Table of content:
- What Is A While Loop In C++?
- Parts Of The While Loop In C++
- C++ While Loop Program Example
- How Does A While Loop In C++ Work?
- What Is Pre-checking Process Or Entry-controlled Loop?
- When Are While Loops In C++ Useful?
- Example C++ While Loop Program
- What Are Nested While Loops In C++?
- Infinite While Loop In C++
- Alternatives To While Loop In C++
- Conclusion
- Frequently Asked Questions
Understand The While Loop In C++ & Its Variations With Examples!
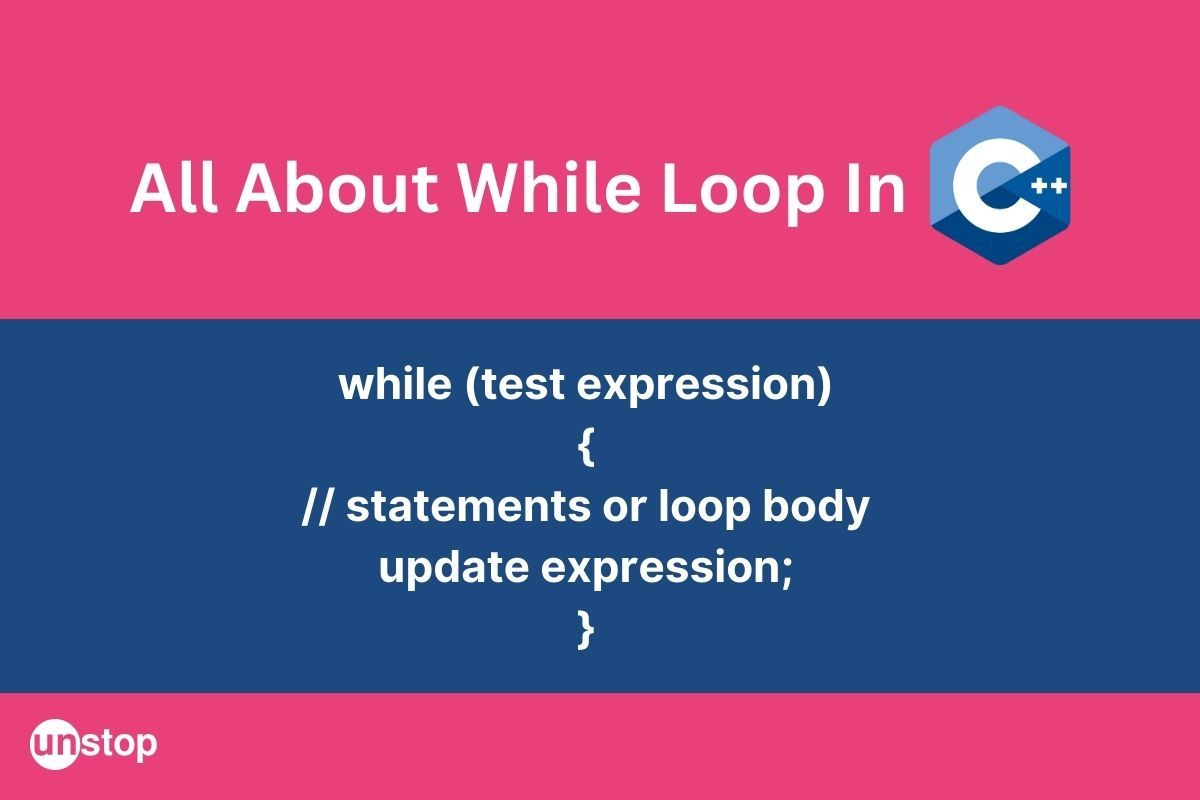
In programming, we make use of different types of statements to make our piece of code efficient as well as fast. Suppose we want to print all numbers from 1-100. Writing a print statement for 100 numbers will be a lengthy and inefficient process. This is where loops in C++ come into play. It is easy to repeatedly execute a block of program statements with the help of loops.
There are three types of loops- for, while, and do-while. In this article, we will focus on while loop in C++ programming, including its syntax and programs. We will also cover the working of the while loop, its uses, execution, and a few alternatives. We will see various code examples along with the explanation to have a better understanding of the topic.
What Is A While Loop In C++?
As mentioned above, loops are used to execute blocks of statements repeatedly. There are two kinds of loops- entry controlled loop and exit controlled loop.
- A while loop in C++ is an example of an entry-controlled loop wherein the condition is checked at the entry of the loop.
- The loop runs until the condition is true, and the statements/ block of code inside the body of the loop are executed.
- The loop terminates as soon as the condition is unsatisfied.
- While loop is mainly used when we do not know how many times a statement will be executed.
- In such a case, the number of iterations is unknown, and it depends on the condition inside the while loop.
In C++, we can make use of nested while loops as well. Also, there are infinite loops, which run forever because the condition in them is always true.
Syntax:
while (test expression)
{
// statements or body of loop
update expression;
}
Here,
- The test expression in the while() is what is evaluated to see its holds true or not.
- If the condition is true, the statements (or the chunk of code) inside the curly brackets are executed.
- The update expression refers to the expression/ statements which update loop variable after every iteration before moving to the next.
- Again the condition is checked, and this is continued until the condition becomes false.
- The control comes out of the loop when the condition becomes false.
Parts Of The While Loop In C++
A while loop in C++ consists of three main parts, as specified below:
The Test Expression
The Test Expression is a condition that decides whether the body of the loop will be executed or not. The result of this is always a boolean statement that is true or false. The loop execution process occurs until the condition is true and stops executing when the loop test expression becomes false.
The Loop Body
The loop body includes the implementation code, which gets executed till the condition in the test expression is true. Most part of the driver code block is written here. This could be anything, including some functions, initialization expressions, blocks of statements, loop blocks, or different algorithms.
Update Expression
The update expression is written inside the body of the loop to increment/decrement the variable value, used in the test expression. If this is missing, the loop will run infinitely.
C++ While Loop Program Example
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgaSA9IDE7Cgp3aGlsZSAoaSA8IDUpewoKY291dCA8PCAiWW91IGFyZSB1bnN0b3BwYWJsZSFcbiI7CgppKys7fQoKcmV0dXJuIDA7fQ==
Output:
You are unstoppable!
You are unstoppable!
You are unstoppable!
You are unstoppable!
Explanation:
- In the above code snippet, we declare and initialize a variable i with the value of 1. We then make use of a while loop to print some statements.
- A test expression (i<5) is checked, and the control moves to the first statement.
- We use the cout statement inside the while loop to print the statement 'You are unstoppable.
- After the statement gets printed for the first time, the value of i then becomes 2 because of the update expression (i++).
- The program again checks the condition, which is true, and the statement is executed for the second time.
- This is continued until i becomes 6, the condition (6<5) becomes false, and we exit the loop.
How Does A While Loop In C++ Work?
The working mechanism of the while C++ loop can be broken into the following steps:
Step 1: The while loop is initiated.
Step 2: The control immediately moves to the test expression or the condition inside the parenthesis.
Step 3: The condition is tested, and-
- The flow of control goes to the body of the loop if the condition is true.
- The control moves outside the loop if the condition is false.
Step 4: The body of the loop gets executed, and the statement inside it gets printed.
Step 5: The update expression updates the value of the control variable after the execution of loop body.
Step 6: The control goes back to Step 2. This continues until the test condition turns false.
Step 7: The while loop ends, and the control goes outside the loop.
Flow chart Of While Loop In C++
The flow diagram below presents a visual presentation of the while loop's working mechanism.
What Is Pre-checking Process Or Entry-controlled Loop?
The pre-checking process or entry-controlled loop refers to the case where the evaluation of the loop condition happens before the execution of the loop body. This type of loop structure is commonly used in languages like C++ and is exemplified by the while and for loops.
In simple words, in an entry-controlled loop, the condition is checked at the beginning of each iteration.
- If the condition evaluates to true, the loop body is executed.
- If the condition is false from the start, the loop body is skipped entirely, and the program continues with the code following the loop.
The key characteristic of an entry-controlled loop is that the loop may not execute at all if the condition is false from the beginning. This makes it suitable for situations where the loop may or may not need to run, depending on the condition.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
When Are While Loops In C++ Useful?
As discussed above, the basic use of a while loop is to execute a body of statements repeatedly.
- They are particularly useful when we do not know the number of iterations beforehand.
- A while loop is used to iterate through an array or a list. We can sort the array, process numbers in the array, or update an array as per our needs.
- A while loop can also be used to iterate through a vector. As we reach the end of the vector, the loop terminates.
- A while loop can also be used to do a pre-checking process before allowing the program to continue.
- A real-world example of a while loop can be seen in video games. The loop continues until the player reaches a certain score or completes a task.
Use Cases:
We can use a while loop to perform some complex algorithms. This includes finding the reverse of a number, printing the first n numbers of the Fibonacci series, checking if a number is palindrome or not, counting the number of digits in a number, and the use of nested while loop.
We will cover the code and explanation to find the reverse of a number for a better understanding of the use cases of a while loop.
Example C++ While Loop Program
The C++ program below reverses a number using the While Loop.
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgbiA9IDc4OTsKCmludCByZXZlcnNlID0gMDsKCmludCBkPTA7Cgpjb3V0IDw8ICJOdW1iZXIgaXM6ICIgPDwgbjsKCndoaWxlIChuID4gMCl7CgpkPSBuJTEwOwoKcmV2ZXJzZSA9IHJldmVyc2UgKiAxMCArIGQ7CgpuID0gbiAvIDEwO30KCmNvdXQgPDwgIlxuUmV2ZXJzZSBpczogIiA8PCByZXZlcnNlO30=
Output:
Number is 789
Reverse is 987
Explanation:
- In the above lines of code, we declare and initialize an integer variable n with the value of 789.
- We declare two more variables reverse and d, and initialize them with the value 0. The reverse variable will store the reverse of n.
- The cout statement is used to print the number to the console. We then use the while keyword to start the while loop in C++ program.
- The test condition is satisfied (789>0), and the code inside the loop is executed. This way, the reverse becomes 9 (reverse = reverse * 10 + d) in the first iteration. The value of n then becomes 79 (n=n/10).
- After this, the condition(78>0) is checked again, and the loop is executed. Here, the reverse becomes 98 in the second iteration, and the value of n is now 9.
- The condition is checked again (9>0), and the value of reverse finally becomes 987. The value of n is 0 at the end of the third iteration.
- In the next iteration, the condition (0>0) becomes false, and the statements outside the body of the loop get executed.
- The cout statement then prints the reverse of the number, and the program is completed.
What Are Nested While Loops In C++?
As per the name, a loop inside a loop is known as a nested loop. There can be any number of nested loops as per the requirements of the program/ problem at hand. The working of nested loops in C++ is similar to any other form of nested loops. It is important to note that in these sorts of loops when the inner loop terminates, the value of the outer loop is incremented.
Syntax:
while(test condition 1)
{
while(test condition 2)
{
// inner loop statement
}
// outer loop statement
}
Here,
- The while keywords begin the while loop (both inner and outer).
- Test condition 1 is the condition for the outer while loop in C++, which, when true, passes the control to the inner loop.
- The test condition 2 is the condition for the inner while loop.
- The inner loop statement refers to the code block that executes when condition 2 is true.
- The outer loop statement refers to the code block that executes when the inner while loop condition turns false, and control is passed outside.
Execution Flow Controls of Nested While Loop
The working mechanism of the nested while loop is as follows:
- In the case of nested loops, we can have as many while loops as the situation requires.
- Say we have 2 loops with one nested inside the other. The outer while loop executes based on test condition 1, and the inner while loop executes based on test condition 2.
- Here, we have the first while() loop, which has test condition 1. If this condition is false, then the entire loop terminates.
- If condition 1 is true, the control goes to the second while() loop, where we have test condition 2.
- If condition 2 is true, the statement in the inner loop executes. After that, the value is updated.
- The condition is checked again, and this continues for the inner while loop until the condition becomes false.
- After the condition is false, the value is updated for the outer while loop. Then again, if condition 1 is true, we go to the inner loop.
- This continues until the condition in both the inner loop and the outer loop becomes false.
- Once that happens, the loop terminates, and the control comes out.
The flow chart below is a visual representation of the working mechanism of the while loop in C++.
C++ Nested While Loop Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgbiA9IDU7CgppbnQgaSA9IDEsIGo9MTsKCndoaWxlIChpIDw9IDUpIHsKCndoaWxlIChqIDw9IGkpIHsKCmNvdXQgPDwgaiA8PCAiICI7CgpqKys7fQoKY291dCA8PCAiXG4iOwoKaSsrO30KCn0=
Output:
1
2
3
4
5
Explanation:
- In the above code snippet, we initialize a variable n as 5 and control variable i and j as 1.
- The outer loop condition is checked (1<=5), and the control goes to the inner loop where the condition (1<=1) is checked.
- The second condition is also true, and the value of j, which is 1, is printed with the use of the cout statement. Simultaneously, the value of j is incremented to 2.
- The inner loop condition is checked again (2<=1), which is false, and then the statement after this loop is executed, and i is incremented to 2.
- The outer loop condition is checked again, and the process continues until both the inner loop and the outer loop become false, and control comes out.
Infinite While Loop In C++
It is clear that an infinite loop is one that never ends. So, an infinite while loop in C++ is one which runs indefinitely until exited explicitly. There are two most common cases that these occur. One is when the test expression always holds true. And the other is when the update expression in the loop is either wrong or missing altogether.
In either case, the test expression always comes back to be true, and the execution of the loop continues till infinity, i.e., it results in an endless loop.
The syntax for Infinite While C++ Loop:
while (test expression) {
// statements or body of the loop
wrong update expression or no update expression;
}
Here,
- The while keyword marks the beginning of the loop.
- The statements or body of the loop refers to the code that gets executed if the test expression is true.
- Note that the updation expression here is wrong or missing.
We check the test expression to start the while() loop since it is an entry-controlled loop. The result of this expression is a boolean, i.e., either true or false.
- If the expression is true, the statements inside the loop are executed.
- After that, ideally, the flow moves to the update expression before moving to the next iteration.
- But in this case, the update expression is missing or wrong; hence the loop becomes an infinite loop.
- Since the condition never becomes wrong, the loop continues forever and never terminates.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgaSA9IDE7Cgp3aGlsZSAoaSA8IDUpewoKY291dCA8PCAiVW5zdG9wIGlzIGZ1biFcbiI7fQoKcmV0dXJuIDA7fQ==
Output:
Unstop is fun!
Unstop is fun!
Unstop is fun!
.... //Infinite times
Explanation:
- In the above code snippet, we initialize a variable i as 1. We then make use of a while loop and the cout statement to print the phrase 'Unstop is fun!'.
- A test expression(i<5) is checked, and the control moves to the first statement.
- Here, the phrase gets printed for the first time.
- There is no update statement, and the value of i remains 1. Hence the condition is always true, and the statement is executed for the second time.
- This continues infinitely since the condition is always true. Also, the \n specifier ensures that every time the phrase is printed in a new line.
Alternatives To While Loop In C++
While loops are used when the number of terminations is unknown. But there are times when we need to know the number of iterations to avoid situations like an infinite loop. In such cases, we make use of alternatives of a while loop, some of which are discussed ahead.
If Statement In C++
We can make use of a simple if statement to replace a while loop. For this, we need to write a nested if statement and keep updating the control variable.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgeCA9IDA7CgppZiAoeCA8IDIpIHsKCmNvdXQgPDwgeCA8PCAiICI7Cgp4Kys7CgppZiAoeCA8IDIpIHsKCmNvdXQgPDwgeCA8PCAiICI7Cgp4Kys7fX0KCnJldHVybiAwO30=
Output:
0 1
Explanation:
- In the above code snippet, we initialize a variable x as 0.
- The keyword if starts the statement, and its condition (x<2) is checked. Since the condition is true, the cout statement prints the value of x that is 0.
- The value of i will now increment to 1, and the condition (x<2) is checked again. Since it is true, the value of x that is 1 is printed.
- The value of x is then incremented to 2, and this time the condition becomes false.
- The loop terminates, and the control comes out of the loop.
Switch Statement In C++
A switch statement can also be used instead of a while loop. In this statement, different cases are written, and when the variable value matches one of these cases, the code corresponding to it is executed. One drawback of the switch is we will have to write all the possible cases that can match, which makes the code lengthy.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgeCA9IDI7Cgpzd2l0Y2ggKHgpIHsKCmNhc2UgMTogY291dCA8PCAiT3B0aW9uIDEiOwoKYnJlYWs7CgpjYXNlIDI6IGNvdXQgPDwgIk9wdGlvbiAyIjsKCmJyZWFrOwoKZGVmYXVsdDogY291dCA8PCAiTm8gb3B0aW9ucyI7CgpicmVhazt9CgpyZXR1cm4gMDt9
Output:
Option 2
Explanation:
- In the above code snippet, we declare an integer variable x and initialize it with the value 2.
- Using the switch case statement, the value of x is matched with the expression of the switch case.
- Case 1 is false, so the control goes to case 2.
- When case 2 matches with the value 2, the statement Option 2 gets printed as output using the cout statement.
- After that, we break out of the loop, and the control comes outside.
Do-While Loop In C++
A do-while loop is similar to the while loop with one major difference. It is an exit-controlled loop in which the condition is checked after executing the body of the loop, unlike the while loop, which is an entry-controlled loop. This can be used as an alternative to a while loop as well.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgeCA9IDk7CgpkbyB7Cgpjb3V0IDw8IHggPDwgIlxuIjsKCngrKzsKCn0gd2hpbGUgKHggPCAxMCk7CgpyZXR1cm4gMDt9
Output:
9
Explanation:
- In the above code snippet, the integer variable x is initialized to 9.
- Since it is a do-while loop, the statement inside is executed first, and the value of x gets printed with the cout statement.
- The value of x is then incremented to 10 as per our update expression (i++).
- The control then moves to the condition(x<10), which is false, and hence the loop terminates.
For Loop In C++
Any while loop in C++ can be written using a for loop as well. This is the easiest way to implement a while loop. The output of a while loop and a for loop is exactly the same since both of them are entry-controlled loops.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7Cgpmb3IoaW50IHg9MDsgeDw9MzsgeCsrKXsKCmNvdXQ8PCB4IDw8ZW5kbDt9CgpyZXR1cm4gMDt9
Output:
0
1
2
3
Explanation:
- In the above code snippet, we initialize a variable x as 0.
- As per the for loop syntax, the condition (x<3) is checked, and the value of x that is 0 is printed.
- The update expression then increments the value of x to 1, and the condition is checked again.
- The new value of x, which is 1, is printed. Now, x is incremented to 2, and the condition is checked again.
- This continues until the condition becomes false (when x=4>3), and the control comes out.
Conclusion
In this article, we conducted a detailed discussion on the while loop in C++.
- The syntax of the while loop contains the while keyword, a test expression, a loop statement, and updating expression.
- We also described the flow of control through the while loop, infinite while loop, and nested while loop.
It is evident that C++ allows for both nested as well as infinite while loops. Some alternatives to the while loop in C++ are the if-statement, the switch case, and the other loop types.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What are the 3 types of common loops?
The three different types of loops provided in C++ are:
- While loop
- Do-while loop
- For loop
They are further classified into an entry-controlled loop and an exit-controlled loop. While loop and for loop are entry control loops, whereas a do-while loop is an exit control loop.
Q. What is the syntax of the while loop?
The syntax of the while loop is mentioned below:
while (test expression)
{
// statements or body of loop
update expression;
}
Here,
- The while keyword initiates the loop.
- Test condition is what determines if the flow will pass to the body or will exit the loop altogether.
- The updation expression updates the value of the loop variable before moving to the next iteration.
Q. What is the output of an infinite loop?
An infinite loop is a loop that continues to execute indefinitely without terminating. It occurs when the loop condition always evaluates to true, or there is no exit condition specified within the loop. The behavior of an infinite loop depends on the programming language and the environment in which it is running. But in any case, the output of the infinite loop will be a recurring result of the code in the loop body.
If you run an infinite loop, it will cause the program to become unresponsive or stuck in an infinite loop state. The program will keep executing the code within the loop repeatedly without ever exiting or until the memory allocated runs out. This can lead to high CPU usage and consume system resources, potentially causing the program or the entire system to freeze or crash.
The syntax of the infinite loop in C++:
while (true) {
// Code block that keeps executing indefinitely
}
In this example, the condition true is always true, so the loop will continue executing endlessly.
Q. Which loop is a while loop? Explain with an example.
While loop is an entry-controlled loop in which the condition is checked first before executing the body of statements. The statements are executed only if the condition is true, and the control comes out when the condition becomes false. In C++, for-loop and while-loop are examples of entry-controlled loops. In such loops, the test expression is checked first, and then the loop body is executed. The loop runs until the condition is true, and the statements inside the body of the loop are executed. The loop terminates as soon as the condition is unsatisfied.
Here is the block of code to understand the same:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgaSA9IDE7Cgp3aGlsZSAoaSA8IDMpewoKY291dCA8PCAiV2hpbGUgbG9vcCBpbiBDKytcbiI7CgppKys7fQoKcmV0dXJuIDA7fQ==
Output:
While loop in C++
While loop in C++
Q. How does a while loop start?
A while loop in most programming languages, including C++, starts by evaluating a condition. If the condition evaluates to true, the code inside the loop is executed. After executing the code block, the condition is checked again. If the condition is still true, the loop iterates and executes the code block again.
This process continues until the condition evaluates to false, at which point the loop terminates, and program execution continues with the code following the loop.
Q. How do you exit a while loop?
A while loop terminates when the test condition becomes false, and the control flows to the next part of the program outside the loop. However, we can make use of conditional statements to terminate the loop. These statements are break, continue, and goto for unconditional transfer of program control from one part of the program to the other. The most widely used is the break statement to come out of the inner loop directly. The break statement allows you to terminate the loop prematurely and continue executing the code after the loop.
Here's a code snippet showcasing the use of break to exit a while loop:
while (condition) {
// Code block inside the loop
if (some_condition) {
break; // Exit the loop if the condition is met
}
// More code inside the loop
}
// Code after the loop
Q. What is the difference between a do-while loop and a while loop in C++?
In a while loop, the condition is checked first, and then the statements are executed since it is an entry-controlled loop. It may be possible in some cases that none of the statements are executed since the condition becomes false and the loop termination occurs. All the variables are initialized before the process of execution of the loop in case of a while loop. Syntax of the while loop is:
while (test expression)
{
// block of statements or body of the loop
update expression;
}
In the case of a do-while loop, a single statement is executed first, and then the condition is checked since it is an exit-controlled loop. Therefore, in this case, even if the condition is wrong, statements will be executed during the loop declaration. Loop variables may be initialized anywhere before or within the loop in case of do while loop. Syntax of the do-while loop in C++ is:
do
{
// statement(s);
}
while(condition);
This compiles the discussion on the while loop in C++. You might also be interested in reading the following:
- Operators In C++ | Types & Precedence Explained (With Examples)
- Comment In C++ | Types, Usage, C-Style Comments & More (+Examples)
- Storage Classes In C++ & Its Types Explained (With Examples)
- Pointers in C++ | A Roadmap To All Types Of Pointers With Examples
- Strings In C++ | Functions, How To Convert & More (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment