Table of content:
- How To Reverse A String In C?
- Reverse A String In C Using strrev() Function
- Other Ways To Reverse A String In C Without strrev() Function
- Reverse A String In C Using For Loop
- Reverse A String In C Using While Loop
- Reverse A String In C Using Recursion Function
- Reverse A String In C Using Pointers
- Reverse A String In C Using Stack
- Reverse A String In C By Printing It Backward
- Reverse A String In C With User-Defined Function To Swap Characters
- Reverse A String In C Using New Character Array
- C Program To Check If Reverse String Is A Palindrome
- Conclusion
- Frequently Asked Questions
Reverse A String In C | 10 Different Ways With Detailed Examples
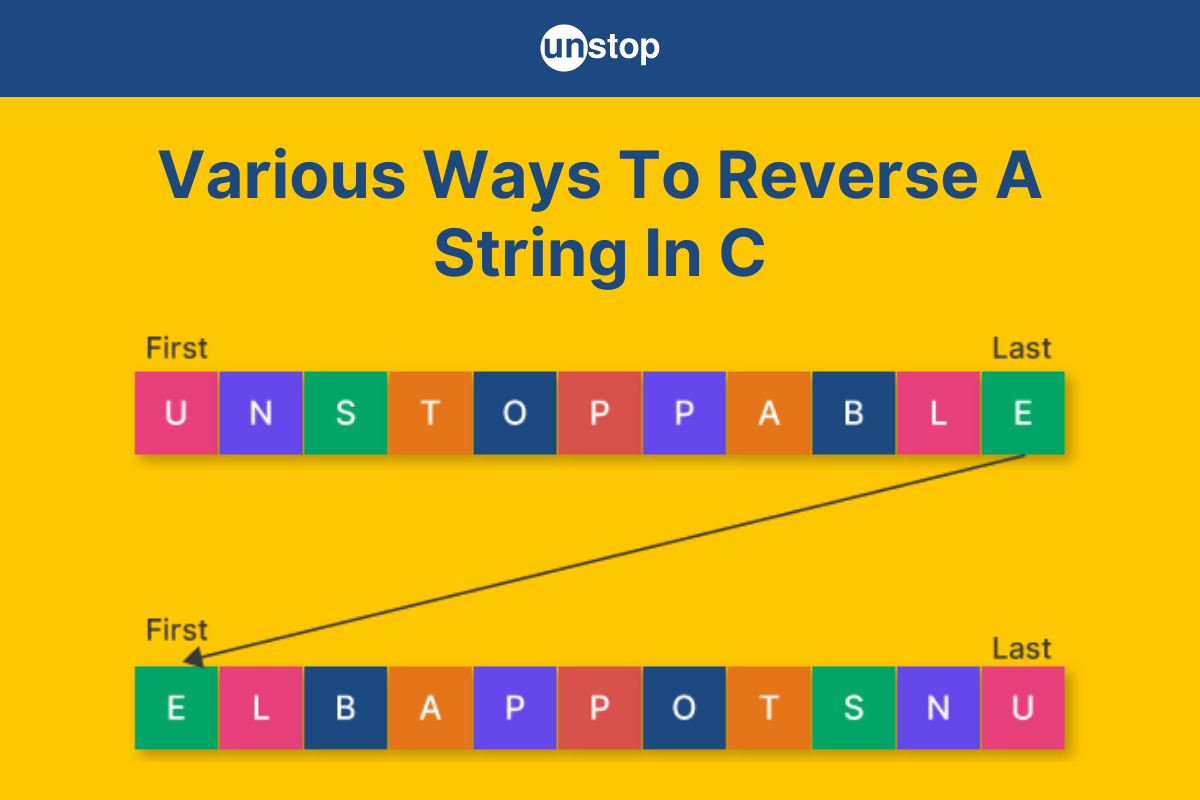
A string is a sequence/ array of characters stored in contiguous memory locations. String reversing is a fundamental operation in programming, and understanding how to perform this task is crucial for any aspiring C programmer. In this article, we'll explore various methods to reverse a string in C programming, catering to both beginners and those looking to enhance their C coding skills.
How To Reverse A String In C?
Reversing a string in C involves rearranging the characters such that their order is reversed. There are two common mechanisms to materialise the reversal of a string. One is to use index values of string characters to traverse the string from both ends and swap the elements till you reach the centre. The other is to create a new string to store the reverse of the string, leaving the original one unchanged. These two mechanisms to reverse a string in C are explained below.
- Auxiliary String Approach: In this approach, the original string remains unchanged, and the reversal/ modification is done on the temporary string. We create a new auxiliary (temporary) string and copy characters from the original string in reverse order.
- In-Place Reversal: In this method, the original string is modified directly to achieve the reversal. We swap characters from both ends of the string towards the middle until the entire string is in the reverse order.
Within these approaches, we can employ various methods to reverse a string in C programming language. We will explore all these methods in the sections below.
Reverse A String In C Using strrev() Function
A straightforward method to reverse a string in C is with the strrev() function. It takes a character array (string) as a parameter and reverses the order of characters in place. The primary purpose of the function is to reverse the string that it takes as a parameter/ argument.
To reverse a string in C using strrev(), you must include the header file containing the function definition, i.e., <string.h>. However, note that it is not part of the standard C library but rather a Microsoft-specific extension. Meaning it may not be available on all platforms and is hence not used frequently.
Syntax:
#include <string.h>
char *strrev(char *str);
Here,
- #include <string.h>: The preprocessor directive to include the standard C library header file for string operations.
- char *: Return data type of the predefined function strrev() indicating that it returns a pointer to a character.
- (char *str): Parameter list indicating the function takes a pointer to a character array (string) that it will reverse.
In short, the function reverses the order of string components/ characters in the provided string, in place. It returns a pointer to the reversed string. Below is simple C program example demostrating how this works.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCmludCBtYWluKCl7CmNoYXIgc3RyWzQwXSA9ICJIZWxsbywgV29ybGQhIjsgLy8gRGVjbGFyaW5nIGFuZCBpbml0aWFsaXppbmcgdGhlIHN0cmluZwpwcmludGYgKCJPcmlnaW5hbCBzdHJpbmc6ICVzXG4iLCBzdHIpOwoKLy8gVXNlIHN0cnJldigpIGZ1bmN0aW9uIHRvIHJldmVyc2UgdGhlIHN0cmluZwpzdHJyZXYoc3RyKTsKcHJpbnRmICgiUmV2ZXJzZWQgc3RyaW5nOiAlc1xuIiwgc3RyKTsKCnJldHVybiAwOwp9
Output:
Original string: Hello, World!
Reversed string: !dlroW ,olleH
Explanation:
In the simple C code example, we include the <stdio.h> and <string.h> header files for input/ output operations and string manipulation functions, respectively.
- In the main() function, we declare a character array str with a size of 40, allowing it to store up to 39 characters (plus the null terminator).
- We assign the value- "Hello, World!" to the string str and display the original string to the console using the printf() library function.
- Here, the %s format specifier is the placeholder for the character array/ string, and the newline escape sequence (\n) shifts the cursor to the next line so that it prints the next output in a new line.
- Next, we call the strrev() function, passing str as an argument. It reverses the characters of the string in place.
- After that, we call the printf() function again to display the reversed string to the console.
- Lastly, the main() function terminates with a return 0 statement, indicating a successful execution.
Note: Since strrev() is not a standard C library function, some compilers might throw a compilation error like this:
main.c: In function ‘main’:
main.c:10:5: warning: implicit declaration of function ‘strrev’; did you mean ‘strsep’? [-Wimplicit-function-declaration]
10 | strrev(str);
| ^~~~~~
| strsep
/usr/bin/ld: /tmp/ccz3Qfmc.o: in function `main':
main.c:(.text+0x72): undefined reference to `strrev'
collect2: error: ld returned 1 exit status
The error indicates that the compiler was confused about the function's definition during the compilation process, and the linker could not find the implementation. Hence, using the strrev() method can be platform-dependent and may not be available in all C environments.
Complexity: The length of the input string determines the complexity of the strrev() function. For a string of length n, it has a linear time complexity of O(n).
Other Ways To Reverse A String In C Without strrev() Function
We've discussed how to use the strrev() function to reverse a string and why it might not be the best approach. There are multiple other ways to write and run programs to reverse a string in C, either in place or by creating a new string. These include:
- For Loop
- While Loop
- Recursion Function
- Using Pointers
- Using a Stack Data Structure
- Printing the String Backwards
- Swapping the Characters of the String
- Creating a New Character Array
Implementing custom string reversal methods provides greater control and flexibility, making it a preferred choice in scenarios where reliance on built-in functions is restricted or discouraged. These methods pose as valuable exercises that enhances programming skills and fosters a deeper understanding of string manipulation algorithms. We will discuss each of these methods to reverse strings in C in greater detail in the sections ahead.
Reverse A String In C Using For Loop
In the for loop approach to reverse a string in C, we iterate through all the characters of the string, swapping the characters at opposite ends. Here is how this works:
- We create a temporary variable (temp) to store the characters of the string (during swapping).
- Using a loop that runs half the length of the string, we exchange the characters at corresponding positions.
- By utilizing a temp variable, the characters are swapped effectively, leading to the gradual reversal of the entire string.
This method allows for an in-place reversal, meaning that the original string is modified directly, resulting in a reversed string. Look at the example C program below, which illustrates the implementation of this method to reverse a string in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCmludCBtYWluKCkgewpjaGFyIGlucHV0U3RyaW5nWzEwMF07CgovLyBJbnB1dCB0aGUgc3RyaW5nCnByaW50ZigiRW50ZXIgYSBzdHJpbmc6ICIpOwpmZ2V0cyhpbnB1dFN0cmluZywgc2l6ZW9mKGlucHV0U3RyaW5nKSwgc3RkaW4pOwoKLy8gUmVtb3ZlIHRoZSBuZXdsaW5lIGNoYXJhY3RlciBmcm9tIHRoZSBpbnB1dCBzdHJpbmcKaW5wdXRTdHJpbmdbc3RyY3NwbihpbnB1dFN0cmluZywgIlxuIildID0gJ1wwJzsKCi8vIEdldCB0aGUgbGVuZ3RoIG9mIHRoZSBzdHJpbmcKaW50IGxlbmd0aCA9IHN0cmxlbihpbnB1dFN0cmluZyk7CgovLyBVc2UgYSBmb3IgbG9vcCB0byBzd2FwIGNoYXJhY3RlcnMgZnJvbSB0aGUgYmVnaW5uaW5nIGFuZCBlbmQKZm9yIChpbnQgaSA9IDA7IGkgPCBsZW5ndGggLyAyOyBpKyspIHsKY2hhciB0ZW1wID0gaW5wdXRTdHJpbmdbaV07CmlucHV0U3RyaW5nW2ldID0gaW5wdXRTdHJpbmdbbGVuZ3RoIC0gMSAtIGldOwppbnB1dFN0cmluZ1tsZW5ndGggLSAxIC0gaV0gPSB0ZW1wOwp9CgovLyBPdXRwdXQgdGhlIHJldmVyc2VkIHN0cmluZwpwcmludGYoIlJldmVyc2VkIHN0cmluZzogJXNcbiIsIGlucHV0U3RyaW5nKTsKCnJldHVybiAwOwp9
Output:
Enter a string: Be Unstoppable
Reversed string: elbappotsnU eB
Explanation:
We begin the example C code by including necessary header files for standard input/output and string manipulation functions like strlen() and strcspn().
- Then, we initiate the main() function, which acts as the program's entry point of execution.
- Inside main(), we declare a string variable/ character array inputString of size 100, which that can store up to 100 characters (size including null terminator).
- Next, we prompt the user to enter a string using printf() function to display a message.
- We then use the fgets() function to read the line of text from standard input (stdin) and store it in the inputString array.
- Here, we use the sizeof() operator to determine the size of the input string, and the function reads [sizeof(inputString) - 1] characters, ensuring that it doesn’t overflow the array. Note that fgets() includes a newline character after the string when the user hits Enter.
- So, we use the strcspn() function which returns the index of first instance of the newline character in inputString, which we then replace with the null termiantor (\0), effectively removing it from the string.
- After that, we call the strlen() function on the inputString to calculate the actual length of the string and store the outcome in the integer type variable length.
- Next, we define a for loop to iterate through the string from 0 (initial value of loop counter variable i) to length/2 (half the number of characters in the string). Inside the loop:
- We create a temporary character variable temp to store the character at the i-th position in the string.
- Then, we assign the character at the [length -1- i]-th position (the corresponding character from the end) to the current position i.
- Next, we store the character in temp variable to the [length -1 -i]-th position, effectively swapping characters from opoosite ends.
- After every iteration, we increment the value of loop count variable i by 1.
- The swapping process continues until half the string is processed, i.e., for (length/2) number of iterations. This effectively reverses the entire string in place.
- After the loop terminates, the inputString variable contains the reversed string, which we display using the printf() statement.
Complexity: For a string of length n, the time complexity of this method is O(n). The for loop iterates through the string once, resulting in a linear time complexity.
Important Note: There are two common built-in functions for reading user input: the gets() and fgets() functions.
- The gets() is dangerous and has been deprecated in modern C standards (C99 and onwards) because it can cause buffer overflows.
- It is recommended to use fgets() instead, which allows you to specify the maximum number of characters to read, preventing overflows. Also, after using fgets(), the input string may contain a newline (\n) which must be removed explicitly.
Check out this amazing course to become the best version of the C programmer you can be.
Reverse A String In C Using While Loop
The while loop approach to reverse a string in C programs involves iterating through the string from both ends towards the centre. In this method:
- We employ a while loop that continues until the start pointer surpasses the end pointer.
- Within each iteration, characters at symmetric positions are swapped using a temporary variable.
- This swapping mechanism effectively reverses the order of characters in the string.
In the while loop, we also increment the start pointer and decrement the end pointer in each iteration, ensuring that the entire string is traversed. Below is a C program example that demonstrates this method.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCmludCBtYWluKCkgewpjaGFyIGlucHV0U3RyaW5nWzEwMF07CgovLyBJbnB1dCB0aGUgc3RyaW5nCnByaW50ZigiRW50ZXIgYSBzdHJpbmc6ICIpOwpmZ2V0cyhpbnB1dFN0cmluZywgc2l6ZW9mKGlucHV0U3RyaW5nKSwgc3RkaW4pOwoKLy8gUmVtb3ZlIHRoZSBuZXdsaW5lIGNoYXJhY3RlciBmcm9tIHRoZSBpbnB1dCBzdHJpbmcKaW5wdXRTdHJpbmdbc3RyY3NwbihpbnB1dFN0cmluZywgIlxuIildID0gJ1wwJzsKCi8vIEdldCB0aGUgbGVuZ3RoIG9mIHRoZSBzdHJpbmcKaW50IGxlbmd0aCA9IHN0cmxlbihpbnB1dFN0cmluZyk7CmludCBzdGFydCA9IDA7CmludCBlbmQgPSBsZW5ndGggLSAxOwoKLy8gVXNlIGEgd2hpbGUgbG9vcCB0byBzd2FwIGNoYXJhY3RlcnMgZnJvbSB0aGUgYmVnaW5uaW5nIGFuZCBlbmQKd2hpbGUgKHN0YXJ0IDwgZW5kKSB7CmNoYXIgdGVtcCA9IGlucHV0U3RyaW5nW3N0YXJ0XTsKaW5wdXRTdHJpbmdbc3RhcnRdID0gaW5wdXRTdHJpbmdbZW5kXTsKaW5wdXRTdHJpbmdbZW5kXSA9IHRlbXA7CgovLyBNb3ZlIHRoZSBpbmRpY2VzIHRvd2FyZHMgdGhlIGNlbnRyZSBvZiB0aGUgc3RyaW5nCnN0YXJ0Kys7CmVuZC0tOwp9CgovLyBPdXRwdXQgdGhlIHJldmVyc2VkIHN0cmluZwpwcmludGYoIlJldmVyc2VkIHN0cmluZzogJXNcbiIsIGlucHV0U3RyaW5nKTsKCnJldHVybiAwOwp9
Output:
Enter a string: Hello, World!
Reversed string: !dlroW ,olleH
Explanation:
In the C code example-
- In the main() function, we declare a character array inputString of size 100 to store user input. The string can store up to 99 characters in length plus a null terminator.
- Then, we use the printf() function to prompt the user to enter a string, followed by the fgets() function to read the input from standard input (stdin) and store it in the inputString array.
- In fgets(), we use the operator sizeof() to ensure that the function reads up to sizeof(inputString) - 1 character, preventing buffer overflow. It's important to note that fgets() includes a newline character when the user presses Enter.
- As mentioned in the code comments, we remove this newline character from the string using the strcspn() function to get index of first occurrence of the character. And then replace that character with the null terminator (\0).
- Next, we calculate the length of the string using the strlen() function and store the result in the integer variable length.
- We also initialize two integer variables (indices): start, which marks the beginning of the string (set to 0), and end, which marks the end of the string (set to length - 1).
- Then, we define a while loop, which iterates through the string as long as the start is less than the end. Inside the loop:
- We create a temporary character variable temp to store the character at the start position in the string.
- Next, we store the character at the end index in the start position and the character in temp at the end position, effectively swapping the characters at the start and end index positions.
- After swapping, we increment the start index and decrement the end index, moving towards the centre of the string.
- This process continues until start is less than end and the loop condition is no longer satisfied, effectively reversing the entire string in place.
- After the loop terminates, the inputString variable contains the reversed string, which we display using the printf() statement.
Complexity: For a string of length n, the time complexity of this method is O(n). The while loop iterates through the string once, resulting in linear time complexity.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Reverse A String In C Using Recursion Function
Recursion is the technique of breaking down a big problem into multiple smaller ones and having a function call itself recursively to solve these problems. This approach to reverse a loop in C involves defining a function that recursively swaps the first and the last characters of a string until the entire string is reversed. In the recursive function method:
- We establish a base case when the length of the string becomes one or less, which signifies an empty string or a single character.
- In each recursive call, the function swaps the first and last characters of the string and progressively moves towards the middle section of the string.
This recursive process continues until the entire string is reversed. It provides an elegant and intuitive solution by reducing the problem into smaller sub-problems, making it a natural fit for recursion. Look at the sample C program below to see the implementaion of this method to rverse a string in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCnZvaWQgcmV2ZXJzZVN0cmluZyhjaGFyICpzdHIsIGludCBzdGFydCwgaW50IGVuZCkgewppZiAoc3RhcnQgPj0gZW5kKSB7CnJldHVybjsgLy8gQmFzZSBjYXNlOiBwb2ludGVycyBoYXZlIGNyb3NzZWQKfQoKLy8gU3dhcCB0aGUgY2hhcmFjdGVycyBhdCBzdGFydCBhbmQgZW5kIHBvc2l0aW9ucwpjaGFyIHRlbXAgPSBzdHJbc3RhcnRdOwpzdHJbc3RhcnRdID0gc3RyW2VuZF07CnN0cltlbmRdID0gdGVtcDsKCi8vIFJlY3Vyc2l2ZSBjYWxsIGZvciB0aGUgbmV4dCBwb3NpdGlvbnMKcmV2ZXJzZVN0cmluZyhzdHIsIHN0YXJ0ICsgMSwgZW5kIC0gMSk7Cn0KCmludCBtYWluKCkgewpjaGFyIHN0clsxMDBdOwpwcmludGYoIkVudGVyIGEgc3RyaW5nOiAiKTsKZmdldHMoc3RyLCBzaXplb2Yoc3RyKSwgc3RkaW4pOwoKLy8gUmVtb3ZlIHRoZSBuZXdsaW5lIGNoYXJhY3RlciBpZiBwcmVzZW50CnN0cltzdHJjc3BuKHN0ciwgIlxuIildID0gJ1wwJzsKCi8vIENhbGwgdGhlIHJlY3Vyc2l2ZSBmdW5jdGlvbiB3aXRoIGluaXRpYWwgaW5kaWNlcwpyZXZlcnNlU3RyaW5nKHN0ciwgMCwgc3RybGVuKHN0cikgLSAxKTsKCi8vIFByaW50IHRoZSByZXZlcnNlZCBzdHJpbmcKcHJpbnRmKCJSZXZlcnNlZCBzdHJpbmc6ICVzXG4iLCBzdHIpOwoKcmV0dXJuIDA7Cn0=
Output:
Enter a string: String Reverse
Reversed string: gnirtS esreveR
Explanation:
In this sample C code:
- We define a function reverseString() to reverse a string using recursion. It takes three parameters: char * (a pointer to a string), start (starting index of the string), and end ( ending index of the string). Inside:
- We use an if-statement to define the base case which checks if value of start is greater than or equal to end (start>=end).
- If this case is met, recursion stops since it means the two pointers have crossed or are in the same position, meaning all characters have been swapped.
- Then, we define what happens in each recursive call, i.e., the character at the start index is swapped with the character at the end index, using a temp variable.
- After swapping, the function calls itself, with the incremented value of start index and decremented value of the end index, moving towards the center of the string, recursively.
- Moving to the main() function, we declare a character array str to store the input string. The size of char string is 100, indicating the number of characters it can have.
- We then prompt the user to input a string using the printf() function and read the input string using the fgets() function.
- Next, we replace the newline character inserted by fgets() with a null terminator using the strcspn() function.
- Then, we call the reverseString() function, passing the string str and the initial indices (0 and strlen(str) - 1), representing the first and last characters of the string as arguments.
- Finally, we print the reversed string using the printf() statement.
Time Complexity: The time complexity is O(n), where n is the length of the string. Each character is swapped once, and the recursion progresses linearly.
Space Complexity: The space complexity is O(n) due to the recursive call stack. For each recursive call, a new function call is added to the stack until the base case is reached.
Reverse A String In C Using Pointers
In this method, pointers are utilized to reverse a string. Pointers are variables that store the address of another variable, allowing direct manipulation of the value in memory. To reverse a string in C using pointers:
- Define two pointers (say start and end) and initialize them to point to the first and last characters of the string, respectively.
- Then, swap the characters at these positions and increment/ decrement the pointer position to move inward, towards the middle, until they meet.
This method is a simple and efficient way to reverse a string. Below is a C program sample to demonstrate this technique.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCnZvaWQgcmV2ZXJzZVN0cmluZyhjaGFyICpzdHIpIHsKY2hhciAqc3RhcnQgPSBzdHI7CmNoYXIgKmVuZCA9IHN0ciArIHN0cmxlbihzdHIpIC0gMTsKCndoaWxlIChzdGFydCA8IGVuZCkgewovLyBTd2FwIGNoYXJhY3RlcnMgdXNpbmcgcG9pbnRlcnMKY2hhciB0ZW1wID0gKnN0YXJ0Owoqc3RhcnQgPSAqZW5kOwoqZW5kID0gdGVtcDsKCi8vIE1vdmUgcG9pbnRlcnMgdG93YXJkcyB0aGUgbWlkZGxlCnN0YXJ0Kys7CmVuZC0tOwp9Cn0KCmludCBtYWluKCkgewpjaGFyIHN0clsxMDBdOwpwcmludGYoIkVudGVyIGEgc3RyaW5nOiAiKTsKCi8vIFVzZSBmZ2V0cyBpbnN0ZWFkIG9mIGdldHMKZmdldHMoc3RyLCBzaXplb2Yoc3RyKSwgc3RkaW4pOwoKLy8gUmVtb3ZlIHRoZSBuZXdsaW5lIGNoYXJhY3RlciBmcm9tIHRoZSBpbnB1dCBzdHJpbmcKc3RyW3N0cmNzcG4oc3RyLCAiXG4iKV0gPSAnXDAnOwoKcmV2ZXJzZVN0cmluZyhzdHIpOwpwcmludGYoIlJldmVyc2VkIHN0cmluZzogJXNcbiIsIHN0cik7CgpyZXR1cm4gMDsKfQ==
Output:
Enter a string: Unstoppable Coder!
Reversed string: !redoC elbappotsnU
Explanation:
In this C code sample-
-
We define a function with the name/ identifer reverseString() that accepts a pointer to a character array pointer as a parameter and reverses the string being pointed to. Inside this function:
- First, we initialize two pointers, start and end, to point to the beginning (str) and end of the string (str + strlen(str) -1), respectively.
- Then, we use a while loop that continues iterations as long as the start is less than the end.
- Within the loop, we swap characters pointed to by start and end using a temporary variable temp.
- After every iteration/ swap, we increment the start ptr and decrement the end ptr to move toward the centre of the string.
- The iterations continue until the two pointers meet in the middle of the string, and the start is no longer less than the end.
- In the main() function, we declare a character array str with a size of 100 to store user input.
- We then prompt the user to enter a string using printf() and read the user input into the str array using the fgets() function. We also replace the newline character from the input string using the strcspn() function.
- After that, we call the reverseString() function, passing the input string as an argument. The function reverses the string by modifying it in place.
- Finally, we use the in-built function printf() to display the reversed string.
Time Complexity: The time complexity is O(n), where n is the length of the string. Each character is accessed and swapped exactly once.
Space Complexity: The space complexity is O(1) since no additional space is used apart from the two pointers and a temporary variable.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Reverse A String In C Using Stack
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. It supports two key operations:
- Push: Adds (pushes) an element onto the top of the stack.
- Pop: Removes (pops) the element from the top of the stack.
In a stack, both insertion and deletion of elements can only happen at one end, known as the top.
When using stack structure to revrse a string in C programs, you first have to push the characters of the string onto the stack in their original order. Then, pop them off the stack, which results in the string being reversed, as elements are retrieved in reverse order.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KI2RlZmluZSBNQVhfU0laRSAxMDAKCnN0cnVjdCBTdGFjayB7CmludCB0b3A7CmNoYXIgaXRlbXNbTUFYX1NJWkVdOwp9OwoKdm9pZCBwdXNoKHN0cnVjdCBTdGFjayAqc3RhY2ssIGNoYXIgaXRlbSk7CmNoYXIgcG9wKHN0cnVjdCBTdGFjayAqc3RhY2spOwp2b2lkIHJldmVyc2VTdHJpbmcoY2hhciAqc3RyKTsKCmludCBtYWluKCkgewpjaGFyIHN0cltNQVhfU0laRV07CnByaW50ZigiRW50ZXIgYSBzdHJpbmc6ICIpOwoKLy8gVXNlIGZnZXRzIGluc3RlYWQgb2YgZ2V0cwpmZ2V0cyhzdHIsIHNpemVvZihzdHIpLCBzdGRpbik7CgovLyBSZW1vdmUgdGhlIG5ld2xpbmUgY2hhcmFjdGVyIGZyb20gdGhlIGlucHV0IHN0cmluZwpzdHJbc3RyY3NwbihzdHIsICJcbiIpXSA9ICdcMCc7CgpyZXZlcnNlU3RyaW5nKHN0cik7CnByaW50ZigiUmV2ZXJzZWQgc3RyaW5nOiAlc1xuIiwgc3RyKTsKCnJldHVybiAwOwp9Cgp2b2lkIHB1c2goc3RydWN0IFN0YWNrICpzdGFjaywgY2hhciBpdGVtKSB7CmlmIChzdGFjay0+dG9wID09IE1BWF9TSVpFIC0gMSkgewpwcmludGYoIlN0YWNrIE92ZXJmbG93XG4iKTsKcmV0dXJuOwp9CgpzdGFjay0+aXRlbXNbKytzdGFjay0+dG9wXSA9IGl0ZW07Cn0KCmNoYXIgcG9wKHN0cnVjdCBTdGFjayAqc3RhY2spIHsKaWYgKHN0YWNrLT50b3AgPT0gLTEpIHsKcHJpbnRmKCJTdGFjayBVbmRlcmZsb3dcbiIpOwpyZXR1cm4gJ1wwJzsKfQpyZXR1cm4gc3RhY2stPml0ZW1zW3N0YWNrLT50b3AtLV07Cn0KCnZvaWQgcmV2ZXJzZVN0cmluZyhjaGFyICpzdHIpIHsKaW50IGxlbmd0aCA9IHN0cmxlbihzdHIpOwoKLy8gQ3JlYXRlIGEgc3RhY2sKc3RydWN0IFN0YWNrIHN0YWNrOwpzdGFjay50b3AgPSAtMTsKCi8vIFB1c2ggY2hhcmFjdGVycyBvbnRvIHRoZSBzdGFjawpmb3IgKGludCBpID0gMDsgaSA8IGxlbmd0aDsgaSsrKSB7CnB1c2goJnN0YWNrLCBzdHJbaV0pOwp9CgovLyBQb3AgY2hhcmFjdGVycyBmcm9tIHRoZSBzdGFjayB0byByZXZlcnNlIHRoZSBzdHJpbmcKZm9yIChpbnQgaSA9IDA7IGkgPCBsZW5ndGg7IGkrKykgewpzdHJbaV0gPSBwb3AoJnN0YWNrKTsKfQp9
Output:
Enter a string: Hello World
Reversed string: dlroW olleH
Explanation:
We begin the C code example by including necessary header files for standard input/output and string manipulation functions.
- Then, we define a macro MAX_SIZE to represent the maximum size of the stack with the value 100.
- Using the struct keyword, we then define a structure called Stack to represent a stack data structure. The structure consists of two members: a top to track the index of the stack's top element and an array items[] to store the characters of the string.
- Next, we declare three functions, i.e., push(), pop(), and reverseString() before the main part and their definition is given later.
- In the main() function, we declare a character array str with a size given by MAX_SIZE to store user input.
- Next, we prompt the user to enter a string using printf() and use fgets() to read the user input and store it in the str array. We also remove the newline character from the input string using the strcspn() function.
- We then call the reverseString() function, passing the input string as an argument. This function uses a stack to reverse the original string.
- Once the function reverses the string, we print the reversed string using printf() in the main function. In this example, we have used the string value- "Hello, World!" as shown in the output.
- The purpose of the push() function is to add a character to the stack. Inside:
- We use an if conditional/ control statement to check whether the stack is full, i.e., stack->top == MAX_SIZE -1. Here, we use the 'this' pointer to access the respective stack element and the equality relational operator in the condition.
- If the condition is false, there is no overflow, and the stack is not full. The function then adds a character from the string to the stack, and the top index is incremented.
- If the condition is true, it means there is stack overflow (stack is full) and the function prints a message to the effect.
- The pop() function is defined to remove an element from the stack as follows:
- First, we check if the stack is empty, i.e., top == -1. If the condition is true, it means the stack is empty, and the function returns a null terminator.
- If the condition is false, meaning the stack is not empty, the function removes and returns the character at the top of the stack. After this, the top index is decremented.
- The reverseString() function accepts a pointer to the string and handles the logic of reversing the string using stack operations:
- We first calculate the length of the string using the strlen() function and store it in the int variable length.
- Then, we create an instance of the Stack called stack and initialize the top member with the value -1.
- After that, we use a for loop and the push() operation to add each character of the string onto the stack in proper order.
- Following this, we remove the character from the top of the stack using the for loop and pop() operation and place them back in the string.
- The string’s characters are pushed one by one onto the stack in their original order. Then, the characters are popped from the stack and placed back into the string, which effectively reverses the string (since the stack follows LIFO).
Complexity: The time complexity of this approach is O(n), where n is the length of the string. Both pushing and popping each character happen in linear time.
Reverse A String In C By Printing It Backward
A simple and efficient way to reverse a string in C is by printing it backwards. In this method, we iterate through the string from the end to the beginning and print each character one by one.
This approach doesn't modify the original string; instead, it prints the reversed version directly to the output. The method is particularly useful when you want to display or analyze the reversed string without altering its contents. Additionally, it avoids the need for any extra data structures or complex logic, making it a clear and concise solution.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCnZvaWQgcmV2ZXJzZVN0cmluZyhjaGFyICpzdHIpOwoKaW50IG1haW4oKSB7CmNoYXIgc3RyWzEwMF07CnByaW50ZigiRW50ZXIgYSBzdHJpbmc6ICIpOwoKZ2V0cyhzdHIpOwpyZXZlcnNlU3RyaW5nKHN0cik7CgpyZXR1cm4gMDsKfQoKdm9pZCByZXZlcnNlU3RyaW5nKGNoYXIgKnN0cikgewppbnQgbGVuZ3RoID0gc3RybGVuKHN0cik7CnByaW50ZigiUmV2ZXJzZWQgc3RyaW5nOiAiKTsKZm9yIChpbnQgaSA9IGxlbmd0aCAtIDE7IGkgPj0gMDsgaS0tKSB7CnByaW50ZigiJWMiLCBzdHJbaV0pO30KcHJpbnRmKCJcbiIpOwp9
Output:
Enter a string: Hello World
Reversed string: dlroW olleH
Explanation:
In the C example above:
- We declare a reverseString() function that takes a character pointer to the string (char *str) as a parameter. The function definition is given after main().
- In the main() function, we declare a character array str with a capacity of 100 characters.
- Then, we prompt the user to input a string value using printf() and read the same using fgets() function. We also remove the newline character introduced by fgets() using the strcspn() function to ensure the string prints cleanly.
- After that, we call the reverseString() function, passing the initial string str as a parameter.
- The function then prints the reversed string to the console, as shown in the output above.
- Inside the reverseString() function:
- We first use the strlen() function calulate the lenght of the string and store the outcome in the variable length.
- Then, we use a for loop to iterate through the string starting from the last index position, i.e., length-1.
- In every iteration, the printf() statement displays the character at the respective position, and the value of the loop counter variable is decremented by 1.
- The loop continues iteration until it reaches the beginning of the string, i.e., i>=0.
- After printing all the characters, a newline character is printed for proper formatting.
- This example shows a simple, non-destructive method to reverse a string in C. The original string remains unchanged, and the reversed version is only printed, making this ideal for cases where you don’t want to modify the original data.
Time Complexity: The time complexity of this approach is O(n), where n is the length of the string. The loop iterates over each character of the string once in reverse order, resulting in a linear time complexity.
Reverse A String In C With User-Defined Function To Swap Characters
Swapping characters is one of the most basic and effective ways to reverse a string in C programs. The essence of this technique is to swap the first character with the last character, the second with the second-to-last, and so on until the middle of the string is reached. We have already seen a form of this mechanism in other methods like loops, recursion, and pointers.
This approach can also be modularized by using a user-defined function to perform the swapping logic. By breaking the problem into a function, we enhance the readability and maintainability of the code, making it easier to reuse and debug. Below is a basic C example, showcasing how toreverse a string using the swapping technique, encapsulated in a user-defined function.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCnZvaWQgcmV2ZXJzZVN0cmluZyhjaGFyICpzdHIpIHsKaW50IGxlbmd0aCA9IHN0cmxlbihzdHIpOwpmb3IgKGludCBpID0gMDsgaSA8IGxlbmd0aCAvIDI7IGkrKykgewovLyBTd2FwIGNoYXJhY3RlcnMKY2hhciB0ZW1wID0gc3RyW2ldOwpzdHJbaV0gPSBzdHJbbGVuZ3RoIC0gMSAtIGldOwpzdHJbbGVuZ3RoIC0gMSAtIGldID0gdGVtcDt9Cn0KCmludCBtYWluKCkgewpjaGFyIHN0clsxMDBdOwpwcmludGYoIkVudGVyIGEgc3RyaW5nOiAiKTsKZ2V0cyhzdHIpOwoKcmV2ZXJzZVN0cmluZyhzdHIpOwpwcmludGYoIlJldmVyc2VkIHN0cmluZzogJXNcbiIsIHN0cik7CgpyZXR1cm4gMDsKfQ==
Output:
Enter a string: Hello World
Reversed string: dlroW olleH
Explanation:
In the example above-
- We define a reverseString function, which takes a single parameter, i.e., a character pointer to a string.
- The function first calculates the length of the string using the strlen() function and stores it in the length variable.
- Next, we use a for loop, which iterates through the initial string with the control variable i set to 0. The loop continues until the value of i is less than half the length.
- In every iteration, the character at the ith position is stored to a variable temp, and the character at (length-1-i)th position is stored at the ith position. Then, the value in the temp variable is resigned to the (length-1-i)th position.
- This is how the loop swaps the characters inside the string. After every iteration, the value of i is incremented by 1.
- Then, in the main() function, we declare a character array str with a capacity of 100 characters.
- Next, we prompt the user to input a string using printf() and read the input string using gets() function. (As mentioned above, it is safer and recommended to use fgets() instead of gets(). The compiler may show an error message when using gets().)
- After that, we call the reverseString() function, which effectively reverses the order of characters in the string.
- Then, using a printf() statement, we display the reversed/ output string to the console.
Time Complexity: The time complexity is O(n), where n is the length of the string. The loop runs for half the length of the string, but this still simplifies to O(n).
Space Complexity: The space complexity is O(1) because only a few extra variables (temp, i, etc.) are used regardless of the string size.
Reverse A String In C Using New Character Array
In this method to reverse of a string in C, you have to create a separate array to store the reversed version of the original array/ string. You the iterate through the original string in a forward direction and simultaneously populate the new array in reverse order.
This method effectively constructs the reversed string in the new array. One key advantage of this approach is that it preserves the original string, as the reversal is done in a new array.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCnZvaWQgcmV2ZXJzZVN0cmluZyhjaGFyICpzdHIpIHsKaW50IGxlbmd0aCA9IHN0cmxlbihzdHIpOwpjaGFyIHJldmVyc2VkU3RyW2xlbmd0aCArIDFdOyAvLyArMSBmb3IgbnVsbCB0ZXJtaW5hdG9yCgpmb3IgKGludCBpID0gMDsgaSA8IGxlbmd0aDsgaSsrKSB7CnJldmVyc2VkU3RyW2ldID0gc3RyW2xlbmd0aCAtIDEgLSBpXTsKfQpyZXZlcnNlZFN0cltsZW5ndGhdID0gJ1wwJzsgLy8gTnVsbC10ZXJtaW5hdGUgdGhlIHJldmVyc2VkIHN0cmluZwpzdHJjcHkoc3RyLCByZXZlcnNlZFN0cik7IC8vIENvcHkgcmV2ZXJzZWQgc3RyaW5nIGJhY2sgdG8gb3JpZ2luYWwKfQoKaW50IG1haW4oKSB7CmNoYXIgc3RyWzEwMF07CnByaW50ZigiRW50ZXIgYSBzdHJpbmc6ICIpOwpmZ2V0cyhzdHIsIHNpemVvZihzdHIpLCBzdGRpbik7CnN0cltzdHJjc3BuKHN0ciwgIlxuIildID0gJ1wwJzsgLy8gUmVtb3ZlIG5ld2xpbmUgY2hhcmFjdGVyIGlmIHByZXNlbnQKCnJldmVyc2VTdHJpbmcoc3RyKTsKcHJpbnRmKCJSZXZlcnNlZCBzdHJpbmc6ICVzXG4iLCBzdHIpOwoKcmV0dXJuIDA7Cn0=
Output:
Enter a string: Hello World
Reversed string: dlroW olleH
Explanation:
- First, we define a function named reverseString that takes a character array as input and reverses it. Inside the function:
- We calculate the length of the input string using the strlen() function and store it in the length variable.
- Then, we declare a new array reversedStr to store the reversed string, which has the length of input string plus 1, for null termiantor.
- After that, we use a for loop to iterate through the characters of the input string. Within the loop, we assign the characters from the original string in reverse order to the reversedStr array. That is, the character at i-th index in input string is assigned to the character at (length-1-0) index position.
- We ensure the reversed string is null-terminated by setting the last character of reversedStr to '\0'. This completes the operation of reversing the input string which is stored in reversedString variable.
- For this example, we use the strcpy() function to copy the reversed string back into the original string. You can skip this step if you want two individual strings that are in reverse of each other.
- Inside the main() function, we first declare a character array str with a size of 100 to store user input.
- We then prompt the user to enter a string using the printf() function, read it using the fgets() function, and store it in the str array.
- Also, we replace the newline character that might have been added to the string by fgets() using the strcspn() function.
- Next, we call the reverseString() function, passing the input string as an argument. This modifies the original string to be its reverse.
- After that, we print the reversed string to the console using a printf() statement.
Complexity: The time complexity of the above method is O(n), where n is the length of the string.
C Program To Check If Reverse String Is A Palindrome
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward as backward. In other words, a palindrome string retains its original meaning even when its characters are reversed. In this method, we check whether a given string is a palindrome by comparing the original string to its reversed version. If all corresponding characters match, the string is classified as a palindrome.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCmludCBpc1BhbGluZHJvbWUoY2hhciAqc3RyKTsKCmludCBtYWluKCkgewpjaGFyIHN0clsxMDBdOwpwcmludGYoIkVudGVyIGEgc3RyaW5nOiAiKTsKZ2V0cyhzdHIpOwoKaWYgKGlzUGFsaW5kcm9tZShzdHIpKSB7CnByaW50ZigiVGhlIHN0cmluZyBpcyBhIHBhbGluZHJvbWUuXG4iKTsKfSBlbHNlIHsKcHJpbnRmKCJUaGUgc3RyaW5nIGlzIG5vdCBhIHBhbGluZHJvbWUuXG4iKTt9CnJldHVybiAwOwp9CgppbnQgaXNQYWxpbmRyb21lKGNoYXIgKnN0cikgewppbnQgbGVuZ3RoID0gc3RybGVuKHN0cik7CmZvciAoaW50IGkgPSAwOyBpIDwgbGVuZ3RoIC8gMjsgaSsrKSB7CmlmIChzdHJbaV0gIT0gc3RyW2xlbmd0aCAtIDEgLSBpXSkgewpyZXR1cm4gMDsgLy8gTm90IGEgcGFsaW5kcm9tZQp9Cn0KcmV0dXJuIDE7IC8vIFBhbGluZHJvbWUKfQ==
Output:
Enter a string: radar
The string is a palindrome.
Explanation:
We begin the C program by including necessary header files <stdio.h> for standard input and output and <string.h> for string manipulation functions.
- First, we declare a function named isPalindrome, which takes a character array as input and returns an integer to indicate whether the string is a palindrome.
- In the main() function, we declare a character array str with a size of 100 to store user input.
- Then, we prompt the user to enter a string using the printf() function and use the gets() function to read the user input into the str array. (Note that safer alternatives to read input like fgets() are recommended).
- We then call the isPalindrome() function inside an if-else statement with the input string. The condition checks if the string is a palindrome using the function.
- If the condition is true, i.e., the function returns 1, the if-block is executed. If the condition is false, i.e., the function returns 0, then the else-block is executed.
- Inside the isPalindrome() function definition:
-
- We calculate the length of the string using strlen() function and store it in the length variable.
- Then, we use a for loop to compare characters at corresponding positions from the beginning and the end.
- If any characters differ (even if it is a single element), the function returns 0, indicating the string is not a palindrome.
- If all characters match, the function returns 1, confirming the string is a palindrome.
Complexity: The time complexity of this approach to reverse a string in C language is O(n/2), which simplifies to O(n), where n is the length of the string.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
We can reverse a string in C using various methods, each with its own advantages. Whether you use a temporary array/ variable, recursion, or an in-place swap, understanding these techniques will enhance your understanding of string manipulation in C. The choice of method depends on the specific use case, balancing memory efficiency and ease of implementation. By experimenting with these examples, you'll deepen your skills as a C programmer.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Why is there no reverse method in string?
In languages like C, there is no inbuilt function/ reverse method for strings as part of the standard library. The absence of the reverse function method can be attributed to several factors:
- Efficiency Considerations: Reversing a string involves rearranging characters in reverse order. Different algorithms exist for accomplishing this task, and the most efficient algorithm might vary based on the specific use case. A generic reverse method might not be optimized for all scenarios.
- Memory Management: Reversing a string in place (i.e., without using additional memory) can be more memory-efficient, especially when dealing with large strings. A generic method to reverse a string in C might not cater to specific memory management requirements.
- Language Design Philosophy: Some programming languages prioritize simplicity and minimalism in their standard libraries. Including a reverse method might be seen as adding unnecessary complexity, given that the task can be achieved with standard programming constructs.
- Flexibility for Custom Implementations: Not having a built-in method to get the reverse of a string in C allows developers to implement their own custom reverse logic based on specific requirements or optimizations.
- Security Considerations: String reversal involves modifying the original string. In some languages, allowing direct in-place modification might raise security concerns, as it could potentially lead to unintended side effects or vulnerabilities.
Q. What are the different approaches to reverse a string in C?
There are several approaches to reverse a string in C, each with its own merits and use cases.
- In-place reversal with pointers/ indices: This method entails traversing the string from both ends towards the middle and swapping corresponding characters along the way. This method is memory-efficient as it doesn’t require additional space.
- Recursion: This approach to reverse a string in C entails breaking down string reversal into smaller subproblems by reversing smaller portions/ substrings.
- New character array: Creating a new string and copying characters in reverse order is a straightforward method. However, this approach requires additional memory.
- Stack-based reversal: It involves pushing characters onto a stack and then popping them off in the reverse order, to achieve reversal.
The choice of method to get the reverse of a string in C depends on factors like memory constraints, desired time complexity, and the specific problem you are trying to solve.
Q. How can I efficiently reverse a string in C without using extra memory?
Reversing a string without using extra memory means modifying the original string in-place. This approach avoids additional memory allocation while performing the reversal. One common approach to get this done is to use pointers or indices to traverse the string from both ends toward the middle, swapping corresponding characters as you go. Below is an example of the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCnZvaWQgcmV2ZXJzZVN0cmluZyhjaGFyICpzdHIpIHsKaW50IGxlbmd0aCA9IHN0cmxlbihzdHIpOwppbnQgc3RhcnQgPSAwOwppbnQgZW5kID0gbGVuZ3RoIC0gMTsKCndoaWxlIChzdGFydCA8IGVuZCkgewovLyBTd2FwIGNoYXJhY3RlcnMKY2hhciB0ZW1wID0gc3RyW3N0YXJ0XTsKc3RyW3N0YXJ0XSA9IHN0cltlbmRdOwpzdHJbZW5kXSA9IHRlbXA7CgovLyBNb3ZlIHBvaW50ZXJzIHRvd2FyZHMgdGhlIG1pZGRsZQpzdGFydCsrOwplbmQtLTt9Cn0KCmludCBtYWluKCkgewpjaGFyIHN0cltdID0gIlVuc3RvcCI7CnByaW50ZigiT3JpZ2luYWwgc3RyaW5nOiAlc1xuIiwgc3RyKTsKCnJldmVyc2VTdHJpbmcoc3RyKTsKcHJpbnRmKCJSZXZlcnNlZCBzdHJpbmc6ICVzXG4iLCBzdHIpOwoKcmV0dXJuIDA7Cn0=
Output:
Original string: Unstop
Reversed string: potsnU
Explanation:
In this example, we define a reverseString() function that accepts the pointer to a character array (char *str) and reverses it in-place. The start pointer is initially at the beginning of the string, and the end pointer is initially at the end. We use a while loop to swap characters at the start and end positions and move pointers towards the middle until they meet.
The function swaps characters at these positions and moves the pointers towards the middle until they meet. This approach efficiently reverses the string with a time complexity of O(n) and uses no extra memory.
Q. Why is the gets() function discouraged for string input in C?
The gets() function is discouraged in C because it poses serious security vulnerabilities/ risks:
- The primary issue lies in the lack of bounds checking, which makes it susceptible to buffer overflow attacks.
- The function reads input from the standard input (keyboard) until a newline character is encountered and stores it in the provided buffer.
- However, without specifying the size of the buffer, the gets() function has no way to limit the number of characters read.
- If the input exceeds the size of the buffer, it may overwrite adjacent memory, leading to undefined behaviour, crashes, or exploitation by attackers to inject malicious code.
Q. What is the time complexity of string reversal algorithms in C?
The time complexity of the various algorithms to reverse a string C depends on the approach used:
- In-place reversal algorithms using pointers or indices typically have a time complexity of O(n), where n is the length of the string. This method is efficient as it only requires traversing the string once.
- Algorithms that reverse a string by creating a new string involve copying the characters in reverse order. This also has a time complexity of O(n), with n indicating the length of the string. However, this string algorithm uses additional memory for the new string.
The time complexity in either approach is linear, but in-place reversal is more memory-efficient.
Q. How to compare two strings in C?
The strcmp() is the C library function from the <string.h> header, used to compare two strings lexicographically (based on the ASCII values of the characters). It returns an integer value that indicates the result of the comparison. Given below is an example code and an explanation of how to compare two strings in C using strcmp() function.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCmludCBtYWluKCkgewpjaGFyIHN0cjFbXSA9ICJIZWxsbyI7CmNoYXIgc3RyMltdID0gIldvcmxkIjsKCmludCByZXN1bHQgPSBzdHJjbXAoc3RyMSwgc3RyMik7CgppZiAocmVzdWx0IDwgMCkgewpwcmludGYoInN0cjEgaXMgbGVzcyB0aGFuIHN0cjJcbiIpOwp9IGVsc2UgaWYgKHJlc3VsdCA+IDApIHsKcHJpbnRmKCJzdHIxIGlzIGdyZWF0ZXIgdGhhbiBzdHIyXG4iKTsKfSBlbHNlIHsKcHJpbnRmKCJzdHIxIGlzIGVxdWFsIHRvIHN0cjJcbiIpOwp9CgpyZXR1cm4gMDsKfQ==
Output:
str1 is less than str2
Explanation:
This C code compares two strings, "Hello" and "World," using the strcmp() function. The result variable holds the comparison result:
- If the result is less than 0, it means str1 is lexicographically smaller than str2.
- If the result is greater than 0, it means str1 is lexicographically greater than str2.
- If the result is 0, it means str1 is equal to str2. The program then prints the appropriate message based on the comparison result.
In this case, the program prints that str1 ("Hello") is less than str2 ("World"), as "H" comes before "W" in ASCII.
We discussed multiple ways to get the reverse of a string in C in this article. Here are a few other informative articles you must read:
-
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
- Array Of Pointers In C & Dereferencing With Detailed Examples
- Ternary (Conditional) Operator In C Explained With Code Examples
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
- Bitwise Operators In C Programming Explained With Code Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment