Table of content:
- Errors In C++
- What Is Exception Handling In C++?
- Exception Handling In C++ Program Example
- C++ Exception Handling: Basic Keywords
- The Need For C++ Exception Handling
- C++ Standard Exceptions
- C++ Exception Classes
- User-Defined Exceptions In C++
- Advantages & Disadvantages Of C++ Exception Handling
- Conclusion
- Frequently Asked Questions
C++ Exception Handling | Try, Catch And Throw (+Code Examples)
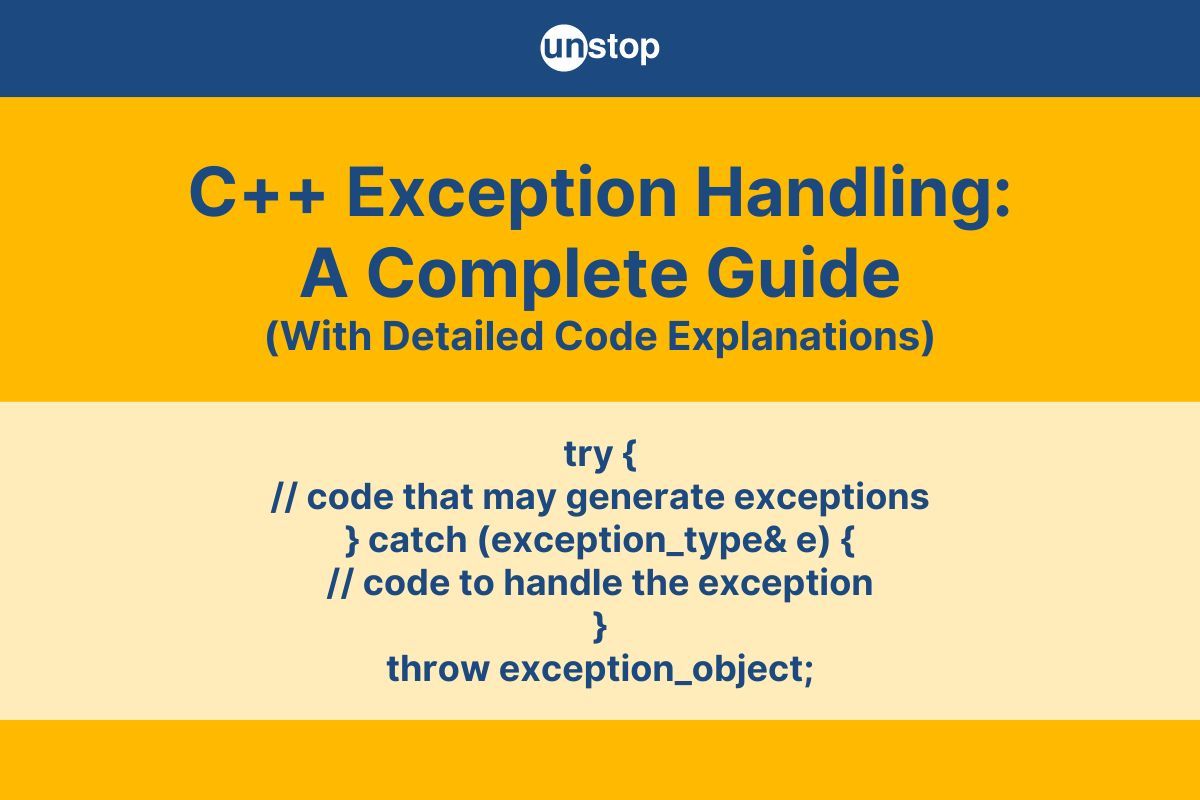
In C++, exception handling is a technique for handling any unexpected errors in programming or code execution. It involves handling exceptions gracefully by utilizing try-catch blocks. While catch blocks deal with particular kinds of exceptions, the try block contains the code where exceptions may occur. Its efficient use improves the maintainability and reliability of code.
In this article, we will explore the key concepts and features of exception handling in C++ programming, including its syntax, best practices, and the advantages and disadvantages it brings to error management in your code.
Errors In C++
In C++ language, errors refer to unforeseen and undesirable events that take place while a program is being executed. These mistakes may occur for a number of reasons, including incorrect input, runtime issues, memory allocation issues, or logical errors in the code. Errors can stop a program from running normally, produce inaccurate results, or even cause the program to crash.
Effective error handling and exception management are hence essential for addressing these errors and ensuring the program's stability and dependability. The errors can be broadly categorized into three main types: syntax errors, runtime errors, and logic errors. Let's take a closer look at each of these basic types.
Syntax Errors In C++
Every language has a set of syntax rules that stipulate the right way to write code in that language. Syntax errors occur when a code violates the rules of the programming language's syntax and are also known as compile-time errors.
- These errors prevent the code from being successfully compiled. Examples of syntax errors include missing semicolons, mismatched parentheses, incorrect variable names, etc.
- The compiler usually provides specific error messages pointing to the location of the syntax error in the code.
Example:
int main() {
cout << "Hello, World!" // Missing semicolon at the end
return 0;
}
Runtime Errors In C++
Runtime errors, as the name suggests, are coding errors that occur while a program is running.
- This means that the compiler does not catch these errors; instead, they are detected during the execution of the program.
- They often arise due to unexpected input, incorrect file operations, division by zero, accessing an out-of-bounds array index, or other situations where the program's behavior is not well-defined.
- The program might crash, terminate abnormally, or produce incorrect results when a runtime error occurs.
Example:
int main() {
int x = 5;
int y = 0;
int result = x / y; // Division by zero
return 0;
}
Logic Errors In C++
Logic errors occur when the code compiles and runs without errors but does not produce the expected or desired output.
- These errors can be tricky to identify because the program behaves as intended, but the outcome is incorrect due to flaws in the logical flow or calculations in the code.
- Logic errors often require careful debugging and code inspection to identify and fix.
Example:
int main() {
int radius = 5;
double area = 3.14 * radius * radius; // Incorrect formula for calculating area of a circle
cout << "The area is: " << area << endl;
return 0;
}
While these are the three major types of errors that occur, our prime focus in exception handling is runtime errors. So, let's explore the concept of C++ exception handling in detail.
What Is Exception Handling In C++?
As we've mentioned before, a code can always throw errors unexpectedly. When this happens, a programmer uses the C++ exception-handling framework to handle and react to unforeseen or extraordinary events that may emerge during the execution of a program.
This framework offers a methodical manner to handle mistakes and uncommon circumstances, assisting in maintaining program stability and averting program crashes. The basic flow of exception handling in C++ is as follows:
- The try block: This block contains code that may potentially throw an exception.
- Exception occurrence: If an exception occurs within the try block, the control is transferred to the appropriate catch block.
- The catch block: This block matches the type of the thrown exception executes. If no suitable catch block is found, the program may terminate or use default exception handling.
Below is the syntax for C++ exception handling, followed by an explanation of its components.
Syntax:
try {
// Code that may throw an exception
} catch (ExceptionType1& e1) {
// Exception handling code for ExceptionType1
} catch (ExceptionType2& e2) {
// Exception handling code for ExceptionType2
} catch (...) {
// Exception handling code for any other exception
}
Here,
- try{} block: The try keyword marks the block containing the code (inside {}) that may raise exceptions. If an exception occurs, it is caught by the corresponding catch block.
- catch block: This keyword marks the block containing the code (inside {}) to handle specific types of exceptions. Multiple catch blocks can follow a try block, each designed to handle different exception types.
- ExceptionType1 and ExceptionType2: Placeholders for the types of exceptions you want to catch. They should match the actual exception types.
- Exception variables e1, e2, etc.: Used inside the catch block to access details about the caught exception.
- catch(...): This is a default catch-all block for exceptions not matched by previous catch blocks. It provides a fallback for unexpected or unhandled exceptions.
Note: When an exception is thrown in a try block, the flow of control moves to the first catch block that matches the exception type. If multiple catch blocks are present, they are evaluated in the order they appear. The first matching catch block handles the exception, and the remaining catch blocks are skipped.
By understanding these components—try, catch, and throw—you can effectively manage exceptions and enhance the reliability of your C++ programs.
Check out this amazing course to become the best version of the C++ programmer you can be.
Exception Handling In C++ Program Example
Here is a C++ program example that showcases the concept of exception handling.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKaW50IG1haW4oKSB7Cgp0cnkgewovLyBDb2RlIHRoYXQgbWF5IHRocm93IGFuIGV4Y2VwdGlvbgppbnQgbnVtZXJhdG9yID0gMTA7CmludCBkZW5vbWluYXRvciA9IDA7CgppZiAoZGVub21pbmF0b3IgPT0gMCkgewp0aHJvdyAiRGl2aXNpb24gYnkgemVybyEiOwp9CgppbnQgcmVzdWx0ID0gbnVtZXJhdG9yIC8gZGVub21pbmF0b3I7CnN0ZDo6Y291dCA8PCAiUmVzdWx0OiAiIDw8IHJlc3VsdCA8PCBzdGQ6OmVuZGw7Cn0KY2F0Y2ggKGNvbnN0IGNoYXIqIGVycm9yTWVzc2FnZSkgewovLyBIYW5kbGluZyB0aGUgZXhjZXB0aW9uCnN0ZDo6Y291dCA8PCAiRXhjZXB0aW9uIGNhdWdodDogIiA8PCBlcnJvck1lc3NhZ2UgPDwgc3RkOjplbmRsOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Exception caught: Division by zero!
Explanation:
In the example code block above, the program uses a try-catch-block scenario for exception handling in C++.
-
We start by including the necessary header file <iostream> for input and output operations.
-
Inside the main() function, we use a try block to enclose the code that might throw an exception.
- Inside the try block, we declare two integer variables, numerator and denominator, and initialize them with the values of 10 and 0, respectively.
- We then use an if-statement to check if the denominator is zero (using the equality relational operator). Note that here, we are attempting division by 0, which is mathematically wrong.
-
If the denominator is zero, we throw an exception with the message "Division by zero!".
-
If the denominator is not zero, we proceed to calculate the result of dividing numerator by denominator and then print the result to the console using std::cout.
- If an exception is thrown, we transfer the control to the catch block, which handles exceptions of type const char*.
- Here, it does so by catching an exception of type const char*, which is a reference to a character array that represents a C-style string. The throw statement's message is stored in the errorMessage variable in the catch block.
- The output procured is an error message informing the user that a division by zero took place inside the catch block.
- The catch block provides a mechanism to manage and catch the exception. As a result, the program outputs the exception message to the console.
- Finally, the main() function returns 0, signalling the successful execution of the program.
Time Complexity: The code has constant or O(1) time complexity.
This code shows how to gracefully manage mistakes via the C++ exception handling framework. Instead of abruptly ending as soon as an exception is thrown, the program enters the catch block to deal with the exception and, if feasible, continues running.
C++ Exception Handling: Basic Keywords
Keywords refer to those words in a programming language that are reserved for specific purposes and must not be used for anything other than what they are reserved for. There are a few keywords that are reserved for C++ exception handling, i.e., they are only meant for handling exceptions, which are unanticipated or exceptional events that happen while a program is being executed.
Programmers can manage and react to such situations with the help of these keywords, which are essential components of the exception-handling mechanism. As mentioned before, there are three keywords when it comes to C++ exception handling, which are described below.
Try Keyword In C++ Exception Handling
In C++, the try keyword is a specialized keyword used to handle exceptions. It encloses a section of code that could result in an exception (i.e., it may contain an error). The try block's function is to locate and manage exceptions that may arise within its boundaries.
Syntax:
try {
// code that may generate exceptions
}
Here, the try block contains code that may throw exceptions, allowing errors to be caught and handled by corresponding catch blocks.
Catch Keyword In C++ Exception Handling
In C++, the catch keyword is used to deal with exceptions that are thrown inside of a try block (discussed above). It offers a block of code to handle the exception and lets us specify the kind of exception we want to catch.
Syntax:
catch (exception_type& e) {
// code to handle the exception
}
Here, the catch block captures exceptions of type exception_type and allows specific code to handle the caught exception using the variable e.
Throw Keyword In C++ Exception Handling
In C++, an exception can be thrown explicitly using the throw keyword. It is used within a block of code to indicate the occurrence of an exceptional condition that cannot be handled in the context at hand. It also indicates that the appropriate catch block should be called if the exception is encountered.
Syntax:
throw exception_object;
Here, the throw statement raises an exception by passing the exception_object, signaling an error that can be caught by a corresponding catch block.
Let's look at a code example to understand the implementation and working of these keywords in the C++ exception handling mechanism.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8c3RkZXhjZXB0PgoKaW50IGRpdmlkZShpbnQgbnVtZXJhdG9yLCBpbnQgZGVub21pbmF0b3IpIHsKCmlmIChkZW5vbWluYXRvciA9PSAwKSB7CnRocm93IHN0ZDo6cnVudGltZV9lcnJvcigiRGl2aXNpb24gYnkgemVybyBlcnJvciIpOwp9CgpyZXR1cm4gbnVtZXJhdG9yIC8gZGVub21pbmF0b3I7Cn0KCmludCBtYWluKCkgewoKdHJ5IHsKaW50IG51bWVyYXRvciA9IDEwOwppbnQgZGVub21pbmF0b3IgPSAwOwoKaW50IHJlc3VsdCA9IGRpdmlkZShudW1lcmF0b3IsIGRlbm9taW5hdG9yKTsKc3RkOjpjb3V0IDw8ICJSZXN1bHQ6ICIgPDwgcmVzdWx0IDw8IHN0ZDo6ZW5kbDsKfQpjYXRjaCAoY29uc3Qgc3RkOjpleGNlcHRpb24mIGUpIHsKc3RkOjpjb3V0IDw8ICJFeGNlcHRpb24gY2F1Z2h0OiAiIDw8IGUud2hhdCgpIDw8IHN0ZDo6ZW5kbDsKfQoKcmV0dXJuIDA7Cn0=
Output:
Exception caught: Division by zero error
Explanation:
In the above code example, we include the <iostream> header for input/output operations and the <stdexcept> header for standard exception classes.
- We then define a function divide() that takes two integer arguments, numerator and denominator. Inside the function:
- We first use an if-statement to check if the denominator is zero.
- If the condition is true, the control moves to the throw statement inside the if-block.
- The throw keyword explicitly throws a runtime error with the message "Division by zero error".
- If the condition is false (the denominator is not zero), we skip the if-block and move on. The function, in this case, divides the numerator by denominator and returns the same.
- In the main() function, we initiate the try block with the code that could contain an exception.
- Inside try, we declare two variables: numerator set to 10 and denominator set to 0.
- We then call the divide() function with these variables to carry out the division, store the outcome in the variable result, and print it to the console using std::cout.
- Note that the divide() function contains a throw statement to help throw/ identify any errors/exceptions. The try block helps handle the potential exception thrown, if any.
- If an exception occurs, it is caught in the catch-block. We will catch exceptions of type std::exception and use the e.what() method to get the details of the exception message.
- Finally, we print the exception message to the console.
The Need For C++ Exception Handling
Programmers can manage and react to exceptional situations that may arise during the execution of a program by using an essential component of programming called exception handling. The advantages and justifications for using C++ exception handling are as follows:
Error Handling:
- Exception handling offers a structured method for dealing with errors and exceptional circumstances.
- It enables programmers to detect errors and take appropriate action in response, avoiding unexpected program termination.
Robustness:
- By giving a program a way to handle and recover from exceptional circumstances, exception handling improves a program's robustness.
- It gives programmers the ability to gracefully handle errors and keep running their programs.
Separation of Concerns:
- Exception handling encourages the division of the logic for the main program from the code for handling errors.
- Developers can keep the primary code focused on its primary functionality while encapsulating error handling within distinct catch blocks, which helps the code be more readable and maintainable.
Diagnostics and Debugging:
- Exception handling helps identify and fix bugs. Whenever an exception is thrown, it frequently includes details about the mistake, like error messages or stack traces.
- With the help of this data, the error's root cause can be found, and the problem can be fixed.
Graceful Termination:
- When critical errors happen, exception handling enables the program to end gracefully.
- Exceptions can be caught and handled at higher levels of the program, allowing for proper cleanup and resource deallocation, as opposed to abruptly crashing the program.
Error Reporting and Logging:
- Reporting and logging errors are made simpler by the C++ exception handling framework.
- Developers can generate error reports, log error information, and alert users or administrators about errors by catching exceptions.
- This assists in software quality enhancement and troubleshooting.
Exception Propagation:
- Handling exceptions makes it easier for them to move up the call stack.
- Centralized error handling is possible when an exception is thrown but not handled within a function because it can propagate to higher levels of the program.
Flexibility:
- Handling exceptions offers flexibility in how different exception types are handled.
- Developers can create custom exception classes to represent particular types of errors and handle them appropriately.
- This makes it possible to develop error-handling techniques that are specific to the type of exception.
Overall, exception handling makes the software more dependable, maintainable, and user-friendly by facilitating efficient error management and offering tools to deal with unforeseen circumstances.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and earn your bragging rights today!
C++ Standard Exceptions
The C++ Standard Exceptions are a collection of predefined exception classes that are available in C++ through the Standard Library. These exception classes are a collection of standard exceptions that can be thrown in various error situations. They are defined in the <stdexcept> exception header file.
Each exception class stands for a particular kind of mistake or exceptional circumstance. The main Standard Exceptions in the C++ exception handling framework are listed below.
The std::exception
This is the base class for all common exceptions. It includes a virtual member function called what() that returns a C-style string describing the exception, as well as a common interface for handling exceptions.
The std::runtime_error
This exception is used to describe runtime errors that are typically brought on by outside factors or improper runtime operations. It is usually thrown along with a string that contains the associated error message.
The std::logic_error
This exception is used to highlight programmatic logic errors. It represents errors that might have been found during design or compile time.
Some of the most common std::logic_error subclasses are as follows:
- std::invalid_argument: Thrown when an invalid argument is passed to a function.
- std::domain_error: This exception is thrown when a mathematical function is called with a value outside of its permitted range.
- std::length_error: Thrown when an object is larger than what is permitted.
- std::out_of_range: Thrown when a value or index is outside of its acceptable range.
- std::bad_alloc: This type of exception is thrown when memory allocation fails, typically because there is not enough memory.
- std::bad_cast: The code throws this exception when a dynamic cast fails to convert a pointer or reference to a particular type.
- std::bad_typeid: This exception is raised when the typeid operator is applied to a null pointer or when it is combined with an expression that has a polymorphic type.
- std::bad_exception: This exception serves as a basic class for managing unanticipated errors that do not fall under the purview of the standard exception hierarchy.
These common exceptions give C++ programmers a uniform way to express and handle common error situations. Programmers may build code that explicitly throws the correct exception when a mistake occurs by making use of these built-in standard exception classes, which improves error handling and code structure.
C++ Exception Classes
Also known as user-defined classes, the exception classes in the C++ programming language are used to represent specific faults or exceptions that may occur while a program is being run. These classes are created by deriving from the C++ Standard Exceptions' base class std::exception or one of its derived classes.
The ability to create their own unique exception classes helps programmers better structure the management and communication of application-specific issues. Given below is a list of definitions and applications of special exception types in C++.
How To Make A Custom Exception Class?
To make a custom exception class, one must first define a class that either derives from std::exception or one of its derived classes.
- It is possible to include extra members or actions that are unique to the exception you're portraying.
- In order to provide your custom exception a relevant error message, you will typically need to modify the what() member method.
Let's take a look at an example of a custom exception class for a better understanding of the same.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8ZXhjZXB0aW9uPgojaW5jbHVkZSA8c3RyaW5nPgoKLy8gRGVmaW5lIHlvdXIgY3VzdG9tIGV4Y2VwdGlvbiBjbGFzcyBieSBpbmhlcml0aW5nIGZyb20gc3RkOjpleGNlcHRpb24KY2xhc3MgTXlFeGNlcHRpb24gOiBwdWJsaWMgc3RkOjpleGNlcHRpb24gewpwdWJsaWM6Ci8vIENvbnN0cnVjdG9yIHdpdGggYW4gZXJyb3IgbWVzc2FnZQpNeUV4Y2VwdGlvbihjb25zdCBjaGFyKiBtZXNzYWdlKSA6IGVycm9yTWVzc2FnZShtZXNzYWdlKSB7fQoKLy8gT3ZlcnJpZGUgdGhlIHdoYXQoKSBtZXRob2QgdG8gcHJvdmlkZSBlcnJvciBtZXNzYWdlCmNvbnN0IGNoYXIqIHdoYXQoKSBjb25zdCBub2V4Y2VwdCBvdmVycmlkZSB7CnJldHVybiBlcnJvck1lc3NhZ2UuY19zdHIoKTsKfQoKcHJpdmF0ZToKc3RkOjpzdHJpbmcgZXJyb3JNZXNzYWdlOwp9OwoKaW50IG1haW4oKSB7Cgp0cnkgewovLyBUaHJvdyB5b3VyIGN1c3RvbSBleGNlcHRpb24KdGhyb3cgTXlFeGNlcHRpb24oIlRoaXMgaXMgYSBjdXN0b20gZXhjZXB0aW9uISIpOwp9CmNhdGNoIChjb25zdCBNeUV4Y2VwdGlvbiYgZXgpIHsKc3RkOjpjb3V0IDw8ICJDYXVnaHQgZXhjZXB0aW9uOiAiIDw8IGV4LndoYXQoKSA8PCBzdGQ6OmVuZGw7Cn0KY2F0Y2ggKGNvbnN0IHN0ZDo6ZXhjZXB0aW9uJiBleCkgewpzdGQ6OmNvdXQgPDwgIkNhdWdodCBhIHN0YW5kYXJkIGV4Y2VwdGlvbjogIiA8PCBleC53aGF0KCkgPDwgc3RkOjplbmRsOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Caught exception: This is a custom exception!
Explanation:
- In this example, we first include iostream and execution files to indicate the import of library functions.
- We then define a custom exception class called MyException, which inherits from the std::exception from the standard library.
- The class contains a constructor to set the error message when creating an instance of the exception.
- Next, inside the class, we override the what() method to return the error message using the override keyword.
- In the main() function,
- We set up the try block to demonstrate throwing and catching the custom exception.
- Then, we include two catch blocks, which contain the code block for catching standard exceptions (std::exception) to provide a fallback for handling other types of exceptions.
- Which of these catch statements are executed depends on the type of exception thrown.
The example shows how to construct exceptions with particular error messages using this unique MyException class, which can then be captured and handled appropriately in C++ code. Note that it is simpler to integrate with current exception-handling procedures since it derives from std::exception, which adheres to the standard exception-handling techniques in C++.
User-Defined Exceptions In C++
In C++ exception handling, you can define your own exception to address certain error situations in the code. These exceptions grant users the ability to construct unique custom exception types that derive from the std::exception base class or any of its derived classes.
For this, one must first construct a new class that derives from std::exception or one of its derived classes and then override the what() method to produce a unique error message. Given below is the basic syntax for these exceptions along with a code example, output, explanation of the code, and information on the time and space complexity.
Syntax:
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "Custom exception message";}
};
Here,
- MyException refers to the name of the new class being defined. This class derives from std::exception.
- The what() method is a virtual function in std::exception, which is being overridden here using the override keyword.
- The const char* signifies the data type of the return value for the overriding function.
Here is a code that illustrates how to utilize a user-defined exception in C++.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgTXlFeGNlcHRpb24gOiBwdWJsaWMgc3RkOjpleGNlcHRpb24gewpwdWJsaWM6CmNvbnN0IGNoYXIqIHdoYXQoKSBjb25zdCBub2V4Y2VwdCBvdmVycmlkZSB7CnJldHVybiAiQ3VzdG9tIGV4Y2VwdGlvbiBtZXNzYWdlIjsKfQp9OwoKaW50IGRpdmlkZShpbnQgYSwgaW50IGIpIHsKCmlmIChiID09IDApIHsKdGhyb3cgTXlFeGNlcHRpb24oKTsKfQoKcmV0dXJuIGEgLyBiOwp9CgppbnQgbWFpbigpIHsKCnRyeSB7CgppbnQgcmVzdWx0ID0gZGl2aWRlKDEwLCAwKTsKc3RkOjpjb3V0IDw8ICJSZXN1bHQ6ICIgPDwgcmVzdWx0IDw8IHN0ZDo6ZW5kbDsKfSBjYXRjaCAoY29uc3QgTXlFeGNlcHRpb24mIGV4KSB7CnN0ZDo6Y291dCA8PCAiRXhjZXB0aW9uIGNhdWdodDogIiA8PCBleC53aGF0KCkgPDwgc3RkOjplbmRsOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Exception caught: Custom exception message
Explanation:
In the example, we first define a class called MyException, which inherits from the exception library. The class overrides the what() method to return a custom exception message.
- We then define a divide() function that takes two integer parameters, a and b and returns an integer. Inside:
- We check if the value of b is zero using an if-statement.
- If the condition is true, we execute the if-block. It contains a throw statement which throws an instance of MyException to signal an error.
- If the condition is false (i.e., b is not zero), we skip the if-block, and the function returns the result of division of a by b.
- In the main() function, we initiate the try block and call the divide() function with values 10 and 0.
- Since here we are dividing something by 0, it triggers an exception, and the control is passed to the catch block.
- Inside catch, once the MyException object is caught, the what() function helps retrieve the custom error message supplied by the MyException class, using the ex variable.
- We use the std::cout statement to display this message to the console.
Advantages & Disadvantages Of C++ Exception Handling
C++ exception handling is a programming technique used to manage and respond to exceptional or unexpected situations that can occur during program execution. Here are some advantages and disadvantages of using exception handling in your code:
Advantages Of Exception Handling:
- Enhanced Readability and Maintainability: C++ exception handling allows you to separate error handling code from the normal program logic. This leads to cleaner, more readable code that is easier to maintain, as error-handling details are isolated.
- Structured Error Handling: With C++ exception handling, you can define a clear structure for handling different types of errors. This helps in maintaining a consistent approach to dealing with exceptions throughout the codebase.
- Centralized Error Handling: C++ exception handling provides a way to centralize error-handling logic in one place, such as a catch block. This makes it easier to manage and update error-handling code when needed.
- Graceful Termination: Instead of abrupt crashes or undefined behavior, exception handling allows for the graceful termination of a program by handling errors in a controlled manner. This can be especially important for long-running applications or critical systems.
- Separation of Concerns: C++ exception handling helps separate the concerns of regular program flow and error handling. This separation can lead to better modularity and easier testing of both components.
-
Propagation of Errors: Exceptions can be propagated up the call stack, allowing you to handle errors at an appropriate level in the program hierarchy. This can help in providing context-specific error handling.
Disadvantages Of Exception Handling:
- Performance Overhead: Exception handling can introduce some performance overhead compared to error-checking techniques, as the system needs to maintain the call stack and perform additional operations during exception propagation and handling.
- Complexity: Exception handling, when not used judiciously, can lead to complex code structures that are harder to understand. Overuse or misuse of exceptions can make the flow of program execution more difficult to debug and maintain.
- Resource Management Challenges: Properly managing resources (memory, file handles, etc.) when exceptions occur can be challenging. It can also lead to resource leaks if resources are not properly released in exception scenarios.
- Unintended Consequences: Improper handling of exceptions or swallowing exceptions without appropriate action can lead to unexpected behavior or data corruption in the program.
- Compiler and Runtime Dependency: Exception handling may depend on compiler and runtime support. Different compilers or runtime environments may have varying levels of support for exception-handling mechanisms.
- Overhead in Embedded Systems: In resource-constrained environments like embedded systems, the overhead introduced by exception handling can be a concern, both in terms of memory usage and performance.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
C++ exception handling is a powerful mechanism that helps manage unexpected situations and errors in a structured and efficient manner. By utilizing try, catch, and throw, developers can gracefully handle errors, prevent program crashes, and ensure that critical resources are properly managed. Exception handling enhances the robustness and maintainability of code by separating error-handling logic from core functionality. Whether dealing with standard exceptions or creating custom ones, C++ provides the flexibility and control necessary for writing reliable and resilient programs, ultimately contributing to better software quality and user experience.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. How do you raise exceptions in CPP?
The throw keyword in C++ allows you to raise or throw exceptions. The throw may be used to create an exception object and pass control to the proper catch block when an unusual circumstance arises in your program. The following steps show how to raise exceptions in C++:
- Create an exception object: First, create an exception object of a certain kind, such as one from a user-defined or built-in exception class (such as std::exception or its related classes).
- Then, use the throw keyword and the exception object to throw the exception.
- This hands-off control to the closest catch block that is capable of handling the exception.
Here is a C++ example showing how to raise exceptions:
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8c3RkZXhjZXB0PiAvLyBJbmNsdWRlIHRoaXMgdG8gdXNlIHN0ZDo6aW52YWxpZF9hcmd1bWVudAoKaW50IG1haW4oKSB7CnRyeSB7CmludCBhZ2U7CgpzdGQ6OmNvdXQgPDwgIkVudGVyIHlvdXIgYWdlOiAiOwpzdGQ6OmNpbiA+PiBhZ2U7CgppZiAoYWdlIDwgMCkgewp0aHJvdyBzdGQ6OmludmFsaWRfYXJndW1lbnQoIkludmFsaWQgYWdlOiBBZ2UgY2Fubm90IGJlIG5lZ2F0aXZlLiIpOwp9CgpzdGQ6OmNvdXQgPDwgIllvdXIgYWdlIGlzOiAiIDw8IGFnZSA8PCBzdGQ6OmVuZGw7Cn0gY2F0Y2ggKGNvbnN0IHN0ZDo6ZXhjZXB0aW9uJiBleCkgewpzdGQ6OmNvdXQgPDwgIkV4Y2VwdGlvbiBjYXVnaHQ6ICIgPDwgZXgud2hhdCgpIDw8IHN0ZDo6ZW5kbDsKfQoKcmV0dXJuIDA7Cn0=
Output:
Enter your age: -10
Exception caught: Invalid age: Age cannot be negative.
Explanation:
We begin by setting up a try block in the main() function.
- Then we declare an integer variable age in the block and use the cout command to prompt the user to enter their age.
- The input is read using the cin command, and the flow is passed on the if-statement, which checks if the ag is less than zero.
- If age<0 holds true, the throw keyword throws a std::invalid_argument exception with a custom error message.
- The catch block then resolves the exception, displaying the error message. And the output is printed to the console using std::cout.
This is how you can efficiently handle uncommon circumstances in your code and give users the proper error handling and feedback by raising exceptions.
Q. What is the difference between exception and exception handling?
The table given below highlights the differences between C++ exception and C++ exception handling.
Aspect | Exception | Exception Handling |
---|---|---|
Definition | An exception is an event that occurs during program execution, often due to an error or unexpected condition. | Exception handling is the mechanism or technique used to manage and respond to exceptions in a controlled and organized manner. |
Nature | It represents an abnormal situation or error that disrupts the normal flow of program execution. | It encompasses the methods, syntax, and constructs used to detect, handle, and recover from exceptions. |
Example | Division by zero, file not found, out-of-memory, etc. | Using try-catch blocks, throwing exceptions, using standard exception classes, etc. |
Role in Program Flow | An exception disrupts the normal sequential flow of the program, potentially causing it to terminate abruptly. | Exception handling aims to gracefully manage exceptional situations, allowing the program to recover or terminate in a controlled manner. |
Responsibility | It's the event or instance that triggers the need for special handling. | It's the process of designing code to handle exceptions and ensure program robustness. |
Mechanism | Exceptions are thrown (generated) when an exceptional situation arises. | Exception handling involves catching and handling exceptions using try-catch blocks and appropriate actions. |
Control Transfer | When an exception is thrown, the program's control transfers to an appropriate catch block. | Catch blocks are designed to handle specific types of exceptions and provide appropriate responses. |
Standard Library Role | C++ provides a set of standard exception classes to represent various types of exceptions. | C++ provides language features (try-catch blocks, throw statements) and standard exception classes to facilitate exception handling. |
Example | throw std::runtime_error("File not found"); | try { // Code that might throw exceptions } catch (const std::exception& ex) { // Handle the exception } |
Q. What are the 3 blocks in exception handling?
The three blocks used in C++ exception handling to deal with exceptions are as follows:
- Try Block: The try block is the initial block in the exception handling process. It encloses the program code that might cause an exception. The associated catch block or blocks will capture and handle any exceptions thrown within the try block. The try block offers a secure area where handling and monitoring of exceptions is possible.
- Catch Block: The try block is followed by one or more catch blocks. They include the code that is run when a matched exception is caught, and they identify the types of exceptions they may handle. We can utilize several catch blocks to handle various kinds of errors. The first catch block that matches each exception type is executed, and each catch block has an associated exception type.
- Throw Block: The throw statement is used to manually throw an exception. It's not always necessary to use throw, as exceptions can be thrown by the C++ standard library or other parts of your program. When you throw an exception, the program will search for a matching catch block to handle it.
Together, the three blocks offer a well-organized method for managing exceptions in C++.
Q. Can we use exceptions in function?
Yes, functions in C++ can utilize exceptions. You can manage extraordinary circumstances and faults that may arise during the execution of a function by using exception handling. For this, a function can use the throw keyword to raise an exception when it runs into a unique circumstance. After that, the function has a choice as to whether to handle the exception internally or pass it on to the caller function for treatment.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8c3RkZXhjZXB0PgoKaW50IGRpdmlkZShpbnQgYSwgaW50IGIpIHsKCmlmIChiID09IDApIHsKdGhyb3cgc3RkOjpydW50aW1lX2Vycm9yKCJEaXZpc2lvbiBieSB6ZXJvIik7Cn0KCnJldHVybiBhIC8gYjsKfQoKaW50IG1haW4oKSB7Cgp0cnkgewppbnQgcmVzdWx0ID0gZGl2aWRlKDEwLCAwKTsKc3RkOjpjb3V0IDw8ICJSZXN1bHQ6ICIgPDwgcmVzdWx0IDw8IHN0ZDo6ZW5kbDsKfQpjYXRjaCAoY29uc3Qgc3RkOjpleGNlcHRpb24mIGV4KSB7CnN0ZDo6Y291dCA8PCAiRXhjZXB0aW9uIGNhdWdodDogIiA8PCBleC53aGF0KCkgPDwgc3RkOjplbmRsOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Exception caught: Division by zero
Explanation:
- We begin by defining a divide() function that takes two interger parameters and performs a division operation on them.
- The function throws an exception of type std::runtime_error with the message 'Division by zero' if the divisor, i.e., b, is zero.
- The catch section of the main() function then handles the exception and displays the error.
- You may distinguish between ordinary conditions and extraordinary ones by using exceptions in functions.
- Exceptions can either be handled inside the function itself or can be sent to higher levels for processing.
Q. What is a friend function in C++?
A friend function in C++ is a unique kind of function that, although not a member of the class in question, is given access to its private and protected members. In other words, the function can access a class's private and protected members as if it were a friend of the class. However, the functions outside the class are normally only allowed to access the public members.
We use the friend keyword "declare friend functions inside the class body, followed by the function prototype. This declaration grants the provided function access to the class's private and protected members as if it were a class member. Here is an illustration:
class MyClass {
private:
int privateData;
public:
MyClass() {
privateData = 0;}
friend void friendFunction(MyClass& obj); // Declaration of friend function};
void friendFunction(MyClass& obj) {
obj.privateData = 42; // Accessing and modifying privateData}
In this illustration, the class MyClass consists of a private data member called privateData, and a friend function called friendFunction. This function can access and modify any of the objects of the privateData member of MyClass.
This is how friend functions may come in handy when external functions need access to a class's secret data members without belonging to that parent class. However, it is typically advised to utilize friend functions sparingly since they can undermine the information-hiding principle and weaken encapsulation.
Q. Can we throw multiple exceptions in C++?
In C++, you cannot throw multiple exceptions at once directly. When an exception is thrown, it travels up the call stack until it is caught by an appropriate exception handler. If another exception is thrown before the first one is caught, the original exception will be lost as the new exception overrides it.
However, you can handle multiple types of exceptions using separate catch blocks. A single try block can be followed by multiple catch blocks, each designed to handle a different type of exception. This allows you to handle various exceptions differently based on their type.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8ZXhjZXB0aW9uPgoKaW50IG1haW4oKSB7CnRyeSB7Ci8vIENvZGUgdGhhdCBtYXkgdGhyb3cgZXhjZXB0aW9ucwppbnQgbnVtZXJhdG9yID0gMTA7CmludCBkZW5vbWluYXRvciA9IDA7CgppZiAoZGVub21pbmF0b3IgPT0gMCkgewp0aHJvdyBzdGQ6OnJ1bnRpbWVfZXJyb3IoIkRpdmlzaW9uIGJ5IHplcm8gaXMgbm90IGFsbG93ZWQuIik7Cn0KCmludCByZXN1bHQgPSBudW1lcmF0b3IgLyBkZW5vbWluYXRvcjsKc3RkOjpjb3V0IDw8ICJSZXN1bHQ6ICIgPDwgcmVzdWx0IDw8IHN0ZDo6ZW5kbDsKfQpjYXRjaCAoY29uc3Qgc3RkOjpydW50aW1lX2Vycm9yJiBleCkgewpzdGQ6OmNvdXQgPDwgIkNhdWdodCBhIHJ1bnRpbWUgZXJyb3I6ICIgPDwgZXgud2hhdCgpIDw8IHN0ZDo6ZW5kbDsKfQpjYXRjaCAoY29uc3Qgc3RkOjpleGNlcHRpb24mIGV4KSB7CnN0ZDo6Y291dCA8PCAiQ2F1Z2h0IGEgZ2VuZXJpYyBleGNlcHRpb246ICIgPDwgZXgud2hhdCgpIDw8IHN0ZDo6ZW5kbDsKfQpjYXRjaCAoLi4uKSB7CnN0ZDo6Y291dCA8PCAiQ2F1Z2h0IGFuIHVua25vd24gZXhjZXB0aW9uLiIgPDwgc3RkOjplbmRsOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Caught a runtime error: Division by zero is not allowed.
Explanation:
In the example, the try block contains code that might throw exceptions.
- If a std::runtime_error is thrown (in this case, due to division by zero), it is caught by the first catch block.
- If a different exception derived from std::exception is thrown, it is caught by the second catch block.
- The third catch block is a catch-all block that handles any exceptions not caught by the previous blocks.
It is essential to arrange catch blocks from the most specific exception types to the most generic. If a more generic catch block appears before a specific one, the specific exception may be caught by the generic block, preventing the specific handler from executing.
You might also be interested in reading the following:
- Inline Function In C++ | Declare, Working, Examples & More!
- 2D Vector In C++ | Declare, Initialize & Operations (+ Examples)
- The 'this' Pointer In C++ | Declaration, Constness, Applications & More!
- Function Overriding In C++ | Examples, Working Mechanism & More!
- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment