- What Is Object-Oriented Programming (OOP)?
- Structure Of Object Oriented Programming
- Principles Of OOP
- OOP Concepts
- Advantages Of OOP
- Disadvantages of OOP
- Applications of OOPs
- Frequently Asked Questions
What Is Object-Oriented Programming? Understanding The Basics
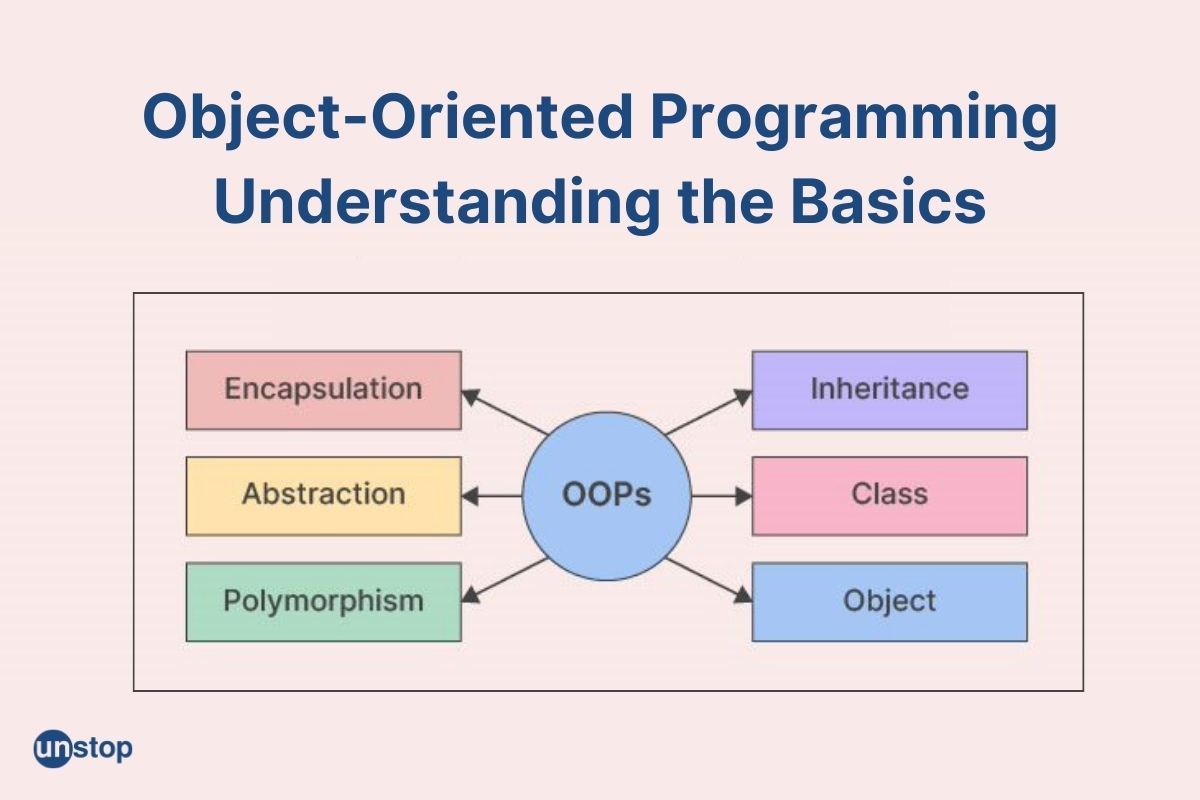
Object-oriented programming models are tools used to write instructions for computers. Object-oriented is a programming model that uses objects that contain data or attributes and code, referred to as methods, that manipulate the attributes. A programming model differs from a language because it provides a deeper structure than a standard set of commands or syntax rules used in most programming languages.
What Is Object-Oriented Programming (OOP)?
OOPs is a popular programming paradigm based on the idea that objects make up the world. Thus, software should be designed as a collection of objects. It's a popular approach to programming, used to achieve complex programming tasks.
Listed below are the core concepts in object-oriented programming:
- Concept of object
- Concept of polymorphism,
- Concept of abstraction,
- Concept of encapsulation,
- Concept of class, and
- Concept of inheritance.
Object Oriented Programming has several key concepts; for example, classes are used to define objects that can have properties or attributes associated with them. Abstraction is an extension of encapsulation of classes of objects that allows one to focus on the most important features of class when implementing high-quality software without worrying about its details and complexity.
Encapsulation ensures that data contained within classes remains secure from external interference or manipulation by allowing only authorized methods to access it. Inheritance makes it possible for one class in a hierarchy structure of classes to inherit features from its generic parent class while providing additional characteristics or improvements that make it unique from other hierarchies or structures. Polymorphism enables developers to create multiple forms of similar functionality using their defined parameters, making codes much simpler than traditional approaches.
With these basic attributes and extra features like hierarchical inheritance, multilevel inheritance, hybrid inheritance, etc., object-oriented programming offers advantages over traditional procedural language models, including improved code readability, scalability and maintenance capability, thus leading toward high-quality of software deliverables.
History of OOP
Object-oriented programming (OOP) was first developed in the late 1960s by computer scientists Odin Dahl and Alan Kay. OOP is based on a model of creating software programs composed of objects, which contain data and actions or behaviours.
Over time, object-oriented programming evolved to include features like abstraction, encapsulation, inheritance classes, and interfaces. Today, OOP is not just a popular programming paradigm, but rather, one of the most widely used approaches for developing modern applications. It enables developers to create feature-rich apps with reusable code structures across multiple platforms quickly and efficiently.
Languages that use OOP
Object-oriented programming (OOP) languages support the concept of classes and objects, encapsulation, data hiding, and inheritance. Examples of OOP languages include:
Java: Java is a widely used general-purpose language developed by Sun Microsystems in 1995. It has powerful library functions for graphical user interface programming and network communication. It supports object-oriented features such as classes, methods, polymorphism, etc.
C++: C++ was originally developed by Bjarne Stroustrup in 1979 at Bell Labs. This multi-paradigm language provides high-level abstraction using Oriented Programming concepts while maintaining the efficiency and control offered by low-level C language operations on memory resources like pointers, etc., making it one of the most popularly used modern-day programming languages today.
C#: Initially released in 2000, aimed mainly at Microsoft’s .NET platform development, C# quickly gained popularity among programmers due to its flexibility when compared to other equally powerful but more complex solutions like Visual Basic 6 or JAVA 2 EE editions so far tailored only towards Windows platforms. Today, this managed code environment runs not only on native Windows machines but can also be compiled into cross-platform .NET Core versions targeting macOS or Linux operating systems.
Python: Python is a scripting language that was introduced in the late 1980s. Since then, it has been widely adopted by developers due to its clear syntax, customizable libraries and other features like dynamic typing, support for object-oriented programming (OOP) style, etc., making it one of the most popular languages amongst students as well as professionals alike.
Ruby: Ruby is an open-source interpreted language developed initially in 1995 and created with ease of use in mind. First targeted at web development, today, it is also used for non-web-related tasks, including application server-side processing or general-purpose computing operations. Its powerful, flexible OOP model helps create maintainable software code, thus reducing debug time significantly compared to C-based solutions.
PHP: While not strictly an Object Oriented Programming (OOP) language, PHP does support the concept of classes, making it one of the most widespread server-side scripting languages today - primarily due to its perfect integration with HTML5 plus JavaScript, allowing developers to quickly deploy full-featured dynamic digital platforms in shortest times ever when compared to other more complex solutions like Java EE or .NET Core versions available for running exclusively under Windows machines.
Scala: Scala is a general-purpose programming language combining object-oriented and functional programming paradigms. Its powerful high-order function feature makes it the perfect choice when you require static typing and reduced lines of code written. It cuts debug time in half, compared to other traditional alternatives like Visual Basic 6 or even C# itself while being interoperable with existing Java code libraries. This allows developers to reuse the same components from previous software releases regardless of whether they run on Android, iOS, Windows, or macOS machines, thanks to the new cross-platform .NET Core version now available.
Structure Of Object Oriented Programming
Object-oriented programming (OOP) organizes and writes code based on the interaction between objects. OOP comprises four basic building blocks: classes, objects, attributes and methods.
Classes
Classes are blueprints for creating objects in an Object-oriented program that define their properties and behaviour through data fields (attributes) and subroutines or functions known as 'methods.' The class name acts as a template name to create other similar objects within that same group defined by the class definition. A real-life example would be if we wanted to make cars - all classes would have certain specifications like four wheels, engines, etc. Still, different car models may also come with unique features such as specific interior details or colour options available only for that model alone.
Objects
Objects are instances created from these blueprinted classes, which contain both data variables & associated functions/subroutines called methods/functions linked together under one identity; this helps bring structure into our programs, making it easier to read & debug later down the line over procedural approaches without any hassle whatsoever! As per the definition of object, it can inherit characteristics from another superclass or parent class, so they don't need to rewrite everything each time when creating similar objects.
Attributes
Attributes are characteristics of an object that define its properties by providing detailed information about it, such as colour or size. An attribute is a type of data field that assigns values to the particular instance created from any given class; this helps us to distinguish each object more accurately and makes debugging easier.
Methods
Methods are functions/subroutines associated with specific classes and their respective objects – they define how these objects behave in certain situations, e.g., if we had a car object, then one method could be for turning on the engine start-up process (which would involve checking oil levels, etc.). These methods can also be used when defining relationships between different classes. Example - You may have multiple cars linked together via some 'group' relationship where common attributes/methods like driving speed limits apply across all instances.
Principles Of OOP
OOP is a model in which programs are organized around objects and data rather than actions and logic. OOP simplifies software development and maintenance using four main principles: Abstraction, Encapsulation, Inheritance, and Polymorphism.
Abstraction
This principle allows us to focus on relevant details of an object while hiding other details from the outside world that are not required or related to it, reducing complexity for the user. It enables developers to create new classes without being concerned with every detail about how the class interacts with existing code.
Encapsulation
This concept ensures that only certain parts of our application can access each object’s internal state information directly—other parts should use public methods or properties instead. Thus, it increases the security of our code by ensuring no one else can fiddle with its internals, unless we allow them to via specified interface/method names, etc.
Inheritance
This principle helps developers build upon existing functionality when writing subclasses, as long as those sub-classes belong within hierarchies; so if you have a parent-child relationship, your child class inherits all behaviour from their base class
Polymorphism
This principle enables us to use a single interface for objects of different types. The developers can write code that works on multiple class hierarchies without writing additional code, leading to less repetition and better maintainability in the long run. Two types of polymorphism in OOP are - method overriding and method overloading.
OOP Concepts
Object Oriented Programming is a programming paradigm that focuses on breaking down complex problems into smaller objects and interactions between these individual objects to solve the problem. It helps developers organize their code better for easier future maintenance and makes the development process more efficient from start to finish. Some core concepts in OOP are:
Coupling
This concept refers to how closely two or more classes are related in an object-oriented program – i.e., whether they need each other's methods and properties. Low coupling is desirable because it makes programs easier to change, maintain and debug over time. High coupling can lead to difficulty in understanding what happens when one part of the system changes, with cascading effects elsewhere that may be difficult to predict at design time.
Cohesion
This concept measures how closely linked each class' behaviour is within itself - whether its members support a single focused purpose or represent greater cohesiveness than if there were multiple disparate behaviours, all lumped together under one class. High cohesion of this type generally leads to resealable code that does only what it needs to do without unnecessarily trying to perform any extraneous tasks. Utility functions can separate less cohesive behaviours and keep the class uniform and focused.
Composition
This OOP concept is a special form of aggregation, whereby one class 'contains' another. It creates part-whole relationships between objects in which the contained object cannot outlive its containing object – it's destroyed as soon as that parent class goes away. This way, composition can help with code reuse and maintainability by creating higher-order structures beyond individual classes or functions.
Association
This term describes how two (or more) different classes interact when they're related to each other logically but don't contain one another. For example, employees working on a project might have an association between them where neither contains the other explicitly. Associations make programs easier to read at design time by helping developers easily identify important logical connections; however, these kinds of implicit connections are usually fragile because their meaning is often lost if either of the associated entities changes substantially over time without updating all references accordingly!
Aggregation
Technically speaking, this type of OOP relationship refers specifically to "has-a" relationships like those described above regarding composition; however, due to its similarities with regular associations, many newer programming languages consider aggregates synonymous with said type relations. Particularity, aggregations require that the contained objects outlive their containers - in other words, they must be able to exist independently of one another even after becoming part of an aggregate.
Advantages Of OOP
Object-oriented programming is a programming method that uses classes, objects, inheritance and encapsulation to model real-world entities and their properties. It follows core concepts such as abstraction, modularity, polymorphism and reusability, making it one of the most powerful paradigms in modern software development.
In OOP, user-defined data types are created using class templates for complex codes with public interface definitions with internal details hidden away from users; runtime polymorphism allows individual instances to improve on basic structures by overriding methods or adding new features through overloading.
By allowing developers to create abstractions of real-world entities, programs become easier to maintain since changes made at the object level propagate throughout the entire system without disrupting its overall structure, thus enabling reusable software designs. Here are some other key advantages of OOP:
- Modularity: OOP allows for the creation of objects that can be reused in different programs by making programming more efficient and less time-consuming.
- Abstraction: By using abstraction techniques, such as classes and subclasses, it is possible to hide certain details while focusing on other aspects of programming without changing your code's overall structure or functionality. This helps developers keep track of complex systems by providing a clear overview instead of an unorganized set of functions and variables used to produce desired outputs from inputs.
- Extensibility: Through inheritance mechanisms like class extensions, it is easy to modify existing codes with minimal effort while continuing to use old functionalities whenever needed; this ensures that you don't have to rewrite entire portions if all you need are small adjustments or additions/deletions here and there throughout your coding project(s).
- Encapsulation: The benefit of encapsulation is to help hide data from external access, ensuring nothing is touched or altered unintentionally, which will invariably lead to program bugs and errors.
- Polymorphism: Through polymorphism, it's possible to send different kinds of messages (in the form of classes) with the same message name but containing varied content outputs. This can be extremely useful when dealing with multitudes of projects where certain elements are repeated multiple times; a single class representing each element would work wonders for streamlining programming efforts across various tasks!
Disadvantages of OOP
OOP also has a few disadvantages, namely:
- Complexity: Object-oriented programming can be complex because it sometimes involves a lot of detail and conceptual structures that are difficult to understand. It also requires additional overhead for the objects’ interactions at runtime, which may lead to slower program execution than procedural coding techniques.
- Cost: Object-oriented programming requires more time upfront in designing an object model since you must consider how all those objects will interact with each other over their lifetime before writing any code. This translates into a higher project cost than traditional programs, especially on large projects where the design costs become significant.
- Difficult Debugging: Debugging OOP programs is often more difficult than debugging procedural programs due to their complexity and potential redefinition of existing variables or classes by derived class definitions from outside sources like libraries or user input data (which could have unexpected effects). Additionally, some errors may only arise when certain objects interact in ways unforeseen during development tests. This is because interdependent relationships between various parts of the system may only be realized in the later stages of testing cycles - leading to potentially costly delays in production releases. At the same time, problems are sorted out after launch day.
Applications of OOPs
The different features of object-oriented programming make it ideal for various applications. Some popular applications that use object-oriented design are:
- Games: Object-oriented programming offers a highly efficient way of creating graphical user interfaces and thus makes for an ideal platform for creating games.
- Business Applications: Being able to model real-world situations using objects is one of OOP's major advantages over procedural programming, making it useful in business application development as well.
- Artificial Intelligence: Creating intelligent programs such as expert systems requires an object-oriented approach in its design since AI involves complex data sets and rule-based actions, which can be more easily managed via encapsulation offered by OOP paradigms.
- Networking Applications: Networking involves multiple layers, each containing specific tasks and roles. Thus, this type of application needs objects or classes representing different types of devices/nodes connected through networks, etc., making understanding easier with the help of inheritance between related subclasses among other features offered by object-oriented languages like Java, C++, etc.
- Image Processing Applications: Complex image processing algorithms rely heavily on objects and classes for efficient working. Creating images through pixels involves organizing large data sets that can be easily handled via encapsulation offered by OOP paradigms.
Frequently Asked Questions
1. What is the basic concept of Object Oriented Programming (OOP)?
Object Oriented Programming (OOP) is a programming paradigm that focuses on defining and creating objects that contain data fields, attributes and methods to represent real-world entities. It emphasizes modularity by breaking down complex problems into smaller pieces while relying heavily on inheritance for code reuse across different classes.
2. How does encapsulation help in software maintenance?
Encapsulation helps in software maintenance as it isolates changes made to one module from affecting other modules or components within the system; thus making sure any new features built in an application are easily integrated without disrupting existing functionality or causing instability over time.
3. What are some common properties of objects?
Common properties of objects include identity, state, behaviour, and encapsulation - all allowing them to be self-contained units that can interact with other objects in meaningful ways, such as sending messages between each other via method calls or changing their internal states through properties defined within its class/prototype.
4. Can you define an object?
An object is an instance of a particular type created at runtime based on definitions written using procedural code for some languages or, more often, using a Class construct found in OOP languages like Java/C++/Python, etc. Objects have specific identities and behaviours depending upon how they were programmed during the development process. They also hold information about their own current 'state.'
5. How do simple structures facilitate OOP programming patterns?
Simple structures help OOP programming patterns by allowing for the easy and organized development of modular code. This means that pieces of an application can be more easily changed or extended without creating a large web of dependencies throughout the whole system - as objects are logically encapsulated from each other with clear boundaries between their blocks, making them easier to maintain & update over time.
6. Please explain dynamic binding and method overriding/overloading in detail.
Dynamic binding is when methods (or functions) are not bound to a given type until they are called at runtime, i.e., it is deferred until execution rather than being set during compile-time. Method overriding/overloading refers to changing existing method definition, which has already been inherited to add new functionality on top while still maintaining previous behaviour if needed; this allows developers extra flexibility when designing applications depending on what specific features need implementation later down the line.
7. In what ways can OOP be applied to solve real-world problems or design a Real-time system?
OOP can be applied in many ways to solve real-world problems, such as modeling complex systems like machine learning algorithms, developing graphical user interfaces where information must be presented visually across components with data manipulation behind the scenes, etc. It is also useful in building Real-time systems due to its ability to handle events quickly through efficient sorting/searching plus concurrent processing capabilities, all thanks to steady flow inheritance pattern-based architectures.
8. Are there any features that make objects unique compared to concrete objects in the real world?
Features that make objects unique include having explicit identities defined within their classes, immutable states that cannot be changed directly, and encapsulation from external forces. It can also be done by focusing on how they interact with other objects through method calls/properties, allowing more robust & tightly coupled designs.
9. What advantages come with developing reusable classes instead of single objects for each application?
Developing reusable classes instead of single objects gives developers extra flexibility in adding new features to existing systems and having greater control over structures. This results in less time spent debugging when making updates or integrating changes due to class-level definitions all working together smoothly.
10. How will collaborative development benefit from using an object-oriented approach when designing solutions for a project?
Collaborative development using an OOP approach typically offers improved security, scalability & performance compared to non-OOP approaches, ensuring multiple teams can work closely on the same project without sacrificing too much speed or efficiency levels while still retaining support for large projects. Thanks to the modular nature of code, it is organized into separate blocks each responsible for different pieces of logic functionality.
You may also like to read:
I am a biotechnologist-turned-writer and try to add an element of science in my writings wherever possible. Apart from writing, I like to cook, read and travel.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
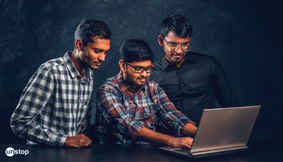
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
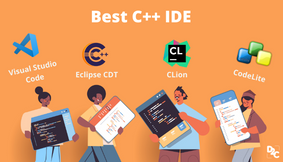
10 Best C++ IDEs That Developers Mention The Most!
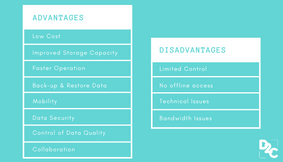
Advantages and Disadvantages of Cloud Computing that you should know!
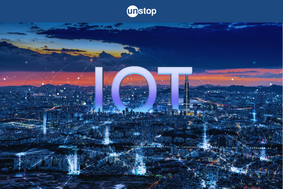
samyak dandge 3 weeks ago