C++ String Find() | Examples To Find Substrings, Character & More!
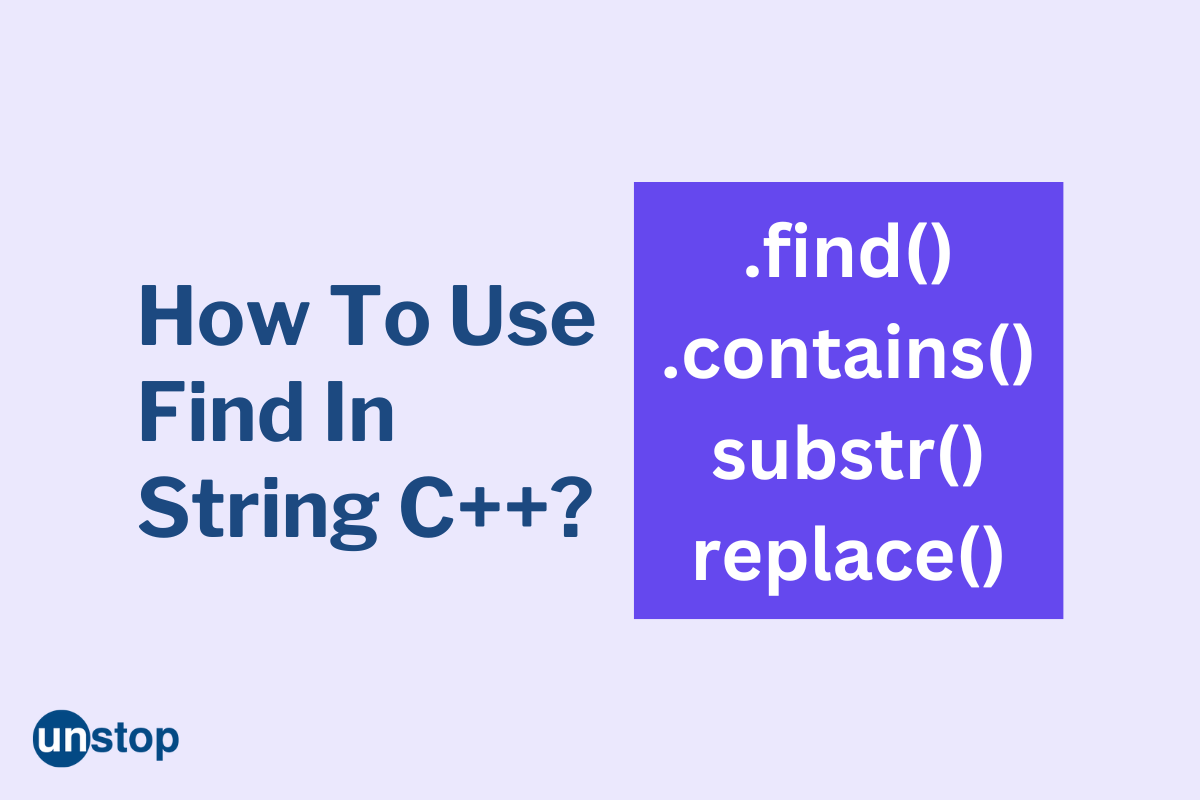
In programming, strings are like sentences that consist of a series of characters and are used to store information. There are different kinds of strings in C++ and we can fulfill a variety of tasks with the help of strings and substring functions. In this article, we will have a look at what are substrings in C++, along with ways to find strings in C++, find substrings, partial strings, a single character of the string, etc. We have provided detailed examples for each to help you better understand these topics.
What Is Find In String C++?
In simple terms, the find() function is the method that returns the index of the first occurrence of a specific string or a character string. Specifically in C++ language, the C++ library provides a .find() function which returns the index of the first occurrence of a substring in a string. And in case a match isn't found, string::npos is returned instead of the string character. Let's look at the syntax and an example for the same.
Syntax of .find() function:
The syntax for declaring .find() function is-
// str1.find(substring);
Knowing the Parameters:
Here,
str1- the input string/ main string/ original string
substring- the string to be matched to the main/ original string
Return value
The function finds the substring that matches str1 and returns the position at which the substring is found. In case, the substring passed as an argument, does not match the input string, a special variable called std:: string :: npos is returned to denote the end of the string.
Implementation example of .find() function-
#include
using namespace std;
int main()
{
string str= "learning at unstop is fun";
cout << str<<'\n';
cout <<" Position of unstop is :";
cout<< str.find("unstop"); Match found at index 12.
}
Output-
learning at unstop is fun
Position of unstop is 12
Explanation-
In the above code snippet, we initialize a string str as ‘learning at unstop is fun’ and then, we simply print the input string. We then choose a substring ‘unstop’ and use str.find() find to get the index of the first character when the substring matches the string.
What Is A Substring?
The short and crisp answer to this question is- part of a string is a substring. But is this the complete answer? No, let’s get into the depth a little more. A substring is a part of a string which is continuous and can be repetitive as well. It could be in the beginning of the original string, anywhere in the middle or sometimes even at the end of the string. Now the question is, how do we know if the substring is present in the specified string or not? Let’s find out the answer below.
How To Find A Substring In A String In C++?
To find a substring in a string, we have a predefined function called substr() function in our C++ programming language. The substr() function takes 2 parameters pos and len as arguments to find and return the substring between the specified position and length.
Syntax of Substr() function:
// string substr (size_t pos, size_t len)const;
Knowing the parameters:
size_t : is an unsigned integral type
pos: starting position/index from where string search begins
len: length of the substring
Example for implementation of substr() function:
#include
#include
using namespace std;
int main()
{
// Random string
string s1 = "Learning at Unstop is fun";
//substr function to find the substring Unstop
string r = s1.substr(12, 6); //index should be 12, and length should be 6.
// result
cout << "Substring is: " << r;
return 0;
}
Output-
Substring is: Unstop
Explanation:
In the above code snippet, we take a random string s1 as input (i.e. your original string). After that, we use the s1.substring() function to find a substring with index as 12 and the length of the string as 6. Once, the substring is found, we store it in a string r and print it as our output.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
How To Find A Character In String C++?
To find a specific/ single character in a string in C++, we can use the std::basic_string::contains function. The return value of this function is boolean, which means it return true is the character is found else false.
Implementation of .contains() function:
#include
#include
intmain()
{
std::stringstr = "find string in C++";
charc = '+';
if(str.contains(c)){ //find if string contains character ‘+’.
std::cout << "Character found" << std::endl; //if yes
}else{
std::cout << "Character not found" << std::endl; //if no
}
return0;
}
Output:
Character Found
Explanation:
In the above example, we initialize str - string with a random string and char c with a random character. We then make use of the str.contains() function in C++, to find if the random string contains the input character. If yes then 'character found' is printed, otherwise 'character not found' is printed.
Also read- Difference Between C And C++| Features | Application & More!
Find All Substrings From A Given String In C++
To find all substrings from a given/ current string in C++, we can use a substring function that takes string s, and the length of string(n) as parameters. This function prints all the substrings up to the length of the string.
Implementation to find all substrings from strings (main)-
#include<bits/stdc++.h>
using namespace std;
// Function to print all sub strings
void subString(string s, int n)
{
for (int i = 0; i < n; i++) //this loop goes till the length of string, in this case 3.
for (int len = 1; len <= n - i; len++) // this loop prints individual substring of all length until n-i, in this case substring of length 1,2 and 3.
cout << s.substr(i, len) << endl;
}
// Main function-
int main()
{
string s = "run";
subString(s,s.length());
return 0;
}
Output-
r
ru
run
u
un
n
Explanation:
In the above example, the subString functions print all substrings of the original string. The outer loop goes to the length of the entire string, in this case, 3. The inner loop prints individual substrings of all lengths until (n-i), in this case, substrings of lengths 1,2, and 3. The main function initializes a string s as run. This main function drives the subString function which in turn prints all the substring in a given string in C++.
Index Substring In String In C++ From A Specific Start To A Specific Length
The substr() function is used to find the index of a substring in string in C++ which is a standard library function. This function returns a string object reference.
Syntax:
string s1 substr(start_index, n);
Here,
start_index refers to the starting index.
n refers to the number of characters to be copied.
Implementation to index substring in a string from specified start to end:
# include
# include
using namespace std;
int main ()
{
//declare string and substring
string s ="find string in C++";
string subs;
int start = 5,
int end=11;
// to access 6 characters from 5th index hence, (end-start)= 11 - 5 = 6
str2 = str1.substr(start, (end - start));
//print strings
cout<<"str1: "<< str1 <<endl;
cout<<"str2: "<< str2 <<endl;
return 0;
}
Output-
s: find string in C++
subs: string
Explanation:
In the above example, we first declare a string s and a substring subs. We then initialize start and end index for the substring as 5 and 11. Then, the function substr() is used to access 6 characters from 5th index hence, (end-start)= 11 - 5 = 6. This gives us our substring which we print as output along with our main string.
Conclusion
In this article, we discussed all the way to find strings in C++. So, we are familiar with the fact that strings are just normal text that we type or read in our day-to-day life, and in programming language we can access these strings using various standard library functions. We have covered all the topics including finding a character in a string, finding a substring, partial strings, and more. We hope this article helped you in understanding the topics better.
Also read- Data Types In C++ | A Detailed Explanation With Examples
Frequently Asked Questions
Q. How do I find the substring of a string in C++?
To find a substring in a string, we have a predefined function called substr() function in C++. The substr() function takes 2 parameters pos (i.e. initial position) and len (i.e. length) as arguments to find and return the substring between the specified position and length.
Syntax of Substr() function:
// string substr (size_t pos, size_t len)const;
Q. How do you check if a string starts with a specific substring?
string::starts_with function is used to find if a string starts with a specified substring. This function returns true if the string starts with a specified substring, else false. It can be illustrated as:
#include
#include
int main()
{
strings = "Unstoppable"; //initialize string s as unstoppable
stringsubstring = "Unstop"; //initialize substring as unstop
if(s.starts_with(substring)){ //check if substring matches string at the beginning.
cout << "String starts with the given substring" <<endl; //print it
}else{
cout << "String doesn't starts with given substring" << endl;
}
return 0;
}
Output:
String starts with the given prefix
Q. How do you find a substring in a string and replace it in C++?
C++ string library provides a function called string replace() function, to replace a portion of string starting from a given index to the end index and replacing that with the new string. The position from where the substring has to be started, the length of the substring, and the new string is passed in the parameter as an argument.
Syntax of replace() function:
// str1.replace(position,length,newStr);
Q. How to find the first occurrence of substring in a string in C++?
C++ library provides a .find() function which returns the index of the first occurrence of substring in string. If case when no substring is found, string::npos is returned.
Syntax of .find() function:
// str1.find(substring);
Q. What is the return value of find_first_of in C++?
The function find_first_of in C++ returns the position of any element from range[first1,last1] that matches with the elements in the range[first2,last2]. In case, there are more than one element that matches both the range, then the position of the first common element is returned. When there is no match found in the entire range, the function returns string :: npos.
Q. What is the use of find_last_of function in C++?
The function find_last_of is used to find the index of the last occurrence of a character in a string. If the character is present in the string, it returns the index of the last occurrence of that character in the string; else it returns string::npos.
We have reached the end of the article on using 'find' in strings in C++. You might also be interested in reading:
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- What Is GitHub? An Introduction, How-To Use It, Components & More!
- History Of C++ | Detailed Explanation (With Timeline Infographic)
- The Structure of C++ Programs Explained With Examples!
- What Is Bash? Features, Major Concepts, Commands, & More!
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
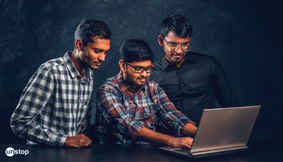
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
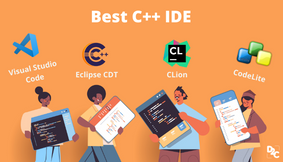
10 Best C++ IDEs That Developers Mention The Most!
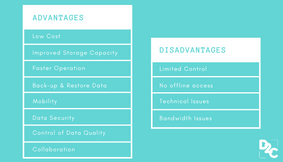
Advantages and Disadvantages of Cloud Computing that you should know!
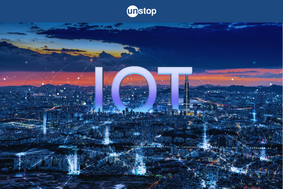
Adolphus “Buli” 1 year ago