- A Brief Intro To C++
- The Timeline Of C++
- Importance Of C++
- Versions Of C++ Language
- Comparison With Other Popular Programming Languages
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Variables In C++?
- Declaration & Definition Of Variables In C++
- Variable Initialization In C++
- Rules & Regulations For Naming Variables In C++ Language
- Different Types Of Variables In C++
- Different Types of Variable Initialization In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Primitive Data Types In C++?
- Derived Data Types In C++
- User-Defined Data Types In C++
- Abstract Data Types In C++
- Data Type Modifiers In C++
- Declaring Variables With Auto Keyword
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Structure Of C++ Program: Components
- Compilation & Execution Of C++ Programs | Step-by-Step Explanation
- Structure Of C++ Program With Example
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What is Typedef in C++?
- The Role & Applications of Typedef in C++
- Basic Syntax for typedef in C++
- How Does typedef Work in C++?
- How to Use Typedef in C++ With Examples? (Multiple Data Types)
- The Difference Between #define & Typedef in C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Strings In C++?
- Types Of Strings In C++
- How To Declare & Initialize C-Style Strings In C++ Programs?
- How To Declare & Initialize Strings In C++ Using String Keyword?
- List Of String Functions In C++
- Operations On Strings Using String Functions In C++
- Concatenation Of Strings In C++
- How To Convert Int To Strings In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is String Concatenation In C++?
- How To Concatenate Two Strings In C++ Using The ‘+' Operator?
- String Concatenation Using The strcat( ) Function
- Concatenation Of Two Strings In C++ Using Loops
- String Concatenation Using The append() Function
- C++ String Concatenation Using The Inheritance Of Class
- Concatenate Two Strings In C++ With The Friend and strcat() Functions
- Why Do We Need To Concatenate Two Strings?
- How To Reverse Concatenation Of Strings In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Find In String C++?
- What Is A Substring?
- How To Find A Substring In A String In C++?
- How To Find A Character In String C++?
- Find All Substrings From A Given String In C++
- Index Substring In String In C++ From A Specific Start To A Specific Length
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Pointers In C++?
- Pointer Declaration In C++
- How To Initialize And Use Pointers In C++?
- Different Types Of Pointers In C++
- References & Pointers In C++
- Arrays And Pointers In C++
- String Literals & Pointers In C++
- Pointers To Pointers In C++ (Double Pointers)
- Arithmetic Operation On Pointers In C++
- Advantages Of Pointers In C++
- Some Common Mistakes To Avoid With Pointers In Cpp
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Understanding Pointers In C++
- What Is Pointer To Object In C++?
- Declaration And Use Of Object Pointers In C++
- Advantages Of Pointer To Object In C++
- Pointer To Objects In C++ With Arrow Operator
- An Array Of Objects Using Pointers In C++
- Base Class Pointer For Derived Class Object In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is 'This' Pointer In C++?
- Defining ‘this’ Pointer In C++
- Example Of 'this' Pointer In C++
- Describing The Constness Of 'this' Pointer In C++
- Important Uses Of 'this' Pointer In C++
- Method Chaining Using 'this' Pointer In C++
- C++ Programs To Show Application Of 'This' Pointer
- How To Delete The ‘this’ Pointer In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What is Reference?
- What is Pointer?
- Comparison Table Of C++ Pointer Vs. Reference
- Differences Between Reference And Pointer: A Detailed Explanation
- Why Are References Less Powerful Than Pointers?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- How To Declare A 2D Array In C++?
- C++ Multi-Dimensional Arrays
- Ways To Initialize A 2D Array In C++
- Methods To Dynamically Allocate A 2D Array In C++
- Accessing/ Referencing Two-Dimensional Array Elements
- How To Initialize A Two-Dimensional Integer Array In C++?
- How To Initialize A Two-Dimensional Character Array?
- How To Enter Data In Two-Dimensional Array In C++?
- Conclusion
- Frequently Asked Questions
- What Are Arrays Of Strings In C++?
- Different Ways To Create String Arrays In C++
- How To Access The Elements Of A String Array In C++?
- How To Convert Char Array To String?
- Conclusion
- Frequently Asked Questions
- What is Memory Allocation in C++?
- The “new" Operator In C++
- The "delete" Operator In C++
- Dynamic Memory Allocation In C++ | Arrays
- Dynamic Memory Allocation In C++ | Objects
- Deallocation Of Dynamic Memory
- Dynamic Memory Allocation In C++ | Uses
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A Substring In C++ (Substr C++)?
- Example For Substr In C++
- Points To Remember For Substr In C++
- Important Applications Of substr() Function
- How to Get a Substring Before a Character?
- Print All Substrings Of A Given String
- Print Sum Of All Substrings Of A String Representing A Number
- Print Minimum Value Of All Substrings Of A String Representing A Number
- Print Maximum Value Of All Substrings Of A String Representing A Number
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Operator In C++?
- Types Of Operators In C++ With Examples
- What Are Arithmetic Operators In C++?
- What Are Assignment Operators In C++?
- What Are Relational Operators In C++?
- What Are Logical Operators In C++?
- What Are Bitwise Operators In C++?
- What Is Ternary/ Conditional Operator In C++?
- Miscellaneous Operators In C++
- Precedence & Associativity Of Operators In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is The New Operator In C++?
- Example To Understand New Operator In C++
- The Grammar Elements Of The New Operator In C++
- Storage Space Allocation
- How Does The C++ New Operator Works?
- What Happens When Enough Memory In The Program Is Not Available?
- Initializing Objects Allocated With New Operator In C++
- Lifetime Of Objects Allocated With The New Operator In C++
- What Is The Delete Operator In C++?
- Difference Between New And Delete Operator In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Types Of Overloading In C++
- What Is Operator Overloading In C++?
- How To Overload An Operator In C++?
- Overloadable & Non-overloadable Operators In C++
- Unary Operator Overloading In C++
- Binary Operator Overloading In C++
- Special Operator Overloading In C++
- Rules For Operator Overloading In C++
- Advantages And Disadvantages Of Operator Overloading In C++
- Function Overloading In C++
- What Is the Difference Between Operator Functions and Normal Functions?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Operators In C++?
- Introduction To Logical Operators In C++
- Types Of Logical Operators In C++ With Example Program
- Logical AND (&&) Operator In C++
- Logical NOT(!) Operator In C++
- Logical Operator Precedence And Associativity In C++
- Relation Between Conditional Statements And Logical Operators In C++
- C++ Relational Operators
- Conclusion
- Frequently Asked Important Interview Questions:
- Test Your Skills: Quiz Time
- Different Type Of C++ Bitwise Operators
- C++ Bitwise AND Operator
- C++ Bitwise OR Operator
- C++ Bitwise XOR Operator
- Bitwise Left Shift Operator In C++
- Bitwise Right Shift Operator In C++
- Bitwise NOT Operator
- What Is The Meaning Of Set Bit In C++?
- What Does Clear Bit Mean?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Types of Comments in C++
- Single Line Comment In C++
- Multi-Line Comment In C++
- How Do Compilers Process Comments In C++?
- C- Style Comments In C++
- How To Use Comment In C++ For Debugging Purposes?
- When To Use Comments While Writing Codes?
- Why Do We Use Comments In Codes?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Storage Classes In Cpp?
- What Is The Scope Of Variables?
- What Are Lifetime And Visibility Of Variables In C++?
- Types of Storage Classes in C++
- Automatic Storage Class In C++
- Register Storage Class In C++
- Static Storage Class In C++
- External Storage Class In C++
- Mutable Storage Class In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Decision Making Statements In C++
- Types Of Conditional Statements In C++
- If-Else Statement In C++
- If-Else-If Ladder Statement In C++
- Nested If Statements In C++
- Alternatives To Conditional If-Else In C++
- Switch Case Statement In C++
- Jump Statements & If-Else In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A Switch Statement/ Switch Case In C++?
- Rules Of Switch Case In C++
- How Does Switch Case In C++ Work?
- The break Keyword In Switch Case C++
- The default Keyword In C++ Switch Case
- Switch Case Without Break And Default
- Advantages & Disadvantages of C++ Switch Case
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A For Loop In C++?
- Syntax Of For Loop In C++
- How Does A For Loop In C++ Work?
- Examples Of For Loop Program In C++
- Ranged Based For Loop In C++
- Nested For Loop In C++
- Infinite For Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A While Loop In C++?
- Parts Of The While Loop In C++
- C++ While Loop Program Example
- How Does A While Loop In C++ Work?
- What Is Pre-checking Process Or Entry-controlled Loop?
- When Are While Loops In C++ Useful?
- Example C++ While Loop Program
- What Are Nested While Loops In C++?
- Infinite While Loop In C++
- Alternatives To While Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Loops & Its Types In C++?
- What Is A Do-While Loop In C++?
- Do-While Loop Example In C++ To Print Numbers
- How Does A Do-While Loop In C++ Work?
- Various Components Of The Do-While Loop In C++
- Example 2: Adding User-Input Positive Numbers With Do-While Loop
- C++ Nested Do-While Loop
- C++ Infinitive Do-while Loop
- What is the Difference Between While Loop and Do While Loop in C++?
- When To Use A Do-While Loop?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are 2D Vectors In C++?
- How To Declare 2D Vector In C++?
- How To Initialize 2D Vector In C++?
- C++ Program Examples For 2D Vectors
- How To Access & Modify 2D Vector Elements In C++?
- Methods To Traverse, Manipulate & Print 2D Vectors In C++
- Adding Elements To 2-D Vector Using push_back() Function
- Removing Elements From Vector In C++ Using pop_back() Function
- Creating 2D Vector In C++ With User Input For Size Of Column & Row
- Advantages of 2D Vectors Over Traditional Arrays
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- How To Print A Vector In C++ By Overloading Left Shift (<<) Operator?
- How To Print Vector In C++ Using Range-Based For-Loop?
- Print Vector In C++ With Comma Separator
- Printing Vector In C++ Using Indices (Square Brackets/ Double Brackets & at() Function)
- How To Print A Vector In C++ Using std::copy?
- How To Print A Vector In C++ Using for_each() Function?
- Printing C++ Vector Using The Lambda Function
- How To Print Vector In C++ Using Iterators?
- Conclusion
- Frequently Asked Questions
- Definition Of C++ Find In Vector
- Using The std::find() Function
- How Does find() In Vector C++ Function Work?
- Finding An Element By Custom Comparator Using std::find_if() Function
- Use std::find_if() With std::distance()
- Element Find In Vector C++ Using For Loop
- Using The find_if_not Function
- Find Elements With The Linear Search Approach
- Conclusion
- Frequently Asked Questions
- What Is Sort() Function In C++?
- Sort() Function In C++ From Standard Template Library
- Exceptions Of Sort() Function/ Algorithm In C++
- The Stable Sort() Function In C++
- Partial Sort() Function In C++
- Sorting In Ascending Order With Sort() Function In C++
- Sorting In Descending Order With Sort Function In C++
- Sorting In Desired Order With Custom Comparator Function & Sort Function In C++
- Sorting Elements In Desired Order Using Lambda Expression & Sort Function In C++
- Types of Sorting Algorithms In C++
- Advanced Sorting Algorithms In C++
- How Does the Sort() Function Algorithm Work In C++?
- Conclusion
- Frequently Asked Questions
- What Is Function Overloading In C++?
- Ways Of Function Overloading In C++
- Function Overloading In C++ Using Different Types Of Parameters
- Function Overloading In C++ With Different Number Of Parameters
- Function Overloading In C++ Using Different Sequence Of Parameters
- How Does Function Overloading In C++ Work?
- Rules Of Function Overloading In C++
- Why Is Function Overloading Used?
- Types Of Function Overloading Based On Time Of Resolution
- Causes Of Function Overloading In C++
- Ambiguity & Function Overloading In C++
- Advantages Of Function Overloading In C++
- Disadvantages Of Function Overloading In C++
- Operator Overloading In C++
- Function Overriding In C++
- Difference Between Function Overriding & Function Overloading In C++
- Conclusion
- Frequently Asked Questions
- What Is An Inline Function In C++?
- How To Define The Inline Function In C++?
- How Does Inline Function In C++ Work?
- The Need For An Inline Function In C++
- Can The Compiler Ignore/ Reject Inline Function In C++ Programs?
- Normal Function Vs. Inline Function In C++
- Classes & Inline Function In C++
- Understanding Inline, __inline, And __forceinline Functions In C++
- When To Use An Inline Function In C++?
- Advantages Of Inline Function In C++
- Disadvantages Of Inline Function In C++
- Why Not Use Macros Instead Of An Inline Function In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Static Data Member In C++?
- How To Declare Static Data Members In C++?
- How To Initialize/ Define Static Data Member In C++?
- Ways To Access A Static Data Member In C++
- What Are Static Member Functions In C++?
- Example Of Member Function & Static Data Member In C++
- Practical Applications Of Static Data Member In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constant In C++?
- Ways To Define Constant In C++
- What Are Literals In C++?
- Pointer To A Constant In C++
- Constant Function Arguments In C++
- Constant Member Function Of Class In C++
- Constant Data Members In C++
- Object Constant In C++
- Conclusion
- Frequently Asked Questions(FAQ)
- What Is Friend Function In C++?
- Declaration Of Friend Function In C++ With Example
- Characteristics Of Friend Function In C++
- Global Friend Function In C++ (Global Function As Friend Function )
- Member Function Of Another Class As Friend Function In C++
- Function Overloading Using Friend Function In C++
- Advantages & Disadvantages Of Friend Function in C++
- What Is A C++ Friend Class?
- A Function Friendly To Multiple Classes
- C++ Friend Class Vs. Friend Function In C++
- Some Important Points About Friend Functions And Classes In C++
- Conclusion
- Frequently Asked Questions
- What Is Function Overriding In C++?
- The Working Mechanism Of Function Overriding In C++
- Real-Life Example Of Function Overriding In C++
- Accessing Overriding Function In C++
- Accessing Overridden Function In C++
- Function Call Binding With Class Objects | Function Overriding In C++
- Function Call Binding With Base Class Pointers | Function Overriding In C++
- Advantages Of Function Overriding In C++
- Variations In Function Overriding In C++
- Function Overloading In C++
- Function Overloading Vs Function Overriding In C++
- Conclusion
- Frequently Asked Questions
- Errors In C++
- What Is Exception Handling In C++?
- Exception Handling In C++ Program Example
- C++ Exception Handling: Basic Keywords
- The Need For C++ Exception Handling
- C++ Standard Exceptions
- C++ Exception Classes
- User-Defined Exceptions In C++
- Advantages & Disadvantages Of C++ Exception Handling
- Conclusion
- Frequently Asked Questions
- What Are Templates In C++ & How Do They Work?
- Types Of Templates In C++
- What Are Function Templates In C++?
- C++ Template Functions With Multiple Parameters
- C++ Template Function Overloading
- What Are Class Templates In C++?
- Defining A Class Member Outside C++ Template Class
- C++ Template Class With Multiple Parameters
- What Is C++ Template Specialization?
- How To Specify Default Arguments For Templates In C++?
- Advantages Of C++ Templates
- Disadvantages Of C++ Templates
- Difference Between Function Overloading And Templates In C++
- Conclusion
- Frequently Asked Questions
- Structure
- Structure Declaration
- Initialization of Structure
- Copying and Comparing Structures
- Array of Structures
- Nested Structures
- Pointer to a Structure
- Structure as Function Argument
- Self Referential Structures
- Class
- Object Declaration
- Accessing Class Members
- Similarities between Structure and Class
- Which One Should You Choose?
- Key Difference Between a Structure and Class
- Summing Up
- Test Your Skills: Quiz Time
- What Is A Class And Object In C++?
- What Is An Object In C++?
- How To Create A Class & Object In C++? With Example
- Access Modifiers & Class/ Object In C++
- Member Functions Of A Class In C++
- How To Access Data Members And Member Functions?
- Significance Of Class & Object In C++
- What Are Constructors In C++ & Its Types?
- What Is A Destructor Of Class In C++?
- An Array Of Objects In C++
- Object In C++ As Function Arguments
- The this (->) Pointer & Classes In C++
- The Need For Semicolons At The End Of A Class In C++
- Difference Between Structure & Class In C++
- Conclusion
- Frequently Asked Questions
- What Are Static Members In C++?
- Static Member Functions in C++
- Ways To Call Static Member Function In C++
- Properties Of Static Member Function In C++
- Need Of Static Member Functions In C++
- Regular Member Function Vs. Static Member Function In C++
- Limitations Of Static Member Functions In C++
- Conclusion
- Frequently Asked Questions
- What Is Constructor In C++?
- Characteristics Of A Constructor In C++
- Types Of Constructors In C++
- Default Constructor In C++
- Parameterized Constructor In C++
- Copy Constructor In C++
- Dynamic Constructor In C++
- Benefits Of Using Constructor In C++
- How Does Constructor In C++ Differ From Normal Member Function?
- Constructor Overloading In C++
- Constructor For Array Of Objects In C++
- Constructor In C++ With Default Arguments
- Initializer List For Constructor In C++
- Dynamic Initialization Using Constructor In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constructor In C++?
- What Is Constructor Overloading In C++?
- Dеclaration Of Constructor Ovеrloading In C++
- Condition For Constructor Overloading In C++
- How Constructor Ovеrloading In C++ Works?
- Examples Of Constructor Overloading In C++
- Lеgal & Illеgal Constructor Ovеrloading In C++
- Types Of Constructors In C++
- Characteristics Of Constructors In C++
- Advantage Of Constructor Overloading In C++
- Disadvantage Of Constructor Overloading In C++
- Conclusion
- Frеquеntly Askеd Quеstions
- What Is A Destructor In C++?
- Rules For Defining A Destructor In C++
- When Is A Destructor in C++ Called?
- Order Of Destruction In C++
- Default Destructor & User-Defined Destructor In C++
- Virtual Destructor In C++
- Pure Virtual Destructor In C++
- Key Properties Of Destructor In C++ You Must Know
- Explicit Destructor Calls In C++
- Destructor Overloading In C++
- Difference Between Normal Member Function & Destructor In C++
- Important Uses Of Destructor In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constructor In C++?
- What Is A Destructor In C++?
- Difference Between Constructor And Destructor In C++
- Constructor In C++ | A Brief Explanation
- Destructor In C++ | A Brief Explanation
- Difference Between Constructor And Destructor In C++ Explained
- Order Of Calling Constructor And Destructor In C++ Classes
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Type Conversion In C++?
- What Is Type Casting In C++?
- Types Of Type Conversion In C++
- Implicit Type Conversion (Coercion) In C++
- Explicit Type Conversion (Casting) In C++
- Advantages Of Type Conversion In C++
- Disadvantages Of Type Conversion In C++
- Difference Between Type Casting & Type Conversion In C++
- Application Of Type Casting In C++
- Conclusion
- Frequently Asked Questions
- What Is A Copy Constructor In C++?
- Characteristics Of Copy Constructors In C++
- Types Of Copy Constructors In C++
- When Do We Call The Copy Constructor In C++?
- When Is A User-Defined Copy Constructor Needed In C++?
- Types Of Constructor Copies In C++
- Can We Make The Copy Constructor In C++ Private?
- Assignment Operator Vs Copy Constructor In C++
- Example Of Class Where A Copy Constructor Is Essential
- Uses Of Copy Constructors In C++
- Conclusion
- Frequently Asked Questions
- Why Do You Need Object-Oriented Programming (OOP) In C++?
- OOPs Concepts In C++ With Examples
- The Class OOPs Concept In C++
- The Object OOPs Concept In C++
- The Inheritance OOPs Concept In C++
- Polymorphism OOPs Concept In C++
- Abstraction OOPs Concept In C++
- Encapsulation OOPs Concept In C++
- Other Features Of OOPs In C++
- Benefits Of OOP In C++ Over Procedural-Oriented Programming
- Disadvantages Of OOPS Concept In C++
- Why Is C++ A Partial OOP Language?
- Conclusion
- Frequently Asked Questions
- Introduction To Abstraction In C++
- Types Of Abstraction In C++
- What Is Data Abstraction In C++?
- Understanding Data Abstraction In C++ Using Real Life Example
- Ways Of Achieving Data Abstraction In C++
- What Is An Abstract Class?
- Advantages Of Data Abstraction In C++
- Use Cases Of Data Abstraction In C++
- Encapsulation Vs. Abstraction In C++
- Conclusion
- Frequently Asked Questions
- What Is Encapsulation In C++?
- How Does Encapsulation Work In C++?
- Types Of Encapsulation In C++
- Why Do We Need Encapsulation In C++?
- Implementation Of Encapsulation In C++
- Access Specifiers & Encapsulation In C++
- Role Of Access Specifiers In Encapsulation In C++
- Member Functions & Encapsulation In C++
- Data Hiding & Encapsulation In C++
- Features Of Encapsulation In C++
- Advantages & Disadvantages Of Encapsulation In C++
- Difference Between Abstraction and Encapsulation In C++
- Conclusion
- Frequently Asked Questions
- What Is Inheritance In C++?
- What Are Child And Parent Classes?
- Syntax And Structure Of Inheritance In C++
- Implementing Inheritance In C++
- Importance Of Inheritance In C++
- Types Of Inheritance In C++
- Visibility Modes Of Inheritance In C++
- Access Modifiers & Inheritance In C++
- How To Make A Private Member Inheritable?
- Member Function Overriding In Inheritance In C++
- The Diamond Problem | Inheritance In C++ & Ambiguity
- Ways To Avoid Ambiguity Inheritance In C++
- Why & When To Use Inheritance In C++?
- Advantages Of Inheritance In C++
- The Disadvantages Of Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Hybrid Inheritance In C++?
- Importance Of Hybrid Inheritance In Object Oriented Programming
- Example Of Hybrid Inheritance In C++: Using Single and Multiple Inheritance
- Example Of Hybrid Inheritance In C++: Using Multilevel and Hierarchical Inheritance
- Real-World Applications Of Hybrid Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Multiple Inheritance In C++?
- Examples Of Multiple Inheritance In C++
- Ambiguity Problem In Multiple Inheritance In C++
- Ambiguity Resolution In Multiple Inheritance In C++
- The Diamond Problem In Multiple Inheritance In C++
- Visibility Modes In Multiple Inheritance In C++
- Advantages & Disadvantages Of Multiple Inheritance In C++
- Multiple Inheritance Vs. Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Multilevel Inheritance In C++?
- Block Diagram For Multilevel Inheritance In C++
- Multilevel Inheritance In C++ Example
- Constructor & Multilevel Inheritance In C++
- Use Cases Of Multilevel Inheritance In C++
- Multiple Vs Multilevel Inheritance In C++
- Advantages & Disadvantages Of Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Hierarchical Inheritance In C++?
- Example 1: Hierarchical Inheritance In C++
- Example 2: Hierarchical Inheritance In C++
- Impact of Visibility Modes In Hierarchical Inheritance In C++
- Advantages And Disadvantages Of Hierarchical Inheritance In C++
- Use Cases Of Hierarchical Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Are Access Specifiers In C++?
- Types Of Access Specifiers In C++
- Public Access Specifiers In C++
- Private Access Specifier In C++
- Protected Access Specifier In C++
- The Need For Access Specifiers In C++
- Combined Example For All Access Specifiers In C++
- Best Practices For Using Access Specifiers In C++
- Why Can't Private Members Be Accessed From Outside A Class?
- Conclusion
- Frequently Asked Questions
- What Is The Diamond Problem In C++?
- Example Of The Diamond Problem In C++
- Resolution Of The Diamond Problem In C++
- Virtual Inheritance To Resolve Diamond Problem In C++
- Scope Resolution Operator To Resolve Diamond Problem In C++
- Conclusion
- Frequently Asked Questions
Operator Overloading In C++ | Detailed Explanation +Code Examples
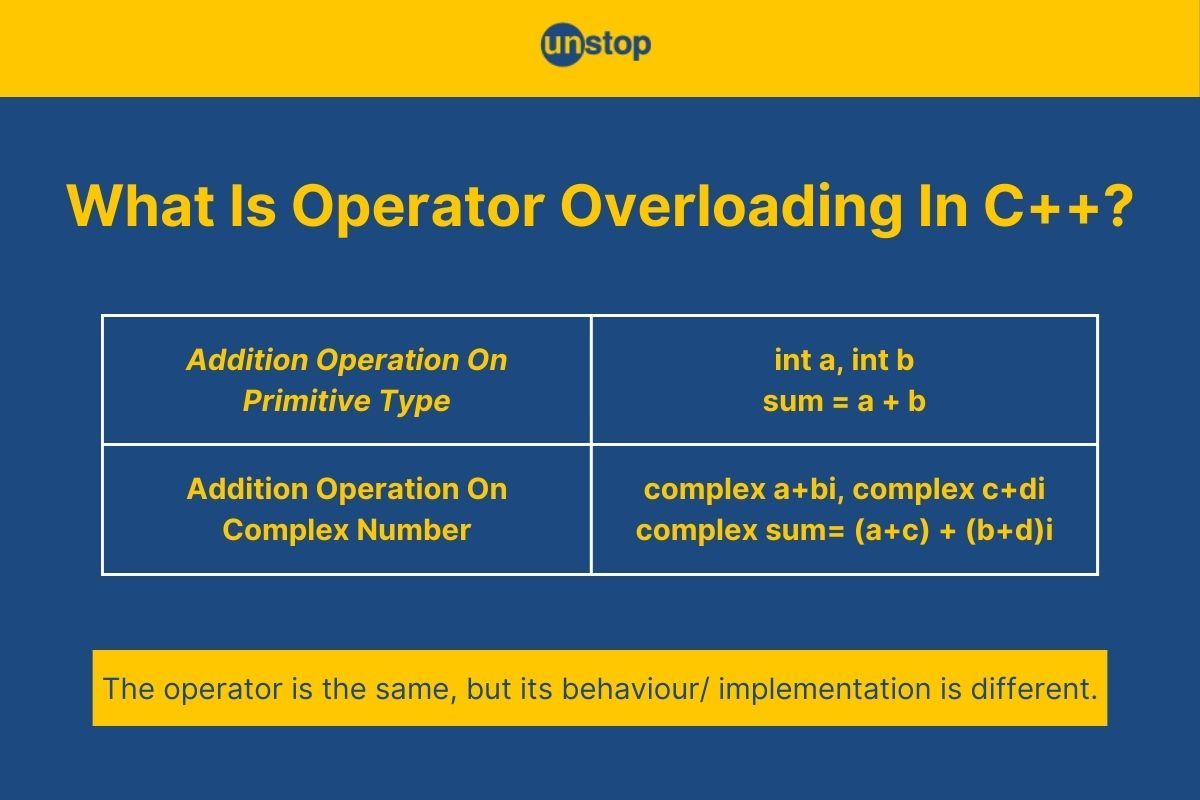
Overloading is an important component of object-oriented programming concepts. It entails redefining an operator or function to have special execution behaviour or use. In this context, operator overloading in C++ programming is a powerful feature that allows developers to redefine the behavior of operators for user-defined types, such as classes and structures. This ability enhances code readability and enables intuitive manipulation of objects in a manner similar to primitive data types.
By overloading operators, you can perform custom operations using familiar syntax, making your code more expressive and easier to understand. In this article, we will discuss everything about operator overloading in C++ with detailed code examples.
Types Of Overloading In C++
There are primarily two types of overloading in C++, including
- Function overloading
- Operator overloading
Both of these compile-time polymorphisms and the underlying mechanism remains the same. That is, we define two or more operators/ functions with the same name but different implementation is terms of operations to perform or parameters in intake. This difference in definition helps select the right behavioural implementation at execution time.
What Is Operator Overloading In C++?
We can easily apply C++ operators to primitive data types (like integers, floating point numbers, characters, etc.). However, to make these operators available for use with user-defined datatypes (like complex numbers and fractions), we need to define their operations explicitly.
- The concept of operator overloading in C++ allows us to extend the abilities of existing operators and give them special meaning while keeping their original meaning intact.
- In other words, operator overloading in C++ is the process of defining new behaviors for existing operators when they are applied to user-defined types.
- This feature allows you to define how operators like arithmetic addition (+), subtraction (-), multiplication (*) operators, and equality relational operators (==), etc., work with objects of your classes or structures.
- This enables you to perform operations on these objects in a natural and intuitive way.
- The three types of operators used for operator overloading in C++ are unary, binary, and special operators.
Let's consider the addition arithmetic operator, for example. In normal arithmetic terms, it adds two numbers. However, if you were to define a custom class representing a complex number, you might want the + operator to add two complex numbers.
- If you have two complex numbers (a+bi) and (c+di), then you would want the addition operator (+) to perform the operation (a+c)+(b+d)i on the numbers.
- For this, you will have to define the functionality, where the basic function is still the same, i.e., addition. But you are adding the real parts and imaginary parts of the numbers together.
In a real-world context, think of operator overloading as customizing a tool for a specific job. Imagine you have a specialized screwdriver designed for a unique type of screw. While the screwdriver's primary function is to turn screws, overloading would be like modifying the screwdriver to fit and turn the unique screw properly.
How To Overload An Operator In C++?
For operator overloading in C++ language, we must define a special operator function inside a class or the structure whose objects/variables we want the overloaded operator to work on. This function can be a member function of a class or a friend function (non-member function).
We must use the operator keyword followed by the operator symbol that we want to overload. The syntax for the same is given below.
Syntax Of Operator Overloading In C++
class className {
public:
return_DataType operator symbol (parameter_list) {
//implementaion
}
//Lines of code
};
Here,
- The class keyword marks beginning of class definition with the name className, and public is an access specifier that controls the level of visibility for class elements.
- return_DataType is the data type of the return value that the function will generate.
- The operator keyword indicates that we are overloading an operator, and the symbol refers to the sign/ symbol of the respective operator we want to overload (i.e., +, <, -, ++, etc.).
- The parameter_list refers to the parameters that the function intakes, and the implementation of the operation is to be provided in place of the code comment.
An alternative method of operator overloading in C++ is to define the overloading special function as a friend function using the friend keyword. This is a special non-member function that has access to the class's private & protected members. However, the member function approach is more commonly used for operator overloading in C++.
Operators Overloading In C++ Example
Let's look at a C++ program example illustrating the overloading of the addition arithmetic operator.
Code Example:
// A program to add two complex numbers using operator overloading
#include <iostream>
using namespace std;
class Complex {
private:
float real;
float imag;
public:
Complex(float r = 0.0, float i = 0.0) : real(r), imag(i) {}
Complex operator+(const Complex& other) {
Complex sum;
sum.real = real + other.real;
sum.imag = imag + other.imag;
return sum;
}
void display() {
if (imag < 0)
cout << "Complex number: " << real << imag << "i" << endl;
else
cout << "Complex number: " << real << "+" << imag << "i" << endl;
}
};
int main() {
Complex c1(2.5, 3.0);
Complex c2(1.2, -0.8);
Complex sum = c1 + c2;
sum.display();
return 0;
}
Output:
Complex number: 3.7+2.2i
Explanation:
In the C++ code example, we first include the <iostream> header file for input/ output operations and use the namespace std.
- We then define a class called complex with private float data type member variables real and imag, representing the real and imaginary parts of a complex number, respectively.
- Then, we have a parameterized class constructor to initialize the real and imag members with default values of 0.0 if no arguments are provided.
- Next, we define a member function to overload the addition operator (+) that takes a constant reference to a complex object other as the parameter.
- Inside, we first create a new complex object called sum.
- It then adds two complex objects together by adding the real and imaginary parts of the current object with those of the other object.
- The addition of the real and imaginary parts are stored in the real and imaginary parts of the sum object, which the function returns.
- That is, the real parts of the two complex numbers (real of the current object and real of the other object) are added and assigned to the real member of the sum object.
- Similarly, the imaginary parts of the two complex numbers (imaginary of the current object and imaginary of the other object) are added and assigned to the imaginary member of the sum object.
- Then, we define a member function called display() to print the complex number in the format real+imaginary(i), where i represents the imaginary unit.
- This function uses the if-else statement to check if the imaginary part is negative and adjusts the output accordingly.
- In the main() function, we create two objects of class complex called c1 and c2 with values (2.5, 3.0) and (1.2, -0.8), respectively. Note that since we have provided value, the constructor body is not implemented.
- Next, we create another Complex class object sum and assign it the total of the other two objects. Here, we use the overload operator (+) to add c1 and c2.
- After that, we use the dot operator to call the display() function on the sum object to print the sum of the complex numbers.
- Finally, the main() function terminates with a return 0 statement.
Overloadable & Non-overloadable Operators In C++
We now know what operator overloading in C++ is, but do you wonder if all operators can be overloaded? The answer to this question is that almost all operators can be overloaded except for a few shown in the table below:
Non-Overloadable Operators |
Symbols |
Scope Resolution Operator |
:: |
Ternary/ Conditional Operator |
?: |
Member Selector Operator |
. |
Sizeof Operator |
sizeof() |
Member Pointer Selector Operator |
* |
Nearly all operators except the ones listed above can be overloaded. We have compiled that information in the table below for convenience.
Overloadable Operators |
Symbols |
Unary Arithmetic Operators |
+, -, ++, — |
Binary Arithmetic Operators |
+, -, *, /, % |
Assignment Operator & Compound Assignment Operators |
=, +=,*=, /=,-=, %= |
&, | |, ! |
|
Relational Operators |
>, < , = =, <=, >= |
& , | , << , >> , ~ , ^ |
|
De-referencing Operator/ Member Access Operator |
->, .,* |
Subscript Operator |
[ ] |
Operator for Dynamic Memory Allocation & |
new(), delete() |
Function call |
() |
In the sections ahead, we will discuss operator overloading for three different types of operators, i.e., unary, binary, and special.
Unary Operator Overloading In C++
Operators that can operate only on a single operand are referred to as unary operators. For example, the increment operator (++) and the decrement operator (--) are unary operators since they have only one operand. We can overload these operators, as shown in the sample ahead.
Example Of Unary Operator Overloading In C++
In the example below, we have illustrated how to overload the pre-increment operator (++). We can follow the same process for the decrement operator and the suffix type of both operators.
Code Example:
// Overload ++ increment operator when used as a prefix
#include <iostream>
using namespace std;
class Counter {
private:
int num;
public:
Counter() : num(9) {} // Initializing counter to 9 using the Constructor
// Overload ++ when operator used as prefix
void operator ++ () {
++num;
}
void print() {
cout << "Counter: " << num << endl;
}
};
int main() {
Counter count;
// Call the overloaded operator ++ function
++count;
count.print();
return 0;
}
Output:
Counter: 10
Explanation:
In the C++ sample code-
- We create a class called Counter with a private data member num (integer type) and a public class constructor to initialize it with default value 9.
- Then, we define a member function to overload the increment operator (++) as a prefix or pre-increment operator.
- Inside this function, we first increment the value of variable num by 1 (since it is a prefix operator). That is, whenever this operator is used with an operand or variable, the value of the respective variable is increased by 1.
- Then, we have a void function print(), which uses the cout statement to print the value of the num data member/ variable of the class.
- In the main() function, we first create a Counter class object called counter without initialization. Since we do not assign a value, the class constructor assigns the default value 9 to it.
- Next, we use the overloaded pre-increment operator on the counter object. This calls/ invokes the overloaded operator ++ function, which increments the value of the count object.
- Then, we call the print() function on the counter object using the dot operator to output its value to the console.
Example 2: Return Value Of Overloaded Operator Function (++ Operator)
In this example, we again overload the increment operator, but this time, we use both its prefix and suffix forms. The same can be applied to the prefix decrement operator and its suffix form.
Code Example:
#include <iostream>
using namespace std;
class Counter {
private:
int num;
public:
Counter() : num(9) {}
Counter operator ++ () {
Counter temp;
temp.num = ++num;
return temp;
}
Counter operator ++ (int) {
Counter temp;
temp.num = num++;
return temp;
}
void print() {
cout << "Count: " << num << endl;
}
};
int main() {
Counter count, output;
output = ++count;
cout<<"Output after pre-increment:";
output.print();
output = count++;
cout<<"Output when applying post-increment:";
output.print();
output = count;
cout<<"Output after post-increment has been applied:";
output.print();
return 0;
}
Output:
Output after pre-increment:Count: 10
Output when applying post-increment:Count: 10
Output after post-increment has been applied:Count: 11
Explanation:
In the example C++ code-
- Just like in the previous example, we create the Counter class with private data member num and a class constructor that initializes it with the default value 9.
- We define two member functions to overload the increment operator (++), but the difference is one uses it as a prefix and the other as a suffix.
- The first operator++() function overloads the pre-increment operator as follows-
- First, we define the function to create a class object temp and then increment the value of num by 1.
- After that, the function returns the temp object with the incremented value of num.
- The second operator++() function overloads the post-increment operator-
- We define the function to first create a Counter object called temp with the current value of num.
- Since we are using the post-increment operator, the value of num is incremented by 1 afterwards, and the function returns the temporary object with this value.
- Then, we have a void print() function, which displays the value of the class data member num using the cout statements.
- Moving on, in the main() function, we create two objects of the Counter class called count and output without initialization.
- Since we did not assign a value, the class constructor initializes both with the data member value 9.
- Note that whenever we use the increment operator on class objects, the Counter class will implement the functions defined in its scope according to the argument or parameter matching.
- If the prefix operator is used, the value is increased before assigning to the output variable.
- If postfix is used, then the value of the count is increased after assigning to the output. Therefore, the change is seen in the next assignment.
- Next, we use the pre-increment operator on the count object and assign that value to the output object.
- As per the function definition in the class, the value of the count object is incremented by 1 and then assigned to the output object.
- Then, we call the print() function on the output object to display the value after pre-increment is applied.
- After that, we apply the post-increment operator on count object and assign the value to output.
- As per the definition, the value of count is assigned to output before increment. So, when we display this value using the print(), the value has not been increased.
- However, when we call the print() function on the output object after that, the value as shown in the output console has increased by 1.
Binary Operator Overloading In C++
The operators that work with two operands to carry out an operation are referred to as binary operators. For example, addition, subtraction, and so on. Look at the example below where we overload the less than and greater than relational operators, to compare the area of squares.
Code Example:
#include <iostream>
using namespace std;
class Square {
public:
int len;
Square(int _len) {
len = _len;
}
int area() {
return len * len;
}
bool operator > (Square a) {
if (area() > a.area()) return true;
return false;
}
bool operator < (Square a) {
if (area() < a.area()) return true;
return false;
}
};
int main() {
Square s1(4);
Square s2(3);
if (s1 > s2) {
cout << "The area of square S1 is larger than area of square S2\n";
}else if (s1 < s2) {
cout << "The area of square S1 is smaller than area of square S2\n";
}else {
cout << "The area of square S1 is equal to area of square S2\n";
}
s2.len = 5;
if (s1 > s2) {
cout << "The area of square S1 is larger than area of square S2\n";
}else if (s1 < s2) {
cout << "The area of square S1 is smaller than area of square S2\n";
}else {
cout << "The area of square S1 is equal to area of square S2\n";
}
return 0;
}
Output:
The area of square S1 is larger than area of square S2
The area of square S1 is smaller than area of square S2
Explanation:
In the example-
-
We first define a class Square representing a square with a single public member len which represents the length of a side of the square.
-
Inside the class, we have a constructor, i.e., Square (int _len), which initializes the len member variable with the value passed to the constructor.
- Then, we have a member function area() that multiplies the len variable by itself to calculate the areas of the square and return the same.
- After that, we define a function to overload the greater than operator (>).
- Inside the function, we use > to compare the area of the current square with another square a.
- It returns true if the current square's area is greater than the area of a.
- If the condition is false, then it returns false.
- Similarly, we define a function to overload the less than operator (<) where it compares the area of the current square with the area of a as follows:
- It returns true if the condition area() < a.area() is met.
- If the condition is not met, it returns false.
- In the main() function, we create two objects of the Square class, s1 with side length 4 and object s2 with side length 3.
- Then, we use an if-else ladder conditional statement to compare the areas of these two squares.
- The program flow first moves to the if condition, s1 > s2 to check if area of s1 is greater than s2. If this is true, the function prints a string message- "The area of square S1 is larger than area of square S2".
- If the condition is false, we move to the next if-condition, s1 < s2, to check if the area of s1 is smaller than s2. If true, the function prints the corresponding message.
- If both conditions are false, the function prints the message in the last else block- "The area of square S1 is equal to the area of square S2".
- After that, we modify the side length of s2 object and assign 5 to it. Now the area os s1 is still 16, but that of s2 is 25.
- We again repeated the comparison process. Since the area of s1 is smaller than the area of s2, the function prints the message - "The area of square S1 is smaller than the area of square S2".
Special Operator Overloading In C++
Besides the unary and binary operators, there are some special operators which may not be used in the traditional sense. For example, the new and delete operators are used for dynamic memory allocation.
The act of overloading these operators can extensively increase their use case. Listed in the table below are special operators that can be overloaded in C++, followed an example.
Operator | Description |
new | This operator is used to allocate memory dynamically. |
delete | This operator is used to free dynamically allocated memory. |
[] |
Subscript operator is used for array declaration and indexing to access array elements. |
() |
The function call operator is used to call a function object as if it were a function. |
-> | Member class operators or 'this' operator is used to access members of a class or structure through a pointer variable. |
In the sample C++ program below, we have illustrated the process of overload the subscript operator []. This example shows how the [] operator can be overloaded to allow intuitive access to elements within a custom array-like class.
Code Example:
#include <iostream>
#include <vector>
class IntArray {
private:
std::vector<int> arr;
public:
IntArray(std::initializer_list<int> init_list) : arr(init_list) {}
int& operator[](size_t index) {
return arr[index];
}
const int& operator[](size_t index) const {
return arr[index];
}
};
int main() {
IntArray myArray = {1, 2, 3, 4, 5};
myArray[2] = 10; // Using overloaded [] operator
std::cout << "Element at index 2: " << myArray[2] << std::endl; // Output: Element at index 2: 10
return 0;
}
Output:
Element at index 2: 10
Explanation:
We begin the example C++ program by including the <iostream> header file for input and output operations, and the <vector> header to use the std::vector class for storing elements.
- Then, we define a class IntArray with a private member arr, which is an vector (i.e., std::vector<int>) used to store integer elements.
- We then have a constructor that initialises the class object, i.e., arr vector, with a list of integers.
- Next, we define two member functions to overload the subscript operator, once as a non-const, and then as a constant.
- The non-const overloaded subscript operator allows non-const access to the elements of the arr vector. It returns a reference to the element at the specified index, allowing modification of the element.
- The const overloaded subscript operator allows const access to the elements of the arr vector. It returns a const reference to the element at the specified index, ensuring the element cannot be modified through this reference.
- This overload is necessary to support the use of the subscript operator on const instances of IntArray.
- In the main() function, we create an object of IntArray class called myArray and initialize it with the list of integers {1, 2, 3, 4, 5}.
- Then, we use the overloaded operator [] to modify the value at index position 2, and assign 10 to it.
- Next, we use cout statements to print this value, and the output shows that the value has infact changed.
- This indictes that we can overload the subscript operator to alter its behaviour such that it can help us access the elements of the vector in the same intuitive ways as in case of arrays.
Rules For Operator Overloading In C++
- Operators (=) and (&) are overloaded by default in C++. For example, if we want to copy objects of the same class, we directly use the assignment operator (=) instead of creating an operator function.
- Operator overloading in C++ does not change the precedence or the associativity of operators, but we can control the order of evaluation with the use of parenthesis.
- Only existing operators can be overloaded, and some of them have to be overloaded using the member function since it can not be done using the friend function.
- Operator overloading in C++ can only be done for user-defined types ( classes and objects) and not for built-in types(char, int, float, etc.).
- At least one operand must be a user-defined class object.
Advantages And Disadvantages Of Operator Overloading In C++
Listed in the table below are some advantages and disadvantages of operator overloading:
Advantages | Disadvantages |
|
|
Function Overloading In C++
Function overloading is a concept in which different functions can have the same name but with different function prototype, i.e., arguments, parameters, datatype, order, and use.
- Just like operator overloading in C++, this is also a compile-time polymorphism and is one of the salient features of the language.
- Having the same name function with different arguments, etc. increases the readability of the program.
For example, suppose we have to add two given numbers which can be of any data type. Then, writing different functions for different types, like- add_int( int a, int b), add_float(float a, float b), etc., can be tedious and lead to errors or unexpected behavior. Here, function overloading can help as it lets us use the same name for the functions with different parameters, like data types.
As discussed before, the compiler can differentiate between the functions based on the parameters/ arguments. Note that for function overloading to work, one or all of the following conditions must be met:
Parameters should have a different type. For example:
add(int a, int b)
add(float a, float b)
Parameters should have a different number of arguments. For example:
add(int a, int b)
add(int a, int b, int c)
Parameters should have a different order of parameters. For example:
add(int a ,float b)
add(float a, int b)
For more, read: Function Overloading In C++ With Code Examples & Explanation
What Is the Difference Between Operator Functions and Normal Functions?
Although operator functions are similar to normal functions, there are some differences between them. The main difference between the two functions is the keyword operator and the operator symbol that is written in operator functions in place of a function name in normal functions.
Here's a table summarizing the differences between operator functions and normal functions:
Operator Functions | Normal Functions | |
---|---|---|
Invocation | Invoked using operators | Called using the function name |
Syntax | Specific syntax based on operators | Follows regular function syntax |
Context | Associated with specific operations | General-purpose functions |
Overloading | It can be overloaded for different operand combinations or types | It can be overloaded based on parameter types |
Return Type | Often have a specific return type | Can have any return type |
Implicit Object | Member functions have an implicit object parameter representing the object on which the operator is applied. | Do not have an implicit object parameter |
Syntax Variations | Syntax requirements based on the operator being overloaded (e.g., unary, binary) | More flexibility in parameter types, numbers, and order |
Take a look at the piece of code below to gain a better understanding of the same:
Normal Function -
return_DataType function_name(arguments){
// Do something.
:::::::::::::::::::::::::
// Return something.
}
Operator Function -
return_DataType operator + (arguments){
// Do something.
:::::::::::::::::::::::::::
// Return type something.
}
Note that addition arithmetic operator (+) can be replaced by other valid operators, i.e., not non-overlodable operators.
Conclusion
Operator overloading in C++ is a runtime polymorphism concept that helps increase the use case or functionality of a built-in operator by redefining their behaviour.
- However, it is important to note that only the built-in operator can be overloaded, and no new operator can be overloaded.
- Overloading operators in C++ or any other language can largely improve the readability of the code.
- Almost all the operators can be overloaded in C++ except for the scope operator (::), pointer operator (.*), dot operator notation (.), and ternary operator (?:).
- Operator overloading can only define an operator's operation; its precedence can't be changed.
- We must make use of the parentheses to change the associativity or precedence between operators.
Frequently Asked Questions
Q. What is operator overloading in C++? Explain with an example.
Operator ovelroading is the process of redefining the behavior of operators when they are to be applied on user-defined data types.
- Say for example, you want to perform addition on complex numbers, the regular addition arithmetic operator cannot work in the traditional sense.
- You will have to define its behavior to add real and imaginary parts individually.
- This process of redefining the implementation behaviour of an operator is referred to as operator overloading in C++ programming.
- It is a compile-time polymorphism that allows us to extend the abilities of existing operators.
Code Example:
// Binary operator (-) overload example
// A program for operator overloading to subtract two complex numbers
#include <iostream>
using namespace std;
class Complex {
private:
float real;
float imag;
public:
Complex(float r = 0.0, float i = 0.0) : real(r), imag(i) {}
Complex operator-(const Complex& other) {
Complex diff;
diff.real = real - other.real;
diff.imag = imag - other.imag;
return diff;
}
void display() {
if (imag < 0)
cout << "Complex number: " << real << imag << "i" << endl;
else
cout << "Complex number: " << real << "+" << imag << "i" << endl;
}
};
int main() {
Complex c1(5.0, 3.5);
Complex c2(2.5, 1.8);
Complex diff = c1 - c2;
diff.display();
return 0;
}
Output:
Complex number: 2.5+1.7i
Explanation:
In the given program-
- The Complex class is defined with private member variables real and imag, representing the real and imaginary parts of a complex number, respectively.
- The constructor of the Complex class initializes the real and imag members with default values of 0.0 if no arguments are provided.
- The operator- function is overloaded as a member function to subtract one Complex object from another. It takes a constant reference to another Complex object (other) as the parameter.
- Inside the operator- function, a new Complex object diff is created to store the difference of the complex numbers. The real parts of the two complex numbers (real of the current object and real of the other object) are subtracted, and the result is assigned to the real member of the diff object.
- Similarly, the imaginary parts of the two complex numbers (imag of the current object and imag of the other object) are subtracted, and the result is assigned to the imag member of the diff object.
- The diff object is then returned.
- The display function is defined to print the complex number in the format real+imaginaryi, where i represents the imaginary unit.
- In the main function, two Complex objects, c1 and c2, are created and initialized with different real and imaginary values.
- The - operator is used to subtract c2 from c1, and the result is stored in the diff object.
- Finally, the display function is called on the diff object to print the difference of the complex numbers.
Q. What are logical operators in C++ with examples?
Logical operators are those that compare and connect various expressions such that the value of the whole expression is dependent on the original expression, variable, and values. While relational operators can only check the relation between two particular variables or values, with logical operators, we can check various conditions simultaneously.
In other words, logical operators provide us with the ability to test multiple conditions at the same time. The general syntax for the use of logical operators is given below.
Syntax:
Operand1 logical_operator operand2 ;
Here, operand1 and operand2 are the two expression which can be any boolean conditions made up using other operators (like relational). And the logical_operator is the respective operator we are using to connect and compararative expressions. The various types of logical operators are listed in the following table:
Operator |
Name |
Form |
&& |
Logical AND |
a && b |
|| |
Logical OR |
a || b |
! |
Logical NOT |
!a |
Q. Is operator overloading a type of polymorphism?
Yes. Operator Overloading in C++ is a compile-time polymorphism that allows us to extend the abilities of existing operators and give special meaning to them while keeping their original meaning intact. Here the name or symbol of the operator remains the same, but the parameters required and implementation/ behaviour change.
Q. Is operator overloading a type of method overloading?
No, they are two distinct concepts. Operator overloading and method overloading may seem similar, there is a prominent point of difference.
- Operator overloading overloads the built-in operators to provide the specific implementation for user-defined data types without changing their original meaning.
- Whereas method/ function overloading refers to the overloading of two or more regular functions (function operator) with the same name but different numbers, datatype, and order of the parameters.
Q. What are the types of operator overloading?
The three types of operators used for operator overloading in C++ are:
- Unary: These operators can operate only on one operand. For example, the increment (++) and decrement (--) operators.
- Binary: These operators need two operands to carry out an operation. For example, arithmetic, logical relational, and other operators like addition, greater than, etc.
- Special operators: Some special operators in C++ are new, delete, [], (), and (-->) operators.
Q. Provide an example of operator overloading in Cpp.
Let’s take an example of how can we overload the logical NOT binary operator (!) and extend its ability to help us find the conjugate of a complex number.
Code Example:
// Logical NOT operator (!) overloaded to conjugate a complex number
#include <iostream>
using namespace std;
class Complex {
private:
float r; //real part of complex number
float i; //imaginary part of complex number
public:
Complex() : r(0), i(0) {} // Constructor to initialize real and imaginary parts to 0
void input() {
cout << "Enter real and imaginary parts:\n ";
cout<< "real:";
cin >> r;
cout<< "\n imaginary:";
cin >> i;
}
Complex operator ! () {
Complex num;
num.r = r ;
num.i = -1 * i;
return num;
}
void print() {
cout << " Complex number: " << r << "+" << i << "i";
}
};
int main() {
Complex comp1, comp2, result;
cout << "Enter first complex number:\n";
comp1.input();
result = comp1;
result.print();
result = ! comp1;
cout<<"\nConjugate";
result.print();
return 0;
}
Output:
Enter first complex number:
Enter real and imaginary parts:
real:7
imaginary:3
Complex number: 7+3i
Conjugate Complex number: 7+-3i
Explanation:
In the given program
- The Complex class is defined with private member variables r and i, representing the real and imaginary parts of a complex number, respectively.
- The default constructor initializes the real and imaginary parts to 0.
- The input function prompts the user to enter the real and imaginary parts of the complex number and stores them in the corresponding member variables.
- The operator! function is overloaded as a member function to find the conjugate of a complex number. It creates a new Complex object, copies the real part (r) of the current object, and assigns the negation of the imaginary part (i) to the new object's imaginary part.
- The print function is defined to display the complex number in the format real+imaginaryi.
- In the main function, three Complex objects (comp1, comp2, and result) are created. The user is prompted to enter the first complex number using the input function, and the print function is called on the result object to display the complex number.
- The operator! is used to find the conjugate of comp1, and the result is assigned to the result object.
- Finally, the print function is called on the result object to display the conjugate of the complex number.
Q. What is the list of operators in C++ programming language?
The most common forms of operators in C++ programming are
- Arithmetic Operators: They are used to perform common arithmetic operations.
- Assignment Operators: They perform variable initialization or value assignment to other variables.
- Comparison Operators: Perform common relational operations generating Boolean-type values.
- Logical Operators: They are used to perform logical calculations or special operations on one or more operands. For example, AND, OR, and NOT.
- Bitwise operators: They perform operations on the bit level of the operands, i.e., they perform Bitwise operations.
Operators |
Symbols |
Arithmetic |
+, -, ++, —,*, /, % |
Assignment |
=, +=,*=, /=,-=, %= |
Logical |
&, | |, ! |
Comparison |
>, < , = =, <=, >= |
Bitwise |
& , | , << , >> , ~ , ^ |
Q. What are the two types of overloading in C++?
The two types of overloading are function overloading and operator overloading in C++. Both of these overloadings are a type of compile-time polymorphism wherein the name of the function/ operator remains the same, but the parameters required, implementation, and the use case change.
- Function Overloading is when different functions have the same name but different arguments, parameters, datatype, order, and use.
- Operator Overloading in C++ allows us to extend the abilities of the existing operators to be applied with user defined data types like complex numbers, arrays, structures, class objects, etc.
Test Your Skills: Quiz Time
Do check out the following:
-
- C++ 2D Array & Multi-Dimensional Arrays Explained (+Examples)
- Typedef In C++ | Syntax, Application & How To Use (+Code Examples)
- C++ Templates | Types, Usage, Overloading & More (+Code Examples)
- Static Member Function In C++: How to Use Them, Properties, & More
- 25+ Difference Between C And C++ | Similarities, Features & More
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Kartik Deshmukh 3 weeks ago
YASH SRIVASTAVA 1 month ago
Avinash Mishra 1 month ago
Sanchit Dhale 1 month ago