Functions In C | Uses, Types, Components & More (+Code Examples)
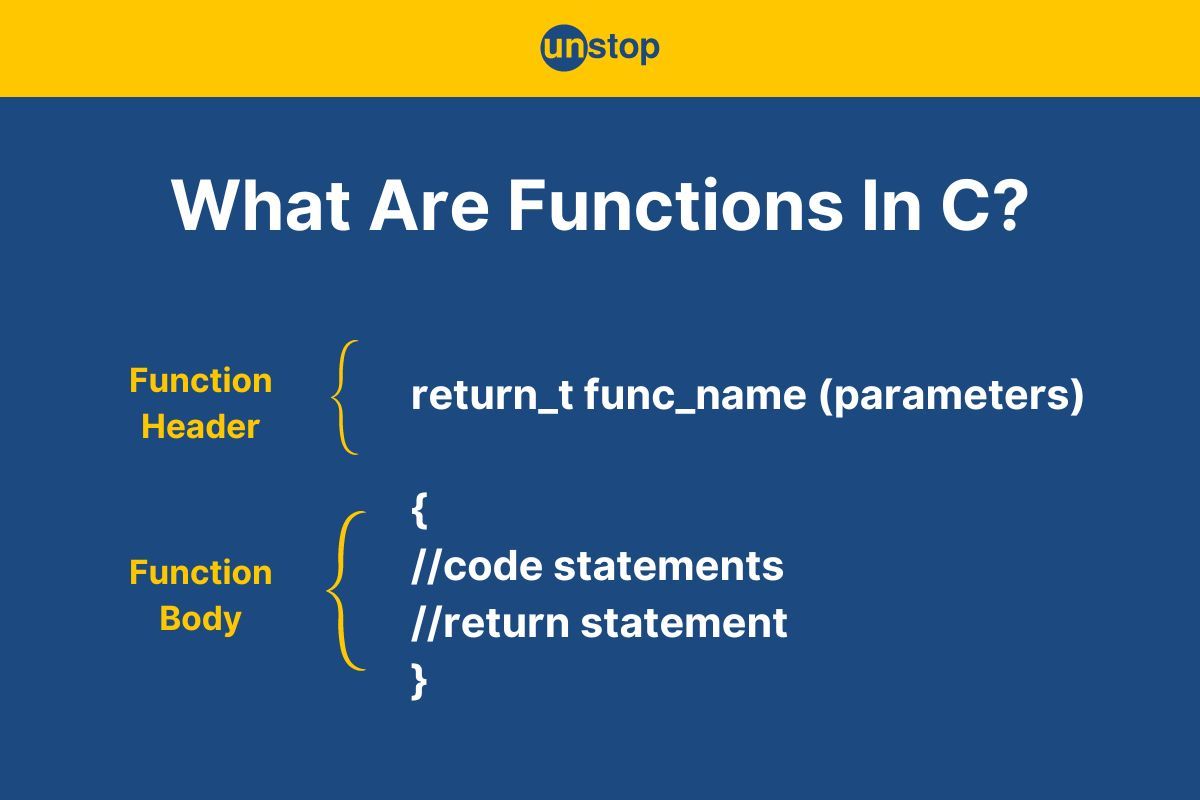
In simplest possible terms, functions are building blocks of a program/ code. They allow us to break complex tasks in C programs into small, meaningful, and reusable blocks of code. In the C programming language, functions play a crucial role in making programs modular, organized, less repetitive, and efficient. In this article, we will cover all that you should know about functions in C with examples and proper explanations. Let’s start with understanding what a function in C is.
What Is A Function In C?
A function is a self-enclosed block of code that performs a specific task. As mentioned before, it allows us to break down a complex program into smaller and reusable pieces of code holding proper meaning. For example, every C program has at least one function, which is the main() function. It acts as the entry point of the program's execution and differentiates any structure definitions or library inclusions from the executable code.
The key purpose of using functions is it allows modularity and reusability in the program. Instead of writing the same code multiple times for different arguments, we can write a function and call it multiple times, passing various arguments to it. Also, writing code assigning various tasks to each function makes debugging easy and code modular.
Why Do We Need Functions In C Programming?
Functions in C serve several purposes, making them fit for writing efficient and easy-to-read code. Here are some of the reasons why we need functions in t:
- They help organize code logically, thus enhancing the program's overall code structure.
- Well-structured code with functions is easier to read and understand.
- Functions can be optimized individually, leading to more efficient code.
- They help break down an extensive program into smaller and manageable pieces.
- Functions in C programs make debugging and troubleshooting easy to detect and fix.
- They enable reusability and reduce redundancy.
Now that we have a basic idea of what a function in C is and why it is important, let's move on to discuss their implementation in C programs.
How To Create & Execute A Function In C?
The purpose of function in C programming, as mentioned before, is to create a block of code that can be used over and over again without the need to rewrite the code. And also to break bigger problems into smaller tasks. The creation and execution of a function in C comprises three parts, namely, the function declaration, function definition, and function call.
In other words, there are three major components/ aspects associated with functions in C. We have listed them in the table below:
Aspects of Function |
Syntax of Function |
Function Declaration |
return_type function_name(parameters list); |
Function Definition |
return_type function_name(parameters list) {function’s body;} |
Function Calling |
function_name(parameters list) |
We will discuss all of these components of function in C individually, but first, let's take a look at a simple C program that shows the implementation of a function to calculate the sum of two integer values.
Code Example:
Output:
Addition of a & b is: 8
Explanation:
In the code example above, we begin by including the <stdio.h> header file for input/ output operations.
- As mentioned in the o, we then define a function addition with return type void.
- The function takes two integer data type variables a and b as paramters.
- It calculates the sum of the two variables using the addition arithmetic operator and assigns the result to variable c.
- We then have a printf() statement, which displays a formatted string with the sum value. Here, the %d format specifier is the placeholder for the integer value.
- Inside the main() function, we initialize two integer variables, a and b, with values 3 and 5, respectively.
- Next, we call the addition() function by passing the variables a and b as arguments.
- This invokes the function to calculate the sum and print the same to the console.
- After executing the addition() function, the flow of control returns to the main() function, and the code terminates.
How To Declare Of A Function In C?
In C, a function needs to be declared before it's used. Declaring a function’s signature before its implementation informs the compiler about its existence, its name, parameters, and return type, enabling you to call it before defining it. The function's name and the list of parameters it takes are also referred to as the function's declaration.
Syntax for Declaration of Function In C:
return_type function_name( parameter list ); // Declaration of function
Here,
- The return_type refers to the data type of the function's return value.
- The parameter list refers to the list of parameters for the function whose name is given by function_name.
It is important to note that the parameter names are not crucial while declaring functions. We can declare it only using the variable's data type without the name. Given below is a short example of function declaration in C programming language.
Example:
#include<stdio.h>
int addition(int a, int b); // Declaration of function
int addition(int, int); // Alternate declaration
Explanation:
In the short example above-
- The return type is int, i.e., the function will return an integer value.
- The function name is addition, which has two integer parameters, i.e., int a and int b.
- There is an alternate declaration made where the parameter name is missing. You can declare a function like this as well.
- Finally, the semicolon marks the end of the declaration.
Now, let’s look at defining and calling a function to invoke the function for its execution.
How To Define A Function In C?
When we say defining a function in a C program, it refers to writing the basic structure of the function along with its logic. This function definition consists of the name of the function for its identification, the values/ variables that we can pass to it (i.e., parameters), the operation or manipulation that the function will perform on the parameters, and the type of value it will return. In other words, the function definition contains the actual working of the function (i.e., statement(s) inside the function’s body).
Generally, a function in C is declared and defined in a single step combined. A function definition already contains the function declaration, so we do not need to declare it explicitly. However, it also is possible to declare a function without giving its definition.
The function definition or the structure of the function in C can be broken into two parts, i.e., the function header and function body., as shown in the image above. Let's see how this translates in the syntax.
The Basic Syntax Of A Function Definition In C
return_type function_name( parameter list ){
// body of the function
}
Here,
- The function header consists of the return type, function name, and parameters list.
- Return type is the data type of the value that the function returns. The return type can be void if no value returns from the function.
- The function_name is the actual name of the function used to make the function call.
- Parameters are the placeholders for catching the value(s) passed to the function. These values are the arguments. Parameters are optional, so a function may not contain any parameters.
- The body of the function contains statements that define the logic behind the function and its functioning. And they mostly close with a return statement.
Note: When declaring and defining a function jointly, you need to define the function before the point of its call in the program. Now, let's take a look at an example that shows how to both declare and define a function in a C program.
Example:
#include<stdio.h>
int addition(int a, int b){
int c = a + b;
return c;}
Explanation:
Here,
- The line int addition(int a, int b) is the function declaration, where the return type is an integer, and the name of the function is addition.
- The function body is the part where we declare a variable c, which holds the sum of the values of a and b, i.e., int c = a + b.
- Lastly, the function returns the value of c, i.e., return c.
- All of these combined make up the function's definition.
How To Call A Function In C?
To use a function, we need to call the function by its name and by passing the required arguments. This call invokes the function and executes it. After execution, the function’s returned value is stored in a variable or used directly. Functions with void return type do not return any value after their execution.
Syntax For Function Call In C:
function_name(arguments);
Here, we call the function by naming it and passing the arguments for the parameters. We know how to declare, define, and call a function in C. Let’s understand it better with a complete code example that combines all three aspects.
Code Example:
Output:
Multiplication of x and y is: 135
Explanation:
In the code example-
- We define a function multiply with return type integer.
- The function takes two parameters, i.e., integer variables a and b, and calculates their product. The outcome is stored in variable c, which the function returns.
- Then, inside the main() function, we declare and initialize two integer variables, x and y, with values 15 and 9, respectively.
- Next, we call the multiply() function by passing x and y as arguments.
- This invokes the function which calculates the product and returns the value back to the main() function.
- We then store the product value in the variable ans and display the value using the printf() function.
- Finally, the main() function terminates with a return 0 statement indicating no errors.
How Does C Function Work?
Working of the C function can be broken into the steps as mentioned below:
- Declaring a function: Declaring a function is a step where we declare the function's existence for the compiler. This consists of defining the return types, name, and parameters of the function.
- Defining a function: After declaring a function, we define it by providing the actual implementation of the function. The definition includes the body of the function, which contains the set of statements and operations to be executed when the function is called.
- Calling the function: Calling the function is a step where we call the function by passing the arguments to it.
- Executing the function: Executing the function is a step where the compiler runs all the statements inside the function to get the final result.
- Returning a value: Returning a value is the step where the calculated value after the execution of the function is returned. Exiting the function is the final step, where all the allocated memory to the variables, functions, etc., is destroyed before giving full control back to the main function.
For a clear understanding of the working of functions in C, let’s consider a simple scenario where we want to calculate the area of a rectangle. We can create a function taking its dimensions as arguments, do the calculations, and return the area. Below is an example where we show the implementation of the same, but the function definition is given after the main() function.
Code Example:
Output:
Enter the length of the rectangle: 6
Enter the width of the rectangle: 8
The area of the rectangle is: 48.00
Explanation:
We begin by including the standard input-output library (stdio.h) to enable the use of functions like printf and scanf.
- Next, we declare a function named Area that takes two parameters, both of type float and returns a float value. This function is intended to calculate the area of a rectangle.
- In the main() function, we declare two float variables, length, and width, which will store the dimensions of the rectangle.
- We then prompt the user to input the length and width of the rectangle using the printf() function and store the values in respective variables using the scanf() function with the address-of operator.
- Next, we make a function call to Area() with the provided length and width values, storing the result in an int variable named area. It's important to note that the variable type should be float to correctly store the result.
- Finally, we use the printf() function to display the calculated area with two decimal places, and the program concludes by returning 0 from the main function, indicating successful execution.
- The actual definition of the Area() function comes after the main function. In this function, the area of the rectangle is computed using the provided length and width, and the result is returned.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Types Of Functions In C
Function in C and many other programming languages can be categorized into two broad categories based on their origin. These two types of functions in C are Standard library functions and User-defined functions. Let's see what these function types entail.
Standard Library Functions In C
Standard or library functions are pre-defined functions provided by the C standard library. These functions offer various functionalities, from input/output operations to mathematical calculations. They can be easily accessed by including the relevant header files in the program.
When we say that these functions are already defined in the C libraries, it means you do not have to define them explicitly. However, you must include the relevant header file at the top of our program to access them. Also, you need to use the proper syntax of the function to call them. E.g., printf(), scanf(), strlen(), floor(), pow(), etc. are library functions.
Syntax:
#include<header_file> // Include header file to use library functions
int main() {
// Using library functions from the header file
return 0;}
As shown in the syntax, we begin by including the header file at the beginning of the program and then use the required function inside the main() function. Below is an example that shows how to use the printf() function from <stdio.h> header file to print the simple Hello, World! message on the screen
Code Example:
Output:
Hello, World!
Explanation:
- First, we import the standard input-output library (stdio.h) using #include<stdio.h>. This allows us to use functions like printf in our program.
- Then, inside the main() function, we use the printf function to display the string message- Hello, World! on the console, i.e., printf("Hello, World!\n").
- Note that the newline escape sequence, i.e., (\n), creates a newline for formatting.
- To conclude the main function, we have the return 0 statement which signals successful execution of the program.
List Of Header Files & Commonly Used Library Functions In C
Header File |
Key Functions |
Description |
<stdio.h> |
printf(), scanf(), getchar(), putchar(), fgets() |
Input/output functions for formatted text and characters. |
<stdlib.h> |
malloc(), free(), exit() |
Functions for memory allocation, deallocation, and program termination. |
<string.h> |
strcpy(), strcat(), strlen(), strcmp(), strtok() |
String manipulation functions, including copying, concatenating, and tokenizing strings. |
<math.h> |
sqrt(), pow(), sin(), cos(), tan(), ceil(), floor() |
Mathematical functions for various calculations, including square roots, trigonometry, and rounding. |
<time.h> |
time(), ctime(), localtime(), strftime() |
Time and date functions, provide access to system time, formatting time, and date information. |
<ctype.h> |
isalpha(), isdigit(), isalnum(), islower(), isupper(), tolower(), toupper() |
Character classification and conversion functions for character type checking and conversion. |
<stdbool.h> |
bool, true, false |
Definitions for boolean data type and constants true and false. |
<stddef.h> |
size_t, NULL |
Definitions for data types and macros, including size_t for size values and NULL for null pointers. |
<limits.h> |
Constants specifying data type limits |
Constants indicating minimum and maximum values for different data types. |
<assert.h> |
assert() |
Macro for including assertions in the code to check assumptions during debugging. |
<stdarg.h> |
Macros and functions for variable arguments list |
Macros and functions for handling functions with a variable number of arguments. |
<errno.h> |
errno |
Global variable that holds the last error code generated by system calls. |
User-Defined Functions In C
User-defined functions are custom-made functions created by programmers to perform specific tasks. They enhance code reusability and readability by encapsulating functionality. User-defined functions are declared, defined, and then called within the program. In C programming, we can add a user-defined function to any library and use it in other programs.
The basic structure/ syntax of a user-defined function is:
return_type function_name ( parameter list ){
// statements - Body of the function
return value;
}
The syntax is the same as that for any regular function. This is probably why the terms normal function and user-defined functions are sometimes used interchangeably. Also because the working mechanism behind both functions is the same.
However, the key difference between them is that normal functions are predefined by the C standard library, while we define user-defined functions to suit specific needs. Now, let's take a look at a C code example, where we create a function to calculate the factorial of a number for a better understanding of this concept.
Code Example:
Output:
Factorial of 5 is 120
Explanation:
In the code example above,
- We define a function named factorial to calculate the factorial of a given integer n.
- This function is implemented using recursion, where it calls itself with smaller instances of the problem until it reaches a base case. Inside the function-
- We have an if-statement that uses relational and logical operators to see if the value of n is equal to 0 or 1.
- If n is 0 or 1, the function returns 1; otherwise, it returns the product of n and the factorial of n - 1.
- Next, inside the main() function, we initialize an integer variable num with the value 5.
- Then, we call the factorial() function with the argument num (5) and store the outcome in the variable result.
- We then use the printf() function to display the calculated factorial result, incorporating the values of num and result in the output message.
- Finally, the main function concludes by returning 0 to the operating system, indicating successful program execution.
Types Of C Functions Based On Arguments & Return Value
Functions in C can be of four types depending upon the arguments passed and value returns. It is evident that functions in C programming can be invoked or called with or without passing the arguments and might not return any value to the calling function. In case of not returning any value, the return_type of the function is written as void. Following are the four conditions that arise based on return type and argument.
- Functions that take arguments and return value.
- Functions that take arguments and return no value.
- Functions that take no arguments and return value.
- Functions that neither take arguments nor return value.
We will cover each and every condition with proper examples in the sections below.
Function In C With Arguments & Return Value
These functions accept arguments, use them to perform calculations and return the result to the calling function. This is the most common type of function. We generally use these functions to perform calculations, operations, and transformations on the values passed to the upon function call.
Syntax:
return_type function_name ( parameter(s) ); // function declaration
function_name (argument(s) x ); // function callreturn_type function_name( parameter(s) x ){ // function definition
// statements;
return value; // return statement
}
Let's take a look at an example of this function type, where we write a function to calculate the length of a string in C.
Code Example:
Output:
The length of the string is: 15
Explanation:
In the sample C code above,
- We define a function named length_cal responsible for determining the length of a given string.
- This function takes a character array str as a parameter. Inside the function-
- We set up two integer variables, i.e., idx and len, with an initial value of 0. These variables keep track of the current index and the length of the string.
- Then, we use a while loop to iterate through the characters of the string until we encounter the null character ('\0'), signifying the end of the string.
- Within the loop, we increment the value of len to calculate the length and advance the index idx.
- Concluding the function, we use a return statement to provide the calculated length of the string.
- Then, in the main() function, we initialize a character array str with the string value- Unstop is best!
- Next, we call length_cal() function by passing the string str as an argument. The resulting length is stored in the variable count.
- To display the outcome, we use the printf() function, incorporating the value of count into the output message.
- Finally, the main function concludes its execution by returning 0.
Function In C With Arguments But No Return Value
Functions that take arguments and do not return any value are commonly known as void functions. These functions perform actions or operations that do not require a result to be returned to the calling function. Some common uses of void functions are:
- Printing information
- Input validation
- Updating variables
- File operations and
- Modifying data structures
Syntax:
void function_name ( parameter(s) ); // function declaration
function_name ( argument(s) x); // function call
void function_name( parameter(s) x ){
// statements;
}
The primary difference in the syntax is that the return type for these functions is void. Take a look at the example below, where we write a function to update the value of a variable without returning it, for a better understanding.
Code Example:
Output:
Updated value: 6
Explanation:
In the code example above,
- We first define the void function updateValue(), which takes an integer pointer as its argument.
- The program starts executing from the main() function.
- Here, we first declare a variable value and initialize it with 5.
- We then call the updateValue() function, passing the address of the value variable as an argument.
- The function gets invoked and updates the variable's value at the passed address, and the flow of control returns to the main() function.
- Lastly, we use the printf() function to print the updated value, as seen in the output window.
Function In C With Return Value But No Arguments
Functions in C that take no arguments but return a value are commonly referred to as parameterless functions or nullary functions. These functions perform actions or calculations that don't require any external input but provide a result - for example, random number generation, counting function calls, getting date and year, etc.
Syntax:
return_type function_name (); // function declaration
function_name (); // function call
return_ type function_name() // function definition
{// statements;
return value; // return statement
}
As is evident, there is no list of parameters in the syntax above. Look at the example below of a C function to generate a random number upon rolling a die to understand this type of function in C.
Code Example:
Output:
You rolled a 5
Explanation:
In the code example above, we include the <stdio.h>, <stdlib.h>, and <time.h> libraries.
- We then define the function rollDice(), which takes no argument and returns a random number between 1 and 6.
- In the main() function, we use the srand() and time() functions to initialize the random seed each time we run the program.
- We then directly call the rollDice() function without passing any argument.
- The function gets invoked, generates a random number between 1 and 6, and returns it to the main() function.
- Lastly, we use the printf() function to directly print the random number, as seen in the output window.
Function In C With Neither Arguments Nor Return Value
Functions in C that take no arguments and return no value are also often referred to as void functions. We use them to perform actions or operations that don't require any input or result to be returned. For example, displaying messages, generating patterns, etc.
Syntax:
void function_name (); // function declaration
function_name (); // function call
void function_name() // function definition
{// statements
}
Let's see an example of a function that prints the welcome message upon calling it, which we define as a void function in C.
Code Example:
Output:
Welcome to Unstop!
Explanation:
In the example above-
- We first define the void function welcomMessage(), which takes an integer pointer as its argument.
- In the main() function, we directly call the welcomeMessage() function without passing any argument.
- The function gets invoked, prints the welcome message, and the control flow returns to the main() function, as seen in the output window.
- Lastly, the program terminates and returns 0.
Types Of Function Calls In C
In C, function calls can be categorized into two main types, i.e., call by value and call by reference.
Function Call-By-Value In C
In call by value, the value of the arguments is passed to the function being called. It means that the function receives a copy of the data (i.e., the formal parameter receives a copy of the actual parameter), and any modifications made to the parameters within the function do not affect the original values outside the function. This method is commonly used for integers, floats, and characters. (delete this portion)
Syntax:
function_name( argument_1, argument_2, … );
Here, the function call passes the value of arguments instead of their address. Given below is an example of the same, where the C function modifies the value of a variable using a void function.
Code Example:
Output:
Original value: 5
After the function call: 5
Explanation:
In the example C code-
- We first define the void function modifyValue(), which takes an integer as an argument.
- It multiplies the given variable by 2 and assigns the value back to the variable itself.
- In the main() function, we first declare a variable num and initialize it with 5.
- We then use the printf() function to display the original value of num before passing it to the function.
- Next, we call modifyValue() function by passing the variable num as an argument.
- The function gets invoked, receives a copy of the num, and stores it in variable x.
- It then modifies the value of x, and the flow of control returns back to the main() function.
- Lastly, we use the printf() function to print the modified value of num, as seen in the output window.
Here, the modifyValue() receives a copy of the num variable. Thus, the value of num remains unaffected outside the main function.
Function Call-By-Reference In C
The method of calling a function in C reference revolves around passing the memory address (reference) of arguments (i.e., the actual parameters and the formal parameters refer to the same memory location) to the function. This empowers the function to modify the original data directly. This approach is harnessed using pointers in C and is particularly useful for altering the original data or working with arrays and complex data structures.
Syntax:
function_name( data_type *argument );
Here,
- The function_name refers to the name of the function.
- The *argument refers to a pointer to the argument whose type is given by data type.
The function call is made by passing the address of arguments instead of their value. Below is an example where we modify the value of a variable using the void function.
Code Example:
Output:
Original value of num = 5
After the function call, value of num = 10
Explanation:
In the code example above-
- We first define the void function modifyValue(), which takes a pointer to an integer as its argument. It multiplies the value of the integer being pointed to by 2 and returns it back to the variable.
- In the main() function, we declare and initialize an integer variable num with the value of 5.
- We then print the original value of num before passing it to the function using the printf() function.
- Then, we call the modifyValue() function By passing the address of the num variable as an argument.
- The function gets invoked, receives the address of the num, and stores it in the pointer variable x.
- It modifies the value of the original variable num in the main() function by dereferencing the pointer with *x, and the flow of control returns back to the main() function.
- Lastly, we use the printf() function to print the modified value of num after the function call, as seen in the output window.
Here, the modifyValue() function receives the address of the num variable and stores it in the integer pointer x. Thus, any modifications done to the value at that address affect the value original variable in the main() function.
The Main() Function In C Programming
This function is the driver of the execution of C programs. It serves as the entry point for program execution. When running a C program, the operating system first looks for the main() function and executes the code within. Being the driver function, it is mandatory to include the main() function in the program.
Syntax:
#include<stdio.h>
int main() { // Parameters are optional
// Your code goes here
return 0;
}
Here,
- We define the main function as the starting point of the program with int main().
- The function returns an integer, typically 0, to indicate successful execution.
- Within the curly braces {}, we have the body of the main function, where executable statements are enclosed.
The Anatomy Of The main() Function
The structure of the main() function follows a specific format.
- int: The main() function returns an integer value. By convention, a return value of 0 indicates successful execution, while non-zero values often signify errors or exceptional conditions.
- main(): This is the function's name, which distinguishes it as the entry point of the program.
- { }: The curly braces enclose the body of the main() function, where your code resides.
- return 0;: At the end of the function, a return statement signifies the end of program execution and communicates a status code (0 in this case) to the operating system.
Role Of The main() Function In C
As mentioned, the main() function is an integral part of any C program. Listed below are some prominent reasons for using the main() function in C:
- Entry Point: When we execute a C program, the operating system locates and invokes the main() function, marking the start of program execution.
- Control Flow: The main() function controls the sequence in which other functions, expressions, and statements within the program are executed.
- User Interaction: It provides a platform for user interaction through input and output operations using functions like printf() and scanf().
- Function Calls: The main() function calls other functions as needed to perform specific tasks, making it a hub for function coordination.
- Program termination: The main() function plays a role in program termination. When the main() function completes execution or reaches the return statement, the program terminates. The value returned from main() can be used to indicate the program's exit status to the operating system.
Command Line Arguments In main() Function In C
While the typical main() function doesn't take arguments, it can handle command-line arguments. These are values passed to the program when it's run from the command line. It can optionally take two arguments, i.e., int argc (argument count) and char *argv[ ] (argument vector). These arguments are used to handle command-line inputs and configurations. Also, argv[0] stores the program name.
Below is an example C program for passing command line arguments to the main() function.
Code Example:
Output:
Number of arguments: 1
Argument 0: /tmp/IvNpn8wnff.o
Explanation:
In the code example, we begin by including the standard input-output library (stdio.h).
- We then define the main() function with two parameters, i.e., int argc and char *argv[]. These parameters facilitate the use of command-line arguments.
- Inside the main(), we use printf() to display the number of command-line arguments passed to the program. The value of argc represents the count of arguments.
- Next, we employ a for loop to iterate over the command-line arguments. The loop runs from i = 0 to i < argc, providing an index for each argument.
- Within the loop, we use a printf() statement to print each argument along with its index. The %d format specifier is used for the index, and %s is used for the argument itself.
- The return 0 statement concludes the main function and signifies successful program execution.
Recursive Functions In C Programming
Recursive functions in C allow functions to call themselves, opening doors to solve a problem in smaller sub-problems. This self-referential property enables a recursive function to break down complex tasks into simpler steps until they can be directly solved.
- Recursive functions provide a more intuitive approach to a problem. Its application in real-world cases includes factorial calculation, binary to decimal conversions, tree traversals, fractals, and mathematical sequences.
- They differ from other functions in terms of code simplicity, time complexity, and errors.
- Recursive functions make code look much simpler, less error-prone, and more understandable, but for complex problems, it might cost a lot of time than a non-recursive implementation.
A recursive function in C comprises two crucial components:
- Base Case: This acts as the terminating condition for the recursion. When the base case is reached, the function stops calling itself and begins returning values. It is essential to define the base case to prevent infinite recursion.
- Recursive Case: In this step, the function calls itself with a modified argument, progressively moving towards the base case.
Recursive calls consume memory for each function call. Thus, if not managed carefully, it may lead to a stack overflow. Let's take a look at an example program where we create a recursive function in C to find the factorial of 7.
Code Example:
Output:
Factorial of 7 is 5040
Explanation:
In this example-
- We define the function factorial(), which takes an integer as its argument and returns an integer value.
- In the main() function, we first declare an integer variable num and initialize it with 7.
- We then call the factorial() function by passing the variable num as an argument.
- The function gets invoked and stores the value of num in the variable n.
- The function keeps calling itself, decrementing the value of n by one with each call until its value reaches either 0 or 1 and satisfies the base condition.
- After returning to the parent condition, the function returns the value of the factorial to the main() function.
- We then use the printf() function to print the factorial, as seen in the output window.
Inline Functions In C Programming
In C Programming, when a function call is made, the flow of control jumps from the calling function to the definition of the function being called. This poses an extra effort to the processor, which is why inline functions came into the picture.
- An inline function in C is a special type of function that is expanded by the compiler at the point of function call rather than being executed through the traditional function call mechanism.
- The expansion of inline functions eliminates the overhead due to function calls, which usually leads to performance optimizations.
To make a function inline, we need to use the inline keyword before the function. When a function is marked as inline, the compiler inserts the function's code directly into the calling code, essentially replacing the function call with the function's body. This is generally beneficial for short and frequently-called functions.
Note: The use of inline functions is completely compiler-dependent. Their behavior and effectiveness depend upon the compiler optimization settings and support for the inline keyword.
Syntax:
inline return_type function_name ( parameters){
// function definition
}
Here,
- The inline keyword marks the function with the name function_name as an inline function.
- The curly braces contain the code statement, and the parameters refer to the variables to be passed as arguments.
Let's take a look at an example of an inline function in C with a program to find the square of 7, given below.
Code Example:
Output:
Square of 7 is 49
Explanation:
In the example-
- We first define the function square(), which takes an integer as its argument and returns an integer value.
- In the main() function, we declare a variable num and initialize it with value 7.
- We then call the square() function by passing num as an argument.
- The function being inline in nature, the compiler puts the body of the function in place of the function call, evaluates it, and returns the value.
- We then use the print() function to print the square, as seen in the output window.
Important Points About Functions In C Programming
Here are a few important points to keep in mind when working with function in a C program:
- If a function is called before its declaration, then the compiler implicitly declares the function with the return type as int and throws an error if the data type of return value is different from int.
- Each C program contains at least the main() function, which is the program's entry point from where the compiler starts executing the program.
- In a C function, if the return type is not provided, then the compiler, by default, treats it as a void return type.
- In C programming, the return type of a function cannot be an array or function type. Thus, we use pointers and function pointers to overcome this.
- In C programming, a function declared with an empty parameter list () can be called with any number of parameters. But, when declared with the void keyword in the parameter list, it can be called without any parameters.
- A function in C can be declared and defined simultaneously togetherly instead of explicitly declaring and defining.
Advantages & Disadvantages Of Functions In C
Functions in C provide a structured and modular approach to programming, and they come with both advantages and disadvantages.
Advantages Of Functions In C
- Modularity: Functions promote modularity by breaking down a program into smaller, manageable units. Each function can focus on a specific task, making the code more organized and easier to understand.
- Reusability: A function in C can be reused in different parts of a program or even in different programs, reducing the need to duplicate code. This enhances maintainability and saves development time.
- Readability: Well-designed functions make code more readable. Instead of dealing with a large and complex code block, developers can understand the program's logic by reading and comprehending smaller, self-contained functions in C programs.
- Easier Debugging: Functions facilitate easier debugging. When an issue arises, focusing on a specific function in C helps isolate the problem, making it easier to identify and fix errors.
- Scoping: Functions provide local scope for variables. This helps prevent naming conflicts between variables in different functions and enhances code reliability.
- Abstraction: Functions allow the abstraction of complex operations. The internal details of a function in C can be hidden, providing a high-level view of the operation to the rest of the program.
Disadvantages Of Functions In C
- Overhead: Function calls introduce overhead in terms of execution time and memory usage. While the impact is generally minimal, it can become a concern in performance-critical applications.
- Complexity: Excessive use of functions in C can lead to a large number of small functions, potentially making the code more challenging to follow. Striking a balance between modularity and simplicity is crucial.
- Global Variables: A function in C can access global variables, and excessive reliance on global variables can lead to code that is harder to understand and maintain. Proper scoping and minimizing the use of globals are essential.
- Parameter Passing Overhead: Passing parameters to functions involves copying data, which can be inefficient for large data structures. This overhead may impact performance, especially in resource-constrained environments.
- Abstraction Overhead: Overly abstracted functions in C may hide important details, making it difficult for developers to understand how certain operations are implemented. Finding the right level of abstraction is crucial.
- Function Proliferation: An excessive number of small functions can lead to a proliferation of function names, making it challenging to choose meaningful and distinct names for each function.
Also read- 100+ Top C Interview Questions With Answers (2023)
Conclusion
Functions in C are an integral part of C programming. They allow us to break bigger problems into smaller parts, with each function handling a specific task or issue. We can either declare a function in C along with its definition or do this separately. The most common types of functions are library functions and user-defined functions. Additionally, functions in C can also be categorized based on the condition or arguments and return values, as well as the way we call them.
The primary advantages of a function in C include modularity, reusability, and abstraction outweigh the disadvantages of overhead and potential complexity. With clear examples and explanations, you are competent enough to try and solve complex and pragmatic problems based on functions in C. Wise use of functions can lead to the development of efficient and elegant code structures.
For more such informative and high-quality content along with new opportunities every day, stay connected with Unstop.
Frequently Asked Questions
Q. What happens if you call a function before its declaration in C?
Suppose a function is called before its declaration. In that case, the compiler implicitly declares the function with the return type as int. If the data type of the value returned from the function is different from int, it will throw an error.
Code Example:
Output:
error
Explanation:
Here, the code will throw an error, as the compiler implicitly declares the function grade() with the return type as int.
- The function definition has the return type of char.
- This conflicts with the int return type from the implicit declaration, and the program throws an error, as seen in the output window.
Q. Where should a function be defined in a C program?
In C programming, a function can be defined in one of three places, i.e., before the point of the function call, after the point of the function call, or in a separate source file. However, the most common and recommended practice is to define the function before the line where it's called. This ensures that the compiler has knowledge about the function’s definition before it’s used.
- Defining before use: This ensures that the function’s definition comes before any point where the function is used in the code so that the compiler becomes aware of the function prototype. It is considered as best practice for function definition.
- Defining after use: In this case, we define the function after the point where it is called in the program. However, we need to declare the function before the calling point to avoid any errors. This method is generally tedious and difficult to understand in some cases.
- In a separate source file: For working on larger projects, it is best to define the functions in a separate source file and include the corresponding header file in the program to use the defined functions.
Q. What are void functions in C?
Functions that do not return any value are commonly known as void functions. These functions are used for performing actions or operations that do not require a result to be returned to the calling function. This case also arises when the return type of a function is not defined; then, the compiler treats it as a void function. These functions are commonly used for printing information, input validation, file operations, and updating variables.
Q. What is a function pointer in C?
A function pointer in C is a variable that stores the memory address of a function. This enables the indirect calling of a function through pointers. Function pointers enable dynamic behavior, such as selecting and invoking functions at runtime based on certain conditions or requirements. In C, functions can be used like any other data type, allowing us to define pointers to functions and invoke them indirectly.
Let’s understand how a function pointer is declared and used with the example shown below.
Code Example:
Output:
Square of 8 is 64
Explanation:
In the code example above,
- We first define the function square(), which takes an integer argument and void return type.
- In the main() function, we first declare a function pointer funcPtr and assign the address of the square() function address to it.
- We then indirectly call the square() function, passing 8 to the funcPtr, equivalent to calling square(8).
- The function gets invoked and prints the square of 8 using the printf() function.
- Lastly, the flow of control returns back to the main() function, and the code terminates.
Q. How to declare a function in C?
Declaring a function’s signature before its implementation informs the compiler about its existence, its name, parameters, and return type, enabling you to call it before defining it.
Syntax:
return_type function_name( parameter list ); // Declaration of function
Parameter names are not crucial while declaring functions. We can declare it only using the variable's data type without the name. Below is an example program consisting of a function to add two integers in C.
Code Example:
#include<stdio.h>
int addition(int a, int b); // Declaration of function
int addition(int, int); // Alternate declaration
Q. What is a forward declaration in C?
In C programming, a forward declaration is a declaration of a function, variable, or type that tells the compiler that the entity exists but doesn't provide its full details. This allows you to use the entity in your code before its actual definition. Forward declarations are particularly useful in scenarios where functions or variables are defined later in the code or in a separate source file.
Here's an example of a forward declaration for a function in C:
Code Example:
In this example, the addNumbers() function is forward-declared at the beginning of the code, so the main function can use it even though its full definition appears later in the code. Forward declarations are essential when functions are defined below their usage or in separate files, ensuring that the compiler knows about them before encountering their implementation.
Q. What is the difference between function arguments and parameters?
Aspect | Parameters | Arguments |
---|---|---|
Definition | Parameters are the variables used in a function declaration or definition. They act as placeholders for the values that will be passed to the function. | Arguments are the actual values or expressions passed to a function when it is called. They correspond to the parameters defined in the function. |
Location in Code | Parameters are part of the function signature and are declared in the function header. | Arguments are supplied during the function call and appear in the parentheses following the function name. |
Example | c void exampleFunction(int x, float y) { /* function body */ } | c int result = calculateSum(3, 5); |
Role | Parameters are used within the function to process the values passed to it. | Arguments provide the actual data that the function will work with. |
Modifiability | Parameters are local variables of the function, and modifications to them do not affect the values outside the function. | Arguments are values passed to the function, and any changes made to them within the function do not affect the original values outside the function. |
Number | The number of parameters is determined by the function declaration or definition. | The number of argument lists must match the number of parameters declared for the function. |
Type | Parameters have types (e.g., int, float) that specify the kind of data they can accept. | Arguments must match the types of the corresponding parameters in the declaration of function in C. |
Purpose | Parameters define the input that a function expects. | Arguments provide the actual data that fulfills the function parameter requirements during the call to the function in C programs. |
You might also be interested in reading the following:
- Ternary (Conditional) Operator In C Explained With Code Examples
- Control Statements In C | The Beginner's Guide (With Examples)
- Bitwise Operators In C Programming Explained With Code Examples
- Do-While Loop In C Explained With Detailed Code Examples
- 5 Types Of Literals In C & More Explained With Examples!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment