Table of content:
- What Is Multilevel Inheritance In C++?
- Block Diagram For Multilevel Inheritance In C++
- Multilevel Inheritance In C++ Example
- Constructor & Multilevel Inheritance In C++
- Use Cases Of Multilevel Inheritance In C++
- Multiple Vs Multilevel Inheritance In C++
- Advantages & Disadvantages Of Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
Multilevel Inheritance In C++ | Syntax, Uses And More (+Examples)
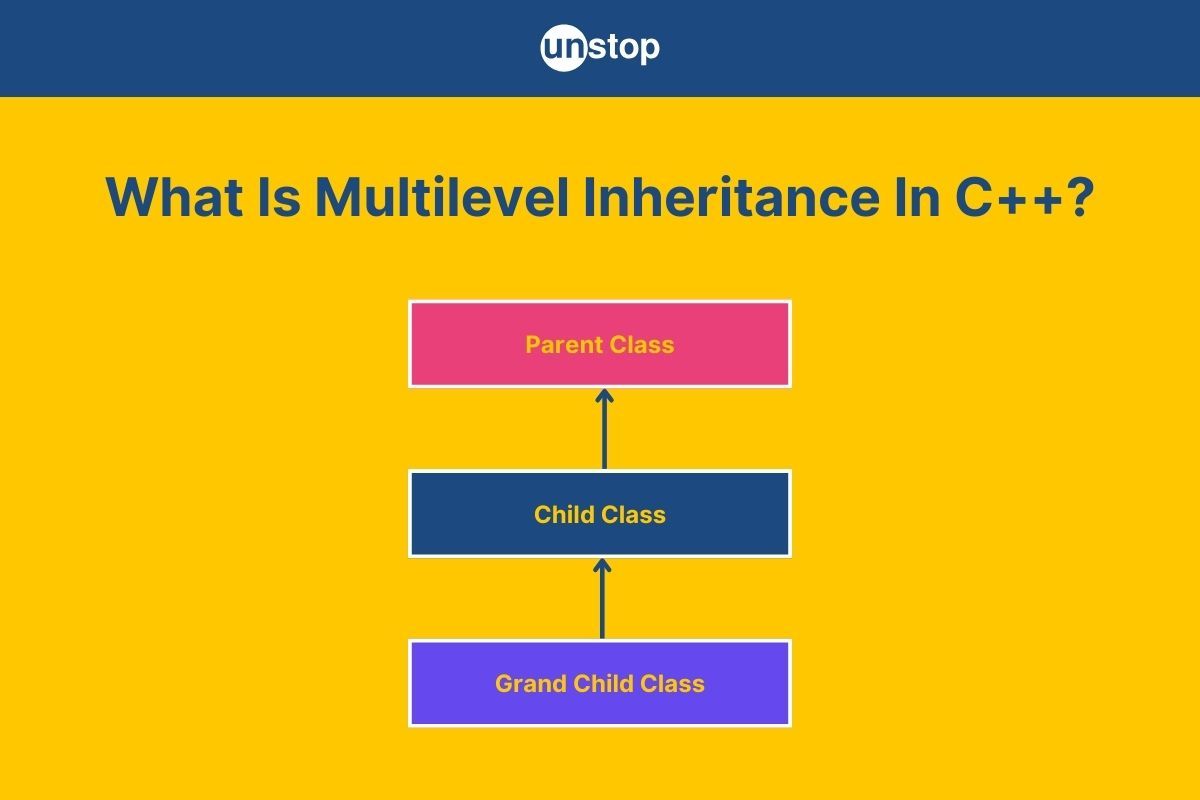
Multilevel inheritance in C++ is a powerful feature that allows classes to inherit from other derived classes. This concept has roots in object-oriented programming, making it easier to manage and organize code. By creating a hierarchy of classes, developers can promote code reusability and enhance maintainability.
Understanding multilevel inheritance is essential for writing efficient C++ programs. In this article, we will explore the intricacies of multilevel inheritance, its advantages and disadvantages, and how it can be effectively implemented in C++ to design robust and maintainable software systems.
What Is Multilevel Inheritance In C++?
Multilevel inheritance in C++ programming is a type of inheritance where a class is derived from another class, which in turn is derived from yet another class. This forms a chain of inheritance across multiple levels, where each class in the hierarchy inherits properties and behaviours from the one above it.
For Example: Imagine a hierarchy of classes representing living beings:
- Class A: The base class that represents the general concept of living beings.
- Class B: Derived from class A, representing animals specifically.
- Class C: Derived from class B, representing a specific type of animal, like birds.
Syntax Of Multilevel Inheritance In C++
class BaseClass {
// Members and methods of the base class
};class DerivedClass : accessSpecifier BaseClass {
// Members and methods of the derived class
};class FurtherDerivedClass : accessSpecifier DerivedClass {
// Members and methods of the further derived class
};
Here:
- The Base Class is the top-level class from which other classes are derived. It defines the common functionality.
- The Derived Class is a class that inherits from the base class and may introduce additional members or methods.
- The Further Derived Class is a class that inherits from the Derived Class, forming a multilevel hierarchy.
Block Diagram For Multilevel Inheritance In C++
The block diagram for multilevel inheritance in C++ visually represents the relationship between the classes to show how the inheritance chain works.
-
BaseClass:
-
The first block at the top (i.e. Class A) represents the BaseClass.
-
This is the foundation of the hierarchy and contains members like variables, methods, etc., that the lower levels will inherit.
-
-
DerivedClass:
-
The second block (i.e. Class B) represents the DerivedClass.
-
This class inherits from the BaseClass (indicated by the arrow from Class B to Class A).
-
DerivedClass can access and reuse the members of the BaseClass and may also introduce its own members and other functions.
-
-
FurtherDerivedClass:
-
The third block (i.e. Class C) represents the FurtherDerivedClass.
-
It inherits from the DerivedClass (indicated by the arrow from Class C to Class B).
-
Since FurtherDerivedClass is two levels below BaseClass, it can access members from both DerivedClass and BaseClass, forming a multilevel inheritance chain.
-
Multilevel Inheritance In C++ Example
Let’s now look at a simple code example to understand the implementation of multilevel inheritance in C++:
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCi8vIEJhc2UgY2xhc3MgKExldmVsIDEpCmNsYXNzIEFuaW1hbCB7CnB1YmxpYzoKICAgIHZvaWQgZWF0KCkgewogICAgICAgIGNvdXQgPDwgIkFuaW1hbCBpcyBlYXRpbmcuIiA8PCBlbmRsOwogICAgfQp9OwoKLy8gRGVyaXZlZCBjbGFzcyAoTGV2ZWwgMikgaW5oZXJpdGluZyBmcm9tIEFuaW1hbApjbGFzcyBNYW1tYWwgOiBwdWJsaWMgQW5pbWFsIHsKcHVibGljOgogICAgdm9pZCB3YWxrKCkgewogICAgICAgIGNvdXQgPDwgIk1hbW1hbCBpcyB3YWxraW5nLiIgPDwgZW5kbDsKICAgIH0KfTsKCi8vIEZ1cnRoZXIgZGVyaXZlZCBjbGFzcyAoTGV2ZWwgMykgaW5oZXJpdGluZyBmcm9tIE1hbW1hbApjbGFzcyBEb2cgOiBwdWJsaWMgTWFtbWFsIHsKcHVibGljOgogICAgdm9pZCBiYXJrKCkgewogICAgICAgIGNvdXQgPDwgIkRvZyBpcyBiYXJraW5nLiIgPDwgZW5kbDsKICAgIH0KfTsKCmludCBtYWluKCkgewogICAgRG9nIG15RG9nOwoKICAgIC8vIEFjY2Vzc2luZyBtZW1iZXJzIG9mIGFsbCBjbGFzc2VzCiAgICBteURvZy5lYXQoKTsgIC8vIEZyb20gQW5pbWFsIGNsYXNzCiAgICBteURvZy53YWxrKCk7IC8vIEZyb20gTWFtbWFsIGNsYXNzCiAgICBteURvZy5iYXJrKCk7IC8vIEZyb20gRG9nIGNsYXNzCgogICAgcmV0dXJuIDA7Cn0K
Output:
Animal is eating.
Mammal is walking.
Dog is barking.
Explanation:
In the above code example, we represent a multilevel inheritance structure in C++:
- We start by defining a base class Animal which represents general animal behavior.
- Next, we define a class mammal which is derived from Animal. It inherits the behavior of Animal and adds specific behaviors for mammals.
- Then we define another class dog which is derived from Mammal. It adds dog-specific behavior to the mammal behaviors.
- Now in the main() function, when an object of the Dog class is created, it can access methods from all levels of the inheritance chain (eat() from Animal, walk() from Mammal, and bark() from Dog).
Constructor & Multilevel Inheritance In C++
A constructor in C++ is a special member function of a class that is automatically called when an object of the class is created. It is used to initialize the object's members. Through the example given below, we will see how constructors can be implemented using multilevel inheritance in C++:
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCi8vIEJhc2UgY2xhc3MgKExldmVsIDEpCmNsYXNzIFBlcnNvbiB7CnB1YmxpYzoKICAgIC8vIEJhc2UgY2xhc3MgY29uc3RydWN0b3IKICAgIFBlcnNvbigpIHsKICAgICAgICBjb3V0IDw8ICJQZXJzb24gY29uc3RydWN0b3IgY2FsbGVkLiIgPDwgZW5kbDsKICAgIH0KfTsKCi8vIERlcml2ZWQgY2xhc3MgKExldmVsIDIpIGluaGVyaXRpbmcgZnJvbSBQZXJzb24KY2xhc3MgRW1wbG95ZWUgOiBwdWJsaWMgUGVyc29uIHsKcHVibGljOgogICAgLy8gRGVyaXZlZCBjbGFzcyBjb25zdHJ1Y3RvcgogICAgRW1wbG95ZWUoKSB7CiAgICAgICAgY291dCA8PCAiRW1wbG95ZWUgY29uc3RydWN0b3IgY2FsbGVkLiIgPDwgZW5kbDsKICAgIH0KfTsKCi8vIEZ1cnRoZXIgZGVyaXZlZCBjbGFzcyAoTGV2ZWwgMykgaW5oZXJpdGluZyBmcm9tIEVtcGxveWVlCmNsYXNzIE1hbmFnZXIgOiBwdWJsaWMgRW1wbG95ZWUgewpwdWJsaWM6CiAgICAvLyBGdXJ0aGVyIGRlcml2ZWQgY2xhc3MgY29uc3RydWN0b3IKICAgIE1hbmFnZXIoKSB7CiAgICAgICAgY291dCA8PCAiTWFuYWdlciBjb25zdHJ1Y3RvciBjYWxsZWQuIiA8PCBlbmRsOwogICAgfQp9OwoKaW50IG1haW4oKSB7CiAgICAvLyBDcmVhdGluZyBhbiBvYmplY3Qgb2YgTWFuYWdlciBjbGFzcwogICAgTWFuYWdlciBtYW5hZ2VyMTsKCiAgICByZXR1cm4gMDsKfQo=
Output:
Person constructor called.
Employee constructor called.
Manager constructor called.
Explanation:
In the above code example:
- We start by defining a base class Person, that represents a general person, and its constructor prints "Person constructor called."
- Next, we define class Employee which is dervied from Person, representing an employee, and its constructor prints "Employee constructor called."
- Similarliy, we define a class Manager which is derived from Employee, representing a manager, and its constructor prints "Manager constructor called."
- When an object of Manager is created, the constructors are called in order: Person, Employee, and then Manager, demonstrating the order of constructor calls in multilevel inheritance.
Use Cases Of Multilevel Inheritance In C++
Multilevel inheritance is useful in many real-world applications. It helps model complex systems clearly. Some of its important features and use cases are as follows:
- Hierarchical Classification: Multilevel inheritance is useful for modelling real-world hierarchies. For instance, in a class hierarchy representing animals, you might have a base class Animal, a derived class Mammal, and further derived classes like Dog and Cat. This allows for a clear representation of relationships and shared behaviors.
- Specialized Functionality: When you want to create specialized versions of a class while retaining the core functionality of its base classes, multilevel inheritance is effective. For example, a base class Shape could have a derived class Polygon, which in turn could have further derived classes like Triangle and Rectangle, each implementing specific behaviors related to their shapes.
- Game Development: In game development, multilevel inheritance can be used to create a hierarchy of game entities. A base class GameObject could be extended by Character, which could then be further derived into classes like Player and NPC (non-playable character).
- User Interface Components: When building graphical user interfaces (GUIs), multilevel inheritance can help organize UI components. A base class Widget can have derived classes like Button, which can be further specialized into ImageButton or TextButton, allowing for shared properties and behaviors while also supporting specific implementations.
- Data Modeling: Multilevel inheritance is beneficial in scenarios where data can be organized in a hierarchical structure, such as in organizational charts or product categories. For example, a base class Product can be extended by Electronics, which in turn can have derived classes like Mobile and Laptop, enabling efficient data handling and organization.
Multiple Vs Multilevel Inheritance In C++
Multiple inheritance in C++ allows a class to inherit from more than one base class. In contrast, multilevel inheritance involves a chain of inheritance, where a class is derived from another class, which is itself derived from yet another class. Given below are the key differences between the two:
Feature | Multiple Inheritance | Multilevel Inheritance |
---|---|---|
Definition | A class inherits from two or more base classes. | A class inherits from a class which is already derived from another class. |
Number of Parent Classes | Multiple base classes are involved. | There is only one parent class per level, but inheritance occurs across multiple levels. |
Structure | Horizontal (one class inherits from multiple classes at the same level). | Vertical (class hierarchy extends across levels). |
Syntax | class Derived : public Base1, public Base2 { }; | class Derived : public Base { }; |
Complexity | It can introduce complexity due to ambiguity in inherited members (resolved using virtual inheritance). | It is simpler than multiple inheritance, with a clear inheritance chain. |
Example | class A {}; class B {}; class C : public A, public B {}; | class A {}; class B : public A {}; class C : public B {}; |
Advantages & Disadvantages Of Multilevel Inheritance In C++
Multilevel inheritance is a powerful feature in C++ that allows classes to be organized hierarchically, facilitating code reuse and specialization. However, it also comes with complexities and challenges that developers must consider when designing their class structures.
Advantages Of Multilevel Inheritance In C++
- Organized Structure: Multilevel inheritance allows for a clear and organized class hierarchy, making it easier to understand relationships between classes and the shared functionality they provide.
- Code Reusability: Common functionality defined in base classes can be reused in derived classes, reducing code duplication and improving maintainability.
- Specialization: It enables the creation of specialized classes that inherit characteristics from multiple levels, allowing for specific behaviors while retaining shared attributes.
- Easy Maintenance: Changes made in the base class can automatically propagate to derived classes, simplifying maintenance and reducing the risk of errors.
- Encapsulation: Multilevel inheritance supports encapsulation by allowing classes to manage their state and behavior while exposing only what is necessary to derived classes.
Disadvantages Of Multilevel Inheritance In C++
- Increased Complexity: The class hierarchy can become complex and difficult to understand, especially with many levels, which may lead to challenges in managing and maintaining the code.
- Tight Coupling: Derived classes can become tightly coupled with their base classes, making it harder to modify one without affecting the others, thus reducing flexibility.
- Difficult Debugging: Tracing bugs can be more challenging due to multiple inheritance levels, complicating the debugging process and making it harder to identify the source of issues.
- Overhead from Virtual Functions: If the classes use virtual functions, there is additional memory overhead for the VTable, which can impact performance and increase memory usage.
- Constructor and Destructor Complexity: The order of constructor and destructor calls can lead to unintended behavior if not managed properly, particularly when dealing with resource management and memory allocation.
- Potential for Inheritance Anomalies: Although less common in multilevel inheritance than in multiple inheritance, issues like the "Diamond Problem" can still arise, leading to ambiguity and conflicts in method resolution.
Conclusion
Multilevel inheritance in C++ is a powerful and flexible feature that facilitates the creation of organized class hierarchies, promoting code reusability and specialization. By allowing classes to inherit properties and behaviors from multiple levels of derived classes, developers can create complex systems that mirror real-world relationships, enhancing both the functionality and maintainability of their applications.
However, it is essential to be mindful of the potential drawbacks, such as increased complexity and tighter coupling between classes, which can complicate maintenance and debugging. By understanding the intricacies of multilevel inheritance and applying best practices, developers can harness its benefits while mitigating its challenges, leading to more efficient and effective software design.
Frequently Asked Questions
Q. What is the difference between multilevel inheritance and single inheritance in C++?
Multilevel inheritance in C++ involves a chain of classes where a derived class inherits from another derived class. In contrast, single inheritance has only one base class and one derived class. This allows for more complex relationships in multilevel inheritance. The key differences are as follows:
Feature | Single Inheritance | Multilevel Inheritance |
---|---|---|
Definition | A class inherits from only one base class. | A class inherits from another derived class. |
Hierarchy Structure | Linear hierarchy (one level). | Hierarchical structure (multiple levels). |
Number of Parent Classes | One parent class only. | More than one class in the inheritance chain. |
Example Structure | class Derived : public Base { }; | class Derived : public Base { }; class FurtherDerived : public Derived { }; |
Complexity | Simpler and easier to understand. | More complex due to multiple levels. |
Use Case | Suitable for simple relationships. | Suitable for representing a hierarchy of related classes. |
Access to Members | Can only access members of the single base class. | Can access members of all ancestor classes. |
Q. Are there any disadvantages to multilevel inheritance?
Multilevel inheritance in C++ comes with several disadvantages that can complicate software design and maintenance. First, it increases complexity, making the class hierarchy harder to understand, especially with more levels. This complexity can lead to tight coupling, where changes in a base class necessitate adjustments in derived classes, complicating maintenance.
Debugging also becomes more challenging due to the multiple levels of inheritance, making it difficult to trace issues back to their source. Additionally, the order of constructor and destructor calls can cause unexpected behaviors if not managed carefully, especially concerning resource allocation and deallocation.
Q. What is multilevel inheritance in C++?
Multilevel inheritance is a type of inheritance in C++ where a class (derived class) inherits from another derived class, creating a hierarchy of classes. This allows for multiple levels of inheritance, enabling more complex relationships between classes.
Q. How does multilevel inheritance affect memory usage in C++?
In multilevel inheritance, memory consumption increases as each derived class adds its own attributes. However, proper design minimizes unnecessary memory usage by ensuring only essential data is inherited. Additionally, if virtual functions are used, there is memory overhead for storing the VTable.
Q. Can virtual functions be used with multilevel inheritance in C++?
Yes, virtual functions can be used with multilevel inheritance in C++. When a base class declares a member function as virtual, it allows derived classes in the multilevel hierarchy to override that function, enabling dynamic polymorphism. This means that the appropriate function to be called is determined at runtime based on the type of the class object, rather than the type of the reference or pointer.
In a multilevel inheritance scenario, if a base class has a virtual function and derived classes override this function, then when a pointer or reference to the base class is used to call the function, the most derived class's implementation will be executed.
Q. Is multilevel inheritance supported in other programming languages?
Yes, many object-oriented languages like Java and Python support multilevel inheritance. While syntax may vary, the core concept remains consistent across these languages, allowing for similar benefits in code structure and reusability.
You might also be interested in reading the following:
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Bitwise Operators In C++ Explained In Detail With Examples
- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
- Friend Function In C++ | Class, Global Use, & More! (+Examples)
- C++ Templates | Class, Function, & Specialization (With Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment