Data Abstraction In C++ | How-To, Types, Uses & More (+Examples)
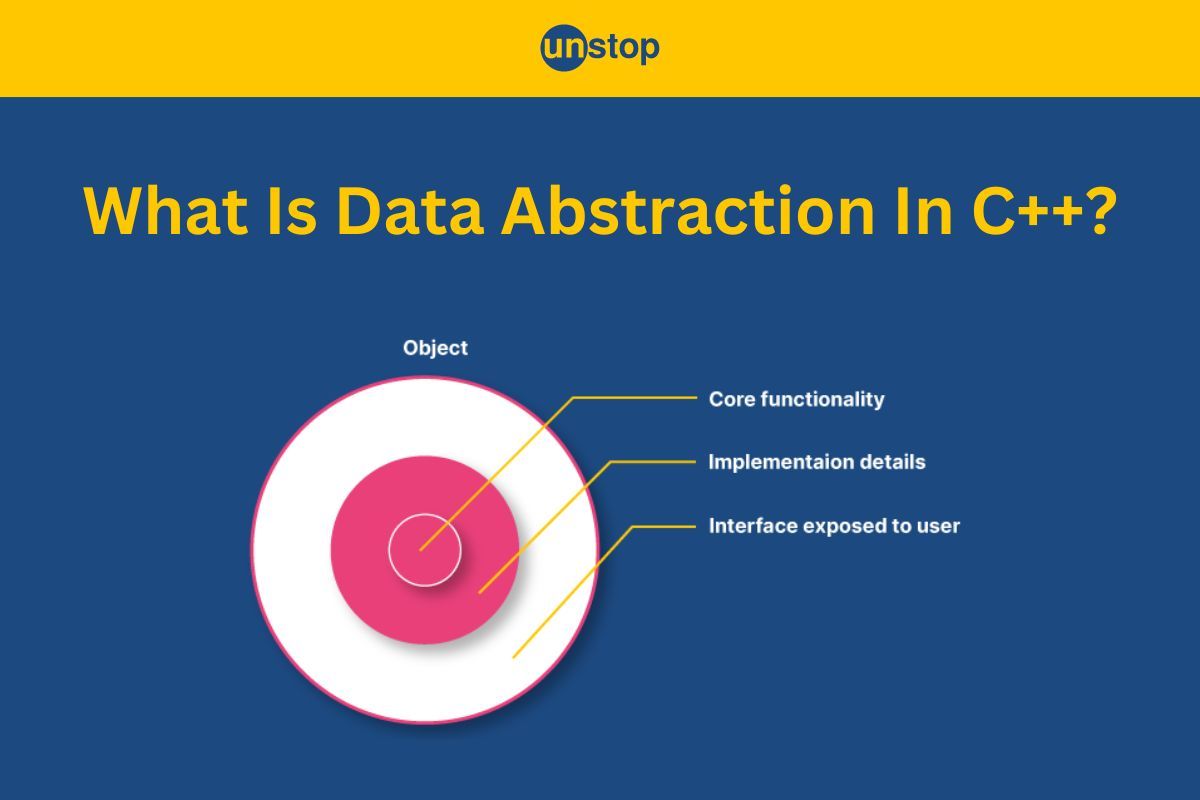
Table of content:
- Introduction To Abstraction In C++
- Types Of Abstraction In C++
- What Is Data Abstraction In C++?
- Understanding Data Abstraction In C++ Using Real Life Example
- Ways Of Achieving Data Abstraction In C++
- What Is An Abstract Class?
- Advantages Of Data Abstraction In C++
- Use Cases Of Data Abstraction In C++
- Encapsulation Vs. Abstraction In C++
- Conclusion
- Frequently Asked Questions
Data abstraction is a fundamental concept in computer science and programming, especially in the context of object-oriented programming languages like C++. It plays a crucial role in designing and developing complex software systems by allowing programmers to hide the implementation details of a data type or a class while exposing only the essential features and functionalities. In this article, we will explore what data abstraction in C++ programming is, its advantages and use cases, and how it is implemented.
Introduction To Abstraction In C++
Abstraction in C++ language is a fundamental concept in reference to object-oriented programming (OOP). It involves simplifying complex systems by breaking them down into smaller, more manageable parts while hiding unnecessary details. It is one of the four key principles of OOP, along with encapsulation, inheritance, and polymorphism.
Abstraction allows you to create abstract classes and interfaces that define a common set of methods and properties that subclasses must implement. These abstract classes and interfaces provide a blueprint for other classes to follow, ensuring consistency in the way objects are constructed and used in a program.
Types Of Abstraction In C++
In C++, there are two primary types of abstraction, namely, control abstraction and data abstraction. These concepts are an integral part of programming and writing efficient code.
What Is Control Abstraction In C++? With Example
Control abstraction refers to the process of hiding the details of control flow (like loops, conditional statements, and recursion) or the logic behind an algorithm. It allows you to encapsulate a sequence of operations into a higher-level function or method, effectively abstracting away the specific steps required to achieve a task.
Common examples of control abstraction in C++ include functions and procedures. When you call a function, you're using control abstraction to perform a specific operation without needing to know the internal implementation details.
Code Example:
Output:
Factorial of 5 is 120
Explanation:
In the above code example-
- We define a function factorial() to calculate the factorial of an integer n using recursion
- Inside the function, we use an if-else statement:
- If the base condition is true i.e n is less than or equal to 1, it returns 1(base case).
- Otherwise, if the base condition is not true it returns n multiplied by the factorial of n - 1(recursive case).
- In the main() function, we set num to 5 and call the factorial() function with this value( demonstrating control abstraction).
- We then print the result to the console, showing the factorial of 5.
When we run the above program, it calculates the factorial of 5 (5!) using the factorial function and displays the result as 'Factorial of 5 is 120' on the console.
What Is Data Abstraction In C++?
Data abstraction in C++ focuses on hiding the internal representation of data while exposing only the essential properties and operations. It allows you to define custom data types (classes) that encapsulate data and provide controlled access through member functions, effectively separating the 'what' (the interface) from the 'how' (the implementation).
We use classes and access specifiers (like public, private, and protected) to implement data abstraction in C++ programs. This means that the private data members store the data, and public member functions provide controlled access and manipulation of that data. Let's take a look at an example of the same.
Code Example:
Output:
Current Balance: $1300
Explanation:
In the above C++ program-
-
We define a class named BankAccount to represent a bank account. It encapsulates the data and operations related to a bank account, demonstrating the concept of data abstraction. Inside the class:
- There is a private data member balance of double data type to store the account balance.
- It also has a constructor that initializes the balance data member when a BankAccount object is created.
- We then define a void deposit() function that allows us to add money to the account's balance using the compound assignment operator.
- Next, we define a withdraw() function, which represents the act of withdrawing money. It contains an if-else statement that checks if the requested amount is less than or equal to the current balance.
- If there is enough balance, the if-block deducts the amount, updates the balance, and returns true.
- If there are insufficient funds, it returns false and prints the string message- "Insufficient funds!".
- Lastly, we define the getBalance() function, which retrieves and returns the current balance.
-
After that, inside the main() function, we create an object of the BankAccount class called account and initialize it with an initial balance of $1000.0. This action triggers the constructor, which sets the balance to $1000.0.
- The concept of data abstraction in C++ is demonstrated through the use of deposit() and withdraw() methods of the account object to interact with the bank account data.
- First, we call the deposit() function on the object account, using the dot operator. Here, we deposit an amount of $500.0 into the account increasing the balance to $1500.0.
- Similarly, we call the withdraw() function on the object and attempt to withdraw $200.0 from the account. Since there is sufficient balance, the withdrawal is successful, and the balance is reduced by $200.0, leaving us with $1300.0.
- Then, we call the getBalance() method on the account object to retrieve the current balance and store it in the variable currentBalance.
- Finally, we print the current balance using the std::cout statement, and the program terminates with a return 0 statement.
When the program is executed, it creates a bank account object, performs deposit and withdrawal operations, and displays the updated balance, which, in this case, is $1300.
Understanding Data Abstraction In C++ Using Real Life Example
As mentioned before, data abstraction in C++ is the process of simplifying complex systems by breaking them down into smaller, more manageable parts and hiding unnecessary details. In programming, data abstraction refers to the practice of representing the essential characteristics of an object while concealing unnecessary or irrelevant details.
This not only helps in reducing complexity but also enhances the maintainability and scalability of software systems. Imagine a television remote control as an analogy for data abstraction in C++ programming.
- When you use a remote control to operate your TV, you don't need to know how intricate electronic components inside the TV work or how the remote control's buttons translate to specific commands.
- Instead, you interact with a simplified interface, i.e., the buttons on the remote control, to achieve desired actions like changing channels or adjusting the volume.
In this analogy:
- The TV remote control represents the abstraction, offering a simplified way to interact with the TV.
- The TV's internal circuitry and mechanisms represent the underlying complexity, which is hidden from the user.
Below is an example of data abstraction in C++ for the television remote control analogy given above.
Code Example:
Output:
Changed to channel 5
Adjusted volume to 60
Explanation:
The above code example is structured around the concept of a TV remote control, with an abstract base class and a concrete derived class-
- We define an abstract class, RemoteControl, as the base class representing the TV remote control. Inside the class-
- We declare two pure virtual functions changeChannel() and adjustVolume(), which represent the actions the remote control can perform.
- Being pure virtual functions (indicated by = 0), these methods do not have implementations in the RemoteControl class itself, making it an abstract class that cannot be instantiated directly.
- Next, we create a concrete implementation (derived class) of the TV remote control called ConcreteRemoteControl. This derived class contains a constructor for creating an object of the class.
- It has two private integer data members, currentChannel and currentVolume which are initialized with default values 1 and 50, respectively.
- It also provides actual implementations for changing channels and adjusting the volume. Both implementations use std::cout to print a message to the console.
- In the main() function, we create an instance of ConcreteRemoteControl named remote and use it to change the channel and adjust the volume.
- We call the changeChannel() method on the remote object to change the channel to 5.
- Similarly, we call the adjustVolume() method to adjust the volume to 60.
The code demonstrates the principles of data abstraction, encapsulation, and polymorphism in C++. It allows users to interact with the TV remote control through a high-level interface (RemoteControl) without needing to understand the internal workings of the TV or the remote control.
Ways Of Achieving Data Abstraction In C++
Here are the primary ways to achieve data abstraction in C++. We have explained both these methods in proper detail, with examples.
Data Abstraction In C++ Using Classes & Access Specifiers
Abstraction using classes and access specifiers is a fundamental aspect of object-oriented programming (OOP) that allows you to define custom abstract data types with attributes (data members) and behaviors (member functions) while encapsulating the internal details. Classes provide a blueprint for creating objects, and access specifiers (public, private, protected) control the visibility of members.
Public Specifier:
Members declared as public are accessible from anywhere in the program, including outside the class.
- This is the interface through which external code interacts with the class.
- Public members typically represent the intended behavior or functionality of the class.
class MyClass {
public:
int publicData; // Public data member
void publicMethod() {
// Public member function
}
};
Private Specifier:
Members declared as private are only accessible within the class itself.
- This hides the internal implementation details of the class from external code.
- Private members are used to store and manage the state of the object.
class MyClass {
private:
int privateData; // Private data member
public:
void setPrivateData(int value) {
// Public member function to set privateData
privateData = value;
}
int getPrivateData() const {
// Public member function to retrieve privateData
return privateData;
}
};
Protected Specifier:
Members declared as protected are similar to private members but are accessible within derived classes. In other words, protected members are used to allow derived classes to inherit and manipulate the base class's behavior and data.
class BaseClass {
protected:
int protectedData; // Protected data member
public:
void setProtectedData(int value) {
protectedData = value;
}
};
class DerivedClass : public BaseClass {
public:
void modifyBaseData() {
protectedData = 42; // Accessible in derived class
}
};
Let's see an example to understand how data abstraction is done using classes and access specifiers in C++.
Code Example:
Output:
Person's Name: Alia
Name: Alia
Age: 26
Explanation:
The code includes two header files: <iostream> for input/output operations and <string> for string manipulation.
- We then define a Person class with two private data members- name (a string type) and age (an integer type).
- It also has a public constructor that initializes the name and age attributes when an object of the Person class is created, accepting name (n) and age (a) as parameters.
- Next, we define a getName() function, which is a getter method that retrieves the person's name.
- We also define a public setter method, setAge(), to modify the person's age. It contains an if-statement, which only allows age modification if the provided value is non-negative.
- Lastly, we define a constant member function, displayInfo(), that displays the person's name and age using the std::cout stream. It is marked as const because it displays information without altering the object's state.
- In the main() function, we create an instance of the Person class named person, with the attributes name and age initialized with values Alia and 25 respectively.
- Next, we call the getName() function (using the person object and dot operator to retrieve the person's name) inside std::cout statement to print the information.
- Similarly, we call the setAge() to update the person's age to 26. This update is conditional, verifying that the provided age is non-negative.
- Finally, we call the displayInfo() member function to display the person's updated information, including their name and age.
Data Abstraction In C++ Using Header Files
Abstraction in header files involves separating the interface (declarations) of a class or functions from their implementations (definitions). Typically, you create a header file (.h) containing class declarations, function prototypes, necessary include guards, and a source file (.cpp) containing the actual code. Let's understand the above concept using an example.
Code Example:
Output:
Original Information:
Name: Alia
Age: 25Updated Information:
Name: Bhaskar
Age: 30
Explanation:
The above C++ code example demonstrates data abstraction using header files by encapsulating the person's details (name and age) within the Person class.
-
As evident from the code comments, the program is divided into three sections, i.e., a header file (Person.h), a source file (Person.cpp), and the main program (main.cpp).
-
Inside the header file (Person.h):
- We declare a Person class, which has two private data members- name (of string type) and age (of integer type), and a public constructor to initialize object members.
- It also has five public member functions— the getter methods (getName, getAge) to get the name and age, the setter methods (setName, setAges) to change the name and age, and a function to display (displayInfo) person information.
- Inside the source file (Person.cpp)-
- We have the constructor implementation to initialize name and age data members when a Person object is created, using initializer lists.
- Then, the implementation of getName() and getAge() methods that access and return the values of private members name and age of the person, respectively.
- The implementation of setName() and setAge() methods enable modificationof name and age data members of person object. Here, we use the 'this' pointer to differentiate between the parameter and the member variable with the same name.
- After that, we have the implementation of displayInfo() method that retrives and prints the person's name and age to the console using std::cout.
-
Lastly, there is the main program (main.cpp), i.e., the main() function, which serves as the entry point of program's execution.
-
Inside main, we create a Person object named person with the name variable initialized with Alia and an age variable with the value of 25.
- Then, we call the displayInfo() function using the person object and dot operator. We use the std::cout stream to print the original information of the object.
- Next, we call the setName() and setAge() methods to update the attribute (name and age) values to Bhaskar and age 30.
- Once again, we call the displayInfo() method to print the updated information.
- Finally, the main() function returns 0, indicating successful execution.
The code demonstrates data abstraction using header files by encapsulating the person's details (name and age) within the Person class
Check this out: Boosting Career Opportunities For Engineers Through E-School Competitions
What Is An Abstract Class?
An abstract class in C++ is a class that cannot be instantiated on its own and is designed to serve as a base or blueprint for other classes. It is a key concept in object-oriented programming and is used to define a common interface or set of methods that must be implemented by its derived (sub) classes. Abstract classes provide a way to enforce a specific structure and behavior among a group of related classes.
Syntax Of Abstract Class
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
// Other member functions and data members (if needed)
};
The syntax of an abstract class in C++ mandates the declaration of at least one pure virtual function in the class. A pure virtual function is a function without any implementation in the base class and is marked with (= 0). Now, let's take a look at an example to see how an abstract class works in a code/ program.
Code Example:
Output:
Drawing a circle.
Area: 78.5398
This is a shape.Drawing a rectangle.
Area: 24
This is a shape.
Explanation:
-
We define an abstract class named Shape, which serves as a base class for geometric shapes.
- The class contains two pure virtual functions and a non-virtual function-
- The draw() and area() are pure virtual functions that represent the action of drawing a shape and calculating the shape's area, respectively.
- These functions are declared without implementation (i.e., as = 0), making Shape an abstract class. This means we cannot instantiate Shape class directly and must create derived classes that provide implementations for these functions.
-
The non-virtual function (non-abstract method) printInfo() prints a message to the console indicating that it's a shape.
-
Next, we define two concrete derived classes, Circle and Rectangle, that publically inherit from the base class Shape.
- The Circle class has a private member variable radius of type double and implementations of the draw() and area() functions. The area() method calculates the area of a circle based on its radius using the formula π*radius*radius.
- Similarly, the Rectangle class has private member variables length and width and implementations of the draw() and area() functions. The area() method calculates the area of a rectangle using the formula length * width.
-
Inside the main() function-
- We create an instance of the Circle class, named circle, and initialize it with a radius of 5.0. Similarly, we create an instance of the Rectangle class, named rectangle, and initialize it with dimensions 4.0 x 6.0, respectively.
- Next, we create an array of Shape pointers called shapes[] to store pointers to the class objects. This demonstrates polymorphism, where objects of derived classes can be treated as objects of the base class Shape.
- Then, we initiate a for loop to iterate through the shape[] array. It first calls the draw() method on each shape, using the arrow operator. This prints a message indicating the type of shape (circle or rectangle).
- The loop then calls the area() method to calculate and print the area of each shape.
- We terminate the loop after calling the printInfo() method on each shape, which prints a general message indicating that it's a shape.
- Each shape is printed with its respective information, followed by an empty line for separation.
-
Finally, the program returns 0, indicating successful execution.
Advantages Of Data Abstraction In C++
Here are some key advantages of abstraction/ data abstraction in C++:
-
Simplification of Complexity: One of the primary purposes of abstraction is to simplify complex systems. It allows developers to focus on high-level structures and concepts while ignoring the lower-level background details. This simplification makes it easier to understand and manage complex systems, reducing cognitive load and the potential for errors, hence making the application secure.
-
Modularity: Data abstraction in C++ encourages the creation of modular code. By breaking down a system into smaller, self-contained components (such as classes or modules), you can work on one piece at a time, which is easier to design, develop, test, and maintain. This modular approach enhances code reusability and collaboration among team members.
-
Encapsulation: Abstraction is closely related to encapsulation, another core concept in object-oriented programming. Encapsulation hides the internal state and implementation details of an object from the outside world. Data abstraction in C++ makes the interface independent of interacting with the object, allowing you to change the implementation without affecting the code that uses it. This separation of concerns enhances security, maintainability, and flexibility.
-
Security: By exposing only the necessary interfaces and hiding implementation details, abstraction helps improve security. It reduces the risk of unintended access to sensitive data or functionality.
-
Reusability: Abstraction promotes code reusability. Abstract classes, interfaces, and generic constructs provide blueprints that multiple classes or components can implement or extend. This reduces low-level code duplication and allows developers to leverage existing abstractions to build new functionality.
-
Flexibility and Extensibility: Abstraction allows for the creation of flexible and extensible systems. By defining abstract classes or interfaces, you can establish contracts that other classes must adhere to. This makes it easier to add new features or functionality by creating new classes that implement the same abstraction. Existing code that depends on the data abstraction in C++ can remain unchanged.
-
Maintenance and Debugging: Abstracting complex systems makes debugging and maintenance more manageable. When issues arise, you can focus on specific components or abstractions rather than having to navigate through a convoluted, monolithic codebase. This saves time and effort in identifying and fixing problems.
-
Communication and Collaboration: Abstraction serves as a common language for communication among developers. It provides a clear and agreed-upon way to describe the structure and behavior of a system. This common understanding is essential for effective collaboration on software projects, especially in larger teams.
-
Adaptation to Change: Data abstraction in C++ and other languages helps software systems adapt to changing requirements. When requirements evolve, or new features are needed, you can often extend or modify existing abstractions rather than rewriting large portions of the codebase. This reduces the risk of introducing errors during changes.
-
Testing and Quality Assurance: Abstraction in C++ code makes it easier to design and conduct tests. You can test individual components or abstractions in isolation, ensuring that each part of the system behaves as expected. This granularity enhances the quality and reliability of the software.
Use Cases Of Data Abstraction In C++
Data abstraction is a fundamental concept in software development and has various use cases across different domains. Here are some common use cases of data abstraction in C++:
-
Database Abstraction:
- In database management systems, data abstraction layers provide a consistent and simplified interface for interacting with various database engines (e.g., MySQL, PostgreSQL, MongoDB).
- Developers can work with a unified API, abstracting the underlying database-specific details.
-
Graphics and UI Libraries:
- Graphics libraries like OpenGL provide an abstraction layer for hardware-accelerated rendering.
- User Interface (UI) libraries abstract the complexities of creating graphical user interfaces, allowing developers to design interfaces using high-level components.
-
File I/O Abstraction:
- Data abstraction in C++ can simplify file input/output operations by providing a consistent interface to read and write files, regardless of their actual storage medium or format.
-
Networking Abstraction:
- Network libraries and protocols (e.g., HTTP, TCP/IP) abstract the complexities of network communication, enabling developers to build networked applications without dealing with low-level networking details.
-
Hardware Abstraction:
- Hardware abstraction layers (HALs) provide a consistent interface for interacting with hardware devices such as sensors, GPUs, and peripherals.
- This simplifies the development of hardware-dependent software, including embedded systems and device drivers.
-
Operating System Abstraction:
- Operating systems abstract the underlying hardware and provide a uniform environment for running applications.
- Developers can write software that runs on different operating systems without worrying about specific OS details.
-
Data Structures and Algorithms:
- Data abstraction in C++ allows developers to work with high-level data structures (e.g., arrays, lists, trees) and algorithmic concepts (e.g., sorting, searching) without needing to implement them from scratch.
-
Object-Oriented Programming (OOP):
- In OOP, the abstraction concept is a core principle, allowing developers to define classes and objects with abstract interfaces (e.g., methods) that hide internal implementation details.
- Inheritance and polymorphism are used to create abstract base classes and concrete derived classes.
-
APIs and SDKs:
- Software development kits (SDKs) and application programming interfaces (APIs) provide abstraction layers for external services, libraries, and platforms.
- Developers can integrate third-party functionality into their applications using well-defined interfaces.
-
Distributed Systems:
- Abstraction is essential in designing and implementing distributed systems, as it allows developers to work with remote services and data sources as if they were local.
- Technologies like Remote Procedure Calls (RPC) and Representational State Transfer (REST) provide abstractions for distributed communication.
-
- Cloud service providers abstract infrastructure and resources, allowing users to deploy and manage applications without dealing with the underlying hardware or data center operations.
-
Database ORMs (Object-Relational Mapping):
- ORMs abstract database tables and queries into high-level object-oriented representations, making database interactions more intuitive for developers.
-
Game Development:
- Game engines abstract the complexities of real-time graphics rendering, physics simulations, and audio processing, enabling game developers to focus on gameplay and content creation.
-
AI and Machine Learning:
- Data abstraction in C++ is used to create high-level machine-learning frameworks that allow developers to build and train complex models without delving into the mathematical details of algorithms and neural networks.
-
Testing and Test Abstraction:
- Test frameworks provide an abstraction for writing and executing tests, making it easier to automate testing processes and evaluate software quality.
In each of these use cases, data abstraction in C++ simplifies the interaction between complex systems and end-users or developers. It enhances code maintainability, modularity, and reusability, and it allows for better management of software complexity in various domains.
Encapsulation Vs. Abstraction In C++
In this section, we will discuss the difference between the two key pillars of OOPs programming- encapsulation and abstraction/ data abstraction in C++. To begin with, let's see what we understand by the term encapsulation.
Encapsulation
Encapsulation in C++ is one of the four fundamental concepts of object-oriented programming (OOP) and involves bundling data (attributes) and the methods (functions) that operate on that data into a single unit called a class. The key idea behind encapsulation is to hide the internal state of an object and provide controlled access to it.
In other words, it restricts direct access to an object's data and requires clients to use accessor and mutator methods to interact with the object's state. Encapsulation helps ensure data integrity and promotes the principle of information hiding.
Key Points About Encapsulation:
- Data members (attributes) of a class are often declared as private to prevent direct access from outside the class.
- Public methods (member functions) are provided to manipulate and access the data safely.
- It enforces the principle of access control, allowing the class to decide what parts of its internal state are exposed to the outside world.
Difference Between Encapsulation & Abstraction In C++
Here's a table summarizing the differences between encapsulation and data abstraction in C++:
Aspect | Abstraction | Encapsulation |
---|---|---|
Definition | Simplifies complex systems by focusing on high-level details and hiding low-level implementation details. | Bundles data and methods into a single unit (class) and controls access to the data. |
Primary Purpose | Reduces complexity, provides a clear and concise interface, and hides unnecessary implementation details. | Protects an object's internal state from unauthorized access and modification while allowing controlled access through methods. |
Implementation | Often implemented using abstract classes and interfaces. Defines a contract for derived classes. | Implemented within a class by declaring data members as private (or protected) and providing public methods (getters and setters) to access or modify the data. |
Access Control | Focuses on providing abstract interfaces with no specific access control. | Emphasizes access control by using private and protected access specifiers for data members and public methods for controlled access. |
Example Syntax (C++) |
class AbstractBase { // Pure virtual function |
class MyClass { public: int getPrivateData() const { |
Conclusion
Data abstraction is a cornerstone of software engineering and plays a crucial role in managing complexity, improving code quality, and facilitating code reuse. In C++, it is achieved through classes and access specifiers or by using header files to declare class interfaces. Real-life examples demonstrate how data abstraction simplifies complex systems. In a world where software systems are growing increasingly intricate, data abstraction remains a valuable tool for software developers. It enables us to build robust, scalable, and maintainable software solutions while keeping the underlying complexity under control. By embracing data abstraction in C++, developers can unlock new possibilities and elevate the quality of their code.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What are the three levels of data abstraction in C++?
In computer science and database management, data abstraction typically consists of three levels:
-
Physical Level:
- This is the lowest level of abstraction.
- It focuses on the physical representation of data on storage devices.
- It is concerned with details like data structures, file organization, and access methods.
- In databases, it deals with how data is stored on disk, including file formats, indexing, and data storage structures.
-
Logical Level:
- This is the middle level of data abstraction in C++ and programming in general.
- It provides a conceptual view of the data without being concerned with physical storage details.
- It defines the structure and organization of data without specifying how it is physically implemented.
- In databases, it involves defining tables, relationships, constraints, and views without detailing how data is stored on disk.
-
View Level (or External Interface Level):
- It is the highest level of data abstraction.
- The focus here is on the user's perspective and how data is presented to applications and end-users.
- It defines user-specific views of the data, hiding the complexities of the logical and physical levels.
- In databases, it includes defining user interfaces, query languages, and access rights for different user roles.
Q. How do access specifiers help achieve data abstraction in C++?
Access specifiers help in data abstraction in C++ by controlling the accessibility of class members. This means that we can hide the implementation details of a class from the user and only expose the essential details.
For example, we can declare a class member as private to make it inaccessible to the user. This means that the user cannot directly access the member, and they must use the public member functions of the class to access it.
This helps to achieve data abstraction by hiding the implementation details of the class from the user. This makes the class more reusable and maintainable, as the user does not need to know how the class works in order to use it.
Here are the three access specifiers in C++ and their meanings:
- Public: The members declared as public can be accessed from anywhere in the program.
- Private: The members declared as private can only be accessed from within the class.
- Protected: The members declared as protected can be accessed from within the class and from its derived classes.
The access specifiers are an important part of data abstraction in C++. By using access specifiers, we can hide the implementation details of a class from the user and only expose the essential details. This makes the class more reusable and maintainable.
Q. What is abstraction vs. abstract class in C++?
Abstraction and abstract class are two different concepts in C++.
- Abstraction is a process of hiding the implementation details of an object or data structure from the user. This allows the user to focus on the essential features of the object or data structure without having to worry about how it works.
- An abstract class is a class that has one or more pure virtual functions. A pure virtual function is a function that has no implementation. This means that any class that inherits from an abstract class must override the pure virtual functions.
Abstraction is a broader concept than abstract class. Data abstraction in C++ can be achieved in many ways, such as using access specifiers, interfaces, and abstract classes. Abstract class is a specific way to achieve abstraction.
Q. What is data abstraction in C++ with example?
Data abstraction in C++ is an object-oriented programming technique that focuses on hiding the implementation details of an object and exposing only the necessary features or interfaces to the user. The goal is to reduce complexity and allow the user to interact with objects at a higher level, without needing to know the underlying implementation.
Here’s a simple example of data abstraction in C++:
Explanation:
In this example, the Circle class uses data abstraction to hide the internal detail of the radius while exposing a public method getArea() that calculates and returns the area of the circle. The user interacts with the Circle object through this simple interface, without needing to know how the area is computed internally.
Q. Why is abstraction used in a class?
Abstraction is used in a class to hide the implementation details from the user. This allows the user to focus on the essential features of the class without having to worry about how it works. Here are some reasons why abstraction is used in a class:
- To reduce complexity: Data abstraction in C++ classes can help to reduce the complexity of a class by hiding the implementation details. This makes the class easier to understand and use.
- To improve reusability: Abstraction can help to improve the reusability of a class by defining the common behavior of a group of classes. This allows us to create concrete classes that inherit from the abstract class and override the behavior as needed.
- To improve maintainability: Data abstraction can help to improve the maintainability of a class by isolating the implementation details from the user. This makes it easier to change the implementation without affecting the user of the class.
- To improve flexibility: Data abstraction in C++ programs can help to improve the flexibility of a class by allowing the user to customize the behavior of the class. This can be done by overriding the pure virtual functions in the abstract class.
You might also be interested in reading the following:
- Function Overloading In C++ With Code Examples & Explanation
- New Operator In C++ | Syntax, Usage, Working & More (With Examples)
- Static Member Function In C++ Explained With Proper Examples
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Sort() Function In C++ & Algorithms Explained (+Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment