- Calculating The Sum Of N Natural Numbers
- How To Find The Sum Of N Natural Numbers In C Program?
- Find The Sum Of N Natural Numbers In C Using For-Loop
- Find The Sum Of N Natural Numbers In C Using While Loop
- Find The Sum Of N Natural Numbers In C Using Do-While Loop
- Find The Sum Of N Natural Numbers In C Using Direct Mathematical Formula
- Find The Sum Of N Natural Numbers In C Using Function
- Find The Sum Of N Natural Numbers In C Between A Given Range
- Find The Sum Of N Natural Numbers In C Using Recursion
- Find The Sum Of N Natural Numbers In C Using Array
- Find The Sum Of N Natural Numbers In C With User Input Until A Positive Integer Is Entered
- Time & Space Complexity Comparison For Programs To Find Sum Of N Natural Numbers In C
- Conclusion
- Frequently Asked Questions
How To Find Sum Of N Natural Numbers In C? (8 Methods + Codes)
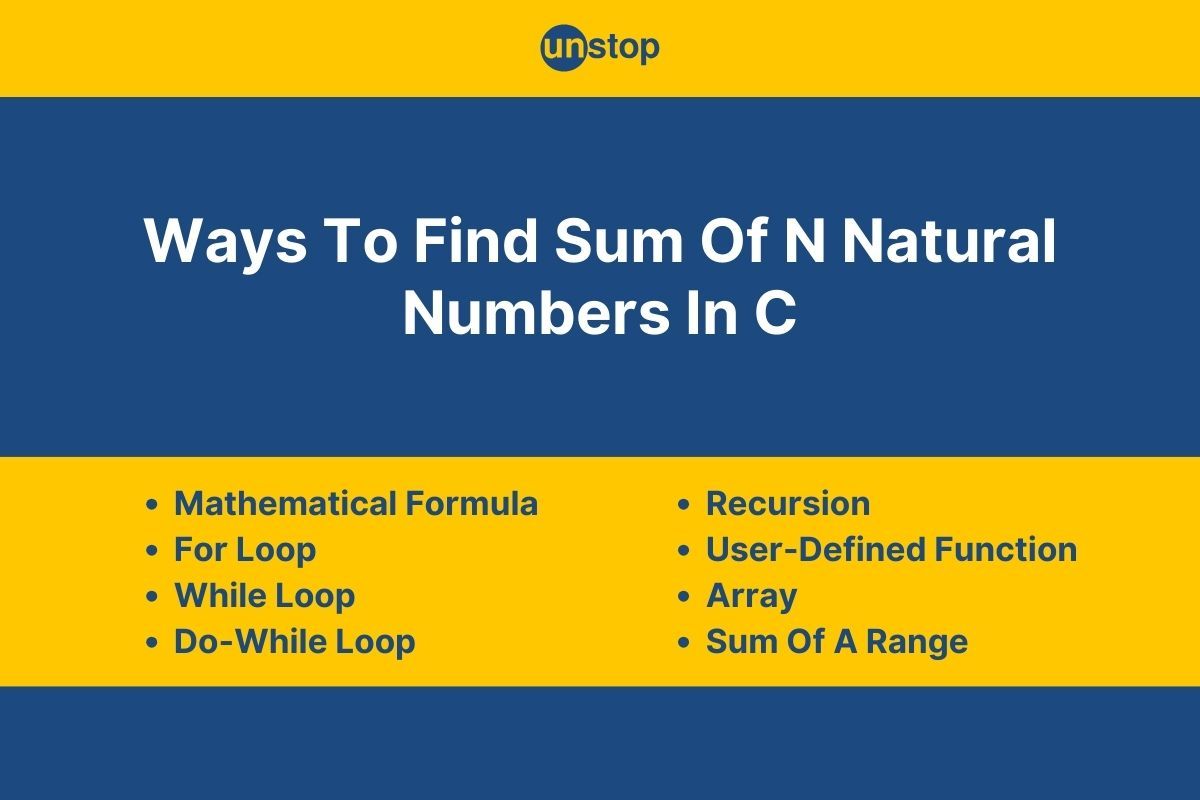
Natural numbers, also called counting numbers, are part of the number system. They include all the positive integers starting from 1 until infinity but exclude 0 and negative integers. The set of natural numbers is denoted by N. These numbers are used in multiple fields of study, including mathematics (arithmetic, algebra, geometry, and calculus), physics, chemistry, and computer science. Naturally, the concept of the sum of n natural numbers also has many applications in these fields.
In this article, we will explore the methods to calculate the sum of N natural numbers in C programming. To begin with, the sum of N natural number refers to the sum of the first n positive integers, starting from 1. The formula for this is Sn = n (n + 1) / 2, where Sn is the sum and n is the number of natural numbers.
Calculating The Sum Of N Natural Numbers
We will discuss various techniques we can use to find out the sum of n natural numbers in C, with examples and in-depth explanations for each of the methods. But before that, let's look at some basic mathematics behind the concept.
The sum of n natural numbers is usually computed using an arithmetic progression (A.P.). It is a sequence of numbers where the difference between any two consecutive numbers is constant (i.e., d), called the common difference. The sum of an A.P. can be found using the below formula:
Sn = (n/2)*[2*a + (n-1)*d] = (n)(n+1)/2;
Here, Sn denotes the sum of the arithmetic progression, n is the number of elements, a is the first element and d is the common difference between elements. In this case, the difference will be 1.
Example:
Suppose we want to find the sum of five natural numbers. Then-
- No of elements (n) = 5.
- The starting element (a) is 1.
- Common difference (d) = 1.
- As Sn = (n/2)*[2*a + (n-1)*d], we put all the values in the formula.
- So Sn = (5/2) [2*1 + (5-1)*1] = (5/2)*(6) = 15
- 1+2+3+4+5 = 15, so the answer we got from the above formula is correct.
Now that we know the mathematical basics, let's learn how to calculate the sum of N natural numbers in C language.
How To Find The Sum Of N Natural Numbers In C Program?
The most common method to calculate the sum of N natural numbers in C is through iteration. We can use loops and other constructs for this. An alternative would be to directly use the mathematical formula in the code. Here are the steps you must follow to write and run a C program to find the sum of N natural numbers through iteration:
- Declare a variable n, which keeps the count of natural numbers whose sum we want to calculate.
- Employ a loop/ iterative construct and define a temporary variable i, which will be used to iterate through 1 to n.
- Define a variable, say, sum, to store the sum as we iterate through the set of numbers. Its initial value will be set to 0.
- The iteration process begins with i=1 and continues till 1=n. At every iteration, we keep adding i to the sum.
- After all the iterations are done, the value of the sum variable will be the sum of N natural numbers.
- Use the printf() function to print the sum of n natural numbers.
Pseudo Code Of Program To Calculate Sum Of N Natural Numbers In C:
Let n: number of natural numbers
Let i: counter variable to iterate from 1 to n
Let sum: variable to store the sum of the n natural numbersinitialize the sum to 0
for i = 1 to n
add i to sumprint the sum
Time Complexity: O(n)
Space Complexity: O(1)
Find The Sum Of N Natural Numbers In C Using For-Loop
As mentioned before, we can use loop constructs and other control statements to calculate the sum of N natural numbers in C language. In this section, we will discuss how to use a for loop to find this sum, where you must begin by initializing a variable to store the sum and iterating through each natural number from 1 to n, adding each number to the sum in each iteration. Once the loop completes, the sum variable holds the total sum of the first n natural numbers.
Syntax:
for(int i=1; i<=n;i++){
sum += i;
}
Here,
- The term n refers to the count of natural numbers whose sum we want to calculate, which is stored in a variable whose name/ identifier is sum.
- The loop variable i is used to control the iterations of the for loop whose initiation is marked by the for keyword.
The simple C program below showcases how to find the sum of N natural numbers using this method.
Code Example:
#include <stdio.h>
int main(){
//Setting variable for total count of numbers and sum variable with initial value 0
int n, sum = 0;
//Prompting user to provide count of numbers whose sum we must calculate
printf("Enter the value of n: ");
scanf("%d", &n);
//Initiating a for loop to calculate the sum of N natural numbers
for (int i = 1; i <= n; i++) {
sum += i;
}
printf("The sum of first %d natural numbers is: %d", n, sum);
return 0;
}
Output:
Enter the value of n: 8
The sum of first 8 natural numbers is: 36
Explanation:
We begin the C code example by including the <stdio.h> header file essential for input-output operations.
- After that, we initiate the main() function, which serves as the program's entry point of execution.
- Inside main(), we declare two integer data type variables, i.e., n to store the user input and sum to store the sum of natural numbers.
- Next, as mentioned in the code comment, we use the printf() function to print a string message prompting the user to enter the value of n.
- Once the user provides input, we use the scanf() function to read the value. The %d format specifier indicates that the value is of integer type, and we store it in the respective variable using the reference/ address-of operator (&).
- Following this, we initiate a for loop, with control variable i iterating from 1 to n. Inside the loop-
- In every iteration, we use the compound addition assignment operator to add previous value of i to the value of sum variable which was 0 intially.
- At the end of every iteration, we increment the value of i by 1. This iterative addition and assgiment of total to sum variable goes on till i<=n. After that, the loop terminates.
- Once the loop completes iterations, the value stored in the sum variable will be the sum of n natural numbers.
- Next, we use the printf function to display this value on the output console.
- Finally, the main() function terminates with a return 0 statement, indicating a successful completion of the program.
Time Complexity: O(n)
Space Complexity: O(1)
Find The Sum Of N Natural Numbers In C Using While Loop
Using a while loop to find the sum of n natural numbers involves initializing a sum variable to store the total sum and an iterator variable to track the current natural number being added. The loop iterates while the iterator is less than or equal to n, continuously adding the iterator value to the sum and incrementing the iterator. Once the loop completes, the sum variable holds the sum of the first N natural numbers.
Syntax:
while(i<=n) {
sum += i;
i++;
}
Here,
- The variable n is the count of natural numbers whose sum we want to calculate iteratively.
- The control/ loop variable i controls the iterations of the loop and the sum of the numbers is stored in the sum variable.
Let's look at a sample C program that shows the implementation of this method.
Code Example:
#include <stdio.h>
int main(){
int n, sum = 0;
printf("Enter the value of n: ");
scanf("%d", &n);
int i = 1;
while(i<=n) {
sum += i;
i++;
}
printf("The sum of first %d natural numbers is: %d", n, sum);
return 0;
}
Output:
Enter the value of n: 7
The sum of first 7 natural numbers is: 28
Explanation:
In the example C code-
- Inside the main() function, we declare two integer variables, i.e., n to store the count of natural numbers and sum to store the sum of natural numbers.
- Next, we use the printf() and scanf() functions to prompt the user to enter the value of n and store it in the variable for use.
- After that, we initialise a loop counter i and set its initial value to 1.
- We then define a while loop to iterate on numbers from 1 to n and calculate the sum. Inside the loop-
- We add the value of i to the sum variable in every iteration and store it back in the sum variable.
- After the iteration, we increment the value of i by 1 and move to the next iteration.
- The loop runs until the condition i <= n is true, which means it iterates from 1 to n.
- After the iterations are done, the loop terminates, and we get the sum of n natural numbers stored in the sum variable.
- We then use the printf() function again to display this value to the console.
- Finally, the main() function returns 0 to indicate successful completion without any errors.
Time Complexity: O(n)
Space Complexity: O(1)
Find The Sum Of N Natural Numbers In C Using Do-While Loop
We can compute the sum of n natural numbers in C easily using a do-while loop, too. The steps involved are similar to the while loop method. The only difference is that the code block will be executed at least once before checking if the condition is true, and then it will repeat the block of code as long as the loop condition is true.
Syntax:
do {
sum += i;
i++;}
while (i <= n);
Here,
- The count of natural numbers whose sum we want to find is given by variable n.
- The variable sum is used to store the sum of the numbers from 1 to n.
- Loop variable i is used to control the iterations of the loop from 1 to n. The first iteration is done without any condition check.
The example C program below shows how you can use the do-while loop to find the sum of N natural numbers. This can be used for any value of N.
Code Example:
#include <stdio.h>
int main(){
int n, i, sum = 0;
printf("Enter a natural number: ");
scanf("%d", &n);
i = 1;
do {
sum += i;
i++;
} while (i <= n);
printf("The sum of first %d natural numbers is: %d\n", n, sum);
return 0;
}
Output:
Enter a natural number: 3
The sum of first 3 natural numbers is: 6
Explanation:
In the sample C code-
- We declare three integer variables inside the main() function. Here, variable n is used to store the count of natural numbers, variable i serves as a counter for the loop, and variable sum is used to store the sum of natural numbers.
- Next, prompt the user to enter a natural number and store it in variable n, using printf() and scanf() functions.
- We initialize the loop counter i to 1 and initiate a do-while loop.
- The loop will execute at least once, unlike a while loop, which may not execute at all if the condition is initially false.
- Inside the loop, we add the value of i to the sum variable and then increment i.
- The loop continues to execute as long as the condition i <= n is true, which means it iterates from 1 to n.
- Once the loop terminates, we print the result using printf() to display the sum of the first n natural numbers to the console.
- Finally, the main() function returns 0 to indicate successful completion of the program.
Time Complexity: O(n)
Space Complexity: O(1)
Find The Sum Of N Natural Numbers In C Using Direct Mathematical Formula
The sum of n natural numbers can be calculated by directly using the mathematical formula as derived from A.P (Arithmetic Progression) at the beginning of the article. The formula for the same is as follows:
Sn = (n/2)*[2*a + (n-1)*d] = (n)(n+1)/2;
Where Sn denotes the sum of the A.P/ first n natural numbers, n is the number of elements in the A.P., a is the starting point of the A.P., and d is the common difference of the A.P. (here d = 1 for natural numbers)
Code Example:
#include <stdio.h>
int main(){
int n;
printf("Enter a natural number: ");
scanf("%d",&n);
int s;
s=(n*(n+1))/2;
printf("Sum = %d", s);
return 0;
}
Output:
Enter a natural number: 9
Sum = 45
Explanation:
In the C example-
- We declare integer variable n to store the count of numbers for sum inside the main() function.
- Then, using printf(), we prompt the user to input a value for n and store it in the respective variable using scanf().
- Next, we declare an integer variable s, without initialization, to store the sum of N natural numbers.
- To calculate the sum of the first n natural numbers, we use the formula (n * (n + 1)) / 2, which uses multiple arithmetic operators.
- This formula is derived from the arithmetic series sum formula. And the outcome of the operation is stored in variable s.
- After that, we display the sum of n natural numbers, i.e., the value of s, to the console using the printf() function.
- Lastly, the main() function terminates with a return 0 statement.
Time Complexity: O(1)
Space Complexity: O(1)
Check out this amazing course to become the best version of the C programmer you can be.
Find The Sum Of N Natural Numbers In C Using Function
Another way to find the sum of N natural numbers in C code is by defining a function. The idea is for the user-defined function to take a value N as a parameter and calculate the sum of the first N natural numbers. The internal calculation can be done using loops or the formula as discussed in the sections above.
The benefit of using this approach, as with functions, is that we can call the function for varied values without having to write the code repeatedly. Let's look at the C program sample given below to better understand this approach to finding the sum of N natural numbers.
Code Example:
#include <stdio.h>
int findSum(int n){
int sum = 0;
for(int i = 1; i <= n; i ++){
sum += i;}
return sum;
}
int main(){
int n, res;
printf("Enter an integer value for count of natural numbers: ");
scanf("%d", &n);
res = findSum(n);
printf("Sum of first %d natural numbers is: %d", n, res);
return 0;
}
Output:
Enter an integer value for count of natural numbers: 10
Sum of first 10 natural numbers is: 55
Explanation:
In the C code sample-
- We define a function called findSum(), which takes an integer variable n as a parameter and calculates the sum of the first n natural numbers. Inside the function-
- First, we initialize an integer variable sum to 0 to store the result.
- After that, we initiate a for loop to iterate from 1 to n, adding each value of i to sum during every iteration.
- After that, we increment the value of i by 1 and move to the next iteration. This continues till condition i<=n becomes false, and the loop terminates.
- Once the loop terminates, the variable sum holds the sum of n natural numbers, which is returned by the function.
- Then, inside the main() function, we declare two variables: n to store user input and res to store the sum of the n natural numbers.
- Next, we take user input for the value n and call the findSum() function with n as an argument.
- The outcome of the function is stored in the variable res, which we then print to the console using the printf() function.
Time Complexity: O(n)
Space Complexity: O(1)
Find The Sum Of N Natural Numbers In C Between A Given Range
We can find out the sum of natural numbers in a given range, i.e. n1 to n2 (n2>n1), by finding the difference between the sum of the first n2 natural numbers and the sum of the first n1-1 natural numbers. The formula for this calculation is:
n1 + (n1+1) + (n1+2) + _ _ _ _ _ n2 = (1+2+3+4+5+_ _ +n2) - (1+2+3+ _ _ _ +(n1-1))
That is, the sum of natural numbers in the given range = (sum of first n2 numbers)-(sum of first n1-1 numbers). Where n1 is the starting number of the range and n2 is the ending number of the range. Look at the example below to understand how to implement this approach to find the sum of N natural numbers in a C program.
Code Example:
#include <stdio.h>
int Sum(int n){
int s = 0;
for(int i = 1; i <= n; i ++){
s += i;
}
return s;
}
int main(){
int n1, n2, sum1 = 0, sum2 = 0;
printf("Enter the starting and ending number of the range: ");
scanf("%d %d", &n1,&n2);
sum1 = Sum(n1-1);
sum2 = Sum(n2);
printf("Sum of numbers in the given range is: %d", sum2-sum1);
return 0;
}
Output:
Enter the starting and ending number of the range: 6 9
Sum of numbers in the given range is: 30
Explanation:
In the C code-
- We define a function Sum(int n) that calculates the sum of the first n natural numbers.
- Inside the function, we initialize a variable s to 0 to store the sum of n natural numbers within the range.
- Next, we initiate a for loop to iterate from 1 to n, adding the value of i to s in every iteration and incrementing the value of i after that.
- Once the loop terminates, the value stored in variable s is the sum of those N natural numbers, which the function returns.
- Then, inside the main() function, we declare four variables, i.e., n1 and n2, to store user input for the range and sum1 and sum2, initialized to 0, meant to store the sums of numbers.
- Following this, we prompt the user to enter the starting and ending numbers of the range and store them in variables n1 and n2 (respectively) using scanf().
- We then call the Sum() function with argument n1-1 to calculate the sum of numbers from 1 to n1-1. The outcome is stored in the sim1 variable.
- Similarly, we calculate the sum of numbers from 1 to n2 and store it in sum2 by calling the Sum() function with the argument n2.
- Next, we subtract sum1 from sum2 to find the sum of numbers in the given range and print the result using the printf() function.
- Lastly, the main() function terminates with a return of 0.
Time Complexity: O(n2)
Space Complexity: O(1)
Find The Sum Of N Natural Numbers In C Using Recursion
We can also find the sum of n natural numbers in C using recursion. Recursion is a method of solving a problem by breaking it down into smaller problems of the same kind. The solution to the original problem is then obtained by combining the solutions to the smaller problems.
Here, the idea behind the approach is that we can find out the sum of n numbers by using the sum of n-1 numbers and adding that to n and similarly for the rest.
Code Example:
#include <stdio.h>
int sum(int n){
if(n == 0) return n;
else{
return n + sum(n-1);
}
}
int main(){
int n, res = 0;
printf("Enter the value of n: ");
scanf("%d", &n);
res = sum(n);
printf("Sum of first %d natural numbers is: %d", n, res);
return 0;
}
Output:
Enter the value of n: 5
Sum of first 5 natural numbers is: 15
Explanation:
In the example code-
- We define a recursive function sum(int n) that calculates the sum of the first n natural numbers. Inside this function-
- We use an if-else statement to define a base case, i.e., if n is 0, we return 0 because the sum of the first 0 natural numbers is 0.
- Else, if n is not 0, we recursively call the sum() function with n-1 and add n to the result. This process continues until the base case is reached.
- In the main() function, we declare two variables, i.e., integer n to store user input and integer res to store the result of the summation.
- We then take user input for the value of n and store it using printf() and scanf() functions.
- After that, we call the sum() function with n as an argument and store the result in the variable res.
- Once the function returns the sum of n natural numbers stored in res, we print the value using a printf() statement.
Time Complexity: O(n)
Space Complexity: O(n)
Find The Sum Of N Natural Numbers In C Using Array
We can also use an array to store the sum of n natural numbers in C. An array is a data structure that stores a collection of elements of the same data type in contiguous memory locations which makes it easy to access the elements using their indexes.
- To do this, you must first make an array of size n+1 and store i at arr[i] from i=1 to i=n.
- Then, traverse the array and add arr[i] to the sum while iterating from i=1 to n.
- This approach requires an extra auxiliary array of size n+1 to compute the sum of first n natural numbers as compared to the methods using loops.
Look at the C program example below to see how we use arrays to find the sum of n natural numbers.
Code Example:
#include <stdio.h>
int main(){
int n, sum = 0;
printf("Enter the value of n: ");
scanf("%d", &n);
int arr[n+1];
for(int i = 1; i <= n; i ++){
arr[i] = i;
}
for(int i = 1; i <= n; i ++){
sum += arr[i];
}
printf("Sum of first %d natural numbers is: %d", n, sum);
return 0;
}
Output:
Enter the value of n: 9
Sum of first 9 natural numbers is: 45
Explanation:
In the code above-
- Inside the main() function, we declare two variables, i.e., int n to store user input for a count of numbers whose sum we will calculate and int sum to store the sum which is initially set to 0.
- Next, we get the value for variable n from the user with the printf() and scanf() functions.
- Then, we declare an array arr with a size of n+1. This array will store the first n natural numbers.
- We then enter a for loop to populate the arr array with natural numbers. The loop iterates from 1 to n, assigning each index i of the array arr with the value i.
- After populating the array, we enter another for loop to calculate the sum of the first n natural numbers.
- Inside this loop, we accumulate the sum by accessing each element of the array arr and adding it to the sum variable.
- Once the sum is calculated, we print the result using the printf() function.
Time Complexity: O(n)
Space Complexity: O(n)
Find The Sum Of N Natural Numbers In C With User Input Until A Positive Integer Is Entered
As we know, natural numbers are positive integers, i.e. greater than 0. So when we want to find the sum of the first n natural numbers, we must ensure that the input must be a positive integer, or the program could throw errors. For this, we can insert a check to keep using users to provide values until they enter a valid natural number. This check can be fused with all other approaches to calculate the sum of N natural numbers as we have discussed above.
In the C example program below, we will use a while loop with an if statement to insert this check. And we will calculate the sum of N natural numbers using a for loop, as discussed in the section above.
Code Example:
#include <stdio.h>
int main(){
int n, sum = 0;
while(1){
printf("Enter the value of n: ");
scanf("%d", &n);
if(n>0) break;
}
for (int i = 1; i <= n; i++) {
sum += i;
}
printf("The sum of first %d natural numbers is: %d", n, sum);
return 0;
}
Output:
Enter the value of n: -3
Enter the value of n: -10
Enter the value of n: 7
The sum of first 7 natural numbers is: 28
Explanation:
In the code-
- Inside the main() function, we declare an integer variable n to store the count of natural numbers from user input. And another variable sum to store the sum of those natural numbers initialized to 0.
- We then define an infinite while loop with the condition while(1), meaning it will loop indefinitely unless a break statement is encountered.
- Inside the loop, we use printf() to prompt the user to enter the value for n and read it using scanf().
- Then, an if-statement checks if n is greater than 0. If the condition is true, then the break statement is encountered, and the loop terminates.
- If the condition is false, i.e., n<0, then the loop continues prompting the user to enter a number until a positive value is entered.
- Once a positive value for n is obtained, the program exits the while loop and moves to the next line.
- Then, we initiate a for loop with the loop variable i starting from 1 and incrementing up to n.
- Inside the for loop, we increment the variable sum by the value of i in each iteration. This calculates the sum of the first n natural numbers.
- After the for loop completes execution, we print the sum of the first n natural numbers using the printf() statement.
- Finally, the main() function returns 0 to indicate successful completion of the program.
- As shown in the output, the program keeps asking the user to input a new value until they enter a positive value.
Time Complexity: O(n)
Space Complexity: O(1)
Time & Space Complexity Comparison For Programs To Find Sum Of N Natural Numbers In C
We have compared the time and space complexity of the various approaches to finding the sum of first n natural numbers in C, which we have discussed in the sections above.
Name of the method |
Time Complexity |
Space Complexity |
Using For Loop |
O(N) |
O(1) |
Using While Loop |
O(N) |
O(1) |
Using Do While Loop |
O(N) |
O(1) |
Using Direct Formula |
O(1) |
O(1) |
Using Function |
O(N) |
O(1) |
Sum of Given Range(N1-N2) |
O(N2) |
O(1) |
Using Recursion |
O(N) |
O(N) |
Using Array |
O(N) |
O(N) |
Until a positive integer is entered |
O(N) |
O(1) |
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
We can calculate the sum of n natural numbers in C using various approaches, including for loop, while loop, do-while loop, function, recursion, array, and mathematical formula directly. The sum of n natural numbers is used in various mathematical calculations, and understanding how to make this calculation using a C program can have wide applications. We also compared the time complexity and space complexity of each of the methods to get an idea of how each approach to calculating the um of N natural numbers in C fairs when pitted against each other.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is the mathematical formula for finding the sum of first n natural numbers?
The mathematical formula for finding the sum of the first n natural numbers is:
S = n(n + 1)/2 where S is the sum and n is the number of natural numbers.
Alternatively, we can find the sum of n natural numbers using the Arithmetic Progression (AP). The sum of an A.P can be found using the below formula:
Sn = (n/2)*[2*a + (n-1)*d] = (n)(n+1)/2;
Where Sn denotes the sum of the A.P. with n denoting the number of elements in it. Also, the term a is the starting point of the A.P., and d is the common difference between two consecutive elements, which will be 1 for natural numbers.
Q. What is the sum of the first 20 natural numbers?
The mathematical formula for finding the sum of the first n natural numbers is S = n(n + 1)/2, where S is the sum and n is the number of natural numbers.
If you want to find the sum of the first 20 natural numbers, then n=20, which, applied in the formula, gives 210-
S = (20)(20+1)/2 = 10*21 = 210
Q. What is += in C?
The += operator in C programming is a compound/ augmented assignment operator which performs the addition of the right operand to the left operand and assigns the result to the left operand. For example-
int x = 10;
x += 5;
This block of code will first add 5 to the value of x and then assign the result (15) back to x. This is equivalent to the expression: x = x + 5. The equality operator can be used with any of the arithmetic operators, such as +, -, *, /, and %, to make a compound assignment operator.
Q. How to find the sum of n natural numbers using recursion in C?
We can find out the sum of n natural numbers in C using recursion. The idea behind the approach is that we use the sum of n-1 numbers and add n to find the sum of n natural numbers. And similarly, for rest until we reach the base case, i.e. n=0 will return 0.
Intuition/ Logic Behind Using Recursion To Find Sum of N Natural Numbers In C:
Suppose n = 6, then-
Sum of first 6 numbers = 6 + sum of first 5 numbers
Sum of first 5 numbers = 5 + sum of first 4 numbers
Similarly,
Sum of first 4 numbers = 4 + sum of first 3 numbers
Sum of first 3 numbers = 3 + sum of first 2 numbers
Sum of first 2 numbers = 2 + sum of first 1 numbers
Sum of first 1 numbers = 1 + 0
Below is a code example that showcases the implementation of this logic.
Code Example:
#include <stdio.h>
int rec_sum(int n){
if(n == 0) return n;
else{
return n + rec_sum(n-1);}
}
int main(){
int n, sum = 0;
printf("Enter the value of n: ");
scanf("%d", &n);
sum = rec_sum(n);
printf("Sum of first %d natural numbers is: %d", n, sum);
return 0;
}
Input/ Output:
Enter the value of n: 21
Sum of first 21 natural numbers is: 231
Explanation:
In this example-
- We first define a recursive function rec_sum() where the base case checks if the value of n is 0. If so, then the function returns zero.
- If not, then the function calls the rec_sum() function within itself with n-1 as an argument to calculate the sum of n-1 natural numbers and adds the value of n to it, which it returns.
- In the main() function, we intilize integer n to store the count of natural numbers without initialization and variable sum to store the result of recursion initialized to 0.
- We then take user input for n and call the rec_sum() function with that as an argument. The function uses a recursive approach to find the sum of the n numbers.
- The function returns the value of the final sum and stores it in sum, which we print to the console using the printf() function.
Q. What is the best method to calculate the sum of n natural numbers in C?
The best method to find the sum of n natural numbers in C is by using the mathematical formula directly in the code, i.e., n*(n+1)/2. This is because the time and space complexity are constant compared to loops where the time complexity is O(n), and for recursion, the time complexity is O(n), and space complexity is O(n).
Q. What would be the time and space complexities when finding the sum of n natural numbers in C programs using loops and recursion?
Complexities when calculating the sum of n natural numbers in C using loops:
Time Complexity: O(n)
Space complexity: O(1)
Complexities when calculating the sum of n natural numbers in C using recursion:
Time Complexity: O(n)
Space complexity: O(n) (due to the function call stack overhead)
Here are a few other interesting articles you must read to expand your knowledge pool on C topics:
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
- 6 Relational Operators In C & Precedence Explained (+Examples)
- Compilation In C | Detail Explanation Using Diagrams & Examples
- Logical Operators In C (AND, OR, NOT, XOR) With Code Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment