Table of content:
- How Are Two Strings Compared In C++?
- Ways to Compare Strings in C++
- Comparing Two Strings Using strcmp() Function in C++
- Comparing Strings In C++ Using compare() Function
- Comparing Strings In C++ Using Relational Operators
- Examples Of String Compare In C++
- This String is Less than Other String
- One String Is Greater Than Other String
- Single Program To Compare Two Strings In C++
- The compare() Function Return Value
- The strcmp() Function: Parameters, Return Value & Undefined Behaviour
- Conclusion
- FAQs
String Compare In C++ | Learn To Compare Strings With Examples
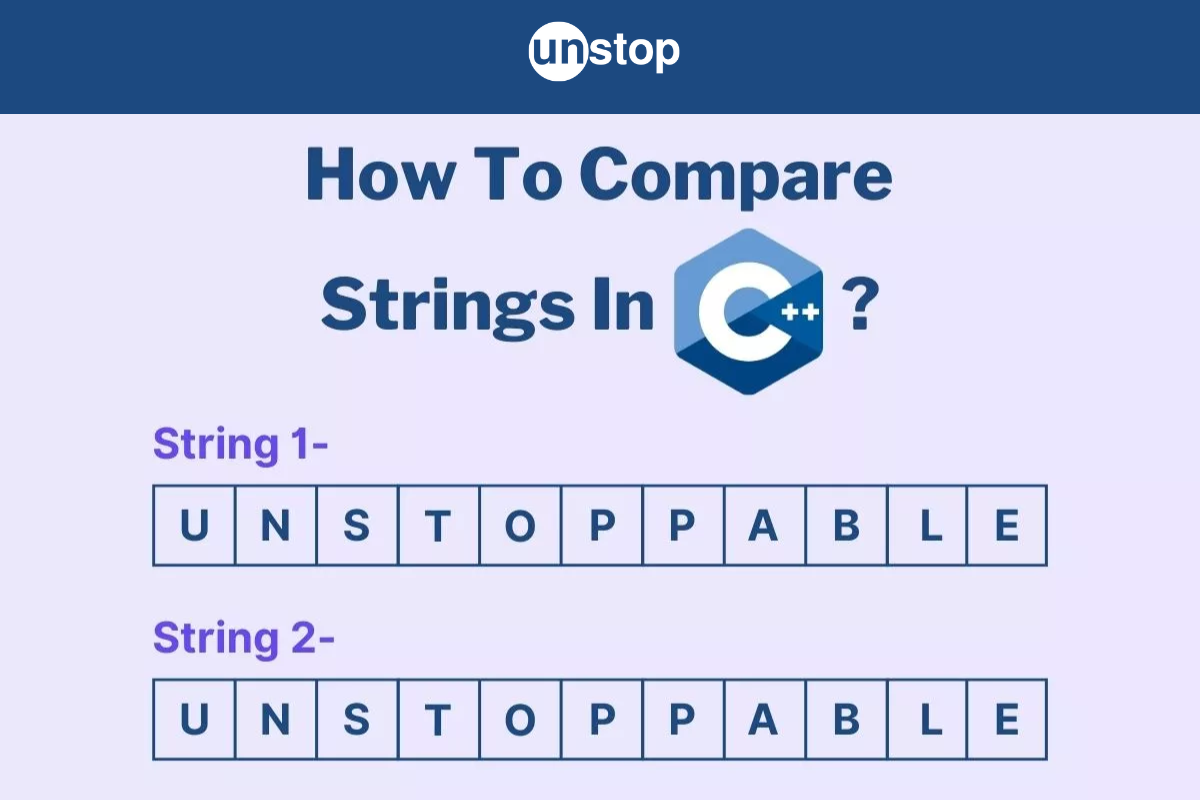
String comparison is an essential and commonly used case in programming. An easy way to understand this is to look at a use case where say, you are creating a password. There are two fields: "password" and "confirm password." We all know that both these must be the same for successful password creation. Now think about what happens behind the scenes here; that is, the respective portal must use a function to compare the sequence of characters specified in the two cases. This is a representation of string comparison. In this article, we will explore this very concept, i.e., string compare in C++. We'll look at various methods to compare strings in C++, the intuition behind the process, and more with the help of detailed examples.
How Are Two Strings Compared In C++?
Before proceeding further, it is essential to understand how two strings are compared. Firstly note that strings in C++ are stored in arrays and have array indexes. A comparison between two strings is done by checking each character from left to right. And we can only compare two strings when they are the same length. For example, take two strings of equal length, string S1 - "slicer" and string S2- "sliced". At index 0, the first character of the two strings is "s," which is the same, so we'll move forward. Moving on, the second, third, fourth, and fifth characters are all the same in both strings. But the sixth character in the first string is "r," whereas, in the second string, it is "d", so the two strings are different.
Note: Every character has its own ASCII value; that's why we can compare strings easily. Also, since upper-case letters have different ASCII values than lower-case letters, string comparison is case-sensitive. This means that Cat and cat are not a string match.
Ways to Compare Strings in C++
There are three commonly used approaches to compare strings in C++, they are:
- Using the strcmp() function in C++
- Using compare() function
- Using C++ relational operators
Let's have a look at these approaches and understand them with the help of examples.
Comparing Two Strings Using strcmp() Function in C++
The header file <string.h> in C++ consists of a pre-defined function called the string compare function i.e., strcmp(). This strcmp() function uses lexicographical comparison to compare two strings. The syntax for this is given below.
Syntax
int strcmp ( const char *str1, const char *str2 );
Parameters
str1: First string
str2: Second string
Return
If the two strings are identical, the program conducting the comparison of strings will return- 'strings are equal'. Otherwise, it will return- 'strings are not equal'.
Now let's have a look at a C++ program that shows the implementation of a string comparison algorithm.
Code Example:
I2luY2x1ZGU8aW9zdHJlYW0+CgojaW5jbHVkZTxzdHJpbmcuaD4KCnVzaW5nIG5hbWVzcGFjZSBzdGQ7CgppbnQgbWFpbigpCgp7CgpjaGFyIHN0cjFbNTBdLCBzdHIyWzUwXTsKCmludCBsZW4xLCBsZW4yOwoKY291dDw8IkVudGVyIHRoZSBGaXJzdCBTdHJpbmc6ICI7CgpjaW4+PnN0cjE7Cgpjb3V0PDwiRW50ZXIgdGhlIFNlY29uZCBTdHJpbmc6ICI7CgpjaW4+PnN0cjI7CgpsZW4xID0gc3RybGVuKHN0cjEpOwoKbGVuMiA9IHN0cmxlbihzdHIyKTsKCmlmKGxlbjE9PWxlbjIpCgp7CgppZihzdHJjbXAoc3RyMSwgc3RyMik9PTApCgpjb3V0PDwiXG5TdHJpbmdzIGFyZSBFcXVhbCI7CgplbHNlCgpjb3V0PDwiXG5TdHJpbmdzIGFyZSBub3QgRXF1YWwiOwoKfQoKZWxzZQoKY291dDw8IlxuU3RyaW5ncyBhcmUgbm90IEVxdWFsIjsKCmNvdXQ8PGVuZGw7CgpyZXR1cm4gMDsKCn0=
String Input 1
Enter the First String: unstop
Enter the Second String: unstopProgram Output 1
Strings are Equal
String Input 2
Enter the First String: slicer
Enter the Second String: slicedProgram Output 2
Strings are not Equal
Explanation
We have taken the two strings as input from users and then found out the lengths of both strings. If the length is the same, the strcmp() function has been used to compare strings. Print strings are equal if the strings are similar; otherwise, print strings are not equal.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Comparing Strings In C++ Using compare() Function
In C++, to compare two strings, we use the compare function. It is a built-in function of C++. This function evaluates the string object's value based on the string of characters specified by its parameter.
Syntax
int compare (const string &str) const;
Parameters
str1: First string
str2: Second string
Return
After comparing the two strings, if-
It returns zero, then it means that both strings are equal
It does not return zero, then it indicates that the strings are not equal.
It provides a positive value, which means string str1 is greater than the str2
It provides a negative value, which means string str1 is less than str2
Code Example
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluICgpCgp7CgpzdHJpbmcgc3RyMSwgc3RyMjsKCmNvdXQgPDwgIiBFbnRlciB0aGUgZmlyc3Qgc3RyaW5nOiAiOwoKY2luID4+IHN0cjE7Cgpjb3V0IDw8ICIgRW50ZXIgdGhlIHNlY29uZCBzdHJpbmc6ICI7CgpjaW4gPj4gc3RyMjsKCgoKCmludCBqID0gc3RyMS5jb21wYXJlKHN0cjIpOwoKaWYgKCBqIDwgMCkKCnsKCmNvdXQgPDwgc3RyMSA8PCAiIGlzIHNtYWxsZXIgdGhhbiAiIDw8IHN0cjIgPDwgIiBzdHJpbmciIDw8IGVuZGw7Cgp9CgplbHNlIGlmICggaiA+IDApCgp7Cgpjb3V0IDw8IHN0cjEgPDwgIiBpcyBncmVhdGVyIHRoYW4gIiA8PCBzdHIyIDw8ICIgc3RyaW5nLiIgPDwgZW5kbDsKCn0KCmVsc2UgLy8gaiA9PSAwOwoKewoKY291dCA8PCAiIFR3byBzdHJpbmdzIGFyZSBlcXVhbC4iOwoKfQoKcmV0dXJuIDA7Cgp9
Input1
Enter the first string: unstop
Enter the second string: unstopOutput1
Two strings are equal
Input2
Enter the first string: ruler
Enter the second string: ruledOutput2
ruled is greater than ruler string
Input3
Enter the first string: peeled
Enter the second string: peelerOutput3
peeled is smaller than peeler string.
Explanation
We compare the two strings by putting the compare() function to use after collecting user input for both strings and printing the output. Since the strings in the first example are identical, you can see that j includes 0. The second string is greater than the first string in the following example because j holds a value greater than zero. The last example indicates that string one is smaller than string two since j contains a value that is less than zero.
Comparing Strings In C++ Using Relational Operators
In C++, we can compare two strings by using the relational operator as well. Relational operators are ones that take two operands, conduct a comparison between them, and then return boolean values. In this section, we will see how to compare strings in C++ by using 2 such operators, i.e.
- The “==” (equal to) operator, and
- The “!=” (not equal to) operator
Syntax for these is as follows:
String1 == string2
Or
String1 != string2
Parameters
string1: First string
string2: Second string
Return
- The “==” operator returns true if both strings are the same. Otherwise, it returns false.
- The “!=” operator returns true when both the strings are different. Otherwise, it returns false.
Now, in order to understand better, let's look at some examples.
Code Example: Compare two strings using the “==” operator
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmludCBtYWluICgpCgp7CgpzdHJpbmcgc3RyMTsKCnN0cmluZyBzdHIyOwoKCgoKY291dCA8PCAiIEVudGVyIHRoZSBmaXJzdCBTdHJpbmc6ICIgPDwgZW5kbDsKCmNpbiA+PiBzdHIxOwoKY291dCA8PCAiIEVudGVyIHRoZSBzZWNvbmQgU3RyaW5nOiAiIDw8IGVuZGw7CgpjaW4gPj4gc3RyMjsKCmlmICggc3RyMSA9PSBzdHIyKQoKewoKY291dCA8PCAiIEJvdGggU3RyaW5ncyBhcmUgZXF1YWwuIiA8PCBlbmRsOwoKfQoKZWxzZQoKewoKY291dCA8PCAiIFN0cmluZyBpcyBub3QgZXF1YWwuIiA8PCBlbmRsOwoKfQoKcmV0dXJuIDA7Cgp9
Input1
Enter the first String: programming
Enter the second String: programmingOutput1
Both Strings are equal.
Input2
Enter the first String: unstop
Enter the second String: unstoppableOutput2
String is not equal
Explanation
Here, we take both strings from the user and compare them using the == (i.e., equal to) operator. In the first example, as both strings are the same, it returns true. But in the last example, the strings are differentiated by the last character, so it returns false.
Code Example: Compare two strings using the “!=” operator
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluICgpCgp7CgpzdHJpbmcgc3RyMTsKCnN0cmluZyBzdHIyOwoKY291dCA8PCAiIEVudGVyIHRoZSBmaXJzdCBTdHJpbmc6ICIgPDwgZW5kbDsKCmNpbiA+PiBzdHIxOwoKY291dCA8PCAiIEVudGVyIHRoZSBzZWNvbmQgU3RyaW5nOiAiIDw8IGVuZGw7CgpjaW4gPj4gc3RyMjsKCgoKCmlmICggc3RyMSAhPSBzdHIyKQoKewoKY291dCA8PCAiIFN0cmluZ3MgYXJlIG5vdCBlcXVhbC4iIDw8IGVuZGw7Cgp9CgplbHNlCgp7Cgpjb3V0IDw8ICJCb3RoIFN0cmluZ3MgYXJlIGVxdWFsLiIgPDwgZW5kbDsKCn0KCnJldHVybiAwOwoKfQ==
Input1
Enter the first String: programming
Enter the second String: programmingOutput1
Both Strings are equal.
Input2
Enter the first String: unstop
Enter the second String: unstoppableOutput2
String is not equal
Explanation:
Now, we are comparing the two strings by using the != operator. Since the strings in the first example are equal, it will return false. However, the second example will return true because the strings are different.
Examples Of String Compare In C++
In this section, we will look at some examples that will help you understand the methods for comparing two strings in C++.
This String is Less than Other String
A string is said to be 'less than' another string if it appears before another string in lexicographic order, sometimes referred to as dictionary order. In other words, while comparing two strings, the comparison is performed character by character, embarking on with each string's initial character. The ASCII value of the characters is compared, and if the character in the first string has a lower ASCII value than the corresponding character in the second string, then the first string is considered less than the second string. Take the strings "bird" and "animal" as an example. The initial letter in the word "animal," "a," has an ASCII value of 97. The initial letter of the word "bird," "b," has an ASCII value of 98. The string "animal" is seen as being "less than" the string "bird" in lexicographic order since 97 is less than 98.
Here we are using the compare function to see the example.
Syntax:
int compare (const string &str) const;
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluICgpCgp7CgpzdHJpbmcgc3RyMSwgc3RyMjsKCmNvdXQgPDwgIiBFbnRlciB0aGUgZmlyc3Qgc3RyaW5nOiAiOwoKY2luID4+IHN0cjE7Cgpjb3V0IDw8ICIgRW50ZXIgdGhlIHNlY29uZCBzdHJpbmc6ICI7CgpjaW4gPj4gc3RyMjsKCgoKCmludCBqID0gc3RyMS5jb21wYXJlKHN0cjIpOwoKaWYgKCBqIDwgMCkKCnsKCmNvdXQgPDwgc3RyMSA8PCAiIGlzIHNtYWxsZXIgdGhhbiAiIDw8IHN0cjIgPDwgIiBzdHJpbmciIDw8IGVuZGw7Cgp9CgplbHNlIGlmICggaiA+IDApCgp7Cgpjb3V0IDw8IHN0cjEgPDwgIiBpcyBncmVhdGVyIHRoYW4gIiA8PCBzdHIyIDw8ICIgc3RyaW5nLiIgPDwgZW5kbDsKCn0KCmVsc2UgLy8gaiA9PSAwOwoKewoKY291dCA8PCAiIFR3byBzdHJpbmdzIGFyZSBlcXVhbC4iOwoKfQoKcmV0dXJuIDA7Cgp9
Input
Enter the first string: animal
Enter the second string: birdOutput
animal is smaller than bird string
Explanation
The two strings were compared employing the compare() approach since we are aware that when compare gives a negative result, it means the first string is smaller than the second string. In the input, a’s ASCII value is smaller than b's; that’s why, after being compared, it returned a negative value, and the printed animal is smaller than the bird string.
One String Is Greater Than Other String
If the ASCII value of the first string is higher than the ASCII value of the second after comparing two strings in C++ with the help of compare function, we can state that compared to the second string, and the first string is greater.
Syntax:
int compare (const string &str) const;
Code Example
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluICgpCgp7CgpzdHJpbmcgc3RyMSwgc3RyMjsKCmNvdXQgPDwgIiBFbnRlciB0aGUgZmlyc3Qgc3RyaW5nOiAiOwoKY2luID4+IHN0cjE7Cgpjb3V0IDw8ICIgRW50ZXIgdGhlIHNlY29uZCBzdHJpbmc6ICI7CgpjaW4gPj4gc3RyMjsKCgoKCmludCBqID0gc3RyMS5jb21wYXJlKHN0cjIpOwoKaWYgKCBqIDwgMCkKCnsKCmNvdXQgPDwgc3RyMSA8PCAiIGlzIHNtYWxsZXIgdGhhbiAiIDw8IHN0cjIgPDwgIiBzdHJpbmciIDw8IGVuZGw7Cgp9CgplbHNlIGlmICggaiA+IDApCgp7Cgpjb3V0IDw8IHN0cjEgPDwgIiBpcyBncmVhdGVyIHRoYW4gIiA8PCBzdHIyIDw8ICIgc3RyaW5nLiIgPDwgZW5kbDsKCn0KCmVsc2UgLy8gaiA9PSAwOwoKewoKY291dCA8PCAiIFR3byBzdHJpbmdzIGFyZSBlcXVhbC4iOwoKfQoKcmV0dXJuIDA7Cgp9
Input
Enter the first string: bird
Enter the second string: animalOutput
bird is greater than animal string
Explanation
We used the compare() function to compare the two strings. As we know that, when the comparison returns a positive value, it implies the first string is greater than the second string. In the input, b’s ASCII value is greater than a's; that’s why, after being compared, it returned a positive value, and the printed bird is greater than the animal string.
Single Program To Compare Two Strings In C++
In this section, we will look at an example to understand how we can use a single program to compare two strings in C++. After looking at the code, take a look at the explanation which describes the working of the said code.
Here is the single program using two built-in functions (compare and strcmp) in C++:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKI2luY2x1ZGUgPHN0cmluZy5oPgoKCgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmludCBtYWluKCkgewoKc3RyaW5nIHN0cjEgPSAidW5zdG9wIjsKCnN0cmluZyBzdHIyID0gInVuc3RvcCI7CgoKCgovLyBVc2luZyBzdHJjbXAoKQoKaW50IHJlc3VsdDEgPSBzdHJjbXAoc3RyMS5jX3N0cigpLCBzdHIyLmNfc3RyKCkpOwoKCgoKaWYgKHJlc3VsdDEgPT0gMCkgewoKY291dCA8PCAiVGhlIHN0cmluZ3MgYXJlIGVxdWFsLiIgPDwgZW5kbDsKCn0gZWxzZSBpZiAocmVzdWx0MSA8IDApIHsKCmNvdXQgPDwgInN0cjEgaXMgbGVzcyB0aGFuIHN0cjIuIiA8PCBlbmRsOwoKfSBlbHNlIHsKCmNvdXQgPDwgInN0cjEgaXMgZ3JlYXRlciB0aGFuIHN0cjIuIiA8PCBlbmRsOwoKfQoKCgoKLy8gVXNpbmcgY29tcGFyZSgpCgppbnQgcmVzdWx0MiA9IHN0cjEuY29tcGFyZShzdHIyKTsKCmlmIChyZXN1bHQyID09IDApIHsKCmNvdXQgPDwgIlRoZSBzdHJpbmdzIGFyZSBlcXVhbC4iIDw8IGVuZGw7Cgp9IGVsc2UgaWYgKHJlc3VsdDIgPCAwKSB7Cgpjb3V0IDw8ICJzdHIxIGlzIGxlc3MgdGhhbiBzdHIyLiIgPDwgZW5kbDsKCn0gZWxzZSB7Cgpjb3V0IDw8ICJzdHIxIGlzIGdyZWF0ZXIgdGhhbiBzdHIyLiIgPDwgZW5kbDsKCn0KCnJldHVybiAwOwoKfQ==
Output
The strings are equal.
The strings are equal.
Explanation
In this case, first, we used the strcmp function to compare two strings. The output for this will be 'strings are equal' if two strings are identical after comparison. So, we use the compare function and store the result's value. If the result is equal to zero, it will print 'the strings are equal'. Str1 is greater than Str2 if the result value is positive; else, it will print that str1 is greater than str2 when the result is negative. In the code, both the strings are "unstop," and as both the strings are the same, it is printed that the strings are equal.
The compare() Function: Return Value
It is a built-in function of C++ which evaluates the string object's value based on the string of characters specified by its parameter. It returns an integer value after comparing two strings, i.e., it returns zero when both strings are equal. If it returns a positive value after comparing, the first string is greater than the second. And when it returns a negative value, that means the first string is smaller than the second string.
Here is a code where, after comparing two strings, it prints its return value.
I2luY2x1ZGUgPGlvc3RyZWFtPgoKI2luY2x1ZGUgPHN0cmluZz4KCnVzaW5nIG5hbWVzcGFjZSBzdGQ7CgoKCgppbnQgbWFpbigpIHsKCnN0cmluZyBzdHIxID0gImRpY2UiOwoKc3RyaW5nIHN0cjIgPSAicmljZSI7CgoKCgppbnQgcmVzdWx0ID0gc3RyMS5jb21wYXJlKHN0cjIpOwoKCgoKY291dCA8PCAiVGhlIHJlc3VsdCBvZiBjb21wYXJpbmcgXCIiIDw8IHN0cjEgPDwgIlwiIGFuZCBcIiIgPDwgc3RyMiA8PCAiXCIgaXM6ICIgPDwgcmVzdWx0IDw8IGVuZGw7CgoKCgpyZXR1cm4gMDsKCn0=
Output
The result of comparing "dice" and "rice" is: -14
Explanation
In this case, the first string is "dice," and the second string is "rice", We have compared both strings using the compare function and stored the results. Next, we printed the value of the result, which is the return value. Since d is the first string and the difference between d and r is 14, the value has been displayed in the negative.
The strcmp() Function: Parameters, Return Value & Undefined Behaviour
In C++, two strings are compared using the strcmp() technique. It takes two parameters, which are pointers to the null-terminated character arrays (i.e., strings), to be compared:
Syntax:
int strcmp( const char *str1, const char *str2 );
The first and second strings to be compared are indicated by the pointers str1 and str2, respectively.
Here is the code to look at how parameters are being used to compare two strings in C++.
I2luY2x1ZGUgPGlvc3RyZWFtPgoKI2luY2x1ZGUgPGNzdHJpbmc+CgoKCgppbnQgbWFpbigpIHsKCmNvbnN0IGNoYXIqIHN0cjEgPSAidW5zdG9wIjsKCmNvbnN0IGNoYXIqIHN0cjIgPSAidW5zdG9wIjsKCgoKCmludCByZXN1bHQgPSBzdGQ6OnN0cmNtcChzdHIxLCBzdHIyKTsKCgoKCmlmIChyZXN1bHQgPCAwKSB7CgpzdGQ6OmNvdXQgPDwgIlRoZSBzdHJpbmcgXCIiIDw8IHN0cjEgPDwgIlwiIGlzIGxlc3MgdGhhbiB0aGUgc3RyaW5nIFwiIiA8PCBzdHIyIDw8ICJcIi4iIDw8IHN0ZDo6ZW5kbDsKCn0gZWxzZSBpZiAocmVzdWx0ID4gMCkgewoKc3RkOjpjb3V0IDw8ICJUaGUgc3RyaW5nIFwiIiA8PCBzdHIxIDw8ICJcIiBpcyBncmVhdGVyIHRoYW4gdGhlIHN0cmluZyBcIiIgPDwgc3RyMiA8PCAiXCIuIiA8PCBzdGQ6OmVuZGw7Cgp9IGVsc2UgewoKc3RkOjpjb3V0IDw8ICJUaGUgc3RyaW5nIFwiIiA8PCBzdHIxIDw8ICJcIiBpcyBlcXVhbCB0byB0aGUgc3RyaW5nIFwiIiA8PCBzdHIyIDw8ICJcIi4iIDw8IHN0ZDo6ZW5kbDsKCn0KCnJldHVybiAwOwoKfQ==
Output
The string "unstop" is equal to the string "unstop".
Explanation
The strings str1 and str2 are compared in this example by the strcmp() function. The result is stored in the result variable. A series of conditional statements are executed to decide whether str1 is less than, greater than, or equal to str2.
Note that \" is used to print the quotation marks around the strings in the output message.
strcmp() Return Value
As we know, we use the strcmp() function in C++ to compare two strings. The two strings that will be compared must be provided as the two parameters. The function returns an integer number that reflects the comparison's conclusion. When both strings are equal, the strcmp() function provides a result of 0. The return value is a negative integer if the first string is smaller than the second string. The return value is a positive integer if the first string is greater than the second string.
Syntax:
int strcmp( const char *str1, const char *str2 );
Code Example
I2luY2x1ZGU8aW9zdHJlYW0+CgojaW5jbHVkZTxzdHJpbmcuaD4KCnVzaW5nIG5hbWVzcGFjZSBzdGQ7CgppbnQgbWFpbigpCgp7CgpjaGFyIHN0cjFbNTBdLCBzdHIyWzUwXTsKCmludCBsZW4xLCBsZW4yOwoKY291dDw8IkVudGVyIHRoZSBGaXJzdCBTdHJpbmc6ICI7CgpjaW4+PnN0cjE7Cgpjb3V0PDwiRW50ZXIgdGhlIFNlY29uZCBTdHJpbmc6ICI7CgpjaW4+PnN0cjI7CgpsZW4xID0gc3RybGVuKHN0cjEpOwoKbGVuMiA9IHN0cmxlbihzdHIyKTsKCmlmKGxlbjE9PWxlbjIpCgp7CgppZihzdHJjbXAoc3RyMSwgc3RyMik9PTApCgpjb3V0PDwiXG5TdHJpbmdzIGFyZSBFcXVhbCI7CgplbHNlCgpjb3V0PDwiXG5TdHJpbmdzIGFyZSBub3QgRXF1YWwiOwoKfQoKZWxzZQoKY291dDw8IlxuU3RyaW5ncyBhcmUgbm90IEVxdWFsIjsKCmNvdXQ8PGVuZGw7CgpyZXR1cm4gMDsKCn0=
Input1
Enter the First String: unstop
Enter the Second String: unstopOutput1
Strings are Equal
Input2
Enter the First String: slicer
Enter the Second String: slicedOutput2
Strings are not Equal
Explanation
We used the compare() function to compare the two strings after receiving the user's input for the two strings and printing the result. As you can see in the first example, j includes 0 because both strings are identical. In the next example, j contains a greater number than zero which means the second string is greater than the first string. In the last example, j contains a lesser number than zero which implies that string one is smaller than string two.
strcmp() Undefined Behavior
The strcmp() function has well-defined behavior for most cases, but there are a few situations in which it can exhibit undefined behavior. Here are some examples:
- Null pointers: If either of the two pointer arguments passed to strcmp() is a null pointer, it will result in undefined behavior. It's important to ensure that both pointers are valid and pointing to valid strings before calling strcmp().
- Non-null terminated strings: The strcmp() function expects null-terminated strings as input. If either of the strings passed to strcmp() is not null-terminated, it will result in undefined behavior.
- Memory overlap: If the two strings passed to strcmp() overlap in memory, it can result in undefined behavior. For example, if one string is a substring of the other and they are being compared, the result may be unpredictable.
- Character encoding: The behavior of strcmp() is not well-defined for strings that contain characters outside the basic character set (i.e., non-ASCII characters) or for strings encoded in a non-standard encoding. In such cases, it may be more appropriate to use a different string comparison function that is designed to handle these cases.
To avoid undefined behavior when using the strcmp() function, it's important to ensure that both input strings are valid, null-terminated strings and that they do not overlap in memory.
Conclusion
In a nutshell, the string compare operation plays an essential role in comparing two strings and determining whether they are the same or not. In C++, it is easy to compare two strings as there are two built-in functions: compare() and strcmp(). You can also use the relational operators to check equality in the fastest way. The strings being compared should be of the same type, and their case sensitivity needs to be taken into account. String comparison is performed by checking both strings character by character. After comparing two strings, strcmp and compare both return 0, if the two strings are equal. But relational operators return a boolean (i.e., true or false) after comparing two functions. Overall, understanding how to compare strings in C++ is a fundamental aspect of programming that is used in a wide range of applications.
FAQs
Q. Can I use == to compare strings in C++?
Yes, the C++ == (equals to) operator allows you to compare two strings. It performs a lexicographical comparison between strings. If two strings are equal, it returns ‘true’. Otherwise, it returns ‘false.’
Q. Can we compare two strings in C++ without using strcmp?
The short answer to this question is- yes; we can compare two strings in C++ without using the strcmp() function. Note that the strcmp() is a predefined function in the string.h header file of the C++ language. You can also compare two strings using the compare function and relational operators == and !=.
Q. Can you use comparison operators on strings?
Comparison operators compare two values. We can easily compare whether two strings match or not using the == and!= operators. Both the == and!= operators compare two strings and return a boolean (true or false) value.
Q. How do you check if a string is contained with another in C++?
If a string contains a substring, we can use the find, boost, and strstr functions in C++ to determine.
1. Find: In C++, the find() function makes it easier to look for the substring within a given string. This function is available in the header file 'algorithm.h'.
2. Boost: The boost library function can be used to find any substring in a string. It gives 1 if the substring was discovered; otherwise, it returns 0.
3. Strstr(): In C++, the strstr function can also be used to determine a substring within a string. It returns a pointer if the substring has been found; if the substring has not been found, it returns a null pointer.
Q. How can we compare two strings in C++?
C++ provides the strcmp() function, compare() function, and relational operator(== and !=) to compare two strings. Here is a little brief on how these methods compare strings in C++:
- Strcmp function:
Strcmp is a built-in function that allows you to compare two strings in C++. The string.h header file contains the strcmp function. After comparing the two strings, 0 will be returned if both strings are identical.
Syntax: int strcmp ( const char *str1, const char *str2 );
- Compare function:
Compare function compares two strings in C++. It is a built-in function of C++. If the two strings match, it returns 0.
Syntax: int compare (const string &str) const;
- Relational operator(== and !=):
Relational operators make the comparison between two strings easier. The “==” operator returns true if both strings are the same; otherwise, it returns false. When both strings are distinct, the "!=" operator returns true. Otherwise, it returns false.
Syntax: String1 == string2
Or
String1 != string2
Q. What does compare () return?
The compare() function is a built-in function that gives the facility to compare two strings in C++. After comparing the two strings, any of the following happens:
- It returns zero, which implies both strings are equal
- It does not return zero. It indicates that the strings are not equal.
- It provides a positive value, which means string str1 is greater than the str2
- It provides a negative value, which means string str1 is less than str2
Q. What happens when you compare two strings?
Since every character holds an ASCII value, when starting to compare two strings of the same length, it starts by checking the first letter of the first string and comparing it with the first letter of the second string. If the ASCII value of both letters is the same, it jumps to the next letter, and this process continues until it encounters the null. When both strings match, it returns 1; otherwise, it returns 0.
Q. Can you compare strings with == in C?
We can't use the == operator to compare two strings in the C language. The reason behind this is when comparing two strings in C, you end up comparing their addresses because a string is nothing more than an array of characters. You can, however, compare two strings in C with strcmp().
Q. What does string compare mean?
The practice of comparing two strings to see if they are equal or not is known as string comparison. Typically, when comparing strings, we are looking to see if the character sequences in the strings match or not. We can claim that two strings are equal when the character sequences are a match. If the strings don't match, we'll declare them not equal.
Q. How to compare two strings using the string function?
The string.h header file in the library features a predefined function called strcmp() to compare two strings. We will look at an implementation example below to get a better understanding of how to compare two strings using the string function.
Code Example:
I2luY2x1ZGU8aW9zdHJlYW0+CgojaW5jbHVkZTxzdHJpbmcuaD4KCnVzaW5nIG5hbWVzcGFjZSBzdGQ7CgppbnQgbWFpbigpCgp7CgpjaGFyIHN0cjFbNTBdLCBzdHIyWzUwXTsKCmludCBsZW4xLCBsZW4yOwoKY291dDw8IkVudGVyIHRoZSBGaXJzdCBTdHJpbmc6ICI7CgpjaW4+PnN0cjE7Cgpjb3V0PDwiRW50ZXIgdGhlIFNlY29uZCBTdHJpbmc6ICI7CgpjaW4+PnN0cjI7CgpsZW4xID0gc3RybGVuKHN0cjEpOwoKbGVuMiA9IHN0cmxlbihzdHIyKTsKCmlmKGxlbjE9PWxlbjIpCgp7CgppZihzdHJjbXAoc3RyMSwgc3RyMik9PTApCgpjb3V0PDwiXG5TdHJpbmdzIGFyZSBFcXVhbCI7CgplbHNlCgpjb3V0PDwiXG5TdHJpbmdzIGFyZSBub3QgRXF1YWwiOwoKfQoKZWxzZQoKY291dDw8IlxuU3RyaW5ncyBhcmUgbm90IEVxdWFsIjsKCmNvdXQ8PGVuZGw7CgpyZXR1cm4gMDsKCn0=
Q. Which string method compares two strings?
Comparing two strings refers to comparing the characters between two strings. We can state the two strings are identical if they contain the same characters; otherwise, they are different. The compare and strcmp functions give us the facility to compare strings in C++. Both of them are built-in functions of C++. Compare and strcmp both return 0 if the strings are identical.
Here is an example of how we use the compare and strcmp functions in code to compare two strings in C++
I2luY2x1ZGUgPGlvc3RyZWFtPgoKI2luY2x1ZGUgPHN0cmluZy5oPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewoKc3RyaW5nIHN0cjEgPSAidW5zdG9wIjsKCnN0cmluZyBzdHIyID0gInVuc3RvcCI7CgoKCgovLyBVc2luZyBzdHJjbXAoKQoKaW50IHJlc3VsdDEgPSBzdHJjbXAoc3RyMS5jX3N0cigpLCBzdHIyLmNfc3RyKCkpOwoKCgoKaWYgKHJlc3VsdDEgPT0gMCkgewoKY291dCA8PCAiVGhlIHN0cmluZ3MgYXJlIGVxdWFsLiIgPDwgZW5kbDsKCn0gZWxzZSBpZiAocmVzdWx0MSA8IDApIHsKCmNvdXQgPDwgInN0cjEgaXMgbGVzcyB0aGFuIHN0cjIuIiA8PCBlbmRsOwoKfSBlbHNlIHsKCmNvdXQgPDwgInN0cjEgaXMgZ3JlYXRlciB0aGFuIHN0cjIuIiA8PCBlbmRsOwoKfQoKCgoKLy8gVXNpbmcgY29tcGFyZSgpCgppbnQgcmVzdWx0MiA9IHN0cjEuY29tcGFyZShzdHIyKTsKCmlmIChyZXN1bHQyID09IDApIHsKCmNvdXQgPDwgIlRoZSBzdHJpbmdzIGFyZSBlcXVhbC4iIDw8IGVuZGw7Cgp9IGVsc2UgaWYgKHJlc3VsdDIgPCAwKSB7Cgpjb3V0IDw8ICJzdHIxIGlzIGxlc3MgdGhhbiBzdHIyLiIgPDwgZW5kbDsKCn0gZWxzZSB7Cgpjb3V0IDw8ICJzdHIxIGlzIGdyZWF0ZXIgdGhhbiBzdHIyLiIgPDwgZW5kbDsKCn0KCnJldHVybiAwOwoKfQ==
Output
The strings are equal.
The strings are equal.
This brings us to the end of our discussion on string compare in C++. Here are some more interesting reads to quench your thirst for knowledge:
- Data Types In C++ | A Detailed Explanation With Examples
- History Of C++ | Detailed Explanation (With Timeline Infographic)
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- Difference Between C And C++| Features | Application & More!
- Decoding The Difference Between Structure And Class In C++
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment