Table of content:
- Why Do We Use Control Statements In C Programs?
- Types Of Control Statements In C
- The If/ Conditional Control Statements In C
- The Switch Case Control Statement In C
- The Conditional Operator Control Statements In C
- The Goto Control Statement In C
- The Loop Iterative/ Control Statement In C
- The Jump Control Statements In C
- Conclusion
- Frequently Asked Questions
Control Statements In C | The Beginner's Guide (With Examples)
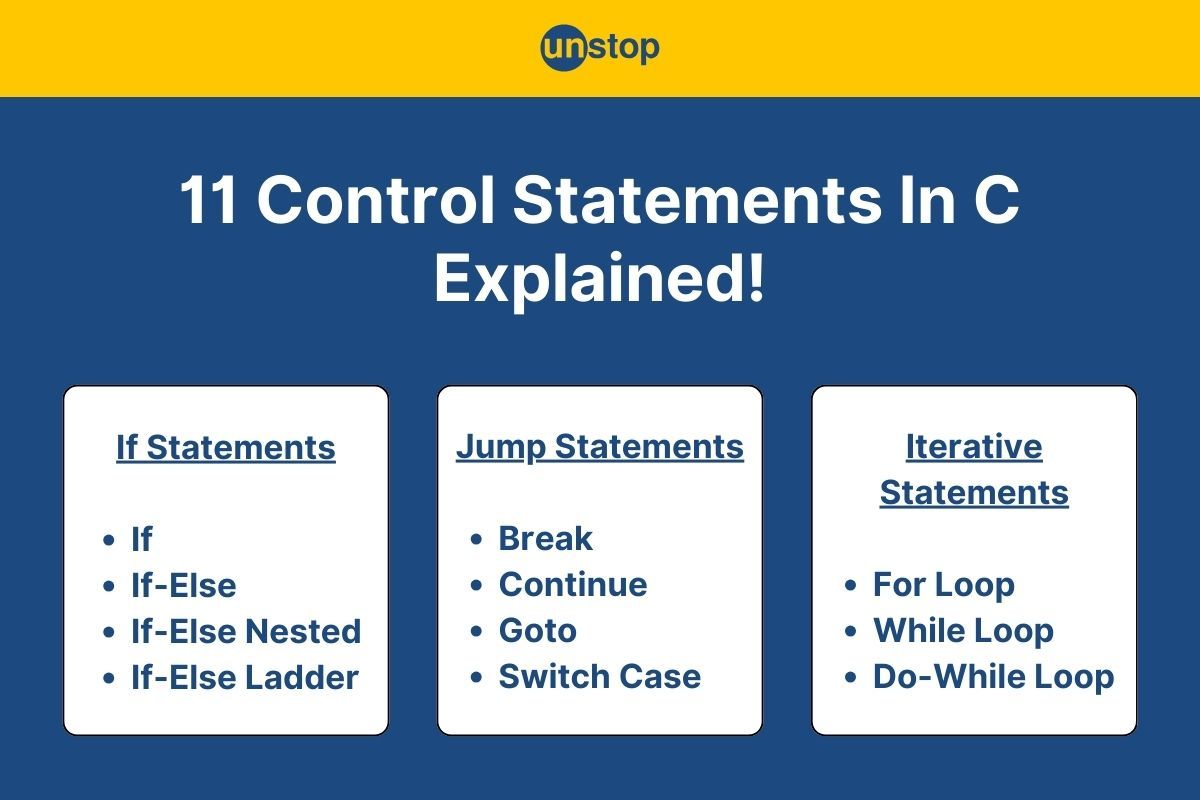
Control statements in C language are essential constructs that enable programmers to control the flow of execution within a program. They dictate which sections of code should be executed based on certain conditions. In other words, the control statements help in making decisions, repeating tasks, and creating logical structures within programs. They provide flexibility and control over the execution of a C program.
By utilizing them effectively, programmers can create efficient and well-structured code, resulting in optimized software performance. This article will provide a comprehensive overview of the various control statements in C, their syntax, use cases, best practices, and more.
Why Do We Use Control Statements In C Programs?
Control statements play a crucial role in C programming, enabling programmers to direct the flow of execution within a program as per their specific requirements. They provide the means to make decisions, iterate over code blocks, and control the program's behavior based on certain predefined conditions. Some of the ways in which they help in writing C programs or are significant are as follows:
- Making Decisions with Conditional Control Statements: Conditional control statements, such as if and switch, empower programmers to make decisions based on certain conditions. These statements allow for branching, where different paths of code execution can be chosen depending on the outcome of the condition. By employing conditional control statements, programmers can introduce logic and create dynamic program behavior.
- Iterating with Loop Control Statements: Loop control statements, including for, while, and do-while, enable the repetition of a block of code until a specified condition is met. They facilitate the implementation of repetitive tasks, such as processing arrays, reading data from files, or performing calculations. Loop control statements provide efficiency and flexibility in handling repetitive operations, reducing code duplication and enhancing productivity.
- Multi-Way Branching: The switch statement allows for multi-way branching based on the value of an expression. This is particularly useful when there are multiple cases to consider, and different code needs to be executed for each case.
- Enhancing Code Readability and Maintainability: Control statements greatly contribute to code readability and maintainability. By utilizing appropriate control statements, programmers can express their intentions clearly, making the code more understandable for themselves and other developers. Well-structured control statements make it easier to trace the program's flow and identify potential bugs or logic errors.
- Handling Exceptional Cases with Control Statements: Control statements in C, such as break and continue, offer mechanisms to handle exceptional cases and alter the normal flow of execution. These statements allow programmers to exit a loop prematurely, skip iterations, or jump to specific code sections. By leveraging these control statements, programmers can manage exceptional scenarios, validate input, or implement error-handling routines effectively.
Types Of Control Statements In C
There are multiple types of control statements in C, including the if-statements and switch statements. These are also known as conditional statements. Other statements are loops (i.e., iterative control statements), break, continue, and goto. The last three are also known as jump control statements. We will explore each of these in the sections ahead.
Control Statement |
Type |
Example |
If Statement |
Selection |
if (condition) { // code block } |
Else Statement |
Selection |
if (condition) { // code block } else { // code block } |
If-Else-If Statement |
Selection |
if (condition) { // code block } else if (condition) { // code block } |
Switch Statement |
Selection |
switch (expression) { case value: // code block break; default: // code block } |
While Loop |
Iterative |
while (condition) { // code block } |
Do-While Loop |
Iterative |
do { // code block } while (condition); |
For Loop |
Iterative |
for (initialization; condition; increment) { // code block } |
Break Statement |
Jump |
break; |
Continue Statement |
Jump |
continue; |
Goto Statement |
Jump |
goto label; |
The If/ Conditional Control Statements In C
The if control statements in the C (also referred to as conditional statements) allow programmers to choose different instruction sets based on specific conditions. These statements enable the execution of specific code blocks only when the condition evaluates to true. If the condition is false, an alternative set of instructions is executed. These statements are also commonly referred to as decision control statements.
Using decision control statements, programmers can create dynamic and adaptive programs that respond to different conditions or inputs. This flexibility allows for the selective execution of code, enhancing the program's efficiency and functionality.
How Do The If Conditional Statements In C Work?
The if control statement evaluates a condition, and if the condition is true, the code block associated with it is executed. If the condition is false, then depending upon the kind of statement, either the code block is bypassed, and the program moves on to the next statement following the if block. Or else the next code block in the statement is executed. We will learn about all the if-statements in the section ahead.
These are four primary types of If control statements in C that we use extensively in programming. They are:
- If
- If-else
- Else if ladder
- Nested if
Let's take a look at what each of these if-statements entails, along with some code examples.
The If Control Statement In C
The if control statement evaluates a condition, and if it is true, the code block associated with it is executed. If the condition is false, the code block is skipped, and program execution continues to the next line after the if-statement.
Syntax
if (condition) {
// Code runs if the condition is true
}
Here,
- The if keyword initiates the if-statement and the condition refers to the expression which is evaluated to deem the output True or False.
- The piece of code inside the curly braces, i.e., {// Code runs if the condition is true } refers to the code that is executed if the output is True.
Code Example:
#include <stdio.h>
int main() {
int age;
printf("Please enter your age: ");
scanf("%d", &age);
if (age >= 18) {
printf("You are eligible to vote.\n");}
return 0;
}
Output:
Enter your age: 21
You are eligible to vote.
Code Explanation:
We begin the sample C program above by including the stdio.h, which contains the input/ output operations.
- Then, we initiate the main() function, which serves as the entry point of the program's execution.
- Inside main(), we declare an integer data type variable called age and then prompt the user to enter a number using the printf() function.
- We then use the scanf() function to read this input and store it in the age variable.
- Here, we use the %d format specifier inside a formatted string to let the program know that it has to read an int number.
- And then reference operator (&age) to indicate that the value must be stored in the age variable.
- Next, we initiate an if-statement to determine whether a person is eligible to vote based on their age. Here-
- The if condition checks if the input number is greater than or equal to 18.
- If the result is true, then the if-block of code prints the respective phrase to the console.
- Here, we input the value 21, which is greater than 18, so the printf() statement inside the if-block prints- You are eligible to vote.
- Finally, the program returns 0 to indicate the successful execution of the main function.
The If-Else Control Statement In C
The if-else statement allows the program to execute a specific block of code if a given condition is true. If the condition is false, an alternative block of code specified in the else clause is executed. It is similar to the if-statement, but here, instead of the flow of the program moving out of the if-statement, the flow stays inside the if-else statement. The flow moves out only if either of the two blocks is executed.
Syntax:
if (condition) {
// executed if the condition is true
} else {
// executed if the condition is false
}
Here,
- The if and else keywords mark the initiation of the if-else statement.
- The condition is what is evaluated to get a boolean result (i.e., true or false).
- Code inside the curly braces following the if keyword is what is executed if the output of condition evaluation is True.
- The code inside the curly braces after the else keyword is executed if the output is False.
- Lines starting with // are code comments, providing explanations for human readability.
Code Example:
#include <stdio.h>
int main() {
int number;
printf("Enter a number: ");
scanf("%d", &number);
if (number % 2 == 0) {
printf("The number is even.\n");
} else {
printf("The number is odd.\n");}
return 0;
}
Output:
Enter a number: 7
The number is odd.
Enter a number: 10
The number is even.
Code Explanation:
We begin by including the essential header files and define the main() function.
- We then declare an integer variable, number, and use the printf() function to prompt the user to enter a number.
- The program then uses the scanf() function to read the input from the user and store it in the number variable.
- Next, we employ an if-else statement to check if the input number is divisible by 2 (i.e. if the remainder of the division operation is 0). Inside the statement-
- The initial condition is number % 2 == 0, which checks if the remainder of dividing the number by 2 is equal to 0.
- If the condition is true, then the program executes the code following the if-keyword, and the printf() statement outputs the message- The number is even.
- If the condition is false, then the program executes the code following the else keyword and prints the message- The number is odd.
- For ease of understanding, we have provided outputs for two different numbers. That is, when we enter the number 7, the if-code block is executed. And when we enter the number 10 as input, the else-code block is executed.
- Finally, the program returns 0, indicating successful execution.
This code demonstrates the use of the if-else control statement in C to perform a conditional check and execute different code blocks based on the outcome. It allows the program to determine whether a given number is even or odd and provides the corresponding output.
By utilizing decision control statements like if and if-else, programmers can create C programs that dynamically respond to different conditions, enhancing the functionality and flexibility of the software.
The Nested If-Else Control Statement In C
The nested if-else statement refers to a situation where one if-statement is placed within another if or else block. It allows for multiple conditions to be evaluated in a hierarchical manner.
Syntax:
if (condition1) {
code block 1 (executed if condition1 is true)
if (condition2) {
coe block 2 (both condition1 and condition2 are true)
} else {
code block 3 (executed if condition1 is true but not condition2)}
} else {
code block 4 (executed if condition1 is false)
}
Here,
- Just like in other statements, the if keywords mark the beginning of the statement being used.
- The condition1 and condition2 refer to the outer/ initial condition we check and the inner condition/ nested condition, respectively.
- The blocks of code 1, 2, and 4 refer to the blocks that are implemented if condition 1 is true, both condition 1 and 2 are true, and if condition 1 is false, respectively.
- Code block 3 refers to the code that is executed if condition 1 is true but condition 2 is false.
- The else keywords mark the alternative statements that determine the flow of the program if the base conditions for if-blocks are false.
Code Example:
#include <stdio.h>
int main() {
int age;
printf("Enter your age: ");
scanf("%d", &age);
if (age >= 18) {
printf("You are eligible to vote.\n");
if (age >= 60) {
printf("You are a senior citizen.\n");}
} else {
printf("You are not of legal age.\n");}
return 0;
}
Output:
Enter your age: 25
You are eligible to vote.
Enter your age: 65
You are eligible to vote.
You are a senior citizen.
Code Explanation:
- We begin the example C program above by defining an integer variable age inside the main() function.
- Next, we prompt the user to input a number using the print() function and then use the scanf() function to read this number and store it in the age variable.
- Then, using a nested if-statement, we determine if the age is eligible for voting and or is of senior citizen status. Inside the statement-
- The initial if-condition (condition1) checks if the age entered is greater than or equal to 18. If this condition is false, the execution flow moves to the last else-block, and the message- You are not of legal age, is printed to the console.
- If the condition holds true, then the code block1 containing a print() function outputs the message- You are eligible to vote.
- After that, the flow of the program moves to the inner if condition, which checks whether the input age is greater than or equal to 60.
- If the inner condition returns true, then the message- You are a senior citizen is printed. And if the inner condition is false, then the flow of execution moves out of the if-else statement.
- Inside the statements, we also use the newline escape sequence (\n) to shift the cursor to the next line before printing a new message.
- In this example, we show the output for two different age inputs. First, when the input age is 25, only the message- You are eligible to vote is printed.
- Second, when the input age is 65, both the first message and the other message (i.e., You are a senior citizen) are printed.
- Finally, the program returns 0, indicating successful execution.
This readable code segment showcases the use of nested "if" statements to perform conditional checks and provide different outputs based on the age input. It allows the program to determine if a person is eligible to vote and, if so, whether they qualify as a senior citizen.
The If-Else Ladder Control Statement In C
The if-else ladder statement is a series of if-statement and else-if control statements in C. These statements are used when multiple conditions need to be checked sequentially. It allows for selecting blocks of code based on the first condition that evaluates to true.
- They differ from the nested if-else statement in that the nested statements check for the else statements that are contained inside a main if-block.
- Whereas in a ladder statement, the if-else statements are checked in a sequential manner.
- In addition to this, the nested if-else statement will be skipped if the initial condition is false, but in the case of a ladder statement, all the statements and conditions will be checked.
The example given below will help clarify the difference between the two better. But first, let's take a look at its syntax.
Syntax:
if (condition1) {
Code block 1 (condition1 is true)
} else if (condition2) {
Code block 2 (condition1 is false and condition2 is true)
} else if (condition3) {
Code block 3 (condition1 and condition2 are false and condition 3 is true)
} else {
Code block 4 (if all conditions are false)
}
Here,
- The if and else keywords mark the different code blocks and conditions.
- Condition 1, 2, and 3 refer to the respective if-conditions that are being checked for a boolean result.
- Note that condition 2 will be checked when condition 1 is false, and condition 3 will be checked if both condition 1 and 2 are false.
- The code block 1 refers to the block of code that will be executed if condition 1 returns true.
- Code block 2 is the section that will be executed if condition 2 is true. (Also note that for flow to move to condition 2, condition 1 must be false, and so on.)
- Code block 3 refers to the block of code that will be executed if condition 3 is true (and both conditions 1 and 2 are false).
- The code block 4 represents the lines of code that will be executed if all the conditions are false.
Code Example:
#include <stdio.h>
int main() {
int marks;
printf("Enter your marks: ");
scanf("%d", &marks);
if (marks >= 90) {
printf("Grade A\n");
} else if (marks >= 80) {
printf("Grade B\n");
} else if (marks >= 70) {
printf("Grade C\n");
} else if (marks >= 60) {
printf("Grade D\n");
} else {
printf("Grade F\n");}
return 0;
}
Output:
Enter your marks: 85
Grade B
Enter your marks: 72
Grade C
Code Explanation:
- We begin the example C code above by declaring an integer variable marks in the main() function.
- Next, the program uses the printf() function to prompt the user to input their marks and the scanf() function to read and store the input in the marks variable.
- Then, we define a ladder if-else statement, which consists of a series of if and else-if statements to check the input marks and assign a grade. Inside this-
- The first if-statement checks if marks are greater than or equal to 90. If the condition is true, it prints Grade A.
- If the first condition is false, the program moves on to the next else-if statement to check the marks are greater than or equal to 80. If this condition is true, it prints Grade B.
- This process continues with subsequent else-if statements, each checking a specific range of marks to assign the corresponding grades C, D, or F.
- If none of the conditions in the statements are true, the program executes the code block within the else statement, which prints- Grade F. This covers the case when the entered marks are below 60.
- Finally, the program returns 0, indicating successful execution.
This code demonstrates the use of conditional statements to assign grades based on the marks entered by the user. By evaluating the marks against different conditions, the program determines the appropriate grade and provides the corresponding output. This is just one of the ways to use the control statements in C programs that dynamically respond to varying input and provide tailored feedback or results.
The Switch Case Control Statement In C
The switch control statement is a programming construct used in various programming languages to execute different code blocks based on the value of a variable or an expression. It provides an alternative to using multiple if-else statements when there are multiple possible conditions to be evaluated.
How Does The Switch Control Statement In C Work?
In general, the switch statement evaluates the given expression or variable and compares it against a series of cases. Each case represents a possible value or a range of values that the expression can match. Once a match is found, the corresponding code block associated with that case is executed.
Syntax:
switch (expression) {
case label 1:
code block 1
break;
case label 2:
code block 2
break;
default:
default_code block
break;}
Here:
- The switch (expression) initiates the switch statement, evaluating the specified expression.
- Label 1 and label 2 are the values against which we match the evaluation of the switch expression. The value is checked for a match with label 2 only if it does not match label 1, and so on.
- The code blocks 1 and 2 are what are executed if the value from the expression matches label 1 or 2, respectively.
- The break keywords indicate that the program will exit the switch statement after that code block before it is executed (if it is executed).
- Note that if the code block before a given break keyword is not executed, then the switch statement continues to look for a match case. In other words, the statement comes into effect only when a specific case is satisfied, and there's no need to evaluate the remaining cases.
- The default value is the match to the expression by default if none of the other cases are a match. In such a case, the default_code block is executed.
The switch statement provides a way to select from multiple choices based on the value of a given expression. Each choice is associated with a different code block.
Code Example:
#include <stdio.h>
int main() {
char grade;
printf("Enter your grade: ");
scanf("%c", &grade);
switch (grade) {
case 'A':
printf("Excellent!\n");
break;
case 'B':
printf("Good job!\n");
break;
case 'C':
printf("Keep it up!\n");
break;
default:
printf("Invalid grade.\n");
break;}
return 0;
}
Output:
Enter your grade: B
Good job!
Enter your grade: D
Invalid grade.
Code Explanation:
- Inside the main() function of the C program, we declare a character variable, grade, and use the printf() function to prompt the user to input a value.
- The program then uses the scanf() function to read the input and save it to the grade variable.
- We then initiate a switch statement to evaluate the value of the grade and execute the code block associated with the matching case label. Here-
- The first case label is A, so if the input grade is A, then the code block following this case is executed, and the message- Excellent! is displayed using the printf() function.
- After that, the break statement is utilized to exit the switch statement and prevent further execution of the code block.
- However, if the grade does not match A, then we compare it against case label 2, which is B. If there is a match, the message- Good job! is printed to the console, and the program exits the switch statement given break.
- Similarly, if the grade does not match label 1 or 2, then case label 3, i.e., C, is checked. If a match is found, then the message- Keep it up! is printed, and the statement is exited.
- If none of the case labels match the grade, the program executes the code block associated with the default label. So the message- Invalid grade is printed to the console.
- In this example, we have shown the output for two different grades. For grade B, case label 2 is met, and the respective code block is executed.
- For grade D, the default block is executed since the value does not match any other cases.
- Finally, the program returns 0, indicating successful execution.
The Conditional Operator Control Statements In C
The conditional operator, also known as the ternary operator, is a unique control statement in C that provides a concise way to make decisions based on a condition. It is denoted by the question mark followed by colon (?:) symbols, and it offers a short way of writing conditional control statements.
How does the Conditional Operators Control Statement work?
The ternary operator is a programming construct that allows for concise conditional expressions. That is, it provides a way to make decisions based on a condition and execute different code blocks or return different values depending on the evaluation of that condition.
In general, a conditional operator consists of three parts, i.e., the condition, the expression to be evaluated if the condition is true, and the expression to be evaluated if the condition is false. The operator evaluates the condition and returns the value of the corresponding expression based on the result of the evaluation.
Syntax:
condition? expression 1: expression 2;
Here:
- The condition represents the Boolean expression or a condition that is evaluated.
- Expression 1 refers to the expression that will be executed if the condition evaluation returns true. It is placed after the question mark symbol.
- Expression 2 refers to the expression that will be executed/ evaluated if the condition returns false.
- The colon (:) separates the true and false expressions.
Note: This means that if the condition is true, the entire expression evaluates to expression 1, and if the condition is false, the entire expression evaluates to expression 2.
Code Example:
#include <stdio.h>
int main() {
int number;
printf("Enter a number: ");
scanf("%d", &number);
// Using the Conditional Operator to determine if the number is positive or negative
(number >= 0) ? printf("Positive\n") : printf("Negative\n");
return 0;
}
Output:
Enter a number: 8
Positive
Code Explanation:
- We begin by declaring an integer variable number inside the main() function.
- Then, we use the printf() function to prompt the user to input a value, and the scanf() function read the input and store it in the number variable.
- Next, we use the ternary operator to determine whether the number is positive or negative. Here-
- The initial condition checks if the number is greater than equal to zero.
- If the condition evaluates to true (i.e., the number is greater than or equal to 0), the program executes the respective expression and the message - Positive is printed.
- If the condition evaluates to false (i.e., the number is negative), the message- Negative, is printed to the console.
- In this example, the input value is 8 which is positive.
- Finally, the program returns 0, indicating successful execution.
Note- By using the conditional operator, the program provides a concise way to make a decision based on the condition. It eliminates the need for an if-else statement when only two outcomes are involved.
The Goto Control Statement In C
The Goto control statement in the C programming language is a powerful feature that enables programmers to transfer control directly to a labeled statement within their code. In other words, when a Goto statement is encountered, the program transfers control to the label statement specified. This can be any valid C statement preceded by a label followed by a colon (:). The labeled statement can be located anywhere within the same function or code block.
How Does The Goto Control Statement In C Works?
The goto control statement is a programming construct that allows for the unconditional transfer of control to a labeled statement within the code. However, it is important to note that the use of goto statements is generally discouraged in modern programming practices due to its potential to make code harder to read and maintain.
The basic working principle of the goto statement is as follows:
- A specific location within the code is labeled with a unique identifier or label.
- The goto statement is used to jump directly to the labeled location, bypassing the normal sequential flow of the program.
Syntax :
label_name
if (condition) {
goto label_name;}
Here:
- The label_name represents a label in the code, which is essentially a marker that the goto statement can use to jump to a specific location in the code.
- The if keyword marks the beginning of an if-statement wherein the condition is what we are evaluating.
- The goto label_name transfers control to the labeled location (label_name) in the code if the condition returns true.
Code Example:
#include <stdio.h>
int main() {
int num;
printf("Enter a positive number: ");
scanf("%d", &num);
if (num <= 0) {
goto error;}
printf("Square of the number: %d\n", num * num);
return 0;
error:
printf("Error: Invalid input. Please enter a positive number.\n");
return -1;
}
Output:
Enter a positive number: -5
Error: Invalid input. Please enter a positive number.
Enter a positive number: 8
Square of the number: 64
Code Explanation:
- We first include the essential header file for input/ output operations. Then, inside the main() function, we declare the integer variable num.
- Then we use the printf() function to prompt the user to input a value and then the scanf() function to read this input and store it in the num variable.
- Next, we initiate an if-statement with a Goto label. In the statement-
- The if condition checks if the value of num is less than or equal to zero (using the relational operator), indicating an invalid input.
- If the condition evaluates to true, the program utilizes the goto statement to jump to a labeled section of code called error.
- Upon reaching the error label, the program executes a printf statement to display an error message, notifying the user about the invalid input they provided.
- However, if the input is determined to be valid (i.e., num is greater than zero), the program proceeds to calculate the square of the number by multiplying num by itself. The calculated result is then printed using the printf statement.
- Finally, the program concludes by returning a value of 0 to indicate successful execution. In case of encountering an error, a return value of -1 may be used instead.
- In this example, we have shown the output for two inputs. The first number, -5, results in the Goto error code block being executed.
- The second number, input 8, results in the execution of the if-block, where the square is printed to the console.
Note- This program allows for user input validation, performing a specific action when an invalid input is detected and providing the desired result when a valid input is received.
The Loop Iterative/ Control Statement In C
In C programming, efficient repetition of instructions or statements is sometimes essential to meet specific requirements. The loop control statements offer a powerful solution to avoid the cumbersome task of rewriting code. By utilizing looping constructs, programmers can improve code efficiency, maintainability, and readability. We will discuss the different types of loop control statements in C in the sections ahead.
How Do Loop Control Statements In C Work?
In general, there are different types of loop statements, including for loops, while loops, and do-while loops. Each type has its own syntax and usage, but they all follow a similar working principle, which is as follows:
- The loop starts by evaluating a condition.
- If the condition is true, the code block associated with the loop is executed.
- If the condition is false, the loop is terminated, and program execution continues with the next statement after the loop.
- After executing the code block, the loop control is returned to the beginning, where the condition is re-evaluated.
- If the condition is still true, the code block is executed again. This process continues until the condition becomes false.
Now, let's discuss the different loop types in detail.
The Do-While Loop Control Statement In C
The do-while loop is a control flow statement that executes a block of code repeatedly until a specified condition becomes false. It ensures that the code block is executed at least once, regardless of the condition.
Syntax :
do {
// Code that is to be executed
} while (condition);
Here,
- The do keyword initiates a do-while loop, which guarantees the execution of the lines of code inside the curly braces at least once before checking the condition.
- The while (condition) refers to the condition that will be checked once the code is executed.
- And the while keyword indicates that the code will be executed repeatedly until the condition remains true.
Code Example:
#include <stdio.h>
int main() {
int i = 1;
do {
printf("Iteration %d\n", i);
i++;}
while (i <= 5);
return 0;
}
Output:
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 5
Code Explanation:
- We declare an integer variable i and initialize it with the value of 1 inside the main() function.
- Then, we initiate a do-while loop, which contains a printf() function to print the iteration number to the console.
- The print statement consists of a formatted string that replaces the number with the value of variable i.
- After the first iteration is done, the value of i is incremented by 1, and the while condition is checked to see if the value is less than or equal to 5.
- The iterations continue till the time the value is less than or equal to 5, and the condition becomes false, indicating that i is no longer less than or equal to 5.
- Finally, the program terminates with a return 0 statement.
Throughout this execution, the code block is guaranteed to be executed at least once due to the do-while loop structure.
The While Loop Control Statement In C
The while loop is a control flow construct that iterates over a block of code repeatedly as long as a certain condition remains true. It provides a way to execute the code block repeatedly until the condition evaluates to false. It evaluates the condition before executing the code block.
Syntax :
while (condition) {
// Code that is to be executed
}
Here,
- The while keyword marks the beginning of the while loop.
- The condition is the expression we evaluate to see if the variable value meets the requirement.
- The lines of code inside the curly braces are what will be executed if the condition returns true. This code is executed repeatedly as long as the condition in the while statement remains true.
Code Example:
#include <stdio.h>
int main() {
int i = 1;
while (i <= 5) {
printf("Iteration %d\n", i);
i++;}
return 0;
}
Output:
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 5
Code Explanation:
- Inside the main() function, we begin by declaring and initializing the integer variable i with the value of 1.
- We then define a while loop with the initial condition i <= 5. This condition checks if the value of i is less than equal to 5.
- If the condition is true, the loop executes. That is, the code block with the print() function is executed, and it prints the number of iteration.
- After that, the value of i is incremented by 1, and the loop once again checks the condition. If it remains true, the loop repeats, executing the code block once more.
- This process continues until the condition eventually becomes false, at which point the loop terminates, and the program moves on to the subsequent code.
The For Loop Control Statement In C
The for loop is a control flow statement that allows executing a block of code repeatedly for a specific number of times. It consists of an initialization, condition, and increment/decrement expression.
Syntax:
for (initialization; condition; increment/decrement) {
// Code
}
Here,
- The for keyword indicates the beginning of the for loop.
- The initialization condition refers to the initial condition/ set of conditions to initialize a loop control variable.
- Next, the condition represents the boolean expression, which is evaluated, and the loop continues to iterate till the time it returns true.
- The increment/ decrement parameter refers to the increment/ decrement operators that update the value of the control variable after each iteration.
- The code inside curly braces gives the code that is executed repeatedly as long as the condition in the for statement remains true.
Code Example:
#include <stdio.h>
int main() {
int i;
for (i = 1; i <= 5; i++) {
printf("Iteration %d\n", i);}
return 0;
}
Output:
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 5
Code Explanation:
- We begin the example by declaring an integer variable i inside the main() function.
- Then, we initiate a for loop, which checks the condition i<=5, i.e., if the value of i is less than or equal to 5.
- If the condition is true, the code block within the loop is executed. It contains the printf() function which displays a message with the number of the iteration.
- Then, the value of i is incremented by 1 using the increment expression i++. The loop once again checks the condition.
- If the condition remains true, the loop repeats, executing the code block once more.
- This iterative process continues until the condition eventually becomes false, causing the loop to terminate, and the program continues with the subsequent code.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
The Jump Control Statements In C
The term jump is often used in programming to refer to control statements that allow for the non-sequential transfer of program execution to a specific location within the code. However, it's important to note that the use of explicit jump control statements in C, such as goto or similar constructs, is generally discouraged in modern programming practices due to the potential negative impact on code readability and maintainability.
There are three primary jump statements in C, i.e.,-
- The Goto Statement (often considered bad practice because it can make code less readable and harder to understand)
- The Break Statement
- The Continue Statement
It's important to note that excessive use of these statements can make code less readable and harder to maintain, so they should be used judiciously. Instead, structured control flow constructs like loops, conditionals, and functions are preferred to achieve clear program flow. These constructs provide a more organized and maintainable approach to controlling program execution.
The Break Control Statement In C
The break statement is commonly used within loops (like for, while, or do-while) and switch statements to exit the enclosing loop or switch block prematurely. It helps in terminating the loop execution or skipping the remaining case statements in a switch.
Syntax:
break;
How Does Break Control Statements In C Work?
In general, the break statement works as follows:
- When encountered within a loop or switch statement, the break statement immediately exits the innermost loop or switch statement.
- Program execution continues with the next statement after the loop or switch block.
Code Example:
#include <stdio.h>
int main() {
int i;
for (i = 1; i <= 10; i++) {
if (i == 6) {
break;}
printf("%d ", i);}
return 0;
}
Output:
1 2 3 4 5
Code Explanation:
- We declare a variable i and assign it the value of 1 inside the main() function (using the equal to assignment operator).
- Next, we create a for loop, which contains a break statement inside an if statement. Inside the loop-
- The for loop is executed with the variable i initially set to 1. It continues until the value of i reaches 10, incrementing by 1 with each iteration.
- At each iteration, a printf() function outputs a number for the console.
- However, when the if condition is met, that is, the value of i becomes 6, the break statement is encountered. This causes the loop to terminate prematurely, and the program proceeds to execute the subsequent code outside the loop.
- Consequently, any remaining iterations of the loop are skipped, and the program continues with the rest of the program logic or statements following the loop.
- This enables the program to control the flow of execution and selectively exit a loop based on certain conditions, allowing for more efficient and flexible program behavior.
The Continue Control Statement In C
The continue statement is used within loops to skip the remaining statements within the loop block for the current iteration. It allows the program to jump back to the loop condition and proceed with the next iteration.
How Do Continue Control Statements In C Work?
In general, the continue statement works as follows-
- When encountered within a loop, the continue statement immediately ends the current iteration and jumps to the next iteration.
- Program execution continues with the next iteration of the loop, skipping any remaining statements within the loop block for the current iteration.
Syntax:
continue;
Code Example:
#include <stdio.h>
int main() {
int i;
for (i = 1; i <= 5; i++) {
if (i == 3) {
continue;}
printf("%d ", i);}
return 0;
}
Output:
1 2 4 5
Explanation:
- In the provided code, we first declare an integer variable i without initialization.
- Then, we create a for loop with the variable i initially set to 1. Inside the loop-
- The loop uses a printf() function to output a number to the console till the condition i <= 5 returns true.
- After every iteration, the value of i is incremented by 1.
- However, there is a continue statement inside the loop, which comes into effect when the if condition i==3 is met.
- So when the value of i becomes 3, the continue statement is encountered, which causes the remaining statements within the loop block to be skipped, and the program proceeds directly to the next iteration of the loop.
- As a result, the statements following the continue statement are not executed for that particular iteration. That is, the value of 3 is not printed to the console.
- The program then resumes with the loop condition check and proceeds with the subsequent iterations until the loop condition eventually becomes false.
- This allows for selective skipping of certain iterations based on specified conditions, facilitating more controlled and efficient loop execution.
Conclusion
The control statements in C are indispensable tools for shaping the execution flow in programs. By mastering the use of if, else, switch, loops, and jump statements, developers can create efficient, readable, and maintainable code. Adhering to best practices ensures that control statements enhance, rather than hinder, the development process. As with any programming concept, practice and experience play key roles in becoming proficient in using control statements effectively in C.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. What are control statements in C programming? Explain the different types of control statements in C.
Control statements in C programming are constructs that manage the flow of execution in a program. They determine the order in which statements are executed based on certain conditions or loops. The main types of control statements in C are given below.
Selection Statements: The statements check a condition whose boolean result determines which set of code will be executed. It consists of-
- If statement: Executes a block of code if a specified condition is true.
- If-Else statement: Provides an alternative block of code to execute if the condition of an if statement is false.
- Switch statement: Allows for multi-way branching based on the value of an expression.
Iteration Statements (Loops): These conditional statements in C allow programmers to perform repetitive tasks without having to write the code over and over again. It consists of-
- For loop: Repeatedly executes a block of code a specified number of times.
- While loop: Repeatedly executes a block of code as long as a given condition is true.
- Do-while loop: Similar to the while loop, but the condition is checked after the block of code is executed, ensuring at least one execution.
Jump Statements: These statements help jump from one section of the code to another, given certain pre-specified conditions. It consists of-
- Break statement: Exits from a loop or switch statement prematurely.
- Continue statement: Skips the rest of the code inside a loop for the current iteration and moves to the next iteration.
- Return statement: Exits from a function and optionally returns a value.
Control statements provide programmers with the tools to create flexible and dynamic program logic by enabling decisions, repetitions, and alterations in the flow of execution based on specified conditions.
Q. How do selection statements work in C?
Selection statements in C are used to control the flow of the program based on certain conditions. There are two main types of selection statements in C, i.e., the if-statement and switch statement.
The if-statement: The if statement is used to make decisions based on a condition. It has the following syntax:
if (condition){
// Code to be executed if the condition is true
}else{
// Code to be executed if the condition is false
}
The switch statement: The switch statement is used when you have multiple possible execution paths based on the value of an expression. It has the following syntax:
switch (expression){
case label 1:
code block 1
break;
case label 2:
code block 2
break;
// ... more cases ...
default:
defaul_code block}
These statements help you control the flow of your program based on certain conditions, allowing for more dynamic and flexible code execution.
Q. What is the purpose of iterative statements in C?
Iterative statements, or loops, enable repetitive execution of code blocks. They provide a way to perform tasks multiple times until a specified condition becomes false.
- The while loop executes the code block while a condition is true.
- The do-while statement executes the code block at least once and repeats as long as the condition is true.
- The for loop provides a structured approach for executing code a fixed number of times.
Q. Are there any limitations or best practices for using control statements in C?
While control statements are essential for creating logic and controlling the flow of a program in C, it's important to be aware of certain limitations and best practices to write efficient and maintainable code. Here are some considerations:
Limitations:
- Nested Control Statements: Excessive nesting of control statements in C programs, especially deep nesting, can make the code harder to read and maintain. It's advisable to keep the nesting levels to a minimum.
- Overuse of Goto: The use of the goto statement for sequential control flow is generally discouraged as it can lead to unreadable and error-prone code. It makes it harder to understand the logical structure of the program.
Best Practices:
- Avoid Complex Conditions: While conditions in control statements are necessary, an overly complex series of conditions can make code difficult to understand. It is best to break down complex conditions into simpler ones or use additional variables to store intermediate results.
- Consistent Indentation: Maintain consistent and clear indentation to enhance code readability. This is particularly important for nested control statements in C.
- Use Switch Wisely: The switch control statement in C is useful for multi-way branching, but it can be misused. Avoid overly complex switch statements and consider using if-else constructs or other alternatives if the logic becomes convoluted.
- Clarity over Cleverness: Prioritize code clarity over clever optimizations. The code should be easy to read and understand by others who may maintain or collaborate on the project.
- Avoid Unconditional Jumps: Minimize the use of unconditional jumps (goto, break, continue) as they can make the code harder to follow. Use them sparingly and only when necessary.
- Error Handling: Properly handle errors and edge cases. Avoid using control statements in C programs for error suppression or ignoring potential issues.
- Modularize Code: Break down complex logic into smaller, modular functions. This enhances code organization and makes it easier to test and maintain.
- Code Reviews: Regularly conduct code reviews to get feedback on control flow and logic. This can help identify potential issues and improve code quality.
Q: Can control statements in C be nested?
Yes, control statements can be nested in C. Nested refers to the situation where one control statement is placed inside another. This means that the body of one control statement (e.g., an if statement, for loop, or while loop) contains another control statement.
For example, you can have an if statement inside a for loop, a while loop inside an if statement, or any combination of nested control statements.
Here's a program code with a nested if-else construct inside a for loop:
Code:
#include <stdio.h>
int main() {
for (int i = 0; i < 5; i++) {
if (i % 2 == 0) {
printf("%d is even.\n", i);
} else {
printf("%d is odd.\n", i);}
}
return 0;
}
Output:
0 is even.
1 is odd.
2 is even.
3 is odd.
4 is even.
Explanation:
In this example, the if statement is nested inside the for loop. The program prints whether each value of i is even or odd during each iteration of the loop.
This brings us to the end of our discussion on control statements in C. Here are a few other important topics you must read:
- Logical Operators In C Explained (Types With Examples)
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
- Arithmetic Operators In C, Precedence & Associativity (+Examples)
- 5 Types Of Literals In C & More Explained With Examples!
- Comma Operator In C | Code Examples For Both Separator & Operator
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment