Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
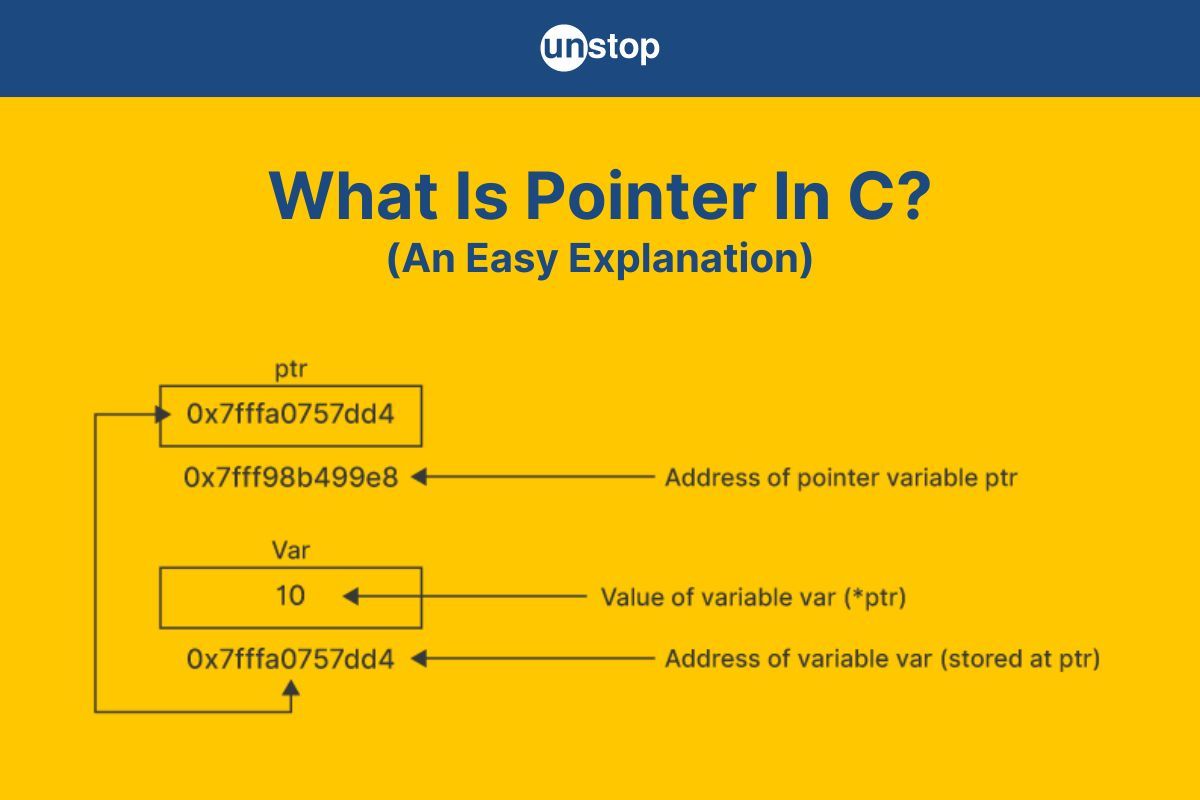
In this article, we will delve into the world of pointers in C covering the basics, their types, uses, advantages, and disadvantages and more with the help of proper examples. Pointers are a fundamental component of the C programming language that enables us to deal with memory addresses of various variables, functions, and even other pointers. And perform data manipulation of these elements. Whether it's dealing with integers, characters, arrays, or functions, pointers prove to be versatile tools.
Mastery of pointers is crucial for any C programmer, as they allow for efficient memory management, dynamic data structures, and low-level access to system resources. Understanding pointers allows us to perform low-level memory access, dynamic memory allocation, and other powerful functionalities in C. Dynamic memory allocation, known as heap memory, becomes possible through pointers, streamlining our code and enabling the return of multiple values from functions.
What Are Pointers In C?
Pointers in C language are special variables that store memory addresses of another variable. Instead of directly containing a value, a pointer allows indirect access to the data stored at a specific location in the computer's memory. And manipulation of these pointers allows us to efficiently manage memory, facilitate dynamic data structures, and optimally run our C programs.
For example, imagine a library with books placed on shelves where each book has a unique identifier. But rather than carrying the entire book, you can use a card (pointer) that holds the location (memory address) of a specific book (variable). This card allows you to indirectly reference and interact with the book without carrying it around. Similarly, pointers in C provide a means to efficiently access and manipulate data indirectly by referencing its memory address.
- When a variable is declared in C, the compiler reserves space in memory to hold its value. We can access the memory address of a variable using the address-of operator (&).
- Pointer variables in C are created using the indirection operator (*). And we can retrieve the value stored at that memory location with the dererencing/ indirection/ value-of operator (*).
- The size of pointers is independent of the data type they point to and depends on the system architecture.
- Using pointers offers faster execution time and helps save memory space in C programming.
- Dynamic memory allocation in the heap provides greater flexibility and efficient memory management for handling variables.
What Is Memory Address In C?
In the C programming language, every variable we define is allocated a specific memory location to store its value. To access this memory location, we use address-of operator, denoted by the ampersand symbol (&). For example, if we have a variable named var in our program, then the memory address of the variable is provided by &var.
The memory addresses are particularly useful when working with functions like scanf(), where we need to read user input and store it in the memory location associated with a variable. For instance, scanf("%d", &var) reads an integer input and stores it in the memory address of the variable var.
The following diagram illustrates the relationship between computers' memory address and content and the variable's name, type, and value used by the programmers.
How To Declare Pointers In C?
Pointers in C follow a syntax similar to variable declarations, but they are denoted by the * (asterisk) symbol. A pointer serves as an indirection, allowing us to access the value stored at the memory location it points to.
Syntax For Pointer Declaration In C:
datatype *ptr;
Here,
- The term ptr represents the name given to the pointer, and the asterisk symbol indicates that it is a pointer.
- The type of data the pointer is pointing to is given by the term datatype. It can also be called the data type of the pointer itself.
Pointers can be defined for various data types, including variables, functions, structures, and more. For instance, to declare a pointer of integer type, we write:
int* p;
In the above statement, a pointer p is defined as an integer-type pointer. There are alternate ways to declare pointers, such as:
int *p1;
int * p2;
Both of these statements achieve the same result as declaring a pointer p1 and a pointer p2 of integer type. If you want to declare multiple pointer variables in a single statement, you need to include the asterisk (*) before each variable name to indicate that they are all pointers. For example:
int *p, *q, *r;
This line declares three pointer variables named p, q, and r, all capable of holding memory addresses of integer data. By understanding pointers in C and their diverse applications, we can unlock powerful memory manipulation and data handling capabilities in our C programs.
How To Initialize Pointers In C?
When we create pointers in C, it doesn't automatically point to any specific memory location. Pointer initialization is the crucial step wherein we assign an initial memory address (hence the value of a variable) to the pointer variable. Usually, we use the address-of (&) operator to retrieve the memory address of a variable and then store it in the pointer in C. Failing to initialize pointers properly can lead to unpredictable and potentially harmful outcomes in a C program.
Let's examine an example to understand this concept better:
int number = 88; // An integer variable with a value
int *pNumber; // Declare a pointer variable called pNumber, which points to an integer
pNumber = &number; // Assign the address of the variable number to the pointer pNumber
int *pAnother = &number; // Declare another integer pointer and initialize it with the address of the variable number
In this illustration, as mentioned in code comments, the integer variable number holds the value 88 and resides at memory address 0x22ccec. The expression &number returns this memory address (0x22ccec), which is then assigned as the initial value to the pointer variable pNumber.
It is essential to note that the address-of operator (&) can only be used on the right-hand side (RHS) of an expression. Properly initialized pointers play a crucial role in accessing and manipulating memory locations, allowing us to efficiently work with complex data structures and dynamic memory allocation in C programming.
We can streamline the process of declaring and initializing pointers in C programs in a single step, known as pointer definition. In this method, the pointer is declared and assigned an initial value simultaneously. For instance:
int *ptr = &var;
In this example, we declare a pointer variable ptr of type int, and we initialize it with the memory address of the variable var.
However, it is essential to emphasize the significance of initializing pointers before usage. Failing to initialize pointers in C properly can result in various errors and unexpected behaviour. Thus, assigning an initial value to pointers is highly recommended to ensure they are pointing to valid memory locations before any operations are performed on them. Proper initialization helps avoid potential issues and ensures the safe and reliable operation of the program.
How To Dereference Pointers In C?
Once we have a pointer that holds the memory address of a specific variable, we can access or modify the value stored at that location by dereferencing the pointer. Dereferencing is the process of obtaining the actual value stored in the memory address pointed to by the pointers in C programs. To dereference a pointer, we use the dereferencing/ indirection operator (*), which we also use during the pointer declaration.
- Dereferencing enables us to refer directly to the value stored at the memory location rather than the memory address itself.
- A useful analogy is the concept of arrays, where we access elements using the brackets operator, like [0], to access the first item.
- Since arrays are essentially pointers, accessing array elements is, in fact, a form of dereferencing.
Now that we know how to declare, initialize and dereference pointers in C to manipulate the data being pointed to, let's look at a C code example that showcases the implementation of all three concepts.
Code Example:
Output:
Address of p variable is 0x7ffcebafa2bc
Value of p variable is 50
Note that the address of the variable may differ when you run the code example above.
Explanation:
We begin the simple C program by including the standard input-output header file <stdio.h> to use functions like printf and scanf.
- We then initiate the main() function, where the program execution begins.
- Inside main(), we declare an integer variable called a number and assign a value of 50 to it. Imagine labelling a box as number and placing a value of 50 inside it.
- Then, we declare an integer type pointer variable p using the indirection operator, i.e., int *p. Think of this as a small piece of paper named p, which can only hold the location of a box (memory address). But right now, it's blank, so we don't know where it points.
- Next, we initialize the pointer and assign the location of the number variable to it, using the address of operator, i.e., p = &number. This can be thought of as writing the address/ location of the box number on the paper p. Now, p knows where to find the number. We can say p is pointing at the box number.
- After that, we use a printf() statement to display the address of number variable to the console.
- Inside the formatted string, we have the %p format specifier indicating that a pointer value will go there and a newline escape sequence, which shifts the cursor to the next line.
- We again use a printf() statement to access and print the value being pointed to by the pointer. For this, we use the dereference operator to insert the value in the formatted string in the output.
- Think of this step like this- we want to know what's inside the box without directly opening it. So, we read the value from the paper p, and following the address written on it, we go to the box number, open it, and find the value 50 inside.
It is important to note that the asterisk sign (*) can be a bit confusing because it serves two different purposes in our code:
- When used in the declaration (int* ptr), it creates a pointer variable.
- When not used in the declaration, it acts as the dereference operator, allowing us to access the value at the memory address pointed to by the pointer. Understanding this distinction is crucial for correctly working with pointers in C programming.
Use Of Pointers In C
We know how to declare, initialize and dereference pointers in C. To use pointers in C, we must first declare a pointer variable using the indirection operator (*) along with the data type (e.g., int *ptr;). We then initialize the pointer by assigning the address of a variable using the address-of operator & (e.g., ptr = &variable;).
Now, let’s see how we can use pointers in C programs to access and alter the value being pointer to by it.
Getting The Value Using Pointers In C
When working with pointers in C, obtaining the value stored at the memory location they point to is achieved through a process called dereferencing. The dereference operator (*) is used to access the value at the address held by the pointer. Below is a sample C program that shows the code implementation of the same.
Code Example:
Output:
Value of number: 42
Explanation:
We begin the C example by including the standard input-output library.
- In the main() function, we declare and initialize an integer variable named number with the value 42.
- Additionally, we declare an integer pointer named ptr and initialize it with the memory address of the number variable.
- The pointer initialization involves assigning the address of the number variable to the pointer using the address-of operator (&).
- Next, we dereference the pointer using the dereferencing cooperator, i.e., int value = *ptr, extracting the value stored at the memory location pointed to by ptr. This obtained value is then stored in the value variable.
- Finally, we print the value of number using the printf() function, displaying the result along with a descriptive message.
- The return 0 statement at the end signals the successful execution of our program to the operating system.
Changing The Value Using Pointers In C
Pointers allow us to indirectly modify the value stored at a specific memory location. This is done by dereferencing the pointer and assigning a new value to it.
- To change the value, we use the dereference operator (*) on the left side of an assignment.
- The new value is assigned to the memory location pointed to by the pointer.
Code Example:
Output:
Modified value of number: 99
Explanation:
In the C program-
- We begin by declaring and initializing an integer variable named number with the value 42, inside the main() function.
- Additionally, we declare an integer pointer named ptr and initialize it with the memory address of the number variable, using the address of operator (&).
- Then, we use the pointer to change the value of number and reassign value 99 to it, i.e., *ptr = 99;. This operation involves dereferencing the pointer, allowing us to modify the value stored at the memory location pointed to by ptr. As a result, the value of number is updated to 99.
- Finally, we print the modified value of number using the printf() function, displaying the result along with a descriptive message.
- The program terminates successfully with a return 0 statement.
Types Of Pointers In C Language
In C, pointers are versatile and can be classified into different types based on the type of data they point to and their usage/behaviour. We will discuss a variety of pointer types in C in the sections ahead.
First, we'll look at six common types of pointers that have special behaviour in a C program. These include:
- Null Pointers In C
- Void Pointers In C
- Wild Pointers In C
- Dangling Pointers In C
- Pointer To Pointer/ Double Pointers In C
- Constant Pointers In C
- Pointer To Constant
What Are Null Pointers In C?
Null pointers in C are pointers that do not point to any memory location. We can create them by assigning a NULL value to the pointer. This can be done using an integer constant expression with the value 0 or by casting such an expression to type void *.
- A null pointer constant, when converted to a pointer type, results in a null pointer, which is guaranteed to compare unequal to a pointer to any object or function.
- Using NULL pointers is a good practice when we want to initialize a pointer without an exact address to assign to it.
- It also helps to identify pointers that are not intended to point to valid data, helping prevent dereferencing errors. Example: Check if a pointer is NULL before using it.
- This ensures that the pointer doesn't accidentally point to a random memory location.
- In C, NULL is a macro constant with a value of zero, defined in various header files like <stdio.h>, <stdlib.h>, and <alloc.h>.
Syntax Of Null Pointers In C:
data_typ *pointer_name = NULL;
or
pointer_name = NULL
By assigning NULL to a pointer variable at the time of declaration, we clearly indicate that the pointer is not currently in use. The convention in most libraries is to set the value of a null pointer to 0 (zero).
Code Example:
Output:
The pointer does not point to anything.
Explanation:
In this C code snippet-
- We first declare an integer pointer named ptr and initialize it to NULL, indicating that it currently does not point to any specific memory address.
- Following this, we use an if-statement to check if the pointer is NULL. If the condition is true, the if-clock is executed, and the printf() statement displays a message stating that the pointer does not point to anything.
- If the condition is false, i.e., the pointer is not NULL, we enter the else block. Inside this block, we dereference the pointer to access and print the value being pointed to.
- It's important to note that dereferencing should be done cautiously only if the pointer holds a valid memory address. Since we've checked for NULL earlier, we can safely assume that the pointer has a valid value.
- Therefore, we proceed to print the value pointed to by the pointer using the printf function.
- Finally, the program completes execution with return 0.
Complexity: The space complexity and time complexity of the code is O(1).
Void Pointers In C
Void pointers in C are unique pointer variables that don't have an associated data type. They are commonly known as generic pointers since they can point to any type of data and be typecasted accordingly.
- The process of creating void pointers in C is straightforward, that is, simply by using the void keyword instead of data type.
- Void pointers in C play a crucial role in implementing generic functions, such as the compare function used in qsort(), allowing for flexible sorting operations.
- However, to display the value stored in this pointer, it is necessary to typecast the pointer into a required type.
- Void pointers offer flexibility and versatility, enabling developers to handle different data types efficiently and facilitating polymorphism in C programming.
Syntax For Void Pointers In C:
void * pointer_name;
Here,
- The asterisk (*) indicates that the variable is a pointer, and the void keyword indicates that it does not have a specific type.
- its name is given by the term pointer_name in the syntax.
Code Example:
Output:
10
Explanation:
- We begin the C code example by declaring an integer variable a and initializing it with the value 10, inside the main() function.
- Next, we declare a void pointer named ptr and initialize it with the memory address of the variable a using the address-of operator (&).
- Since ptr is a void pointer, it can point to any data type. However, to access the value stored at the memory location pointed to by ptr, we need to cast it to the appropriate type.
- In this case, we cast ptr to an integer pointer using the casting operator, i.e., (int*)ptr. This allows the compiler to treat ptr as a pointer to an integer.
- After that, we dereference the pointer to obtain the value stored at the memory location it points to, i.e., *(int*)ptr.
- Finally, we print this value using the printf() function, with the %d format specifier indicating that we're printing an integer.
Complexity: The space complexity and time complexity of the code is O(1).
Read this to learn more- Void Pointer In C | Referencing, Dereferencing & More (+Examples)
Wild Pointers In C
Wild Pointers in C are pointers that have not been assigned any valid memory address, resulting in their unpredictable behaviour. These uninitialized pointers can cause issues in our programs, leading to crashes or unexpected behaviour.
Since they point to arbitrary memory locations, they lack the necessary information to access valid data, posing a significant risk to the stability and reliability of our code. It is essential to initialize pointers properly to avoid such wild pointer scenarios and ensure the integrity of our programs.
Syntax:
int *wildPtr; // Declaration of a wild pointer (not initialized)
Code Example:
Output:
Segmentation fault
Explanation:
In the example C code-
- In the main() function, we declare an uninitialized pointer named wildPtr, often referred to as a wild pointer.
- Next, we dynamically allocate memory using the malloc() function and assign the address of the allocated memory to wildPtr. This provides a valid memory location for the pointer.
- Following the allocation, we store the value 42 in the allocated memory using the dereferencing operator, i.e., *wildPtr = 42.
- Then, we print the value stored at the memory location pointed to by wildPtr, using a printf() statement and dereferencing operator.
- We then deallocate the dynamically allocated memory using the free() function. However, the pointer still contains the address of the deallocated memory, leading to a dangling pointer situation.
- Finally, attempting to access the value pointed to by the wildPtr after deallocation results in undefined behaviour.
- The return 0 statement indicates the successful execution of our program.
Complexity: The space complexity and time complexity of the code is O(1).
Dangling Pointers In C
A dangling pointer in C refers to a pointer that points to a memory location that has been deallocated or freed. Accessing or modifying the value through a dangling pointer can lead to undefined behaviour, as the memory it points to is no longer guaranteed to be valid.
- Dangling pointers in C often arise from errors in memory management, such as accessing a pointer after the memory it points to has been freed.
- Handling dangling pointers is crucial to ensure the stability and reliability of our programs.
- It is essential to be cautious when dealing with pointers and avoid using them after the memory they are pointing to has been deallocated or when their referenced variables go out of scope.
Syntax:
int *danglingPtr; // Declaration of a dangling pointer
Example:
Output:
No output is explicitly provided due to the program exhibiting undefined behavior
Explanation:
In this example C code snippet-
- We first define a function getDanglingPointer() that returns a pointer. Inside-
- We declare an integer variable localValue and initialize it with the value 123.
- Then, we create and initialize a pointer ptr with the memory address of the localValue variable.
- It's important to note that localValue is a local variable, meaning it exists only within the scope of the getDanglingPointer() function.
- The function returns the pointer ptr, which points to the local variable localValue. However, returning a pointer to a local variable leads to the creation of a dangling pointer.
- In the main() function, we call getDanglingPointer() and assign the returned pointer to danglingPtr.
- This pointer now points to a deallocated memory location, which results in undefined behaviour when accessed or modified.
- The return 0 statement at the end of the main() function indicates the successful execution of the program.
Complexity: The space complexity and time complexity of the code is O(1).
Read this to lean more- Dangling Pointer In C Language Demystified With Code Explanations
Double Pointers Or Pointer To Pointers In C
In C language, we have the concept of double-pointers, also known as pointers-to-pointers. Instead of pointing directly to a data value, these pointers point to the memory address of another pointer in C program. This creates a form of multiple indirection or a chain of pointers.
- When we define a pointer to a pointer, the first pointer holds the address of the second pointer, which in turn points to the location containing the actual value.
- This allows for more complex memory addressing and manipulation.
- To declare a variable that is a pointer to a pointer, we simply place an additional asterisk in front of its name.
Syntax For Pointer-to-Pointer In C:
datatype ** pointer_name;
Here,
- The double asterisk sign (**) indicates that the pointer variable whose name is given by pointer_name is a pointer pointing to another pointer.
- The term datatype refers to the type of pointer it is pointing to, which in turn reflects the data type of the value stored.
With this concept, we can create multi-level pointers with any number of levels, such as ***ptr3, ****ptr4, ******ptr5, and so on. These multi-level pointers provide increased flexibility and allow us to access and modify memory locations at various levels of indirection.
Code Example:
Output:
Value using double pointer: 42
Modified value using single pointer: 99
Explanation:
In this C code above-
- Inside the main() function, we first declare and initialize an integer variable, num with the value 42.
- Then, we declare and initialize an integer pointer variable ptr1, pointing to the location of num variable.
- Following that, we create a double pointer ptr2 (pointer to an integer pointer) and initialize it with the memory address of ptr1.
- Using a printf() statement, we access and print the value pointer to by ptr2 (which is, in turn, the value pointed to by ptr1, i.e., the value of num).
- Next, we modify the value of the num variable through the double pointer ptr2, i.e., **ptr= 92.
- After modifying the value through the double pointer, the program prints the modified value of value using the original pointer ptr1.
- The modified value turns out to be 99, which shows that we can use both double and single pointers to access and modify values of variables stored at respective memory locations.
- Finally, the program returns 0, indicating successful completion to the operating system.
Complexity: Space complexity and time complexity of the code is O(1)
Constant Pointers In C
A constant pointer is a special type of pointer in C that points to a memory location whose value cannot be changed through that pointer.
- This means we cannot modify the value stored at the memory address pointed to by a constant pointer.
- However, the constant pointer itself can be reassigned to point to different memory addresses, allowing for flexibility in memory allocation.
These constant pointers are beneficial when we want the address of a variable to remain fixed or when we don't want to allocate any other memory location to a pointer. Like a constant variable in C, a constant pointer will keep pointing to the same memory location it is initially assigned to.
Note: Constant pointers can change the value of the variable they point to but cannot change the address they hold. It is important to initialize the constant pointer during the declaration itself, unlike a normal pointer that can be left uninitialized.
Syntax Of Constant Pointers In C:
const data_type *pointer_variable;
//Example: int *const constPtrX =constX;
- The const keyword indicates that the pointer is constant and is pointing to a variable whose type is given by the term data_type.
- The term pointer_variable represents the name of the constant pointer variable.
Code Example:
Output:
Value of num using constant pointer: 42
Explanation:
In the sample C code-
- We first declare and initialize an integer variable num with the value 42 inside the main function.
- Then, we declare a constant integer pointer ptr and initialize with the memory address of the num variable. The const keyword indicates that the value pointed to by ptr should not be modified through this pointer.
- We then proceed to access the value of value through the constant pointer ptr. The value pointed to by ptr (which is the value of num) is accessed and printed using a printf() statement.
- However, as mentioned in the comments, if we attempt to modify the value through the constant pointer it would result in a compilation error. This is because the const qualifier indicates that the value pointed to by ptr is read-only, and attempting to modify it leads to a violation of const-correctness.
- Finally, the program returns 0, indicating successful completion to the operating system.
- In summary, this code snippet demonstrates the use of a constant pointer, which restricts the modification of the pointed-to value.
Pointer To Constant In C
A pointer to constant is a specialized type of pointer in C that points to a memory location whose value is treated as constant.
- Through this pointer, you cannot modify the value of the variable it points to.
- However, you have the flexibility to change the pointer itself to point to different memory addresses.
- The key distinction is that the pointer will always point to an address where the value cannot be changed. While you cannot assign values to the pointer to constant, you can increment or decrement its value.
The pointer is designed to point to constant data objects, ensuring that the values at those addresses remain unchanged. Unlike constant pointers in C, it is not mandatory to initialize the value of a pointer to constant at the time of declaration, offering some convenience.
Syntax of Pointer To Constant In C:
data_type const *pointer_variable;
Here,
- data_type: The data type of the constant variable that the pointer points to.
- pointer_variable: The name of the pointer variable.
Code Example:
Output:
Value at index 0: 10
Value at index 1: 20
Value at index 2: 30
Value at index 3: 40
Value at index 4: 50
Explanation:
In this C code example-
- We first declare and initialize an array of constant integers, i.e., numbers with values {10, 20, 30, 40, 50} inside the main() function.
- Then, we declare and initiate a pointer to a constant integer ptr with the memory address of the first element of the array numbers.
- We then use a for loop to access the values of the array through the pointer ptr using pointer arithmetic inside the loop.
- Inside the for loop, the printf() statement accesses and prints each value at a given index, starting from i=0 and ptr+i thereafter.
- The value of index i is increcremented after every iteration and the loop runs until i<5.
- In the code comments, we have shows that any attempt to modify the value through the constant pointer (by uncommenting the line *(ptr + 2) = 99;), will result in a compilation error.
- This is because the const qualifier indicates that the values pointed to by ptr are read-only.
Some other types of pointers include the near pointer, far pointer, and huge pointers in C. While they are not commonly used in modern programming, it will be good to have a basic idea of what they are.
Near Pointers In C
A near pointer in C is a special type of pointer that grants access to a limited amount of small data, usually around 64kb.
- It has the capability to utilize bit addresses, typically up to 16 bits, within a specific section of memory.
- It's like having a small window to peek into a tiny portion of the computer's memory.
- Each address in this memory section represents a single byte, which amounts to eight bits of storage, making it suitable for handling compact data.
For more extensive data, the information can be cleverly stored in a sequence of addresses, like pieces of a jigsaw puzzle, allowing us to assemble and retrieve the larger picture when needed.
Far Pointers In C
The far pointer in C, unlike its near counterpart, stores memory addresses using two 16-bit registers, enabling it to access memory beyond the current segment. The compiler assigns a segment register to hold the segment address and another register to store the offset within the current segment. By adding the offset to the shifted segment address, the far pointer obtains the actual address.
- It's important to note that the segment part cannot be directly modified in far pointers, as incrementing or decrementing only affects the offset and not the segment address. As a result, the size of a far pointer is 4 bytes.
- However, a limitation of far pointers is that different far pointers may have different values but still point to the same address in memory. This aspect renders pointer comparison on far pointers futile.
Far pointers were once a solution to work with larger memory spaces, but modern programming practices and advances in memory management have made them less relevant today. Consequently, using far pointers in contemporary programming is rare, as they have largely become a vestige of the past. Nevertheless, exploring the historical context of far pointers in C can be intriguing for those interested in the evolution of programming techniques.
Huge pointers In C
The huge pointer in C, like the far pointer, utilizes two separate registers to store memory addresses. It possesses unique characteristics that distinguish it from other pointer types:
- Unlike the far pointer, the offset and segment address can be modified in a huge pointer. This flexibility allows us to traverse from one segment to another using a huge pointer, making it a powerful tool for navigating large memory spaces.
- Huge pointers always compare the absolute addresses, enabling relational operations to be performed on them. This feature offers greater versatility when dealing with memory addresses.
- The size of a huge pointer is 4 bytes, making it relatively compact and efficient in memory usage.
Note: However, it's essential to mention that the concept of near, far, and huge pointers is not commonly used in modern compilers. It's like a rare gem from the past, cherished by old-time programmers but seldom encountered in the present-day coding landscape.
As technology advances, these special pointers have gradually faded into obscurity, and most modern compilers don't recognize or support them. But just like reminiscing about vintage technology, the idea of near pointers brings a touch of nostalgia for those who once worked with them in a bygone era of computing.
Other Types Of Pointers In C
Besides the types of pointers in C we have discussed above, the are a few other types that you must be familiar with. Let's have a look at these in the sections ahead.
Integer Pointers In C
As the name suggests, an integer pointer in C is a pointer variable that holds the memory address of an integer variable. It allows for efficient manipulation and indirect access to the integer's value through memory addressing. The syntax for declaring an integer pointer is the same as a regular pointer, with the data type being int.
int *ptr; // Declaration of an integer pointer
Code Example:
Output:
The value of a = 10
The value stored at ptr = 10
The address of a = 0x7ffc335d350c
The pointer ptr points to the address = 0x7ffc335d350c
The address of pointer ptr = 0x7ffc335d3510
Explanation:
In this C code sample-
- Inside the main() function, we declare an integer variable a and initialize with the value 10. We also declare an integer pointer ptr.
- Next, we use the address-of operator (&) to assign the address of a to ptr.
- We then use a set of printf() statements to display various values and addresses as follows:
- First, we print the value of a variable with direct access inside a formatted string. Then, use the pointer ptr to access the same and print it to the console.
- After that, we print the address/ memory location of the a variable using the address of operator.
- Again, we print the same address, i.e., location of the variable a, but this time we access it using the pointer ptr.
- Lastly, we print the address of the ptr variable itself using the address-of operator.
- Finally, the program returns 0, indicating successful completion to the operating system.
Complexity: The space and time complexity is O(1).
Structure Pointers In C
A structure pointer, also known as a pointer to a structure, is a fascinating concept in C programming. It refers to a special kind of pointer that points to the memory block containing a structure. This powerful feature allows us to create complex data structures like linked lists, trees, graphs, and more.
- When we work with structures, we often need to access and manipulate their values efficiently.
- This is where a structure pointer in C language can some to the rescue. It reveals the address of a structure in memory, making it easier to work with the entire structure.
- When a pointer points to a structure, it's like having a map that guides us directly to the location of the structure variable.
- This enables us to access and modify the structure members with ease.
- We can even pass the entire structure to functions using structure pointers, making it a powerful tool for managing data efficiently.
Syntax Of Structure Pointers In C:
struct StructName *ptrToStruct;
Here,
- The struct keyword indicates that the data type being pointed to by the pointer ptrToStruct is a structure.
- The term StructName refers to the name given to the structure being pointed to.
Code Example:
Output:
Name: Amitav Ghosh
Age: 67
Gender: M
Explanation:
In the code above-
- We first define a structure named Person to represent a person's information. It has three members, i.e., name (an array of characters), age (an integer), and gender (a character).
- Inside the main() function, we create a structure variable person1 of type Person.
- Next, we use the dot operator (.) to initialize the members of the structure variable.
- We assign values 67 and M to the age and gender fields, respectively.
- Then, we use the strcpy() function to copy the string value- Amitav Ghosh, to the name field/character array.
- We then declare a pointer to a structure of type Person named ptrToStruct. And assign the address of person1 to the structure pointer ptrToStruct using address of operator.
- After that, we use a set of printf() statements to access the structure members and print their values to the console. Here, we use the structure pointer and the 'this'/ arrow pointer (->) to gain access to member values.
- Finally, the program terminates and returns 0 to the operating system.
Complexity: The space and time complexity is O(1).
Array Pointers In C
Pointers and arrays share a close relationship in C programming. In fact, the array name itself acts as a pointer to its first element, and this concept is known as a pointer to arrays.
- Pointers to an array store the memory block's address where the array variable is located.
- When we dereference a pointer expression, we access the value pointed to by that pointer. I
- n the case of a pointer to an array, the dereferencing should give us the array itself, and the array name denotes the base address.
So, when we dereference a pointer to an array, we obtain the base address of the array it points to. Take the following array declaration, for example:
int arr[5] = { 1, 2, 3, 4, 5 };
Suppose the base address of arr is 1000, and each integer requires two bytes of memory. The five elements of the array will be stored in memory as follows:
- The variable arr contains the base address 1000, which acts as a constant pointer pointing to arr[0]. Hence, arr holds the address of arr[0], which is 1000.
- The name arr serves two purposes, first it is the name of the array itself. And second, it acts as a pointer pointing towards the first element in the array.
- By default, arr is equal to &arr[0], meaning it holds the address of the first element in the array.
This connection between pointers and arrays enables efficient manipulation and access to array elements in C programming.
Syntax For Array Pointers In C:
data_type (*pointer_variable)[size];
Here,
- The square brackets indicate that the pointer given by the name pointer_variable is pointing to an array with element number given by size.
- And the type of data stored in the array (hence the data type of the pointer) is given b y the term data_type.
Code Example:
Output:
Original array elements: 1 2 3 4 5
Modified array elements: 10 10 10 10 10
Explanation:
In the above code-
- We begin by defining a function modifyArray that takes a pointer to an array and a value as parameters. The function iterates through the elements of the array and sets each element to the specified value.
- In the main() function, we declare an array arr of size 5 and initialize it with values 1 to 5.
- We then declare a pointer ptr to an array of 5 integers and initialize it with the address of the array arr using the address-of operator (&).
- After that, we use the a for loop with a printf() statement to display the original elements of the array arr. Here, inside the formatted string, we use the pointer ptr and the index value of elements to access them.
- Next, we call the modifyArray function, passing the pointer ptr and the value 10 as arguments. The function modifies all the elements of the array arr using the pointer to an array.
- Finally, we print the modified elements of the array arr using the pointer ptr and a printf() statement inside another for loop.
Complexity: The space and time complexity is O(1).
Function Pointers In C
In C, a function pointer is a variable that stores the memory address of a function. It allows for the indirect invocation of functions, providing flexibility and enabling dynamic function calls. Imagine them as little bookmarks that store the memory addresses of functions. With these bookmarks, we can easily navigate to specific functions and invoke them whenever we need their superpowers.
The syntax for declaring a function pointer involves specifying the function's return type and parameter types, followed by an asterisk (*) and the pointer variable's name.
Syntax For Function Pointers In C:
int (*ptr)(int, char);
This declaration clearly indicates that the function pointer with the name ptr will be pointing to a function that takes two arguments (int and char) and returns an int.
Code Example:
Output:
Result of addition: 8
Result of subtraction: 4
Explanation:
In the C code above-
- We define two functions, i.e., add() and subtract() that take two integers and parameters.
- The first function uses the arithmetic addition operator to add the two numbers and returns their sum.
- The second function uses a subtraction arithmetic operator and returns the difference of the interger numbers.
- Inside the main() function, we declare a function pointer called addPtr, which points to the add() function. It takes two integers and returns an integer.
- Similarly, we declare function pointer subtractPtr pointing to the function subtract(), which takes two integers and returns an integer value.
- We initialize these function pointers by assigning the addresses of the add() and subtract() functions, respectively, using the address-of operator (&).
- Following this, we use these function pointers to dynamically invoke the functions:
- We use addPtr to call the add function with arguments 5 and 3. The outcome of the operation is printed to the console with the respective printf() statement.
- Similarly, we use subtractPtr to call the subtract function with arguments 8 and 4. The outcome of this operation is also printed to the console using printf().
- Finally, the program returns 0, indicating successful completion without any errors.
What Are Complex Pointers In C?
In C, a complex pointer refers to a pointer that points to another pointer or has more than one direction and indirection. This allows for additional levels of indirection, enabling more complex memory management and data structures. This concept is useful in scenarios where you need to dynamically allocate memory for multi-dimensional arrays or create linked data structures like linked lists or trees. Essentially, a complex pointer holds the address of another pointer rather than directly pointing to a variable's value. For example:
int x = 10; // Integer variable
int *ptr_x = &x; // Pointer to x
int **ptr_ptr_x; // Complex pointer to a pointer to an integer
ptr_ptr_x = &ptr_x; // Assign the address of ptr_x to ptr_ptr_x
How To Read A Complex Pointer In C?
Complex pointers in C consist of several operators with varying associativity and precedence (as in the snipper example above).
- The highest precedence and left-to-right associativity are attributed to brackets, i.e., () and [].
- While the asterisk/ indirection operator (*) and the identifier of the pointer hold the second highest precedence with right-to-left associativity.
- The data type operator has the lowest precedence among all.
It's important to take note of the following:
Operator | Symbol | Purpose |
---|---|---|
Bracket Operator | () | Used to declare and define functions |
Array Subscript | [] | It allows to access elements within an array |
Pointer Operator | * | It is used for declaring and handling pointers |
Identifier | Refers to the name of the pointer and is always given the highest priority | |
Data Type | Specifies the variable type the pointer points to, may include modifiers like signed int, long, etc. |
Keeping track of the precedence and associativity of these operators is crucial when dealing with complex pointers in C. Understanding the order in which these operators are applied is essential for writing accurate and efficient code involving pointer-based operations in C programming. For example:
int (*p)[10]
The pointer declaration int (*p)[10] can be analyzed as follows, considering the precedence and associativity of the operators involved:
- Inside the brackets (), both the pointer operator * and the pointer name p have the same precedence. Since their associativity is right to left, the priority goes to p, and the second priority goes to *.
- The [] operator is assigned the third priority, as the data type has the lowest precedence.
- Based on this analysis, the pointer can be read as follows: p is a pointer to an array of integers of size 10.
How To Pass Pointers To Functions In C?
In C, passing pointers to functions allows for the efficient manipulation of data and provides a means to modify variables outside the function's scope.
- When a pointer is passed as a function parameter, the function gains access to the memory address of the variable, enabling the direct modification of its value.
- This is particularly useful when working with large data structures or arrays, as it avoids the need to pass the entire data set, reducing memory overhead.
- Additionally, passing pointers facilitate dynamic memory allocation, allowing functions to modify memory outside their local scope.
- However, one must ensure proper handling and dereferencing of pointers within the function to prevent unintended consequences or undefined behaviour.
Syntax:
void functionName(dataType *ptr);
Here,
- functionName: The name of the function.
- dataType: The data type of the variable that the pointer will point to.
- ptr: The name of the pointer parameter that will receive the address of a variable.
Code Example:
Output:
Modified value of number: 99
Explanation:
In this C code above-
- We begin by defining a function called modifyValue that takes an integer pointer (int *ptr) as a parameter.
- Inside the modifyValue function, the value pointed to by the passed pointer is modified. Specifically, the value at the memory address pointed to by ptr is set to 99.
- In the main() function, an integer variable named number is declared and initialized with the value 42.
- We then call the modifyValue() function by passing the address of the number to it using the address-of operator (&). This means the function receives a pointer pointing to the memory location of the number variable.
- As a result of the function call, the value of number is indirectly modified through the pointer.
- After that, we use a printf() statement to display the modified value of the number variable being pointed to by ptr.
- Finally, the program closes with a return 0 statement.
Complexity: The space and time complexity is O(1).
Applications Of Pointers In C
Pointers in C find applications in various scenarios, contributing to the language's flexibility and efficiency. Here are some key applications of pointers in C language:
- Dynamic Memory Allocation: Pointers enable dynamic memory allocation using functions like malloc(), calloc(), and realloc(). This allows for the creation of flexible data structures such as linked lists, trees, and dynamic arrays.
int *dynamicArray = (int *)malloc(5 * sizeof(int));
- Function Pointers: C supports function pointers, allowing for the creation of arrays of functions, callbacks, and dynamic function invocation. This feature enhances modularity and flexibility in program design.
int add(int a, int b) {
return a + b;
}
int (*ptrFunction)(int, int) = &add;
- Arrays and Strings: Pointers and arrays in C are closely related. Pointers in C programs simplify array operations and traversal, making tasks like string manipulation more efficient.
char str[] = "Pointer Example";
char *ptr = str;
- Data Structures: Pointers are fundamental for implementing complex data structures like linked lists, trees, and graphs. They allow for efficient traversal and manipulation of data, contributing to optimized algorithms.
struct Node {
int data;
struct Node *next;
};
- Passing Parameters to Functions: Pointers enable the passing of parameters by reference, allowing functions to modify the original data directly. This is particularly useful when dealing with large data structures.
void modifyValue(int *ptr) {
*ptr = 42;
}
Direct & Indirect Access Using Pointers In C
There are two ways to access data in C programs, i.e., direct and indirect. Direct access involves using the respective variable name to access and manipulate the data. The indirect access method involves using a pointer in C programs with the help of the dereference operator (*). This allows us to retrieve or modify data at that specific location in memory, indirectly. This method provides a level of abstraction, enabling the traversal and manipulation of complex data structures.
Code Example:
Output:
Direct Access, var=5
Indirect Access, var=5
Explanation:
In this C code snippet-
- Inside the main() function, an integer variable var is declared and initialized with the value 5.
- We then declare an integer pointer ptr and assign the memory address of the variable var to it, using the address-of operator (&). Now, ptr holds the address where the value of a is stored in memory.
- After that, we use two printf() statements to access and print the value stored in var.
- In the first statement, we show the direct access method, we use the variable name to access the data, i.e., printf("Direct Access, a=%d\n", var)
- In the second statement we show indirect access to data using a pointer in C, which here is ptr. That is, printf("Indirect Access, a=%d\n", *ptr).
- Finally, the program terminates by returning 0 to the operating system.
Complexity: The space and time complexity is O(1).
Printing Pointers In C Language
Printing pointers in C can be a useful debugging technique to check the memory addresses that the pointers are pointing to. When you print a pointer, you are displaying the memory address it holds rather than the value it points to.
- We must use the %p format specifier when printing pointers in C.
- It's important to remember that the memory address represented by the pointer may vary each time you run the program due to the nature of memory allocation in your system.
- Therefore, don't be surprised if you see different memory addresses on different runs.
- Keep in mind that dereferencing a pointer and trying to print its value when it is uninitialized or pointing to an invalid memory location can lead to undefined behaviour.
- Always ensure that a pointer is pointing to valid memory before dereferencing or printing its value.
Code Example:
Output:
Address of x: 0x7ffc3ffac9fc
Explanation:
Inside the main() function of the C program example above, we declare an integer variable x and initialize it with the value 42.
- We also declare an integer pointer ptr and initialize it to hold the memory address of variable x using the address-of operator (&).
- Next, we use the printf() function to print the memory address of variable x by dereferencing the pointer ptr.
- The format specifier %p is used to represent the memory address in hexadecimal format.
- Finally, the program returns 0, indicating successful completion to the operating system.
Complexity: The space and time complexity is O(1).
In summary, this code snippet demonstrates the use of a pointer to obtain and print the memory address of a variable (x) in the program.
Pointer Arithmetic In C
Pointer arithmetic refers to the set of valid arithmetic operations that can be performed on pointers in C programming. As we know, a pointer variable stores the memory address of another variable rather than storing an actual value. Consequently, only a limited number of operations are allowed on pointers.
The C pointer arithmetic operations differ slightly from the standard mathematical calculations we are accustomed to. These operations include:
- Increment/Decrement of a Pointer: You can increment or decrement a pointer in C to make it point to the next or previous memory location of its data type.
- Addition of Integer to a Pointer: You can add an integer value to a pointer, which will cause the pointer to point to a memory location offset by the value multiplied by the size of its data type.
- Subtraction of Integer from a Pointer: You can also subtract an integer value from a pointer in C, moving the pointer backwards in memory by the value multiplied by the size of its data type.
- Subtracting Two Pointers of the Same Type: You can subtract two pointers of the same data type, which will give you the number of elements between the two pointers in terms of their data type size.
- Comparison of Pointers of the Same Type: You can compare two pointers in C, of the same data type using relational operators to check their relative positions in memory.
Certain arithmetic operations are considered invalid when working with pointers in C. These include:
- Addition of Two Pointers: Adding two pointers in C is not allowed as they don't have a meaningful interpretation.
- Division of Two Pointers: The division of pointers is also not supported in C.
- Multiplication of Two Pointers: We also cannot multiple to pointers in C language.
By adhering to the rules of valid pointer arithmetic, we can safely perform operations on pointers in C programs without encountering undefined behaviour or errors.
Code Example:
Output:
Element at index 1: 20
Element at index 2: 30
After incrementing, element at index 1: 20
After decrementing, element at index 0: 10
After subtracting 1, element at index 1: 20
Explanation:
In the C code example-
- We declare and initialize an integer array arr with values {10, 20, 30, 40, 50} inside the main() function.
- Additionally, we declare an integer pointer ptr and initialize it to point to the array, arr (essentially the first element of the array arr).
- We use a set of printf() statements to display the values of array elements. Here, we use pointer addition arithmetic as follows:
- We first print the value at index 0 (i.e., 10) using the pointer *ptr.
- Then, we use pointer addition to add 1 to the ptr and access the value at index 1 of the array, which prints 20.
- After that, we add an offset of 2 to the pointer, i.e., ptr + 2, effectively moving it two elements ahead in the array. This prints the value at index 2 of the array, which prints 30.
- Next, increment the pointer using ptr++, which moves it to the next element in the array. Then, we use *ptr to print the value at the new index 1, which prints 20.
- Following this, we decrement the pointer using ptr--, moving it back to the previous element. Then, we use *ptr to print the value at the original index 0 again, which prints 10.
- We add an offset of 2 to the pointer using ptr = ptr + 2, which means that the pointer points to the value at index 2.
- Building on that, we show pointer subtraction by deducting 1 from the pointer, i.e., ptr-1, moving it one element back to index 1. Using this inside the printf() statement we display the value at this index to the console.
- The program returns 0, indicating successful completion of the operating system.
Complexity: The space and time complexity is O(1).
The pointer arithmetic demonstrates how a pointer can be manipulated to navigate through the elements of an array.
What is Pointer Casting in C?
Pointer casting in C involves the explicit conversion of a pointer from one data type to another. This operation allows programmers to reinterpret the data at a specific memory location as belonging to a different type.
Syntax:
new_data_type *new_ptr = (new_data_type *)old_ptr;
Here,
- new_data_type: The desired data type to which the pointer is cast.
- new_ptr: The new pointer variable that will store the casted value.
- old_ptr: The existing pointer variable or expression to be cast.
The syntax for pointer casting includes placing the target data type in parentheses before the pointer variable or expression.
- While pointer casting provides flexibility, it demands careful consideration of data compatibility to avoid unintended consequences or undefined behaviour.
- Common use cases include converting between different pointer types or adjusting the interpretation of data at a memory location.
- For example, an integer pointer should only point to integer data, and a character pointer should only point to character data.
- Another reason is related to pointer arithmetic, where the size of the data type affects the memory address incrementation.
Code Example:
Output:
Original int value: 42
Casted double value: 0.000000
Explanation:
In this C code above-
- First, inside the main() function, we declare an integer variable intValue and initialize it with the value 42. We also declare a double variable doubleValue without initializing it.
- Next, we declare two pointers, i.e., an integer pointer intPtr, which we initialize with the memory address of the intValue variable. The second pointer, double pointer doublePtr, isn't explicitly initialized.
- We then perform a pointer casting operation, converting the integer pointer intPtr to a double pointer doublePtr.
- For this, we use the casting operator () with a new data type inside it, i.e., (double*)intPtr. While this is allowed, we must be cautious as it assumes that the memory layout and interpretation of the data are compatible.
- After the casting, we dereference the casted pointer doublePtr to access the value stored at the memory location pointed to by intPtr.
- Then, we assign the value being pointed to by the doublePtr to the doubleValue variable.
- Finally, we use two printf() statements, one to print the original integer value and the other to print the casted double value. We use %d and %lf format specifiers to indicate integer and double values, respectively.
Complexity: The space and time complexity is O(1).
Address Of (&) Operator In C
The address-of operator, denoted by the ampersand symbol (&), is a unary operator used to obtain the memory address of a variable.
- When applied to a variable, the address-of operator returns the hexadecimal representation of the memory location where the variable is stored.
- This address is crucial for various operations involving pointers in C, allowing programmers to manipulate memory directly, pass variables by reference to functions, and dynamically allocate memory.
- The address-of operator enhances the versatility of C language, enabling efficient memory management and direct access to the underlying hardware, providing a level of control and flexibility appreciated in systems programming and low-level operations.
Syntax:
&variable_name;
Here, the ampersand sign is for the address-of operator, and the variable_name refers to the variable whose address we are using.
Code Example:
Output:
5
0x7ffc7ac25ba4
Explanation:
In this C code-
- We decalre and initialize an integer variable x with the value 5, inside the main() function. Then, we use a printf() statement with a formatted string to display this value.
- After that, we use another printf() statement to display the address/ memory location of the variable x using the address-of operator, i.e., &x.
Complexity: The space and time complexity is O(1).
The Size Of Pointers In C
In C programming, the size of a pointer variable is influenced by the underlying system architecture. It's amazing to learn that a memory address, which aids the computer in keeping track of where data is kept in memory, is internally represented as an integer value.
- It is interesting to note that regardless of the data type the pointers in C are referencing, its size remains constant.
- That is, whether the pointer points to an integer, character, or any other data type, it occupies the same amount of memory.
- In C, we use the sizeof operator to ascertain the size of a pointer, which reveals the number of bytes allocated for the pointer variable.
- With this information, we can efficiently manage memory and handle memory addresses consistently, regardless of the data types involved. It's comparable to understanding how a computer works and utilizing its memory management tools.
Syntax:
sizeof(operand)
Here, the keyword sizeof is the operator itself, and the operand refers to the element whose size we want to determine.
Code Example:
Output:
Size of NULL Pointer: 8 Bytes
Size of Integer Variable: 4 Bytes
Size of Integer Pointer: 8 Bytes
Size of Float Variable: 4 Bytes
Size of Float Pointer: 8 Bytes
Explanation:
In this C example-
- We declare and initialize an integer variable count with the value 10 and a floating-point variable sum with the value 20.5.
- After that, we declare three pointer variables, i.e., null_ptr pointing to NULL, int_ptr initialized with address-of the count variable, and float_ptr pointing to the sum variable.
- Following this, we use a set of printf() statements with the sizeof() operator to calculate and print the sizes of these variables in bytes.
- The %zu format specifier is used for the sizeof operator, which is appropriate for printing the size of objects.
- Finally, the program completes execution with a return of 0.
Complexity: The space and time complexity is O(1).
To know more about the sizeof() operator, read- The Sizeof() Operator In C | A Detailed Explanation (+Examples)
Advantages Of Pointers In C
Here are some of the most common advantages that come with using pointers in C programming:
- Memory Efficiency: Pointers allow for dynamic memory allocation and deallocation, enabling more efficient use of memory. This is particularly beneficial when dealing with data structures of varying sizes or when precise control over memory is required.
- Efficient Function Parameter Passing: Passing pointers to functions instead of the actual data can be more efficient, especially for large data structures. This avoids the overhead of copying the entire data set, contributing to better performance.
- Dynamic Data Structures: Pointers facilitate the creation and manipulation of dynamic data structures such as linked lists, trees, and dynamic arrays. This flexibility allows for the efficient management of memory resources during program execution.
- Array Manipulation: Arrays and pointers in C are closely related, allowing for efficient traversal and manipulation of array elements using pointer arithmetic. This relationship simplifies array operations and enhances code readability.
- Function Pointers: C supports function pointers, enabling the creation of callbacks and dynamic function invocation. This feature contributes to the development of modular and extensible code structures.
- String Handling: Strings in C are typically represented as arrays of characters, and pointers provide an efficient way to manipulate and process strings. Pointers simplify tasks like concatenation, comparison, and copying of strings.
Disadvantages Of Pointers In C
Some common disadvantages of using/ working with pointers in C are as follows:
- Dangling Pointers: Dangling pointers occur when a pointer points to a memory location that has been deallocated. Dereferencing such pointers can lead to undefined behavior, crashes, or security vulnerabilities.
- Memory Leaks: Improper memory management, such as failing to deallocate dynamically allocated memory, can result in memory leaks. Over time, this can degrade system performance and exhaust available memory.
- Null Pointers: Dereferencing a pointer that points to NULL can cause runtime errors, crashes, or unexpected behavior. Careful initialization and checking for null pointers are essential to avoid such issues.
- Complexity: Pointers introduce an additional layer of complexity to the code. Novice programmers may find it challenging to understand and manage pointer-related concepts, leading to errors and bugs.
- Pointer Arithmetic Pitfalls: Incorrect usage of pointer arithmetic can lead to accessing invalid memory locations, causing unpredictable results and compromising program stability. Careful handling is necessary to avoid such pitfalls.
- Security Concerns: Pointers can be vulnerable to security threats, such as buffer overflows and pointer manipulation attacks. Developers need to implement proper bounds checking and input validation to mitigate these risks.
Conclusion
In conclusion, pointers in C serve as a foundational concept empowering us to harness the potential of memory management effectively. They act as a double-edged sword, offering unparalleled power and flexibility but demanding careful and responsible use. Understanding the nuances of manipulating pointers in C is crucial for effective memory management and efficient code.
By mastering pointers, C programmers can unlock the full potential of the language, paving the way for optimized algorithms, data structures, and modular code structures. As with any powerful tool, proper handling and vigilance are essential to harness the benefits while avoiding the pitfalls associated with pointers in C.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Explain pointers and their characteristics.
Pointers in C are variables that store memory addresses. They enable dynamic memory allocation, efficient manipulation of data structures, and support features like function pointers. A pointer is declared with a data type and an asterisk (*), and it can be assigned the address of another variable using the address-of operator (&).
- Characteristics include the ability to perform pointer arithmetic, which facilitates array manipulation, and the distinction between constant pointers (whose address is fixed) and pointers to constants (which point to immutable data).
- While powerful, pointers require careful management to avoid issues like dangling pointers, memory leaks, and null pointer dereferences.
- Understanding pointers is fundamental for effective memory control and optimized C programming.
Q. Describe the concept of an array of pointers in C.
In C, an array of pointers is a structure that holds multiple pointers, where each element of the array points to a memory location. This arrangement allows for the creation of arrays that can dynamically manage different types of data.
For instance, an array of pointers to integers can be used to represent a dynamic array of integers, providing flexibility in memory allocation and manipulation. Array of pointers is often employed in scenarios like managing strings, creating dynamic data structures, or facilitating efficient sorting algorithms, enhancing the versatility and memory efficiency of C programs.
Q. Where is pointer memory allocated in C?
In C, the memory for a pointer variable is allocated on the stack or in the data segment, depending on its declaration and usage. If the pointer is a local variable within a function or block, it is typically allocated on the stack. However, when the pointer is employed to point to dynamically allocated memory using functions like malloc() or calloc(), the memory for the pointer itself is allocated on the stack or in the data segment, while the memory it points to is allocated on the heap.
Q. Do pointers consume memory in C?
Yes, pointers in C do consume memory. A pointer is a variable that stores the memory address of another variable. The size of a pointer depends on the architecture of the system.
- On most modern systems, the size of a pointer is typically 4 bytes in 32-bit systems and 8 bytes in 64-bit systems.
- When you declare a pointer, the memory required to store the memory address it points to is allocated. For example:
int *ptr;
Here, ptr is a pointer to an integer, and it consumes memory to store the memory address of an integer variable.
Q. Distinguish between a constant pointer and a pointer to a constant in C.
In C, a constant pointer and a pointer to a constant are two distinct concepts that involve the immutability of either the pointer or the data it points to.
A constant pointer is a pointer whose address cannot be changed once it is initialized. The data it points to, however, can be modified. This means that while the pointer itself remains fixed to a particular memory location, the content at that location can be altered.
int x = 10;
int *const ptr = &x; // Constant pointer to an integer
*ptr = 20; // Valid - changes the value at the memory location pointed by ptr
// ptr = &y; // Invalid - attempting to change the address pointed by ptr
On the other hand, a pointer to a constant is a pointer that can be reassigned to different memory addresses, but the data it points to is treated as constant and cannot be modified through that pointer.
int x = 10;
const int *ptr = &x; // Pointer to a constant integer
// *ptr = 20; // Invalid - attempting to change the value at the memory location pointed by ptr
ptr = &y; // Valid - the pointer itself can be reassigned to another memory location
Q. Identify the dissimilarities between an array and pointers in C.
Arrays and pointers in C share some similarities, but they also have significant differences. Here are key dissimilarities between an array and a pointer:
-
Fixed Size vs. Dynamic Size:
- Array: Has a fixed size determined at compile time. Once declared, the size remains constant throughout the program execution.
- Pointer: Can point to a single memory location or to dynamically allocated memory, allowing for flexibility in size and dynamic memory management.
-
Initialization and Assignment:
- Array: Must be initialized with a specific set of values or assigned another entire array.
- Pointer: Can be initialized with a memory address, assigned dynamically allocated memory, or assigned the address of another variable.
-
Size Information:
- Array: Carries size information internally, and the size is known at compile time.
- Pointer: Does not inherently store size information. The size must be managed separately, leading to potential issues if not handled carefully.
-
Memory Allocation:
- Array: Memory is allocated for all its elements at once when the array is declared.
- Pointer: Memory is allocated separately for the data pointed to by pointers in C, which can be dynamically allocated during runtime.
You might also be interested in reading the following:
- Control Statements In C | The Beginner's Guide (With Examples)
- Comma Operator In C | Code Examples For Both Separator & Operator
- Bitwise Operators In C Programming Explained With Code Examples
- Jump Statement In C | Types, Best Practices & More (+Examples)
- Length Of String In C | 7 Methods Explained With Detailed Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment