast entire structures. Type casting is generally applied to individual members of a structure rather than the entire structure itself.
Example:
Output:
Before type casting: Ritu, 25
After type casting: Ritu, 25.00
Explanation:
In this C code, we first define a structure named Person with members, i.e., name and age.
- In the main() function, we declare and initialise a variable person1 of type Person with the name Ritu and age 25.
- We then print the values of person1.name and person1.age before typecasting.
- Next, we perform type casting by converting the simple integer age to a float using (float)person1.age.
- The float value is stored in floatAge, and the updated values are printed.
The output demonstrates the effect of type casting in C programming, with the age now displayed as a floating-point number.
Q. How is type conversion done in C?
Type conversion in C involves converting one data type to another. The compiler performs type conversion only for those data types where conversion is possible. It is important to note that the destination data type cannot be smaller than the source data type in type conversion. This process occurs at compile time and is often referred to as widening conversion due to the restriction on the destination data type.
Q. What is type casting syntax?
Type casting in C refers to the process of converting the data type of a variable to another desired one, as per program requirements. The type casting syntax in C is as follows:
(type) expression
Here, type represents the desired data type to which you want to cast the expression, and expression is the value or variable that you want to cast.
Q. What is type casting vs type?
The table below summarizes the difference between type and type casting in C programming.
Feature | Type | Type Casting |
---|---|---|
Definition | Refers to the data type of a variable, return value, etc. | Refers to the process of converting one data type to another. |
Purpose | Specifies the kind of data a variable can hold. | Used to change the data type of a variable or value |
Example | int, float, char, etc. | Converting an integer to a float, or vice versa |
Immutability | Once assigned, it remains constant | This can result in a new value or variable with a different data type |
Implicit/Explicit | It may be implicit or explicit based on the programming language | Explicitly performed by the programmer (explicit type casting) |
Compatibility | Ensures compatibility between operations and operands | Required when performing operations involving different data types |
Q. Why is type casting in C needed?
Here are a few reasons why type casting is useful in C programming:
- Type casting in C provides the means to convert data between varying types, guaranteeing harmony among variables and expressions.
- Facilitating the use of data from one type in operations or assignments expecting a different type, promotes seamless integration.
- It empowers us to govern how data is perceived and manipulated, assuring calculations and conversions occur with the intended precision and accuracy.
- Type casting in C language permits the broadening of a variable's value range, allowing for greater versatility in data storage.
Here are a few more topics you must read:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment