Table of content:
- What Is Format Specifier In C?
- List Of Format Specifiers In C
- Types Of Format Specifiers In C
- What Is Double Format Specifier In C?
- Float Data Type Vs. Double Data Type In C
- Why Should I Use Format Specifiers In C?
- Conclusion
- Frequently Asked Questions
Format Specifiers In C | A Complete Guide With Detailed Examples
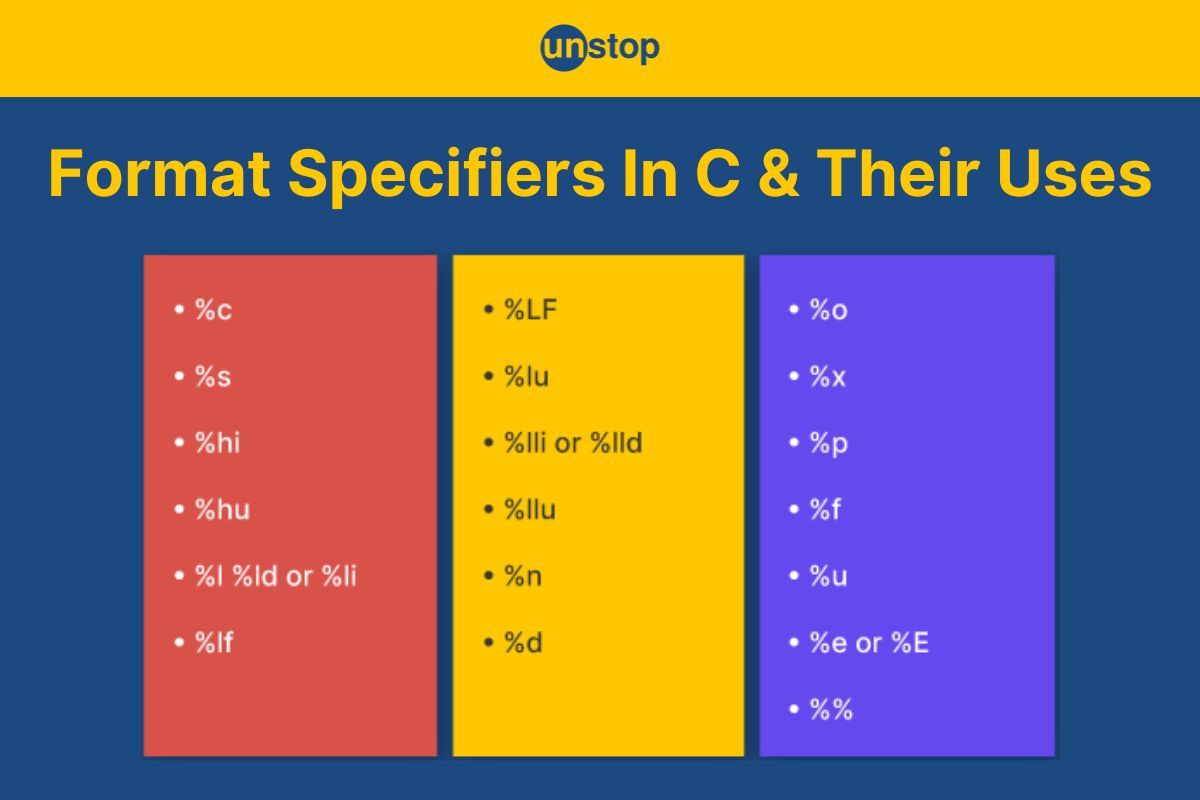
One of the most powerful and versatile programming languages, C is known for its low-level capabilities and efficiency. To work effectively in the C, developers must understand various features and concepts. This includes format specifiers in C programming that are essential for handling input and output operations. These specifiers help define the data type of values to be read or written.
In this article, we will explore the concept of format specifiers in C programming, explaining what they are and how to use them with practical examples.
What Is Format Specifier In C?
Format specifiers, also known as format codes or format strings, are placeholders used in input and output functions to represent data types. They instruct the compiler on how to interpret and display data when using functions like printf() and scanf().
- Format specifiers are used to ensure that the program correctly reads and displays data based on the specified data type.
- In C, format specifiers are special characters that begin with the modulus/per cent symbol (%), followed by a character indicating the data type.
- For example, the format specifier symbol %d represents a decimal integer/ integer data type, %f represents a floating-point number, and %c represents a character.
Each format specifier corresponds to a specific data type, ensuring that the program handles the data correctly.
List Of Format Specifiers In C
It is important to correctly use format specifiers in C programming language. Incorrect usage can lead to errors and unexpected results.
- To prevent this, it is recommended to use appropriate format specifiers according to the type of data that needs to be displayed or read.
- If a specific format specifier is not available for the data type being used, it may be necessary to write custom code or functions properly.
The table below lists some of the most commonly used format specifiers in C, along with a description, range, and size.
Symbol/ Notation |
Format Specifier Name |
Description |
Data type |
Range |
Size |
%d or %i |
Decimal integer |
Signed integer in base 10 |
int |
-2147483648 to 2147483647 |
4 bytes |
%f |
Float |
Floating point number with six digits of precision |
float |
1.2E-38 to 3.4E+38 |
4 bytes |
%Lf |
Long double |
Floating point number with extended precision |
long double |
3.4E-4932 to 1.1E+4932 |
10 or 16 bytes |
%c |
Character |
Single character |
char |
-128 to 127 |
1 byte |
%s |
String |
String of characters |
char[] |
- |
- |
%p |
Pointer |
Address in memory |
void * |
- |
4 or 8 bytes |
%Id |
Long integer |
Signed long integer |
signed long |
-2147483648 to 2147483647 |
4 bytes |
%lu |
Unsigned Long |
Unsigned long integer |
unsigned long |
0 to 4294967295 |
4 bytes |
%lld |
Long Long |
Signed long long integer |
long long |
-9223372036854775808 to 9223372036854775807 |
8 bytes |
%llu |
Unsigned Long Long |
Unsigned long long integer |
unsigned long long |
0 to 18446744073709551615 |
8 bytes |
%x |
Hexadecimal |
Unsigned integer in base 16 |
unsigned int |
0 to 4294967295 |
4 bytes |
%E |
Scientific notation |
Floating point number in scientific notation |
double |
2.2E-308 to 1.8E+308 |
8 bytes |
%o |
Octal |
Unsigned integer in base 8 |
unsigned int |
0 to 4294967295 |
4 bytes |
%u |
Unsigned Decimal |
Unsigned integer in base 10 |
unsigned int |
0 to 4294967295 |
4 bytes |
%hd |
Short |
Short signed integer |
short |
-32768 to 32767 |
2 bytes |
%m |
Error message |
Error message corresponding to the error number in the argument |
int |
- |
- |
%n |
Output assignment |
Stores the number of characters written so far into the pointer argument |
int * |
- |
- |
%hu |
Unsigned Short |
Short unsigned integer |
unsigned short |
0 to 65535 |
2 bytes |
Note: The sizes listed here are for a typical 32-bit or 64-bit system and may vary depending on the system and compiler used to run the C program. Also, note that the size of a pointer depends on the system architecture. On a 32-bit system, pointers are typically 4 bytes, while on a 64-bit system, pointers are typically 8 bytes.
Types Of Format Specifiers In C
Integer Format Specifier In C (%i or %d)
The %i and %d format specifiers are used to represent integer values when the data type is a signed integer. There are many kinds of integer format specifiers, of which %d is used to format an integer as a signed decimal.
This means that it represents a value that is decimal, regardless of whether it is positive or negative. Overall, both %i and %d serve the same purpose in the context of the printf() function.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IG51bSA9IDEwOwpwcmludGYoIlRoZSB2YWx1ZSBvZiBudW0gaXMgJWRcbiIsIG51bSk7CgpyZXR1cm4gMDsKfQ==
Output:
The value of num is 10
Explanation:
We begin the simple C program example by including the header file <stdio.h> for standard input-output operations.
- We then initiate the main() function, which is the program's entry point.
- Inside the main, we declare an integer type variable num and initialize it with the value 10.
- Next, we use the library function printf() to display the value with a message on the console. The message includes the format specifier %d, which indicates where the integer value will be inserted.
- The formatted string also contains the newline escape sequence (\n), which shifts the cursor to the next line.
- The num variable is provided as an argument to the printf() function, which replaces the %d format specifier with the value of num.
- Finally, the return 0 statement signifies a successful execution of the program, and the program terminates.
Floating Format Specifier In C ( %f )
This format specifier is used to print floating-point numbers, that is, numbers with a fractional part like 2.345343 or 3.14159, etc. The format specifier symbol %f, when used inside the formatted string for input and output, instructs the function to replace it with a floating-point value. Let's look at an example of this.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKZmxvYXQgbnVtID0gMy4xNDE1OTsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgdGhlIG51bSBpcyAlZlxuIiwgbnVtKTsKCnJldHVybiAwOwp9
Output:
The value of the num is 3.141590
Explanation:
In the C program example above-
- We once again include the stdio.h header file and start the main() function.
- Then, we declare a variable, called num, of floating-point data type and initialize it with the value 3.14159. This value represents the mathematical constant π (pi).
- Next, we use the printf() function to display a message on the console, where the message includes the format specifier %f, indicating that a floating-point number will be inserted in its place.
- The variable num is passed as an argument to the printf function. The %f format specifier is replaced with the value of num.
Character Format Specifier In C ( %c )
The %c format specifier in C is used to print an individual character in a program. It specifies that the value to be printed is of character data type, i.e., single letters, digits, or symbols.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKY2hhciBsZXR0ZXIgPSAnQSc7CnByaW50ZigiVGhlIHZhbHVlIG9mIHRoZSBsZXR0ZXIgaXMgJWNcbiIsIGxldHRlcik7CgpyZXR1cm4gMDsKfQ==
Output:
The value of the letter is A
Explanation:
In the example C program-
- We declare a character variable called letter and initialize it with the value 'A', inside the main() function.
- Then, we use the printf() function to display the value of the letter using the %c format specifier, which formats the character value as a single character.
Strings Format Specifier In C ( %s )
Strings in C differ from characters in that they are a sequence of characters as opposed to a single character. The format specifier %s is used in C to print strings literals, which are arrays of characters terminated by a null character (\0).
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKY2hhciBzdHJbXSA9ICJIZWxsbywgd29ybGQhIjsKcHJpbnRmKCJUaGUgdmFsdWUgb2Ygc3RyIGlzICVzXG4iLCBzdHIpOwoKcmV0dXJuIDA7Cn0=
Output:
The value of str is Hello, world!
Explanation:
In the C code example-
- Inside the main() function, we declare a character array str and initialize it with the string Hello, world!
- We then use the printf() function to print a message with the value of the string.
- The message is enclosed in double quotes and contains a format specifier %s, indicating that a string (character array) will be inserted in its place.
- The str variable is passed as an argument to the printf function. The %s format specifier is replaced with the value of str.
- Finally, the return 0 statement signifies that the program has been executed successfully, and it exits with a return code of 0.
Pointers Format Specifier In C ( %p )
A pointer is a variable that stores the memory address of another variable it is pointing to. Given its unique nature, we use a separate format specifier, i.e., %p, to refer to it inside input-output operations. In other words, the %p format specifier is used to print the memory address of a variable.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IG51bSA9IDEwOwppbnQgKnB0ciA9ICZudW07IC8vQXNzaWduaW5nIGFkZHJlc3Mgb2YgbnVtIHZhcmlhYmxlIHRvIHBvaW50ZXIgcHRyCnByaW50ZigiVGhlIHZhbHVlIG9mIHB0ciBpcyAlcFxuIiwgcHRyKTsKCnJldHVybiAwOwp9
Output:
The value of ptr is 0x7ffcc90b2914
Explanation:
In the example C code-
- Inside the main() function, we declare an integer variable num and initialize it with the value 10.
- As mentioned in the code comment, we declare a pointer variable, ptr, i.e., a pointer to an integer (int *), and assign the memory address of num to it using the address-of operator (&).
- Then, we use the printf() function to display a message and the address of the num on the console.
- The message includes a format specifier %p, indicating that a pointer's address will be inserted in its place.
- The ptr variable, which holds the memory address of num, is passed as an argument to the printf function. The %p format specifier is replaced with the memory address held by ptr.
Long Integers (%ld) Format Specifier In C
The %ld format specifier is used to print integer values in C, which are larger than the standard integer size. Below is an example of the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKbG9uZyBudW0gPSAxMjM0NTY3ODkwOwpwcmludGYoIlRoZSB2YWx1ZSBvZiBudW0gaXMgJWxkXG4iLCBudW0pOwoKcmV0dXJuIDA7Cn0=
Output:
The value of num is 1234567890
Explanation:
In the sample C program-
- We declare a long integer variable x and initialize it with the value 123456789 inside the main() function.
- Then, we print the value of x to the console using the printf() function and the %ld format specifier, which formats the long integer value as a decimal integer.
- The num variable is passed as an argument to printf, and the %ld format specifier is replaced with the value of num.
Unsigned Integers Format Specifier In C (%u)
The %u format specifier is used to print unsigned decimal integers in C. It is a placeholder for representing an unsigned integer value.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKdW5zaWduZWQgaW50IHggPSA0MjsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgeCBpcyAldVxuIiwgeCk7CgpyZXR1cm4gMDsKfQ==
Output:
The value of x is 42
Explanation:
In the C program sample-
- Inside the main() function, we first declare an unsigned integer variable x and then initialize it with the value 42.
- We then use the printf() function to display the value of x variable using the format specifier %u, indicating where the unsigned integer value will be inserted.
- The value of x (42) is passed as an argument to printf, and the %u format specifier is replaced with the unsigned integer value.
Unsigned Long Integer (%lu) Format Specifier In C
The %lu format specifier is used to print unsigned long integer values in C. It is similar to the %ld specifier but for long unsigned decimal integers. That is, this format specifier is used to print unsigned integer values that are larger than the standard integer size.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKdW5zaWduZWQgbG9uZyBudW0gPSAxMjM0NTY3ODkwOwpwcmludGYoIlRoZSB2YWx1ZSBvZiBudW0gaXMgJWx1XG4iLCBudW0pOwoKcmV0dXJuIDA7Cn0=
Output:
The value of num is 1234567890
Explanation:
In the sample C code-
- We declare an unsigned long integer variable num inside the main() function and initialize it with the value 1234567890.
- We then print this value with a message to the console using the printf() function.
- In the message, we use the %lu format specifier to indicate that an unsigned long integer value will be inserted in its place.
- The num variable is passed as an argument to the printf function. The %lu format specifier is replaced with the value of num.
- When the program is executed, the output will display 'The value of num is 1234567890', showing the value of num as an unsigned long integer.
Long Long Integers Format Specifier In C ( %lld )
Sometimes, you might need to print/ use extra long numbers in a program. The %lld format specifier is used in C to print signed long long integer values in input-output operations with ease. These are larger than regular long integers, providing a greater range of values.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKbG9uZyBsb25nIG51bSA9IDEyMzQ1Njc4OTAxMjM0NTsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgbnVtIGlzICVsbGRcbiIsIG51bSk7CgpyZXR1cm4gMDsKfQ==
Output:
The value of num is 123456789012345
Explanation:
In the C code sample-
- In the main() function, we declare and initialize a long long integer variable num to the value 123456789012345.
- Then, we use the printf() function to display a message on the console, with the format specifier %lld enclosed in the double quotes, indicating that a long long integer will be inserted in its place.
- The value of num is passed as an argument to printf, and the %lld format specifier is replaced with the value of num.
- The return 0 statement marks a successful program execution.
Unsigned Long Long Integers ( %llu )
This format specifier can be considered a variation of the %lld specifier because it is used to specify that the value is larger than a long decimal integer. The only difference is that the %llu specifier is used for unsigned integer values that are larger than unsigned long integers.
The %llu format specifier is used to print unsigned long long integer values in C, allowing for even larger values than unsigned long integers.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKdW5zaWduZWQgbG9uZyBsb25nIG51bSA9IDEyMzQ1Njc4OTAxMjM0NTsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgbnVtIGlzICVsbHVcbiIsIG51bSk7CgpyZXR1cm4gMDsKfQ==
Output:
The value of num is 123456789012345
Explanation:
- Inside the main() function, we declare and initialize an unsigned long long integer variable called num with the value 123456789012345.
- Then, using the printf() function, we display a message on the console, with the format specifier %llu, to indicate that an unsigned long long integer value will be inserted in its place.
- The value of num is passed as an argument to printf, and the %llu format specifier is replaced with the value of num.
Hexadecimal Integers Format Specifier In C (%x)
The %x and %X format specifiers are used to print integer values in hexadecimal form, which uses the digits 0-9 and A-F to represent values from 0 to 15. The specifier is %x for lowercase letters and %X for uppercase alphabets.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IG51bSA9IDI1NTsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgbnVtIGlzICV4XG4iLCBudW0pOwoKcmV0dXJuIDA7Cn0=
Output:
The value of num is ff
Explanation:
- Inside the main() function, we declare an integer variable num and initialize it with the value 255.
- We then use the printf() function to display value of num in hexadecimal format using the %x format specifier, indicating that an integer value will be inserted in its hexadecimal representation.
- The value of num (which is 255 in decimal) is passed to printf(), and the %x format specifier is replaced with the hexadecimal value ff.
Note- If the notation were to be %2x, then it would indicate to the compiler that the value should be printed in hexadecimal form, with at least two characters (or padded with at least two characters). Also, if %X were used instead, the output would be FF instead of ff.
Scientific Notation Format Specifiers ( %e or %E )
The %e and %E format specifiers are used to print floating-point numbers in scientific notation (exponential notation), where the format is mantissa * 10^exponent. The character 'e' represents the exponential power, which here, by default, is considered to be 10. It is a placeholder for the corresponding argument that represents a floating-point value.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKZG91YmxlIHggPSAxMjM0LjU2Nzg5OwpwcmludGYoIlRoZSB2YWx1ZSBvZiB4IGluIHNjaWVudGlmaWMgbm90YXRpb24gaXMgJWVcbiIsIHgpOwoKcmV0dXJuIDA7Cn0=
Output:
The value of x in scientific notation is 1.234568e+03
Explanation:
- We declare a double-precision floating-point variable x in the main() function, and initialize it with the value 1234.56789.
- Then, we use the printf() function to output a message with the value for the variable x. The message contains the format specifier %e, indicating that a floating-point number will be inserted in scientific (exponential) notation.
- The value of x (1234.56789) is passed as an argument to printf, and the %e format specifier is replaced with the value in scientific notation, which might look like 1.234568e+03.
Uppercase Variant: If %E were used instead, the output would be in the form 1.234568E+03.
Octal Integers Format Specifier In C (%o)
The %o format specifier is used to print unsigned octal integer numbers/ values as input in functions. It is used when working with octal representation (base-8) of numbers.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHggPSA0MjsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgeCBpbiBvY3RhbCBpcyAlb1xuIiwgeCk7CgpyZXR1cm4gMDsKfQ==
Output:
The value of x in octal is 52
Explanation:
- We declare an integer variable x and initialize it with the value 42.
- Then, we use the printf() function to display the value of x in an octal format using the %o format specifier.
- The decimal value 42 is represented as 52 in octal, which is printed in the output.
Short Integers Format Specifiers ( %hd )
We know the %i or %d specifiers that print integer values. Then what is this for?
The %hd format specifier is used to print short integer values in C. The h indicates that the value is short, and the d specifies that it is a short integer.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKc2hvcnQgaW50IHggPSAzMjc2NzsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgeCBpcyAlaGRcbiIsIHgpOwoKcmV0dXJuIDA7Cn0=
Output:
The value of x is 32767
Explanation:
- We begin the example above by including the standard input-output library and initiating the main() function.
- Inside the main, we declare a short integer variable x and initialize it by assigning a value 32767. Note that short int data types have a limited range compared to regular integers.
- Next, we use the printf() function to display a message on the console, with the format specifier %hd, indicating that a short integer value will be inserted.
- The value of x (32767) is passed as an argument to printf, and the %hd format specifier is replaced with the short integer value.
- Finally, the return 0 statement signifies a successful program execution.
Check out this amazing course to become the best version of the C programmer you can be.
Unsigned Short Integers Format Specifier ( %hu )
This format specifier is a variation of the short integer format specifier in C we discussed above. The %hu specifier is used to format and print unsigned short integers in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKdW5zaWduZWQgc2hvcnQgaW50IHggPSA2NTUzNTsKcHJpbnRmKCJUaGUgdmFsdWUgb2YgeCBpcyAlaHVcbiIsIHgpOwoKcmV0dXJuIDA7Cn0=
Output:
The value of x is 65535
Explanation:
- In the main() function of the C program above, we declare an unsigned short integer variable x and initialize it with the value 65535.
- We then use the printf() function and pass the value of x to it as an argument.
- The function prints a message to the console containing the format specifier %hu, indicating that an unsigned short integer value will be inserted in that place.
- When the program is executed, the value 65535 is printed as-is, since it’s within the range of unsigned short integers.
Printing Error Message Format Specifier In C (%m)
The %m format specifier is used to print the error message corresponding to the value of errno in C. Note that this specifier is specific to the GNU C library and is not standard C, which can affect portability.
This is not a valid specifier in C, but since C89/90, using %m in a formatted string for functions acts as the placeholder for the result of an implied strerror(erno) call. So, the value inserted in its place is the text string/ error message corresponding to the current value of the errno variable (Linux std error code).
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxlcnJuby5oPgoKaW50IG1haW4oKSB7CkZJTEUgKmZwOwpmcCA9IGZvcGVuKCJub25leGlzdGVudGZpbGUudHh0IiwgInIiKTsKaWYgKGZwID09IE5VTEwpIHsKcHJpbnRmKCJFcnJvciBvcGVuaW5nIGZpbGU6ICVtXG4iKTsKcmV0dXJuIDE7fQpmY2xvc2UoZnApOwoKcmV0dXJuIDA7Cn0=
Output:
Error opening file: No such file or directory
Explanation:
- In the above code, we have tried to open a file that does not exist using the fopen() function.
- As the file does not exist, fopen will return NULL and the errno variable is automatically set to ENOENT, which indicates "No such file or directory".
- We have used the %m format specifier to print the error message corresponding to the value of errno.
- The program returns 1 to indicate an error condition, and the error message is displayed.
The %n Format Specifier In C
This is a special format specifier whose broad purpose is to print the number of characters read/ displayed up until the %n appears. In other words, the %n format specifier is used to store the number of characters printed so far into an integer variable.
In this sense, when used with the printf() function, the specifier loads the variable pointed to by the argument and equates its value to the number of characters before occurrence of %n.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHgsIHk7CnByaW50ZigiVGhpcyBpcyBhIHNhbXBsZSBzdHJpbmclblxuIiwgJngpOwpwcmludGYoIlRoZSBudW1iZXIgb2YgY2hhcmFjdGVycyBwcmludGVkIGJlZm9yZSB0aGlzIGlzICVkXG4iLCB4KTsKcHJpbnRmKCJUaGlzIGlzIGFub3RoZXIgc2FtcGxlIHN0cmluZyVuXG4iLCAmeSk7CnByaW50ZigiVGhlIG51bWJlciBvZiBjaGFyYWN0ZXJzIHByaW50ZWQgYmVmb3JlIHRoaXMgaXMgJWRcbiIsIHkpOwoKcmV0dXJuIDA7Cn0=
Output :
This is a sample string
The number of characters printed before this is 23
This is another sample string
The number of characters printed before this is 29
Explanation:
- We first declare two integer variables x and y, inside the main() function using the comma operator.
- Next, we use the printf() function to print the string- This is a sample string, with the %n format specifier.
- As a result, the %n specifier format specifier counts the number of characters printed up until its occurrence and stores that count in the specified variable, here variable x, using the address-of operator.
- Next, we use printf() to display another phrase along with the value for x, where the %d is replaced with the value of x as counted by %n earlier.
- The process is repeated, where we print another phrase using printf(). The %n specifier counts the characters and this time stores the value in the variable y.
- Finally, we print the value of y, indicating the number of characters printed for the second string.
What Is Double Format Specifier In C?
In C, the double data type is used for storing high-precision floating-point numbers. It is named double because it typically holds twice the size of data compared to the float data type. A double in C ranges between 1.7E-308 - 1.7E+308, and it can store decimal numbers, real numbers, negative values, etc. Doubles provide greater precision and can represent a wider range of values, making them essential in applications requiring accurate and large-scale numerical computations.
Some key characteristics of the double data type in C are as follows:
- Size: Typically, a double type is stored in 8 bytes (64 bits) of memory, providing greater precision compared to the float data type, which is usually 4 bytes (32 bits).
- Precision: It offers double precision, representing values with a greater number of significant digits, typically about 15-17 significant digits. This precision makes them suitable for scientific calculations where accuracy is critical.
- Range: Due to its increased precision, the double type can represent a wider range of values, both very large and very small, compared to the float type.
- Suffix: In C, you can specify a double type constant by adding the double suffix d or D, though this is optional. For example, you can write a double constant like 3.14159265359 or 3.14159265359D.
- Usage: The double is commonly used in applications where precision is essential, such as scientific calculations, financial software, and engineering simulations. It is also the default floating-point type for many C functions and libraries.
Syntax For Declaration & Initialization
To declare and initialize a double variable in C, you must use the following syntax:
double variable_name = initial_value;
Here, the double keyword marks the type of the variable whose name is given by the identifier variable_name, and the value assigned is given by intial_value.
Representation Of Double In C
Double-precision floating-point numbers (doubles) are typically represented using 64 bits of memory according to the IEEE 754 standard. This standard defines the format for representing doubles, which includes a sign bit, an exponent, and a significant (mantissa) part.
- Sign bit (1 bit): Indicates whether the number is positive (0) or negative (1).
- Exponent (11 bits): Represents the power of 2 used to scale the mantissa.
- Mantissa (52 bits): Stores the significant digits of the number.
This representation allows doubles to store values with higher precision and a wider range than single-precision floats.
Here's an example of a double's binary representation (simplified for illustration):
0 | 10000000000 | 1100000000000000000000000000000000000000000000000000000
^ Exponent ^ Mantissa
Here, the sign bit (0) indicates that the number is positive, the exponent (10000000000) represents the power of 2 used to scale the mantissa which stores the significant digits of the number.
How To Print Double Value In C?
You can print the value of a double variable using the printf() function with the %f format specifier, which is used for formatting floating-point numbers. For example:
double num = 123.456;
printf("The value of num is: %f\n", num);
Example Of Double In C
Below is an example of using a double variable to calculate the area of a circle using the radius and then using the %f format specifier to print the value with double precision.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKZG91YmxlIHJhZGl1cyA9IDUuMDsKZG91YmxlIGFyZWEgPSAzLjE0MTU5MjY1MzU5ICogcmFkaXVzICogcmFkaXVzOwoKcHJpbnRmKCJUaGUgYXJlYSBvZiB0aGUgY2lyY2xlIGlzOiAlZlxuIiwgYXJlYSk7CgpyZXR1cm4gMDsKfQ==
Output:
The area of the circle is: 78.539816
Explanation:
In the example-
- Inside the main() function, we declare two double variables, radius and area with the former intialised to the value of 5.
- We calculate the area variable representing the area of the circle by multiplying π (approximately 3.14159265359) by the square of the radius.
- Next, we use the printf() function to display the calculated area with the %f format specifier, which formats the double as a floating-point number.
- Finally, the return 0 statement signifies a successful program execution.
This example illustrates the power and flexibility of the double data type in handling precise and complex numerical computations in C.
Float Data Type Vs. Double Data Type In C
In C programming, both float and double are used to store floating-point numbers, but they differ in precision, range, memory usage, and computational efficiency. Here’s a comparison of these two data types:
Aspect | float | double |
---|---|---|
Data Type Size | Typically 4 bytes | Typically 8 bytes |
Precision | Single precision float number with 6-9 significant digits | Double-precision, 15-17 significant digits |
Range | Limited range compared to double | Larger range compared to float |
Memory Usage | Less memory is required | More memory is required |
Default Suffix | Default is f (e.g., 3.14f) | Default is either none or d (e.g., 3.14 or 3.14d) |
Use Case | Suitable for most common use cases | Used for high-precision calculations |
Computational Speed | Generally faster due to smaller size | Slightly slower due to the large size |
Portability | Provides faster calculations on some platforms | Provides greater precision on all platforms |
Example | float pi = 3.14159f; | double pi = 3.14159265359; |
Why Should I Use Format Specifiers In C?
Format specifiers in C are essential for handling input and output operations. They serve as placeholders in formatted strings, ensuring that the data is correctly interpreted and displayed. Here’s why they are important: Some key reasons why format specifiers are used in C are as follows-
- Data Type Specification: Format specifiers in C specify the expected data type during input and output operations, ensuring that the program interprets and handles the data correctly.
- Type Safety: They enhance type safety by enforcing consistency between the format string and the actual data. This reduces the risk of type-related errors, such as passing a float where an integer is expected.
- Data Formatting: Format specifiers in C allow for the precise formatting of data, enabling developers to control how numbers, dates, and other values are displayed. For instance, you can control the number of decimal places displayed in a floating-point number using %.2f.
- Internationalization and Localization: They facilitate internationalization and localization by adapting data presentation to different regions and languages, accounting for variations in numeric formats and character encoding.
- Code Clarity: Format specifiers in C make code more readable and maintainable by clearly indicating the expected data types and how they should be formatted.
- Error Prevention: Proper use of format specifiers in C helps prevent runtime errors, which can lead to program crashes or unexpected behavior. For example, format mismatches, invalid type casting/ conversions, or data-related issues.
- Security: Correct use of format specifiers contributes to program security by preventing vulnerabilities such as format string attacks, where an attacker could exploit a poorly formatted string to execute arbitrary code.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
Format specifiers are a fundamental concept in C programming as they play a crucial role in handling input and output operations. Understanding the use of format specifiers in C is essential for displaying and reading data accurately in your C programs. By using the correct format specifier for each data type, you can ensure that your programs work as expected and produce well-formatted output.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What are format specifiers in C?
Format specifiers in C are placeholders used in functions like printf() and scanf() to specify the format and type of data to be displayed or read. They allow programmers to control the formatting of input and output operations. For example, when we want to print an extra-long decimal integer value, we must use the format specifier %lld inside the function. This indicates that the value to be replaced is a long long integer.
long long num = 2342345435646532431232; \\declaring and initializing a long long variable
printf("The value of num is %lld\n", num);
Q. Why are format specifiers important in C programming?
As the name suggests, the format specifiers specify the type of value stored inside a variable. It is essential to use these specifiers in the program because-
- Format specifiers are essential for accurate and controlled data formatting.
- They ensure that data is displayed or read in the correct format and type, preventing errors and ensuring proper data handling.
- Format specifiers also enable localization and internationalization efforts, making software adaptable to different languages and regions.
- Properly used format specifiers contribute to program security.
- Format specifiers help prevent errors by enforcing consistency between the format string and the actual data.
Q. What are some common format specifiers in C?
In C, format specifiers are used to specify the data type when displaying or reading data using functions like printf() and scanf(). Here are some common format specifiers-
- %d: Represents a signed decimal integer.
- %u: Represents an unsigned decimal integer.
- %f: Represents a floating-point number in decimal notation.
- %lf: Represents a double-precision floating-point number.
- %c: Represents a character.
- %s: Represents a string (null-terminated array of characters).
- %p: Represents a pointer address.
- %x: Represents an integer in hexadecimal notation.
- %o: Represents an integer in octal notation.
- %ld: Represents a long integer.
- %lu: Represents an unsigned long integer.
- %lld: Represents a long long integer.
- %llu: Represents an unsigned long long integer.
Q. How do format specifiers in C ensure type-checking and error prevention?
Format specifiers in C play a crucial role in ensuring type-checking and error prevention during input and output operations.
- When using format specifiers in functions like scanf(), they specify the expected data type for the input.
- This ensures that the data provided by the user or a file is of the correct type, preventing unintended type-related errors, such as attempting to read a string into an integer variable.
- Likewise, when using format specifiers in printf(), they dictate how data should be displayed, helping to avoid formatting errors and mismatches between the format string and the actual data, which can lead to runtime issues.
In conclusion, format specifiers in C contribute to safer and more reliable programs/ code by enforcing type consistency and formatting.
Q. Can format specifiers be modified for localization and internationalization in C?
In C programming, format specifiers serve as placeholders for data types, instructing how to format and display values in functions like printf() and scanf(). While format specifiers themselves don't directly handle localization and internationalization, they play a pivotal role within the broader strategy for achieving these goals.
- For internationalization, C developers often employ internationalization libraries like GNU gettext or ICU, and they set the locale using setlocale to accommodate different cultural and linguistic preferences.
- Format specifiers come into play when formatting numeric, date, and time values, ensuring that these values are presented in a manner consistent with the user's chosen locale.
Thus, format specifiers in C, when used in conjunction with internationalization techniques, contribute to the development of software that can seamlessly adapt to diverse languages and regions, providing a more inclusive and user-friendly experience.
Q. How should format specifiers in C be used for professional software development and SEO?
Format specifiers have various benefits, like making the code more manageable, clearer, and concise. Here are a few additional benefits of using format specifiers in professional software development:
- Code Readability and Maintainability: Proper use of format specifiers in your code enhances readability. When other developers review or work on your code, well-formatted output and input statements with correct format specifiers make it easier for them to understand the program's logic and data handling.
- Data Validation: Using the correct format specifiers in C programs is crucial for ensuring data integrity and preventing errors. In professional software development, data validation and error handling are critical to delivering reliable and secure software. Format specifiers help ensure that the right data types are processed during input operations, reducing the risk of runtime errors.
- Internationalization and Localization: In global software development, using format specifiers correctly is important for internationalization (i18n) and localization (l10n). Different languages and regions have specific formatting requirements for numbers, dates, and currencies. Properly utilizing format specifiers allows for easier adaptation to various locales.
Some benefits of using format specifiers in C from the SEO (Search Engine Optimization) point of view are-
-
Page Load Times: In web development, efficient coding practices can affect page load times. If your code is optimized and well-structured, it can lead to shorter loading times. This indirectly contributes to a better user experience, and Google's search algorithms consider page speed as a ranking factor.
-
Structured Data Markup: While not format specifiers in the traditional sense, structured data markup (e.g., Schema.org) helps search engines understand your content better. Properly structured data can enhance how your content appears in search engine results, potentially increasing click-through rates.
-
User Experience: When you adhere to good coding practices (format specifiers included) it produces a well-structured and user-friendly website, thus reducing bounce rates and improving user engagement. These factors are indirectly considered by search engines as signals for ranking.
-
Content Quality: High-quality content is a critical aspect of SEO. Maintaining high-quality content can be facilitated by using programming best practices, which include clear and well-structured code and are indirectly related to the correct use of format specifiers in C or other programming languages.
You might also be interested in reading:
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
- Logical Operators In C (AND, OR, NOT, XOR) With Code Examples
- Control Statements In C | The Beginner's Guide (With Examples)
- Recursion In C | Components, Working, Types & More (+Examples)
- Union In C | Declare, Initialize, Access Member & More (Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment