Length Of String In C | 7 Methods Explained With Detailed Examples
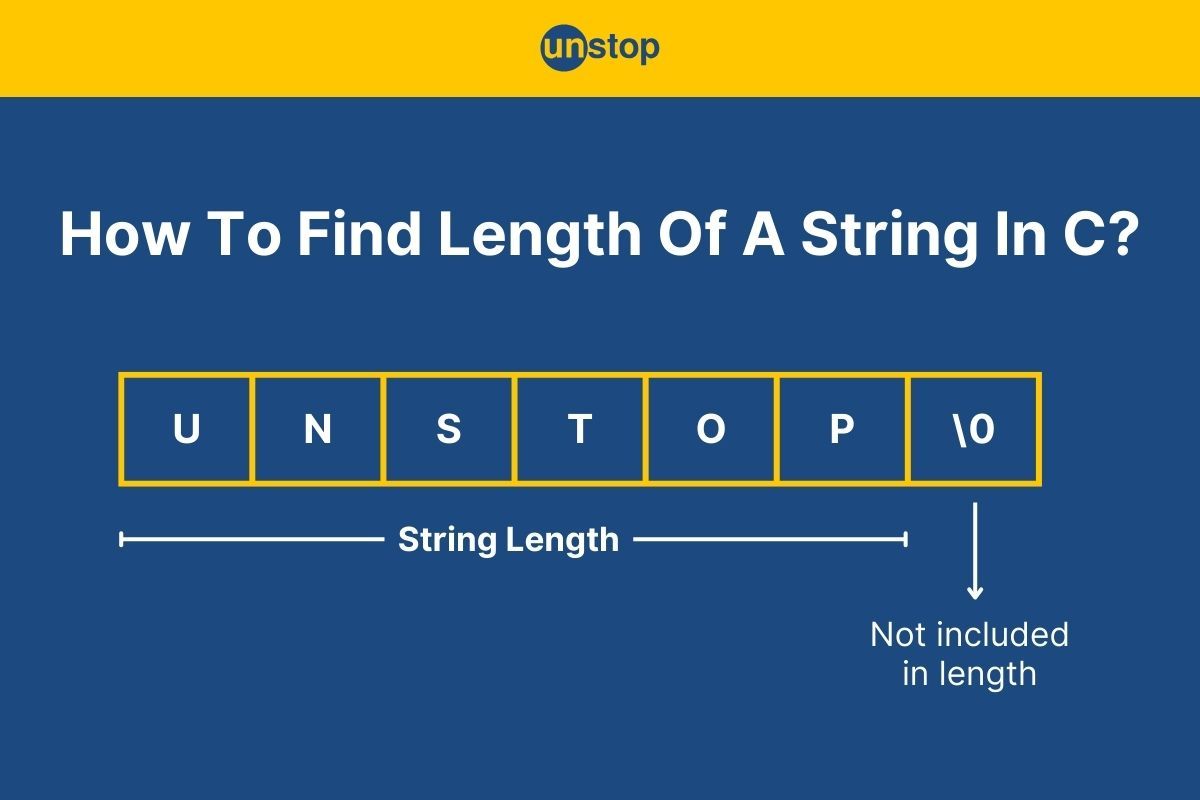
Table of content:
- What Is String In C?
- How To Find Length Of String In C?
- Find String Length In C Using Built-In Library Function Strlen()
- How To Use Sizeof() Operator To Find Length Of String In C?
- How To Use Loops To Find Length Of String In C?
- How To Find Length Of String In C Using Recursion?
- Find String Length In C Using Pointers
- String Length In C Using User-Defined Function
- Conclusion
- Frequently Asked Questions
A string in C programming language is a collection of characters stored in a character array, which is terminated by a null character (\0). Strings are one of the most commonly used data types in programming, especially when working with textual data. Naturally, we often need to perform a variety of manipulations on strings, and one of the important string manipulation procedures is to determine the length of a string in C.
Since the termination of strings happens with a null character, its length is determined by the number of characters in the array except the null character (\0). Knowing the length of a string has a set of wide applications, such as input validation for passwords, text comparison, data filtering, and pattern recognition. In this article, we will discuss the various ways of computation of string length in C programs with proper code examples and explanations.
What Is String In C?
Before we begin learning about the ways of string length determination, let's discuss the basics of strings. As mentioned before, in C (and most other programming languages), a string is a sequence of characters that terminates with a null character (\0). Strings are often referred to as character arrays, with each element of the array denoting a specific character of the string.
The last element of the array contains a null element (‘\0’) or terminator character, which indicates the end of the string. Every character of a string can be accessed separately using the index of the character array.
The Basic Syntax For Declaring A String In C:
char string_name[size]
Here,
- The term char reflects the data type of the element, which here is the character type (since strings are a sequence of characters).
- The name of the string is given by string_name, and the number of characters is given by size.
For example, consider the following line-
char myString[ ] = "Hello, World!";
In this example, myString is an array of characters that contains the string- "Hello, World!". We have used the double quotes method to initialize it. The size of the array is automatically determined by the length of the string plus one for the null character.
To learn more about strings in C, read- Strings In C | Declare, Initialize & Use String Functions (+ Examples)
How To Find Length Of String In C?
There are multiple string length methods we can use in C to determine the length of a string, including built-in standard library functions, operators, and control flow statements. The general approach to finding the length of the string in C is as follows:
- We begin by declaring a string/ character array str[ ] to store the given string.
- We then use two basic methods to find out its length:
- The built-in library functions and operators.
- Count each character of the array to get the length of the string. This can be done using loop, recursion, user-defined functions, and pointers.
- Finally, we will store the obtained length in a variable and output it.
The methods to carry out string length calculation in C programming are as follows:
- The streln() Function
- The sizeof() Operator
- For Loop
- While Loop
- Recursion
- Pointers
- User-defined Functions
We will discuss each of these string length methods in detail, with code explanations in the sections ahead.
Find String Length In C Using Built-In Library Function Strlen()
In C programming, strlen() is a predefined function from the standard C library and is used to find the length of a null-terminating string. This function belongs to the <string.h> header file, which contains many other efficient string processing methods. The sterln() function simply counts the number of characters until it reaches the end of the string, i.e., the null character (‘\0’).
Syntax Of sterln() Function To Find Length Of String In C:
#include<string.h>
strlen(str);
Here,
- We must include the <string.h> header file to use the sterln() function, i.e., #include<string.h>.
- The term str is the string's name/ identifier, which is the parameter taken by the sterln() function. The string, as usual, is terminated by a null character.
Let’s understand it better with the help of an example, as illustrated below.
Code Example:
Output:
The length of the String is: 6
Explanation:
We begin the sample C program by including the <stdio.h> and <string.h> header files for input/ output operations and string methods, respectively.
- Then, we initiate the main() function, which is the program's entry point.
- Inside main(), we define a character array/ string str and assign the string value Unstop to it using the double quotes method. This is a null-terminated string/ sequence of characters in memory.
- Next, we define an integer variable len to hold the length of the string, i.e., the result obtained from the strlen() function.
- We initialize the len variable by calling the sterln() function and passing the string str as an argument.
- The function iterates through the characters in the array until it encounters the null character, counting the number of characters in the process. The resulting length is then assigned to the len variable.
- Then, we use a printf ()statement to display the calculated length of the string. We use the %d format specifier to indicate that an integer value will be printed.
- Finally, the main function concludes with a return 0 statement, signaling that the program has been executed successfully.
Time Complexity: The time complexity of the strlen() function in C is generally O(n), where n is the length of the input string argument.
Also read- How To Run C Program | Step-by-Step Explanation (With Examples)
How To Use Sizeof() Operator To Find Length Of String In C?
In C programming language, sizeof() is a built-in unary operator that returns the size (in bytes) of a data type, variable, or expression. For a character array, it returns the size of the array, including the null character (\0). So, the string length will be one less than the value returned by the sizeof() operator. Let's look at a code implementation of this operator to understand how it works.
Code Example:
Output:
String Length is: 13
Explanation:
In the C code example above-
- We define and initialize a character array/ string str with the string literal- Unstop Rocks!, inside the main() function.
- This array is essentially a sequence of characters (character array) in memory, terminated by the null character (\0), marking the end of the string.
- Then, we define an integer variable length to store the length of the string.
- Then, we use the sizeof() operator to obtain this length, passing the string str as the operand. This operator calculates the memory space occupied by the string in bytes.
- However, since the size obtained also includes the null character, we subtract 1 to accurately represent the actual length of the string.
- We then use a printf() statement to display the calculated length of the string.
- Finally, the main function concludes by returning 0, indicating there were no errors in the program's execution.
How To Use Loops To Find Length Of String In C?
We can iteratively calculate the length of a string using a loop. This involves counting each character of the array using a counter variable until the null character is reached. Upon reaching the null character, the loop gets terminated, and the counter variable will have the length of the string. The general approach to using loops to determine string length is as follows:
Step 1- Declare Variables: We begin by declaring an integer variable to store the length of the string (e.g., length). And an integer variable/ control variable for iterating through the characters in the string (e.g., i).
int length = 0;
int i;
Step 2- Initialize Variables: Next, we initialize the length variable by setting it to 0.
Step 3- Loop Through the String: Then we use a loop (e.g., for or while) to iterate through each character in the string. The loop continues until the null character ('\0') is encountered.
for (i = 0; str[i] != '\0'; i++) {
// Code inside the loop
}
Step 4- Increment Length & Check For Null Character: Within the loop, we increment the length variable for each character to get the final count. The loop condition (str[i] != '\0') must also check whether the current character is not the null character.
Step 6- Complete Length Calculation: As soon as the null character is found (i.e., the loop condition is met) the loop terminates and the outcome is the length of the string. Now, you can print or use the length value as needed.
We can use both a for loop and a while loop to calculate the length of a string in C programs. Let's take a look at the examples of both these methods.
For Loop To Find Length Of String In C
A for loop consists of three elements, i.e., the initialization condition which sets the starting point for control variable, a loop condition which determines if the loop will be executed, and an updating condition to update the value of the control variable. All in all, it helps iterate through the string (input variable) to determine its length.
Given below is an example demonstrating the code implementation of a for loop to determine the length of a string in C language.
Code Example:
Output:
String Length is: 15
Explanation:
In the sample C code-
- Inside the main() function, we declare a string str and initialize with the string value- Unstop is 11/10.
- We then declare an integer variable count utilized to 0 to serve as a counter to track the length of the string.
- Then, we use a for loop to iterate through each character in the string until the null character (\0) is encountered. Within the loop-
- The count variable i begins with 0, and the loop checks if the character at position i is null using not equal to relational operator, i.e., str[i] !=0.
- If the condition is true, i.e., the character is not null, the loop code is executed. That is, it increments the value of the count variable.
- After every iteration, the value of the control variable is also increased by 1, and this goes on till the condition becomes false.
- When this happens, the count variable reflects the number of characters up till the null character. This is the length of the input string stored in the count variable and returned by the loop.
- Next, we use a printf() statement to display the calculated length of the string. The format specifier %d indicates that an integer value will be printed, corresponding to the value stored in the count variable.
- Conclusively, the main function returns 0, signifying the successful execution of the program.
While Loop To Find Length Of String In C
Just like a for loop, the while loop also helps iterate through the characters of the string to determine its length. The loop condition is a boolean expression that helps determine if the code block is executed. Look at the code implementation example below to understand how to use a while loop to calculate the length of a string in C language.
Code Example:
Output:
The length of string is: 12
Explanation:
In the C code sample-
- We first declare the string variable str and initialize it with the string- Hello world!, inside the main() function.
- Then, we declare a length counter variable len, to hold the length of the string and an index/ loop control variable i, and initialise them both with 0.
- We then employ a while loop that checks if the current character is null, i.e., the looping condition str[i] != ‘\0’ at the start of every iteration.
- If the condition is true, the loop's body will execute. If the condition is false, the loop will terminate.
- In each iteration, the value of variables len and i increases by 1 until the loop encounters the null character.
- Upon reaching the null character, the loop terminates, and the len variable provides the length of the string.
- Lastly, we use the printf() function to print the length of the string, as seen in the output window.
- The main function returns 0, signifying the successful execution of the program.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
How To Find Length Of String In C Using Recursion?
A recursive function is one that calls itself again and again (directly or indirectly) till a condition is specified. We can calculate the length of a string in C program by creating a recursive function that traverses the string character by character until the null character ('\0') is encountered.
The base case for such a recursive function would be when the null character is reached, signaling the end of the string. In each recursive call, the function advances to the next character and increments a counter. The length is accumulated as the function unwinds its recursive calls. Look at the code implementation example below for a better understanding.
Code Example:
Output:
The string length is: 6
Explanation:
In the above code example-
- We define a rec_length function to calculate the length of a str string using recursion.
- It takes two parameters, i.e., a character array/ string str and an integer index, representing the current position of string element in the array. In the function-
- We have a conditional if-statement that checks if the character at the current index is the null character, i.e., str[index]== '\0'.
- As mentioned in the code comment, this is the base condition for the recursion function. If the null character is encountered, i.e., the condition is true, the function returns 0, indicating the end of the function string.
- If the base condition is not met, the function recursively calls itself with an incremented index (index + 1).
- The returned value is then incremented by 1, representing the current character's contribution to the overall string length.
- In the main() function, we declare a character array str and initialize it with the string value- Unstop.
- Next, we initialize an integer variable len to 0, which will ultimately store the length of the string.
- Then, we call the rec_length function with the string and an initial index of 0. The result is assigned to the len variable, representing the total length of the string.
- Finally, we use a printf() statement to display the calculated length of the string.
- The main function concludes by returning 0, indicating the successful execution of the program.
Find String Length In C Using Pointers
We use a pointer to store a variable's memory address in C programming. When we assign a character array (string) to a pointer, it automatically stores the memory address of the first character of the array. To determine the length of the string, we need to count all the characters of the array leaving the null character, i.e., increment the pointer by one in a loop and terminate it upon reaching the null character. An example of the above implementation is explained below.
Code Example:
Output:
The length of the String is: 22
Explanation:
In the example C code-
- We declare and initialize a string str with the string value- Unstop is Unstoppable! inside the main() function.
- This string represents a sequence of characters/ character array in memory, concluded by the null character ('\0'), signifying the end of the string.
- Next, we declare an integer variable, len, and initialize it with the value 0. This variable will be used to store the length of the string.
- Then, we define a character pointer ptr and set it to point to the first character of the string str. This pointer will be used to iterate through the characters in the string.
- We then use a while loop to iterate through the string characters until the null character ('\0') is encountered. Inside the loop-
- The loop condition checks if the character being pointed to by the ptr variable is not a null character.
- If the condition is true, i.e., the character is not null, then the loop body is executed. The value of the len variable is incremented by 1 for each character/ iteration, effectively tallying the length of the string.
- The value of the ptr variable is also incremented by 1 in every iteration to move to the next character in the array.
- The loop terminates when the condition becomes false, i.e., the null character is encountered. At this point, the len variable has the count of characters in the string.
- Then, we use a printf() statement to display the calculated length of the string.
- Finally, the main function returns 0, indicating the successful execution.
An alternative approach where we can use pointers to get the length of a string is pointer subtraction. Here, we traverse to the last pointer and then subtract the pointer pointing to the element/ character at the first position of the string from the pointer pointing to the last element of the string. The difference will give us the number of characters/ elements between the characters, i.e., the length of the string.
String Length In C Using User-Defined Function
The length of string in C can also be determined by employing a user-defined function. This function can be implemented using loops, pointers, and built-in library functions. take a look at the example below, where we use a while loop inside a user-define function to find the length of a string.
Code Example:
Output:
The length of the string is: 13
Explanation:
In the code example above,
- First, we create a user-defined function named length_cal, which takes a character array str as an argument and calculates the length of the string using a while loop. Inside the function-
- We have two integer variables, idx (representing the index of the array) and len (representing the length of the string), both initialized to 0.
- Then, we have a while loop that iterates through the characters in the string until the null character ('\0') is encountered.
- In every iteration, the loop increments the values of both the len variable and the idx index. The function then returns the final value of len.
- In the main() function, we declare a character array str and initialize it with the string- I am an Otaku.
- We also declare an integer variable count and initialize it to 0.
- Next, we call the user-defined function length_cal with the str array as an argument, and the result is assigned to the count variable. This effectively calculates the length of the string.
- Then, we use a printf() statement to display the calculated length of the string. The format specifier %d indicates that an integer value will be printed, corresponding to the value stored in the count variable.
- Finally, the main function returns 0, signifying the successful execution of the program.
Conclusion
Understanding how to calculate the length of a string is crucial for working with text data in C programming. One of the most convenient and widely used methods to get the length of string in C is the strlen() function. It provides a straightforward way to obtain the length of a string without the need for manual iteration. Other methods include the sizeof() operator, for loop, while loop, pointers, pointer subtraction, recursion, and user-defined functions.
Whether using the strlen function or manually iterating through the characters, the goal is to determine the number of characters in the string, excluding the null character at the end. Choose the method that best suits your needs and coding style.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. How to find string length in C without using strlen()?
The strlen() function is a standard library function that iterates through the loop and returns its length. An alternative approach to this is using a loop to determine the string length. Below is an example showcasing the implementation specifics of this method.
Code Example:
Output:
The length of string is: 12
Explanation:
In the code example above-
- We first declare a string str and initialize it with the string value- Hello world! inside the main() function.
- Then, we declare a counter variable len to hold the length of the string and an index control variable i and initialize them both with 0.
- We then start a while loop, which first checks the condition str[i] != ‘\0’ at the start of every iteration. If the condition is true, the loop's body will execute; otherwise, the loop will terminate.
- In each iteration, the value of len and i increases by 1 until it reaches the null character. Upon reaching the null character, the loop terminates, and the len will have the length of the string.
- Lastly, we use the printf() function to print the length of the string, as seen in the output window.
Q. Is length() a string function?
No, the length() function is not an inherent library function in C programming language or its string library. Instead, we have a strlen() function to find the length of a string in C, which is included in the <string.h >header file.
However, the length() function does exist as a member function in the std::string class from the C++ standard library. As the name suggested, it is used to calculate the length of a string in the C++ programming language.
Q. What is the use of the strlen() function in C?
The strlen() function is a predefined function from the standard C library used to find the length of a null-terminated string. It can be accessed by including the <string.h> header file at the beginning of the code. The pre-existing library function 'strlen()' simply counts the number of characters in a string until it reaches the end of the string, i.e., the null character (‘\0’).
Take a look at the code below to understand how the strlen() function is implemented inside a C program sample to get the length of a string.
Code Example:
Output:
The length of the String is: 6
Explanation:
In the code example above, we include the essential header files for string manipulations and input/ output operations.
- Inside the main() function, we declare a string/ character array str and initialize it with the value- Unstop.
- We then call the strlen() function by passing str to it as an argument. The function calculates the length of the string and stores it in the len variable.
- We print this length to the console using the printf() function.
Q. How can you count characters in C?
Characters stored in a character array in C can be counted using a loop. To count the number of characters, we need to iterate through the loop and increment the value of a counter variable by one for each iteration until we reach the end of the character array, i.e., the null character.
The above approach is explained with the help of an example as shown below,
Code Example:
Output:
Number of characters: 12
Explanation:
In the above code example-
- We declare a string/ character array str and initialize it with the value- Hello world!
- Then, we declare two integer variables, len and i, where the former stores the length and the latter is the index counter/ control variable. We set them both to 0 to begin with.
- Next, we use a while loop where the condition determines if the current character is not null, i.e., str[i] != ‘\0’, at the start of every iteration. If the condition is true, the loop's body will execute; otherwise, the loop will terminate.
- In each iteration, the value of variables len and i is increased by 1 until it reaches the null character. Upon reaching the null character, the loop terminates, and the len will have the number of characters in the array.
- Lastly, we use the printf() function to print the number of characters, as seen in the output window.
Q. How to take a string with blank space as input in C?
There are various ways to input a string with blank space in the C programming language. One such method of taking input is the fgets() function. The syntax for this function is:
char *fgets(char *str, int size, FILE *stream)
Here, str is a pointer to the character array where the input string will be stored, size is the maximum number of characters to be read. This will prevent the buffer overflow and the stream for reading the input. This is typically stdin for taking the standard input.
Code Example:
Output:
Enter the string: Unstop Rocks!
The string entered is: Unstop Rocks!
Explanation:
In the above code example,
- We first declare a character array str of size 20, i.e., the string can hold 20 characters at max, including null and space characters.
- Then, we call the fgets() function by passing the string str, character count, and stdin as parameters. The function automatically adds a newline character at the end of the input string.
- Finally, we use the printf() function to print the string as shown in the output window.
Q. How to find the string length in C?
The length of a string in C can be determined by using either built-in library functions or counting each character of the array to get the length of the string. The ways to calculate the length can be categorized as follows:
- Using the built-in library function strlen(), that returns the length of the string as an integer value.
- By using the built-in sizeof() operator, which returns the length of the string in terms of the size of the character array (in bytes).
- By iterating through the string, counting every character (including the space character) and terminating upon reaching the null character (‘\0’) to get the length of the string. This can be done using -
- Loop - while and for loop
- Recursive functions
- Pointers
- User-defined functions
As mentioned, there are multiple ways to calculate the length of string in C.
You might also be interested in reading the following:
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
- Do-While Loop In C Explained With Detailed Code Examples
- Comma Operator In C | Code Examples For Both Separator & Operator
- Ternary (Conditional) Operator In C Explained With Code Examples
- Dangling Pointer In C Language Demystified With Code Explanations
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment