C++ Constructors | Default, Parameterised, Copy & More (+Examples)
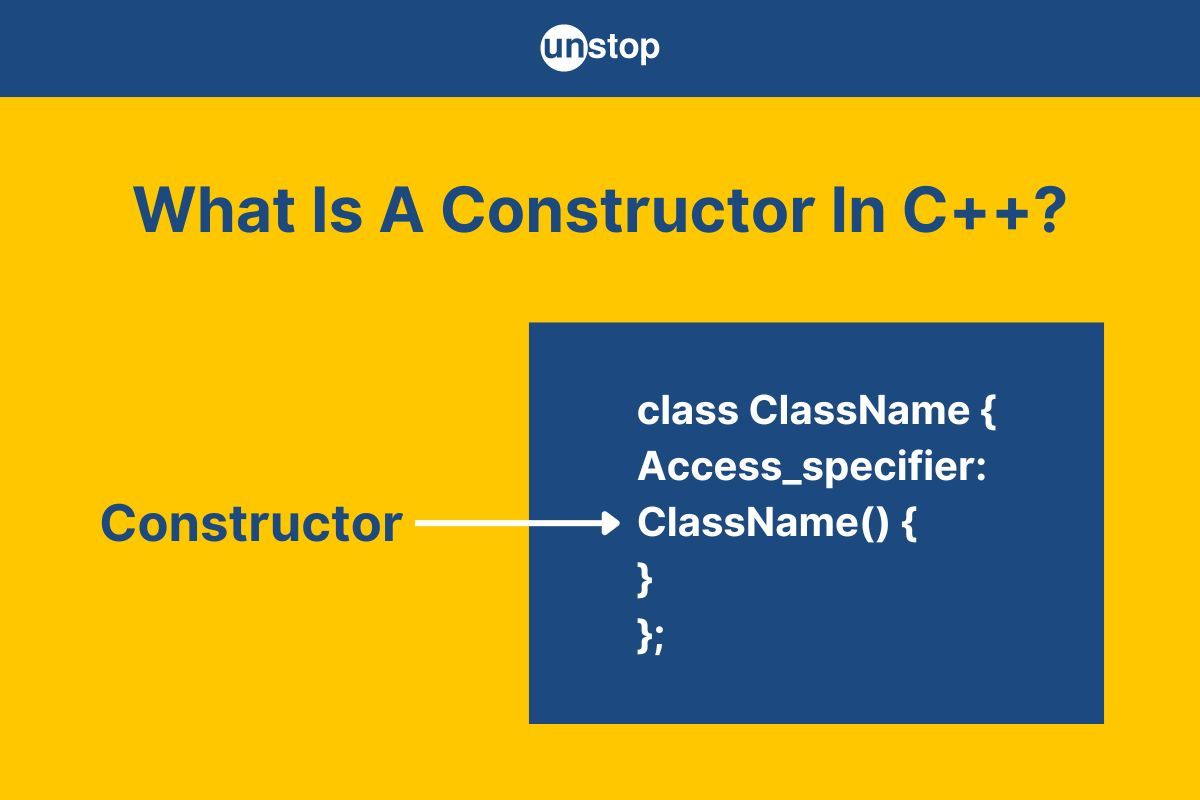
The C++ programming language's ability to support OOPs concepts sets it apart from its predecessors. Two core concepts that make this OOP implementation possible are classes and objects. As you may know, classes enclose attributes and behaviours that materialize using objects. An important part of any class is a constructor, which is functional in the creation of these objects and their attributes. In this article, we will explore the concept of constructors in C++, its types, characteristics, overloading, and more.
What Is Constructor In C++?
A constructor in C++ programming is a special member function that is called automatically when an object belonging to the respective class is created. It is the responsibility fo the constructors to initialize the object's data members and establish its initial state. In other words, it lets you instantiate the initial state of an object. It is just like a recipe that you follow when creating the object, making sure the object starts with the right characteristics and values.
- They make sure that an object is created with valid and consistent values, establishing the foundation for the object's functionality and behavior.
- Constructors in C++ are particularly helpful for allocating resources, setting initial values, and setting up the object for use immediately after creation.
- They have the same name as the class name and don't have the return type, not even void.
Given below is a syntax followed by an example of a constructor in C++.
Syntax Of Constructor In C++:
class ClassName {
Access_specifier:
ClassName() {
}
};
Here,
- The class keyword is used for class declaration whose name is given by ClassName.
- The access_specifier specifies the data visibility. This can be public, private, or protected.
- The constructor is given by ClassName(). As you can see, the name/ identifier is the same as the class.
Code Example:
Output:
Person 1: Name: Unknown, Age: 0
Person 2: Name: Unstop, Age: 7
Explanation:
In this basic C++ program example, we begin by including the <iostream> library for input/output operations, <string.h> for string operations and use the namespace std directive to avoid needing std:: prefixes for standard library elements.
- We then define a class called Person to represent a person with two private attributes/ data members, i.e., name (string data type) and age (integer data type). This means that these members cannot be accessed directly from outside the class.
- The Person class has two constructors. As mentioned in the code comments, the first constructor is the default constructor, which initializes the name attribute to Unknown and the age attribute to 0.
- The second constructor is a parameterized constructor that takes a constant reference to the name variable (string type) and the age variable (integer type) as parameters and sets the respective attributes using the 'this' pointer.
- We also define a member function of the class called display(), which prints the name and age attributes using cout statement.
- In the main() function, we create two objects of the Person class. Here, we use the default constructor for creation of object person1.
- We use the parameterized constructor with values "Unstop" and 7 for creation of object person2.
- Then, we call the display() method on both person1 and person2 to print their information.
- Finally, the program terminates with a return 0 statement indicating successful execution to the operating system.
Another Way Of Defining Constructor In C++
In the section above we discussed how to declare and define a constructor in C++ inside the class. Alternatively, you can also define it outside of a class when writing a C++ program. However, the constructor declaration must be made inside the class itself.
When a constructor is defined outside of a class, there are specific syntax changes that need to be observed. This involves defining the constructor's implementation outside the class definition, often using the scope resolution operator(::).
Constructor in C++ Syntax (Defined Outside The Class):
class ClassName {
Access_specifier:
ClassName(); // Default constructor
};
// Constructor definitions (outside class)
ClassName::ClassName() {
// Initialize data members and allocate resources here
}
Here, the change in the syntax can be seen as the addition of a scope resolution operator, i.e., (::), which is used to define the class constructor outside the class.
Code Example:
Output:
Person 1: Name: Unknown, Age: 0
Explanation:
We are using the same example as above, with a Person class, with some changes.
- We define a class called Person, with two private data members, name and age.
- Then, we declare the default constructor inside the class without any definition.
- We also have a class member function displayInfo(), which uses cout to print the object's information.
- Next, we define the default constructor outside the class (as mentioned in the comment) using the scope resolution operator(::).
- This constructor initializes the data member name to 'Unknown' and age to 0 for any new object of the Person class.
- In the main() function, we create an instance of the Person class called person1 default constructor.
- We then call the displayInfo() method on person1 using the dot operator. This prints the output to the console, as given above.
This example shows that even if we define the constructor outside of the class since the declaration was made inside, it can still initialize the object attributes as regular.
Characteristics Of A Constructor In C++
A constructor in C++ plays a critical role in classes and the creation of objects. Here are some of its most important characteristics:
- Class Name Matching: A constructor's name must match the class name in which it is specified. It improves the compiler's ability to recognize and associate the constructor with the class.
- Overloading: We can overload constructors in C++, just like standard functions. This implies that a class may have numerous constructors, each with a unique list of parameters, providing various ways to initialize objects. It provides flexibility in code.
- Default Constructor: In case we do not explicitly define a constructor in C++ classes, the compiler creates a default constructor. The object's attributes are initialized with default values in the default constructor, such as 0 for numbers and null for object references.
- No Return Type: A constructor in C++ doesn't have any return types, not even void. This is because their primary function is to initialize objects instead of returning values.
- Automatic Invocation: When an object is formed, the constructors are automatically called. Unlike an ordinary method, a constructor in C++ does not require an explicit call.
- Initialization: An object's attributes, usually referred to as instance variables, are initialized using constructors. They guarantee that an item is in a usable state as soon as it is created.
- Access Modifiers: Access modifiers for constructors include public, private, and protected. The constructor in C++ can only be invoked from certain places depending on the access modifier selected.
- Inheritance: Unlike ordinary methods, constructors cannot be overwritten or inherited by subclasses. However, as part of their direct initialization procedure, constructors in a subclass normally invoke a constructor of the superclass. That is, the constructor in C++ of a derived class invoked the base constructor.
Types Of Constructors In C++
Constructors in C++ can be divided into a number of types according to their parameters, functions, and characteristics. The three main types of constructors in C++ are:
- Default Constructor In C++
- Parameterized Constructor In C++
- Copy Constructor In C++
We will explore each of these types of constructor in C++ in detail in the sections ahead.
Default Constructor In C++
The default constructor in C++ is a unique constructor that is created and called automatically by the compiler when no other user-defined constructor is explicitly declared within a class.
- The concept of default constructors is used to construct objects with initial default values for its data members and is called without any arguments.
- In other words, it is called for the default initialization of class internal members.
The default constructor in C++ also known as the argument constructor is, hence, crucial when creating objects without specifying any particular values.
Syntax Of Default Constructor In C++:
class ClassName {
Access_specifier:
ClassName(){
}
};
The syntax for the default constructor is the same as the one given in the sections above. However, this syntax is for when the constructor in C++ is defined inside the class.
Code Example:
Output:
Width: 0, Height: 0
Explanation:
- In the example above, we first define a class called Rectangle, containing two private data members, i.e., width and height.
- It also has a default constructor Rectangle(), where we use initializer lists (:) to initialize the data member variables width and height to 0. These lists are more efficient than assigning the values within the constructor body.
- The class also has a member function displayDimensions() that uses the cout command to output the values of width and height to the console.
- In the main() function, we first create an instance of the Rectangle class named rect using the default constructor. The default constructor initializes the width and height of the rect object to 0.
- We then call the displayDimensions() method on the object rect. The method prints the width and height of the rectangle.
Parameterized Constructor In C++
In C++, a parameterized constructor is a particular kind of constructor that accepts one or more parameters as arguments when creating an object. It enables you to supply unique values when initializing an object's data members. Parameterized constructors in C++ are especially helpful when you want to create objects with specific beginning states based on the supplied inputs.
Syntax For Parameterized Contractor In C++:
class ClassName {
Access_specifier:
ClassName(parameter_type parameter1, parameter_type parameter2, ...){
// Constructor body
}
};
Here,
- The class keyword indicates that we are defining a class.
- The className is the name of the class, as well as the parameterized constructor in this case.
- Access_specifier is a data visibility controller that can be public, private, or protected.
- The terms paramater1 and parameter_type represent the name of the parameter and its type.
Code Example:
Output:
Width: 10, Height: 5
Explanation:
The example continues the one above with the Rectangle class, two private data members, i.e., width and height, and the member function displayDimensions().
- But instead of a default constructor, we have a parameterized constructor that takes two integer parameters (w for width and h for height).
- Inside the constructor, the width and height attributes of the object are initialized using the provided parameters.
- In the main() function, we create an instance of the Rectangle class named rect by passing the values
- In the main() function, we create an instance of the Rectangle class named rect by passing values 10 and 5 to the constructor, i.e., Rectangle rect(10, 5).
- We then call the displayDimensions() member function on the rect object to display the width and height of the rectangle.
Copy Constructor In C++
A copy constructor is a special constructor in C++ that makes a copy of an existing object while creating a new one. It is utilized whenever an object declaration is passed as a value to a function, returned by value from a function, or when an object is given the value of another object belonging to the same class. The copy constructor in C++ makes sure that the new object's data members are set to the same values as those of the source object.
Syntax For Copy Constructor In C++:
class ClassName {
Access_specifier:
ClassName( ClassName &source){
// Copy data members from the source object
}
};
Here,
- class is the keyword used for the class definition.
- ClassName is the name of the class in which the copy constructor is defined and also the name of the constructor.
- Access_specifier controls the data visibility of the constructor, which could be public, private, protected
- ClassName &source is a parameter of the copy constructor in C++ that accepts a constant reference to the objects of the same class.
Code Example:
Output:
Name: Shivani, Age: 25
Explanation:
- In this example, we first define a Person class with two private data members, the name of the type string, and the age of the type int.
- The class contains a parameterized constructor that accepts a string n for the name and integer a for the age.
- It also has a copy constructor that takes a reference to another Person object, i.e., const Person &source, as a parameter.
- We also define a member function displayInfo() inside the class to print the name and age of the person using the cout stream.
- In the main() function, we create an instance of Person class named person1 using the parameterized constructor with the values "Shivani" and 25.
- We then create another instance of Person named person2 by copying the contents of person1 using the copy constructor. This happens when Person person2 = person1 is executed.
- Next, we call the displayInfo() method on person2, which prints the information of person2 on the console.
The output shows that the copy constructor in C++ has copied the attributes and values of the data members for person1 object to person2 object.
Dynamic Constructor In C++
A dynamic constructor is a type of constructor in C++ that allocates memory dynamically using the new keyword and pointer variables. This allows objects to be initialized at runtime with memory allocated on the heap.
The new operator in C++ enables dynamic memory allocation, creating object at runtime. However, you must explicitly manage (release) this memory by using the delete keyword to deallocate it when it is no longer required.
Syntax For Dynamic Constructor In C++:
class className {
//Data members
char* p;Access_specifier:
className(){
// Allocating memory at run time
p = new char[6]; }
};~ClassName() {
delete[] p; // Deallocate the memory
}
};
Here,
- class: It is the keyword used to define the class.
- ClassName: It refers to the name of the class as well as the constructor, which here is used to initialize the data member dynamically.
- char* p: The pointer variable that points to the memory location of the data member.
- Access_specifier: This is a data visibility controller (public, private, or protected) that specifies who can access the class members.
- The new keyword is used to allocate memory dynamically inside the constructor.
- The delete keyword inside the destructor ~ClassName() deallocates or releases memory.
Code Example:
Output:
Unstop
Example:
- We define a class named company with a private member p, which is a pointer to a character type. This pointer will hold the dynamically allocated memory for our string.
- Inside the class, we define a default constructor. In this constructor-
- We allocate memory for a character array of size 7 using the new operator. This size includes space for the null terminator(/0) escape sequence at the end of the string.
- After allocating the memory, we use the strcpy() function to copy the string literal "Unstop" into the allocated memory. This ensures that our pointer p points to a valid string stored in the allocated memory.
- We also define a destructor for the class to free the allocated memory using the delete[] operator. This is important to avoid memory leaks by ensuring that any memory allocated with new is properly deallocated when the object is destroyed.
- Next, we define a member function display() that prints the string pointed to by p to the console using cout.
- In the main() function, we create an object of the company class named obj. When this object is instantiated, the constructor is called, allocating memory and copying the string "Unstop" into it.
- We then call the display() method on the obj object to print the string to the console.
- After the main() function completes, the destructor is called to destroy the obj object, freeing the allocated memory.
Benefits Of Using Constructor In C++
Some of the key benefits of using the constructor in C++ are as follows:
- Initialization: A constructor in C++ provides a mechanism to initialize an object's data members when it is created, ensuring the entity starts in a valid and consistent state.
- Customization: Parameterized constructor in C++ allows distinct objects to be initialized with specific values, enabling customization based on the requirements of the program.
- Readability of the Code: Constructors in C++ improve code readability by centralizing object initialization logic with the class specification rather than spreading it throughout the program.
- Default Values: Implicit default constructor in C++ allow object creation with default values for their data members, especially when some values are commonly used.
- Resource Management: When creating an object, constructors in C++ can be used to allocate and control resources (dynamic memory), ensuring that they are properly cleaned up after the object's lifespan.
- Object Initialization: A constructor in C++ offers a simple mechanism to guarantee that an object's setup is completed at creation, preventing uninitialized or inconsistent states.
- Constructor Overloading: Using multiple constructors with different argument lists allows objects to be created and initialized in a variety of ways, adapting to different scenarios.
- Inheritance and Derived Classes: Constructors facilitate inheritance by allowing derived classes to use base class constructors in C++ for initializing base class objects and data members, ensuring proper object creation across the inheritance hierarchy.
- Code Reuse: By using constructors to generate many objects with related properties, unnecessary code can be reduced, and maintainability is improved.
How Does Constructor In C++ Differ From Normal Member Function?
Constructors and member functions both belong to a class but serve different purposes and have distinct characteristics.
Constructors in C++ classes are special member functions used to initialize objects of a class. They are automatically called when an object is created, setting up the initial state of the object. On the other hand, member functions define the operations that can be performed on objects of the class and need to be called explicitly.
The table below highlights the difference between a member function and a constructor in C++.
Aspect |
Constructor |
Member function |
---|---|---|
Purpose |
Initialize object properties/ attributes during creation |
Define operations and behaviors of objects |
Naming |
Same as the class name |
Any valid function name |
Return type |
No return type (not even void) |
Can have any valid return type |
Arguments |
Can accept parameters only for initialization |
Can accept parameters for operation |
Invocation |
Constructor in C++ is automatically invoked during object creation |
Member functions must be called explicitly using the object name |
Overloads |
Can have multiple overloads |
Can have multiple overloads |
Initialization |
Primarily focused on initial state setup |
Perform operations on data members |
Constructor Overloading In C++
Overloading is the technique of constructing numerous functions with the same name but various parameter lists. This is also application on constructor in C++.
Constructor overloading allows you to have multiple constructors in a class, each with a different parameter list. This enables objects to be initialized in various ways based on the provided arguments.
Syntax:
class ClassName {
access_specifier:
// Default constructor
ClassName() {}
// Parameterized constructor with 1 parameter
ClassName(parameter_type parameter1) {}
// Parameterized constructor with 2 parameters
ClassName(parameter_type parameter1, parameter_type parameter2) {}
};
Here,
- The class keyword is used to define the class.
- className is the name of the class in which the constructor is defined, as well as the name of all constructors, i.e., default and parameterized.
- The access_specifier specifies the visibility of the data members and member functions in the class.
- The term parameter refers to the parameter taken by the constructor. And parameter_type refers to their data type.
- className(parameter_type parameter1): It is a parametrized constructor with 1 parameter.
- className(parameter_type parameter1,parameter_type parameter2): It is a parametrized constructor with 2 parameters.
Read the following to know more- Constructor Overloading In C++ Explained With Real Examples
Constructor For Array Of Objects In C++
An array of objects is a collection of objects of the same class type stored in a single array. When an array of objects is created, the constructor for each object in the array is called to initialize each element.
An array of objects is a collectionof objects of the same class type stored in a single array data structure. In other words, it's an array with each entry being a class instance, i.e., an array of class objects.
- The constructor of each object in an array of objects is called to initialize each element of the array.
- This allows you to store and manage multiple objects of the same class in a structured manner.
Here is how a constructor in C++ work with an array of objects:
- The first step is to declare an array of items. This only allocates memory for the array it hasn't called the constructor.
- The second step is constructor invocation, i.e., the constructor of each object is automatically called as you create them in the array. This occurs if you initialize the array's objects using the array declaration.
Syntax:
class ClassName {
Access_specifier:
ClassName(data_type parameter1, data_type parameter2) {
// body of the Constructor
}
};int main() {
ClassName objectsArray[size] = {
ClassName(param1_value, param2_value), // Object 1
ClassName(param1_value, param2_value), // Object 2
};
//Additional code, if any
return 0};
Here,
- The class keyword indicates that a class is being defined.
- ClassName is the name of the class as well as the constructor.
- The access_specifier is a data visibility controller that can be public, private, or protected.
- The term parameter1 specifies the name of the parameter, and data_type specifies the type of parameter.
- param1_value: It denotes the value of the parameter.
Code Example:
Output:
Name: Unknown, Age: 0
Name: Alice, Age: 20
Name: Bob, Age: 22
Name: Charlie, Age: 21
Explanation:
In the C++ code example-
- We first define a class named Student with two attributes/ private data members- name and age.
- The class has a default constructor that initializes attributes to default values and a parameterized constructor that takes values for name and age.
- Inside the main() function, we declare an array of Strudent objects named students with a size of 3. This allocates memory for three Student objects but doesn't initialize them yet.
- We then call the display() function on the first element of the array of objects, i.e., display().students[0].
- As a result, the default constructor is called for each element in the students array initializing name with value "Unknown" and age with value 0.
- The values for the first object are printed to the console.
- We then create three individual Student objects (s1, s2, and s3) using the parameterized constructor. These objects have specific values for name and age:
- The first object has the name Aditi and age 20, and the second object has the name Josh and age 22.
- The third object has name Deepak and age 21.
- After that, we declare another array named studentsWithValues of Student objects initialized using parameterized objects (s1, s2, and s3). This creates a new array with objects that have specific values.
- We then call the display() method on individual objects to show their attributes.
- This is done using a for loop that begins with the control variable I set to zero and continues till I <3. After every iteration, the value of i is incremented by 1.
The array students shows the default values, while the array studentsWithValues displays the initialized values. The output demonstrates the usage of constructors and arrays of objects.
Constructor In C++ With Default Arguments
A constructor with default arguments allows you to provide default values for some or all of its parameters. This means that you can skip some arguments from the constructor when creating an object, and the constructor will still use the default values (garbage values) for those arguments. This gives you freedom when creating objects and lets you avoid explicitly giving values for parameters that frequently have the same value.
Syntax:
class ClassName {
Access_specifier:
// Constructor with default arguments
ClassName(data_type parameter1 = default_value1, type parameter2 = default_value2, ...);
};
Here,
- The class keyword defines the class whose name is given by className, which is the same as the name of its constructor.
- The access_specifier reflects the visibility of the class members.
- The paramter1, data_type, and default_value1 refer to the name of the parameters, its type, and its default value, respectively.
Code Example:
Output:
Width: 0, Height: 0
Width: 5, Height: 0
Width: 3, Height: 8
Explanation:
- Here, we first define a Rectangle class with a constructor that has default arguments for both width and height, i.e., (int w = 0, int h = 0).
- This constructor allows object creation with default values (here, 0) for width and height if no arguments are provided during object creation.
- In the main() function, we create three Rectangle objects as follows:
- Object rect1 is created using the default constructor, resulting in both width and height being set to 0.
- Object rect2 is created with a width of 5 and the default height of 0,
- Object rect3 is created with a width of 3 and a height of 8.
- For each object, we then call the displayDimensions() method to display the width and height of the respective rectangles.
Initializer List For Constructor In C++
The constructor initializer list in C++ provides a way to initialize class members directly as part of the object construction process. It is especially useful for initializing constant members or when dealing with non-default constructor calls.
- The data members are initialized directly with the values provided in the initializer list.
- Members are initialized in the order they are declared in the class, not the order in the initializer list. This order is crucial, especially if one member depends on another.
- The constructor body executes after the members have been initialized by the initializer list.
Syntax:
ClassName::ClassName(parameters) : member1(value1), member2(value2), ... {
// Constructor body
}
Here,
- ClassName: It is the name of the class.
- Parameters: It denotes the arguments taken by the constructor.
- member1: It specifies the name of the class member.
- value1: It is a value that needs to be assigned to member1.
We have used this approach to define constructor in C++ in many examples above.
Dynamic Initialization Using Constructor In C++
Dynamic initialization involves setting up objects or variables at runtime based on user input or calculations. This often includes allocating memory dynamically within the constructor. Let's look at the syntax for dynamic initialization inside a constructor in C++ followed by an example.
Syntax:
class ClassName {
Access_specifier:
// Constructor for dynamic initialization
ClassName(data_type parameter1, data_type parameter2, ...) {
// Allocate memory or perform dynamic initialization
// Initialize data members using dynamic values
}// Destructor to release any dynamically allocated resources
~ClassName() {
// Deallocate memory or perform cleanup
}
};
Here,
- The class keyword indicates that we are defining a class.
- The className is the name of the constructor and the class.
- Access_specifier is a data visibility controller which could be public, private, or protected.
- The className with a tilde sign (~) indicates that it is a destructor used to deallocate the memory.
- The parameter1 and data_type refer to the name of the parameter and its type.
Code Example:
Output:
0 2 4 6 8
Explanation:
- The DynamicArray class is defined in the example above with a constructor that takes an int size as a parameter, indicating the size of the dynamically allocated array.
- Inside the constructor, memory is dynamically allocated for the data array using the new operator.
- The array elements are initialized based on their indices, with each element set to twice its index value.
- The class also has a displayData() method, which displays the elements of the initialized array using the cout command.
- Additionally, the class includes a destructor that deallocates the memory allocated for the data array using the delete[] operator to prevent memory leaks.
- In the main() function, we create an object of the DynamicArray class called arr, whose elements are dynamically initialized based on the constructor parameter.
- We then use the object to call the diplayData() function, which prints the array elements to the console.
- After the program finishes using the object, the destructor is automatically called to free the dynamically allocated memory.
Conclusion
A constructor in C++ is a fundamental building block that facilitates the creation and initialization of objects. They ensure that objects start in a consistent state and play a crucial role in achieving encapsulation, flexibility, and code readability. There are three primary types of constructors in C++, i.e.-
- Default Constructor: A default constructor in C++ is automatically generated if no constructors are defined. It initializes class members to their default values.
- Parameterized Constructors: Parameterized constructors accept arguments to customize object initialization.
- Copy Constructor: A copy constructor in C++ creates a new object as a copy of an existing object. It's used for object initialization and passing by value.
Understanding constructors in C+ is essential for writing efficient and maintainable code, and mastering their usage is key to becoming proficient in object-oriented programming with C++.
Frequently Asked Questions
Q. What is constructor overloading?
The technique of declaring multiple constructors within a class, each with a different argument list, is known as constructor overloading. This makes it possible to build and initialize objects in a variety of ways depending on the arguments given during object creation. The flexibility of object initialization is improved via constructor overloading.
Code Example:
Explanation:
In this example, we define a Calculator class with private attributes length, breadth, and height.
- It has two constructor methods to initialize these attributes, one with two parameters and another with three parameters. (These are overloaded constructors)
- In the main() function, two Calculator objects (calc1 and calc2) are created using different constructors.
- Since no operations are performed on these objects, the program doesn't produce any visible output.
Q. What are the 4 main types of constructors in C++?
Constructors are special methods that help initialize the objects of the respective class. There are four main types of constructors in C++, including:
- Default Constructor: A default constructor in C++ is automatically generated by the compiler if no constructors are defined explicitly in the class. It does not take arguments and initializes class members to their default values. If a custom constructor is defined, the default constructor must be explicitly defined if needed.
Example: ClassName() {} - Parameterized Constructor: A parameterized constructor in C++ takes one or more arguments to initialize class members with specific values during object creation.
Example: ClassName(type param1, type param2) {} - Copy Constructor: A copy constructor in C++ creates a new object as a copy of an existing object. It's used when an object is initialized using another object, passed by value to constructor functions, or returned by value from functions. The implicit copy constructor ensures proper initialization and avoids shallow copies of dynamically allocated memory.
Example: ClassName(const ClassName& other) {} - Constructor with Default Arguments: A constructor with default arguments allows you to provide default values for some or all data members. It provides flexibility in object creation and is especially useful when you want to create objects with common property values.
Example: ClassName(type param1 = default_val1, type param2 = default_val2) {}
Q. How can a constructor call another constructor?
Chaining of constructor in C++ is a feature that allows one constructor to call another constructor of the same class. As a result, you can reuse the same initialization logic in other constructors of the same class. The constructor initializer list is used to implement constructor chaining.
Code Example:
Output:
Constructor with parameters
Number 1: 10, Number 2: 20
Constructor with parameters
chaining constructor
Number 1: 0, Number 2: 0
Explanation:
- The code includes the <iostream> library for input/output operations and uses the namespace std; directive to avoid the need for std:: prefixes.
- The Example class has two constructors, one with parameters and another without.
- The parameterized constructor initializes num1 and num2.
- The chaining constructor calls the parameterized constructor using the initializer list.
- When the obj2 object is created using the default constructor, the delegating constructor is called, which in turn calls the parameterized constructor.
- The output demonstrates the constructor chaining and the order in which constructors are called.
Q. Is it mandatory to use a constructor in a class in C++?
No, it is not mandatory to use a constructor in C++. The compiler will create a default constructor for you if you don't define any constructors in your class.
- Each data member of the class is initialized using the implicit default constructor in C++.
- However, you must specify your own constructors if you want to carry out special initialization.
- You can manage how the objects in your class are initialized and increase the flexibility of your code by defining a custom constructor in C++.
Q. Can constructors throw exceptions?
Yes, a constructor in C++ can throw exceptions if it encounters an error that prevents it from properly initializing an object. It can throw an exception to signal that the construction process has failed.
In other words, when a constructor throws an exception, the object being created is considered not to have been successfully constructed. As a result, the object's destructor will not be called because the object was never fully constructed. However, the destructors of any fully constructed base or member subobjects will be called to clean up any resources allocated before the exception is thrown.
Q. Can a constructor in C++ be private?
Yes, a constructor in C++ can be declared as private. However, this will restrict direct instantiation from outside the class. A private constructor is declared private within a class template. By doing this, the constructor of the class cannot be used to instantiate it directly.
- Encapsulation is improved, and greater control over class instances is maintained with the use of private constructors.
- By making the constructor private, you can also enforce certain rules or patterns for creating default member initializers and objects of that class.
Q. What is the difference between a constructor and a destructor?
Here are some of the differences between a constructor and a destructor in C++:
Aspect |
Constructor |
destructor |
Purpose |
Initializes object's state |
Cleans up object's resources |
Syntax |
ClassName(Same as the class name) |
~ClassName (preceded by tilde) |
Invocation |
Automatically, at the time of object creation |
Automatically on object destruction |
Default Behavior |
The compiler generates a default if absent |
The compiler generates a default if absent |
Memory Management |
Allocates memory, initializes members |
Deallocates memory and releases resources |
Number |
Multiple constructors allowed |
Only one destructor per class is allowed |
Q. What is the purpose of a constructor in programming?
These are some of the uses of constructors in programming language:
- Effective Object Initialization: Constructors are used to set up the initial state of an object when it is created.
- Customization: Constructors can be parameterized to allow different ways of creating objects, allowing customization based on specific needs.
- Attribute Initialization: Constructors assign initial values to the object's attributes, ensuring they have valid and meaningful values from the start.
- Data Consistency: Constructors help to prevent uninitialized or inconsistent data within an object
- Immediate Usability: By initializing necessary properties, explicit constructors ensure that objects are ready to be used as soon as they are instantiated.
This compiles the discussion on the constructor in C++. You might also be interested in reading the following:
- C++ Templates | Class, Function, & Specialization (With Examples)
- C++ If-Else & Other Decision-Making Statements (+Examples)
- 2D Vector In C++ | Declare, Initialize & Operations (+ Examples)
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- Bitwise Operators In C++ Explained In Detail With Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment