Table of content:
- A Brief Intro To C++
- The Timeline Of C++
- Importance Of C++
- Versions Of C++ Language
- Comparison With Other Popular Programming Languages
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Variables In C++?
- Declaration & Definition Of Variables In C++
- Variable Initialization In C++
- Rules & Regulations For Naming Variables In C++ Language
- Different Types Of Variables In C++
- Different Types of Variable Initialization In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Primitive Data Types In C++?
- Derived Data Types In C++
- User-Defined Data Types In C++
- Abstract Data Types In C++
- Data Type Modifiers In C++
- Declaring Variables With Auto Keyword
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Structure Of C++ Program: Components
- Compilation & Execution Of C++ Programs | Step-by-Step Explanation
- Structure Of C++ Program With Example
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What is Typedef in C++?
- The Role & Applications of Typedef in C++
- Basic Syntax for typedef in C++
- How Does typedef Work in C++?
- How to Use Typedef in C++ With Examples? (Multiple Data Types)
- The Difference Between #define & Typedef in C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Strings In C++?
- Types Of Strings In C++
- How To Declare & Initialize C-Style Strings In C++ Programs?
- How To Declare & Initialize Strings In C++ Using String Keyword?
- List Of String Functions In C++
- Operations On Strings Using String Functions In C++
- Concatenation Of Strings In C++
- How To Convert Int To Strings In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is String Concatenation In C++?
- How To Concatenate Two Strings In C++ Using The ‘+' Operator?
- String Concatenation Using The strcat( ) Function
- Concatenation Of Two Strings In C++ Using Loops
- String Concatenation Using The append() Function
- C++ String Concatenation Using The Inheritance Of Class
- Concatenate Two Strings In C++ With The Friend and strcat() Functions
- Why Do We Need To Concatenate Two Strings?
- How To Reverse Concatenation Of Strings In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Find In String C++?
- What Is A Substring?
- How To Find A Substring In A String In C++?
- How To Find A Character In String C++?
- Find All Substrings From A Given String In C++
- Index Substring In String In C++ From A Specific Start To A Specific Length
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Pointers In C++?
- Pointer Declaration In C++
- How To Initialize And Use Pointers In C++?
- Different Types Of Pointers In C++
- References & Pointers In C++
- Arrays And Pointers In C++
- String Literals & Pointers In C++
- Pointers To Pointers In C++ (Double Pointers)
- Arithmetic Operation On Pointers In C++
- Advantages Of Pointers In C++
- Some Common Mistakes To Avoid With Pointers In Cpp
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Understanding Pointers In C++
- What Is Pointer To Object In C++?
- Declaration And Use Of Object Pointers In C++
- Advantages Of Pointer To Object In C++
- Pointer To Objects In C++ With Arrow Operator
- An Array Of Objects Using Pointers In C++
- Base Class Pointer For Derived Class Object In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is 'This' Pointer In C++?
- Defining ‘this’ Pointer In C++
- Example Of 'this' Pointer In C++
- Describing The Constness Of 'this' Pointer In C++
- Important Uses Of 'this' Pointer In C++
- Method Chaining Using 'this' Pointer In C++
- C++ Programs To Show Application Of 'This' Pointer
- How To Delete The ‘this’ Pointer In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What is Reference?
- What is Pointer?
- Comparison Table Of C++ Pointer Vs. Reference
- Differences Between Reference And Pointer: A Detailed Explanation
- Why Are References Less Powerful Than Pointers?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- How To Declare A 2D Array In C++?
- C++ Multi-Dimensional Arrays
- Ways To Initialize A 2D Array In C++
- Methods To Dynamically Allocate A 2D Array In C++
- Accessing/ Referencing Two-Dimensional Array Elements
- How To Initialize A Two-Dimensional Integer Array In C++?
- How To Initialize A Two-Dimensional Character Array?
- How To Enter Data In Two-Dimensional Array In C++?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Arrays Of Strings In C++?
- Different Ways To Create String Arrays In C++
- How To Access The Elements Of A String Array In C++?
- How To Convert Char Array To String?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Memory Allocation in C++?
- The “new" Operator In C++
- The "delete" Operator In C++
- Dynamic Memory Allocation In C++ | Arrays
- Dynamic Memory Allocation In C++ | Objects
- Deallocation Of Dynamic Memory
- Dynamic Memory Allocation In C++ | Uses
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A Substring In C++ (Substr C++)?
- Example For Substr In C++
- Points To Remember For Substr In C++
- Important Applications Of substr() Function
- How to Get a Substring Before a Character?
- Print All Substrings Of A Given String
- Print Sum Of All Substrings Of A String Representing A Number
- Print Minimum Value Of All Substrings Of A String Representing A Number
- Print Maximum Value Of All Substrings Of A String Representing A Number
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Operator In C++?
- Types Of Operators In C++ With Examples
- What Are Arithmetic Operators In C++?
- What Are Assignment Operators In C++?
- What Are Relational Operators In C++?
- What Are Logical Operators In C++?
- What Are Bitwise Operators In C++?
- What Is Ternary/ Conditional Operator In C++?
- Miscellaneous Operators In C++
- Precedence & Associativity Of Operators In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is The New Operator In C++?
- Example To Understand New Operator In C++
- The Grammar Elements Of The New Operator In C++
- Storage Space Allocation
- How Does The C++ New Operator Works?
- What Happens When Enough Memory In The Program Is Not Available?
- Initializing Objects Allocated With New Operator In C++
- Lifetime Of Objects Allocated With The New Operator In C++
- What Is The Delete Operator In C++?
- Difference Between New And Delete Operator In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Types Of Overloading In C++
- What Is Operator Overloading In C++?
- How To Overload An Operator In C++?
- Overloadable & Non-overloadable Operators In C++
- Unary Operator Overloading In C++
- Binary Operator Overloading In C++
- Special Operator Overloading In C++
- Rules For Operator Overloading In C++
- Advantages And Disadvantages Of Operator Overloading In C++
- Function Overloading In C++
- What Is the Difference Between Operator Functions and Normal Functions?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Operators In C++?
- Introduction To Logical Operators In C++
- Types Of Logical Operators In C++ With Example Program
- Logical AND (&&) Operator In C++
- Logical NOT(!) Operator In C++
- Logical Operator Precedence And Associativity In C++
- Relation Between Conditional Statements And Logical Operators In C++
- C++ Relational Operators
- Conclusion
- Frequently Asked Important Interview Questions:
- Test Your Skills: Quiz Time
Table of content:
- Different Type Of C++ Bitwise Operators
- C++ Bitwise AND Operator
- C++ Bitwise OR Operator
- C++ Bitwise XOR Operator
- Bitwise Left Shift Operator In C++
- Bitwise Right Shift Operator In C++
- Bitwise NOT Operator
- What Is The Meaning Of Set Bit In C++?
- What Does Clear Bit Mean?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Types of Comments in C++
- Single Line Comment In C++
- Multi-Line Comment In C++
- How Do Compilers Process Comments In C++?
- C- Style Comments In C++
- How To Use Comment In C++ For Debugging Purposes?
- When To Use Comments While Writing Codes?
- Why Do We Use Comments In Codes?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Storage Classes In Cpp?
- What Is The Scope Of Variables?
- What Are Lifetime And Visibility Of Variables In C++?
- Types of Storage Classes in C++
- Automatic Storage Class In C++
- Register Storage Class In C++
- Static Storage Class In C++
- External Storage Class In C++
- Mutable Storage Class In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Decision Making Statements In C++
- Types Of Conditional Statements In C++
- If-Else Statement In C++
- If-Else-If Ladder Statement In C++
- Nested If Statements In C++
- Alternatives To Conditional If-Else In C++
- Switch Case Statement In C++
- Jump Statements & If-Else In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A Switch Statement/ Switch Case In C++?
- Rules Of Switch Case In C++
- How Does Switch Case In C++ Work?
- The break Keyword In Switch Case C++
- The default Keyword In C++ Switch Case
- Switch Case Without Break And Default
- Advantages & Disadvantages of C++ Switch Case
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A For Loop In C++?
- Syntax Of For Loop In C++
- How Does A For Loop In C++ Work?
- Examples Of For Loop Program In C++
- Ranged Based For Loop In C++
- Nested For Loop In C++
- Infinite For Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A While Loop In C++?
- Parts Of The While Loop In C++
- C++ While Loop Program Example
- How Does A While Loop In C++ Work?
- What Is Pre-checking Process Or Entry-controlled Loop?
- When Are While Loops In C++ Useful?
- Example C++ While Loop Program
- What Are Nested While Loops In C++?
- Infinite While Loop In C++
- Alternatives To While Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Loops & Its Types In C++?
- What Is A Do-While Loop In C++?
- Do-While Loop Example In C++ To Print Numbers
- How Does A Do-While Loop In C++ Work?
- Various Components Of The Do-While Loop In C++
- Example 2: Adding User-Input Positive Numbers With Do-While Loop
- C++ Nested Do-While Loop
- C++ Infinitive Do-while Loop
- What is the Difference Between While Loop and Do While Loop in C++?
- When To Use A Do-While Loop?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are 2D Vectors In C++?
- How To Declare 2D Vector In C++?
- How To Initialize 2D Vector In C++?
- C++ Program Examples For 2D Vectors
- How To Access & Modify 2D Vector Elements In C++?
- Methods To Traverse, Manipulate & Print 2D Vectors In C++
- Adding Elements To 2-D Vector Using push_back() Function
- Removing Elements From Vector In C++ Using pop_back() Function
- Creating 2D Vector In C++ With User Input For Size Of Column & Row
- Advantages of 2D Vectors Over Traditional Arrays
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- How To Print A Vector In C++ By Overloading Left Shift (<<) Operator?
- How To Print Vector In C++ Using Range-Based For-Loop?
- Print Vector In C++ With Comma Separator
- Printing Vector In C++ Using Indices (Square Brackets/ Double Brackets & at() Function)
- How To Print A Vector In C++ Using std::copy?
- How To Print A Vector In C++ Using for_each() Function?
- Printing C++ Vector Using The Lambda Function
- How To Print Vector In C++ Using Iterators?
- Conclusion
- Frequently Asked Questions
Table of content:
- Definition Of C++ Find In Vector
- Using The std::find() Function
- How Does find() In Vector C++ Function Work?
- Finding An Element By Custom Comparator Using std::find_if() Function
- Use std::find_if() With std::distance()
- Element Find In Vector C++ Using For Loop
- Using The find_if_not Function
- Find Elements With The Linear Search Approach
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Sort() Function In C++?
- Sort() Function In C++ From Standard Template Library
- Exceptions Of Sort() Function/ Algorithm In C++
- The Stable Sort() Function In C++
- Partial Sort() Function In C++
- Sorting In Ascending Order With Sort() Function In C++
- Sorting In Descending Order With Sort Function In C++
- Sorting In Desired Order With Custom Comparator Function & Sort Function In C++
- Sorting Elements In Desired Order Using Lambda Expression & Sort Function In C++
- Types of Sorting Algorithms In C++
- Advanced Sorting Algorithms In C++
- How Does the Sort() Function Algorithm Work In C++?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Function Overloading In C++?
- Ways Of Function Overloading In C++
- Function Overloading In C++ Using Different Types Of Parameters
- Function Overloading In C++ With Different Number Of Parameters
- Function Overloading In C++ Using Different Sequence Of Parameters
- How Does Function Overloading In C++ Work?
- Rules Of Function Overloading In C++
- Why Is Function Overloading Used?
- Types Of Function Overloading Based On Time Of Resolution
- Causes Of Function Overloading In C++
- Ambiguity & Function Overloading In C++
- Advantages Of Function Overloading In C++
- Disadvantages Of Function Overloading In C++
- Operator Overloading In C++
- Function Overriding In C++
- Difference Between Function Overriding & Function Overloading In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is An Inline Function In C++?
- How To Define The Inline Function In C++?
- How Does Inline Function In C++ Work?
- The Need For An Inline Function In C++
- Can The Compiler Ignore/ Reject Inline Function In C++ Programs?
- Normal Function Vs. Inline Function In C++
- Classes & Inline Function In C++
- Understanding Inline, __inline, And __forceinline Functions In C++
- When To Use An Inline Function In C++?
- Advantages Of Inline Function In C++
- Disadvantages Of Inline Function In C++
- Why Not Use Macros Instead Of An Inline Function In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Static Data Member In C++?
- How To Declare Static Data Members In C++?
- How To Initialize/ Define Static Data Member In C++?
- Ways To Access A Static Data Member In C++
- What Are Static Member Functions In C++?
- Example Of Member Function & Static Data Member In C++
- Practical Applications Of Static Data Member In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constant In C++?
- Ways To Define Constant In C++
- What Are Literals In C++?
- Pointer To A Constant In C++
- Constant Function Arguments In C++
- Constant Member Function Of Class In C++
- Constant Data Members In C++
- Object Constant In C++
- Conclusion
- Frequently Asked Questions(FAQ)
Table of content:
- What Is Friend Function In C++?
- Declaration Of Friend Function In C++ With Example
- Characteristics Of Friend Function In C++
- Global Friend Function In C++ (Global Function As Friend Function )
- Member Function Of Another Class As Friend Function In C++
- Function Overloading Using Friend Function In C++
- Advantages & Disadvantages Of Friend Function in C++
- What Is A C++ Friend Class?
- A Function Friendly To Multiple Classes
- C++ Friend Class Vs. Friend Function In C++
- Some Important Points About Friend Functions And Classes In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Function Overriding In C++?
- The Working Mechanism Of Function Overriding In C++
- Real-Life Example Of Function Overriding In C++
- Accessing Overriding Function In C++
- Accessing Overridden Function In C++
- Function Call Binding With Class Objects | Function Overriding In C++
- Function Call Binding With Base Class Pointers | Function Overriding In C++
- Advantages Of Function Overriding In C++
- Variations In Function Overriding In C++
- Function Overloading In C++
- Function Overloading Vs Function Overriding In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Errors In C++
- What Is Exception Handling In C++?
- Exception Handling In C++ Program Example
- C++ Exception Handling: Basic Keywords
- The Need For C++ Exception Handling
- C++ Standard Exceptions
- C++ Exception Classes
- User-Defined Exceptions In C++
- Advantages & Disadvantages Of C++ Exception Handling
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Templates In C++ & How Do They Work?
- Types Of Templates In C++
- What Are Function Templates In C++?
- C++ Template Functions With Multiple Parameters
- C++ Template Function Overloading
- What Are Class Templates In C++?
- Defining A Class Member Outside C++ Template Class
- C++ Template Class With Multiple Parameters
- What Is C++ Template Specialization?
- How To Specify Default Arguments For Templates In C++?
- Advantages Of C++ Templates
- Disadvantages Of C++ Templates
- Difference Between Function Overloading And Templates In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Structure
- Structure Declaration
- Initialization of Structure
- Copying and Comparing Structures
- Array of Structures
- Nested Structures
- Pointer to a Structure
- Structure as Function Argument
- Self Referential Structures
- Class
- Object Declaration
- Accessing Class Members
- Similarities between Structure and Class
- Which One Should You Choose?
- Key Difference Between a Structure and Class
- Summing Up
- Test Your Skills: Quiz Time
Table of content:
- What Is A Class And Object In C++?
- What Is An Object In C++?
- How To Create A Class & Object In C++? With Example
- Access Modifiers & Class/ Object In C++
- Member Functions Of A Class In C++
- How To Access Data Members And Member Functions?
- Significance Of Class & Object In C++
- What Are Constructors In C++ & Its Types?
- What Is A Destructor Of Class In C++?
- An Array Of Objects In C++
- Object In C++ As Function Arguments
- The this (->) Pointer & Classes In C++
- The Need For Semicolons At The End Of A Class In C++
- Difference Between Structure & Class In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Static Members In C++?
- Static Member Functions in C++
- Ways To Call Static Member Function In C++
- Properties Of Static Member Function In C++
- Need Of Static Member Functions In C++
- Regular Member Function Vs. Static Member Function In C++
- Limitations Of Static Member Functions In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Constructor In C++?
- Characteristics Of A Constructor In C++
- Types Of Constructors In C++
- Default Constructor In C++
- Parameterized Constructor In C++
- Copy Constructor In C++
- Dynamic Constructor In C++
- Benefits Of Using Constructor In C++
- How Does Constructor In C++ Differ From Normal Member Function?
- Constructor Overloading In C++
- Constructor For Array Of Objects In C++
- Constructor In C++ With Default Arguments
- Initializer List For Constructor In C++
- Dynamic Initialization Using Constructor In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constructor In C++?
- What Is Constructor Overloading In C++?
- Dеclaration Of Constructor Ovеrloading In C++
- Condition For Constructor Overloading In C++
- How Constructor Ovеrloading In C++ Works?
- Examples Of Constructor Overloading In C++
- Lеgal & Illеgal Constructor Ovеrloading In C++
- Types Of Constructors In C++
- Characteristics Of Constructors In C++
- Advantage Of Constructor Overloading In C++
- Disadvantage Of Constructor Overloading In C++
- Conclusion
- Frеquеntly Askеd Quеstions
Table of content:
- What Is A Destructor In C++?
- Rules For Defining A Destructor In C++
- When Is A Destructor in C++ Called?
- Order Of Destruction In C++
- Default Destructor & User-Defined Destructor In C++
- Virtual Destructor In C++
- Pure Virtual Destructor In C++
- Key Properties Of Destructor In C++ You Must Know
- Explicit Destructor Calls In C++
- Destructor Overloading In C++
- Difference Between Normal Member Function & Destructor In C++
- Important Uses Of Destructor In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constructor In C++?
- What Is A Destructor In C++?
- Difference Between Constructor And Destructor In C++
- Constructor In C++ | A Brief Explanation
- Destructor In C++ | A Brief Explanation
- Difference Between Constructor And Destructor In C++ Explained
- Order Of Calling Constructor And Destructor In C++ Classes
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Type Conversion In C++?
- What Is Type Casting In C++?
- Types Of Type Conversion In C++
- Implicit Type Conversion (Coercion) In C++
- Explicit Type Conversion (Casting) In C++
- Advantages Of Type Conversion In C++
- Disadvantages Of Type Conversion In C++
- Difference Between Type Casting & Type Conversion In C++
- Application Of Type Casting In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Copy Constructor In C++?
- Characteristics Of Copy Constructors In C++
- Types Of Copy Constructors In C++
- When Do We Call The Copy Constructor In C++?
- When Is A User-Defined Copy Constructor Needed In C++?
- Types Of Constructor Copies In C++
- Can We Make The Copy Constructor In C++ Private?
- Assignment Operator Vs Copy Constructor In C++
- Example Of Class Where A Copy Constructor Is Essential
- Uses Of Copy Constructors In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do You Need Object-Oriented Programming (OOP) In C++?
- OOPs Concepts In C++ With Examples
- The Class OOPs Concept In C++
- The Object OOPs Concept In C++
- The Inheritance OOPs Concept In C++
- Polymorphism OOPs Concept In C++
- Abstraction OOPs Concept In C++
- Encapsulation OOPs Concept In C++
- Other Features Of OOPs In C++
- Benefits Of OOP In C++ Over Procedural-Oriented Programming
- Disadvantages Of OOPS Concept In C++
- Why Is C++ A Partial OOP Language?
- Conclusion
- Frequently Asked Questions
Table of content:
- Introduction To Abstraction In C++
- Types Of Abstraction In C++
- What Is Data Abstraction In C++?
- Understanding Data Abstraction In C++ Using Real Life Example
- Ways Of Achieving Data Abstraction In C++
- What Is An Abstract Class?
- Advantages Of Data Abstraction In C++
- Use Cases Of Data Abstraction In C++
- Encapsulation Vs. Abstraction In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Encapsulation In C++?
- How Does Encapsulation Work In C++?
- Types Of Encapsulation In C++
- Why Do We Need Encapsulation In C++?
- Implementation Of Encapsulation In C++
- Access Specifiers & Encapsulation In C++
- Role Of Access Specifiers In Encapsulation In C++
- Member Functions & Encapsulation In C++
- Data Hiding & Encapsulation In C++
- Features Of Encapsulation In C++
- Advantages & Disadvantages Of Encapsulation In C++
- Difference Between Abstraction and Encapsulation In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Inheritance In C++?
- What Are Child And Parent Classes?
- Syntax And Structure Of Inheritance In C++
- Implementing Inheritance In C++
- Importance Of Inheritance In C++
- Types Of Inheritance In C++
- Visibility Modes Of Inheritance In C++
- Access Modifiers & Inheritance In C++
- How To Make A Private Member Inheritable?
- Member Function Overriding In Inheritance In C++
- The Diamond Problem | Inheritance In C++ & Ambiguity
- Ways To Avoid Ambiguity Inheritance In C++
- Why & When To Use Inheritance In C++?
- Advantages Of Inheritance In C++
- The Disadvantages Of Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Hybrid Inheritance In C++?
- Importance Of Hybrid Inheritance In Object Oriented Programming
- Example Of Hybrid Inheritance In C++: Using Single and Multiple Inheritance
- Example Of Hybrid Inheritance In C++: Using Multilevel and Hierarchical Inheritance
- Real-World Applications Of Hybrid Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Multiple Inheritance In C++?
- Examples Of Multiple Inheritance In C++
- Ambiguity Problem In Multiple Inheritance In C++
- Ambiguity Resolution In Multiple Inheritance In C++
- The Diamond Problem In Multiple Inheritance In C++
- Visibility Modes In Multiple Inheritance In C++
- Advantages & Disadvantages Of Multiple Inheritance In C++
- Multiple Inheritance Vs. Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Multilevel Inheritance In C++?
- Block Diagram For Multilevel Inheritance In C++
- Multilevel Inheritance In C++ Example
- Constructor & Multilevel Inheritance In C++
- Use Cases Of Multilevel Inheritance In C++
- Multiple Vs Multilevel Inheritance In C++
- Advantages & Disadvantages Of Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Hierarchical Inheritance In C++?
- Example 1: Hierarchical Inheritance In C++
- Example 2: Hierarchical Inheritance In C++
- Impact of Visibility Modes In Hierarchical Inheritance In C++
- Advantages And Disadvantages Of Hierarchical Inheritance In C++
- Use Cases Of Hierarchical Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Access Specifiers In C++?
- Types Of Access Specifiers In C++
- Public Access Specifiers In C++
- Private Access Specifier In C++
- Protected Access Specifier In C++
- The Need For Access Specifiers In C++
- Combined Example For All Access Specifiers In C++
- Best Practices For Using Access Specifiers In C++
- Why Can't Private Members Be Accessed From Outside A Class?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Diamond Problem In C++?
- Example Of The Diamond Problem In C++
- Resolution Of The Diamond Problem In C++
- Virtual Inheritance To Resolve Diamond Problem In C++
- Scope Resolution Operator To Resolve Diamond Problem In C++
- Conclusion
- Frequently Asked Questions
Strings In C++ | Create, Manipulate, Functions & More (+Examples)
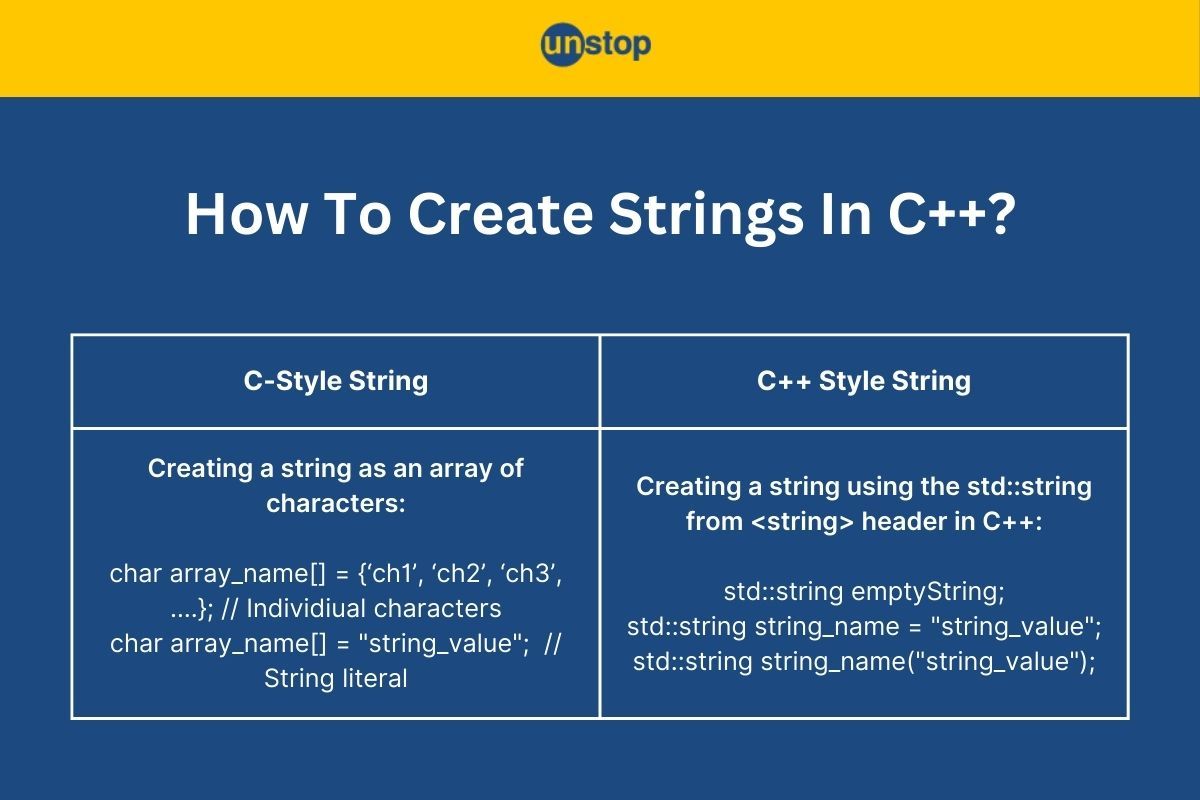
In everyday life, we often think of a string as a piece of thread or fiber. In molecular biology, it might represent a database of protein interactions. Clearly, the term “string” holds various meanings. But what does it mean in programming?
In programming languages like C++, strings refer to a sequence of characters. They are commonly used to store words (i.e., text) or characters (i.e., letters or numbers). In this article, we will discuss everything you need to know about strings, string functions in C++, how to convert integers to strings, and more.
What Are Strings In C++?
As we've mentioned, strings in C++ are used to store textual data made up of a sequence of characters.
- A string variable is a group of characters (including special characters and whitespaces) enclosed in single or double quotation marks.
- For instance, “UNSTOP” and ‘Unstoppable’ are both examples of strings in cpp.
- There are two ways to create a string in C++ programs: by using the C-style strings (character array) and the C++ string using the header file <string> (which offers more functionality and ease).
- The <string> header is also essential when using string functions to manipulate strings,
Note that including the <string> header is essential for working with C++ string functions, allowing us to manipulate strings efficiently.
For Example:
std::string str_name = “ This is the Unstop Blog” ;
Here, we use the string keyword (from <string> header> to indicate that the type of the variable is of type string, followed by the identifier/ name of the variable and the value. We will discuss the declaration process for strings in C++ in the section ahead.
Types Of Strings In C++
As mentioned, strings in C++ language can be represented in two ways:
- C-Style Strings: These are character arrays used to represent strings. This method comes from C programming and requires manual handling of the string's termination using a null character (\0).
- C++ String Class: By using the std::string class, available through the <string> header, you gain access to many powerful string-handling functions that make working with strings easier and safer.
Next, we’ll explore these two methods in greater detail.
How To Declare & Initialize C-Style Strings In C++ Programs?
In C programming language, a string is an array of characters that ends with a null character (\0). The null terminator tells the compiler where the string ends, as arrays don’t inherently know their own length.
C++ also supports C-style character string manipulation with functions like strcpy(), strcmp(), and strcat(). While functional, this method is less common in modern C++ due to the increased complexity and lower safety compared to the C++ string class. The syntax and an example C++ program for this are given below.
Syntax For C-Style Strings In C++:
char array_name[] = {‘ch1’, ‘ch2’, ‘ch3’, ….}; // Array of individual characters
char array_name[] = "string_value"; // String literal
Here,
- The term array_name refers to the name of the array and the char keyword signals that its elements are of character type.
- The square brackets [] or array notation indicate that it is an array, and they can optionally contain the size of the array.
Also seen in the syntax above, you can assign a value to a character array/ C-style string in two ways:
- By listing individual characters enclosed in single quotes inside the curly braces {}. These braces are known as the initializer list.
- By assigning a string value enclosed in double quotes (“ ”).
Examples:
char s1[7] = "unstop"; // C-style string, including null character
char s2[] = "unstop"; // Size deduced automatically
char s3[7] = {'U', 'n', 's', 't', 'o', 'p', '\0'}; // Explicitly adding null character
Here, we initialize the s1 and s2 strings using the double quotes string literal method. We use the individual character inside the curly braces to initialize the last string, s3. The size of these arrays will automatically be 7 characters, where the last is the null terminator (\0), added by the compiler.
Look at the simple C++ program example below, which illustrates how to declare and initialize C-style strings.
Code Example:
#include <iostream>
int main() {
char str[] = "Hello, world!"; // C-style string declaration
std::cout << str << std::endl; // Print the C-style string
return 0;
}
Output:
Hello, world!
Code Explanation:
We begin the C++ code example by including the <iostream> header file for input/ output functionality.
- In the main() function, we declare a C-style string or character array named str and assign the value “Hello, world!” to it (using double quotes). The string's end is indicated by the automated addition of the null character (/0) in C++.
- Then, we use the std::cout stream to display the value to the console.
- Finally, the main() function returns 0, indicating successful execution to the operating system.
Note: C-style strings are less common in modern C++ code due to their complexity and lack of safety features.
How To Declare & Initialize Strings In C++ Using String Keyword?
In C++, strings can be declared and initialized using the std::string class, which is part of the C++ Standard Library. Unlike C-style strings (character arrays), the std::string class provides a variety of built-in functions that simplify string manipulation, making it a safer and more flexible option.
To use the string class, you need to include the <string> header in your program. Once included, you use the string keyword to declare and initialize string variables in C++ programs.
Syntax For Creating Strings In C++:
std::string string_name = "string_value";
std::string string_name("string_value");
Here,
- In std::string, the string keyword indicates that the following variable is of string type. The std:: is used to indicate that it is from a library class.
- The name of the string is given by string_name and the value is given by string_value.
As you can see, there are two ways of assigning values to strings in C++ programs:
- In the first method, we use the simple assignment operator (=) to assign the string value enclosed in double quotes.
- In the second method, we directly assign the string value (inside parentheses) using the constructor provided by the std::string class. This approach offers more flexibility, as the string class automatically manages memory, and you don’t need to worry about null terminators.
The basic C++ program example below illustrates both the methods of declaring and initializing a string variable using the string keyword from <string>.
Code Example:
#include <iostream>
#include <string> // Required to use std::string
int main(){
std::string s1 = "Hello"; // String initialization using the assignment operator
std::string str("Unstop"); // String initialization using the constructor
std::cout << "s1 = " << s1 << std::endl;
std::cout << "str = " << str << std::endl;
return 0;
}
Output:
s1 = Hello
str = Unstop
Code Explanation:
In the C++ code example, we include the <iostream> header for input operations and the <string> header to use the C++ string functionalities.
- In the main() function, we use the string keyword to declare two variables, s1 and str of type string.
- As mentioned in the code comments, we initialize s1 with the value "Hello" using the assignment operator and str with the value "Unstop" using the constructor from the string class.
- The strings are encased in double quotes and are automatically terminated with the null character /0 at the end.
- We then print the values to the console using std::cout statements.
Note: Alternatively, you can include the statement– using namespace std; – after the #include preprocessor directive for header files, to avoid typing std:: every time. However, it's recommended to use the std:: prefix explicitly in larger programs to prevent potential naming conflicts, especially in projects that use multiple libraries.
Declaring & Initializing Empty Strings (Uninitialized String In C++)
You can also declare an empty string, which is simply a string with no initial value. In other words, you can declare a string variable without initializing it at the same point. This is what the syntax would look like:
std::string emptyString;
In this case, emptyString remains uninitialized until a value is assigned to it later in the program.
Check out this amazing course to become the best version of the C++ programmer you can be.
Advantages Of Using The std::string Class
- Automatic Memory Management: The std::string class dynamically manages memory, reducing the risk of buffer overflow and making it safer compared to C-style strings.
- Rich Functionality: The string class offers various built-in functions such as concatenation, comparison, searching, and modifying strings, which are easier to use than C-style functions like strcpy() or strcat().
- Null-Termination: Unlike C-style strings, you don’t need to manually ensure that the string ends with a null character (\0); this is handled automatically.
By utilizing the std::string class, you can handle strings more efficiently and securely in C++ programs. The ability to use operators like = and various constructors offers flexibility when initializing and manipulating strings, making the std::string class the preferred option in modern C++ programming.
List Of String Functions In C++
C++ provides a variety of built-in functions to perform different operations on strings. These functions make it easy to manipulate strings without manually managing memory or handling null characters, as is required with C-style strings. Here are some commonly used string functions in C++:
Function |
Description |
Syntax |
size() |
Returns the size of the string in bytes. |
string_name.size() |
length() |
Returns the length/ number of characters in the string, including spaces. (same as size()). |
string_name.length() |
push_back() |
Appends a character to the end of the string. |
string_name.push_back('char') |
pop_back() |
Removes the last character of the string. |
string_name.pop_back() |
resize() |
Resizes the string to the specified number of characters. If the new size is larger, it adds default characters ('\0'). |
string_name.resize(new_size) |
swap() |
Swaps the contents of two strings. |
string_name1.swap(string_name2) |
find() |
Searches for/ finds the first occurrence of a substring and returns its index. If not found, it returns std::string::npos. |
string_name.find("substring") |
erase() |
Removes/ erases a part of the string, starting from the given position. |
string_name.erase(pos, len) |
compare() |
Compares two strings lexicographically. |
string_name.compare("another_string") |
substr() |
Extracts a substring from the string. It returns a substring starting from a given position and of a specified length. |
string_name.substr(pos, len) |
at() |
Accesses the character at a specified position. |
string_name.at(pos) |
copy() |
Copies characters from a string into a char array. |
string_name.copy(char_array, len, pos) |
capacity() |
Returns the total capacity of the string, i.e., how many characters it can hold without reallocating memory. |
string_name.capacity() |
replace() |
Replaces part of the string with another string. |
str.replace(pos, len, "new_str") |
empty() |
Checks if the string is empty. |
str.empty() |
append() |
Adds another string to the end of the current string. |
str.append("another_str") |
shrink_to_fit() |
Reduces the capacity of the string to fit its size, releasing unused memory. |
str.shrink_to_fit() |
data() |
Returns a pointer to the underlying character array. |
str.data() |
c_str() |
Returns a C-style string (null-terminated character array) equivalent to the string object. |
str.c_str() |
begin() |
Returns an iterator to the first character of the string. Useful for iteration through the string. |
string_name.begin() |
end() |
Returns an iterator to the end of the string. (Marks the end of iteration.) |
string_name.end() |
rbegin() |
Returns a reverse iterator to the end of the string. Starts reverse iteration from the last character. |
string_name.rbegin() |
rend() |
Returns a reverse iterator to the beginning of the string. |
string_name.rend() |
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Operations On Strings Using String Functions In C++
Now, let’s take a look at some examples of how you can perform various operations on strings in C++ using these functions.
Input String Functions In C++
The following functions are commonly used for input operations on strings:
- getline( ): It is used to read and store an input stream of characters (string including spaces) in the object memory.
- push_back( ): Using this function, you can add the single character passed to the end of the string.
- pop_back( ): This function is used to remove the last character from the string.
Code Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string s;
char c;
cout << "Please enter a string: ";
getline(cin, s);
cout << "Your string is: " << s << endl;
cout << "Please enter a character to add to the string: ";
cin >> c;
s.push_back(c);
cout << "Your string with the added character is: " << s << endl;
s.pop_back(); \\Removing last character of the string
cout << "Your string with the last character removed is: " << s << endl;
return 0;
}
Output:
Please enter a string: Hello, world!
Your string is: Hello, world!
Please enter a character to add to the string: ?
Your string with the added character is: Hello, world!?
Removing the last character from the string…
Your string with the last character removed is: Hello, world!
Code Explanation:
- In this example, we declared a string s and initialized it by taking user input using the getline() function.
- We then declared a character variable c and took a single character input from the user.
- After that, we called the push_back() function, which appended the character c to the end of the string s.
- Lastly, we used the pop_back() function, which removed the last character of the string s.
Capacity String Functions In C++
These functions are related to managing the storage capacity of strings in C++:
- capacity( ): This function returns the capacity that the compiler assigned to the string passed as argument.
- resize( ): Used to resize the string to the specified length/ number of characters.
- shrink_to_fit( ): This function reduces the current storage capacity of the string to its current size, releasing the excess space.
Code Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string s = "Hello, world!";
cout << "Original string: " << s << endl;
cout << "Original capacity: " << s.capacity() << endl;
s.resize(5);
cout << "After resizing: " << s << endl;
cout << "Capacity after resizing: " << s.capacity() << endl;
s.shrink_to_fit();
cout << "Capacity after shrink_to_fit: " << s.capacity() << endl;
return 0;
}
Output:
Original string: Hello, world!
Original capacity: 15
After resizing: Hello
Capacity after resizing: 15
Capacity after shrink_to_fit: 5
Code Explanation:
In the C++ example code,
- We declared and initialized string variable s with the value "Hello, world!".
- Then, we called the capacity() function to display the current memory capacity allocated for the string.
- Next, we called resize(5), which truncated the string to the first 5 characters. Even though the string was resized, the memory capacity remained unchanged.
- Finally, we called shrink_to_fit(), which adjusted the memory capacity to match the new size of the string.
Iterator String Functions In C++
These functions provide access to string elements through iterators:
- begin(): This is one of the most commonly used iterator functions that returns an iterator to the start of the string.
- end(): It returns the iterator to the end of the string.
- rend( ): The outcome of this function is a reverse iterator that points to the string's beginning.
- rbegin( ): It retruns a reverse iterator that starts at the end of a string.
Code Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string s = "Hello, world!";
// Using forward iterator
cout << "Using forward iterator: ";
for (auto it = s.begin(); it != s.end(); ++it) {
cout << *it;
}
cout << endl;
// Using reverse iterator
cout << "Using reverse iterator: ";
for (auto it = s.rbegin(); it != s.rend(); ++it) {
cout << *it;
}
cout << endl;
return 0;
}
Output:
Using forward iterator: Hello, world!
Using reverse iterator: !dlrow ,olleH
Code Explanation:
In this program,
- We declared a string s with the value "Hello, world!".
- Then, we called the begin() function to create an iterator that starts at the first character of the string. We used this iterator in a for loop to print the string in its original order.
- Next, we called the rbegin() function to obtain a reverse iterator starting from the last character of the string and used it in another for loop to print the string in reverse order.
Manipulating String Functions In C++
These functions allow you to copy and swap strings in C++ programs:
- copy(“char array”, len, pos ): Copies a portion of the string into a char array. Three inputs are typically required: the target char array, the length to be copied, and the starting place inside the string to begin copying.
- swap( ): It swaps the contents of one string with another string.
Example C++ program to show manipulating string functions
#include <iostream>
#include <string>
using namespace std;
int main() {
string my_string = "Hello, world!";
// Copying a substring
string copied_string = my_string.substr(7, 5);
cout << "Copied substring: " << copied_string << endl;
// Swapping two characters in the string
swap(my_string[0], my_string[7]);
cout << "After swapping: " << my_string << endl;
return 0;
}
Output:
Copied substring: world
After swapping: wello, Horld!
Code Explanation:
In this example,
- We declared a string my_string and initialized it with the value "Hello, world!".
- Then, we used the substr() function to copy a portion of the string, starting from index 7 and extracting 5 characters, resulting in the substring "world", and print it to the console.
- Next, we performed a swap operation between the first character (my_string[0]) and the eighth character (my_string[7]), which resulted in the string changing from "Hello, world!" to "wello, horld!".
- Finally, we printed the updated string to the console.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and claim the bragging rights, now!
Concatenation Of Strings In C++
In programming, the concatenation of strings is the process of joining two strings with each other, end to end. For example, say we have two strings- 'Unstop' and 'pable'. Then, the result of string concatenation of these two will be 'Unstoppable'.
For more, read: C++ String Concatenation | All Methods Explained (With Examples)
How To Convert Int To Strings In C++
In C++, converting an integer to a string can be essential when you need to concatenate numbers with text, display numerical values in a textual format, or store integers as strings. In this section, we will explore three common methods for converting integers to strings in C++ langauge:
- to_string()
- boost::lexical_cast
- stringstream class
We will discuss each method with examples and explanations.
Method 1: Using to_string()
The to_string() function in C++ converts an integer (or other primitive types) to its corresponding string representation. It is straightforward and part of the string library.
Syntax:
string to_string (data_type variable_name);
Code Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
int num = 123;
string str_num = to_string(num);
cout << str_num << endl;Â // Output: "123"
return 0;
}
Output:
123
Code Explanation:
- In this example, we first declare an integer variable num and assigned th evalue 123 to it.
- Then, we use the to_string() function to convert it into a string, and store the outcome in the variable str_num.
- After that, we print the string using the cout command.
- Note that the to_string() function is part of the string header, so we included the #include <string> directive at the top of the program.
Method 2: Using boost::lexical_cast
The boost::lexical_cast is part of the Boost library and allows for converting between different types, including strings and integers. Before using it, you need to install Boost on your system.
Syntax:
boost::lexical_cast<data-type>(argument)
Code Example:
#include <iostream>
#include <boost/lexical_cast.hpp>
using namespace std;
int main() {
string str_num = "42";
int num = boost::lexical_cast<int>(str_num);
cout << "The string \"" << str_num << "\" as an integer is: " << num << endl;
return 0;
}
Output:
The string "42" as an integer is: 42
Code Explanation:
- In this program, we first declare a string str_num and assign the value “42” to it.
- Then we use the boost::lexical_cast method to convert it to an integer, indicating conversion to integer type (<int>).
- We store the converted value in the variable num and then print it using the cout command.
- Note that if the string is invalid (e.g., "hello"), boost::lexical_cast will throw a boost::bad_lexical_cast exception.
Method 3: Using stringstream Class
The stringstream class allows you to manipulate strings as streams, making it easy to insert and extract values. It’s useful for converting between different types.
Code Example:
#include <iostream>
#include <sstream>
using namespace std;
int main() {
int num = 42;
stringstream ss;
ss << num;Â // Insert integer into the stream
string str_num = ss.str();Â // Extract as a string
cout << "The integer " << num << " as a string is: " << str_num << endl;
return 0;
}
Output:
The integer 42 as a string is: 42
Code Explanation:
- In this example, we first declare an integer num and create a stringstream object ss.
- Then, we insert the integer into the stream using the shift operator (<<).
- After that, we extracte the string version using the str() function and store it in the str_num variable.
- Finally, we printed the result using the cout command.
Looking for guidance? Find the perfect mentor from select experienced coding & software experts here.
Conclusion
Strings in C++ are versatile tools for handling textual data/ information. They can be declared and initialized in two primary ways, either using C-style character arrays or by leveraging the std::string class, which offers enhanced functionality. The latter provides several in-built methods for manipulation, comparison, and processing, making it more versatile for modern programming needs.
When dealing with strings, a range of functions like size(), length(), and swap() simplify common operations such as checking length or swapping values between strings. Functions like push_back() and pop_back() help with adding or removing characters, resize() lets you adjust the string size dynamically, and capacity() and shrink_to_fit() allow optimum memory management. In addition we can also convert integers to string, using the simple std::to_string() method or other advanced options like using stringstream for stream-based conversion or boost::lexical_cast for flexible data type conversions.
Mastering these tools are essential when dealing with strings in C++ and will allow you to efficiently manage text, optimize memory usage, and handle data type conversions with ease.
Also read: 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. Can I use string as a function in C++
No, a string cannot be used as a function in C++. The std::string class represents sequences of characters and includes various methods for handling text (in the string header file), but it does not function as a callable object.
Q. How to declare and initialize a string in C++?
The most common and preferred method of creating strings in C++ language is using the std::string class. For this, you must include the <string> header in the preprocessor directive at the beginning of the program.
Once you have done that, you can create a string like in the following example:
std::string myString = "Hello, world!";
Here, we declared a string variable called myString, using the std::string keyword and assing it a value– “Hello, world!”.
Alternatively you can simply declare a string using the same method, without assigning it any value. This will result in an empty string, as follows:
std::string myString;
The variable myString will remain an empty string until it is assigned a value.
Q. How to concatenate two strings in C++?
There are two ways to concatenate two strings in C++. The most common is the concatenation operator (+), also known as the addition arithmetic operator. Alternatively, you can use the append() method to combine two strings. An example of using the concatenation operator is:
std::string fullName = firstName + " " + lastName;
Here, we are creating a new string called fullName, which combines the strings firstName and lastName with a white space in between
Q. How to get the length of a string in C++?
You can use either length() or size() to get the length of a strings in C++. Both the functions belong to the <string> header.
std::string myString = "Hello";
int len = myString.length();
Here, we first created a string variable called myString and assigned it the value “Hello”. We then call the length() function on mystring using the dot operator. This will return the string length which in this case is 5.
Q. How to convert a string to an integer in C++?
There are multiple ways to transform a string into an integer in C++. You can use either the std::stoi() function or stringstream to convert a string to an integer. For example:
int num = std::stoi("123");
This function will conver the string “123” into the integer value 123. However, then using this function you must ensure proper error handling to avoid exceptions like std::invalid_argument or std::out_of_range.
Q. How to extract a substring from a string in C++?
Use the substr() function to extract part of a string or a substring from a string in C++ program. The function generally takes two arguments: the starting position (inclusive) and the length of the substring.
std::string sub = myString.substr(2, 5); // Extracts a substring starting at index 2 with length 5
In the snippet above, we extract a substring that begins at the 2nd index position (3rd character) and continues for 5 characters.
Q. How to replace a substring in a string in C++?
You can use the replace() function from the std::string class to replace a part of a string in C++. The function takes three parameters: the starting position from where you want to replace, the length of the substring you want to replace, and the new substring that will replace the original one. For example:
std::string myString = "Hello";
myString.replace(1, 2, "Hi"); // Output:HHilo
Q. Give the difference between a string and a character array.
The table below highlights the key differences between character arrays and strings in C++.
String |
Character Array |
Immutable once defined |
Mutable after creation |
A sequence of characters |
A collection of char values |
Static memory allocation |
|
Stored in the String Constant Pool |
Stored in heap or stack memory |
This compiles our discussion on strings in C++ programming.
Test Your Skills: Quiz Time
You might also be interested in reading the following:
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- OOPs Concept In C++ | A Detailed Guide With Codes & Explanations
- Defining Constant In C++ | Literals, Objects, Functions & More
- C++ If-Else Statement | Syntax, Types & More (+Code Examples)
- C++ Find() In Vector | How To Find Element In Vector With Examples
- Difference Between C And C++| Features | Application & More!
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Ujjwal Sharma 1 week ago
Anjali Nimesh 1 week ago
Samruddhi Deshmukh 2 weeks ago
Kartik Deshmukh 2 weeks ago
Sanchit Dhale 1 month ago
RAVI PRATAP 1 month ago