Hello World Program In C | How-To Write, Compile & Run (+Examples)
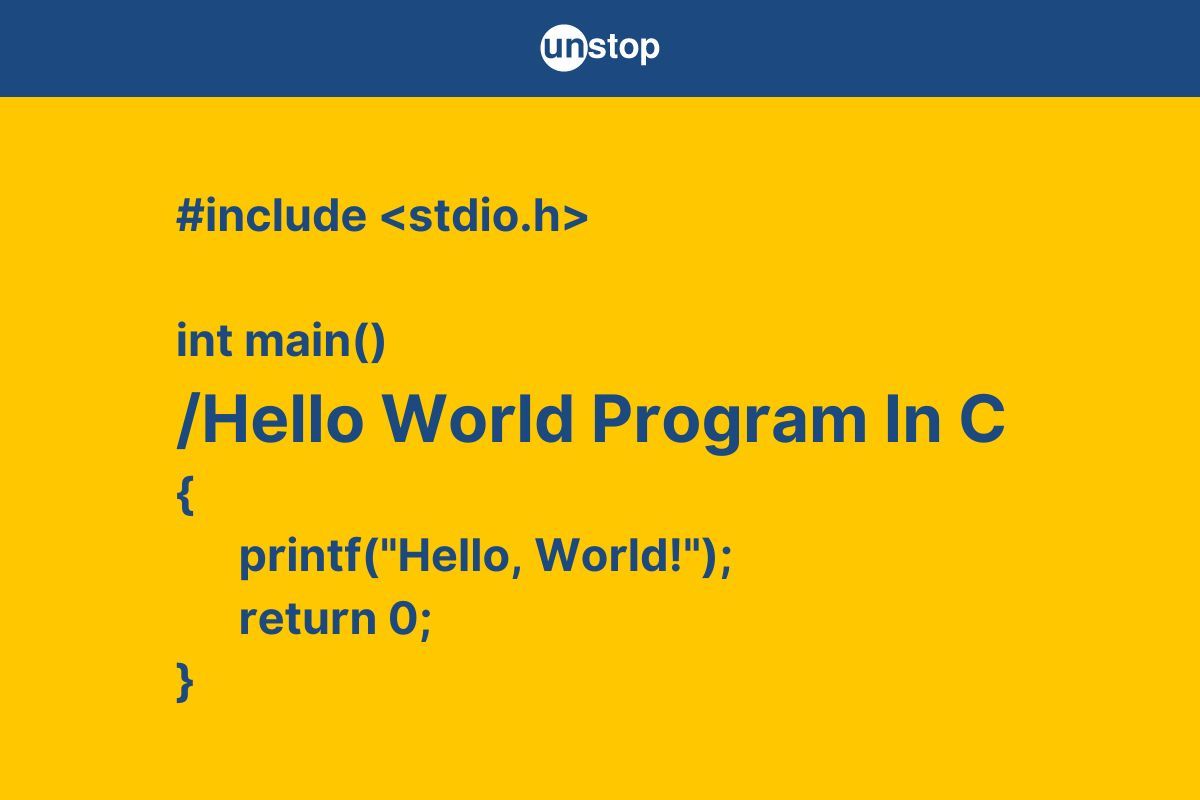
The C language first made its appearance in 1972 and has since been one of the most popular programming languages around. It serves as the foundational basis for many other languages. It is safe to say that attaining mastery over this general-purpose programming language will make it easier for you to learn other languages with similar syntaxes, like Java, Python, C++, and others.
In this article, we will take you through writing the most basic program ever. Yes, we are talking about the Hello World program in C. We have explained every step in detail to help you write and execute the Hello World! introductory program. This is especially useful if you are learning to program for the first time. So let's get started!
How To Write C Program (Hello, World)?
To write robust C programs, you must follow a specific logical and fundamental structure. Let’s examine the basic structure of the "Hello World" program in C language.
1 |
#include<stdio.h> |
Including the header files |
2 |
int main() |
The main method |
3 |
{ |
Opening Curly Brackets |
4 |
printf(“Hello World”); |
Print statement |
5 |
return 0; |
Return statement |
6 |
} |
Closing Curly Brackets |
Here is a detailed description of the various program components:
Header File- Line 1 [#include <stdio.h>]
Every C program starts by including essential header files. These executable files have the .h extension and contain function declarations and macro definitions shared across C programs.
- The hashtag symbol (#) with include keyword forms the pre-processor directive (#include). It tells the preprocessor to include the content of the specified header file in the source file (preprocessor program) before the compilation process.
- In this example, the <stdio.h> header contains declarations for input/ output library functions like printf() and scanf().
- So, the preprocessor copies the preprocessed code from <stdio.h> to our source file.
Main Declaration- Line 2 [int main()]
In a simple program (without any union or structure definitions), the main() function declaration comes next.
- It serves as the entry point for program execution. Meaning, it is where the program begins execution. Think of the beginning of the main() function to be like a door that, when opened, allows for the executable program to enter, and the program execution begins.
- The main() function does not take any parameters, as indicated by the empty parentheses.
- The int keyword specifies that the function returns an integer data type value to the operating system upon completion, typically indicating the program's success or failure.
Body – Line 3 to 6 {}
The next part of the program is the statements/ blocks of code known as the function body. The body of the main function is enclosed in a pair of curly brackets { }. This block of code contains all the executable statements of the function. In this example, the body includes a single printf function. It is important to note that every separate function must start with and terminate with curly brackets.
Statements- Line 4 [printf(“Hello World”);]
Once we enter the body, the statements inside are forwarded to the program compiler as machine-understandable code instructions.
- Within the main function body, we have a printf() statement, which is the standard output function in C. It is used to print text to the console.
- The string "Hello World" is enclosed in double quotes and passed as an argument to the printf() function. The statement ends with a semicolon (;), which marks the end of the instruction in C.
Return Statement- Line 5 [return 0;]
The return statement indicates the end of the function execution and returns a value to the calling process. In the main() function, return 0 signifies successful program termination. The operating system uses this return value to determine the program's exit status, with 0 typically indicating success.
Compiling Your First C Program
The successful execution of a C program depends on using a dedicated compiler that translates C code into executable machine code. A compiler is a software used to convert and run programs written in a specific programming language, such as C. There are two main approaches to installing and running the compilation process for C programs on personal computing devices like desktop computers or laptops:
- Download a complete IDE that includes a C language compiler, such as Turbo C++, Microsoft Visual (Visual Studio Code) C++, or DevC++.
- Alternatively, you can download the C compiler separately, change the program files using any text editor, and then run the C program via the command line.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Steps To Run Hello World Program In C With An IDE (Like Turbo C++)
Here are the steps you must follow to run the Hello World program in C when using an IDE:
- Launch the Turbo C IDE: Open the Turbo C IDE (Integrated Development Environment) and navigate to the File menu, then click New.
- Write the "Hello World" program. Ensure to add the <conio.h> header file and getch(). The getch() function, found in <conio.h>, is a non-standard function mainly used in MS-DOS compilers like Turbo C to wait for a key press before closing the console window.
- Compile the Code: Either click on the Compile menu's Compile option or press Alt + F9, to compile the code.
- Run the Code: Click the Run feature or press Ctrl + F9 to execute the compiled code. Note that the C program is first compiled to create an object code file, which is then executed.
-
View the Output: The program output will be displayed in the output window.
Steps To Run Hello World Program In C Without An IDE
There is an alternative way to run the Hello World program in C without using an IDE. The steps to get this done are as follows:
- Download the GCC Compiler: Visit the GCC website and download the GCC C compiler if you prefer a manual setup process without an IDE.
-
Write and Save the Program: Open any text editor, copy and paste the C program code (here, the "Hello World" program), and save it as helloworld.c. Ensure the file extension is .c. This is similar to how you would save any other output file with a name after downloading and installing the gcc compiler.
- Open the Command Prompt or Terminal: Navigate to the directory where you saved the helloworld.c program file and open the command prompt or console terminal.
- Compile the Code: Use the command gcc helloWorld.c to compile the code. If the code is error-free, it will compile and produce an executable file, typically named a.exe. (Note the file extension .exe is for executable)
The said process involves taking an ordered set of instructions written in C language through various steps to obtain executable source code files. - Run the Executable: Enter ./a.exe in the command prompt to start the program, and your screen will display "Hello, World."
Simple C Program To Display Hello World Message
The "Hello, World!" introductory program is a fundamental lesson that offers valuable insight into understanding programming languages. It serves not only as an entry-level step in learning any language but also as one of the simplest programs. Below is a C program example for printing Hello World.
Code Example:
Output:
Hello, World!
The workings of this example C program are explained in detail in the next section.
How The Hello, World! Program In C Works?
The Hello, World program in the code above is a straightforward, error-free C program. We have used the printf() function to generate an output on a screen, with "Hello, World!" as the central theme.
- Preprocessor Command: The pre-processor directive/ command #include <stdio.h> at the start of the program instructs the compiler to include the standard input/output library file in the code.
- Main Function: The program's entry point is the int main() function, which provides the operating system with an integer type value upon completion.
- Print Statement: Inside the main() function, the printf() function prints a character stream of data to the standard output console. The expression/message is "Hello, World!".
- Output Display: The output device receives a display of everything inside the double quotes (" ").
- Return Statement: Finally, the program is terminated, and the operating system is informed that the program ran successfully with the use of the return 0 statement.
How To Write Hello World Program In C With User-Defined Functions?
Let us see how the "Hello, World!" program in C executes with a user-defined function. These are functions that we can define to serve a specific purpose, and can be reused with ease. So, we can create a function called printMessage() that prints the message on the screen. It will be built into the program below and will be called in the main() function.
Syntax to define a function:
return_type function_name(argumentsIfAny) {
//function_body (code)
}
Here,
- The return_type refers to the data type of the function's return value.
- Function_name refers to the name/ identifier of the function you are defining, which takes parameters represented by the term argumentsIfAny.
- The function_body refers to the lines of code to be executed when the function is called.
Now, let's take a look at a C code example that showcases the Hello World program in C with a user-defined function.
Code Example:
Output:
Hello, World!
Explanation:
In the example C code-
- We first define the printMessage() function, which consists of a printf() statement that displays the string message- Hello, World! to the console.
- Note that the void keyword used when declaring the function indicates the lack of the return value.
- Next, we begin the main() function, the program's entry point, which provides the operating system with an integer value.
- Then, as mentioned in the code comments, we call the printMessage() function. It prints the message on the screen, as shown in the output above.
- Lastly, the program terminates, and the operating system is informed that the program ran successfully with the use of the return 0 statement.
Also read: Functions In C | Uses, Types, Components & More (+Code Examples)
How To Write Hello World Program In C Using Character Variables?
In our previous sections, we have demonstrated how to write and execute the "Hello, World!" program in C using direct string literals and user-defined functions. Now, we will explore an alternative approach by using character variables.
Syntax to declare variables in C:
variable_type variable_name = initial_value;
Here,
- The variable_type and variable_name refer to the data type of the variable and its name, respectively.
- The initial_value refers to the value we assign during variable initialization.
In our previous "Hello, World!" programs, we directly used strings inside the printf() function. In the current C program, we will use the char data type variables to construct the same message.
This approach involves declaring individual character variables and using the character format specifier (%c) in the printf() function to output the complete message. Below is an example that shows how to write the Hello World program in C using character variables.
Code Example:
Output:
Hello world
Explanation:
We begin the sample C program with the preprocessor directive/ command to include the header file for input-output operations.
- Then, we initiate the main() function, which is the program's entry point.
- Inside, we declare 7 unique character variables using the comma operator. Here, a, b, c, d, e, f, and g are assigned characters- H, e, l, o, w, r, and d, respectively.
- These characters combine to create the message "Hello World," which is the theme of the program.
- Next, we use the printf() function with a formatted string to display the required message to the console.
- Inside the printf() statement, the %c format specifiers are the placeholders for character values, which are given after the comma in the formatted literal string.
- As you can see, the required output is printed to the console, and the program terminates with a return 0.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Hello World Program In C To Print Specific Number Of Times
A possible approach to print the message "Hello, World!" repeatedly in a C program is by utilizing looping statements. Loops are control structures/ statements that allow repeated execution of a block of code until a pre-defined condition is met.
There are three types of loops: for loops, while loops, and do-while loops. In this case, we will use the for loop to write a Hello, World! program in C to print a fixed number of times.
For Loop Syntax:
for (initialization; testExpression; updateStatement){
// code statements inside the body of loop
}
Here,
- The for keyword marks the beginning of the loop, where the initialization statement specifies the value from where the loop begins.
- The testExpression refers to the loop condition, which we check for True or False, and the updateStatement specifies how to update/ increment or decrement the loop variable after every iteration.
Given below is a C program sample that shows how to output the Hello, World! message four times.
Code Example:
Output:
Enter the number of times to print the message: 4
Hello, World!
Hello, World!
Hello, World!
Hello, World!
Explanation:
In the C code sample above-
- We declare two integer variables, i.e., i and n, inside the main() function.
- Next, we prompt the user to give the number of times they want to print the message using the printf() function.
- We use the scanf() function to read this input and the address-of operator (&) to store the value in variable n. In this example, the input we are taking is 4.
- Then, we initiate a for loop containing a printf() statement that displays the message- Hello, World! in every iteration.
- The loop begins with the control variable i set to 0 after which the program checks the loop condition, i less than n (i<n).
- Since loop condition (0<4) is true, the loop body is executed and it prints the message to the console.
- After that, the value of the loop control variable is incremented by 1 (as per the update condition) and the loop begins again.
- Note that the newline escape sequence (\n) inside the printf() statement shifts the cursor to the next line after every iteration.
- After the first iteration, i becomes 1, and the process of condition checking, then printing, and then updation continues until i becomes more than n.
- The loop terminates after four iterations, and the program ends with the statement return 0.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
The Hello World Program In C To Print Indefinitely
We've explored how loops can be used to repeat a task, such as printing a message, as long as a specified condition holds true. In the previous example, a for loop was used to print the "Hello, World!" message four times. But what if you want the message to be printed without stopping?
The solution is to use an infinite loop. An infinite loop continuously repeats a block of code until it is terminated by an external action. In this section, we'll use an infinite while loop to make the "Hello, World!" program in C print the message indefinitely.
While Loop Syntax:
while(condition) {
statement(s);
}
Here,
- The while keyword marks the beginning of the loop, while the statements inside curly brackets are the code/ loop body.
- The condition here will always remain true.
Code Example:
Output:
Hello, World!
Hello, World!
Hello, World!
Hello, World!
...... (indefinitely)
Explanation:
In the sample C code-
- We include the essential standard input-output library and then begin the main() function.
- Inside, we create an infinite while loop where the test condition is 1, which is always true.
- Since the condition is always true, the printf command indefinitely displays the message to the console, each one in a new line.
- Note that the loop will terminate only when someone stops it externally, for example, by pressing (Ctrl + C).
- There is a return statement at the end of the main() function, but it is never reached since the loop runs indefinitely.
Check out this amazing online course to become the best version of the professional software developer you can be.
Conclusion
C is a foundational language in the world of programming and is often considered the "mother" of modern programming languages. Learning how to write and execute the "Hello, World!" program in C is an excellent way to start honing your programming skills. This essential program can be executed in multiple ways: using an IDE, without an IDE, with character variables, and with a user-defined function. Additionally, the "Hello, World!" program in C can be run repeatedly or indefinitely using loops, showcasing the versatility and power of the C programming language.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. How to print the Hello World program in C?
The most straightforward way to print the message "Hello, World!" in C is by using the printf() function. Alternatively, you can also create and employ a user-defined function to achieve the same result. Below is a simple C program showcasing the use of printf() to write the Hello, World! program.
Code Example:
Output:
Hello, World!
Q. How can you declare and initialize variables in C?
Declaring a variable involves creating or defining a variable by indicating to the compiler the variable's name and data type. Assignment of a value to the variable is not required during declaration. Initialization, however, involves assigning a value to the variable.
There are two primary ways to declare and initialize a variable:
- Separately declaring and initializing the variable.
- Assigning a value to the variable at the time of declaration.
When declaring a variable without initialization, you specify the type and name, then assign a value using the simple assignment operator.
The syntax for initializing and declaring variables in C is as follows:
// Declaration: type variable_name;
int age;
// Initialization: variable_name = value;
age = 16;
// Declaration and initialization combined: type variable_name = value;
float pi = 3.14159;
In the snippet above, we first declare a variable age and then assign it a value in the next line. The first step is the declaration, while the second step is the initialization. In the final line, we create a variable pi of type float and initialize it simultaneously with a value of 3.14159.
Q. How to write an if-else statement in C?
In C programming, the if-else statement is a fundamental decision-making structure to help write powerful programs. It allows the execution of certain blocks of code based on if specific conditions are met. Generally, if the condition is true, the code within the if block is executed; otherwise, the code within the else block is executed.
Syntax Of If-Else Statement In C:
if (condition) {
// code executed when the condition is true
}else {
// code executed when the condition is false
}
Q. How to write for loop in C?
The for loop is a control structure that initializes a condition, tests it, and performs statements based on the condition before updating the data.
Syntax of for loop in C-
for(initialization; condition; increment){
// multiple statements
}
Q. How to print the Hello World in C 5 times?
You can write a Hello, World! program in C to print the message five times by using a for loop and the printf command. Below is an example of a simple C program that demonstrates this:
Code Example:
Output:
Hello World
Hello World
Hello World
Hello World
Hello World
Q. What are the 4 types of functions in C?
In C programming, functions can be classified into four primary types:
- Built-in functions- These simple functions come built-in with the libraries of the programming language. For example, the standard output function printf().
- User-defined functions- These are custom functions created by the user to perform specific tasks.
- Recursive functions- Functions that call themselves recursively to perform iterative operations.
- Function pointers- These are pointer variables that point to functions, enabling indirect function calls.
Q. What is the main function in C programs?
The execution of a sample program code in C begins with the main() function. It is commonly referred to as the entry point of a program. Every program written in C must use this predefined function. The main() function starts the program's execution and returns an integer value to the operating system.
Had fun writing your own Hello World program in C? We are sure you did. Here are a few more interesting topics you must explore:
- Continue Statement In C | Working & Use Explained (+Code Examples)
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
- C Program To Find Roots Of Quadratic Equation With Detailed Examples
- Decimal To Binary In C | 11 Methods (Examples + Complexity Analysis)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment