substr() In C++ | Definition And Functions Explained With Examples
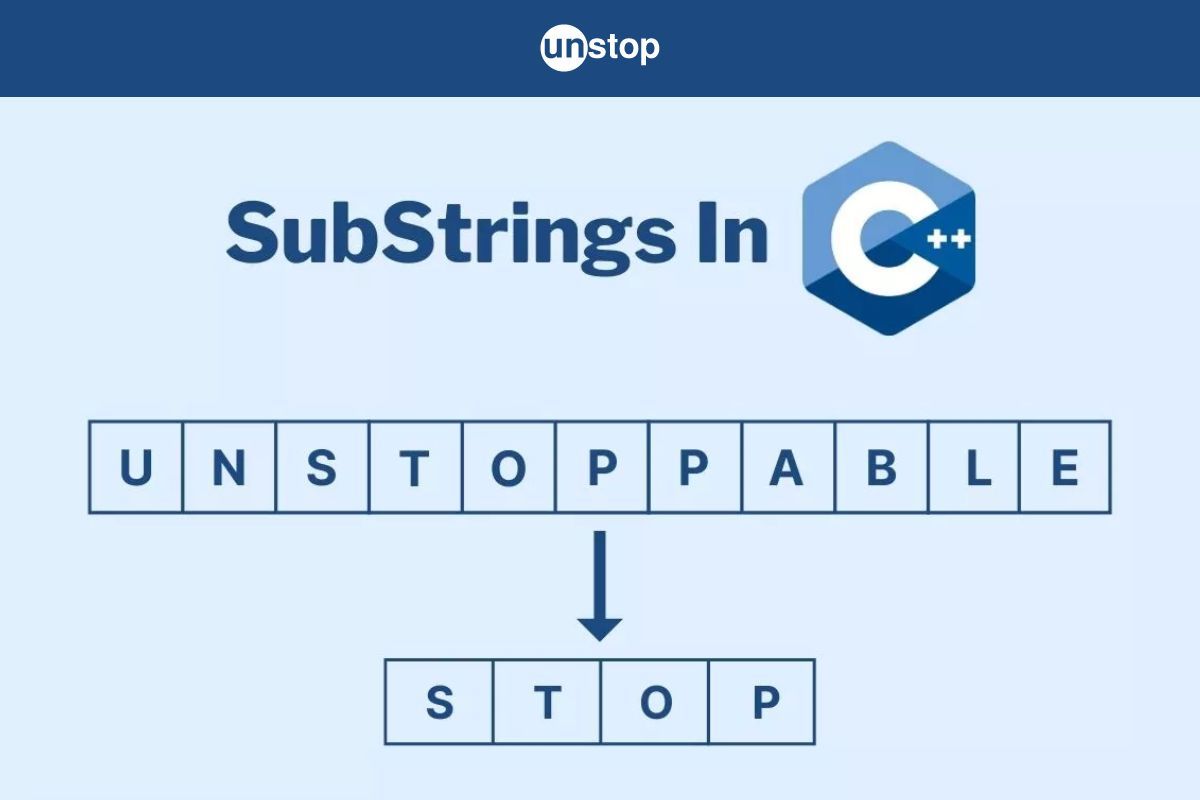
The Substring function or substr in C++ programming language is a part of the string function that is used to get a part of the string from the original string. It is a predefined method in the “string.h” header file (i.e., string header file) used for string slicing and handling other string operations like append(), strcat(), etc.
In this blog, we will discuss the structure, syntax, parameters, and return type of substr function in C++ with the help of examples and exercises. At the end of this tutorial, we will discuss the top 8 FAQs and solutions to better understand the topic. So let’s get started!
What Is A Substring In C++ (Substr C++)?
In C++, a part of a string is referred to as a substring. The substr() function in C++ is defined in the “String.h” header file and is used for string extraction or string slicing. The substr in C++ takes two parameters, the starting index and the length of the substring we want from that index, and then it returns a new string object to construct a substring from the original string.
Syntax of Substr Function in C++
Here is the basic syntax of substr() function:
string substr(starting_pos, len_substr)
It consists of three main parameters:-
- String keyword - indicates that the return type of substr function is a string.
- starting_pos - an unsigned Integer type input parameter. It denotes the position of the first character to be copied from the original string. (Note that the pos parameter is the position parameter, i.e., it refers to the position from which we want the substring to start.)
- len_substr - an unsigned Integer type input parameter denotes the length of the substring.
Return Type
Similar to a string type variable, the substr function also returns a newly constructed object of type String. You can store this newly constructed string object in the following way:
string substring=str.substr(firstParameter,secondParameter);
Here str is the variable name in which the original string has been stored. And substring is the variable name for the newly extracted substring.
Example For Substr In C++
Let's understand the substr in C++ using the following example:
#include
#include
using namespace std;
int main() {
string originalString = "#Unstoppable"; //original string
string substr1 = originalString.substr(1, 6); //substring starting from index 1 and length 6
string substr2 = originalString.substr(8, -2); //substring starting from index 8 and length -2
cout << "Substring1 starting at position 1 and length 6 is: " << substr1 << endl;
cout << "Substring2 starting at position 8 and length -2 is: " << substr2 << endl;
return 0;
}
Output:
Substr1 starting at position 1 and length 6 is: Unstop
Substr2 starting at position 8 and length -2 is: able
Explanation:
In the first case, since the starting index is 1, it implies that the substring1 starts from ‘U’ and ends at the 6th index, which is ‘p’, giving a substring of length 6.
Meanwhile, in the second case, since the length is -2, which is a negative number, the substr function will take all the characters till the end of the string. This is because the parameters of substr function only take Unsigned_integer type values.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
A Few More Examples
Consider a string s = “Unstoppable”. The following figures shows the string and respective indexes of each character in the sequence of characters in the string-
string: U n s t o p p a b l e
index: 0 1 2 3 4 5 6 7 8 9 10
Now if we write:
s.substr(s.begin(),5) the result wil be a compilation error, because the s.begin() is an iterator datatype and not an integer datatype.
s.substr(2,3) the result is 'stt'. That is, it returns a string of three letters starting from the 2nd index
s.substr(*s.begin()-s[0],6) the result will be Unstop. That is s.begin() is U, then 'h'-s[0] gives 'U'-'U'=0, means subtr(0,6) or sixth letter from the zeroth index.
s.substr(2,0) the result will be (no output) since it selects zero letters from the second index.
Points To Remember For Substr In C++
- The index of the first character is always taken as 0 (not 1).
- The starting_pos and substr_len parameters only accept unsigned integer numbers and no other data type.
- If starting_pos equals (=) the original string length, the function returns an empty string.
- If starting_pos is greater than (>) original string length, it throws an ‘out_of_range’ exception, and there are no changes in the string.
- If substr_len is greater than (>) the size of the original string, then returned sub-string is [starting_pos, size()).
- If substr_len is not passed as a parameter, then returned sub-string is [starting_pos, size()).
Important Applications Of substr() Function
In this section, we will look at the code implementation examples to show various applications of the substring function, subtr() in C++.
How to Get a Substring Before a Character?
Let’s assume that you have been given a string and a character. Your task is to print the sub-string followed by the given character. The example below shows how this can be done:
Sample Code:
// C++ program to demonstrate functioning of substr()
#include
#include
using namespace std;
int main()
{
// Take any string
string s = "Hardwork:Success";
// Find position of ':' using find()
int pos = s.find(":");
// Copy substring before pos
// Extract everything before the ":" in the given string
string sub = s.substr(0, pos);
// prints the result
cout << "String is: " << sub;
return 0;
}
Output:
String is: Hardwork
Explanation:
A string is taken, and we take the input of a character. To print the sub-string followed by the given character, we first find the position of the given character. Then we copy the intended substring using the substr function starting from the 0th index until the position of the character, which is the length of the intended substring.
Print All Substrings Of A Given String
Let’s learn how to obtain all possible substrings from a given string.
Example Code:
// C++ program to print all possible substrings of a given string
#include <bits/stdc++.h>
using namespace std;
// Function to print all the sub strings
void subString(string s, int length)
{
// Pick the starting point in outer loop and vary the lengths of
// different strings for a given starting point in the inner loop
for (int i = 0; i < length; i++)
for (int len = 1; len <= length - i; len++)
cout << s.substr(i, len) << endl;
}
// Driver program to test the above function
int main()
{
string s = "code";
subString(s, s.length());
return 0;
}
Output:
c
co
cod
code
o
od
ode
d
de
e
Explanation:
The possible substrings of string “code” are { c, co, cod, code, o, od, ode, d, de, e}. We vary the starting point in the outer loop and the lengths of the substring in the inner loop.
Print Sum Of All Substrings Of A String Representing A Number
Suppose we have been provided with an integer represented as a string and we have been asked to get the sum of all possible substrings of this string. We can do this using the following:
Code:
#include <bits/stdc++.h>
using namespace std;
// Method to convert digit in character format to integer digit
int toDigit(char ch)
{
return (ch - '0');
}
// Returns sum of all substring of string num
int sum_Substrings(string num)
{
int n = num.length();
// allocating memory equal to length of the string
int sumofdigits[n];
// initialize first value with first digit in the string
sumofdigits[0] = toDigit(num[0]);
int result = sumofdigits[0];
// loop over all digits of string
for (int i = 1; i < n; i++) {
int numi = toDigit(num[i]);
// update each sumofdigits with the previous value
sumofdigits[i] = (i + 1) * numi + 10 * sumofdigits[i - 1];
// adding current value to the result
result += sumofdigits[i];
}
return result;
}
// Driver code to test above methods
int main()
{
string num = "423";
cout << sum_Substrings(num) << endl;
return 0;
}
Output:
497
Explanation: All substrings are { 4, 42, 423, 2, 23, 3 }, and the sum of these substrings is 497.
Print Minimum Value Of All Substrings Of A String Representing A Number
We have been given an integer represented as a string. Now our task is to get the minimum of all possible substrings of the given string, which is representing a number.
Code:
// C++ program to print the minimum of all the
// substrings of a given string representing a number
#include <bits/stdc++.h>
using namespace std;
void subString(string s, int length)
{
vector v;
// finding all possible substrings
for (int i = 0; i < length; i++){
for (int len = 1; len <= length - i; len++){
string sub_str =(s.substr(i, len));
int x=stoi(sub_str); // converting to integer format
v.push_back(x);//inserting into a vector
}
}
cout<<*min_element(v.begin(),v.end())<<endl;
}
// Driver program to test above function
int main()
{
string s = "9328";
subString(s, s.length());
return 0;
}
Output
2
Explanation:
All substrings are { 9, 93, 932, 9328, 3, 32, 328, 2, 28, 8 }, and the minimum value substring is 2. The function subString accepts two parameters, the string s and the length of the string. We then create a vector to store the substrings in integer format. A for loop is created to calculate all possible substrings and convert them to integers, subsequently inserting this into a vector. We finally find the minimum element using C++ STL.
Print Maximum Value Of All Substrings Of A String Representing A Number
We have been given an integer represented as a string. Now our task is to get the maximum of all possible substrings of the given string, which is representing a number.
Example Code:
// C++ program to find maximum of all possible substrings of given string
#include <bits/stdc++.h>
using namespace std;
void subString(string s, int length)
{
vector v;
// finding all possible substrings
for (int i = 0; i < length; i++){
for (int len = 1; len <= length - i; len++){
string sub_str =(s.substr(i, len));
int x=stoi(sub_str);// converting to integer format
v.push_back(x);//inserting into a vector
}
}
cout<<*max_element(v.begin(),v.end())<<endl;
}
// Driver program to test above function
int main()
{
string s = "423";
subString(s, s.length());
return 0;
}
Output
423
Explanation: All substrings are { 4, 42, 423, 2, 23, 3 }, and the maximum value substring is 423. The function subString accepts two parameters, the string s and the length of the string. We then create a vector to store the substrings in integer format. A for loop is created to calculate all possible substrings and convert them to integers, subsequently inserting this into a vector. We finally find the maximum element using C++ STL.
Conclusion
- This substr() function in C++ is the method of the string.h library.
- The substr() function takes two parameters a starting_pos and substr_len, which takes only unsigned integer values.
- If the second parameter is negative or greater than the length of the original string, the parameter automatically assigns to the total length-1 value.
- The first parameter cannot be of a negative number or any other data type.
Frequently Asked Questions
Q. What is a substr() in C++?
Substr() is a function in C++ included in the “string.h” header file. It is used to get a copy of the desired length or a part of the string from the original string.
The substr function takes two input arguments:
1) Starting_pos: Indicating the Position of the first character to be copied from the original string.
2) Substr_len: Indicates the desired length of the substring from the first position.
Here is the basic syntax of substr function:-
string substr(starting_pos, len_substr)
Q. How to get a substring of a string in CPP?
To get the substring of a string in CPP, you can use the substr() function. To include the substr function in C++, you also have to include “string.h” library in the program.
Here is an example:
#include
#include // including string.h library
using namespace std;
int main() {
string originalString = "Substring";
string sub_string = original_string.substr(0,6);
// starting_pos = 0 and length = 6
// Implies it prints from index=0 to index=5 of the original string
cout << sub_string <<endl;
return 0;
}
Output:
Substr
Q. How to extract a substring from a string?
To extract a substring from a string, you can use the substr() function, which takes the starting position and length of the substring as input parameters and returns a string type value.
Let's understand this using an example.
#include
#include
using namespace std;
int main() {
string originalString = "#Unstoppable"; //original string
string sub_str = originalString.substr(1, 6); //substring starting from index 1 and length 6
cout << "Extrcated substring starting at position 1 and length 6 is: " << sub_str << endl;
return 0;
}
Output:-
Extracted substring starting at position 1 and length 6 is:: Unstop
Q. What method removes a substring from a string in CPP?
Here is an example of how you can remove a substring from the original string using the substr function in C++.
#include
#include
Using namespace std;
int main() {
string original ="how are you?";
//getting the substring to be deletd that is “are “
string substring = original.substr(3,4);
original.erase(3,substring.length());
// erase function takes two parameter, the starting index in the string from where you want to erase characters and the length of the substring
cout<<original<<"\n";
return 0;
}
Output:-
how you?
Q. How do I get the first character of a string using a substring?
To get the first character of a string, you can use the substring function, substr() in C++ as follows:
#include
#include
Using namespace std;
int main() {
String original = “hello world” ; // original string
// to get the first character starting position=0 and length of substr = 1 .
Char first_ch = original.substr(0,1);
// now ‘h’ character is stored in first_ch variable
cout<<first_ch;
return 0;
}
Output:-
h
Q. Does the substr start at 0 or at 1?
Similar to string datatype, the substr function also returns a normal string type value. Therefore like anyother string ,the starting index of the substr or substring is also 0.
Q. Does a substring include the first character?
The substr function in c++ includes two input parameters:
1)Starting_pos: Indicating the position of the first character to be copied from the original string.
2)Substr_len: Indicates the desired length of the substring form the first position.
Therefore it includes the character present in the original string whose index is mentioned in place of starting_pos.
Q. How do I get the first n characters from a string?
We can use the substr() function to get the first n characters from a string. Let’s learn using the following example:
#include
#include
Using namespace std;
int main() {
String original = “hello world” ; // original string
int n=5; // n is the length of characters, from first position, that you want.
// to get the first n characters starting position=0 and length of substr = n;
// here n=5 therefore substr_len=5; .
string first_n_ch = original.substr(0,n);
// now first n character are stored in first_n_ch string type variable
cout<<first_n_ch;
return 0;
}
Output:
hello
You might also be interested in reading the following:
- Pointers in C++ | A Roadmap To All Types Of Pointers With Examples
- Decoding The Difference Between Structure And Class In C++
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- Difference Between C And C++| Features | Application & More!
- Find In Strings C++ | Examples To Find Substrings, Character & More!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment