What Is C++ Programming? Syntax, Application, Job Prospects & More!
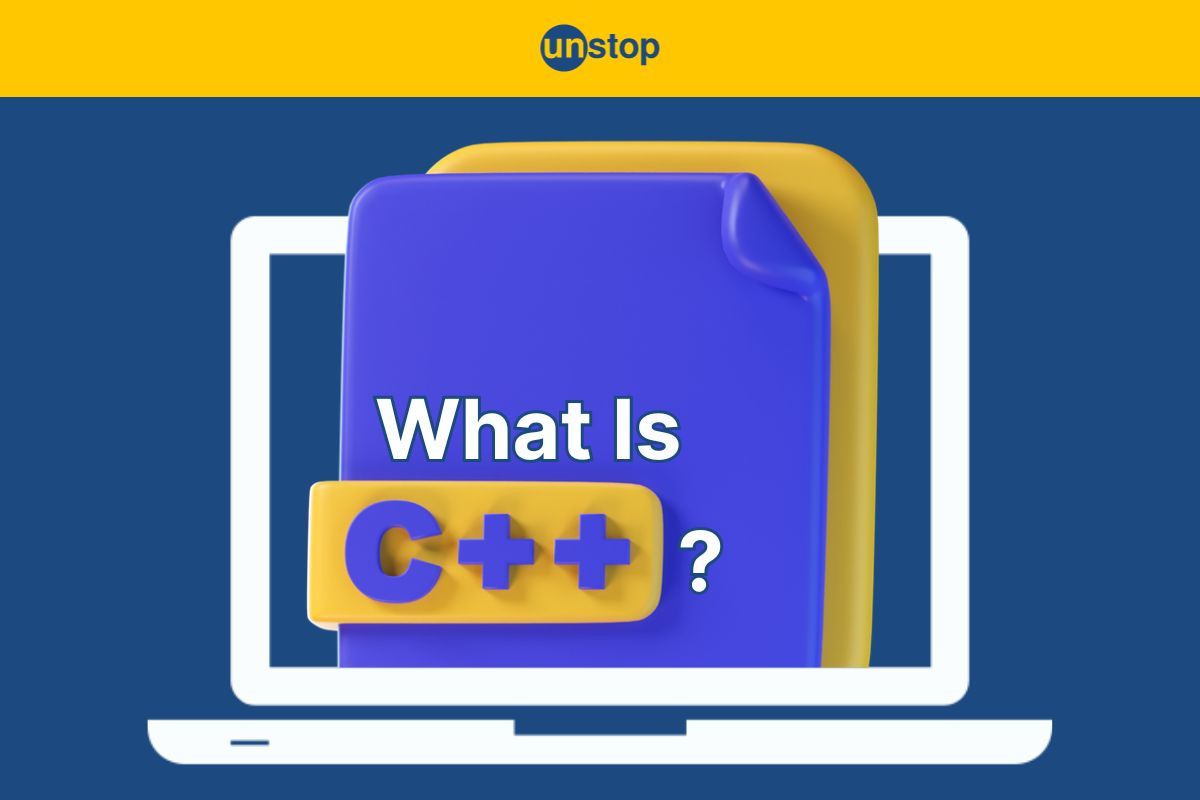
Every one of us who is interested in programming or even computer science must have heard of C++, one of the most popular programming languages out there. But how many of us know everything about the language and its applications? Well, if you don't know a lot about this amazing language as yet, then you are in the right place. In this blog, we will discuss what is C++, its features, libraries, and applications, and even look at an example and programming interview questions for better understanding.
What Is C++ Programming Language?
C++ (full form C plus plus) is a multi-paradigm, general-purpose programming language that is an extension of C. It was designed to include general-purpose, object-oriented programming language and other functions along with low-level memory manipulation. Also, C++ is a middle-level language, that is, it encapsulates features of both low-level language and high-level language. The idea to develop C++ as an extension of C with added features struck Danish computer scientist Bjarne Stroustrup while he was working at Bell Labs, USA. And as they say, the rest is history!
C++ Introduction And History
The coding language first made its appearance in the programming world in 1979. Today, it is one of the most versatile programming languages used for a wide variety of applications. Some common applications of C++ are software development, game programming/ development, operating systems, graphics applications, embedded systems, etc.
To know more about the history and timeline of this language, read- History Of C++ | Detailed Explanation (With Timeline Infographic)
Important Features Of C++ Programming Language
The robust and useful features packed in this single, core programming language are probably one of the main reasons behind its popularity. But what makes it so good? Well, listed below are some key features that you must know of.
- Simple & Easy: C++ facilitates the breakdown of program code into smaller and more logical units. Add to that the fact that it comes with rich library support; it's much easier and simpler to use this popular language.
- High Performance: C++ works in a manner where C++ code is converted into machine code, which can then be run directly on the hardware. This helps provide faster results and, at the same time, high performance.
- Mid-Level Language: This feature implies that C++ can be used for low-level system programming, such as for networking, kernels, drivers, etc. And also for high-level application programming such as for game engines, applications like Photoshop, and more.
- Object-Oriented Programming Language: This means that C++ supports the concepts of encapsulation, polymorphism, inheritance, etc. The inclusion of these object-oriented programming principles allows the programmer to write more organized and reusable code. It is just as suitable for all types and sizes of application design.
- Large Active Community & Rich Library: C++ has an extensive peer community of developers, resulting in plenty of resources for troubleshooting and learning. Also, it comes with rich libraries and frameworks, which result in speedy development.
- Cross-Platform Language: C++ is a platform-independent language, meaning that code written in this particular language can be compiled and run on different operating systems and hardware without any modification in regulation.
- Low-Level Memory Manipulation: C++ provides greater control over memory allocation and management by directly accessing and modifying memory addresses in our code. This further converts into efficient memory usage.
- Compatibility with C: C++ can use C libraries and codes in C++ language programs, making it backward compatible with the C programming language.
- Compiled Language: This means that the source code in C++ gets converted into an executable file, i.e., machine code, entirely before execution. The execution speed for C++ is high, and also there is no additional processing overhead in C++. This makes it one of the fastest programming languages out there, adding to its popularity.
How To Use C++ Programming Language?
Anyone interested in programming usually begins with C/ C++ to understand the basic logic behind writing efficient code. Here is a brief into how to use C++ in programming-
- Using C++ involves the process of creating, writing, and executing C++ code. You begin by writing your code in a text editor or an integrated development environment (IDE) and save it with a .cpp extension.
- Essential header files are included using #include directives to access functionalities like input/output operations and data structures.
- The program's execution starts with the main function, where you write the core logic of your program.
- Declare and initialize variables as needed, using appropriate data types.
- Input and output operations are facilitated through cout for output and cin for input.
- After successful compilation, you run the program in a terminal or command prompt, generating the desired output.
- Debugging tools may be employed to identify and rectify errors. Testing the program with various inputs helps ensure its functionality, and you may iterate on the code to make improvements.
C++ Syntax
C++ syntax refers to the set of rules that dictate how C++ programs are written. It encompasses the correct arrangement and combination of symbols, keywords, and expressions that make up valid C++ code. Here are some general syntax rules for writing C++ code:
Comments: Single-line comments are preceded by //, and multi-line comments in C++ are enclosed between /* and */. They are needed to make the code more readable and understandable for other programmers.
// This is a single-line comment
/*
This is a
multi-line comment
*/
Header Files: You must include necessary header files at the beginning of the program using #include directives. These files supply us with functions/ elements essential for the program to run properly.
#include <iostream>
using namespace std;
Main Function: Every C++ program must have a main function where execution begins. The return 0 statement marks the end of this function, indicating a successful run without errors.
int main() {
// Code goes here
return 0; // Indicates successful program execution
}
Statements and Semicolons: Statements are terminated with a semicolon (;). They tell the compiler that a respective line has ended and ensure that there is no carryover to the next line of code.
cout << "Hello, World!" << endl; // Statement ending with a semicolon
Variables: Declare variables with a data type followed by the variable name. We can initialize and declare a variable in one go or even separately.
int age; // Declaration
age = 25; // Initialization
Data Types: Use appropriate data types for variables (e.g., int, double, char, string).
int number = 42;
double pi = 3.14;
char grade = 'A';
string name = "John";
Input/Output: Input is obtained using cin, and output is displayed using cout. These are part of the <iostream> library.
cout << "Enter your age: ";
cin >> age;
C++ Standard Libraries: What You Need to Know
The STL or standard libraries supported by C++ are an integral part of the language since they provide users with a range of tools and functions to simplify the development process.
There are three most commonly used STLs:
- The Standard Template Library (STL)- This library consists of a range of generic algorithms and data structures that help in building complex applications.
- The C Standard Library- This library consists of a range of functions for working with strings, files, and other low-level programming tasks.
- The Boost C++ Libraries- This comprises a set of open-source libraries that extend the functionality of C++.
Some benefits of the standard libraries are:
- The STL is a collection of templates providing a wide range of generic algorithms and iterators. It also includes algorithms like searching, sorting, and manipulating sequences.
- The C Standard Library provides macros for performing repetitive and everyday tasks such as I/P-O/P operations, exception handling, threading, etc.
- The C++ Standard Library contributes by providing additional functionality to the C Standard Library.
- We also have namespaces, within which all functions, classes, and objects are defined. We need an adequate header file to make proper use of position and types.
- The C++ Standard Library is designed to be compatible with different compilers and platforms and is efficient in performance simultaneously.
All in all, the standard libraries add a whole lot of wealth of functionality to the language.
How To Write C++ Code?
Writing C++ code involves several steps. Here is the process that you should follow while writing a code in C++:
Step 1: Set Up Your Development Environment
- Install a C++ compiler (e.g., GCC, Visual C++ for Windows, Xcode for macOS).
- Choose a text editor or an integrated development environment (IDE) for coding (e.g., Visual Studio Code, Eclipse, Code::Blocks).
Step 2: Create a C++ Source File- Create a file with a .cpp extension (e.g., main.cpp). This file will contain your C++ code.
Step 3: Include Necessary Header Files- Use #include directives to include necessary header files for input/output operations (<iostream>), data structures (<vector>, <string>), and other functionalities.
Step 4: Define the main Function
- Every C++ program starts with the main function.
- Declare the main function as int main().
- Include the code that will be executed when the program runs within curly braces {}.
Step 5: Declare Variables
- Declare variables for storing data and using appropriate data types (e.g., int, double, char, string).
- Initialize variables with values if and when needed.
Step 6: Perform Input/Output- Use cout for output (printing to the console) and cin for input.
Step 7: Error Handling- Implement error handling mechanisms, such as try, catch, and throw, for handling exceptions.
Step 8: Memory Management
- Be mindful of memory allocation and deallocation, especially when using pointers.
- It is a good idea to use smart pointers or containers to manage memory automatically when possible.
Step 9: Comments- Add comments to explain complex logic or to provide documentation for your code.
Step 10: Compile and Run
- Compile your code using the chosen compiler.
- Execute the compiled program to test its functionality.
Step 11: Debugging
- Use debugging tools or techniques to identify and fix errors in your code.
- Print statements, breakpoints, and debugging tools can be helpful.
C++ Program: A Practical Example
To better understand how C++ program works, let’s have a look at a simple program that calculates the sum of two numbers on the console.
Code:
Output:
Enter the first number: 6
Enter the second number: 8
The sum of 6 and 8 is 14
Explanation:
In the simple C program above-
- We begin by including the iostream header and using namespace std.
- Next, we initiate the main() function, which is the entry point and is responsible for the execution of the instruction.
- Then, we declare three integer variables, i.e., num1, num2, and sum.
- We then use the cout object for outputting a message to the console asking the user to feed in the two numbers (i.e., num1 and num2) one by one.
- The cin object reads the user input for the first number and stores them in the respective variables.
- Next, we initialize the sum variable and use the addition arithmetic operator to add them. The result of this is stored in the sum variable.
- We once again use the cout object to output the final result of the sum calculation to the console.
- The program then returns a value of 0, indicating that the program ran successfully.
The Practical Applications Of C++
Listed below are some of the most common applications of the C++ programming language:
Programming Of Game Development Engines
Programming of game development engines and building games is a resource-intensive process. The C++ features like high performance and low-level memory control, execution speed, etc., make it the preferred choice for game development companies. C++ allows programmers to manipulate hardware and memory, optimize the use of resources, and allow procedural programming. Many popular video gaming systems engines, including Unity and Unreal, are written in C++ competitive programming language.
Web Development
C++ is less standard than other backend programming languages like JS and Python for web development but is still the language of choice for high-performing server applications. Many core web browsers, like Mozilla, Chrome, etc., are written in C++.
Embedded Systems & IoT
Embedded systems are where the program is embedded with the hardware inside the object itself. For example, smartwatches, smart appliances, medical equipment, etc. These devices then operate on limited computing resources. C++ is often the choice of programming language for programmers to control and ensure that these programs run efficiently even in with limited resources. C++ is also used for real-time processing and control system development.
Operating Systems
C++ is one of the fastest and most robust languages, which is widely used in developing many operating systems. This includes Windows, Microsoft Office, iOS, IDE Visual Studio, etc.
Scientific Computing
C++ can handle large datasets and efficient complex calculations. There are many libraries in C++ for this purpose, like Boost, Armadillo, etc. This makes it the language of choice when conducting scientific computation.
Finance Industry
It also has applications in the finance industry, like developing risk management software, trading algorithms, and also banking applications.
Compilers and Interpreters
C++ is used in the development of compilers and interpreters for various programming languages. Its low-level capabilities make it suitable for creating tools that analyze and translate code.
3D Graphics Software
C++ is widely used in the development of 3D graphics software, including computer-aided design (CAD) applications and 3D modeling tools. Its performance benefits contribute to real-time rendering and complex graphics computations.
AI and Machine Learning
C++ is used in AI and machine learning/ deep learning applications, particularly for performance-critical components. Libraries like TensorFlow and OpenCV have C++ interfaces adding to its popularity for this segment.
Multimedia Applications
C++ is employed in the development of desktop applications and multimedia applications, including audio and video processing software, due to its efficiency and ability to handle complex data structures.
Advantages And Disadvantages of C++
By now, you must have a clear idea about what is C++ and why it is so popular. But nothing in the world is perfect. In this section, we will discuss some important advantages as well as disadvantages of this quick programming language.
Advantages of C++
C++ is a versatile and powerful programming language that offers several advantages, making it a popular choice for various applications. Here are some key advantages of C++:
- C++ allows for low-level memory manipulation and direct access to hardware, providing high performance and efficiency. This makes it suitable for system-level programming and resource-intensive applications.
- C++ supports the principles of object-oriented programming, including encapsulation, inheritance, and polymorphism. OOP enhances code organization, reusability, and maintainability.
- C++ programs can be written to be platform-independent, allowing software developers to write code that can run on different operating systems with minimal modifications.
- The STL in C++ provides a rich set of generic algorithms, containers, and utilities. This library simplifies complex programming tasks, improves code reuse, and enhances productivity.
- C++ is a multi-paradigm programming language supporting procedural, object-oriented, and generic programming. Developers can choose the most suitable paradigm for their project, providing flexibility in coding styles.
- C++ has a strong connection with the C language. It allows developers to integrate and use existing C code seamlessly, facilitating interoperability between C and C++.
- C++ has a large and active community, resulting in extensive documentation, libraries, and online resources. Developers can easily find support, tutorials, and solutions to common problems.
- C++ is a general-purpose language used in diverse fields, including systems programming, game development, embedded systems, RDBMS applications, networking, graphics programming, and application development. Its versatility makes it applicable across various industries.
- C++ provides low-level access to memory and hardware resources, making it suitable for programming tasks that require direct interaction with hardware components.
Disadvantages of C++
Some common drawbacks of C++ are as follows:
- C++ has a more complex syntax compared to some other programming languages. This complexity can make it more challenging for beginners to learn and understand.
- While manual memory management provides flexibility, it also introduces the risk of memory leaks and dangling pointers, which can be difficult to debug and manage.
- Unlike some modern programming languages, C++ does not have automatic garbage collection. Developers are responsible for managing memory allocation and deallocation, which can be error-prone.
- C++ lacks reflection capabilities, making it more difficult to inspect and manipulate objects at runtime compared to languages that support reflection.
- While there is a standard for the C++ language, the implementation of features can vary between compilers. This lack of strict standardization may sometimes lead to portability issues.
- Due to its complex features and syntax, C++ has a steeper learning curve, especially for beginners. Mastery of the language often requires time and experience as against some other programming languages like Python.
- C++ allows for low-level operations, making it possible to write code that can lead to security vulnerabilities, such as buffer overflows and memory corruption.
While it’s a great language, its drawbacks must be taken into consideration when deciding whether to use it for a particular object.
Why Should You Learn C++?
C++ is a widely used programming language with several features and advantages (as discussed above) that make it suitable for various applications. Here are key reasons why C++ is chosen:
- Efficiency: C++ provides low-level access to memory, allowing for efficient manipulation and control, which is crucial for system-level programming and performance-critical applications.
- Performance: C++ allows for close-to-the-hardware programming, making it well-suited for high-performance applications like game development, real-time systems, and embedded systems.
- Portability: C++ programs can be written to be platform-independent, allowing code to run on different operating systems with minimal modifications.
- Object-Oriented Programming (OOP): C++ supports object-oriented programming, enabling the creation of modular, reusable, and maintainable code through concepts like classes and inheritance.
- Standard Template Library (STL): The STL in C++ provides a rich set of generic algorithms, data structures, and utilities, enhancing productivity and code quality by offering pre-built components.
- Flexibility: C++ is a multi-paradigm language supporting procedural, object-oriented, and generic programming. This flexibility allows developers to choose the most suitable paradigm for their project.
- Legacy Code Compatibility: C++ has a strong connection with the C language, allowing developers to integrate and use existing C code within a C++ program seamlessly.
- Community and Resources: C++ has a large and active community, leading to extensive documentation, libraries, and online resources. Developers can easily find support and solutions to common problems.
- Wide Range of Applications: C++ is used in diverse fields, including systems programming, game development, embedded systems, networking, graphics programming, and application development. Its versatility makes it a valuable language across industries.
- Control Over Hardware: C++ provides control over hardware resources, making it suitable for programming tasks that require direct interaction with hardware components.
- Memory Management: C++ allows manual memory management, providing developers with control over memory allocation and deallocation. This feature is essential for applications requiring fine-tuned memory usage.
- Job Prospects: Given the immense uses and advantages of this language, the demand for programmers proficient in C++ is high. Also, C++ forms the basis for many other programming languages, making it easier for programmers to diversify. This further improves their scope of employability and leads to higher pay packages.
Learn C++ | Amazing References
There are many excellent books for learning C++. Here are some highly recommended ones are as follows:
- C++ Primer by Stanley B. Lippman, Josée Lajoie, and Barbara E. Moo- An excellent book for beginners that covers the fundamentals of C++. It is known for its clear explanations and hands-on examples.
- Accelerated C++ by Andrew Koenig and Barbara E. Moo- Focuses on practical programming and is suitable for those who want to quickly get up to speed with C++.
- C++ Primer Plus by Stephen Prata- A comprehensive guide that covers C++ basics and progresses to more advanced topics, suitable for those looking to deepen their understanding.
- The C++ Programming Language by Bjarne Stroustrup- Authored by the creator of C++, this book is for readers who want a deep understanding of the language. It covers C++ features and design principles.
C++ Career Prospects
C++ is a powerful and versatile programming language that has been widely used in various industries for decades. It offers a combination of low-level and high-level programming capabilities, making it suitable for a broad range of applications, from system-level programming to high-performance software development. As a result, there are numerous career opportunities for C++ programmers in India.
Average Salary for C++ Programmers in India:
The salary of C++ programmers in India can vary based on factors such as experience, location, industry, and the specific skills of the individual. The average salary for C++ developers in India can range from ₹4 lakh to ₹15 lakh per annum. However, these figures can vary significantly.
Salary Based on Experience:
The salary of C++ programmers often increases with experience. Here's a rough breakdown:
- Entry-Level (0-2 years): ₹4 lakh to ₹8 lakh per annum
- Mid-Level (2-5 years): ₹8 lakh to ₹12 lakh per annum
- Senior-Level (5+ years): ₹12 lakh and above per annum
Keep in mind that these figures are approximate, and the actual salary can vary based on factors like the company's size, location, and the programmer's specific skill set. Additionally, the demand for C++ programmers in industries such as gaming, finance, embedded systems, and software development contributes to competitive salary packages.
Important Topics In C++ Programming
Now that you know what is C++, let's take a look at a few important concepts that you must know if you too want to make the most of the amazing opportunities that come with knowing how to work with this language.
Difference Between C And C++
Here's a table that highlights the key differences between C and C++:
Feature | C | C++ |
---|---|---|
Paradigm | Procedural programming | Multi-paradigm (Procedural, OOP, Generic) |
Object-Oriented | Does not support OOP | Fully supports OOP with classes, objects, inheritance, polymorphism, and encapsulation |
Abstraction | Low-level programming, less abstraction | Higher-level abstractions with classes and objects |
Syntax and Features | Simpler syntax, fewer keywords | Expanded syntax with additional keywords and features |
Memory Management | Manual memory management using malloc and free | Supports manual memory management but also provides features like constructors and destructors |
Standard Template Library (STL) | Lacks a standardized template library | Includes the Standard Template Library (STL) with generic algorithms, containers, and utilities |
Namespace | Does not have namespaces | Introduces namespaces for better organization and avoiding naming conflicts |
Error Handling | Relies on error codes and manual error-checking | Supports exceptions for more robust error handling |
File I/O | Uses standard I/O functions (printf, scanf) | Uses the iostream library for file I/O (cin, cout) |
Compatibility | Older, with a large codebase in various industries | Evolved from C and includes C compatibility. Widely used in modern software development |
Use Cases | Systems programming, embedded systems, low-level programming | Application development, game development, and projects benefitting from OOP design. |
For a more in-depth understanding of how these languages differ, read- Difference Between C And C++ Explained With Code Example
Conclusion
Still, wondering about what is C++? Surely you are not! C++ programming stands as a cornerstone in the ever-evolving landscape of software engineering. Its efficiency, support for multiple functional programming paradigms, and compatibility with C contribute to its enduring popularity. From crafting high-performance games to driving the intricacies of operating systems, C++ continues to play a pivotal role in diverse domains.
As technology advances, the versatility and power of C++ ensure its relevance, making it a language of ideal choice for developers aiming to build robust and efficient software solutions. Whether you are a seasoned developer or a newcomer to the programming world, the journey into the realm of C++ promises a rich and rewarding programming experience.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What language is CPP?
CPP is a programming language that stands for C Plus Plus. It is an extension of the C programming language and includes additional features such as OOP, templates, and exception handling.
Q. Is cpp a complex language?
C++ can be challenging to learn and use effectively, especially for beginners. Some might consider it a complex language with many features and concepts, such as pointers and memory management, that can be difficult to understand.
Q. How to learn cpp?
Learning C++ involves understanding the language syntax, concepts, and best practices. There are many online resources, books, and courses available that can help beginners learn C++. You can check out the C++ course at Unstop here- Learn Cpp.
Q. How do I create a cpp file in C++?
To create a .cpp file in C++, you can use any text editor or integrated development environment (IDE) such as Visual Studio or Code::Blocks. Then, create a new file with the .cpp extension and write your C++ code.
Q. What is header and cpp file?
In C++, header files contain declarations of functions, variables, and other entities used in a program.cpp files contain the implementation of those entities. Header files are included in .cpp files using the #include preprocessor directive.
Q. How do I run a cpp file in g++?
To run a cpp file in g++, compile it using the g++ command in the terminal or command prompt. For example, the order is as follows: g++ file.cpp -o output_file_name. This will create an executable file with the name specified after (-o). Then, you can run the executable file using the command given as- ./output_file_name command.
Q. What is the difference between .H and .CC files?
The .h file extension is used for header files in C++. These files contain function declarations, class declarations, and other important information that the .cc or .cpp files need to compile the program. The .cc or .cpp file extension is used for source files that contain the actual implementation of the C++ functions and classes declared in the header files.
This compiles our insightful discussion on what is C++, but it's not the end. Here are a few interesting topics from the plethora of advanced concepts/ languages for you to immerse in:
- Compilation In C | A Step-By-Step Explanation & More (+Examples)
- Similarities And Differences Between C++ And Java
- 10 Important Features Of Python That You Must Know!
- Difference Between Abstract Class And Interface In C# (In Detail)
- Data Structure Interview Questions For 2024 [With Detailed Answers]
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment