Typedef In C++ | Syntax, Application & How To Use (+Code Examples)
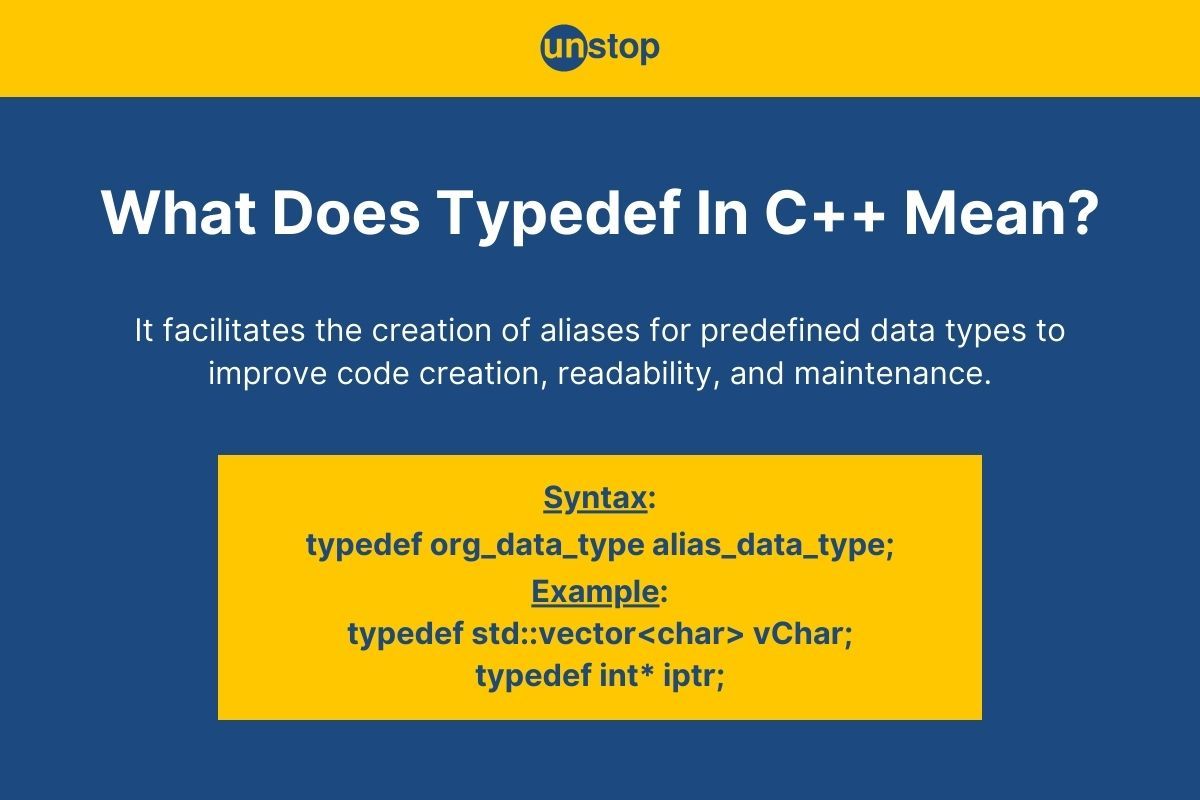
The typedef in C++ programming is a reserved keyword used to give more descriptive and simple names to standard pre-defined data types, which may be too complex to write repeatedly. Data types represent the type of data stored in a variable or any other construct. In this blog, we will discuss the syntax, working mechanism, and uses of typedef in C++ with the help of examples.
What is Typedef in C++?
Typedef is short for 'Type-Definition' and is the reserved keyword used to create an alias name (or, in simple words, a short and meaningful name) for specific pre-defined or user-defined data types, pointers, or structures. The primary use of typedef in C++ language is to make it easy for developers to write programs by shortening complex type names. It aids in making the code cleaner while keeping the purpose served by the new datatype unchanged.
The Role & Applications of Typedef in C++
The primary role and application of typedef in C++ are to facilitate the aliasing of specific datatypes.
- It helps make the code easier to work with, as it gives a more descriptive name, which helps the programmers understand the use of the specified function or wrapper class.
- The purpose served by the new data type remains intact and unchanged.
- It reduces the complexity of code writing by facilitating the process of declaring higher-level data types.
- Typedef in C++ can be used with arrays, normal and function pointers, and other STL data structures like vectors, strings, maps, etc.
For example, say you want to create a function collect_data() that takes different inputs and returns an error message. Defining the function in the usual way-
bool collect_data(char alpha_data, vector<long> &data);
While this is the usual and correct way to declare a function, this declaration does not give the programmer an idea of the function’s exact purpose or what it will return. To avoid this situation, we use typedef declaration to make the code self-explanatory and modification easier.
typedef bool error_msg; // Using typedef to rename the bool data type
error_msg collect_data(char alpha_data, vector<long> &data); //Using error_msg instead of bool
In the example above, the renamed data type clearly indicates that the collect_data() function will return an error message.
Basic Syntax for typedef in C++
typedef <existing_data_type> <aliased_name>;
Here,
- The typedef keyword indicates that we are defining a new identifier for a data type.
- The existing type specifier/ data type that we want to rename is given by existing_data_type, and the replacement identifier is given by aliased_name
How Does typedef Work in C++?
As mentioned, typedef is a reserved keyword that helps replace complex data types with simpler and more explanatory names. Here is how typedef in C++ works:
- The typedef keyword creates an alias name for a data type while providing the same level of abstraction from both the actual data types and the modified data types.
- It allows us to create aliases that focus more on what the variable should actually mean.
- The use of destroy() and other default ways garbage collectors work makes it easy to delete unnecessary codes and optimize the use of memory space locations. In simpler terms, it makes it easy for programmers to write clean code.
- It also helps in computing the size based on data types and allocating memory space for both large storage data type variables and small storage variables.
- Using typedef helps simplify variable declarations for many compound types, including union, struct, etc.
- We can also use the typedef in C++ with structure type to shorten the declaration statements needed each time a new structure is created. This also minimizes code complexity, code readability, and the chances of any possible errors.
- Typedef allows us to shorten long names of complex data structures with easy modification.
For example, say we want to declare a map of strings to characters; then the syntax would be as follows:
Map <string, char> mp;
However, this statement does not provide enough information about the map and is tedious to write repeatedly whenever we need a new map. With the use of typedef in C++, we can redeclare the map type with a more symbolic name as follows:
typedef map <string, char> GradeByName;
The above statement clearly shows that the map represents the grades of various students. Also, if you decide to give a grading point, you just need to change the character type to float.
How to Use Typedef in C++ With Examples? (Multiple Data Types)
As mentioned, we can use typedef in C++ programs to create alias names for types. In this section, we will discuss, with the help of examples, how to implement typedef on different type specifiers.
Typedef in C++ With Pre-Defined Data Types
The most commonly used pre-defined/ built-in types in C++ are int, float, char, etc., which are rather easy to use and write repeatedly. However, some derived types are long, long long, unsigned long, etc. can be cumbersome to use.
In such cases, typedef in C++ can be used to create aliases for the actual types. And we can use that alias name to declare new variables of the respective data types. The syntax for this remains the same as mentioned above. Look at the example C++ program below to understand how this can be done.
Code Example:
Output
The unsigned long int value is: 54321
Explanation:
In the example C++ code, we first include the essential header file, i.e., <iostream>, for input-output operations and use namespace std.
- Inside the main() function, we create an alias for the unsigned long int type (which is a built-in int type), i.e., uli.
- Next, we declare a variable a1, which is of uli type and initialize it with the value 54321 using curly braces.
- Then, we use the cout command to print the same to the console.
- Lastly, the main() function returns 0, indicating successful execution without any errors.
Typedef in C++ With STL Data Structures
Suppose your code needs many new Standard Libraries (STL) data structures such as vectors, maps, strings, etc. Then, writing the expression- std::vector<int> repeatedly will be very time-consuming. It is beneficial to use the typedef in C++ in such cases, making it easier to write the code.
Syntax:
typedef <STL_data_structure_name> <alias_name>;
Here, the basic syntax remains the same, with the only difference being that the original data type has been replaced with the data structure type. Look at the example C++ code below to understand how typedef can be used with STL data structures (vector in this case).
Code Example:
Output:
T y p e D e f
Explanation:
In the C++ code example-
- We first include header files for input-output operations and using vectors, i.e., <iostream> and <vector>, respectively.
- Then, we typedef vector creation STL notation to create an alias, i.e., an alias for std::vector<> is vChar.
- Next, we use the alias to declare and initialize a vector named v1 and then use a for loop with the cout command to display the vector elements to the console.
Typedef in C++With Array Types
Just like in STL data structures, typedef can be used with an array to create a custom data type that acts as its alias. You can easily create 2D and 3D arrays using the alias typedef.
Syntax:
typedef <array_data_type> <alias_name> [size];
Here, the deviation in the syntax is that for the original data type that we want to create an alias of, we take the data type of the elements stored in the array given by array_data_type. We also specify the size of the array/ number of elements given by size.
Code Example:
Output
Array output:
1 2 3
Matrix output:
0 0 0
0 1 2
0 2 4
Explanation:
- In the main() function, we use typedef to create an alias for the integer array type called arr with the size specified as 3 elements.
- We then initialize an array called array1 using the alias notation and print its elements by employing a for loop and cout command.
- Using the same notation, we then create a 2D array called mat[3], which will be a 3*3 matrix.
- After that, we first initialize the elements of the matrix (array of arrays) using a set of nested for loops. In that, the outer loop focuses on the row number, and the inner loop focuses on the column number.
- Next, we again use a set of nested loops and print the matrix to the console.
Typedef in C++ With Pointers
Typedef in C++ is commonly used for the faster creation of pointers. It can be used with normal pointers and function pointers as well.
Syntax:
typedef <data_type>* <alias_name>;
Here, the term <data_type>* indicates the data type of the pointer, which is given the asterisk symbol (*).
Code Example:
Output:
p is: 10
Explanation:
- We declare and initialize an integer variable p with the value 10 inside the main() function.
- Then, we use typedef to create an alias name for the integer pointer type, i.e., iptr.
- Using this notation, we create a pointer called pointer_p and assign the address of p variable to it using the address-of operator (&).
- Next, we use the cout command to print the value stored in the variable.
Typedef in C++ With Function Pointers
Function pointers are those pointer variables that point to a function instead of a variable or a data structure. The general syntax for the declaration of a function pointer can be long. We can hence use typedef to create an alias name for it; the syntax for this is given below.
Syntax:
typedef <return_type_of_function>(*<alias_name>) (<parameter_type>,<parameter_type>,....);
For Example:
typedef int (*function_ptr)(int, int);
function_ptr new_ptr = &function;
Code Example:
Output
5
6
Explanation:
As mentioned in the code comments at every step, the example shows how we generally create function pointers. In comparison to using an alias name for the same, created using typedef.
Note: The “func_ptr2” can not be used as an independent function pointer. It can be used only to create new function pointers, which can only point to the function that takes two integers as its input parameters and returns an integer sum.
The Difference Between #define & Typedef in C++
Typedef |
#define |
Typedef can only be used to assign alternative/ symbolic names to types, pointers, and structures. It cannot do so with values. |
It can provide alternative or alias names for values as well. E.g., we can define 3.142 as pi, etc. |
Typedef statements are compiler-based, i.e., they are interpreted by the compiler. |
#define statements are pre-processor based, i.e., they are interpreted by the pre-processor. |
Typedef statements are to be terminated using a semicolon. (;) |
#define does not need a semicolon for termination. |
It is the actual definition of a new datatype (or pointer or structure). |
It is simply an indirect way to copy-paste the definition values. |
The typedef keyword follows the scope rule. E.g., if a new type is defined within a scope or a function, the new type is visible only until the scope is present. |
#define does not follow any scope rules. When the pre-processor encounters it, all its occurrences are replaced. |
Conclusion
Typedef stands for 'type definition', and it is the reserved keyword used to create an alias name for built-in data types. It is especially helpful in simplifying variable declarations for complex data types and compound structures, unions, etc. The syntax of typedef in C++ is:
typedef <existing_data_type> <aliased_name>;
It differs from the #define macro, as the compiler interprets typedef statements while #define statements are interpreted by the pre-processor. Also, we can use typedef in C++ to assign alias names only to data types and structures where, whereas #define can be used to provide alias names to values as well (e.g., pi for 3.142)
Frequently Asked Questions
Q. What is the data type of typedef?
There is no data type of the typedef in C++ since it is not a new data type. Instead, it is a reserved keyword used to create an alias name or alternative and more descriptive name for a predefined/ built-in data type.
Q. What is the syntax of typedef in C++?
The syntax of typedef in C++ is divided into three parts. The first is the typedef keyword itself, followed by the existing data type that you want to create an alias for and lastly the alias name. The syntax is-
typedef <existing_data_type> <aliased_name>;
Q. Difference between struct and typedef struct in C++ programs?
The keyword Struct is used to define a structure comprising many other data types as its component elements. Now, typedef struct is the same as the struct keyword type because, in C++, all declarations are implicitly typedef’ ed until hidden by some other declaration with the same name. The only difference is that typedef cannot be forward declared.
Q. How to use typedef struct in C/C++?
Typedef struct in C++/C is mostly used to make code cleaner and more readable by giving short and meaningful names to complex data structures. The syntax for declaring a typedef struct:
typedef struct <struct_data_type_name>{
/* different component of multiple data types declared */
} <alias_name>
<alias_name> <object_name>;
// object of structure is created with a need for typing struct again and again
Code Example:
Output:
Welcome to #Unstop
Enter student name: Rajesh
Enter student age: 25
Student name is: Rajesh
Student age is: 25
Q. What is typedef enum in C?
A typedef is a way of declaring an alternative name for a standard data type. An enumerated data type or enum type is a user-defined data type with an associated set of symbolic constants representing the valid values of that type.
Q. How to use typedef with a pointer?
Typedef can be used to declare a number pointer of the same type. Let’s understand this with the help of an example.
Syntax:
typedef <data_type>* <alias_name>
Code Example:
Output:
x= 10.5 *px= 10.5
y= 8.6 *py= 8.6
z= 12.5 *pz= 12.5
Q. Can you use typedef in a class in C++?
Yes, we can use typedef in a class in C++, but the typedef name should be different from any class type name declared in that scope. The typedef name can be the same as the class type name only if that typedef is a synonym of the class name. A C++ class that is defined in typedef definition without being named can be given a dummy name. This class can’t have constructors or destructors.
For example:
Typedef class {
~Trees();
} Trees;
Here, Trees is an alias for an unnamed class that is defined with the use of typedef in C++ class. It is not a class type name, and therefore, we cannot define a ~Trees() destructor for this class, or else the compiler throws an error.
This brings us to the end of our discussion on typedef in C++. Here are a few other articles you must read:
- Logical Operators In C++ | Use, Precedence & More (With Examples)
- C++ Type Conversion & Type Casting Demystified (With Examples)
- String Compare In C++ | Learn To Compare Strings With Examples
- C++ If-Else | All Conditional Statements Explained With Examples
- Operators In C++ | Types & Precedence Explained (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment