Table of content:
- What Are Operators In C Program?
- Types Of Operators In C
- Arithmetic Operators In C
- Assignment Operators In C
- Increment And Decrement Operators In C
- Relational Operators In C
- Logical Operators In C
- Bitwise Operators In C
- Ternary Or Conditional Operators In C
- Shift Operators In C
- Special Operators In C
- The Operators Precedence In C
- Conclusion
- Frequently Asked Questions
Operators In C Programming | All Types Explained (+Code Examples)
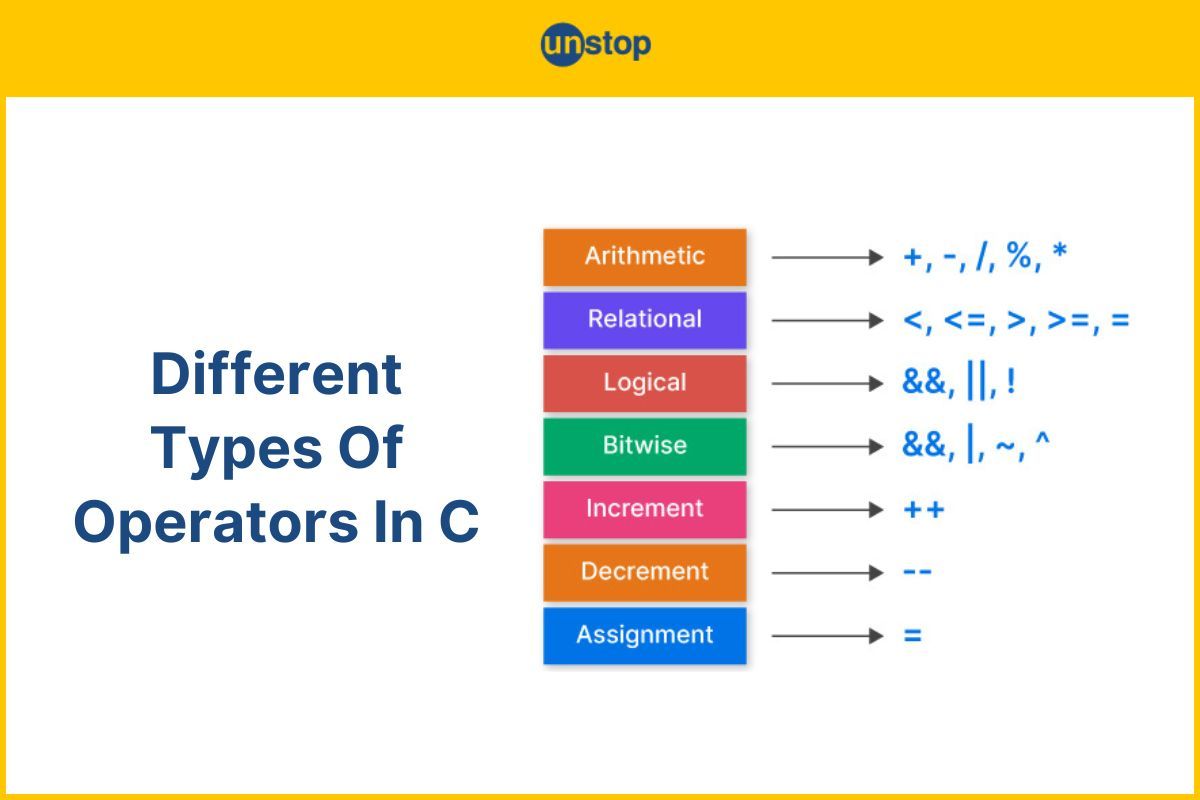
Operators are the symbols in programming that help perform operations on data/values stored in variables. They act as the building blocks of programming languages, enabling users to perform specific logical and mathematical operations on operands (data).
In this article, we will discuss what operators in C programming language are and all its types, with the help of examples.
What Are Operators In C Program?
In simple words, operators are a collection of symbols used to give commands to a compiler to implement unique mathematical or logical operations. The built-in operators can be classified into two types based on the number of operands they take:
- Unary Operators- As the name suggests, unary operators act on or carry out operations on a single operand only. For example, the increment and decrement operators.
- Binary Operators- These are the operators that work on two numeric operands/ expressions. Examples of binary operators include arithmetic operators (addition, subtraction, multiplication, etc.), relational operators, etc.
Types Of Operators In C
The table below lists the different types of operators in C language, classified based on the operations they perform on numeric operands.
Arithmetic Operators |
Assignment Operators |
Relational Operators |
Special Operators |
Logical Operators |
Shift Operators |
Bitwise Operators |
Miscellaneous Operators |
Ternary or Conditional Operators |
|
Each of these types comprises a range of operators, as we will see in the individual sections below.
Arithmetic Operators In C
These are binary operators used to perform mathematical/ arithmetic operations on numeric operands. Listed in the table below are the different types of arithmetic operators in C, along with a description and example.
Operator |
Description |
Example |
+ |
This is the additive operator that calculates the sum of the two operands. |
C + D = 30 |
− |
It subtracts the value of the right-hand operand from the left side operand. |
C − D = -10 |
* |
This operator multiplies the two operands. |
C * D = 200 |
/ |
Divides the operand in the numerator by the operand in the denominator. |
D / C= 2 |
% |
The modulus operator gives out the remainder for an integer division. |
D % C = 0 |
++ |
The increment operator increases the integer value by one. |
C++ = 11 |
-- |
The decrement operator decreases the integer value by one. |
C-- = 9 |
Below is a simple C program example illustrating the use of the arithmetic operators.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IGExID0gMTA7CmludCBiMSA9IDEwOwoKCmludCBjMSA9IGExICsgYjE7CnByaW50ZigiQWRkaXRpb246ICVkXG4iLCBjMSk7CgpjMSA9IGExIC0gYjE7CnByaW50ZigiU3VidHJhY3Rpb246ICVkXG4iLCBjMSk7CgpjMSA9IGExICogYjE7CnByaW50ZigiTXVsdGlwbGljYXRpb246ICVkXG4iLCBjMSk7CgpjMSA9IGExICUgYjE7CnByaW50ZigiTW9kdWx1czogJWRcbiIsIGMxKTsKCmZsb2F0IGQxID0gKGZsb2F0KWExIC8gKGZsb2F0KWIxOwpwcmludGYoIkRpdmlzaW9uOiAlLjJmXG4iLCBkMSk7CgpyZXR1cm4gMDsKfQ==
Output:
Addition: 20
Subtraction: 0
Multiplication: 100
Division: 1.00
Modulus: 0
Explanation:
In the simple C code example, we first include the essential header file <stdio.h> for input/ output operations.
- Inside the main() function, we initialize two integer variables, a1 and b1, both with a value of 10.
- Next, we perform a series of arithmetic operations on the two variables as operands.
- First, we add a1 and b1 to obtain the sum c1 using the addition operator (+) and then print it to the console using the printf() statements with formatted string.
- Here, the %d format specifier is the placeholder for an integer value, and the newline escape sequence (\n) shifts the cursor to the next line after printing.
- Then, we subtract b1 from a1, using the subtraction arithmetic operator (-) and print the same to the console.
- Similarly, we calculate the product and the modulus (remainder of division operation) of a1 and b1 using the multiplication (*) and modulo operators (%), respectively.
- We then use the division operator (/) to divide a1 by b1. Note that here, we cast a1 and b1 to float to ensure the division yields a floating-point result rather than an integer. The printf() statement displays output with two decimal places.
- Finally, the main() function returns 0, indicating successful execution to the operating system.
For more, read: Arithmetic Operators In C | Types & Precedence Explained (+Examples)
Assignment Operators In C
Assignment operators are used to assign a value to variables. When used in an expression, the assignment operator leads the left operand to receive the value on the right side. There are six types of assignment operators in C.
- The common assignment operator, represented by the equal to sign (=), is the most frequently used of these.
- Besides this, we have five compound assignment operators that perform an arithmetic operation and then assign the revised value to the left-side operand.
- For instance, you can add a value (right-hand operand) to an existing variable (left operand) by using the (+=) operator and assign the value back to the left operand, in a single expression.
Listed in the table below are the assignment operators, along with an example and an elaborate version of the expression.
Operator |
Example Expression |
Elaborate Expression |
= |
c = d |
c = d |
+= |
c += d |
c = c+d |
-= |
c -= d |
c = c-d |
*= |
c *= d |
c = c*d |
/= |
c /= d |
c = c/d |
%= |
c %= d |
a = a%b |
The C example program below illustrates the use of all six assignment operators.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHggPSAxMDsgLy8gVXNpbmcgdGhlIGNvbW1vbiBhc3NpZ25tZW50IG9wZXJhdG9yCmludCB5ID0gNTsgLy8gVXNpbmcgdGhlIHNpbXBsZSBvciBiYXNpYyBhc3NpZ25tZW50IG9wZXJhdG9yCgp4ICs9IHk7IC8vIC8vIFVzaW5nIHRoZSBhZGRpdGlvbiBjb21wb3VuZCBhc3NpZ25tZW50IG9wZXJhdG9yCnByaW50ZigieCA9ICVkXG4iLCB4KTsKCnggLT0geTsgLy8gVXNpbmcgdGhlIHN1YnRyYWN0aW9uIGNvbXBvdW5kIGFzc2lnbm1lbnQgb3BlcmF0b3IKcHJpbnRmKCJ4ID0gJWRcbiIsIHgpOwoKeCAqPSB5OyAvLyBVc2luZyB0aGUgbXVsdGlwbGljYXRpb24gY29tcG91bmQgYXNzaWdubWVudCBvcGVyYXRvcgpwcmludGYoInggPSAlZFxuIiwgeCk7Cgp4IC89IHk7IC8vIFVzaW5nIHRoZSBkaXZpc2lvbiBjb21wb3VuZCBhc3NpZ25tZW50IG9wZXJhdG9yCnByaW50ZigieCA9ICVkXG4iLCB4KTsKCnggJT0geTsgLy8gVXNpbmcgdGhlIG1vZHVsbyBjb21wb3VuZCBhc3NpZ25tZW50IG9wZXJhdG9yCnByaW50ZigieCA9ICVkXG4iLCB4KTsKCnJldHVybiAwOwp9
Output:
x = 15
x = 10
x = 50
x = 10
Explanation:
In the C example code,
- We declare two integer variables, x and y, and initialize them with values 10 and 5, respectively, using the simple assignment operator (=).
- Then, as the , we use various compound assignment operators (+=, -=, *=, /=, and %=) to perform arithmetic operations between left and right operands (x and y) and then assign the value back to the left-hand operand (x):
- The addition assignment operator (x += y) adds y to x and then assigns the value to x, i.e., 15.
- The subtraction assignment operator (x -= y) subtracts y from x and sets x back to 10.
- The multiplication assignment operator (x*=y) multiplies x by y and then updates x to 50.
- The division assignment operator (x /= y) divides x by y and then sets x back to 10.
- The assignment modulo operator (x %= y) finds the remainder of x divided by y, resulting in x = 0.
- We print the outcome of the operations side by side, using the printf() statements.
For more, read: Assignment Operators In C | A Complete Guide With Detailed Examples
Increment And Decrement Operators In C
The increment and decrement operators in C are unary operators that increase and decrease the value of an operand by 1, respectively. The decrement and increment operators can be divided into two groups as follows:
- Prefix- The prefix operator is applied/ placed before the variable. When a prefix increment operator is applied (e.g., ++a), the value of the operand is increased by 1 before it is assigned or used in an expression. Conversely, when a prefix decrement operator is applied (e.g., --a), the operand is decreased by 1 before being assigned or used.
- Postfix- The postfix operator is applied/ placed after the variable. When a postfix increment operator is applied (e.g., a++), the current value of the operand is used in the expression first, and then the operand is increased by 1 afterwards. In the case of a postfix decrement operator (e.g., a--), the current value is used first, and then the operand is decreased by 1.
Have a look at the table below for a better understanding of the same:
Type of Operator |
Sample Operator Expression |
Description/ Explanation |
Prefix Increment Operator |
++q |
Here, the (++) first increases the initial value of q by 1, after which it is used in the code. |
Postfix Increment Operator |
q++ |
Here, the initial value of q is used in the code first, and then it is increased by 1. |
Prefix Decrement Operator |
–q |
Here, the value of q is first decreased by 1 first, and then it is used in the program/ code. |
Postfix Decrement Operator |
q– |
Here, the value of q would be used as is in the program first and then decrease the value of q by 1. |
Look at the basic C program example that illustrates the use of the increment and decrement operators.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IGEgPSA1OwppbnQgYiA9IDI7CmludCBjOwoKYyA9ICsrYTsKcHJpbnRmKCJjID0gJWQsIGEgPSAlZFxuIiwgYywgYSk7CgpjID0gLS1iOwpwcmludGYoImMgPSAlZCwgYiA9ICVkXG4iLCBjLCBiKTsKCmMgPSBhKys7CnByaW50ZigiYyA9ICVkLCBhID0gJWRcbiIsIGMsIGEpOwoKYyA9IGItLTsKcHJpbnRmKCJjID0gJWQsIGIgPSAlZFxuIiwgYywgYik7CgpyZXR1cm4gMDsKfQ==
Output:
c = 6, a = 6
c = 1, b = 1
c = 6, a = 7
c = 1, b = 0
Explanation:
In the basic C code example, we declare three integer variables a, b, and c, and initialize the first two with values 5 and 2, respectively.
- Then, we use the pre-increment operator (++a), assign that value to variable c and print the values of both a and c afterwards. As seen in the output, both a ad c are 6, meaning the value of a was increased by 1 before being assigned to c.
- We then apply the pre-decrement operator to b (--b), assign the value to c, and print both. As seen in the output, the value of both c and b is 1, meaning b's value was decreased by 1 before being assigned to c.
- Next, we apply the post-increment operator to a (a++), assign that to c and print both the values. As seen in the output, the value of c is 6, and the value of a is 7. This means that a's current value (6) was first assigned to c, and then it was increased by 1 (raising a to 7).
- Lastly, the post-decrement operator b--, first, the value of 'b' is assigned to 'c' and is then decreased by 1. So the post-decrement operator b-- reduces b's value by 1. Consequently, 'c' changes to 1 and 'b' to 0.
For more, read: Increment And Decrement Operators In C With Precedence (+Examples)
Relational Operators In C
The main purpose of relational operators in C is to conduct a value comparison of two operands. For instance, they can be used to determine if an operand is greater than the other, or to determine if one operand is equal to the other, and other such similar cases. In the truth table below, we have listed the different types of relational operators with examples.
Operator |
Operator Name |
Example |
Result |
== |
Equal to |
6 == 5 is evaluated to 0 |
False |
> |
Greater than |
6 > 5 is evaluated to 1 |
True |
< |
Less than |
6 < 5 is evaluated to 0 |
False |
!= |
Not equal to |
6!= 5 is evaluated to 1 |
True |
>= |
Greater than or equal to |
6 >= 5 is evaluated to 1 |
True |
<= |
Less than or equal to |
6 <= 5 is evaluated to 0 |
False |
The C program example below illustrates the use of all six relational operators.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHggPSA0OwppbnQgeSA9IDI7CgppZiAoeCA+IHkpIHsKcHJpbnRmKCJ4IGlzIGdyZWF0ZXIgdGhhbiB5XG4iKTsKfQoKaWYgKHggPj0geSkgewpwcmludGYoInggaXMgZ3JlYXRlciB0aGFuIG9yIGVxdWFsIHRvIHlcbiIpOwp9CgppZiAoeCA8IHkpIHsKcHJpbnRmKCJ4IGlzIGxlc3MgdGhhbiB5XG4iKTsKfQoKaWYgKHggPD0geSkgewpwcmludGYoInggaXMgbGVzcyB0aGFuIG9yIGVxdWFsIHRvIHlcbiIpOwp9CgppZiAoeCA9PSB5KSB7CnByaW50ZigieCBpcyBlcXVhbCB0byB5XG4iKTsKfQoKaWYgKHggIT0geSkgewpwcmludGYoInggaXMgbm90IGVxdWFsIHRvIHlcbiIpOwp9CgpyZXR1cm4gMDsKfQ==
Output:
x is greater than y
x is greater than or equal to y
x is not equal to y
Explanation:
In the C code example, we first declare two integer variables, x and y and assign them with values 4 and 2, respectively. Next, we use a set of if-statements along with relational operators to perform comparison operations of these values.
- First, we check if the value of x is greater than y (i.e., x>y) using the greater than operator. Since x = 4 and y = 2, the statement condition is true, and if-block executes, printing the string message– "x is greater than y" to the console.
- Then, we perform the comparison operation to check if the value of x is greater than or equal to y (i.e., x>=y), using greater than equal to operator. Since x (4) is greater than y (2), the statement condition is true; the if-block is executed, printing the respective message.
- We then check if the value of x is less than y (x<y), using the less than operator. Since the x (4) is not less than y (2), the statement condition is false, and the flow skips the if-block, moving to the next line in the code.
- Next, we check if the value of x is less than or equal to y (x<=y), using the less than equal to operator. Since x (4) is greater than y (2), the statement condition is false; we skip the if-block, and no message is printed.
- After that, we use the equality operator to check if the value of x is equal to y (x==y). Since x (4) and y (2) are not equal, the statement condition is false, and we skip the respective if-block.
- Lastly, we perform the not equal-to comparison operation to check if x is not equal to y (x!=y). Since x (4) and y (2) are not equal, the condition is true, and we execute the if-block, printing the respective message.
For more, read: 6 Relational Operators In C & Precedence Explained (+Examples)
Logical Operators In C
The logical operators are used to combine two or more conditions, constraints, or expressions. The combined expression may be referred to as a logical expression.
- The result of logical operations is always a boolean value, i.e., true (1) or false (0).
- Let's take, for example, a logical/ conditional expression containing the logical AND operator. Here, the entire expression returns true only if both the conditions/ expression results true and false if the expression on either side is false.
There are three types of logical operators in C. We have listed these logical operators in the table below, with a short description and example.
Operator |
Name/ Description |
Example |
Result |
&& |
Logical AND operator |
If c= 6 and d = 5 then, expression ((c==5) && (d>5)) equals to 0. |
False |
|| |
Logical OR operator |
If c = 6 and d = 5 then, expression ((c==6) || (d>5)) equals 1. |
True |
! |
Logical NOT operator |
If c = 6 then, expression !(c==6) equals to 0. |
False |
The example C program below illustrates the implementation of the logical operators.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHggPSA1OwppbnQgeSA9IDI7CmludCB6ID0gMDsKCmlmICh4ID4geSAmJiB4ID4geikgewpwcmludGYoInggaXMgdGhlIGxhcmdlc3QgbnVtYmVyXG4iKTsKfQoKaWYgKHkgPCB4IHx8IHogPCB4KSB7CnByaW50ZigieCBpcyBub3QgdGhlIHNtYWxsZXN0IG51bWJlclxuIik7Cn0KCmlmICghKHggPT0geSkpIHsKcHJpbnRmKCJ4IGlzIG5vdCBlcXVhbCB0byB5XG4iKTsKfQoKcmV0dXJuIDA7Cn0=
Output:
x is the largest number
x is not the smallest number
x is not equal to y
Explanation:
In the example C code, we declare three integer variables x, y and z and assign them with values 5, 2 and 0 respectively. Next, we use a set of if statements to perform logical operations on the values.
- First, we check if the value of x is greater than both y AND z (i.e., x>y && x>z), using the logical AND operator. Since x is 5, which is greater than both y and z, the logical conditional expression evaluates to true, and the if-block is executed, printing the message– "x is the largest number".
- Second, we check if the value of y is less than x OR if z is less than x (i.e., y<z || z<x) using the logical OR operator. Since x is 5, which is greater than both y and z, the entire expression evaluates to true, and the if-block prints the message "x is not the smallest number".
- Last, we check if the value of x is NOT equal to y (i.e., !(x==y)) using the logical NOT operator. Since x (5) is not equal to y (2), the entire expression evaluates to true, and the if-block prints the message- "x is not equal to y".
For more, read: Logical Operators In C (AND, OR, NOT, XOR) With Code Examples
Bitwise Operators In C
Bitwise operators, as the name suggests, are used to carry out bit-level operations on the operands. In other words, these operators operate on individual bits of the operands.
- In this, the operands are first translated to bit-level prior to performing the operation on them.
- The advantage of carrying out operations like addition, subtraction, multiplication, etc., at the bit level is that it makes for quicker processing times.
- For example, the bitwise AND operator takes two consecutive integers as operands and executes AND on each bit of the two numbers. It returns 1 (i.e., True) only when both the bits are set to 1 (True).
- The bitwise operators are often used in low-level programming for performance optimization, such as in system-level programming or embedded systems.
There are six bitwise operators in C, as listed in the table below.
Operators |
Meaning of operators |
& |
Bitwise AND |
| |
Bitwise OR |
^ |
Bitwise exclusive OR |
~ |
Bitwise complement |
<< |
Shift left |
>> |
Shift right |
The sample C program below showcases the use of these operators.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IGExID0gNTsKaW50IGIxID0gNjsKCmludCBhbnNfYW5kID0gYTEgJiBiMTsKcHJpbnRmKCJSZXN1bHQgb2YgYTEgJiBiMSBpcyAlZFxuIiwgYW5zX2FuZCk7CgppbnQgYW5zX29yID0gYTEgfCBiMTsKcHJpbnRmKCJSZXN1bHQgb2YgYTEgfCBiMSBpcyAlZFxuIiwgYW5zX29yKTsKCmludCBhbnNfeG9yID0gYTEgXiBiMTsKcHJpbnRmKCJSZXN1bHQgb2YgYTEgXiBiMSBpcyAlZFxuIiwgYW5zX3hvcik7CgppbnQgYW5zX3NoaWZ0X2xlZnQgPSBhMSA8PCAxOwpwcmludGYoIlJlc3VsdCBvZiBhMSA8PCAxIGlzICVkXG4iLCBhbnNfc2hpZnRfbGVmdCk7CgppbnQgYW5zX3NoaWZ0X3JpZ2h0ID0gYjEgPj4gMTsKcHJpbnRmKCJSZXN1bHQgb2YgYjEgPj4gMSBpcyAlZFxuIiwgYW5zX3NoaWZ0X3JpZ2h0KTsKCmludCBhbnNfbm90ID0gfmExOwpwcmludGYoIlJlc3VsdCBvZiB+YTEgaXMgJWRcbiIsIGFuc19ub3QpOwoKcmV0dXJuIDA7Cn0=
Output:
Result of a1 & b1 is 4
Result of a1 | b1 is 7
Result of a1 ^ b1 is 3
Result of a1 << 1 is 10
Result of b1 >> 1 is 3
Result of ~a1 is -6
Explanation:
In the sample C code, we initialize two integer variables, a1 and b1, with values 5 and 6, respectively. Next, we perform bitwise operations on them:
- First, we perform the bitwise AND operation (&) between a1 and b1 and assign the outcome to variable ans_and. This operation returns 1 for each bit position where both a1 and b1 have a 1.
- The outcome of this operation is 0100 (binary) and 4 (decimal representation), which we print to the console using a printf() statement.
- Second, we perform the bitwise OR operation (|) between a1 and b1, and assign the value to variable ans_or. This operation returns 1 for each bit position where either a1 or b1 has a 1. The result is 0111 (binary representation) and 7 (decimal representation).
- Then, we perform bitwise XOR operation (^) between a1 and b1, which returns 1 for each bit position where either a1 or b1 has a 1, but not both. The result is 0011 (binary representation) and 3 (decimal representation), stored in variable ans_xor.
- Next, we perform the bitwise left shift (<<) operation on a1, shifting each bit by 1, effectively multiplying it by 2. The result is 1010 (binary representation) and 10 (decimal representation) stored in variable ans_shift_left.
- After that, we perform the bitwise right shift (>>) operation on b1, shifting each bit by 1. The outcome is 0011 (binary representation), and 3 (decimal representation) is stored in ans_shift_right.
- Lastly, we perform the bitwise NOT operation (~) on a1 and store the result in variable ans_not. The outcome is -6, as the bitwise complement of 5 (Binary: 0101) is 1010, interpreted as -6 in two's complement representation.
For more, read: Bitwise Operators In C Programming Explained With Code Examples
Ternary Or Conditional Operators In C
The ternary operator (also called the conditional operator) is a shorthand way to evaluate a condition and return one of two values based on whether the condition is true or false. It’s called "ternary" because it requires three components: a condition to check, a value if the condition is true, and a value if it’s false. It is often used in place of an if-else statement.
Syntax
condition ? value_if_true : value_if_false
Here,
- The condition refers to the initial expression being evaluated, and the symbols (? and :) denote the ternary operator.
- The value_if_true and value_if_false, refer to the values to be returned if the condition evaluates to true or false, respectively.
The C program sample below demonstrates how this operator works in code.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHggPSA2OwppbnQgeSA9IDEyOwoKaW50IHJlc3VsdCA9ICh4ID4geSkgPyB4IDogeTsKcHJpbnRmKCJUaGUgbGFyZ2VyIHZhbHVlIGlzOiAlZFxuIiwgcmVzdWx0KTsKCnJldHVybiAwOwp9
Output:
The larger value is: 12
Explanation:
In the C code sample, we initialize integer type variables x and y with the values 6 and 12, respectively.
- Then, we use the ternary operator to determine the larger of the two values. The expression (x > y) ? x : y checks if x is greater than y.
-
- If this condition is true, it means x is greater than y, and the variable result is assigned the value of x.
- If the condition is false, it means y is greater than x, and the result is assigned the value of y.
- Since y (12) is greater than x (6), the result gets the value 12, which we print to the console.
For more, read: Ternary (Conditional) Operator In C Explained With Code Examples
Shift Operators In C
Shift operators in C manipulate the bits of a binary number, moving them left or right by a specified number of positions. These operators are commonly used for low-level programming tasks, such as bit manipulation, data compression, and certain arithmetic operations.
- Left Shift Operator (<<): Moves bits of the left operand to the left by the number of positions specified by the right operand. Empty positions on the right are filled with zeros. This operation effectively multiplies the original number by 2 raised to n, where n is the number of positions shifted.
- Right Shift Operator (>>): Moves bits of the left operand to the right by the number of positions specified by the right operand. The behavior of the empty positions on the left varies:
-
- For unsigned types, empty positions are filled with zeros (logical shift).
- For signed types, they may be filled with either zeros or the sign bit, depending on the implementation (arithmetic shift).
We have already covered these in the section on bitwise operators, which encompass all operators that perform bit-level manipulations.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Special Operators In C
In addition to the operators in C discussed above, the programming language offers several unique operators designed for specific memory-related tasks, including obtaining variable sizes, addresses, and dereferencing pointer variables. These are essential for tasks involving memory management, data type manipulation, and parameter passing.
Also sometimes referred to as miscellaneous operators, we have listed them in the table below with a brief description and example.
Operator |
Description |
Example |
sizeof() |
Returns the number of bytes used by a data type or variable (for example, int, char, float, double, etc.). |
sizeof(x), where x is an integer, will return 4. |
& |
Yields the memory address of a variable. |
&x returns the actual address of the variable x, which could be any valid memory address (e.g., 0x7ffeefbff5c8). |
* |
Dereferences a pointer variable to access or modify the value at the pointed-to address. |
*ptr, helps access the value stored at address ptr. |
In the following sections, we will discuss these special/ miscellaneous operators in a little more detail.
The Size-of Operator In C
This is a unary operator that returns the size (in bytes) of a data type or variable at compile time.
- The result of the sizeof() operator is of the unsigned integral type, typically represented by size_t.
- It’s commonly used to determine memory allocation requirements for data types or data structures.
For Example:
int x;
printf("Size of int: %zu bytes\n", sizeof(int)); // Output may vary by system
printf("Size of x: %zu bytes\n", sizeof(x));
For more, read: The Sizeof() Operator In C | A Detailed Explanation (+Examples)
The Address-of Operator In C
The address-of operator, represented by the ampersand sign (&), is used for two primary reasons:
- Parameter Passing by Reference: It allows us to call functions by passing variables through reference/ address.
- Establishing Pointer Values: The address-of operator gives the memory address of a variable. The value of a pointer is the memory address where the data item resides.
For Example:
int y = 10;
int *ptr = &y; // ptr now holds the address of y
printf("Address of y: %p\n", (void*)&y);
printf("Value at ptr: %d\n", *ptr); // Dereferencing pointer to get value
The Dereference(*) Operator
Also known as the asterisk operator (*), it is used to access the value at the memory address held by a pointer. It allows direct manipulation of variable values through pointers.
Look at the C program example below, which illustrates the use of all three special operators discussed.
Code example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKCmludCB4ID0gMTA7CgpwcmludGYoIlNpemUgb2YgaW50OiAlenUgYnl0ZXNcbiIsIHNpemVvZihpbnQpKTsgLy8gVXNpbmcgc2l6ZW9mIHRvIGdldCB0aGUgc2l6ZSBvZiBpbnQgdHlwZQpwcmludGYoIlNpemUgb2YgeDogJXp1IGJ5dGVzXG4iLCBzaXplb2YoeCkpOyAvLyBVc2luZyBzaXplb2YgdG8gZ2V0IHRoZSBzaXplIG9mIHggdmFyaWFibGUKCmludCAqcHRyID0gJng7IC8vIFVzaW5nIHRoZSBhZGRyZXNzLW9mIG9wZXJhdG9yICgmKSB0byBhc3NpZ24geCdzIGFkZHJlc3MgdG8gcHRyCgoKcHJpbnRmKCJBZGRyZXNzIG9mIHg6ICVwXG4iLCAodm9pZCopJngpOyAvLyBVc2luZyAmIHRvIGdldCB0aGUgbWVtb3J5IGFkZHJlc3Mgb2YgeApwcmludGYoIkFkZHJlc3Mgc3RvcmVkIGluIHB0cjogJXBcbiIsICh2b2lkKilwdHIpOyAvLyBQcmludGluZyB0aGUgYWRkcmVzcyBzdG9yZWQgaW4gcHRyCnByaW50ZigiVmFsdWUgYXQgcHRyICh2YWx1ZSBvZiB4KTogJWRcbiIsICpwdHIpOyAvLyBVc2luZyAqIHRvIGRlcmVmZXJlbmNlIHB0ciBhbmQgZ2V0IHZhbHVlIG9mIHgKCnJldHVybiAwOwp9
Output:
Size of int: 4 bytes
Size of x: 4 bytes
Address of x: 0x7ffcde08601c
Address stored in ptr: 0x7ffcde08601c
Value at ptr (value of x): 10
Explanation:
In the code example,
- We declare an integer variable x and initialize it with the value 10.
- Then, we use the sizeof() operator inside printf() statements to determine the size of the int data type and the size of the variable x and print them to the console.
- Next, we use the address-of operator (&) to get the memory address of the variable x and assign it to the pointer variable ptr.
- We then print the address of x twice, first accessing it using the address of operator and then using the pointer variable itself.
- After that, we use the dereference operator with ptr to access the value of x and print it to the console.
Check out this amazing course to become the best version of the C programmer you can be.
The Operators Precedence In C
Just like BODMAS in mathematics, programming languages like C use a predefined set of rules to determine the order in which operators are evaluated in an expression. This hierarchy is called operator precedence, and it ensures that operators with higher precedence are evaluated before those with lower precedence.
For example, the multiplication operator has a higher precedence than the addition operator. So, in an expression like x = 7 + 4 * 4, C will first multiply 4 * 4 (yielding 16) and then add 7, giving a final result of 23 rather than 44.
In addition to precedence, operators in C program also follow associativity rules, which determine the order in which operators of the same precedence level are evaluated, typically from left to right or right to left.
For clarity, below a table showing operator precedence in C, with operators at the top having the highest precedence with those towards the bottom have the lowest precedence. We have also mentioned the associativity of operators in the last column.
Category |
Operator |
Associativity |
Postfix |
() [] -> . ++ - - |
Left to right |
Unary |
+ - ! ~ ++ - - (type)* & sizeof |
Right to left |
Multiplicative |
* / % |
Left to right |
Additive |
+ - |
Left to right |
Shift |
<< >> |
Left to right |
Relational |
< <= > >= |
Left to right |
Equality |
== != |
Left to right |
Bitwise AND |
& |
Left to right |
Bitwise XOR |
^ |
Left to right |
Bitwise OR |
| |
Left to right |
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
Operators in C serve as essential building blocks for performing computations, from basic arithmetic to complex bitwise manipulations. In this article, we've explored different types of operators in C, along with their subtypes.
Understanding these operators—arithmetic, relational, logical, bitwise, and others—allows programmers to write efficient and readable code. Mastering them can help optimize performance, enhance accuracy in comparisons, and manage control flow effectively. As with any skill in C programming, proficiency with operators grows through practice and a solid grasp of these fundamental concepts.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is the difference between prefix and postfix operators in C?
In C, prefix operators and postfix operators indicate where an operator is placed relative to its operand.
- A prefix operator precedes the operand. There are two types of prefix operators, i.e., prefix increment (++) and prefix decrement (--). For instance, ++a increments value of a before using its value, so if a is 4, ++a would yield 5. This is the prefix increment operator.
- A postfix operator follows the operand. Again, there are postfix increments and postfix decrement operators. For example, a++ increments the value of a after its current value is used, so if a is 4, b = a++ assigns 4 to b and then increments a to 5. This is the postfix increment operator.
Q. What are the types of C operators?
The primary types of operators in C are:
- Arithmetic operators: These operators perform mathematical calculations like addition, subtraction, multiplication, division, and modulus (remainder of division).
- Relational Operators: They are used to compare values of two operands and return a true or false Boolean value. It includes ==, !=, <, >.
- Logical Operators: These operators are used to apply logical operations to Boolean values, such as AND, OR, and NOT.
- Bitwise operators are used to perform different types of operations on the operands' binary values (at bit-level).
- Assignment Operators: These operators are used to Assign values to variables, like =, +=, -=.
- Conditional Operator: This is used for making decisions based on a condition being met and implementing an expression.
Q. What is a special operator in C?
There is no specific "special operator" in the C programming language. However, certain operators have a reputation for being "special" due to their particular or specialized capability. These consist of:
- The sizeof operator returns a data type's or variable's size in bytes.
- The comma operator (,) allows multiple expressions to be evaluated sequentially, returning the value of the last expression.
- The pointer operators– dereference (*) and address-of (&) help with memory access. The former dereferences a pointer to access a value, and later retrieves a variable's memory address.
Q. What are the different data types in C?
The basic data types in C include:
- int: Stores integers (or whole numerical values) like -1, 0, 1. Typically, int occupies 4 bytes on 32-bit systems, allowing values between -2,147,483,648 and 2,147,483,647.
- float: Stores floating-point numbers like 3.14 with up to six decimal places, covering a wide range from approximately 1.2E-38 to 3.4E+38.
- double: Similar to float, but with more precision (up to 15 decimal places) and a range from 2.3E-308 to 1.7E+308.
- char: Holds single characters like 'a' or '1', typically occupying one byte with a range of 0 to 255 (unsigned) or -128 to 127 (signed).
- void: Represents the absence of a value, used in functions that do not return a value.
Q. What is a loop in C?
Loops are special control statements that allow us to repeatedly execute a block of code while a specific condition holds true. The three different kinds of loops in the C programming language:
1. The for loop: When we know the number of iterations in advance, we utilize the for loop. An initialization statement, a check condition, and an increment/decrement statement make up its syntax:
for(condition; initialization; updation){
// loop body
}
2. While loop: When we have a condition that needs to be verified before each iteration but does not know the number of iterations in advance. The while loop syntax is as follows:
while(condition){
// loop body
}
3. do-while loop: Unlike the while loop, which checks the condition at the start of each iteration, the do-while loop checks the condition after each iteration. This means that even if the condition is initially false, the code inside the loop will be executed at least once. The do-while loop syntax is as follows:
do {
// loop body
} while (condition);
Q. What is a variable in C?
A variable in C is a storage space in memory with a specified data type used to hold values. In other words, it is named location for data.
- Variables must be declared before use, specifying both the type of data being stored and the name/ identifier being given to the location/ variable. Once declared, values can be assigned using the simple assignment operator (=).
- We can then use these variable names to access the data, allowing us to dynamically manipulate and process data within programs.
For example:
int a;
This line of code tells the compiler that we want to create a variable named "a" that could keep integer values. This is referred to as variable declaration, which can be done without initialization. Then, we can initialize the variable by assigning it an initial value using the simple assignment operator (=). For example:
a = 5;
By now, you must know about the different types of operators in C and their uses. Here are some other topics you must explore:
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
- Recursion In C | Components, Working, Types & More (+Examples)
- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
- Null Pointer In C | Creation, Dereferencing & More (+Examples)
- Constant In C | How To Define, Rules & Types (+Detailed Examples)
- Union In C | Declare, Initialize, Access Member & More (Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment