Arithmetic Operators In C | Types & Precedence Explained (+Examples)
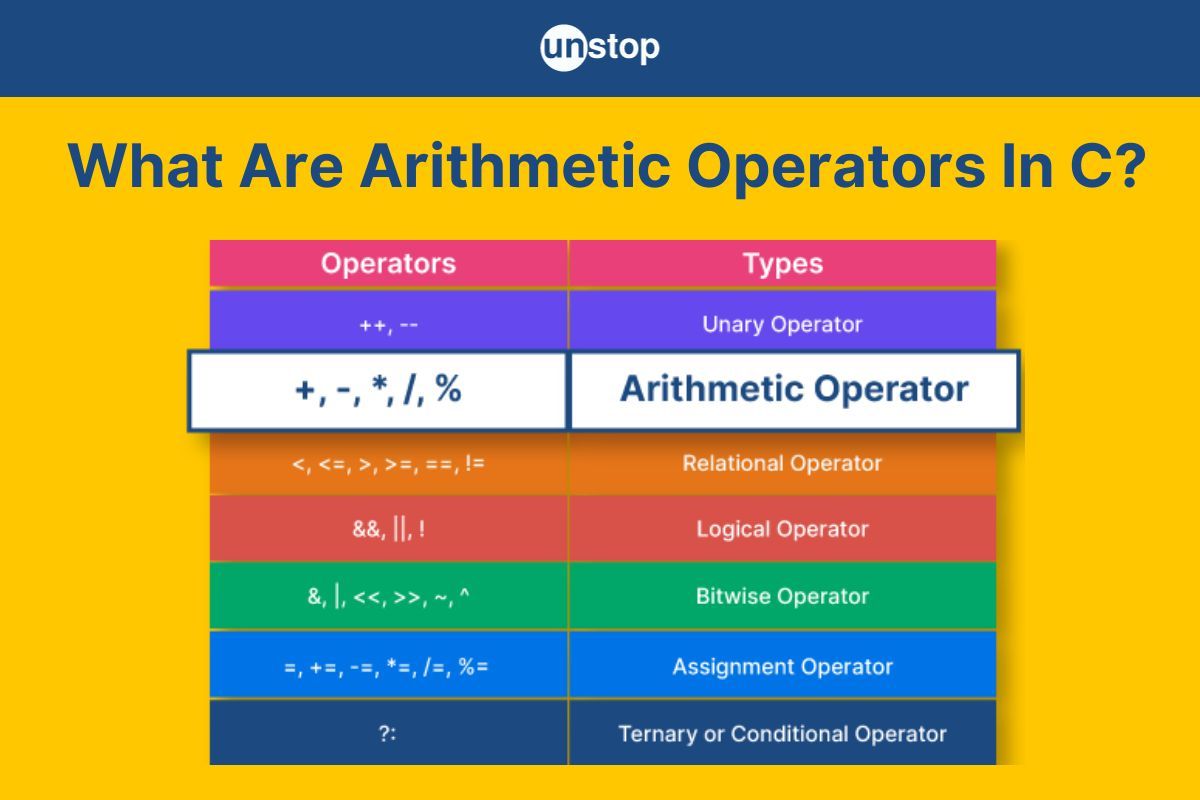
Operators are integral for data manipulation in programming as they define the operations that must be conducted on given operands. There are multiple types of operators, including arithmetic operators, logical, bitwise, relational, conditional operators, etc.
In this article, we will focus on the arithmetic operators in C and demonstrate how they can be used in your programming endeavours. They are fundamental to C programming, allowing developers to perform mathematical computations easily. So let's get started.
What Are Arithmetic Operators In C?
Arithmetic operators in C are fundamental components that enable developers to perform basic arithmetic/ mathematical calculations within C programs. These operators include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
In other words, these operators are used to manipulate numeric data types, such as integers and floating-point numbers, allowing us to perform tasks like addition, subtraction, multiplication, division, and finding remainders.
Types Of Arithmetic Operators In C
The arithmetic operators in C language can be classified into two broad types depending on the number of operands they can take. These types are binary operators, i.e., those that take two operands, and unary operators, i.e., those that work with a single operand.
In total, there are eight arithmetic operators that you can use to perform mathematical operations when writing and running a C program. In this section, we will look at these two type of operators and explain all arithmetic operators with examples in sections ahead.
Binary Operators In C
The term binary operators can be used in two contexts, one in general programming and the other in binary arithmetic. In the context of binary arithmetic, a binary operator can used to perform operations on binary data only.
But in general programming, a binary operator is one that takes two operands and performs the respective calculations on their values. The table below lists the binary arithmetic operators in C, with a brief description, and an example of arithmetic calculations.
Operator |
Name of the Operator |
Arithmetic Operation |
Syntax |
+ |
Addition |
It calculates the sum of two operands. |
A + B |
– |
Subtraction |
It subtracts the second operand from the first operand. |
A – B |
* |
Multiplication |
It calculates the product of or multiplies the two operands. |
A * B |
/ |
Division |
It divides the first operand by the second operand. |
A / B |
% |
Modulus |
It calculates the remainder when the first operand is divided by the second operand. |
A % B |
Now that we know what binary arithmetic operators in C are, let's look at an example. The simple C program example below shows how to use these operators in practice. We will also discuss these operators individually in later sections.
Code Example:
Output:
57
11
782
1
11
Explanation:
We begin the simple C code example by including the standard header file for input-output <stdio.h>.
- Then, we define the main() function, which serves as the program's entry point.
- Inside the main, we declare two integer variables, x and y, and assign the values 34 and 23 to them, respectively.
- Next, as mentioned in the code comments, we perform arithmetic operations, i.e., addition and subtraction on variables x and y, the results of which are stored in variables sum and diff, respectively.
- Similarly, we apply other binary arithmetic operators, i.e., multiplication, division, and modulus on variables x and y, and store the results in variables product, divi, and modulus, respectively.
- We use multiple printf() statements to print the results of the arithmetic operations to the console side by side.
- Here, we use the %d format specifier to signify integer value and the newline escape sequence (\n) to shift the cursor to the next line inside the formatted string for printf().
- Finally, the main() function closes with a return 0 statement indicating successful execution.
Unary Operators In C
Unary operators, as the name suggests, operate on a single operand and alter its value as stipulated. This includes the unary plus, unary minus, increment and decrement operators. The last two of these can be used as prefixes or postfixes, depending on whether they appear before or after the operand.
Operator |
Symbol |
Operation |
Implementation |
Decrement Operator |
-- |
Decreases the integer value of the variable by one. |
--A or A-- |
Increment Operator |
++ |
Increases the integer value of the variable by one. |
++A or A++ |
Unary Plus Operator |
+ |
Returns the value of its operand. |
+A |
Unary Minus Operator |
– |
Returns the negative of the value of its operand. |
-A |
The C program example below illustrates how to use unary operators in programming to get desired results.
Code Example:
Output:
a = 5
b = -5
c = 6
d = 5
Explanation:
The C code example-
- Inside the main() function, we declare a variable a, of integer data type and set it to the value 5.
- Next, we declare variables b, c, and d and assign values to them as follows:
- Variable b is assigned the negation of a using the unary minus operator, making it -5.
- Variable c is assigned the value of a incremented by 1, using the increment operator.
- Variable d is assigned the value of a decremented by 1 using the decrement operator.
- Then, we use the printf() function from <stdio.h> file to print the values of these variables.
- The program returns 0, indicating successful execution.
Learn more about increment/ decrement operators here- Increment And Decrement Operators In C With Precedence (+Examples)
Addition Arithmetic Operator In C
The plus/ addition arithmetic operator (+) in C is a binary operator that sums (or adds) two operands. The operands may be characters, integers, or floating-point numbers of various sorts. Note that the data type of the resulting value will be the same as the data type of the operands.
The plus operator is frequently combined with variables or constants in programming languages to carry out basic operations. For instance, the formula a = b + c would assign the value of b plus c to the variable a.
Syntax for addition arithmetic operator in C:
data_type result = operand1 + operand2
Here,
- The data_type refers to the data type of the operands and the outcome value.
- Operand1 and operand 2 are the main operands on which we perform the addition operator, indicated by the addition operator (+).
- The result variable is used to store the outcome of the operation.
The example C program below illustrates the use of the addition arithmetic operator in C.
Code Example:
Output :
The sum of 5 and 10 is 15
Explanation:
In the example C code-
- We declare two integer variables, x and y, and initialize them with values 5 and 10 inside the main() function.
- Then, we use the addition operator to calculate the sum of x and y, the result of which is stored in variable c.
- After that, we use the printf() function to display the sum of x and y and their values to the console.
Subtraction Arithmetic Operator In C
The subtraction arithmetic operator is represented by the minus (-) symbol. It is a binary operator that subtracts the value of one operand from another, i.e., the amount being subtracted from is the minuend, and the amount being subtracted is the subtrahend.
As an illustration, the single expression 5 - 3 would equal 2 because 5 is decreased by 3. As 7 is deducted from 10, the expression 10 - 7 would also evaluate to 3 and have the value 3.
Syntax:
data_type result = operand1 - operand2
Here,
- Operand1 and operand2 are the two main operands where the value of operand2 is deducted from the value of operand1.
- The (-) sign represents the subtraction operator, and its outcome is stored in the variable result.
Look at the sample C program below, which highlights the implementation of this arithmetic operator.
Code Example:
Output:
The subtraction of 10 and 5 is 5
Explanation:
In the sample C code-
- In the main() function, we declare the two integer variables x and y and initialize them with the values 5 and 10, respectively.
- Then, we use the subtraction (-) operator to subtract the value of x from y and store the result in the variable c.
- Next, we display the result of the subtraction to the console using the printf() function from the <stdio.h> header.
- The program returns 0, which signifies successful execution.
Multiplication Arithmetic Operator In C
The multiplication operator, denoted by the asterisk symbol (*), is used to multiply two values. It is a binary arithmetic operator, which means that the multiplicand and the multiplier are its two operands. For instance, since 3 is multiplied by 4, the expression (3 * 4) would evaluate to 12.
Syntax:
data_type result = operand1 * operand2
Here,
- Operand1 and operand2 refer to the main operands on which the multiplication operator, i.e., * symbol, is applied.
- The result variable stores the outcome of the operation, and the data type of all the variables/ values is given by data_type.
Below is a C program sample to showcase the use of this arithmetic operator.
Code Example:
Output:
The multiplication of 10 and 5 is 50
Explanation:
In the C code sample-
- Inside the main() function, we declare two integer variables, x and y, and initialize them with the values 5 and 10, respectively.
- Then, using the multiplication operator, we multiply the value of x by the value of y. The result of this operation is stored in a third variable, c.
- Next, we employ the printf() function to output the result of the multiplication, with a message, to the console.
- The program returns 0, indicating successful execution.
Division Arithmetic Operator In C
The division operator is another binary arithmetic operator in C used to divide two values/ operands. In other words, we use the division operator, denoted by the sign forward slash sign (/), to divide one operand (the dividend) by the other operand (the divisor).
For instance, when 10 is divided by 5, the expression (10/5) would be evaluated to 2. As 7.5 is divided by 2.5, the expression (7.5 / 2.5) would be evaluated to 3, a positive number.
Syntax:
data_type result = operand1 / operand2
Here,
- Variables operand1 and operand2 refer to the dividend and divisor, respectively, while variable result stored the outcome of the division operation.
- The (/) symbol represents the division operator.
Code Example:
Output:
The Division of 10 and 5 is 2
Explanation:
- We declare two integer variables, x, and y, inside the main() function and initialize them with the values 5 and 10, respectively.
- Then, using the division operator, we divide the value of y by the value of x. The result of this division is stored in variable c.
- Since both y and x are integers, this operation performs integer division, resulting in a quotient of 2.
- Next, we call the printf() function to display the result of the division along with the value of variables x and y.
- The program returns 0, indicating successful execution.
Modulus % Arithmetic Operator In C
The modulus operator, denoted by the percentage symbol (%), takes two operands and returns the remainder value after dividing the two values. In short, this arithmetic operator in C returns the remainder of its two operands. For instance, the expression (10% 3) would equal 1 because 10 divided by 3 leaves 1 as the remainder. Similarly, the expression (15% 6) would result in 3 since 3 remains after dividing 15 by 6.
Syntax
data_type result = operand1 % operand2
Here,
- Variables operand1 and operand2 refer to the operands on which we apply the modulus operation, represented by the % symbol.
- The result represents the variable where we store the result of the operation.
Code Example:
Output:
The modulus of 10 and 5 is 0.
Explanation:
For the simple C code example above-
- Inside the main() function, we declare two integer variables, x and y, and initialize them with the values 5 and 10, respectively.
- Then, we use the modulus operator to calculate the remainder of the division between x and y. The result of this mathematical operation is stored in variable c.
- The modulus operation calculates the remainder when y is divided by x. Since here, 10 is perfectly divisible by 5, the remainder after division is 0.
- Next, we use the printf() function to display the result of the modulus operation with a message to the console.
- The program returns 0, indicating successful execution.
Increment Arithmetic Operator In C
This is a unary operator, i.e., it works with only one operand. The increment operator is used to raise the value of a variable by one and is denoted by the double plus symbol (++). It can be used as both a prefix and a postfix operator. Note that the pre-increment operator and the post-increment operator are the two different kinds of increment operators used in programming.
Pre-increment operator: A unary operator known as the pre-increment operator is denoted by the symbol (++) applied before the operand. It increases the value of the operand by 1 before evaluating the expression that contains the operand. For example-
int x = 5;
int y = ++x;
In this example, the value of x is increased by 1 before it is assigned to the variable y, thus resulting in the value of y becoming 6.
Post-increment operator: The unary post-increment operator is denoted by the symbol (++) applied after the operand. It increases the value of the operand after the expression containing the operand is evaluated. For example-
int x = 5;
int y = x++;
In this example, the initial value of variable x is 5, and the variable y is assigned its value with the post-increment operator on x. As a result, the initial value of x is allocated to y, i.e., 5, after which the value of x is raised to 6.
Code Example:
Output:
After Pre-Increment: x = 6, y = 6
After Post-Increment: x = 7, z = 6
Explanation:
The C program above begins by including the standard input-output library.
- Inside the main() function, we initialize the integer variable x with the value of 5.
- Then, we declare two more integer variable y and z (without initialization) in a single line using the comma operator.
- Next, we assign the value x with a pre-increment operator to variable y, i.e., the value of x is increased to 6. The result is then printed to the console using the printf() function.
- Similarly, we assign the value of x with the post-increment operator to the variable z (i.e., z = x++). As a result, the value of z goes to 6, and x becomes 7. This output is also printed to the console using the printf() function.
- The program returns 0 to indicate successful execution.
Decrement Arithmetic Operator In C
The decrement operator is used to reduce the value of a variable by one. Since it works with only a single operand, it is a unary operator. Just like the increment operator, this can also be used as both a prefix and a postfix operator and is denoted by the double minus symbol (--).
In other words, there are two different kinds of decrement operators used in programming, i.e., the pre-decrement operator and the post-decrement operator.
Pre-decrement operator: The unary pre-decrement operator is applied by applying the symbol (--) before the operand. This operator reduces the value of the operand by 1 before evaluating the conditional expression containing the operand. For example-
int x = 5;
int y = --x;
In this snippet, the initial value of variable x is set to 5, after which we assign its value to the variable y with the pre-decrement operator. As a result, the value of y becomes 4.
Post-decrement operator: This unary operator is applied when we position the basic symbol (--) after the operand. It reduces the value of the operand by 1 after the expression containing the operand is evaluated. For example-
int x = 5;
int y = x--;
In this example, the initial value of variable x is set to 5. Then, variable y is assigned the value of x with the post-decrement operator. As a result, the value of y becomes 5, whereas the value of x becomes 4.
Let's look at a code where we showcase the application of both these decrement operators.
Code Example:
Output:
After Pre-Decrement: p = 4, q = 4
After Post-Decrement: p = 3, r = 4
Explanation:
In this example, we define the main() function after including the <stdio.h> file.
- Inside the main, we declare three integer variables, i.e., p, q, and r. Of these, we set the initial value of p to 5.
- Next, we initialize variable q with the value of p with the pre-decrement operator applied to it, i.e., q = --p. This decreases the value of p by 1 before assigning it to q.
- We use the printf() function to display the result of this operation to the console with a string message. The value of both p and q becomes 4.
- Then, we use the post-decrement operator on p and assign that value to variable r, i.e., r = p--. This assigns the initial value of p to r before decrementing the value of p. As a result, r gets the value of 4, but p decreases to 3.
- Another printf statement displays the effect of post-decrement on p and the value assigned to r.
- The program returns 0, indicating successful execution.
Check out this amazing course to become the best version of the C programmer you can be.
Precedence & Associativity | Evaluation Of Arithmetic Expressions
The evaluation of expression with arithmetic operators in C is affected by multiple factors, including the precedence of operators, their associativity, and the data types of the variables on which we are applying the operators. In this section, we will discuss these three aspects of the arithmetic expression evaluation.
Precedence Of Arithmetic Operators In C Language (From Highest To Lowest)
In an expression, operator precedence refers to the order in which operators are assessed/ evaluated. Some operators are evaluated earlier than others because they have higher precedence. The table below lists the precedence of arithmetic operators in C in reference to all other operator types.
Precedence Level | Operators |
1 | Postfix Operators (), [], ->, ++, -- |
2 | Unary Operators ++, --, +, - |
3 | Cast Operator (type) |
4 | Arithmetic Operators *, /, %, +, - |
6 | Shift Operators <<, >> |
7 | Relational Operators <, <=, >, >= |
8 | Equality Operators ==, != |
9 | Bitwise Operators AND, OR, XOR |
10 | Logical Operators AND, OR, NOT |
11 | Conditional Operator ?: |
12 | Assignment Operators =, *=, /=, %=, +=, -= |
13 | Comma Operator (,) |
In addition to precedence in reference to other operands, there are different precedence levels within every operator group. For instance, in the arithmetic expression (2 + 3 * 4), the multiplication operation is evaluated first since it has precedence over addition (3 * 4 = 12). Once that is done, the addition operation is assessed as 2 + 12 = 14.
The following table lists the precedence within arithmetic operators in C from highest to lowest.
Operators |
Precedence |
Brackets () |
Highest |
Increment and Decrement operators (++, --) |
2nd Highest |
Unary plus and minus (+, -) |
3rd Highest |
Multiplication, division and modulus operators (*, /, %) |
4th Highest |
Binary plus and minus (+, -) |
5th Highest |
Assignment/ equal to operator (=) |
Lowest |
Associativity Of Arithmetic Operators In C
In an expression, associativity describes the sequence in which operators with the same precedence are evaluated. Some operators evaluate from left to right because they are left-associative. Others are right-associative, which means they are analyzed right to left.
For example, the exponentiation operator is right-associative, as a result the expression (2 ** 3 ** 2) is evaluated as- 2 ** (3 ** 2) = 2 ** 9 = 512.
The table below lists the associativity of arithmetic operators in C, with the same precedence.
Operators |
Associativity |
Brackets () |
Left-to-right |
Increment and Decrement operators (++, --) |
Right-to-left |
Unary plus and minus (+, -) |
Right-to-left |
Multiplication, division and modulus operators (*, /, %) |
Left-to-right |
Binary plus and minus (+, -) |
Left-to-right |
Assignment/ equal to operator (=) |
Right-to-left |
Data Type
The type of variables/ data values used in an expression are referred to as their data type. For instance, although floating-point arithmetic uses decimal values, integer arithmetic exclusively uses whole integers. When using values in an expression, one must ensure that the data type of the values and the return value are the same.
If this is not the case, then type conversion may be required to make sure the operands are compatible when evaluating expressions with various complex data types. While this occurs automatically in some programming languages, it must be explicitly converted in others.
Multiple Arithmetic Operators In A Single Expression
There is a possibility that multiple operators may be used in a single expression. When this happens, the operators are ranked according to their precedence and associativity. In other words, when an expression contains numerous operators, their precedence, associativity, and occasionally the usage of brackets to group sub-expressions affect the order in which they are evaluated.
For example, consider the following expression-
4 + 5 * 6 - 2 / 2
The four operators included in this expression are addition, multiplication, subtraction, and division. The operator precedence and associativity principles will define how to evaluate this expression. Thus, the multiplication and division operations are performed first since they take precedence over addition and subtraction.
Because multiplication and division have the same precedence and are left-associative, we evaluate them from left to right. Similarly, since both addition and subtraction operators also share the same precedence and are left-associative, we evaluate addition and subtraction from left to right.
Applying these rules, the expression given above is evaluated as follows-
4 + 5 * 6 - 2 / 2 // evaluate multiplication first
4 + 30 - 2 / 2 // evaluate division next
4 + 30 - 1 // evaluate addition and subtraction last
33
Hence, the result of the expression turns out to be 33.
It's important to note that using brackets to group sub-expressions, forces the enclosed section to be evaluated first. This can override the operator precedence rules, thus modifying the order in which operators are evaluated. For example, consider the following expression-
(4 + 5) * 6 - 2 / 2
The brackets indicate that the addition operation should be evaluated before the multiplication. Therefore, we evaluate the expression as follows-
(4 + 5) * 6 - 2 / 2 // evaluate addition inside parentheses first
9 * 6 - 2 / 2 // evaluate multiplication next
54 - 1 // evaluate division and subtraction last
53
In this case, the result of the expression turns out to 53.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
Arithmetic operators in C provide the foundation for many mathematical computations in programming. Understanding how these operators work and being mindful of basic data types ( integer data type, floating-point data types, etc.), precedence, and potential issues like overflow and underflow is crucial for writing robust and reliable code. By mastering these operators, you'll be well-equipped to perform a wide range of mathematical tasks in your C programs and build efficient and error-free software.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. How does one prefix the — and ++ operators?
The symbols (--) and (++) represent the decrement and increment operators, respectively. They are used to decrease or increase the value of a variable by 1, respectively. The placement of the operators before or after an operand determines if they are being used in the prefix or postfix form.
- When the operator is applied before the operand, it becomes a prefix operator, meaning the operation is performed before the value is used in the expression.
- When the operator is applied after the operand, it is referred to as a postfix operator, meaning the operation is performed after the value is used. For example:
int x = 5;
int y = --x;
In this example, the decrement operator (--) is placed before the variable x. This means that the value of x will be decremented by 1 before the value of y is assigned. As a result, the value of x becomes 4, and the value of y will also be 4. Similarly:
int x = 5;
int y = ++x;
In this snippet, the increment operator (++) is placed before the variable x. This means that the value of x will be incremented by 1 before the value of y is assigned. As a result, the value of x will become 6, and the value of y will also be 6.
Also note that when the operator is applied after the operand in an expression, it is called post-fix. That is, post-increment or post-decrement.
Q. What types of operands can arithmetic operators in C accept?
In C, arithmetic operators primarily operate on numerical operands, such as integer operands (int, long) and floating-point numbers (float, double). Ideally, the type of both operands must be the same, which will also be the type of the value achieved after the respective numerical calculation is done. For example, if both your operands are float data type numbers, then the returned value must also be of the same type.
There may be situations when you might have to perform arithmetic operations on non-numerical operands. However, when operands of different types are involved in an operation, typecasting may be used to convert one operand to a compatible type for the operation.
Q. How do you perform increment and decrement in the C programming language?
In C programming language, you use the increment (--) and the decrement (++) operators to increase or decrease the value by one, respectively.
- These operators are commonly used in loops and other control statements/ structures to modify the value of a variable.
- The increment operator is used to increment the value of a variable by one, and the decrement operator is used to decrement the value of a variable by one. These operators can be used as either a prefix or a postfix operator.
Q. How can one discover the (modulus) remainder?
The modulus arithmetic operator in C is used to find the remainder of an integer division. In C programming language, the modulus operator is represented by the % symbol. Given below is a snippet example showcasing how to use the modulus operator to find the remainder of an integer division in C-
int dividend = 17;
int divisor = 5;
int remainder = dividend % divisor;
In this example, we declare two variables, dividend and divisor, and initialize them with the values 17 and 5, respectively. We then carry out an integer division of 17 by 5, which results in 3 with a remainder of 2. Here, the value of the remainder will be 2, i.e., the remainder of the integer division of dividend by divisor. The modulus operator finds this remainder and stores it in the variable remainder.
Note- The modulus operator can only be used with integer types, such as int, long, short, and char. It cannot be used with floating-point types like float and double.
Q. What is the difference between the unary minus and subtraction operators?
In C programming, both unary minus and subtraction operators are used to perform subtraction operations on operands. However, there are a few important differences between these two operators.
The subtraction operator (-) is a binary operator used to subtract two values. It requires two operands, one on either side of the operator. For example-
int x = 10;
int y = 5;
int z = x - y;
In this instance, the subtraction operator is used to subtract the value of y from the value of x, and the result is assigned to the variable z.
In comparison, the unary minus operator (-) is used to negate the value of a single operand. It only requires one operand, which appears immediately after the operator. For example-
int x = 10;
int y = -x;
In this illustration, the unary minus operator negates the value of x, and the result is assigned to the variable y.
As is evident from the example, the primary difference between the two operators is that the subtraction operator requires two operands to calculate the difference. In contrast, the unary minus operator demands only one operand. The unary minus operator is also used to negate the value of the operand, while the subtraction arithmetic operator in C subtracts one value from another.
Q What comes first in operation, the decrement (--), the equal to (=), or the increment (++) operator?
In C programming, the order of evaluation is governed by operator precedence and associativity. The decrement (--) and increment (++) operators have higher precedence than the assignment (=) operator. Meaning, when used in a single expression, the decrement operator (--) is evaluated first, and then the assignment (=) operator is evaluated.
For example, in an expression such as x = --y, the value of y is first incremented by 1 and then the value is assigned to the variable x.
int x, y;
y = 5;
x = --y;
In this code snippet, the initial value of y is set to 5, which is decremented by 1 before being assigned to x. So, the evaluation result of the expression is- the value of x will be 4, and the value of y will also be 4.
It is worth noting that the same rule applies to the increment operator (++). So, the expression (x = ++y) results in the value of y being incremented by 1, which is then assigned to the variable x. Therefore, the increment (++) and decrement (--) operators have higher precedence than the assignment (=) arithmetic operator in C.
You might also be interested in reading the following:
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Convert Binary To Decimal In C | 4 Ways Explained (+Code Examples)
- Functions In C | Uses, Types, Components & More (+Code Examples)
- Union In C | Declare, Initialize, Access Member & More (Examples)
- Conditional/ If-Else Statements In C | The Ultimate Guide
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment