Classes & Objects In C++ | A Detailed Explanation (With Examples)
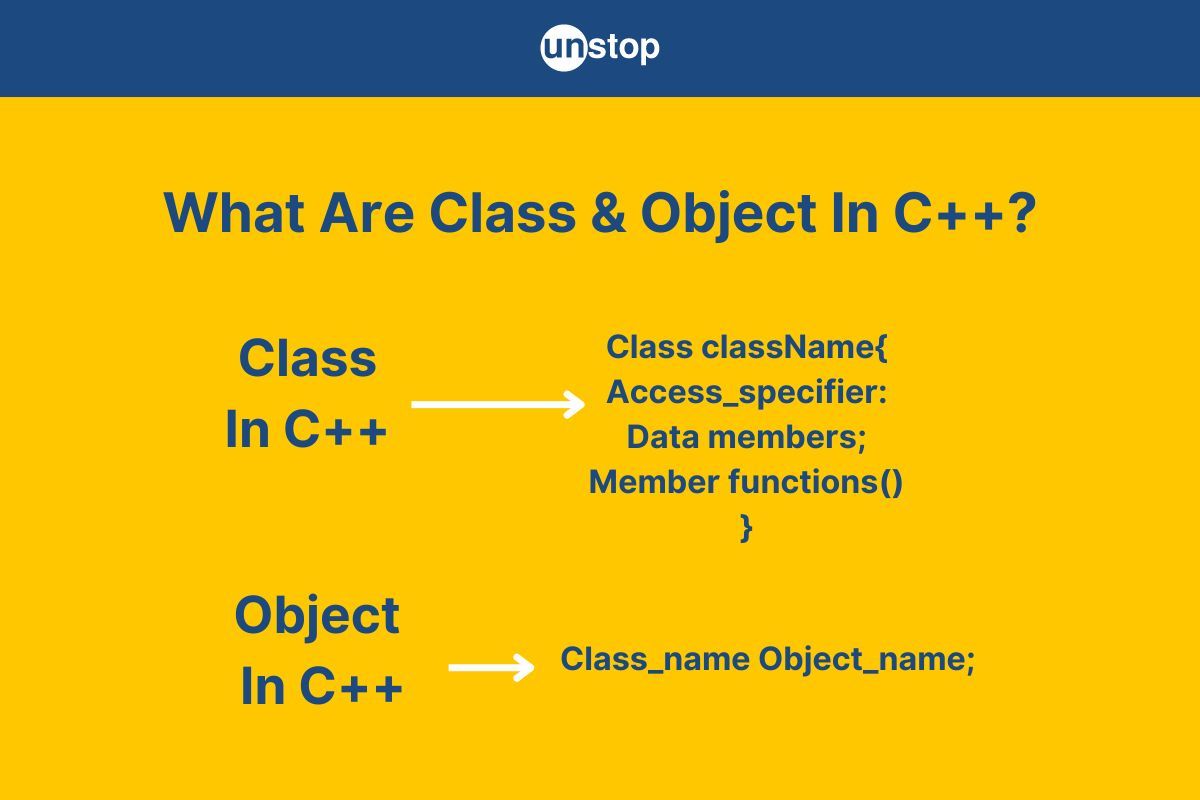
Classes and objects are integral components for the implementation of object-oriented programming language concepts. They help implement OOP features like inheritance, polymorphism, encapsulation, etc. A class in C++ (and other object-oriented languages) encapsulates objects (instances) and methods that help manipulate the data in these objects.
In other words, it is a blueprint for creating and manipulating objects. An object in C++, on the other hand, is an instance of that class. In this article, we will discuss the concept of classes and objects in C++ and their other components.
What Is A Class And Object In C++?
A class in C++ is a fundamental OOPs concept that enables you to create user-defined data types. It acts as a blueprint or template to specify how objects belonging to the class should be constructed and behave.
- A class encapsulates data members (attributes or properties) and member functions (methods or operations) that manipulate the data.
- In other words, a class in C++ acts like a template for building objects, where objects are instances.
- Each object has a unique set of data members and can be manipulated with the help of the class functions (i.e., class methods/ member functions).
Real-Life Example Of Class & Object In C++
Consider the category Car as a class in C++ programming. This class can define characteristics such as brand, model, year, and color, and functions such as ignition, acceleration, and braking.
- Each individual car, with its specific brand, model, and year, represents an object of the Car class.
- Thus, Car is a blueprint from which various car objects are created, each having its own unique set of characteristics and behaviors (functions).
Similarly, in the animal kingdom, consider Dog as a class. This class would encapsulate common characteristics such as having a tail, barking, and sniffing, and behaviors typical of dogs.
- Rather than creating a separate class for every breed of dog (e.g., Labrador, Beagle, Bulldog), we use a single Dog class to represent the common features and functionalities.
- Each specific breed would then be an object of the Dog class, inheriting the general characteristics while potentially having additional specific attributes or methods.
In summary, classes in C++ language act as blueprints or templates for creating objects with similar features and behaviors, allowing for efficient and organized code management.
Syntax To Define A Class In C++
Class className{
access_specifier:
//Data_members;
//Member_functions()
}
Here,
- The keyword class is used to define a class in C++, where the class identifier is given by className.
- The access_specifier is the access controller which defines the visibility of class members. It can be private, public, and protected; we will elaborate on these in a later section.
- Data_members are the variables that hold data related to an object of the class.
- Member_functions() are class methods that define what operations can be performed on objects and how.
What Is An Object In C++?
An object in C++ is a self-contained element that contains data and behavior. It is an instance of a class that combines both data members and the class methods/ functions that operate on that data into a single unit.
- The class functions, often referred to as methods, will allow the object to perform actions and manipulate its data.
- This bundling of data and methods within an object and class in C++ programming supports code reuse and simpler maintenance, especially in large software systems.
Let's look at the syntax below to know how to create an object in C++, followed by a detailed explanation of the process.
Syntax Of Object In C++
Class_name Object_name;
Here,
- Class_name: It is the name of the class for which the object is created
- Object_name: It is the name of the object. Note that having a meaningful object name enhances code readability
As shown in the syntax, when declaring/ creating an object of C++ class, you must specify the class name followed by the name you want to give to the object. Ideally, both these names should be descriptive.
How To Create A Class & Object In C++? With Example
The first step in the process of creating a class and object in C++ is to define the class itself. This is usually done at the beginning of the program, i.e., before the main() function. Then, you can declare an object of the C++ class inside the main function to perform modifications/ carry out manipulations.
The process of creating class & object in C++ is as follows:
- Declare and Define a Class in C++ Program: The first step is to create a class, as mentioned in the syntax given above. The C++ class definition must specify-
- Data members, i.e., the attributes or member variables of objects in C++.
- Member functions, i.e., C++ class methods that define how we can manipulate the data stored in the member variables to full required tasks.
- A C++ class definition also includes two more elements or special functions, i.e., the constructor and destructor.
- A constructor helps in the creation of the class object, and a destructor helps destroy the C++ class objects once they are no longer needed.
- Create an Object in C++ Program: After a class has been defined, an object of that class can be declared by writing the class name and then the object name. The memory for the object is assigned after this declaration.
- The creation of a C++ class object is usually done in the main() part of the program.
- Once declared and initialized, the C++ class object can be used to invoke special functions in the class.
Example Of C++ Class & Object Creation
Given below is an object and class in C++ example illustrating how to create and use them in practice.
Code Example:
Output:
Area of the rectangle: 15
Explanation:
We begin the C++ program example by including the <bits/stdc++.h> header file (which encompasses all libraries) and use the namespace std.
- Then, we define a class named Rectangle, which represents the characteristics of a rectangle.
- The class contains has two private data members (class attributes), i.e., length and width, both of which are of double data type.
- We also declare a class constructor inside the Rectangle class to initialize the data members when an object is created.
- Then, we define a public member function named calculateArea(), which calculates the area of the rectangle using the multiplication operator and returns the same.
- In the main() function, we create an object of type class Rectangle, called rect, with values 3 and 5, as mentioned in the code comments.
- As you can see, we use the class name followed by the object name for declaration and put the values of data members in the brackets.
- Next, we call the calculateArea() function, passing the rect object as an argument using the dot operator.
- This function call invokes the member function to calculate the area of the rectangle which we store in the area variable.
- Then, we use the cout statement to print the value of the area along with a string message describing the same.
- Finally, the main() function terminates with a return 0 statement.
Access Modifiers & Class/ Object In C++
Access specifiers, also known as access modifiers, are keywords used in C++ to manage the visibility and accessibility of class members, including data members and member functions, to users outside the class. They are essential to the implementation of inheritance and encapsulation. There are three main access specifiers used with a class in C++:
- Public: C++ class members declared with public access specifier are accessible from any part of the program. They form the class's public interface, allowing the functions and code outside the class to interact with them. For example, an object in C++ class (say publicData) and member function (say publicFunction) with the public modifier can be accessed directly from objects of the class.
- Private: The C++ class members and functions declared as private are accessible only within the class itself. That is, functions or objects outside the class in C++ cannot access them (even derived classes). This is used to protect sensitive data and methods so that only the class's methods may directly edit or access them. For example, a C++ object and method declared as private (say privateData and privateFunction) can only be accessed by methods within the class.
- Protected: Members declared as protected are inaccessible from outside the class, just like private members. However, derived classes that inherit from the base class can access them. This provides a way to allow derived classes to use or modify the base class's data while preserving encapsulation. For example, function and object in C++ parent class (say protectedData and protectedFunction) can be accessed within its derived classes.
Modifiers |
Base/ Own Class |
Derived Class |
Main() Function |
public |
Yes |
Yes |
Yes |
protected |
Yes |
Yes |
No |
private |
Yes |
No |
No |
Let's take a look at an example to get a better understanding of access attributes of class in C++.
Code Example:
Output:
This is a public member function.
This is a public member function.
This is a protected member function.
Explanation:
In the example above-
- We first define a class called MyClass, which has a data member and a member function defined under all three access modifiers.
- Public members: We have public data member publicData and member function publicFunction, which prints a string message stating the same. They are accessible from anywhere, including outside the class, such as in the main() function.
- Private Members: We have private data member and member function called privateData and privateFunction(), respectively. They are only accessible within MyClass.
- Protected Members: We have protected data member and member function called protectedData and protectedFunction(), respectively. They are accessible within MyClass and derived classes like Derived, but not from outside the class hierarchy.
- Next, we define a derived class called Derived which inherits from MyClass using the keyword public. This means public and protected members of MyClass are accessible in Derived, but private members are not.
- In the main() function, we create an object obj of MyClass. The public members of MyClass, like publicData and publicFunction(), can be accessed using obj.
- However, the private and protected members like privateData, privateFunction(), protectedData, and protectedFunction() are not accessible from outside the class.
- Next, we create an object derivedObj of the Derived class.
- We then use the object of class Derived with the dot syntax/ operator to call accessBaseMembers(), the member function of the derived class.
This example demonstrates that only public members of MyClass can be accessed directly. Derived class objects can access public and protected members through member functions of the derived class.
Member Functions Of A Class In C++
A function that is defined inside a class in C++ is referred to as its member function. In object-oriented programming (OOP), they are also known as methods.
- Member functions have access to the class members' data and describe the behavior or operations that objects of the class are capable of performing.
- This means we can use an object in that C++ class to call these member functions.
There are two ways to define these member functions in relation to a class in C++. Here is a more detailed explanation of member functions and how they relate to classes.
Defining Member Functions Inside Class In C++
When a member function is declared inside a class in C++, it is implicitly treated as an inline function, which means that the function definition is given right when the function is declared. It describes the specific behavior of the object in C++ class and performs action by accessing the data members/ class attributes for the respective object.
Syntax:
Class class_name{
return_type member_function(){
// body of the function definition
}
}
Here,
- Class: It is a keyword used to define a class.
- class_name: It is the name of the class.
- return_type: It is the data type of the member function’s return value
- member_function: It is the name of the member function defined inside the class.
Code Example:
Output:
Value: 42
Explanation:
- In this example, we define a class named MyClass with a private integer data member called data and two public member functions, setData() and getData().
- The setData() function initializes the data variable with the argument value, and the getData() function retrieves the value.
- In the main() function, we create an object obj of type MyClass.
- We then call the setData() function on the obj object, passing 42 as an argument. This initializes the data member of the obj object to 42.
- Next, we call the getData() function using the same obj object and assign the retrieved data to a variable value.
- Finally, we use the cout statement to print the integer number stored in the variable value.
Note: Both setData() and getData() are member functions defined inside the MyClass class. Being member functions of the class in C++ program, they have direct access to the private data member data, allowing them to manipulate and retrieve its value.
The member functions setData() and getData() are implicitly declared as inline functions and defined inside the class in C++ example.
Defining Member Functions Outside Class In C++
A class's member functions can be declared inside the class without definition. Then, we can give the definition of the function outside of the class in C++ using the scope resolution operator (::) and the class name to indicate the class to which they belong.
- We usually define member functions outside of a class in C++ to effectively organize and separate the class interface from its implementation.
- This is especially done when handling bigger and more complicated functions.
- The syntax given below shows the difference between the definition of member function inside and outside the class in C++.
Syntax:
Class class_name{
return_type member_function() // function declaration
}
return_type class_name::member_function(){
//body of the function definition
}
Here,
- Class and class_name refer to the keyword to declare a class and its name, respectively.
- The terms member_ function and return_type refer to the function's name and the data type of its return value, respectively.
- The double colon (::) is the scope resolution operation used to define a member function outside the class in C++.
Code Example:
Output:
Value: 42
Explanation:
This example is similar to the one above, with the same class, MyClass, and the same data members and member functions.
- However, here, we have merely declared the member functions with their signatures inside the class. We have then given the actual function definition outside the class using the scope resolution operator.
- In the main() function, we created an object obj of type MyClass, which behaves as an instance of the class and can call the member functions.
- Just like before, we call the member functions setData() and getData() on the obj object to set and retrieve the value of the data member. Finally, the value is printed on the console.
Also read: Member Function In C++ | Definition, Declaration, Types & More
How To Access Data Members And Member Functions?
The first step to access data members and member functions of a class in C++, you need to create an object of that class. You can then use this object in C++ class with the dot operator to access specific data members.
- We can access the data members and member functions using objects of that class in C++.
- This is possible because each object of the class behaves independently, allowing the storage and manipulation of different data.
- The same class blueprint can be used to initialize multiple objects in C++, each of which can preserve its own set of data.
Example: Object and Class in C++ Programming
As we discussed above, we can use object in C++ class to access the data members. For this, we must use the object name followed by the dot operator and the name of the data member. Let's look at an example of the same.
Code Example:
Output:
Name: Anahita, Age: 20, Roll Number: 12345
Name: Arav, Age: 23, Roll Number: 0
Explanation:
In the example C++ program-
- We define a class named Student to represent student information.
- The C++ class contains three public data members: name, age, and rollNumber to store the student's details.
- We also define a public member function called displayInfo() to display student information in reference to a specific instance/ object in C++ class, using cout.
- In the main() function, we create an object student1 of the Student class. The object represents a specific instance of a student with its own set of data members and member functions.
- We then access the data members of this object of the Student class and assign them values using the dot operator (.).
- Similarly, we create another object of class Student, called student2 and initialize two of its data members (name and age).
- Next, we call the displayInfo() member function using both the objects student1 and student2.
- When the function is executed in the context of the student1 object, and it displays the student's information (name, age, and roll number) on the console.
- It does the same for the student2 object; however, since we did not initialize the last data member, it prints the garbage value 0. This is given by the constructor of the C++ class, which we will discuss in a later section.
As seen in the example, multiple objects can be created from the same class blueprint, each maintaining its own set of data and enabling the representation of different students with their unique details.
Significance Of Class & Object In C++
We already know what is class and object in C++. However, it is important to note that classes and objects are fundamental ideas that are necessary for object-oriented programming (OOP). In other words, the importance of classes and objects in C++ lies in the foundation that they offer for object-oriented programming (OOP).
- Data Hiding: In object-oriented programming (OOP), data hiding, also known as information hiding, involves encapsulating the internal implementation details of a class and exposing only the necessary interfaces to interact with objects in C++ classes.
- Data Binding: The process of associating data (properties or attributes) with their corresponding behavior (methods or functions) within a class in C++ is known as data binding. It creates a connection or link between data and the possible operations on that data.
- Code Reusability: Classes encourage reusable code. A single class declaration can be used to produce many objects in C++ programs, allowing you to reuse the same code structure while using different data values. It also makes code maintenance easier and avoids code duplication and code redundancy.
- Flexibility: The concept of classes and objects in C++ offers a scalable architecture for the construction of complicated systems. You can add new classes or enlarge existing ones as the program's requirements increase without changing the structure of the C++ program.
- Inheritance: Inheritance is a mechanism that allows you to create a new C++ class based on an existing class. The new class inherits the properties (data and methods) of the existing class, and you can extend or modify its behavior as needed. This promotes code reuse and hierarchical modelling.
- Polymorphism: Polymorphism enables you to treat objects of different classes in a uniform way. This is achieved through inheritance and virtual functions. Polymorphism allows you to write more generic and flexible code that can work with different types of objects in C++ without knowing their specific implementations.
- Encapsulation and Access Control: Classes provide access control modifiers like public, private, and protected, which determine the visibility of members (attributes and methods) from outside the class in C++. This encapsulation prevents unauthorized access and modification of internal data, thus enhancing data security and integrity.
- Constructor and Destructor: Constructors are special member functions that initialize objects in C++ classes when they are created. Destructors are used to clean up resources and perform necessary actions when the objects are destroyed. These functions play a crucial role in managing the lifecycle of an object in C++.
- Dynamic Memory Management: Objects in C++ can be created dynamically using the new keyword, allowing you to allocate memory for objects at runtime. This can be useful when dealing with objects whose lifetimes can't be determined at compile time.
- Class Templates: C++ supports the concept of class templates, which allows you to create generic classes that can work with multiple data types. This increases code flexibility and reusability.
What Are Constructors In C++ & Its Types?
Constructors are unique member functions that are called automatically when an object of a class is created in C++.
- They are used to set up the C++ object's requirements and initialize its data members.
- Constructors are defined without a return type and have the same name as the class.
- There are multiple types of constructors, all of which we will discuss in this section.
Default Constructor Of Class In C++
A constructor that is automatically created by a compiler is known as a default constructor of a class in C++. That is, if a class doesn't have any defined constructors, the compiler will create a default one.
- Its goal is to initialize the object with default values, such as setting pointer variables to null or numerical data members to zero.
- For built-in data types (like int, double, pointers), if you don't explicitly initialize them in the constructor, they can hold garbage values.
- By definition, a default constructor does not accept parameters. In other words, it is a constructor that can be called with no arguments.
Given below is the syntax for the default constructor of a class in C++.
Syntax:
class MyClass {
public:
// Default constructor (automatically generated if not defined)
MyClass() {
// Initialize data members here
}
};
Here,
- Myclass is the name of the class as well as the default constructor, which should be the same.
- Public is the access controller of data inside the MyClass class.
Parameterized Constructor Of Class In C++
A constructor that accepts one or more parameters as arguments is known as a parameterized constructor. It enables you to provide particular values to initialize the object when it is created.
Syntax:
class MyClass {
public:
// Parameterized constructor
MyClass(arguments) {
// Initialize data members using the provided arguments
}
};
Here,
- Myclass is the name of the class as well as the parameterized constructor.
- Public is the public access controller of data inside the class.
- The arguments refer to the parameters that the constructor takes. They are used to initialize the data members of the class/ object of the C++ class.
Copy Constructors Of Class In C++
The copy constructor creates a new object by copying the data of an existing object of the same class. It is called in several situations, such as when an object is passed by value, returned by value, or explicitly copied.
Syntax:
class MyClass {
public:
// Copy constructor
MyClass(const MyClass& other) {
// Perform a deep copy of data members from 'other' to this object
}
};
Here,
- Myclass is the name of both the class and the copy constructor.
- Public is the access specifier that makes the copy constructor accessible from outside the class.
- The parameter const MyClass& other is a reference to another object of the same class in C++. The const keyword ensures that the original object is not modified.
Example Of Default, Parameterized, Copy Constructor For Objects In C++
Output:
Default constructor called.
Parameterized constructor called.
Copy constructor called.
Name: Unknown, Age: 0
Name: Apurva, Age: 30
Name: Apurva, Age: 30
Explanation:
In the example program above-
- We first define a class called Person, with two private data members, i.e., the name and age of a person.
- The class has a default constructor, which is automatically called when an object is created without any arguments. It sets the name to Unknown and the age to 0 for objects created using the default constructor.
- We also define a parameterized constructor, which is called when objects are created with specific values for the name and age. It allows us to provide custom values during object creation.
- Then, we define a copy constructor, which is used when a new object is created as a copy of an existing object. It initializes the new object with the same name and age as the existing object.
- The copy constructor is invoked during object creation, assignment, or when passing an object by value.
- In the main() function, we create three objects of the Person class using different constructors. Here,
- The person1 is created using the default constructor.
- The person2 is created using the parameterized constructor with the values "Apurva" and 30.
- The person3 is created using the copy constructor initialized as a copy of person2.
- Next, we call the member function displayInfo() for each object to print the information (name and age) of each person, i.e., 3 times. The function is declared as const to indicate that it does not modify the object's state.
When the program is executed, it displays messages showing which constructors are called for each object creation. The information confirms that the objects are successfully created and initialized based on the respective constructors. The information for each person is then displayed using the displayInfo() function.
For more, read: Constructor In C++ | Types, Benefits, Uses & More (With Examples)
What Is A Destructor Of Class In C++?
A destructor is a special member function that is automatically called when an object goes out of class scope in C++ or is explicitly deleted.
- It is used to carry out cleanup tasks and deallocate resources that were obtained throughout the lifetime of the object in C++ classes.
- The class name is used for destructors, distinguished by the tilde (~) symbol.
A destructor's main function is to free up any resources that the C++ object owns, including dynamically allocated memory, file handles, network connections, or other resources that require proper cleanup.
Syntax:
class ClassName {
public:
// Destructor
~ClassName() {
// Cleanup and deallocation of resources
}
};
Here,
- The ClassName is the name for both the destructor and the class, which must be the same.
- The tilde symbol (~) is used to specify the destructor.
For more information including example, read: Destructor In C++ | Understanding The Key To Cleanups (+ Examples)
Note that both constructors and destructors play a critical role when working with classes and objects in C++. They ensure proper initialization and cleanup of resources, thus maintaining the integrity and efficiency of the program.
An Array Of Objects In C++
An array is a collection of contiguous memory that can store both primitive data types and user-defined data types. It is one of the most commonly used data structures. In C++, we can declare an array of any data type, including classes.
When combining the concept of array with class in C++, we can create an array of objects. The refers to an array of elements where each element is of class type (in other words, an object of C++ class).
Syntax:
class _name array_name[size];
Here,
- class_name is the name of the class
- size is the number of objects we need to store.
- array_name is the name of the array
Code Example:
Output:
Constructor: Book "Book1" created.
Constructor: Book "Book2" created.
Constructor: Book "Book3" created.
Book Title: Book1
Book Title: Book2
Book Title: Book3
Destructor: Book "Book3" destroyed.
Destructor: Book "Book2" destroyed.
Destructor: Book "Book1" destroyed.
Explanation:
In the sample C++ program-
- We first define a class called Book with one private data member, i.e., title of string data type.
- The class includes a constructor and a destructor for initialization and cleanup tasks.
- It also has a member function to display the book title called displayTitle().
- In the main() function, we create an array of Book objects named books of a size of 3. (Creation of the array of objects)
- The constructor is called for each Book object, initialising them with different titles, i.e., Book1, Book2, and Book3.
- Next, we access the elements of the array of objects. For this, we create a for loop to access each book in the array and call the displayTitle() member function to print the title of each book using cout.
- When the program is executed, the constructors for each Book object in the array are called, printing messages indicating the creation of each book.
- The titles of all three books are then displayed using the displayTitle() member function.
- Finally, when the main() function ends, the Book objects in the books array go out of scope, and their destructors are called automatically, printing messages indicating the destruction of each book object.
Object In C++ As Function Arguments
Class objects in C++ can be passed as arguments in the same manner that variables can be passed to functions.
- A member function, a friend function, or a non-member function can all accept an object as an argument.
- The member function and the friend function have access to the object's private and public members.
- However, the non-member function can only access the object's public members.
There are two ways in which we can pass an object in C++ as an argument to a function (just like regular variables) which we will discuss in this section.
Passing Object In C++ By Value
Passing a class object in C++ by value means that a copy of the entire object is made and passed to the function as an argument.
- This means that the function receives its own independent copy of the original object.
- As a result, any modifications made to the copy inside the function will not affect the original object of C++ class.
- However, passing an object by value can be inefficient due to the time consumed in generating a copy of the object.
Let's look at a sample C++ program illustrating this approach of passing object as function arguments.
Code Example:
Output:
Passing by Value:
Book Title: Original Book Title
Book Title: Modified Book Title (Passed by Value)
Book Title: Original Book Title
Explanation:
- In this example, we define a Book class with a constructor, a displayTitle() member function, and a setTitle() member function.
- We then create a function named modifyBookTitleByValue, which takes the object of the Book class as argument by value, creating a copy.
- In the main() function, we create a Book object book1 with the title data member initialized with "Original Book Title".
- We then pass the object book1 by value as an argument and call the modifyBookTitleByValue() function.
- Inside the function, a copy of book1 is created, and modifications are made to this copy.
- A message indicating the modification is printed on the console. Note that the original book1 object remains unchanged.
- After that, we once again call displayTitle() function on book1 object. The output shows the original object was not affected by the modification made since it was passed by value, which creates a copy.
Alternatively, passing by reference allows direct modification of the original object in C++ classes, which we will discuss in the following section.
Passing Object In C++ By Reference
Passing class objects in C++ (as an argument) by reference means that a reference or alias to the original object is passed to the function.
- In other words, when you pass an object by reference, you are not making a replica of the full object; instead, you are passing a reference or alias to the original object.
- This allows the function to use the original object's data without making a separate copy and to access and alter it directly.
Passing by reference is more efficient than passing by value. Let's look at an example of the same.
Code Example:
Output:
Passing by Reference:
Book Title: Original Book Title
Book Title: Modified Book Title (Passed by Reference)
Explanation:
- Just like in the previous example, we define a Book class with a constructor, a displayTitle() member function, and a setTitle() member function.
- We then create a function named modifyBookTitleByReference. The function takes a Book object by reference using the ampersand/ reference operator (&).
- In the main() function, we create an object of the Book class, named book1, with the title "Original Book Title".
- We then call the modifyBookTitleByReference() function by passing the object book1 to it by reference.
- Inside the function, the original book1 object is modified directly using the setTitle() member function.
- Finally, we call the displayTitle() function on object book1. The output shows the modified title for book1 object.
Note: Comparing the outputs of the two programs shows that passing by value creates a copy that does not affect the original object, while passing by reference allows direct modification of the original object in C++.
The this (->) Pointer & Classes In C++
The this pointer (->) is a special pointer available within non-static member functions of a class in C++. That is, a class's non-static member functions automatically have access to the this pointer, which is implicitly declared by the compiler. It points to the current object for which the member function is being called, allowing access to the object's data members and other member functions.
Usage of the this Pointer in Reference to Class & Object In C++:
-
Accessing Data Members: The this pointer helps differentiate between class data members and local variables or parameters with the same name. For instance, if a member function's parameter name conflicts with a data member's name, you can use the this pointer to clarify that you are referring to the object's data member.
-
Calling Member Functions: You can use the this pointer to call other member functions of the same class. This is particularly useful when chaining member function calls within a single member function.
-
Returning the Object: The this pointer can be returned from a member function to allow method chaining, where multiple member functions are called in a single statement.
-
Comparing Objects: The this pointer is also useful for comparing the current object with another object of the same class.
Also read: Static Member Function In C++: How to Use Them, Properties, & More
The Need For Semicolons At The End Of A Class In C++
A semicolon is required at the end of a class definition to indicate the end of the class declaration.
- This semicolon is not optional; it is a crucial part of the syntax for defining a class in C++ and many other languages.
- It tells the compiler that the class definition is complete and is necessary for proper code compilation.
Basic Syntax:
class ClassName {
// Data members and member functions
};
Example:
class Person {
int age;
int height;
}; // Semicolon is required hereint main() {
// Creating an object of class Person
Person person1; // This is correct
return 0;
}
In the snippet example above-
- Semicolon After Class Definition: The semicolon after the closing brace } of the class Person is mandatory. It signifies the end of the class declaration. Omitting this semicolon will result in a compilation error.
- Object Creation: The semicolon at the end of the class definition does not influence the creation of objects. Objects of the class can be instantiated in the main function or elsewhere after the class definition is complete.
- Syntax Requirement: The semicolon is needed after the class definition to ensure that the compiler correctly processes the class declaration.
Difference Between Structure & Class In C++
Listed in the table below are the key differences between structure and class in C++:
Feature |
Class |
Structure |
Definition |
A class in C++ is a user-defined type that encapsulates data and functions, providing abstraction and encapsulation. |
A structure is a user-defined data type that groups related variables but does not encapsulate functions. |
Default Access Levels |
The default access level is private. |
The default access level is public. |
Class in C++ can use all inheritance modes (public, protected, private). |
Structures can use inheritance but traditionally have been used without inheritance. | |
Memory Allocated |
Memory can be allocated on both the stack and the heap, depending on how the object is instantiated. |
Memory is generally allocated on the stack for structure objects, but heap allocation is also possible. |
Usage Convention |
Typically used for objects with complex behavior, encapsulation and data abstraction. |
Typically used for simpler data structures with public data access and minimal behavior. |
Null values |
Class in C++ can contain pointers and can have null values. |
Not compatible with null values. However, can contain pointers that are set to NULL. |
Interchangeability |
It can be used interchangeably with structures in modern C++. |
It can be used interchangeably with classes in modern C++. |
Conclusion
- Class: The class in C++ is a user-defined data type, that acts like a blueprint or a template for creating objects. It defines the structure and behaviour of object and is essential for object-oriented programming (OOP).
- Object: It is a real-time scenario of a class where memory is allocated once the class is instantiated. We pass objects as arguments the same way as we pass any other primitive data like int, string, etc. However, passing objects by reference is more suitable than passing objects by value.
- Data Members: A class may have data members that express an object's state. These data members, which may be of different data types, can record details about the properties of the object.
- Member Functions: These functions specify how an object of the class should behave. They can be used to perform actions, change the data members, and even give users a mode to communicate with the object.
- Object Creation: As mentioned before, classes serve as a blueprint for the creation of objects, which is done by instantiating a class.
- Access Control: The concept of access specifiers (i.e., public, protected, and private) helps control the visibility and accessibility of class members. This guarantees data encapsulation and data hiding.
- Constructors and Destructors: When an object in C++ is created, a constructor, which is a special member function, initializes the object's data members. Destructors, on the other hand, are responsible for releasing any resources utilized by the object and are called when the object leaves its scope.
Also read: 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What is the difference between class and object in C++?
Listed in the table below are the differences between a class and an object in C++.
Basis |
Class |
Object |
Definition |
A class in C++ is a blueprint or a user-defined data type that defines the structure and behavior of objects. |
An object in C++ is an instance of a class created based on the class blueprint. |
Role |
It acts like a template that specifies the data members (attributes) and member functions (methods) that objects of that class will have. |
It is an entity that represents a specific real-world entity with actual data and functionality. |
Memory |
Classes provide the design or layout for creating objects, but they don't consume any memory themselves. |
Objects consume memory. They contain the actual data members and can execute the member functions defined in the class. |
Declaration |
Class definitions are declared using the class keyword. |
Objects are defined by instantiating a class using its constructor. |
Q. In C++, is a class object stored in a heap or stack?
The memory allocated to the object depends upon how it is created. Meaning, an object in C++ can be allocated either in stack or heap memory, depending on creation and class scope.
Stack: A class object is kept in the stack memory when it is created as a local variable inside a function or a block. The program automatically allocates and deallocates the object's memory when it enters and leaves the defined scope of the object. For example:
void someFunction() {
MyClass obj; // Object 'obj' is stored in the stack
}
Here, Object 'obj' is automatically deallocated when the function exits
Heap: A class object created using dynamic memory allocation (using the new operator) is kept in the heap memory. To prevent memory leaks, the object's memory must be manually deallocated using delete. For example:
void someFunction() {
MyClass* objPtr = new MyClass(); // Object is created in the heap
delete objPtr;}
Here, Object ‘objPtr’ must be manually deallocated using the delete keyword.
Q. Is there an object class in C++?
No, there is no specific object class in C++. Objects are instances of user-defined class data types. Classes serve as blueprints (template) for creating objects. In other words, an object in C++ is a specific instance of a class containing both data and behavior defined by the class. When the object is instantiated, the compiler allocates the memory to the object.
Q. What are the types of classes in C++?
There are different types of classes in C++ based on how they interact with other classes and functionalities. Here are some of the commonly used classes:
- Base Class: A class that acts as a foundation/ building block for other classes. It contains common characteristics that are inherited by derived classes.
- Derived Class: A class that inherits attributes and behavior from the base class. It can extend or modify the behavior and properties of the base class by introducing new members.
- Abstract Class: A class that cannot be instantiated directly and contains one or more pure virtual functions. It acts as the basis or interface for other classes to derive from. Although pointers and references to abstract classes can be utilized, abstract class objects cannot be generated.
- Friend Class: A class that has access to another class's private and protected members is said to be its friend class. It is declared as a friend in the class whose data it wants to access.
Q. How do you declare a class in C++ and explain each component in it?
The syntax to declare a class in C++ is as follows:
class className{
access_specifier
//data members
//member functions
};
Explanation of syntax:
- The class keyword is used to define a class in C++.
- The access_specifier indicates the visibility of the following elements/ members. There are three specifiers, i.e., public, private, and protected.
- Data members of a class refer to the variables that hold the data related to the object of that class.
- Member functions are methods that define the behavior of the class and operate on data members.
Q. What is the use of the 'this' pointer in C++?
The this pointer is a special pointer available in non-static and const member functions. It points to the current object for which the member function is called. It helps in distinguishing between member variables and parameters with the same name. For example:
class MyClass {
public:
int value;
void setValue(int value) {
// Using "this" pointer to differentiate between data member and local variable
this->value = value;}
};
In the above example, the class MyClass has a data member value and member function setValue() that takes an integer parameter named value.
Inside the function, there is ambiguity between the parameter name value and the data member name value. We use the this pointer (i.e., this->value) to resolve this conflict and refer to the specific data member.
Q. What are data members and member functions in a class in C++?
- Data Members: Variables that represent the state or attributes of an object in C++. They store the data related to the object.
- Member Functions: Methods defined within a class that perform operations or manipulations on the data members. They determine the behavior of objects created from the class. Member functions work on data members to change the state of an object in C++.
This compiles our discussion on object and class in C++. You must also check out the following:
- Multiple Inheritance In C++ & Diamond Problem Resolution (Examples)
- Constructor Overloading In C++ Explained With Real Examples
- C++ If-Else | All Conditional Statements Explained With Examples
- Logical Operators In C++ | Use, Precedence & More (With Examples)
- C++ 2D Array & Multi-Dimensional Arrays Explained (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment