C Program To Reverse A Number | 6 Ways Explained With Examples
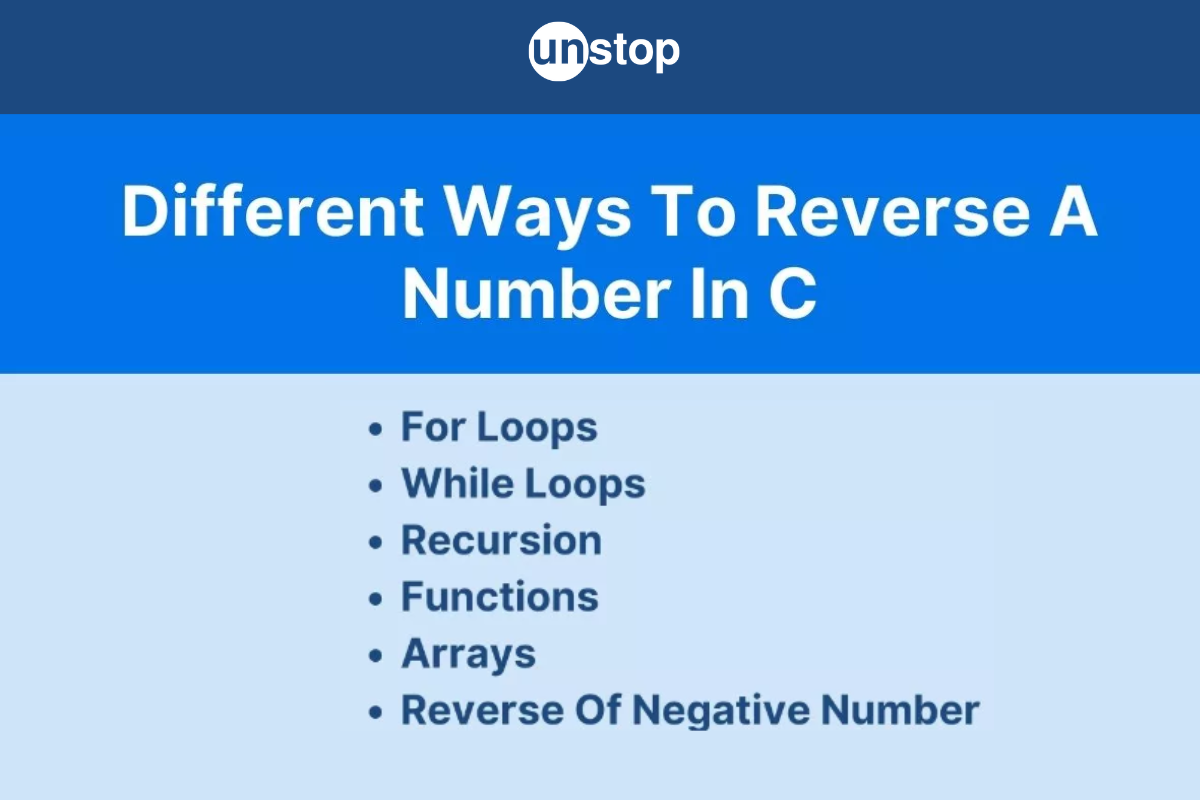
Table of content:
- Understanding Algorithm & Approach To Reverse A Number In C
- Flowchart Of C Program To Reverse A Number
- C Program To Reverse A Number
- C Program To Reverse A Number Using While Loop & User Input
- C Program To Reverse A Number Using For Loop
- C Program To Reverse A Number Using Recursion
- C Program To Reverse A Number Using Function
- C Program To Find Reverse Of Negative Number Inputs
- Using Arrays For C Program To Reverse A Number
- Conclusion
- Frequently Asked Questions
Reversing a number is a common task in programming that involves rearranging the digits of a given number in the opposite order. This operation can be useful in various scenarios, such as checking for palindrome numbers or solving mathematical puzzles to check your coding skills and clarity of various programming topics. In this article, we will explore an efficient approach to reverse a number using the C programming language.
We will try to understand the algorithm, logic, and necessary programming skills. We will also examine the algorithm’s space and time complexity. Let’s first start by understanding the algorithm of a C program to reverse a number and its logic.
Understanding Algorithm & Approach To Reverse A Number In C
Textually (or visually), you can simply write the number in the reverse sequence of digits. For example, if the number is– 12345, the reverse number will be 54321.
But when it comes to programming, you need to use proper logic to get a computer to do the same. There are naturally many ways to get this done. Below, we have explained an algorithm or the logic behind a C program to reverse a number.
Approach:
The following steps outline the approach to reversing a number in C, using some basic mathematical operations along with loops:
- Declare two variables:
-
- The first integer variable, say num, will hold the original number.
- The second integer variable, say reverseNum, will store the reversed number.
- Take user input: Use printf() and scanf() functions to prompt the user for input and read it to be stored in the variable num.
- Reverse the number:
-
- Initialize variable reverseNum with 0, as it will store the reversed number.
- Next, use a loop, say a while loop with a condition that num is greater than 0.
- Inside the loop:
-
- Multiply reverseNum by 10 to shift the digits one place to the left.
- Add the last digit of num to reverseNum using the modulo operator (%) and division operator (/).
- Update num by dividing it by 10, discarding the last digit.
- Repeat these steps until num becomes 0.
- Output the reversed number: Use the printf() function to display the reversed number reverseNum to the user.
To achieve this in C programming, we will use the following arithmetic operators:
- The Modulus Operator (%): This provides the remainder and will be used to get the last digit of the num. E.g., 2765 % 10 = 5
- The Division Operator (/): This provides the quotient and will be used to remove the last digit of the num. E.g., 2765 / 10 = 276
The table below gives a step-by-step explanation of how we reverse the number 3425, for example.
Iteration |
num |
(num % 10) |
rev_num * 10 + (num % 10) |
num = num/10 |
1 |
3425 |
5 |
0 * 10 + 5 = 5 |
342 |
2 |
342 |
2 |
5 * 10 + 2 = 52 |
34 |
3 |
34 |
4 |
52 * 10 + 4 = 524 |
3 |
4 |
3 |
3 |
524 * 10 + 3 = 5243 |
0 |
As we can see, when the value of num gets to zero at the end of the 4th iteration, we have our reversed number stored in rev_num.
Let’s understand how to reverse a number in C with the help of a flowchart.
Flowchart Of C Program To Reverse A Number
As shown in the flowchart, we begin the process to reverse a number in C by reading the user input for the number num (which we will reverse).
- Then, we initialize the variable rev_num to 0 so that we can iteratively assign it the reversed digits.
- The program flow then passes to the loop, which continues as long as the num is greater than 0.
- Within each iteration, the last digit of num is extracted using the modulo operator (%) and added to rev_num after shifting its digits one place to the left.
- The num is updated by dividing it by 10, effectively removing the last digit.
- Finally, the rev_num will contain the reversed number which print to the console.
This is the general logic behind writing and running a C program to reverse a number. Though we discussed the approach using the while loop, there are many ways we can reverse a number in C.
In the following sections, we will discuss these approaches along with detailed examples and explanations, as well as the time and space complexity of the source code.
C Program To Reverse A Number
The code below is an implementation of the flowchart given and the algorithm to reverse a number in C for a better understanding of the concept.
Code Example:
Output:
Reversed Number: 54321
Explanation:
In the simple C code example, we first include the essential header file <stdio.h> for input/ output operations.
- We declare two variables, num, and rev_num, inside the main() function and initialize them with the values of 12345 and 0, respectively.
- Next, we start a while loop that first checks if value of num is not equal to zero (num !=0) before every iteration. If the condition is true, the loop's body will execute. If it is false, then the loop will terminate.
- The while loop will run for five iterations since there are five digits in the num value.
- After 5 iterations, the value of num becomes 0, and the loop terminates.
- Then, we use the printf() function to print the reversed number, which is the value of rev_num after five iterations.
- Here, the %d format specifier is the placeholder for an integer value and the newline escape sequence shifts the cursor to the next line, after printing the output.
- Lastly, the main() function returns 0, indicating successful execution.
Time and Space Complexity Analysis:
Time Complexity
For an n-digit number, the loop/recursive function will execute n times. The number of digits of a number K is given by log10(K). So the time complexity of the above-used algorithm will be O(log10(K)).
Space Complexity
Since we are not using any extra space for storing the last digit and the reversed number, thus the Space complexity will be O(1).
C Program To Reverse A Number Using While Loop & User Input
A while loop checks the condition first and keeps executing the body till the time the loop condition returns true. In the example below, we will take user input and then reverse the number using a while loop.
Code Example:
Output:
Enter an integer to be reversed: 186549
Reversed Number: 945681
Explanation:
In the simple C program example,
- We declare two variables, num, and rev_num, in the main() function, separated by comma. We initialize rev_num with 0, while num will store user input.
- Then, we prompt the user to provide a number to reverse using printf() and read it using scanf(), storing it in num.
- Next, we start a while loop where it first checks the condition (num != 0) at the start of every iteration. If the condition is true, the loop's body will execute; otherwise, the loop will terminate.
- The while loop will run for six iterations since there are six digits in the num value (186549). Look at the step-by-step iteration breakdown in the table below:
Iteration
num != 0
(Condition/ at start of iteration)Last Digit
(num % 10)Updated rev_num
[rev_num * 10 + (num% 10)]Updated num
(num = num/10)1
true
9
0 * 10 + 9 = 9
18654
2
true
4
9 * 10 + 4 = 94
1865
3
true
5
94 * 10 + 5 = 945
186
4
true
6
945 * 10 + 6 = 9456
18
5
true
8
9456 * 10 + 8 = 94568
1
6
true
1
94568 * 10 + 1 = 945681
0
7
false
- After 6 iterations, the value of num becomes 0, and the loop terminates.
- Finally, we use printf() to display value of rev_num, which after six iterations holds the reversed number. As seen in the console, the output of code is 945681.
C Program To Reverse A Number Using For Loop
In this section, we will use a for loop instead of a while loop to execute the algorithm. Unlike, the while loop, this looping statement consists of three parts– the initialization condition, the loop condition and the updation condition.
Below is an example C program to reverse a number using a for loop.
Code Example:
Output:
Reversed Number: 54021
Explanation:
In the code example above,
- We declare two variables, num, and rev_num, in the main() function and initialize them with the values of 12045 and 0, respectively.
- We then start a for loop where it has three components:
-
- In the first statement we set the initial value for the loop counter variable i to 0 (i=0).
- The second is the loop condition (num != 0), which is checked at the start of every iteration. If the condition is true, the loop's body will execute; otherwise, the loop will terminate.
- In the third statement we set the updation condition, i.e., to increment the value of the counter variable by 1 after every iteration.
- The for loop will run for five iterations since there are five digits in the num value. The table below gives a step-by-step breakdown of the iterations:
Iteration
num != 0 (Condition/ at start of iteration)
Last Digit (num % 10)
Updated rev_num [rev_num * 10 + (num% 10)]
Updated num (num = num/10)
1
true
5
0 * 10 + 5 = 5
5402
2
true
4
5 * 10 + 4 = 54
540
3
true
0
54 * 10 + 0 = 540
54
4
true
2
540 * 10 + 2 = 5402
5
5
true
1
5402 * 10 + 1 = 54021
0
6
false
- After 5 iterations, the value of num becomes 0, and the loop terminates.
- Lastly, we use the printf() function to print the reversed number, which is the value of rev_num after five iterations.
- As seen in the window, the program output is 54021.
Check out this amazing course to become the best version of the C programmer you can be.
C Program To Reverse A Number Using Recursion
In this section, we will discuss the recursive approach to reverse a number in C. To begin with, a recursive function calls itself repeatedly until a specific condition/subtask is satisfied. Recursion helps simplify complex problems by breaking them down into smaller, manageable steps.
Let's take a look at a sample C program to reverse a number using recursion for a better understanding of the concept.
Code Example:
Output:
Reverse Number: 12389
Explanation:
In the C example program above,
- We begin by defining a recursive function called reverse, which takes two integer variables n (original number) and k (will hold the reversed number) as inputs.
- The function successively removes the last digit in n and appends it to k, which effectively reversing the number.
-
- As mentioned in the code comments, we set a base case using the if-statement to check if value of n is equal to 0. If yes, then the function returns the value of k.
- If not, then the function appends the last digit of n to k by calculating (k * 10 + n % 10).
- And then it removes the last digit from n by updating it to n / 10
- The process repeats (passing updated values to the function) until the base case is true.
- Next, inside the main() function, we declare two variables, num and rev_num, and initialize them with the values of 98321 and 0, respectively.
- We then call the recursive function reverse() passing num and rev_num as its arguments. The result of the function will be an integer data type stored in the variable ans.
- The recursive function runs for five iterations, as shown below in the table:
Iteration
n-value
k-value
return value
0
98321
0
reverse(98321,0)
1
9832
1
reverse(9832,1)
2
983
12
reverse(983,12)
3
98
123
reverse(98,123)
4
9
1238
reverse(9,1238)
5
0
12389
As n=0, Instead of calling reverse, k is returned with the Value 12389
- After 5 iterations, the reversed number is stored in the ans variable as returned from the recursive function.
- Lastly, we use the printf() function to print the reversed number, which is the value of ans.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
C Program To Reverse A Number Using Function
A function is a modular block of code designed to take inputs, process them, and return an output. We can use functions (much like the reverse function before) in the C program to reverse a number.
The function body will enclose the logic you want to use to reverse the function. You can then call the function passing the number you want to reverse as argument. In the C code example below, we have used the while loop inside the function to reverse a number.
The example provides a clearer understanding of how to use functions in a C program to reverse a number.
Code Example:
Output:
Reversed Number is: 4321
Explanation:
In the example C code,
- We define a function reverse() that takes two integer parameters: n (the number to be reversed) and rev (which will hold the reversed number), and uses a while loop for reversing logic.
-
- The while loop begins by checking if the value of n is not equal to 0 (n != 0). As long as this condition holds true, the loop will execute.
- Within the loop, the last digit of n is appended to rev using the expression rev = rev * 10 + n % 10, effectively building the reversed number step by step.
- The last digit is then removed from n by performing integer division (n /= 10).
- In the main() function, we declare two integer variables: num, initialized to 1234 (the number we want to reverse), and rev_num, initialized to 0.
- We then call the reverse() function, passing num and rev_num as arguments, which allows the function to manipulate the values.
- The while loop inside the reverse() function will run for four iterations, corresponding to the four digits in num.
- After four iterations, n becomes 0, the loop terminates, and the reversed number is returned to the main function.
- Finally, we use the printf() function to display the reversed number stored in the result variable, which outputs 4321.
C Program To Find Reverse Of Negative Number Inputs
Can we reverse a negative number in C directly? If not, then what is the issue? This question arises can we reverse a negative number in C directly? Sadly, the answer is yes, there are issues when reversing a negative number. In this section, we will explore this in depth.
The main issue with applying the above algorithm for negative numbers is that some compilers ignore the negative numbers and provide wrong results. This is because there is a change in the binary representation of negative numbers. So in the case of negative numbers, we first have to multiply it by -1 to make it positive and then reverse it. This reversed number is again multiplied with -1 to obtain its negative value, our answer.
Code Example:
Output:
Reversed Number is: -4321
Explanation:
In the code example above,
- In the main() function, we declare two integer variables, n and rev_num, initialized to -1234 and 0, respectively.
- We also declare a third integer variable called flag, initialized to 1, indicating that n is initially treated as positive.
- Next, we use an if statement to check if n is negative. If it is, we convert it to positive by multiplying by -1 and update flag to 0.
- Then, we start a while loop which first checks if n is not equal to 0, i.e., loop condition n!=0.
- As long as this condition holds true, the loop will execute, appending the last digit of n to rev_num. After four iterations, n becomes 0, and the loop terminates.
- We then check the value of flag. If it equals 0 (indicating that the original number was negative), we multiply rev_num by -1 to convert it back to a negative number.
- Finally, we print the reversed number using printf(), which outputs -4321.
Using Arrays For C Program To Reverse A Number
An array is a collection of elements (of same data types) that are stored in contiguous memory locations. It provides easy ways to store, manage and manipulate the collection of data. In this section, we will discuss how to write a C program to reverse a number, using arrays.
Essentially, we will first store number in an array and then print the array to get the reversed number. Each digit of the number is stored in an array using a loop or recursion so that the time complexity will remain the same as in other methods.
The sample below showcases how to write a C Program to reverse a number using an array.
Code Example:
Output:
Reversed Number is: 54321
Explanation:
In the sample C code,
- In the main() function, we declare an interger variable called num and initialize it to 12345.
- Then, we declare an integer array arr[5] to hold the 5 digits of the integer and initialize all elements to 0.
- Next, we begin a for loop with loop variable initially set to 0 and will run until num is equal to 0 (i.e., n!=0).
-
- During each iteration, we store the last digit of the numbers num (i.e., num%10) in arr[i].
- After than we remove that digit from the num variable using integer division (i.e., num/10).
- The for loop will run for five iterations since there are five digits in the original value of num. After five iterations, num becomes 0, and the loop terminates.
- Lastly, we use the printf() function along with a fresh loop to print the value at each index of the array arr, which prints the number in reversed order.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
Reversing a number in C language is a simple yet fundamental task that illustrates key programming concepts. By utilizing basic mathematical operations and loops, we can efficiently implement a C program to reverse a number.
The various methods discussed, including handling negative inputs and utilizing arrays, serve as foundational techniques for more complex number manipulation tasks. Feel free to experiment with the provided code snippets to deepen your understanding and apply these concepts to tackle diverse programming challenges.
Also Read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. How to reverse a 5-digit number in C?
There are multiple ways in a C program to reverse a number. The example below shows how we use one of these methods to reverse a 5-digit number in C.
Code Example:
Output:
Reversed Number: 94568
Explanation:
In the code example above,
- Inside the main function, we declare two variables num and rev_num, and initialize them with the values of 86549 and 0, respectively.
- We then start a while loop where it first checks the condition (num != 0) at the start of every iteration. If the condition is true, the loop's body will execute; otherwise, the loop will terminate.
- The while loop will run for five iterations since there are five digits in the num value. After 5 iterations, the value of num becomes 0, and the loop terminates.
- Lastly, we use the printf() function to print the reversed number, which is the value of rev_num after five iterations.
Q. How to remove a digit from a number in C?
To remove the last digit from a number in C, we can directly use the divide (/) arithmetic operator and divide the number by 10 to get the quotient which will be our required number.
For example, Consider removing the last digit from the number 176496. On dividing the number by 10, we get the quotient as 176496 / 10 = 17649, which is our required answer.
Code Example:
Output:
17649
Q. What does *= do in C?
The asterisk sign followed by an equal sign (*=) is a compound assignment operator. It working mechanism is two-fold, where in it first multiplies the right operand’s value with the left operand’s value, and the result is assigned to the left operand.
That is, A *= B or A = A * B. An example of how this is used in a C program is given below.
Code Example:
Output:
Value of b is: 123
Q. What is n /= 10 in C?
The operator /= is simply an assignment operator that divides the rightmost digit operand’s value with the left operand’s value, and the result is assigned to the left operand. Now n /= 10 means n = n/10, which simply divides the value of n by 10 and assigns it to n.
Source Code:
Output:
Value of n /= 10 is: 1234
Q. How do you reverse a number without a loop in C?
To reverse a number in C without a loop, we can use the recursive approach which breaks a problem into smaller tasks to be handled one at a time. For this we define a recursive function which calls itself again and again, until the base case is satisfied. The following code shows a C program to reverse a number using recursion.
Source Code:
Output:
Reverse Number: 12389
Explanation:
In the code example above,
- We define a reverse() function which takes two integers as parameters, n for original number and k to store the reversed number.
- In the base case, it checks if the value of n is 0, if so, it returns k. If not, it removes the last digit of n and appends it to the k, effectively reversing the number, recurssively.
- In the main() function, we declare two variables, num, and rev_num, and initialize them with the values of 98321 and 0, respectively.
- We then call the recursive() function passing num and rev_num as its arguments.
- The function recursively calls itself 5 times, after which the function returns the reversed number stored in the rev_num variable.
- Lastly, we use the printf() function to print the value of rev_num.
Q. How to reverse negative integers in C?
The main issue with reversing negative numbers is that some compilers altogether ignore the negative numbers and provide wrong results. This is because there is a change in the binary representation of negative numbers compared to positive ones.
So in the case of negative numbers, we first have to multiply it by -1 to make it positive and then reverse it. This reversed number is again multiplied by -1 to obtain its negative value, which will be our answer.
Source Code:
Output:
Reversed Number is: -4703
Explanation:
In the code example above,
- We declare two variables n and rev_num in the main function, and initialize them with the values of -1234 and 0, respectively.
- A flag variable is declared and initialized to 1 for positive values of n.
- Then check if value of n is negative, if yes, we make it positive by multiplying it by -1 and update the value of flag to 0 for n being negative.
- We then start a while loop which checks if n is not equal to zero(n != 0) before every iteration. If the condition is true, the loop's body will execute; otherwise, the loop will terminate.
- The while loop will run for four iterations since there are four digits in the n value. After which, the value of n becomes 0, and the loop terminates.
- We then check for the value of the flag. If it is equal to 0, then the value of rev_num is made negative.
- Lastly, we use the printf() function to print the reversed number, which is the value of rev_num after four iterations.
This shows how to write a C program to reverse a number with a negative sign.
You might also be interested in reading the following:
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Control Statements In C | The Beginner's Guide (With Examples)
- Strings In C | Declare, Initialize & String Functions (+Examples)
- Type Casting In C | Cast Functions, Types & More (+Code Examples)
- Union In C | Declare, Initialize, Access Member & More (Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment