25+ Difference Between C And C++ | Similarities, Features & More!
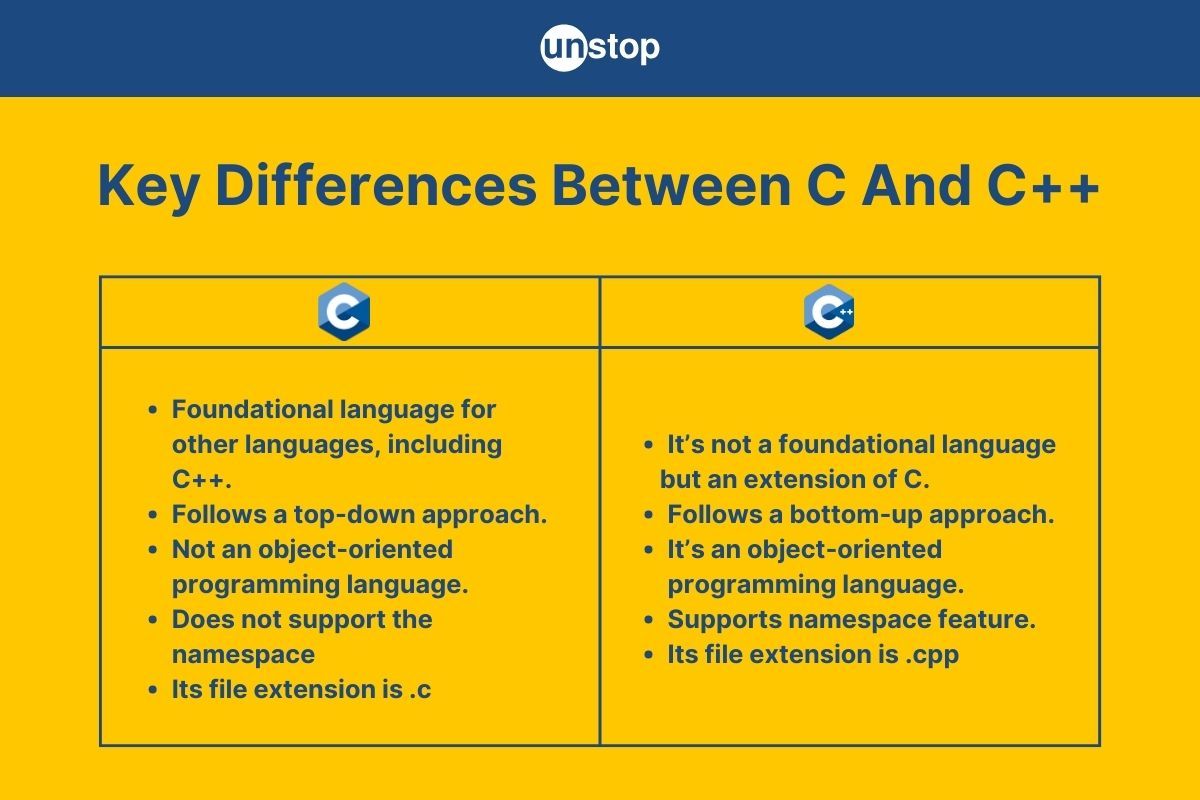
Two of the most popular and widely used programming languages, C and C++, are the centre of many discussions. One of the most important discussions revolves around the difference between C and C++. There are many people out there who get confused between these languages, which leads them to assume that they are the same. But that ain't true!
In this article, we will discuss everything about C vs. C++ by exploring the differences between C and C++, their features, similarities, and more.
The Difference Between C and C++ Languages
Before we begin looking at the differences between C and C++, it is important to note that as the languages have evolved over the years, so have the differences between the two. That is, when C++ was originally created, it was considered a superset of C (this may be so even today). However, as things evolved, the C programming language took on numerous additional features. Not all of these features have been adapted in C++, which shows that there are numerous prominent and subtle differences between the two languages.
That being said, let's move forward with the discussion. In the table below is a snapshot view of the difference between C and C++:
Basis | C | C++ |
Origin | Developed in 1972-73 at Bell Labs by Dennis Ritchie. | Developed at Bell Labs in 1979 by Bjarne Stroustrup. |
Basic type | It is a combination of a function-driven language and a procedural programming language. | It is a combination of object-driven language and procedural language. It is also considered a hybrid language (procedural and object-oriented programming language). |
Set | It is a subset (for the most part) of C++. | It is a superset (for the most part) of C. |
Foundational | C is the foundational language for many other languages, including C++. | Is not the foundational language, rather is an extension of C. |
Keywords | Has approximately 32 keywords. | Has approximately 60 keywords. |
Object-oriented | It is not an object-oriented programming language, i.e., it does not support polymorphism, encapsulation, and inheritance. | It is an object-oriented programming language, i.e., it supports concepts of classes and objects, as well as polymorphism, encapsulation, and inheritance. |
Data and Functions | Data and functions are separated in this procedural programming language | Data and functions are instead encapsulated in objects in this object-oriented language. |
Approach | It follows a top-down approach i.e. programmers first develop the main module of the program and then the next modules. | It follows a bottom-up approach which means bottom-level modules are developed first then upper levels. It is helpful for the reusability of code. |
Security Features | C is not a secure language since the absence of data encapsulation and hiding features makes it vulnerable to outside manipulation. | C++ is a secure language since it has security features like encapsulation and data hiding. |
Programs | The program is divided into smaller parts known as functions. | Programs are divided into objects and classes in this OOP language. |
File Extension | Its file extension is .c | Its file extension is .cpp |
Compatibility | It is not compatible with other generic programming languages. | It is compatible with other generic programming languages. |
Focus | Focuses on method/ process rather than data. | Focuses on data rather than process. |
Namespace | Does not support the namespace feature. | It supports the namespace feature hence reducing the possibility of name collision. |
Overloading | Does not support function overloading and operator overloading. | Supports function and operator overloading. |
Data types | Supports only built-in data types i.e. does not allow string or boolean data type. | Supports both built-in and user-defined data types i.e. allows boolean data type as well as other generic data. |
Reference variables | It does not support the concept of reference variables. | It supports the concept of reference variables. |
Inline functions | Does not support inline functions. | Supports inline functions. |
Header files | The IO header in C is <stdio.h> | The IO header in C++ is <iostream.h> |
Friend/ Virtual Functions | C supports neither virtual nor friend functions. | C++ supports both friend functions as well as virtual functions. |
Allocation of memory | It uses calloc() and malloc() for dynamic memory allocation. | It uses new() and free() for dynamic memory allocation. |
Memory de-allocation | C uses free() for memory de-allocation. | C++ uses the delete operator for memory de-allocation. |
Variables in dynamic memory allocation |
Variables are stored in RAM. | Variables are stored in heap. |
Input/Output Functions | It uses scanf and printf as standard input-output functions. | It uses cin and cout as standard input-output functions. |
Exception handling | It does not support exception handling. | It supports exceptional handling. |
Type checking | There is no strict type checking in C. | There is strict type checking in C++. |
Access modifiers | In C, structures do not have any access modifiers. | Structure access modifiers are present in C++. |
It is evident that while both languages were developed at Bell Laboratories, there are many C and C++ differences that you must know about.
Key Difference Between C And C++ Explained
1. Object Oriented Programming (OOP):
- C is a procedural programming language and does not support OOP concepts natively. It lacks features such as classes, objects, inheritance, polymorphism, and encapsulation.
- C++ is designed as a multi-paradigm language and fully supports OOP concepts. It allows the creation of classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
2. Overloading:
- C does not support function overloading, which means you cannot define multiple functions with the same name but different parameter lists.
- C++ supports function overloading, enabling you to define multiple functions with the same name but different parameter lists. This feature allows for more expressive and readable code.
3. Memory Allocation and Deallocation:
- In C, memory allocation and deallocation are typically managed using functions like malloc, calloc, realloc, and free from the <stdlib.h> library.
- C++ provides additional memory management features through the use of the new and delete operators. These operators are used to dynamically allocate and deallocate memory for objects. Additionally, C++ introduces the concept of constructors and destructors, which are automatically called when objects are created and destroyed, respectively.
4. Exception Handling:
- C does not have built-in support for exception handling. Error handling in C is typically done using error codes or return values to indicate errors.
- C++ supports exception handling using try, catch, and throw keywords. Exceptions provide a way to handle runtime errors and exceptional conditions in a structured manner, allowing for cleaner and more robust error handling compared to traditional error code-based approaches.
5. Access Modifiers:
- C does not have access modifiers such as public, private, and protected.
- C++ provides access modifiers to control the accessibility of class members. By default, class members are private in C++, meaning they can only be accessed within the class.
Now, let's have a look at a brief description of the languages before moving on to discuss the similarities they share.
What is C Programming Language?
C is a procedural/ structured language developed at Bell Laboratories some 50 years ago and is one of the most popular programming languages even today. This basic programming language is machine-independent and has numerous applications across operating systems (like iOS, Windows, etc.) and simple as well as complex programs (like Git, Oracle database, etc.).
Fun fact: C is referred to as a 'God' language since it forms the basis for many other languages like C++.
Features of C Programming Languages
- The syntax of C language is very simple and, hence, easy to learn and implement, thus making it efficient and fast.
- It is considered a middle-level language since it comprises both high-level and low-level language features.
- It is a compiler-based language, which implies that the speed of code compilation and execution is high.
- It is portable, i.e., machine-independent, and can be run across hardware architectures and platforms.
- C is easily extendible, i.e., it is easy to modify and extend the code written in this language to add new features and functionality to the program.
- It comes with extensive function-rich libraries that support and make the job of developers easier when working with C programs.
Applications of C
Some of the applications of this structural programming language are:
Operating system development | Kernels development |
Compiler development | GUI design |
Embedded systems | Game development |
System development | Web browser design |
Assembly language code |
Code Example:
Here is an example code for a basic C program that calculates the sum of two numbers:
Output:
Enter the first number: 10
Enter the second number: 20
The sum of 10 and 20 is 30
Explanation:
- This program declares three integer variables: num1, num2, and sum.
- It then prompts the user to enter two numbers and reads them using the scanf() function.
- The printf() function is then used to output the result after computing their total.
- Finally, it returns 0 to signify that the programme has been successfully finished.
This is only a simple illustration, and C is capable of being used for a wide variety of purposes, such as operating systems, assemblers, text editors, print spoolers, etc.
What is C++ Programming Language?
C++ is a general-purpose high-level language developed about 37 years ago at Bell Laboratories as an extension to C. The intent was to add the concept of OOPs (object-oriented programming) and provide support for the same. Today, the language has expanded far beyond the initial intent with numerous functional, object-oriented, and general features. This has given rise to more differences between C and C++ and it has become one of the most in-demand programming languages.
Features of C++
- It is an OOP language that also facilitates structured programming.
- It is platform-dependent; however, C++ programs can be run on multiple machines with minor alterations.
- It has an auto-keyword feature where users can define any variable without declaring its datatype.
- C++ is a multi-threading language, i.e., it allows a system to multitask/ execute more than one program at a given point in time.
- It is a simple, machine-independent, and compiler-based language.
- It is an efficient programming language that is case-sensitive and has a dynamic memory allocation feature.
Applications of C++
Operating systems | Game/ Gaming application development/ animation |
Web browsers like Google, Mozilla, etc. | Cloud/ distributed systems |
Database management software development | Banking application development |
Code Example:
Output:
Enter a number: 5
Factorial of 5 is 120
Explanation:
- It includes the <iostream> header to use input and output functions.
- It uses the using namespace std; directive to simplify the use of the cout and cin objects.
- It declares two integer variables, num and fact, where num stores the user's input, and fact stores the factorial result, initialized to 1.
- It prompts the user to enter a number with the cout statement and reads the input into the num variable using cin.
- It calculates the factorial of the entered number using a for loop that iterates from 1 to num, multiplying the fact variable by each value of I.
- Finally, it displays the calculated factorial using cout.
Similarities Between C and C++
In contrast to the difference between C and C++, some points of similarity between C and C++.
- Basic syntax: Fundamentally, the syntaxes of C and C++ are identical, and most of the operators and keywords in C++ are also present and carry out the same functions in C.
- Compilation and code structure: C and C++ have extremely similar general syntax, code structure, and compilation.
- Basic grammar: Similarities between the basic grammar of C and C++ include the usage of semicolons to terminate sentences, curly brackets to construct code blocks, and keywords like if, else, for, while, and switch.
- Basic memory model: The basic memory model of C and C++ is very close to the hardware, and they share similar notions of heap, stack, static, and file-scope variables.
- Keywords and operators: Nearly all C++ operators and keywords are also present in C.
Conclusion
Even though these languages share similarities at a basic level, there are many points of difference between C and C++. These come in the form of unique characteristics and features, some of which they have adapted over the years. Nonetheless, both C and C++ are popular commercial programming languages with a wide range of uses varying from simple application software creation to complex application development.
Frequently Asked Questions
Q. How does C++ extend the features of C programming language?
Nearly everything in C++ is also in C, but it has more features. Many developers consider C++ as a superset of C, adding functions like object-oriented programming, machine independence, dynamic memory allocation, memory management, and multithreading. Function overloading is one of the extra features offered by C++.
Q. How does memory management differ between C and C++?
Memory management in C and C++ is different in the following ways:
- Memory allocation in C++ is done using new and delete, but in C we use malloc() and free().
- While C++ has exception handling, C does not directly offer error handling in memory allocation.
- Compared to C, C++'s Standard Template Library (STL) contains a wider variety of container classes, such as vectors, lists, sets, maps, and more.
Q. What is the role of classes and objects in C++?
The main elements that facilitate object-oriented programming in C++ are classes and objects. A user-defined data type called a class combines methods for manipulating data with data representation in a single, tidy package. It serves as a tool for defining an object's shape and outlines the attributes and actions that a class's objects are capable of. For creating objects, a class functions as a blueprint or template.
In contrast, an object is a specific instance of a class. It is an actual entity with state and behaviour, where state is defined as data and behaviour is defined as functionality.
Q. What are the advantages of using C++ over C for software development?
There are several advantages of using C++ over C for software development, including:
- Exception Handling: Handling exceptions is available in C++ but does not exist in C. This makes it simpler to deal with problems and produce more durable and dependable code.
- Function Overloading: Function overloading is supported in C++. This makes it simpler to design more adaptable and reusable code by enabling developers to declare several functions with the same name but different arguments.
- Standard Template Library (STL): The Standard Template Library (STL) is one of several helpful libraries available in the C++ programming language. It offers a large selection of container classes, algorithms, and other features.
Q. In C, can you declare variables anywhere in a function?
Variables in C must be declared before they are utilised, often at the start of the function before any statements can be executed. This means that you cannot declare variables anywhere in a function. All variables must be declared at the beginning of the function before any executable statements.
Q. How do you deal with dynamic memory allocation in C?
In C, dynamic memory allocation is managed using functions like malloc, calloc, realloc, and free from the <stdlib.h> header. You allocate memory using malloc() or calloc(), which return pointers to the allocated memory. When done, use free to release the allocated memory to prevent memory leaks. Make sure to check if allocation was successful (the returned pointer is not NULL) and handle errors gracefully to ensure robust memory management.
This compiles our discussion on the difference between C and C++. Here are a few other topics you must explore:
- Hello World Program In C | How To Write, Compile & Run (+Examples)
- Operators In C Programming: Explained With Examples
- Comment In C++ | Types, Usage, C-Style Comments & More (+Examples)
- Operators In C++ | Types & Precedence Explained (With Examples)
- Comma Operator In C | Code Examples For Both Separator & Operator
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment