Table of content:
- What Is For Loop In C?
- The Structure Of For Loop In C
- How Does The For Loop In C Work?
- Example Of For Loop In C Programming
- Various Forms Of For Loop In C
- Multiple Initializations Inside For Loop In C
- Nested For Loop In C
- Infinite For Loop In C
- Advantages & Best Practices When Using For Loop In C
- Conclusion
- Frequently Asked Questions
For Loop In C | Structure, Working & Variations With Code Examples
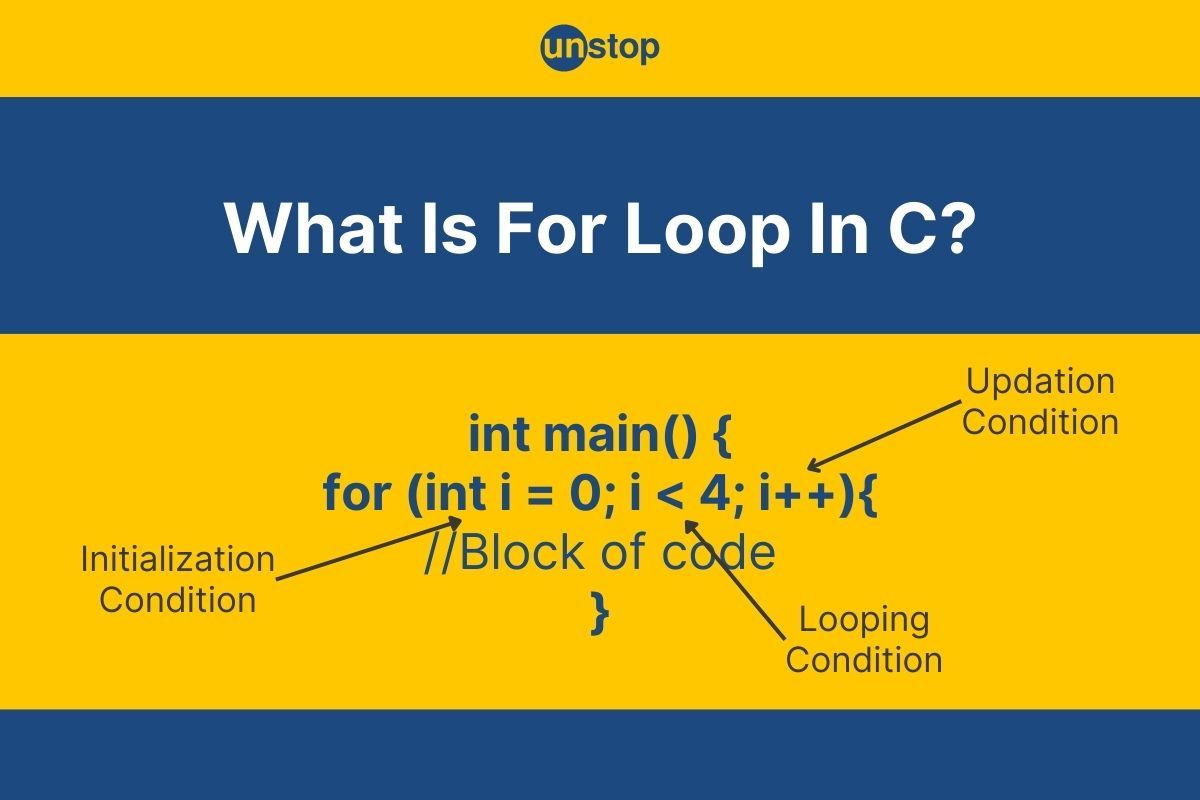
Programming languages are built on the foundation of powerful constructs that simplify the implementation of repetitive tasks, and the C programming language is no exception. Loops are programming constructs that allow the repetition of a set of instructions until a specific condition is met. They are used to efficiently execute a block of code multiple times without duplicating the code. One such indispensable construct is the for loop in C.
It is a versatile tool that facilitates efficient iteration through a set of instructions, allowing developers to write concise and structured code. In this article, we will delve into the key concepts of the for loop, explore its syntax and functionality, and provide practical examples to demonstrate its utility in various scenarios.
What Is For Loop In C?
In the C programming language, a for loop is a control flow construct used for the iterative execution of a block of code. It allows you to repeat a set of instructions a specific number of times or until a certain condition is met. The for loop is an entry-controlled loop and has a well-defined structure consisting of three main components, i.e., initialization, condition, and iteration expression.
Other loop constructs in C include the while loop and the do-while loop. The former of these is also an entry control loop or a pre-checking loop since the condition is checked at the beginning. The latter is an exit control loop or a post-checking loop since the condition is checked after the initial code block has been executed.
The Structure Of For Loop In C
As mentioned before, the for loop in C has a well-defined structure comprising three essential components, i.e., initialization (or the starting value of the control variable), condition, and iteration expression.
The for-loop syntax in C is as follows:
for (initialization; condition; iteration_expression) {
// Code block to be repeated
}
The for keyword marks the beginning of this loop in a C program. Here is a description of the three main components of a for loop-
Initialization
This part is executed only once at the beginning of the loop. It is used to initialize one or more loop control variables in an expression. These variables are crucial for controlling the loop and keeping track of the current state.
One important thing to note is that, with certain compiler exceptions, we can choose not to declare the variable in Expression 1. However, would have to modify our code accordingly.
For example:
#include <stdio.h>
int main(){
int i = 1;
for(; i < 5; i++){
printf("%d", i);}}
Here, the first condition, i.e., the initialization, is missing since we have initialized the control variable outside of the main() function.
Condition/ Test Expression
The loop continues executing as long as this specified condition is true (it is also known as the looping condition). If the condition becomes false, the loop terminates. In this sense, the looping condition acts as a gatekeeper, determining when the loop should stop or the program should exit the loop.
Note that we can also have more than one condition. However, the loop will iterate according to the last condition, and the other conditions will be treated as statements.
Iteration Expression/ Updation Condition
This part is executed after each iteration of the loop and is typically used to update the loop control variable(s). In other words, allows you to specify how the loop variables should change with each iteration. We generally use the increment and decrement operators to update the value of the control variable.
Loop body
This refers to the part of code written within the curly brackets {} of the for loop. This is the code that will be repeated again and again as per the conditions of the for loop, i.e., till the looping condition remains true. Note that it is possible to not use the curly braces ( {} ) if the body is of single line only. This is because curly braces actually define the scope of the for loop.
For example:
#include<stdio.h>
void main (){
for(int i=0; i<10; i++)
printf("%d ", i);}
How Does The For Loop In C Work?
The for loop flowchart in C, given below, explains the working mechanism of the loop visually. Take a look-
Here is a detailed explanation of the working of a for loop in C in a step-by-step format:
- Initialization Expression: The loop begins with the initialization of the control variable. This refers to the initial value taken by the variable
- Test Expression: Next, the program checks the looping condition given the initial value expression.
- If the condition returns false, the for loop gets terminated.
- If the condition returns true, the piece of code inside the body gets executed.
- Update Expression: After the successful execution of code statements/ code blocks inside the body of the loop, the loop variable is incremented or decremented. In other words, the value of the variable is changed in other ways depending on the operation.
- The loop continues execution until the loop condition returns false.
Example Of For Loop In C Programming
Let's take a look at a code example that showcases the implementation of a for loop in a C program. The code explanation following the example highlights the flow of control throughout the example.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHN1bSA9IDA7IC8vIEluaXRpYWxpemUgc3VtIHRvIHplcm8KCi8vIENyZWF0aW5nIGEgZm9yIGxvb3AgdG8gY2FsY3VsYXRlIHRoZSBzdW0gb2YgdGhlIGZpcnN0IDUgbmF0dXJhbCBudW1iZXJzCmZvciAoaW50IGkgPSAxOyBpIDw9IDU7IGkrKykgewpzdW0gKz0gaTsgLy8gQWNjdW11bGF0ZSB0aGUgc3VtIGluIGVhY2ggaXRlcmF0aW9uCgovLyBQcmludCB0aGUgY3VycmVudCB2YWx1ZSBvZiBpIGluIGVhY2ggaXRlcmF0aW9uCnByaW50ZigiSXRlcmF0aW9uICVkOiBDdXJyZW50IHN1bSA9ICVkXG4iLCBpLCBzdW0pOwp9CgovLyBQcmludCB0aGUgZmluYWwgc3VtIGFmdGVyIHRoZSBsb29wIGNvbXBsZXRlcwpwcmludGYoIkZpbmFsIHN1bTogJWRcbiIsIHN1bSk7CgpyZXR1cm4gMDsKfQ==
Output:
Iteration 1: Current sum = 1
Iteration 2: Current sum = 3
Iteration 3: Current sum = 6
Iteration 4: Current sum = 10
Iteration 5: Current sum = 15
Final sum: 15
Explanation:
In the simple C program above-
- We first include the <stdio.h> header file, which contains the essential input/ output operations. And then initiate the main() function, which is the entry point for the program's execution.
- Inside main(), we initialize a variable of integer data type, sum with the value 0. This variable will be used to accumulate the sum of numbers.
- We then create a for loop, as mentioned in the code comments, to iterate over a sequence of numbers and calculate the sum of the first 5 of them. Here-
- The loop starts from 1 (int i = 1) and continues as long as i is less than or equal to 5 (i <= 5).
- During each iteration, we use the compound addition assignment operator to iteratively calculate the accumulated sum of the variable values.
- After each iteration, the value of i is incremented by 1.
- We begin with sum=0, which after 1st iteration becomes, sum= 0+1 =1, after 2nd iteration sum = 1+2 =3, after 3rd iteration sum = 3+3=6, and so on.
- The printf() statement inside the loop consists of a formatted string, which prints the iteration number (i) and the current sum.
- After the loop runs five times, we use another printf() statement to display the final sum of the first five natural numbers to the console.
- The program terminates with a return 0.
Time Complexity: O(n) - The loop runs a constant number of times (5 in this case), leading to linear time complexity.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Various Forms Of For Loop In C
In C, the for loop is a versatile construct, and it can be used in various forms to suit different programming needs. Let's explore some common forms of the for-loop flow control statements.
Simple Increment For Loop In C
The simple increment for loop is a fundamental structure that initializes a loop control variable (i in this case) to zero, continues as long as the condition (i < end) is true, and increments i by 1 (i.e., the increment counter) after each iteration.
Syntax:
for (int i = start; i < end; i += step) {
// Code to be executed for each iteration
}
Here,
- Initialization: The loop variable is initialized to a starting value (start).
- Condition: The loop continues as long as the specified condition (i < end) is true.
- Update: The loop variable is incremented by a specific value (step) in each iteration.
Simple Decrement For Loop In C
The decrement for loop is similar to the simple increment loop but starts with a higher initial value and decrements i by 1 after each iteration until the condition (i > end) becomes false.
Syntax:
for (int i = start; i > end; i -= step) {
// Code to be executed for each iteration
}
Here,
- Initialization: The loop variable is initialized to a starting value (start).
- Condition: The loop continues as long as the specified condition (i > end) is true.
- Update: The loop variable is decremented by a specific value (step) in each iteration.
Custom Step For Loop In C
A custom step loop is a type of loop where the iteration variable (loop variable) is updated by a specific value other than 1 in each iteration. This allows for more flexibility in controlling the progression of the loop. Below is a code example to explain the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKZm9yIChpbnQgaSA9IDA7IGkgPCAxMDsgaSArPSAyKSB7CnByaW50ZigiJWQgIiwgaSk7Cn0KCnJldHVybiAwOwp9
Output:
0 2 4 6 8
Explanation:
In this C code, a for loop iterates over even numbers from 0 to 8 (inclusive) with a step size of 2. The values of i are printed in each iteration, resulting in the output- 0 2 4 6 8. The loop increments i by 2 in each iteration, and the program returns 0 upon completion.
For Loop To Iterate Through Arrays
The for loop for iterating through arrays is a common use case. It initializes an index variable (i), continues as long as the index is less than the length of the array, and increments the index after each iteration. Below is a code example to explain the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IG51bWJlcnNbXSA9IHsxLCAyLCAzLCA0LCA1fTsKaW50IGxlbmd0aCA9IHNpemVvZihudW1iZXJzKSAvIHNpemVvZihudW1iZXJzWzBdKTsKCmZvciAoaW50IGkgPSAwOyBpIDwgbGVuZ3RoOyBpKyspIHsKcHJpbnRmKCIlZCAiLCBudW1iZXJzW2ldKTsKfQoKcmV0dXJuIDA7Cn0=
Output:
1 2 3 4 5
Explanation:
In this C code, we first define an array of numbers containing elements 1 to 5.
- Then, we calculate the variable length as the total size of the array divided by the size of a single element using the sizeof() function.
- Next, we create a for loop to iterate through each element of the array, printing its value followed by a space.
- The program returns 0 after completing the loop.
The loop ensures that every element in the array is processed, making it a concise way to iterate over arrays.
Infinite For Loop In C
The infinite for loop has no specified condition, leading to continuous iteration until manually terminated. Such an endless loop is a powerful construct but should be used with caution.
Syntax:
for (;;) {
// Code for the infinite loop
// This will keep running indefinitely
}
Here, the only difference between the syntax of a regular for loop in C and this is that there is no initialization, looping, and updating conditions in infinite loops. We will discuss this in detail in the section ahead.
Nested For Loops In C
Nested for loops involve one for loop type inside another. This can be useful for iterating over two-dimensional structures, such as matrices. The syntax for a simple nested loop is:
Syntax:
for (initialization; condition; update) {
for (inner initialization; inner condition; inner update) {
// Code for inner loop
}
// Code after inner loop (optional)
}
Here, the basic syntax of the for-loop in C remains the same; we just add one loop inside another. We will discuss this with the help of a code example in a section ahead.
Note: The for loop in C can also change form with the use of other conditional statements/ control statements inside it. For example, we can use an if-statement inside the loop to add an additional condition. We can also use break statements inside the loop control instructions to jump out of the loop after a certain requirement is met. The possibilities are endless!
Multiple Initializations Inside For Loop In C
In C, the for loop allows for multiple initializations within its syntax. This feature is helpful when you need to initialize and work with multiple loop control variables simultaneously.
The syntax for a for loop with multiple initializations is as follows:
for (initialization_1, initialization_2; condition; iteration_expression) {
// Code to be repeated
}
Here,
- The expression initialization_1 and initialization_2 are two different initializations separated by the comma operator.
- Both initializations are executed at the beginning of the loop, and the loop control variables introduced by these initializations can be used within the loop body.
- The condition and iteration_expression refer to the loop condition and the increment/ decrement/ updation condition for the control variable after every iteration, respectively.
Let's look at an example to illustrate the concept of multiple initialization with a for loop in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKZm9yIChpbnQgaSA9IDAsIGogPSAxMDsgaSA8IDU7IGkrKywgaiAtPSAyKSB7Ci8vIENvZGUgdG8gYmUgcmVwZWF0ZWQKcHJpbnRmKCJpOiAlZCwgajogJWRcbiIsIGksIGopOwp9CgpyZXR1cm4gMDsKfQ==
Output:
i: 0, j: 10
i: 1, j: 8
i: 2, j: 6
i: 3, j: 4
i: 4, j: 2
Explanation:
In the example C program above,
- We begin by including the input/ output library and start the main() function.
- Then, we create a for loop with two control variables, i and j, to print the values of these variables. Here-
- The loop begins by initializing the control variable with the value i to 0 and j to 10 and continues as long as the condition i < 5 is true.
- For every iteration where the condition remains true, the printf() statement inside the loop body displays the values of the variable at that point.
- The print statement consists of the %d format specifier as a placeholder for the integer value and a newline escape sequence to ensure that the output for the next iteration is printed in a new line.
- After each iteration, the value of i is incremented by 1, and j is decremented by 2, using the increment/ decrement operators.
- The loop runs for five iterations, and the output shows the values of i and j in each iteration.
- Finally, the program terminates with a return 0 statement, indicating a successful implementation without any errors.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Nested For Loop In C
In C programming, nested for loops refers to the practice of placing one or more for loops inside another. This allows for the creation of complex loop structures, where the inner loop is executed multiple times for each iteration of the outer loop.
The syntax for nested for loops consists of one or more for loop constructs within the body of another. This structure is particularly useful when dealing with multi-dimensional arrays or when a repetitive task needs to be performed in a systematic way.
The general syntax for a nested for loop is as follows:
for (initialization_1; condition_1; updation_expression_1) {
for (initialization_2; condition_2; updation_expression_2) {
// Code to be repeated
}
//Code for outer loop (optional)
}
Here,
- The outer loop is controlled by the expressions- initialization_1, condition_1, and updation_expression1. They have the same relevance as in the syntax for a regular for loop in C.
- Inside the outer loop, there is an inner loop with its own set of expressions initialization_2, condition_2, and updatation_expression2.
- The code inside the inner loop is executed for each iteration of the outer loop.
- The optional code after the inner loop is the code block for the outer loop, which can be mentioned either before or after the inner loop.
Now, let's take a look at a simple example that prints a multiplication table using a nested for loop in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKZm9yIChpbnQgaSA9IDE7IGkgPD0gNTsgaSsrKSB7CmZvciAoaW50IGogPSAxOyBqIDw9IDU7IGorKykgewpwcmludGYoIiVkXHQiLCBpICogaik7Cn0KcHJpbnRmKCJcbiIpOwp9CgpyZXR1cm4gMDsKfQ==
Output:
1 2 3 4 5
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
5 10 15 20 25
Explanation:
In the example C code-
- We create a nested for loop inside the main() function to iteratively print the multiplication table of the values of two control variables.
- The control variable for the outer loop is i, whose initial value is 1, and it runs till the value is 5.
- The inner loop is controlled by variable j, whose initial value is 1, and it runs till j=5.
- The inner loop executes for each value of i and runs 5 times every time.
- It contains a printf() statement, which displays the product of i and j followed by a tab character/ escape sequence, creating a multiplication table-like output.
- After every iteration of the inner loop, the value of j is incremented by 1, and the flow of control passes to the outer loop after the value of j=5.
- After every iteration of the inner loop, the printf() statement in the outer loop moves the cursor to a new line, i.e., printf("\n"). The escape sequence in the formatted string here creates a new row in the output.
- This nested loop structure generates a multiplication table where each row corresponds to a value of i, and each column within the row corresponds to a value of j.
- The program returns 0 to indicate successful termination.
Infinite For Loop In C
An infinite for loop continues to execute indefinitely. In C, this is commonly achieved by creating a loop without any explicit termination condition. Infinite loops are typically used in scenarios where continuous execution is required until an external action interrupts the program, such as manual termination or an interrupt signal.
The syntax for an infinite for loop in C is as follows:
for (;;) {
// Code block to be repeated indefinitely
}
Here,
- The initialization, condition, and update expressions in the for loop are all empty (;;), indicating that there is no initialization, no condition to check, and no update.
- The absence of a condition ensures that the loop runs indefinitely.
- The code inside the loop will be executed continuously until the program is manually terminated.
Let's look at an example with an infinite loop that prints "Hello, World!" indefinitely for a better understanding of the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKZm9yICg7OykgewpwcmludGYoIkhlbGxvLCBXb3JsZCFcbiIpOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Hello, World!
Hello, World!
Hello, World!
... (continues indefinitely)
Explanation:
In the C code example above-
- We include the standard input-output library and define the main() function, which is the start of the program's execution.
- Then, we create an infinite for loop that has no initialization, condition, or update expressions, resulting in indefinite execution.
- Inside the infinite loop, there is a printf() statement that contains a string value, i.e., Hello, World!. This is followed by a newline character to push the cursor to the next line after every iteration.
- The loop runs indefinitely until manually terminated by an external action, such as interrupting the program.
- The return 0 statement indicates a successful program termination. However, in this case, due to the infinitive for loop condition, the return statement is never reached.
How To Avoid Infinite Loops In C?
Avoiding infinite loops is crucial to prevent programs from running indefinitely and to maintain proper program execution. Here are some points to consider for avoiding infinite for loops in C:
- Define a Clear Exit Condition: Always define a clear and logical exit condition in the loop's condition part. And ensure that the exit condition is reachable and will eventually be satisfied.
- Use Logical Conditions: Ensure that the loop condition correctly uses logical operators (&&, ||, etc.) to avoid unintended behavior. Be especially cautious with complex conditions that might lead to unexpected results.
- Initialize Loop Control Variables Properly: Initialize loop control variables before the loop to prevent undefined behavior. And avoid using uninitialized variables in the loop condition.
- Avoid Infinite Increments/Decrements: Ensure that the iteration expression contributes to reaching the exit condition. In other words, avoid scenarios where the loop control variable never reaches the exit condition due to improper increment steps or decrement steps.
- Use a Counter and Limit Iterations: Use a counter variable to limit the number of iterations, especially in situations where the exact exit condition is challenging to determine. Set a maximum number of iterations and exit the loop when the counter reaches that limit.
- Avoid Nested Loops Without Clear Termination: If using nested loops, ensure that each loop has a clear termination condition. Avoid complex nested loop structures that can lead to unintended infinite loops.
- Test and Debug: Regularly test and debug your code to identify potential issues with loop conditions and exit conditions. You can use a variety of available debugging tools and techniques to step through the code and understand its behavior.
- Code Review: Conduct code reviews to ensure that multiple eyes have reviewed the logic and identified potential issues. It is a great idea to encourage peer reviews to catch logical errors that might lead to infinite loops.
Advantages & Best Practices When Using For Loop In C
The for loops in C are one of the most essential constructs in controlling the flow of the program. Here are some of the benefits of using these constructs and best practices when writing C code-
- Conciseness and Readability: The for loop's concise syntax allows for a clear representation of repetitive tasks, enhancing code readability.
- Efficiency: Well-constructed for loops can lead to more efficient and optimized code execution, especially when handling large datasets or arrays.
- Scope Control: The scope of loop control variables is limited to the loop block, minimizing unintentional variable clashes and enhancing code modularity.
- Avoiding Infinite Loops: Careful consideration of initialization, condition, and iteration expressions helps prevent unintended infinite loops. That is, it is simple to avoid infinite loops by paying attention to a few parameters.
- Separate Loop Logic from Loop Body: These loops clearly separate the loop logic (initialization, condition, and iteration) from the actual code to be repeated. This improves code organization and makes it easier to maintain.
Conclusion
The for loop in C is a versatile and indispensable tool for handling repetitive tasks with efficiency and clarity. Its structured syntax, encompassing initialization, condition, and iteration expressions within a single line, contributes to code readability and conciseness. The for loop's adaptability makes it suitable for scenarios where the number of iterations is known in advance, allowing for optimized and efficient code execution.
Whether you are a beginner learning the basics of C programming or an experienced developer crafting intricate algorithms, the for loop in C remains an essential building block, offering a balance of control and simplicity. By embracing its advantages and adhering to established best practices, programmers can wield the for loop effectively, transforming repetitive tasks into elegant and efficient solutions.
Also Read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What is a loop in C?
In C programming, a loop is a control structure that allows a set of instructions to be executed repeatedly while a specified condition is true. Loops are essential for performing repetitive tasks efficiently and are fundamental to the concept of iteration in programming. They help avoid code duplication and enhance the overall readability and maintainability of the code.
Q. What are the 3 types of loops?
In C, there are three primary types of loops, namely, for loop, while loop, and do-while loop. The syntax and a brief explanation of these are given below.
For Loop: The for loop is a versatile and widely used loop structure in C. It allows you to execute a block of code repeatedly for a specified number of times.
The syntax is as follows:
for (initialization; condition; iteration_expression) {
// Code to be repeated
}
While Loop: The while loop repeats a block of code as long as a specified condition is true. It is particularly useful when the number of iterations is not known beforehand.
The syntax is as follows:
while (condition) {
// Code to be repeated
}
Do-While Loop: The do-while loop is similar to the while loop, but the conditional expression is evaluated after the execution of the loop body. This ensures that the loop body is executed at least once, even if the condition is initially false.
The syntax is as follows:
do {
// Code to be repeated
} while (condition);
These three types of loops provide different ways to control the flow of a program and execute a set of instructions repeatedly based on certain conditions.
Q. What is the syntax of the for loop in C?
The syntax of the for loop in C is centered around a control variable, a test condition and a code of block to be executed repeatedly as per set conditions. The syntax is as follows:
for (initialization; condition; iteration_expression) {
// Code to be repeated
}
Here,
- Initialization Statement: Provides the initial value of the control variable and is executed only once, i.e., at the beginning of the loop.
- Conditional Statement: Loop continues as long as this condition returns true. It is checked at every iteration. The loop terminates when this condition returns false.
- Iteration Expression: This provides the condition based on which the control variable is updated, and is implemented after each iteration.
Q. Can I nest for loops in C?
Yes, you can nest for loops in C. Nesting refers to the practice of placing one for loop inside another. This is a common technique used in programming to handle multidimensional data structures or to perform repeated tasks with a more complex pattern.
The general syntax for a nested for loop looks like this:
for (int i = 0; i < outer_limit; i++) {
for (int j = 0; j < inner_limit; j++) {
// Code to be repeated for each combination of i and j
}
}
Here, the outer loop (for (int i = 0; i < outer_limit; i++)) contains an inner loop (for (int j = 0; j < inner_limit; j++)). The code inside the inner loop will be executed for each combination of i and j.
A common use case for nested for loops is when working with two-dimensional arrays or matrices. Each iteration of the outer loop can represent a row, and each iteration of the inner loop can represent a column in a two-dimensional array.
Q. What are the applications of loops in C?
Loops in C are fundamental constructs that facilitate the repetition of a specific block of code. Their applications are diverse, ranging from simple tasks to complex algorithms. Here are some common applications of loops in C:
- Iterating Through Arrays: Loops are used to traverse and process elements in arrays efficiently.
- Printing Patterns: Loops facilitate the creation of patterns, such as triangles or squares, by repeating specific print statements.
- Performing Arithmetic Operations: Loops are employed for mathematical computations, like calculating sums or products with the use of arithmetic operators.
- Data Processing and Analysis: Loops are crucial for iterating through datasets and performing data processing tasks.
- Matrix Manipulation: Nested loops are commonly used for working with two-dimensional arrays or matrices.
- Searching and Sorting Algorithms: Loops play a key role in searching and sorting algorithms, enabling the repeated examination and manipulation of data.
- Menu-Driven Programs: Loops are used in menu-driven programs where the user interacts through a menu, allowing the program to repeatedly respond to user input.
- File Handling: Loops are employed when reading or writing data to files, iterating through file contents or records.
- Implementing Algorithms: Loops are essential in implementing various algorithms, from basic to complex, by repeating specific steps until a condition is met.
Q. What is the purpose of the for loop's initialization and iteration expressions?
The initialization expression of the for loop in C sets or initializes the control variable and is executed once at the beginning of the loop. The iteration expression, also referred to as the updation condition, defines how to update the loop control variable after each iteration, thus controlling the progression of the loop. These expressions help manage the loop's state and determine when the for loop in C should terminate.
Here are a few more interesting topics for you to immerse in:
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment