Do-While Loop in C++: How It Works, Syntax, and Examples
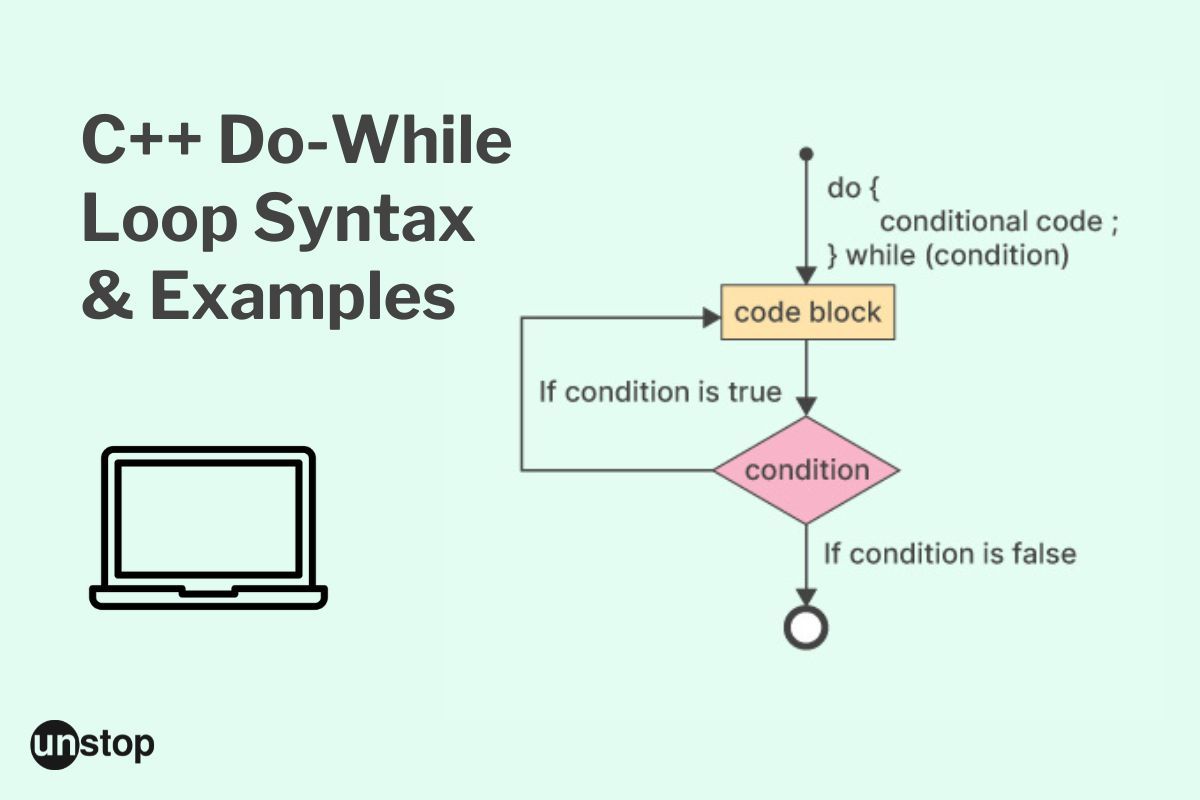
When it comes to iterative programming, loops are a fundamental construct. In C++ programming language, the do-while loop stands out as a powerful tool for repetitive tasks. Unlike the traditional while loop, the do-while loop guarantees the execution of its body at least once, making it particularly useful in certain scenarios. In this article, we will explore the do-while loop statement in C++, its syntax, functionality, and best practices.
What Are Loops & Its Types In C++?
Loops are programming constructs that allow for the repetitive execution of a block of code. They provide a convenient way to automate tasks and iterate over collections of data. In C++, there are three main types of loops, as mentioned below:
- While Loop: The while loop repeatedly executes a block of code as long as a specified condition is true.
- Do-While Loop: The do-while loop is similar to the while loop but with one crucial difference: the condition is checked after the loop body is executed. This guarantees that the loop body is executed at least once.
- For Loop: The for loop is used when you know the exact number of iterations in advance. It consists of three parts: initialization, condition, and update.
What Is A Do-While Loop In C++?
A do-while loop is a particular kind of loop structure used in C++ that enables a block of code to be run repeatedly until a specific condition is satisfied. The condition for the loop is checked at the end of each iteration rather than at the beginning, which is a key distinction from a while loop.
Do-While Loop Syntax In C++
do {
// Code block to be executed
} while (condition);
Here,
- The do keyword initiates the loop
- The code inside the curly brackets is what must be executed.
- While (condition) is the statement that must be verified as true for the loop to keep running.
Whether the condition is initially true or false, the code block enclosed by the curly braces will be run at least once in this case. The condition is verified following the execution of the code block. The loop will run again and repeat the process if the condition is true. If the predicate is false, the loop will end, and control will move on to the next statement.
Do-while loops come in handy when you want to guarantee that a block of code is run at least once, whether the condition is true or false at first.
Do-While Loop Example In C++ To Print Numbers
Using a do-while loop in C++ to display numbers from 1 to 10 is demonstrated here:
Output:
1
2
3
4
5
6
7
8
9
10
Explanation:
- Here, we declare the integer variable i and set its initial value to 1, inside the main function.
- Then we enter the do block and use the cout statement to print the value of i to the console.
- The increment operator and i++ statement are then used to increase the value of i by 1.
Note: The increment statements are also known as update expressions since they update the loop by a pre-specified value after the execution of the loop body. - Then the loop condition i=10 is verified following the execution of the code block inside the do block.
- The loop will run once more and repeat the process as long as the value of i is less than or equal to 10.
- The loop ends when the value of i reaches 11. At that point, the loop's condition is no longer true.
How Does A Do-While Loop In C++ Work?
Here is a detailed explanation of how a C++ do-while loop functions and runs:
- Regardless of whether the condition is true or false, the code block contained within the do statement is executed first.
- The condition inside the while statement is then assessed after the code block inside the do statement has been run. This initial condition is also referred to as the test expression.
- The loop returns to step 1 and runs the code block once more if the condition is satisfied. Every time the loop gets updated by a certain value, the expression used to get this done is the update expression. For example- increment and decrement statements.
- The loop ends, and the control moves to the statement that follows it if the condition is false.
- Up until the condition in the while statement turns out to be false, steps 1 and 2 are repeated.
The key distinction between a while loop and a do-while loop is that a while loop only executes the code block inside if the condition is initially true. Whereas a do-while loop executes the code block inside at least once, whether the condition is initially true or false.
Here is an illustration of how the loop works:
As is evident from the diagram above,
- The do-while loop first executes the code block before determining whether the loop is still in place. That is, the loop body is executed at least once regardless of the loop condition being met.
- If the loop condition is satisfied, the code block is run once more, and the condition is tested/ checked again. The loop condition is verified following each execution of the code block.
- This procedure iterates, and the loop ends only when the condition is false. At this point, the loop is broken, and the remainder of the program resumes execution.
Various Components Of The Do-While Loop In C++
Here are the components of a C++ do-while loop:
The word "do": The code block that will be run at least once comes after this and begins the do-while loop.
Code block: This block of code is enclosed in curly braces and will be run at least once.
The word "while": The loop condition, which is checked following each execution of the code block, is specified using this.
The expression in the loop condition determines whether the loop should keep running or end. The code block is run once more if the loop condition is satisfied. The loop ends, and the program continues running if the condition is false.
Semicolon: A semicolon (;) marks the conclusion of the do-while loop statement.
Here is an illustration of a C++ do-while loop that asks the user to enter a number and keeps asking until the correct number is entered:
Code:
Output:
Enter a number: 0
Enter a number: 11
Enter a number: 5
You entered 5
Explanation:
- We begin by including the iostream file and namespace std.
- Next, we declare an integer variable num inside the main() function.
- Then the do keyword initiates the do-while loop, and the code block uses the cout statement to prompt the user to enter a number.
- The program uses the cin statement to scan and read the input fed in.
- The loop then checks the condition following the while keyword to determine if the input number is greater than 10 or less than 0.
- If this condition is met, then the code block is run once more.
- Here, since the input is 0 and 11, which meets the condition, the loops keep on running.
- When the user inputs a value that breaks the condition, like 5 here, the condition turns false, and the loop ends.
- The program then continues running after the loop.
Example 2: Adding User-Input Positive Numbers With Do-While Loop
The example below shows how we can add positive numbers (provided by users) using the do-while loop in C++.
Code:
Output:
Enter a positive number (or enter 0 to exit): 10
Enter a positive number (or enter 0 to exit): 25
Enter a positive number (or enter 0 to exit): 5
Enter a positive number (or enter 0 to exit): 0
The sum of the positive numbers entered is: 40
Explanation:
- The program in this example first initializes two variables, num to store the user-entered number and sum to record the sum of any positive numbers entered.
- Then, the do keyword initiates the do-while loop, which runs the code block at least once.
- The code block uses the cout statement to print Enter a positive number (or enter 0 to exit) to prompt the user to input numbers.
- It then uses the cin statement is used to read the input.
- We initiate an if statement inside the do-while loop, which defines sum as the addition of the inputted numbers until the numbers are greater than 0. We use the (+=) operator for this.
- The do-while loop continues until the user enters 0. Here, the user input is 10, 25, 5, and 0. At this point, the loop terminates, and the next line of the program is executed.
- Next, we use the cout statement once again to print the sum total of the input numbers. This is 40, as shown in the output window.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
C++ Nested Do-While Loop
A do-while loop inside another loop is referred to as a nested do-while loop in C++. Note here the inner loop executes multiple times for each iteration of the outer loop.
Syntax:
In C++, a nested do-while loop has the following basic structure:
do {
// Outer loop code block
do {
// Inner loop code block
} while (inner_loop_condition);
} while (outer_loop_condition);
Note: The inner loop is executed first in this structure, followed at least once by the outer loop. For each iteration of the outer loop, the inner loop is run multiple times or until the inner loop condition is false. The outer loop condition is examined after the inner loop has finished. The process is repeated, starting with the inner loop, if the outer loop condition is met.
How Nested Do While Loop Work In C++?
The operation of a nested do-while loop is described in the following detail:
- The outer do-while loop is run for the first time by the program. If the outer loop condition is true, it is checked, and the inner loop's code is then run.
- The program runs the inner loop for the first time inside the outer loop. When the inner loop condition is verified as true, the inner loop's code is run.
- The control returns to the outer loop after the inner loop has finished its iteration.
- If the outer loop condition is still true, the inner loop is run once more by the program.
- This process keeps repeating until the inner loop condition turns false.
- When the outer loop condition is true, and the inner loop condition is false, control returns to the outer loop. The process is repeated, starting with the inner loop, if the outer loop condition is still true.
After the nested do-while loop, the program continues running if the outer loop condition is false. Otherwise, the loop ends.
Example of a Nested Do-While Loop in C++
The multiplication table of numbers from 1 to 5 is shown in the following nested do-while loop in the C++ example.
Code:
Output:
The program prints the multiplication tables of 1, 2, 3, 4, and 5.
Explanation:
- We begin by including the iostream file to use the cout statements.
- Then inside the main() function, we declare two variables, i and j, and initialize them with a value of 1.
- The variable i is used in the outer loop to track the number that the multiplication table is being displayed for.
- The inner loop cycles through the numbers 1 through 10 and displays the multiplication table using the variable j.
- For each iteration of the outer loop, the outer loop executes values of i from 1 to 5. And the inner loop executes for values of j from 1 to 10.
- The value of i is increased by 1 when the inner loop ends, using the increment operator. And the value of j is set back to 1 for the outer loop's subsequent iteration.
- Here we have used the cout print statement to print the output in the window.
C++ Infinitive Do-while Loop
In C++, an infinite do-while loop occurs when the loop's condition is always true. When this occurs, the loop will keep running indefinitely until all available memory has been used. Setting the condition statement to true (while(true)) or omitting the increment/decrement statement from the body are two approaches to create an endless do-while loop.
Syntax
In C++, the syntax of an infinite do-while loop is similar to that of a regular do-while loop. The only exception is the absence of an exit/ termination condition.
do {
// code to be executed repeatedly
} while(true);
Here,
- The word do denotes the start of the loop block, which contains the code that will be run repeatedly.
- The keyword while is then followed by the requirement for breaking the loop, in this case, just true.
- The loop will continue to run infinite times until an outside event happens or the program is stopped because true is always true.
It's crucial to remember that an infinite do-while loop can be risky if used carelessly because it can result in an infinite loop that uses up all the computer's resources and crashes the program. As a result, having a way to exit the loop using a break statement or another control structure is crucial.
Setting the condition as true:
An infinite do-while loop in C++ is a loop that runs without an end condition indefinitely. When you want to repeatedly run a section of code until an outside event happens, like pressing a certain key or getting a signal, an infinite do-while loop can be helpful.
Let's look at an illustration to better understand this concept. Below are two code examples where a C++ infinite do-while loop asks the user for a number up, and then a sum is calculated. The first code does not have an exit condition and runs infinitely. The second code has an exit condition, that is- entering a negative number.
Code:
Explanation:
Since the do-while loop condition is true in this illustration, the loop will continue to run indefinitely unless an outside event occurs. The loop uses the cin statement to read numbers from the user, adds them to the sum variable with the += operator, and then repeats this process indefinitely.
Using a break statement inside the loop will immediately end the loop and move control outside of it, allowing you to escape the infinite loop. For instance, you could change the aforementioned program so that it ends the loop when the user enters a negative number as follows:
In this modified program, until the user enters a negative number, the loop runs endlessly. The "break" statement is executed when the user enters a negative number, ending the loop and moving the control outside of it.
Eliminating The Increment/ Decrement Statement
It's also possible to create an infinite do-while loop execution in C++ without using any increment or decrement block of statements. When you want to repeatedly run a section of code without changing any values, this can be helpful. The example above showcases this concept.
What is the Difference Between While Loop and Do While Loop in C++?
The while loop and the do-while loop are two examples of loops in C++ that let you repeatedly run a section of code. The order in which these two loops check the loop condition is the primary distinction between them.
The distinctions between the C++ while loop and do-while loop are listed in the following table:
While Loop |
Do-While Loop |
|
Syntax |
while (condition) { code } |
do { code } while (condition); |
Condition Check |
The loop condition is checked at the beginning of the loop. If the condition is false, the loop code is never executed. |
The loop condition is checked at the end of the loop. The loop code is always executed at least once, even if the condition is false. |
Use Case |
Use it when you want to execute the loop code zero or more times, depending on the condition. |
Use when you want to execute the loop code at least once, regardless of the condition. |
Let's examine a few instances to understand this distinction better:
Example 1: While loop
Output:
The count is: 0
The count is: 1
The count is: 2
The count is: 3
The count is: 4
Explanation:
According to the circumstances, the while loop runs the loop code zero or more times in this example. At the start of the loop, the condition is verified. The loop code is run if the condition is satisfied. The loop is ended, and the control is moved outside if the condition is false.
Example 2: Do-While Loop
Output:
The count is: 0
The count is: 1
The count is: 2
The count is: 3
The count is: 4
Explanation:
The do-while loop is used to run the loop code at least once in this example, regardless of the condition. At the conclusion of the loop, the condition is examined. Once the loop's code has run, the condition is then verified. The loop code is repeated if the condition is true. The loop is ended, and the control is moved outside if and when the condition is false.
When To Use A Do-While Loop?
When you want to run a block of code at least once in C++ and then keep running the loop until a specific condition is satisfied, you can use a do-while loop. Listed below are some scenarios in which a do-while loop might be a wise decision:
- User Input: A do-while loop can be used to obtain user input while also ensuring that the input is valid. For instance, you can use a do-while loop to keep prompting the user until they enter a valid number if you're asking them to enter a number between 1 and 10.
- When creating a menu-driven program, write a do-while loop to display the menu options and keep asking the user for input until they choose to quit the program.
- Game Loops: A do-while loop can be used to keep a game running until the player decides to stop playing it. To display the game screen, update the game state, and await the player's input, for this instance, it will be beneficial to use a do-while loop.
- Processing Input: You can use a do-while loop to read the input and keep processing it until you reach the end of the file or stream when processing input from a file or a network stream.
A do-while loop is typically a wise choice when you need to run a block of code at least once before continuing to do so until a specific condition is met. Remember that a do-while loop wastes time by executing the loop code once when the loop condition is false at the first check, making it less efficient than a while loop.
Conclusion
The do-while loop is a valuable addition to the programmer's toolkit, offering a reliable way to execute code at least once and allowing for versatile control flow. By understanding its syntax and best practices, you can leverage the power of the do-while loop to write efficient and robust programs in C++. Happy coding!
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. Can you do a while loop within a for loop?
In C++, a while loop can indeed be inserted inside a for loop. Such a loop is referred to as a nested loop. In a nested loop, the inner while loop repeatedly runs its code block until its condition is met, at which point control is returned to the outer for loop. The process continues until the for loop's condition is met, at which point the for loop iterates once more.
Such nested loops are useful when performing a repetitive task that calls for multiple iterations, each of which involves a different set of repetitive tasks.
For example, you can use a nested loop to print a multiplication table where the outer loop iterates through the rows. And the inner loop iterates through the columns. In general, nested loops can be applied to any circumstance in which it is necessary to carry out a repetitive task inside another repetitive task.
Q. What are the three types of loops?
There are three primary kinds/ types of loops in C++. They are- the while loop, the do-while loop, and the for loop. These loops enable you to repeatedly run a block of code until a predetermined condition is satisfied.
The simplest kind of loop in C++ is- the while loop. Here, the loop condition is checked at the start of each iteration, and the code is executed only if the condition is true. The while loop syntax is as follows:
while (condition) {
// loop code
}
While the while loop checks the loop condition at the start of each iteration, the do-while loop does so at the end of each iteration. This indicates that even if the condition is false, the loop code is run at least once. A do-while loop's fundamental syntax is as follows:
do {
// loop code
} while (condition);
One line can contain the loop condition, the initialization code, and the update code when using the more complex for loop. When you know the number of iterations in advance, you usually use it. Here is a for loop's basic syntax:
for (initialization; condition; update) {
// loop code
}
You can control the loop's progression in all three types of loops by using the break and continue statements. You can skip to the next iteration with the continue statement, while you can exit the loop early with the break statement.
In general, loops are an effective tool in C++ that enables you to repeatedly run a block of code until a specific condition is met. You can write C++ code that is more effective and efficient if you know the various loop types and how to use them.
Q. What type of loop is a while loop in C++?
While a specific condition is true, a while loop, a type of conditional loop, repeatedly runs a block of code. If the condition is true, the loop's associated code block is then executed. The loop begins by evaluating the condition. The cycle repeats until the condition is no longer true, at which point it ends, and the control statement is transferred to the following program statement.
While loops come in handy when you are unsure of how many times you will run a code block or when you want to run a code block indefinitely until a specific condition is met. However, it's crucial to make sure that the condition eventually fails; otherwise, the loop will run forever and become infinite.
Q. What is the difference between a for loop and a do while loop in C++?
A do-while loop executes its block of code at least once before checking the loop condition, in contrast to a for loop, which depends on a condition that is checked before the loop and executes its block of code zero or more times.
Before the body of loop is carried out in a for loop, the condition is checked, and if it is initially false, the loop body is not carried out at all. In contrast, a do-while loop runs the loop body at least once before checking the loop condition to see if the loop should continue or not.
Another distinction is that in a do-while loop, the body of the loop is executed first, followed by the iteration statement before the loop condition is checked, whereas in a for loop, the initialization and iteration looping statements are only executed after the loop condition is checked.
In general, while do-while loops are useful when you want to perform an action at least once and then repeat it based on a condition, for loops are useful when you want to iterate over a fixed range or collection of elements.
Q. What are the four parts of a loop in C++?
A loop in C++ typically consists of four components:
- Initialization: You initialize any variables that will be used in the loop during this section of the loop. Just once, just before the loop begins, this step is carried out.
- Condition: In the second section of the loop, the condition that will determine whether or not the loop should continue to run is specified. As long as this condition is met, the loop will keep running.
- Body: In the third iteration of the loop, you specify the code block that will be run repeatedly for the duration of the true condition.
- Iteration: The code that will be run after each iteration of the loop is specified in the fourth section of the loop. In order to eventually make the condition of the loop false and end the loop, this step is used to change the variables used in the condition.
In C++, these four elements combine to form a looping construct that enables you to run a block of code repeatedly so long as a specific condition is met. The iteration step modifies the loop variables used in the condition so that the loop will eventually come to an end. The initialization step creates any necessary variables; the condition determines when the loop should end, and the body executes the code inside the loop.
You might also be interested in reading the following:
- References In C++ | Declare, Types, Properties & More (+Examples)
- Logical Operators In C++ | Use, Precedence & More (With Examples)
- Strings In C++ | Functions, How To Convert & More (With Examples)
- Pointers in C++ | A Roadmap To All Types Of Pointers With Examples
- Storage Classes In C++ & Its Types Explained (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment