Table of content:
- What Is A Matrix?
- What Is The Transpose Of A Matrix?
- Algorithm For Finding The Transpose Of A Matrix In C
- C Program To Find The Transpose Of A Matrix (Using For Loop)
- Transpose Of A Square Matrix In C
- Transpose Of A Rectangular Matrix
- Conclusion
- Frequently Asked Questions
Transpose Of A Matrix In C | Properties, Algorithm & More (+Codes)
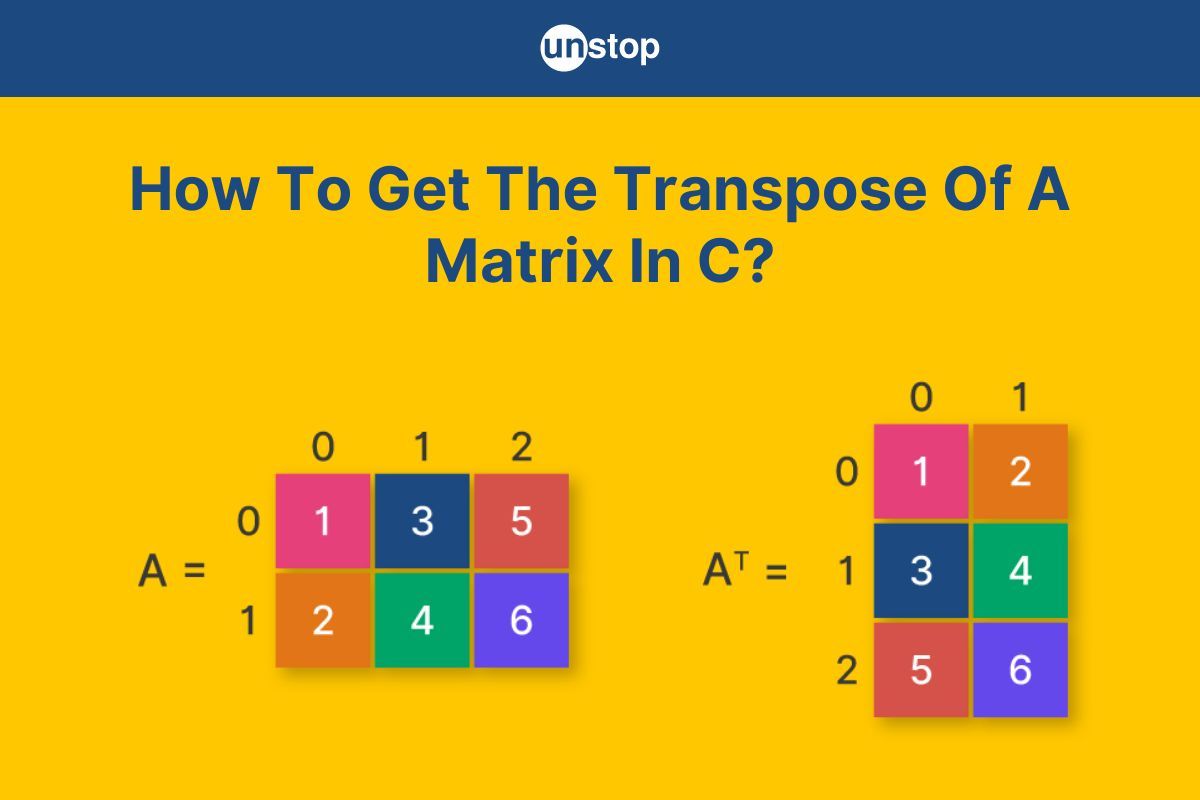
Matrices play a crucial role in various mathematical and scientific computations. One fundamental operation performed on matrices is the transpose, which involves swapping the rows and columns of a matrix. In C programming, understanding and implementing the transposition of a matrix is essential for handling data transformations and manipulations efficiently.
In this article, we will learn about the working algorithm for creating the transpose of a matrix in C programming language. Whether you're new to matrices or an experienced programmer aiming to enhance your programming skills, learning how to transpose a matrix in C can amplify your data handling and computation capabilities.
What Is A Matrix?
A matrix is a set of elements arranged in a rectangular grid or array within two-dimensional (and occasionally three-dimensional) space. Sometimes, a matrix and an array are mistakenly interpreted as the same, but in fact, they aren’t technically the same. While all matrices are arrays, not all arrays are matrices.
In simple terms, all matrices are a type of array, but not all arrays are organized like matrices containing rows and columns. Some arrays can be like a mixed-up collection of different things, while matrices are special because they have a structured table-like arrangement.
In C programming language, a matrix is represented as a multi-dimensional array, specifically a two-dimensional (2D) array consisting of rows and columns, representing a table-like structure.
For example, A [3][2] is a non-square matrix of three rows and two columns. We will then say that matrix A is a '3 by 2' matrix, which is written as 3x2. The elements in a matrix are stored in contiguous memory locations.
Properties Of A Matrix
When working with matrices, like creating the transpose of a matrix in C programs, one must consider the basic properties and characteristics of matrices. Here are some key matrix properties that you must be aware of:
- Order or Dimensions: A matrix has an order or dimension given by the number of rows and columns (m x n). It is often denoted as mxn or m*n, where m represents the number of rows and n represents the number of columns.
- Elements: The individual values within a matrix are called elements. We can refer to or identify an element by its row and column indices.
- Equality: Two matrices are equal if and only if they have the same order and corresponding elements are equal.
- Addition: Matrix addition is defined for matrices of the same order. It involves adding corresponding elements together.
- Scalar Multiplication: A matrix can be multiplied by a scalar (a single number). Each element of the matrix is multiplied by the scalar.
- Transpose: The process of creating the transpose of a matrix involves swapping its rows into columns and columns into rows. That is, if the dimensions of matrix A, are m*n, then the transpose of matrix A will have dimensions n*m.
- Symmetric Matrix: A square matrix is symmetric if its transpose is equal to itself.
- Diagonal Matrix: A square matrix is diagonal if all its non-diagonal elements are zero.
- Identity Matrix: The identity matrix is a square matrix with ones on its main diagonal and zeros elsewhere. Multiplying any matrix by the identity matrix does not change the original matrix.
What Is The Transpose Of A Matrix?
The transpose of a matrix in C refers to a new matrix obtained by swapping its rows and columns. To visualize this, imagine a classroom where desks are originally arranged in x rows and y columns. The transpose of this arrangement would involve switching the rows to columns and columns to rows.
Original seating arrangement | Transposed seating arrangement |
A B C D E F G H I J K L |
A E I B F J C G K D H L |
In the table above, you can see how the students have changed their row order to column order, resulting in the transposition of the original matrix/ seating order. When working on getting the transpose of a matrix in C programming, it is important to note that the order of the matrix changes for non-square matrices.
Here’s an example for understanding the concept of matrix transposition better:
Matrix |
Transpose |
10 20 30 Order=3x3 |
10 40 70 Order=3x3 |
Here, the order of the matrix remains the same even after the transposition because this is a square matrix. However, the same will not hold for a rectangular matrix. Take a look at the example below:
Matrix |
Transpose |
10 20 30 Order=2x3 |
10 40 Order=3x2 |
Here, the order has changed after transposing. That is, the order of the resultant matrix is n*m, which has changes from m*n of the input matrix.
Now that we know about the basics of transposing, let's look at the working algorithm and a sample program to get the transpose of a matrix in C language.
Algorithm For Finding The Transpose Of A Matrix In C
The algorithm for finding the transpose of a matrix in C involves swapping the rows and columns. Here's a step-by-step explanation of the workings of the algorithm:
Step 1: Input
We begin with an input matrix, usually represented as a 2D array in C. Say the dimensions of the matrix are m*n.
Step 2: Initialize Transpose Matrix
Next, create a new matrix to store the resultant matrix, say transpose. We know that the dimensions of this matrix will be n*m, the reverse of the original matrix.
Step 3: Nested Loop for Computation
Next, use two nested loops to traverse each element of the original matrix. For each element A[i][j] in the original matrix, assign its value to the corresponding position transpose[j][i] in the transpose matrix.
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
transpose[j][i] = A[i][j];
}
}
This swapping process effectively transposes the matrix, exchanging rows with columns.
Step 4: Display the Transpose Matrix
Use another set of nested loops to print the elements of the transpose matrix.
for (int i = 0; i < cols; ++i) {
for (int j = 0; j < rows; ++j) {
printf("%d\t", transpose[i][j]);
}
printf("\n");
}
The output will be the transpose of the original matrix, with rows becoming columns and vice versa.
Also read- How To Run C Program | Step-by-Step Explanation (With Examples)
C Program To Find The Transpose Of A Matrix (Using For Loop)
Let's look at the example below that finds the transpose of a matrix in C using a function containing nested for loops.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+Cgp2b2lkIHRyYW5zcG9zZU1hdHJpeChpbnQgbWF0cml4W11bM10sIGludCByb3dzLCBpbnQgY29scykgewppbnQgdHJhbnNwb3NlW2NvbHNdW3Jvd3NdOyAvLyBDcmVhdGUgYSBuZXcgbWF0cml4IHRvIHN0b3JlIHRoZSB0cmFuc3Bvc2UKCi8vIFRyYW5zcG9zZSB0aGUgbWF0cml4CmZvciAoaW50IGkgPSAwOyBpIDwgcm93czsgaSsrKSB7CmZvciAoaW50IGogPSAwOyBqIDwgY29sczsgaisrKSB7CnRyYW5zcG9zZVtqXVtpXSA9IG1hdHJpeFtpXVtqXTsgLy8gUGxhY2UgdGhlIGVsZW1lbnQgaW4gdGhlIHRyYW5zcG9zZWQgcG9zaXRpb24KfQp9CgovLyBQcmludCB0aGUgdHJhbnNwb3NlZCBtYXRyaXgKcHJpbnRmKCJUcmFuc3Bvc2UgTWF0cml4OlxuIik7CmZvciAoaW50IGkgPSAwOyBpIDwgY29sczsgaSsrKSB7CmZvciAoaW50IGogPSAwOyBqIDwgcm93czsgaisrKSB7CnByaW50ZigiJWRcdCIsIHRyYW5zcG9zZVtpXVtqXSk7Cn0KcHJpbnRmKCJcbiIpOwp9Cn0KCmludCBtYWluKCkgewppbnQgbWF0cml4WzNdWzNdID0gewp7MSwgMiwgM30sCns0LCA1LCA2fSwKezcsIDgsIDl9Cn07CgovLyBQcmludCB0aGUgb3JpZ2luYWwgbWF0cml4CnByaW50ZigiT3JpZ2luYWwgTWF0cml4OlxuIik7CmZvciAoaW50IGkgPSAwOyBpIDwgMzsgaSsrKSB7CmZvciAoaW50IGogPSAwOyBqIDwgMzsgaisrKSB7CnByaW50ZigiJWRcdCIsIG1hdHJpeFtpXVtqXSk7Cn0KcHJpbnRmKCJcbiIpOwp9CgovLyBDYWxsIHRoZSB0cmFuc3Bvc2UgZnVuY3Rpb24KdHJhbnNwb3NlTWF0cml4KG1hdHJpeCwgMywgMyk7CgpyZXR1cm4gMDsKfQ==
Output:
Original Matrix:
1 2 3
4 5 6
7 8 9Transpose Matrix:
1 4 7
2 5 8
3 6 9
Explanation:
We begin the simple C program above by including the necessary <stdio.h> header file.
- We then define a function named transposeMatrix() to handle the transposition of a matrix.
- This function takes a 2D array named matrix with a fixed number of columns (3 in this case), along with the parameters rows and cols specifying the number of rows and columns.
- Inside the function, we initialize a new matrix called transpose to store the transposed elements.
- Then, we have two nested for loops that iterate through the original matrix. Each element at position (i, j) is placed in the transposed position (j,i). The result of this is stored in the transpose matrix.
- After that, we have a printf() statement to print the string message- Transpose Matrix. The newline escape sequence is used to shift the cursor to the next line.
- We then have another set of nested for loops with printf() statements to display the transposed/ resultant matrix. The %d format specifier represents an integer value.
- Inside the main() function, we declare and initialize a 3x3 matrix named matrix.
- Next, we print this matrix using nested for loops with print() statements.
- We then call the transposeMatrix, passing the original matrix along with its dimensions (3 rows and 3 columns). This operation transposes the matrix and prints the resulting transposed matrix.
- The program concludes with a return 0 statement to the main function, indicating successful execution without errors.
Time Complexity: The time complexity of the program is O(rows * columns) since we need to visit each element of the matrix once.
Transpose Of A Square Matrix In C
A square matrix is a type of matrix that has an equal number of rows and columns. In other words, a matrix that has n rows and n columns is considered a square matrix. The number n is also often referred to as the order or size of the square matrix. Square matrices are denoted as n*n, where n represents both the number of rows and columns. For example, a 2x2 matrix or a 3x3 matrix is a square matrix.
How To Get Transpose Of A Square Matrix In C?
To transpose a square matrix in C, we typically use a nested loop structure. The outer loop iterates over the rows, and the inner loop iterates over the columns, swapping the elements across the main diagonal. This process continues until all elements have been swapped, resulting in a transposed matrix.
For instance, take a square matrix A, as shown in column 1 of the table below. Then, the transpose of the matrix A, denoted by A^T, will be as shown in the next column.
A = | 10 20 30 | |
A^T = | 10 40 70 | | 20 50 80 | | 30 60 90 | |
Below is an example C code to find the transpose of a square matrix in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgojZGVmaW5lIE51bWJlcnIgMwoKdm9pZCB0cmFuc3Bvc2VNYXRyaXgoaW50IG1hdHJpeFtOdW1iZXJyXVtOdW1iZXJyXSkgewppbnQgaWksIGpqOwoKZm9yIChpaSA9IDA7IGlpIDwgTnVtYmVycjsgaWkrKykgewpmb3IgKGpqID0gaWkgKyAxOyBqaiA8IE51bWJlcnI7IGpqKyspIHsKLy8gU3dhcHBpbmcgZWxlbWVudHMgYWNyb3NzIHRoZSBkaWFnb25hbAppbnQgdGVtcCA9IG1hdHJpeFtpaV1bampdOwptYXRyaXhbaWldW2pqXSA9IG1hdHJpeFtqal1baWldOwptYXRyaXhbampdW2lpXSA9IHRlbXA7Cn0KfQp9CgppbnQgbWFpbigpIHsKaW50IG1hdHJpeFtOdW1iZXJyXVtOdW1iZXJyXSA9IHsKezEsIDIsIDN9LAp7NCwgNSwgNn0sCns3LCA4LCA5fQp9OwppbnQgYWksIGFqOwoKLy8gUHJpbnQgdGhlIG9yaWdpbmFsIG1hdHJpeApwcmludGYoIk9yaWdpbmFsIE1hdHJpeDpcbiIpOwpmb3IgKGFpID0gMDsgYWkgPCBOdW1iZXJyOyBhaSsrKSB7CmZvciAoYWogPSAwOyBhaiA8IE51bWJlcnI7IGFqKyspIHsKcHJpbnRmKCIlZCAiLCBtYXRyaXhbYWldW2FqXSk7Cn0KcHJpbnRmKCJcbiIpOwp9CgovLyBUcmFuc3Bvc2UgdGhlIG1hdHJpeAp0cmFuc3Bvc2VNYXRyaXgobWF0cml4KTsKCi8vIFByaW50IHRoZSB0cmFuc3Bvc2VkIG1hdHJpeApwcmludGYoIlxuVHJhbnNwb3NlIE1hdHJpeDpcbiIpOwpmb3IgKGFpID0gMDsgYWkgPCBOdW1iZXJyOyBhaSsrKSB7CmZvciAoYWogPSAwOyBhaiA8IE51bWJlcnI7IGFqKyspIHsKcHJpbnRmKCIlZCAiLCBtYXRyaXhbYWldW2FqXSk7Cn0KcHJpbnRmKCJcbiIpOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Original Matrix:
1 2 3
4 5 6
7 8 9
Transpose Matrix:
1 4 7
2 5 8
3 6 9
Explanation:
In the above C code example, we get the transpose of a square matrix and print both the original and transposed matrices.
- We begin with including the standard input-output library and defining a constant named Numberr with a value of 3.
- Next, we define a function named transposeMatrix(), which takes a 2D array representing a square matrix of size Numberr x Numberr as a parameter.
- Inside this function, we declare two control variables, ii and ij, of integer data type.
- Then, we have two nested for loops to iterate through the upper triangle of the matrix (above the main diagonal).
- The outer loop controls the number of the row and the inner loop controls the number of the column.
- For each pair of elements at positions (ii, jj) and (jj, ii), their values are swapped and stored in a temporary variable temp.
- The outer loop begins with row 0 since the initial value of ii is set to 0. The control then flows to the inner loop, where the control variable is ii+1, i.e., 1 to begin with.
- The inner loop first stores the element at position [0][1] in the temp variable. It then assigns the value to position number [1][0] to the indice [0][1] and the value of the temp variable to the indice position [0][1].
- After this, the value of jj is incremented by 1 (i.e., jj++), and the inner loop continues to be executed till the value of jj is less than NUMBERR.
- Once the condition becomes false, the control flows back to the outer loop, and the process repeats for row 1.
- We get the transpose of the matrix once all the nested loop iterations are done, i.e., when the outer loop condition becomes false.
- Then, inside the main() function, we declare and initialize a square matrix of size 3x3.
- We then display the original matrix to the console using two nested for loops and printf() statements.
- Next, we call the transposeMatrix() function, modifying the original matrix in place to obtain its transpose.
- Following the transposition, we use another set of nested for loops containing printf() statements to display the transpose of the matrix.
Time Complexity: The code has a time complexity of O(N^2), where N is the size of the square matrix.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Transpose Of A Rectangular Matrix
A rectangular matrix is a type of matrix that does not have an equal number of rows and columns. In other words, if a matrix has m rows and n columns, where m is not equal to n, it is considered a rectangular matrix. Rectangular matrices are denoted as m*n. For example, a 2x3 matrix has 2 rows and 3 columns.
How To Get Transpose Of A Rectangular Matrix In C?
For a rectangular matrix (having a different number of rows and columns), the transpose operation is similar but requires a slight modification to handle the different dimensions. The transpose of a rectangular matrix involves interchanging its rows and columns, resulting in a new matrix with dimensions n rows and m columns, where n ≠ m. In the case of a rectangular matrix, the process to get the transpose of a matric in C essentially flips the original matrix along its main diagonal.
Example: Suppose we begin with an input matrix with dimensions 2x3, i.e., a rectangular matrix. Then, the transpose of this matrix will be of the order 3x2, as shown in the table below.
Original Matrix | Transpose/ Resultant Matrix |
1 2 3 |
1 4 2 5 3 6 |
Notice how the rows and columns have been swapped to create the transpose. The original matrix had 2 rows and 3 columns, but the transpose has 3 rows and 2 columns. This is the characteristic behavior of a rectangular matrix transpose.
Below is a code example of how to find the transpose of a rectangular matrix in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgojZGVmaW5lIE1BWF9ST1dTIDEwCiNkZWZpbmUgTUFYX0NPTFMgMTAKCnZvaWQgdHJhbnNwb3NlTWF0cml4KGludCBtYXRyaXhbTUFYX1JPV1NdW01BWF9DT0xTXSwgaW50IHJvd3dzLCBpbnQgY29scykgewppbnQgdHJhbnNwb3NlW01BWF9DT0xTXVtNQVhfUk9XU10sIGlpLCBqajsKCi8vIEZpbmRpbmcgdGhlIHRyYW5zcG9zZSBvZiB0aGUgbWF0cml4CmZvciAoaWkgPSAwOyBpaSA8IGNvbHM7IGlpKyspIHsKZm9yIChqaiA9IDA7IGpqIDwgcm93d3M7IGpqKyspIHsKdHJhbnNwb3NlW2lpXVtqal0gPSBtYXRyaXhbampdW2lpXTsKfQp9CgovLyBQcmludGluZyB0aGUgdHJhbnNwb3NlIG1hdHJpeApwcmludGYoIlRyYW5zcG9zZSBNYXRyaXg6XG4iKTsKZm9yIChpaSA9IDA7IGlpIDwgY29sczsgaWkrKykgewpmb3IgKGpqID0gMDsgamogPCByb3d3czsgamorKykgewpwcmludGYoIiVkXHQiLCB0cmFuc3Bvc2VbaWldW2pqXSk7Cn0KcHJpbnRmKCJcbiIpOwp9Cn0KCmludCBtYWluKCkgewppbnQgcm93LCBjb2x1bW4sIGFpLCBiajsKaW50IG1hdHJpeFtNQVhfUk9XU11bTUFYX0NPTFNdOwoKLy8gSW5wdXQgbnVtYmVyIG9mIHJvd3MgYW5kIGNvbHVtbnMKcHJpbnRmKCJFbnRlciB0aGUgbnVtYmVyIG9mIHJvd3M6ICIpOwpzY2FuZigiJWQiLCAmcm93KTsKcHJpbnRmKCJFbnRlciB0aGUgbnVtYmVyIG9mIGNvbHVtbnM6ICIpOwpzY2FuZigiJWQiLCAmY29sdW1uKTsKCi8vIElucHV0IHRoZSBlbGVtZW50cyBvZiB0aGUgbWF0cml4CnByaW50ZigiRW50ZXIgdGhlIGVsZW1lbnRzIG9mIHRoZSBtYXRyaXg6XG4iKTsKZm9yIChhaSA9IDA7IGFpIDwgcm93OyBhaSsrKSB7CmZvciAoYmogPSAwOyBiaiA8IGNvbHVtbjsgYmorKykgewpzY2FuZigiJWQiLCAmbWF0cml4W2FpXVtial0pOwp9Cn0KCi8vIFByaW50IHRoZSBvcmlnaW5hbCBtYXRyaXgKcHJpbnRmKCJPcmlnaW5hbCBNYXRyaXg6XG4iKTsKZm9yIChhaSA9IDA7IGFpIDwgcm93OyBhaSsrKSB7CmZvciAoYmogPSAwOyBiaiA8IGNvbHVtbjsgYmorKykgewpwcmludGYoIiVkXHQiLCBtYXRyaXhbYWldW2JqXSk7Cn0KcHJpbnRmKCJcbiIpOwp9CgovLyBUcmFuc3Bvc2UgYW5kIHByaW50IHRoZSBtYXRyaXgKdHJhbnNwb3NlTWF0cml4KG1hdHJpeCwgcm93LCBjb2x1bW4pOwoKcmV0dXJuIDA7Cn0=
Output:
Enter the number of rows: 2
Enter the number of columns: 3
Enter the elements of the matrix:
100 200 300 400 500 600
Original Matrix:
100 200 300
400 500 600Transpose Matrix:
100 400
200 500
300 600
Explanation:
In this sample C code we carry out the transposition of a rectangular matrix. It starts with including the standard input-output library.
- We define constants MAX_ROWS and MAX_COLS to set the maximum size of the matrix.
- Then, we define a function named transposeMatrix, which takes a two-dimensional array representing a matrix and the number of rows and columns as parameters, i.e., rowws and cols.
- Inside the function, we declare a new array, i.e., transpose, to store the transposed matrix, and two integer variables, ii and jj, to represent the indices position of matrix elements. The variables are separated using commas.
- As mentioned in code comments, we then use two nested loops to traverse the original matrix and swap the elements to generate the transposed matrix.
- The outer loop control variable ii determines the column that will be traversed, and the control moves to the inner loop, which determines the row number that is being traversed.
- In the first iteration, the outer loop sets the column to 0, and the inner loop swaps all the elements in that column row-wise. The value of control variables is incremented after each iteration to get the updated value.
- Each element at the indices [jj][ii] is stored in the transpose matrix at the indices [ii][jj].
- Once the transpose is calculated, the function prints it using another set of nested loops.
- Then, inside the main() function, we declare variables are declared for the number of rows, columns, loop counters (i.e., ai and bj), and the array matrix itself.
- Next, we prompt the user to input the number of rows, columns and matrix values (elements) using printf() statements.
- Using the scanf() function with the reference/ address-of operator, we read and store the input values in respective variables.
- We then use another set of nested loops to print the original matrix.
- Next, we call the transposeMatrix function with the original matrix and its dimensions as arguments.
- The function carries out the transpose operation and prints the transpose of the matrix to the console, as shown in the output.
- The program concludes with a return 0 statement in the main() function, indicating the successful execution.
Time Complexity: The code iterates through all elements in the original matrix to calculate the transpose, resulting in a time complexity of O (N * M).
Conclusion
Understanding how to get the transpose of a matrix in C or mathematics is a fundamental concept and is crucial for various applications in computer science, physics, and engineering. The transpose of a matrix is basically the output matrix we get by switching the rows and columns of the original matrix. It is essential to keep the properties and characteristics of matrices in mind when working with matrices, square or rectangular. Delving deeper into the concepts of matrix operations/ matrix transposition process and algorithms is invaluable when manipulating and analyzing data efficiently in real-life programming situations.
Frequently Asked Questions
Q. What is matrix transposition?
Matrix transposition is a fundamental operation in linear algebra where the rows and columns of a matrix are interchanged. Given a matrix A with dimensions m x n, its transpose, denoted as Aᵀ, is obtained by flipping the matrix over its main diagonal, effectively swapping its rows with columns.
The resulting transposed matrix has dimensions n x m. This operation rearranges the matrix elements to create a new matrix, where each element A[i][j] in the original matrix becomes Aᵀ[j][i] in the transposed matrix.
Matrix transposition is widely used in various mathematical and computational applications, such as solving linear systems, matrix multiplication, and data manipulation in fields like signal processing, image analysis, and machine learning. It plays a crucial role in optimizing algorithms and facilitating operations involving matrices.
Q. Can I use pointers for matrix transposition in C?
Yes, you can use pointers for transpose of a matrix in C. Pointers provide a convenient and efficient way to access and manipulate matrix elements. When transposing a matrix using pointers, you would typically use nested loops to iterate through the elements and swap them accordingly.
Here's a simple example of a function to transpose a matrix using pointers in C:
Code:
I2luY2x1ZGUgPHN0ZGlvLmg+Cgp2b2lkIHRyYW5zcG9zZShpbnQgcm93cywgaW50IGNvbHMsIGludCBtYXRyaXhbcm93c11bY29sc10pIHsKZm9yIChpbnQgaSA9IDA7IGkgPCByb3dzOyBpKyspIHsKZm9yIChpbnQgaiA9IGkgKyAxOyBqIDwgY29sczsgaisrKSB7Ci8vIFN3YXAgZWxlbWVudHMgdXNpbmcgcG9pbnRlcnMKaW50IHRlbXAgPSAqKCoobWF0cml4ICsgaSkgKyBqKTsKKigqKG1hdHJpeCArIGkpICsgaikgPSAqKCoobWF0cml4ICsgaikgKyBpKTsKKigqKG1hdHJpeCArIGopICsgaSkgPSB0ZW1wOwp9Cn0KfQoKaW50IG1haW4oKSB7CmludCByb3dzID0gMywgY29scyA9IDM7CmludCBtYXRyaXhbM11bM10gPSB7CnsxLCAyLCAzfSwKezQsIDUsIDZ9LAp7NywgOCwgOX0KfTsKCi8vIFByaW50IHRoZSBvcmlnaW5hbCBtYXRyaXgKcHJpbnRmKCJPcmlnaW5hbCBNYXRyaXg6XG4iKTsKZm9yIChpbnQgaSA9IDA7IGkgPCByb3dzOyBpKyspIHsKZm9yIChpbnQgaiA9IDA7IGogPCBjb2xzOyBqKyspIHsKcHJpbnRmKCIlZFx0IiwgbWF0cml4W2ldW2pdKTsKfQpwcmludGYoIlxuIik7Cn0KCi8vIFRyYW5zcG9zZSB0aGUgbWF0cml4CnRyYW5zcG9zZShyb3dzLCBjb2xzLCBtYXRyaXgpOwoKLy8gUHJpbnQgdGhlIHRyYW5zcG9zZWQgbWF0cml4CnByaW50ZigiXG5UcmFuc3Bvc2VkIE1hdHJpeDpcbiIpOwpmb3IgKGludCBpID0gMDsgaSA8IGNvbHM7IGkrKykgewpmb3IgKGludCBqID0gMDsgaiA8IHJvd3M7IGorKykgewpwcmludGYoIiVkXHQiLCBtYXRyaXhbaV1bal0pOwp9CnByaW50ZigiXG4iKTsKfQoKcmV0dXJuIDA7Cn0=
Output:
Original Matrix:
1 2 3
4 5 6
7 8 9
Transposed Matrix:
1 4 7
2 5 8
3 6 9
Explanation:
This example demonstrates a simple matrix transposition function using pointers. The transpose function swaps the elements in place by utilizing pointer arithmetic to access and modify matrix elements.
Q. How do I declare a transpose function in C?
To declare a transpose function in C, you need to specify the function prototype in your code. Here's an example of how you can declare a transpose function:
void transpose(int rows, int cols, int matrix[rows][cols]);
This declaration indicates that you are defining a function named transpose, which takes three parameters:
- rows: The number of rows in the matrix.
- cols: The number of columns in the matrix.
- matrix: A 2D array (matrix) where the transpose operation will be performed.
The void return type indicates that the function does not return any value; instead, it performs the matrix transposition in place.
Q. What is the time complexity of matrix transposition?
The time complexity of matrix transposition is O(m * n), where m is the number of rows and n is the number of columns in the matrix. The reason for this time complexity is that, in the worst case, each element of the matrix needs to be visited once to perform the transposition operation.
During matrix transposition, you typically iterate over each row and column of the matrix, swapping the elements along the main diagonal. This requires visiting all m*n elements of the matrix. Since each element is accessed once, the time complexity is proportional to the total number of elements in the matrix, which is m*n. Therefore, the time complexity is O(m * n).
Q. Is there a standard library function for matrix transposition in C?
No, the C standard library, i.e., <stdlib.h>, <string.h>, <math.h>, etc., does not contain a specific function for matrix transposition. So, a user must implement the process of calculating the transpose of a matrix in C programming based on various algorithms. For example, creating functions or routines to get the transpose of a matrix in C which often involves using nested loops to iterate through the matrix and swap elements appropriately.
Q. What is the fastest method to transpose a matrix in C?
The fastness of a technique to get the transpose of a matric in C programming or any other language depends on improving memory access patterns and leveraging hardware capabilities. Optimum strategies often include loop unrolling to reduce loop overhead, blocking or tiling to enhance cache locality, and optimizing loop order based on the matrix storage format (row-major or column-major). Compiler optimization flags and efficient data types, tailored to the matrix characteristics and target platform, can further enhance the performance of techniques used to get the transpose of a matrix in C programs.
Want to enhance your understanding of C concepts? Do read the following articles:
- Ternary (Conditional) Operator In C Explained With Code Examples
- Conditional/ If-Else Statements In C | The Ultimate Guide
- Keywords In C Language | Properties, Usage, Examples & Detailed Explanation!
- 6 Relational Operators In C & Precedence Explained (+Examples)
- Do-While Loop In C Explained With Detailed Code Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment