While Loop In C, Infinite, Nested And More! (+Code Examples)
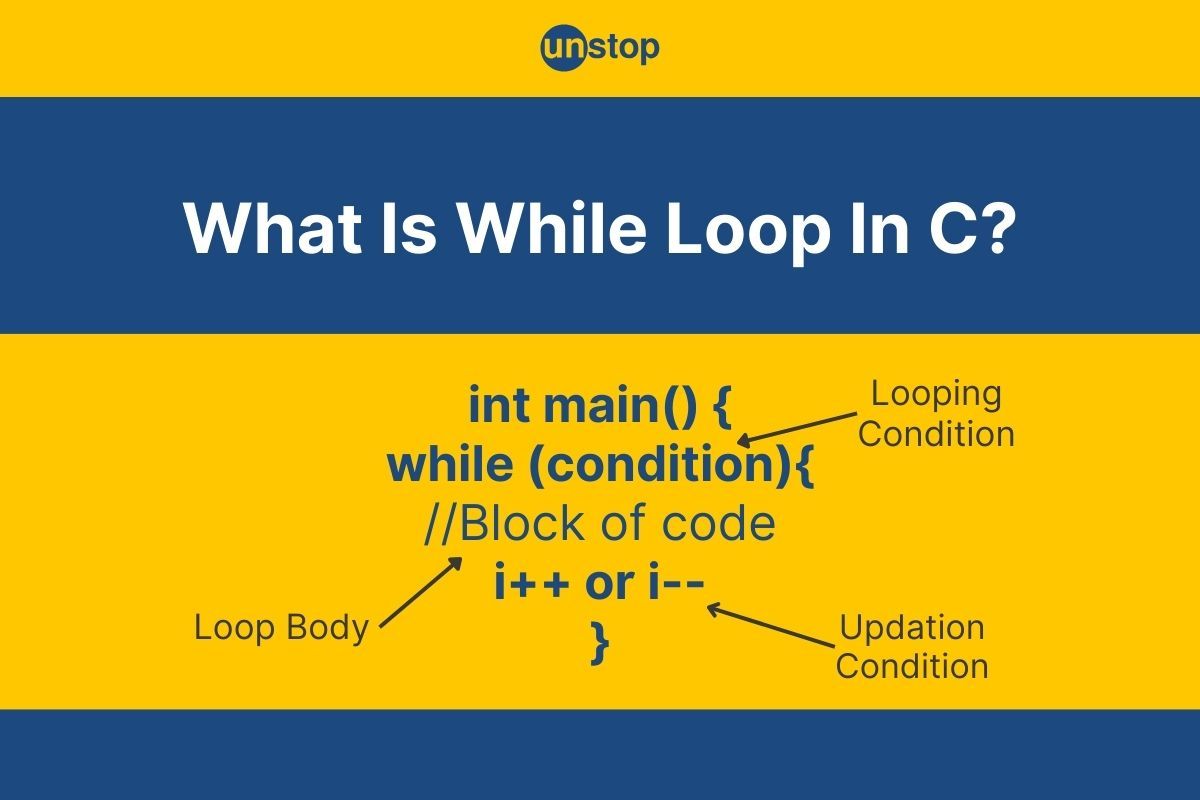
Looping statements, loop control statements, or iteration statements are all used to refer to loops in programming. These contracts play a vital role in logical programming by allowing users to run a segment of code iteratively under specific conditions. In this article, we will focus our discussion on the while loop in C programming and help you understand how to use it in your own codes.
We will provide comprehensive definitions, syntax explanations, flow charts, and practical examples. To effectively grasp the application of the while loop in C, it is essential to first comprehend the concept of loops, their significance, and the diverse types of loops available for use in C programs. So let's get started!
What Is Looping In C?
Looping is the act of executing a statement or series of statements repeatedly until a specific condition has been fulfilled or met. In other words, the loop continues to run until the condition evaluates to false. Iteration statements, commonly known as loops, are named as such because they iterate or cycle through the execution of the statement, creating a circular pattern.
The C language offers three main types of loops
- The for loop
- while loop
- do...while loop
The Need For Loops In C Programming
Looping is a fundamental concept in programming that allows a set of instructions to be repeated multiple times. There are several reasons why looping statements are essential in programming, such as:
- Repetition: They allow the execution of a set of instructions multiple times without having to write the code again and again.
- Efficiency: The loop constructs reduce redundancy, making code more concise and maintainable.
- Iterating Collections: Facilitates the traversal of arrays, lists, or sets.
- Automation: Essential for automating repetitive tasks or operations.
- User Interaction: These constructs help us handle repeated user input or display menus until a condition is met.
- Algorithm Implementation: They are integral for the efficient implementation of algorithms.
- Dynamic Program Flow: Enables programs to adapt to different scenarios based on runtime conditions.
- Control Flow: Works in conjunction with decision-making structures to control program flow.
- Encapsulation: Encapsulates repetitive logic, enhancing code modularity and organization.
What Is While Loop In C Language?
In most programming languages, the while loop is a control flow statement that enables the repeated execution of code based on a specified boolean expression. The boolean condition can be either true or false. The while loop is also referred to as a pre-tested or entry-controlled loop due to its nature.
While Loop Syntax In C Language
while (test expression){
// loop body or block of statements
}
Here,
- The while keyword marks the beginning of the pre-tested loop/ while loop.
- The test expression refers to the looping condition, which is checked before the loop body is executed.
- The curly brackets {} contain the piece of code that will be executed till the test expression remains true.
Note that the loop's body can have a single statement or a group of statements. Once the test condition becomes false, the loop will end, and the program control moves to the line immediately after the loop.
The Structure Of The While Loop In C
As is evident from the while loop syntax, it follows a structured top-down approach. Its structure can be divided into the following sections-
- Initialization: In this phase, the loop variable is assigned an initial value. While not mandatory in the while loop in C syntax, initialization becomes crucial when using a variable in the test condition.
- Conditional Expression/ Test Condition: This step plays a crucial role in determining whether the code block within the while loop will be executed. Because the loop body will be executed only if the test condition specified in the conditional expression evaluates to true.
- Body: The body comprises the actual set of statements that are executed until the desired condition is met. Typically enclosed within curly braces, this section carries out the necessary tasks within the loop.
- Updation: The updation step involves modifying the loop variable's value after each iteration. Although not explicitly specified in the syntax, the updation expression is typically placed within the loop's body to ensure the variable is appropriately updated.
By following this systematic approach, the while loop in C provides an effective mechanism for the repetitive execution of code while fulfilling the specified condition.
How Does While Loop In C Work?
The working mechanism of a while loop is similar to that of a for loop or many other control statements, for that matter. The loop flowchart given below gives a visual impression of how the flow of control jumps/ moves as we move along in a while loop. Take a look-
The execution/ working of the while loop, as also evident in the flow diagram above, is as follows:
- When the program initially enters the loop, it evaluates the test condition, which presents two possibilities, i.e., true or false.
- If the test condition is false, the program bypasses the loop body and proceeds to the next step or line of the program. That is, the flow of programs exits the loop.
- If the test condition evaluates to true, the program executes the block of statements within the loop body.
- Upon completing the execution of the loop body, program control returns to step 1. That is, execution does not stop after the statements are completed; instead, the condition is checked again.
- This process continues as long as the test expression remains true, ensuring repeated execution of the loop body.
- Once the condition evaluates to false, the loop terminates.
Example Of While Loop In C
Now that we know what a while loop is, its structure, and its working mechanism, let's take a look at a while loop example. Here, the C program takes user input and prints the Fibonacci series up to that number using a while loop in C.
Code Example:
Output:
Enter a Number: 5
0 1 1 2 3 5
Explanation:
In this basic C program, we begin by including the standard input-output library (<stdio.h>) and then create the main() function, which is the entry point of the program.
- Inside main(), we declare three variables, i, n, j, and k, of integer data type.
- Then, as mentioned in the code comments, we use the printf() function to prompt the user to enter a number.
- The value is stored in the variable n using the scanf() function. Here, we use the reference operator (&) to indicate the address of variable n.
- Next, we initialize variables i, j, and k with the values of 0, 1, and i+j (sum of i and j using addition arithmetic operator), respectively.
- We then use the printf() statement with a formatted string and %d format specifier to display the initial values of i and j.
- Then, we create a while loop that continues as long as k is less than or equal to n. Inside the loop:
- At every iteration, the loop prints the current value of k using a printf() statement.
- After every iteration, the values of variables are updated for the next iteration. Here, the value of i is updated to j, the value of j is updated to k, and the value of k is updated to the sum of i and j.
- This continues until the value of k reaches 5, at which point the loop terminates.
- After the loop, the program returns 0 using return 0 to indicate an error-free execution to the operating system.
Time Complexity: O(F(n)) - Fibonacci sequence growth
Space Complexity: O(1) - Constant space
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Properties Of The While Loop In C
The while loop possesses several important characteristics that you must know when working with these constructs. The properties are as follows:
- The condition is evaluated using a conditional expression. The while loop's designated statements continue to execute as long as the specified condition remains true.
- A condition is considered true if the result of its evaluation is 0. Conversely, any non-zero value results in the condition being considered false.
- The condition expression is a mandatory component of the while loop structure.
- It is possible to have a while loop without a body, meaning there may not be any statements within the loop's block.
- The while loop allows for the inclusion of multiple conditional/ test expressions to evaluate different conditions.
- The use of brackets is optional when the loop body consists of only one statement.
- The execution of a break, goto, or return statement within the loop's body can lead to the termination of the while loop. On the other hand, if you wish to end a specific iteration while keeping the while loop active, the continue statement can be used. This allows control to be handed back to the while statement for the subsequent repetition.
These properties and behaviors of the while loop will enable you to create flexible and controlled iterative processes within their programs.
When To Use While Loop In C?
The while loop in C is used when you want to repeatedly execute a block of code as long as a certain condition is true. Listed below are some common situations where you might choose to use a while loop.
Indefinite Iteration: Use a while loop when the number of iterations is not known in advance, and the loop should continue until a specific condition is met.
while (condition) {
// Code to be executed while the condition is true
}
Input Validation: When you need to repeatedly prompt the user for input until valid data is entered.
while (invalidInput) {
// Prompt user for input
// Check if input is valid, update invalidInput
}
Processing Collections: Iterating over elements in an array, list, or other data structures when the size of the collection is not fixed.
int i = 0;
while (i < arraySize) {
// Process array elements
i++;
}
Dynamic Memory Allocation: When working with dynamically allocated memory, and you want to perform an operation until a specific condition is satisfied.
while (ptr != NULL) {
// Perform operations on dynamically allocated memory
// Update ptr as needed
}
File Processing: Reading lines from a file until the end of the file is reached.
while (feof(file) == 0) {
// Read lines from the file
}
Event Handling: In event-driven programming, you might use a while loop to continuously handle events until a termination condition is met.
while (!exitRequested) {
// Handle events
}
Infinite While Loop In C
An infinite while loop is created when the given condition is always true, leading to continuous execution of the loop. Programmers may encounter this situation due to the following reasons:
- Incorrect test condition: If the condition specified in the while loop is incorrect or never evaluates to false, the loop becomes infinite.
- Missing updation statement: If the updation statement is absent within the loop body, the condition remains constant, resulting in an infinite loop.
- Non-zero return value: If the expression within the while loop returns a value other than zero, the loop will continue to execute indefinitely.
Let's take a look at an example of an infinite while loop in C to gain a better understanding of this concept.
Code Example:
Output:
1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 ...... (infinitely)
Explanation:
We begin the d by including the essential header file for input/ output operations.
- Inside the main() function, we declare and initialize an integer variable var with the value of 1.
- We then create a while loop, where the initial test condition var <= 2.
- If the condition returns true, then the printf() statement inside the loop will display the value of var to the output console. If the condition is false, the loop will terminate.
- But since here the condition is always true, the loop body repeatedly prints the value of the var variable.
- The loop will run indefinitely, creating an endless loop because the value of var is constant (1), and there are no statements inside the loop that change the value of var.
- So, in this instance, the program will repeatedly print the number 1.
Note: The code demonstrates an infinite while loop, which will never end since the value of the variable var is constant (there is no increment/ decrement operator to update the value of this variable). An infinite loop continues executing until the program is terminated externally (e.g., manually stopping the program execution).
Time Complexity: Because the loop is infinite, it is impossible to calculate the time complexity. The program won't stop running unless it is manually stopped.
Space Complexity: Because the code only utilizes a fixed amount of memory to keep the var variable, its space complexity is constant (O(1)).
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Which Will Be Faster- While(1) Or While(2)?
An infinite loop refers to a loop that continues endlessly until a break statement is encountered. This can be achieved by using constructs such as while(1), while(2), or any non-zero integer as the loop condition. Here, the condition for the while loop is the constant number 1, 2, or any non-zero number. In the absence of a break statement, these loops will continue to execute indefinitely.
However, the use of while(1) or while(any non-zero integer) in practical programming scenarios is generally discouraged. This is because such constructs can lead to increased CPU consumption and code blocking, meaning the loop cannot be exited until the program is manually terminated. While(1) is typically employed when a condition needs to remain perpetually true. It is important to exercise caution when utilizing infinite loops and ensure proper control mechanisms, such as break statements, are employed to prevent unintended consequences.
Code Example:
Output:
1 2 3 4 5
Explanation:
- In the sample C code above, we initialize an integer variable i to the value 0 inside the main() function.
- We then create an infinite while loop using while(1), which will continue to execute indefinitely unless explicitly terminated. Inside the loop-
- We have a printf() statement that displays the current value of i, but the prefix increment operator (++i) increments the value of i before printing it.
- Then, there is an if-statement that checks if the value of i is equal to 5, using the equality relational operator.
- If the condition is true so, the code block containing the break statement comes into execution which causes the program to exit the loop and move on to the next part of the program.
- After the loop, the program returns 0 using return 0 to indicate successful execution to the operating system.
Time Complexity: This code has an O(n) time complexity, where n is the number at which the loop breaks (in this example, 5). The number of iterations is precisely proportional to the value of n because the loop iterates until the break condition is satisfied.
Space Complexity: Because the code only utilizes a fixed amount of memory to store i variable, its space complexity is constant (O(1)).
Nested While Loop In C
Nested while loops are formed by placing one while loop inside another, creating a hierarchical structure where one loop resides within the scope of another loop. This nesting of loops is commonly known as loops inside the loop.
- Depending on the complexity of the problem at hand, any number of loops can be nested within one another, with various combinations of while loops.
- Nested loops are employed when there is a requirement to repeat the loop body a specific number of times based on the problem's intricacy.
- By utilizing nested loops, multiple levels of iteration can be achieved, allowing for more precise control over the repetition process.
It is worth noting that nested loops can be implemented with up to 255 blocks, providing ample flexibility for solving complex problems that necessitate intricate loop structures.
Syntax:
While (outer condition) {
// outer while code statements
While (inner condition) {
// inner while code statements
}
Outer while code-block;}
Here,
- The while keywords indicate the beginning of the respective while loop.
- Conditions refer to the test expression to be checked for the respective loop, i.e., inner or outer.
- The code statements inside curly brackets refer to the code/ loop body, which will be executed if the condition is met.
Important Note:
The number of iterations in a nested while loop is determined by multiplying the number of iterations in the outer loop by the number of iterations in the inner loop, just like in a nested for loop. Nested while loops are commonly utilized in pattern-based C programs, particularly those involving number patterns or shape formations. They provide an effective approach for achieving intricate patterns and complex repetitions within the program's execution flow.
Execution Flow Of Nested While Loop In C Language
The nested while loop consists of an inner while loop and an outer while loop, each operating based on their respective conditions. Let's delve into how the nested while loop functions.
- The program begins by evaluating the test condition for the outer while loop.
- If the outer loop condition is not satisfied (i.e., False), the loop terminates, and the flow of the program moves to code outside of the nested loop.
- If the outer loop condition is met (i.e., True), then any code statements inside the outer loop preceding the inner loop are executed.
- After that, the flow of execution moves to the inner while loop, and its test condition is evaluated.
- If the inner loop condition holds true, the control enters the inner loop, and the associated statements are executed.
- After executing the inner loop statements, the inner loop condition is re-evaluated. This continues till the inner condition remains true.
- Once the inner loop condition becomes false, the control exits the inner loop and moves back to the outer loop.
- If the outer loop condition remains true, the entire procedure repeats from step 3.
- This cycle continues until either the outer loop condition becomes false or a termination condition is encountered within the loops.
Now, let's take a look at an example to see the implementation of nested while loop in C.
Code Example:
Output:
ENTER A NUMBER: 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
Explanation:
- We begin the example by including the input-output library and declare three integer variables, i.e., i, n, and in, inside the main() function.
- Next, we use the printf() function to prompt the user to input a value. Then, we store it in the n variable using the scanf() function and a reference operator to indicate its address.
- We then create a nested while loop to print a triangular pattern of numbers. Here-
- The control variable for the outer loop refers to the number of lines in the pattern. The condition checks if the value of i is less than or equal to n.
- As long as this condition remains true, the newline escape sequence inside the printf() statement shifts the cursor to the next line, ensuring that every execution of the inner loop happens in a new line.
- When the outer condition is true, the control moves to the inner loop, where the loop variable controls the numbers printed on each line.
- The inner loop condition first checks if the value of in variable is less than equal to i.
- If this condition is true, the corresponding code block prints the value of in, and then increments both in and i by 1.
- This inner loop continues till in is less than equal to i, after which the control moves back to the outer loop.
- For every time the outer loop condition is true, the inner loop executes till n<=i is true.
- After both the loops are done executing, and both the conditions become false, the control moves out of the nested while loop.
- The program returns 0 to indicate successful execution to the operating system.
Time Complexity: O(n^2) - Quadratic time complexity
Space Complexity: O(1) - Constant space complexity
Do-While Loop In C
The do-while loop statement in the C programming language is utilized to repeatedly execute a block of code until a certain condition is satisfied. It is classified as an exit-controlled or post-tested loop, where the test condition is evaluated after the execution of the loop's main body.
This distinctive feature ensures that the statements within the do-while loop are always executed at least once, regardless of the condition. This is also what primarily differentiates a do-while loop from a while loop in C.
Basic Syntax of Do-While Loop in C
do {
// body of do-while loop
} while (condition);
Example of Do-While Loop In C:
Output:
0
1
2
3
4
Explanation:
- We begin this example C program by declaring an integer variable i and initializing it with the value of 0 inside the main() function.
- Then, we create a do-while loop that executes the statements inside the do block at least once. Inside the loop-
- The value of variable i is displayed using the printf() function. It contains a format specifier for integer value followed by the newline escape sequence, which shifts the cursor to the next line.
- After the iteration, the value of i is incremented by 1, and the condition is checked again.
- If it is true, the loop continues to the next iteration. If the condition is false, the loop terminates.
- The loop continues to execute as long as the specified condition (i < 5) is true.
- After the loop, the program returns 0 using return 0 to indicate successful execution to the operating system.
Conclusion
The while loop in C programming is a versatile and powerful tool for implementing repetitive execution of code. Its concise syntax and flexibility make it a fundamental construct in a programmer's toolkit. it enables efficient handling of various programming tasks by providing a clear and structured way to repeat a block of code based on a specified condition.
However, it's essential to use while loops judiciously to avoid potential pitfalls, such as infinite loops. Careful consideration of the loop's condition and appropriate updates within the loop body is crucial for ensuring correct program behavior.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. Is it possible to combine a for loop and a while loop? If so, how?
Yes, it's entirely possible to combine a for loop and a while loop in a C program. The key to combining them effectively lies in understanding that they are both constructs for controlling the flow of execution, and you can use them in tandem to achieve specific programming goals.
Below is an example that demonstrates combining a for loop and a while loop in C.
Code Example:
Output:
Iteration 1 (using for loop)
Extra iteration 1 (using while loop)
Extra iteration 2 (using while loop)
Iteration 2 (using for loop)
Extra iteration 1 (using while loop)
Extra iteration 2 (using while loop)
Iteration 3 (using for loop)
Extra iteration 1 (using while loop)
Extra iteration 2 (using while loop)
Explanation:
In this example, the for loop is responsible for iterating three times. Within each iteration, a while loop is used to perform additional iterations. This combination allows for a more intricate control flow, especially when you need different types of iterations or conditions within each iteration of the outer loop.
Q. How does a while loop differ from a do-while loop in C?
The main difference between the while loop and the do-while loop lies in the order of condition checking and statement execution. In a while loop, the condition is evaluated first before executing any statements, determining whether the loop body should be executed at all. On the other hand, a do-while loop executes the statements at least once before checking the condition. This guarantees that the loop body will be executed before condition evaluation.
While loops are considered entry-controlled loops since the condition is checked at the beginning, while do-while loops are classified as exit-controlled loops due to the condition being verified at the end.
Feature | While Loop | Do-While Loop |
---|---|---|
Initialization | Requires initialization of loop control variable before the loop. | Initialization is not required before entering the loop. |
Condition Checking | Condition is checked before the loop body is executed. | Condition is checked after the loop body is executed. |
First Execution | May not execute at all if the initial condition is false. | Guaranteed to execute at least once, regardless of the initial condition. |
Syntax | c while (condition) { // code } | c do { // code } while (condition); |
Q. What are entry and exit control loops? Give their examples.
To begin with, loops are constructs that help control the flow of a program's execution. They are primarily divided into two broad categories, i.e., entry-controlled and exit-controlled.
Entry-Controlled Loop: An entry-control loop is a loop where the condition is checked before the execution of the loop body. The loop body is executed only if the condition is initially true. The while loop is a classic example of an entry-controlled loop in C. For instance, consider a while loop that prints numbers from 1 to 5:
int i = 1;
while (i <= 5) {
printf("%d\n", i);
i++;
}
In this example, the condition i <= 5 is checked before entering the loop body, and the loop continues executing as long as the condition remains true.
Exit-Controlled Loop: An exit-controlled loop is a loop where the condition is checked after the execution of the loop body. The loop body is executed at least once, and the condition is evaluated afterward to determine whether the loop should continue. The do-while loop is an example of an exit-controlled loop. For example, consider a do-while loop that prints numbers from 1 to 5:
int i = 1;
do {
printf("%d\n", i);
i++;
} while (i <= 5);
In this case, the loop body is executed at least once because the condition is checked after the initial execution. The loop continues as long as the condition i <= 5 is true. Exit-controlled loops are useful when you want to ensure that the loop body runs at least once, regardless of the initial condition.
Q. What are loop control statements?
Loop control statements are used to alter the flow of execution within loops. They provide programmers with the ability to control the iteration process, exit loops prematurely, or skip certain iterations based on specific conditions. In C programming, there are three primary loop control statements: break, continue, and goto. Each of these statements serves a distinct purpose in controlling the behavior of loops.
Break Statement: Terminates the loop prematurely. Used to exit a loop based on a specific condition. For example
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break;}}
Continue Statement: Skips the rest of the loop body for the current iteration and moves to the next iteration. For example:
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue;}}
The Goto Statement: Allows an unconditional jump to a labeled statement within the same function. Generally less preferred due to potential code complexity. For example:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (i == 2 && j == 2) {
goto endLoop;}}}
endLoop:
Q. What approaches can we use to calculate the best, worst, and average case complexity of a code?
You can employ many strategies to determine a code's complexity in the best, worst, and average cases.
- Using an analytical method, the complexity of the code is assessed by looking at its organization, loops, conditionals, and recursive calls. The complexity equation is derived through mathematical analysis by using mathematical properties and proofs.
- The asymptotic analysis uses notations like Big O, Omega, and Theta to describe how the algorithm scales with input size.
- Empirical analysis entails running the code with various inputs and calculating the amount of time or resources used during execution. It offers information on the typical case complexity.
- Approximations or upper bounds for sophisticated algorithms may be used in complexity calculations.
The combination of these methods aids in estimating the code's effectiveness and scalability. Importantly, hardware and language optimizations that affect real execution time are not taken into account by complexity analysis, which instead concentrates on algorithmic effectiveness.
This brings us to the end of our discussion on the while loop in C. Here are a few other topics you must read:
- Logical Operators In C Explained (Types With Examples)
- Ternary (Conditional) Operator In C Explained With Code Examples
- Comma Operator In C | Code Examples For Both Separator & Operator
- Identifiers In C | Rules For Definition, Types, And More!
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment