Hybrid Inheritance In C++ | Syntax, Applications & More (+Examples)
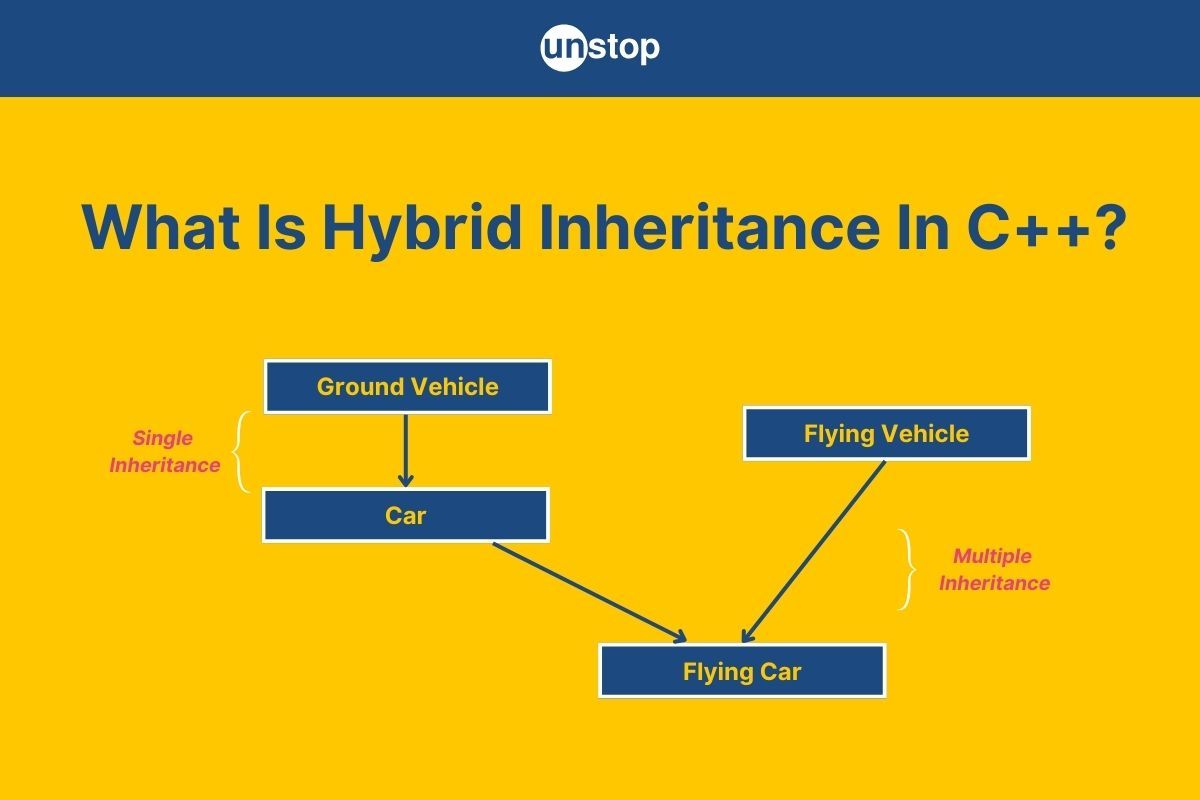
Hybrid inheritance in C++ is a type of inheritance where a class can inherit from more than one base class by combining different types of inheritance—single, multiple, hierarchical, or multilevel. This allows a class to inherit features from several base classes, providing a flexible and organized approach to modelling complex real-world relationships. By blending these inheritance types, hybrid inheritance helps developers create more dynamic and reusable code structures.
In this article, we will explore what hybrid inheritance is, how it works, why it is important in object-oriented programming, and how we can use it effectively in C++ with examples and best practices.
What Is Hybrid Inheritance In C++?
Hybrid inheritance in C++ programming is a combination of multiple inheritance types within a class hierarchy. It merges features from both single and multiple inheritance. This approach allows developers to create complex class structures.
- By using hybrid inheritance in C++, programmers can integrate different inheritance forms. For instance, a derived class can inherit from two or more base classes.
- This flexibility enhances the design of software applications. It enables developers to utilize various functionalities from different classes.
- Hybrid inheritance's main purpose is to enhance code reusability. It simplifies programming tasks by allowing one class to inherit methods and attributes from multiple sources.
Syntax Of Hybrid Inheritance In C++
class A {
// block of statement(s)
}; // Single class Aclass B : public A { // Single inheritance (B derives from A)
// block of statement(s)
};class C {
// block of statement(s)
}; // Independent class Cclass D : public B, public C { // Multiple inheritance (D derives from both B and C)
// block of statement(s)
};
Here:
- class B : public A represents single inheritance, where B inherits from A.
- On the other hand, class D : public B, public C represents multiple inheritance, where D inherits from both B (which indirectly inherits from A) and C.
Thus, class D follows hybrid inheritance as it inherits through a combination of single and multiple inheritance patterns.
Importance Of Hybrid Inheritance In Object Oriented Programming
Hybrid inheritance in object-oriented programming plays a crucial role in building flexible and reusable class structures.
- Enhanced Reusability: Hybrid inheritance in C++ allows reusing code from multiple base classes, reducing duplication and improving maintainability.
- Flexible Design: It supports complex relationships between classes by combining multiple inheritance types, leading to versatile and modular designs.
- Efficient Code Organization: Hybrid inheritance in C++ facilitates the organization of code into smaller, reusable components that can be combined in various ways to build more complex systems.
- Improved Abstraction: It enables higher-level abstraction by allowing a derived class to inherit characteristics from diverse base classes, hiding implementation details.
- Better Extensibility: It provides flexibility to extend functionality by adding new derived classes without altering existing base class code, supporting scalable and adaptable systems.
- Promotes Real-World Modeling: Hybrid inheritance in C++ helps model real-world systems more accurately by allowing objects to inherit behaviors from different sources, reflecting multiple facets of real-world entities.
Overall, hybrid inheritance in C++ enhances the efficiency of software development in OOP. It streamlines processes and fosters a clearer understanding of class hierarchies, allowing developers to create easier-to-maintain applications.
Example Of Hybrid Inheritance In C++: Using Single and Multiple Inheritance
In this example of hybrid inheritance, we will demonstrate how classes can inherit features from multiple base classes using a combination of single and multiple inheritance in C++.
Code Example:
Output:
This is a Vehicle.
This Vehicle has an Engine.
This is a Car.
This is a Sports Car.
Explanation:
In the above code example-
- We start by defining the base class Vehicle, which contains a function showVehicle() that outputs "This is a Vehicle." Next, we define another base class Engine with the function showEngine(), which prints "This Vehicle has an Engine."
- Now, we create the derived class Car, which inherits from Vehicle. It has an additional method showCar() that outputs "This is a Car." This is an example of single inheritance, where the Car class inherits from one base class.
- To introduce multiple inheritance, we create another derived class, SportsCar, which inherits from both Car and Engine. This means that SportsCar has access to methods from both the Vehicle and Engine classes. In addition, we add a method showSportsCar() to SportsCar, which prints "This is a Sports Car."
- In the main() function, we create an object obj of the class SportsCar. Using this object, we call the following methods: showVehicle() (inherited from Vehicle), showEngine() (inherited from Engine), showCar() (inherited from Car), showSportsCar() (from the SportsCar class itself).
The above example demonstrates how SportsCar inherits features from both its parent classes (Car and Engine), showcasing the power of hybrid inheritance in combining features from multiple sources.
Example Of Hybrid Inheritance In C++: Using Multilevel and Hierarchical Inheritance
In this example of hybrid inheritance in C++, we will demonstrate the implementation of both multilevel inheritance and hierarchical inheritance:
Code Example:
Output:
This is an Animal.
This is a Mammal.
This is a Dog.
This is an Animal.
This is a Mammal.
This is a Cat.
Explanation:
In the above code example-
- We start by defining the base class Animal, which includes a method showAnimal() that prints "This is an Animal."
- Next, we create the derived class Mammal, which inherits from Animal (multilevel inheritance). The Mammal class adds a method showMammal() that outputs "This is a Mammal."
- We then define the Dog class, which inherits from Mammal. It includes its own method, showDog(), that prints "This is a Dog." This showcases another level of multilevel inheritance.
- Similarly, we create the Cat class, which also inherits from Mammal. It contains a method showCat() that outputs "This is a Cat." This represents hierarchical inheritance since both Dog and Cat share the same parent class, Mammal.
- In the main() function, we create objects dogObj and catObj. Using dogObj, we call the following methods: showAnimal() (inherited from Animal), showMammal() (inherited from Mammal), showDog() (specific to Dog).
- Similarly for catObj, we call: showAnimal() (inherited from Animal), showMammal() (inherited from Mammal), showCat() (specific to Cat).
- Finally, the main() function returns 0, indicating successful program execution.
Real-World Applications Of Hybrid Inheritance In C++
Hybrid inheritance in C++ shines in complex software systems. It allows programmers to combine multiple classes effectively. For example, a restaurant management system can use hybrid inheritance to manage different aspects like meals, orders, and customers.
Here are some other real-world applications of hybrid inheritance in C++:
- Gaming Engines: We can use hybrid inheritance to create complex character models. For example, a base class Character can have subclasses like Player and NonPlayer, with specific classes like Hero inheriting from Character.
- Vehicle Management Systems: In a vehicle management system, we can have a base class called Vehicle. Classes like Car and Truck can inherit from it. We can then create specialized classes like ElectricCar from Car.
- E-commerce Applications: In an e-commerce platform, we can use a base class Product for different product types. Classes like Electronics and Clothing can inherit from it, and we can have specific classes like Smartphone and Shirt.
- Healthcare Systems: We can create a base class called Person for people in healthcare. Classes like Patient and Doctor can inherit from it. Then, we can have specialized classes like Surgeon from Doctor.
- Education Management Systems: In an education management system, we can use a base class Person for students and teachers. Classes like Student and Teacher can inherit from it, and we can create subclasses like Undergraduate and FullTime.
- Financial Applications: In financial applications, we can have a base class BankAccount. We can create subclasses like SavingsAccount and CheckingAccount, and then further specialize them with classes like StudentAccount.
Conclusion
Hybrid inheritance in C++ language provides a flexible and powerful way to design class hierarchies by combining multiple types of inheritance, such as single, multiple, multilevel, and hierarchical. While it enables more complex models that reflect real-world scenarios, it also comes with challenges like increased complexity, ambiguity, and potential maintenance difficulties. By carefully designing class structures and understanding the implications of using hybrid inheritance, we can build efficient, reusable, and scalable systems. However, it's important to strike a balance to ensure clarity and maintainability in the code.
Frequently Asked Questions
Q. What is hybrid inheritance in C++? Why is it important?
Hybrid inheritance in C++ combines various inheritance types, such as single, multiple, multilevel, and hierarchical inheritances, within a single class hierarchy. In this structure, a derived class can inherit from different types of base classes, allowing for more complex and flexible class relationships. For example, one class might inherit from a single base class, while another class might inherit from multiple base classes.
- Hybrid inheritance is important because it offers greater design flexibility, enabling us to model real-world scenarios more accurately by inheriting features from multiple sources.
- It also promotes code reusability by allowing different classes to share common functionalities, reducing redundancy and simplifying code maintenance.
- Moreover, hybrid inheritance in C++ supports the creation of more complex systems, such as game development or management software, where relationships between classes need to be more intricate.
Q. How does hybrid inheritance differ from other types?
Hybrid inheritance differs from other types of inheritances by combining multiple forms of inheritance, such as single, multilevel, hierarchical, and multiple inheritance structure to create more complex class hierarchies. While single inheritance involves a class inheriting from one base class, and multiple inheritance allows a class to inherit from multiple base classes, hybrid inheritance blends these approaches, enabling a class to inherit features from several inheritance structures. This flexibility makes hybrid inheritance powerful but can also introduce challenges like ambiguity and increased complexity, which are less common in simpler inheritance models.
Q. Can you provide a real-world example of hybrid inheritance?
Here’s a real-world example of hybrid inheritance in C++ using a Company Management System:
Scenario: In a company, we have employees who can either be part-time or full-time. There are also different types of managers. Using hybrid inheritance in C++, we can model this system.
Code Example:
Output:
This is an Employee.
This is a Full-Time Employee.
This is a Manager.
This is a Technical Lead Manager.
Explanation:
In a company, a technical lead (TechLead) is a specialized type of manager who is also a full-time employee. The class structure reflects this real-world scenario, allowing us to model both the general characteristics of employees and the specific roles within the company.
Q. What are the challenges of using hybrid inheritance?
Here are some challenges of using hybrid inheritance:
- Increased Complexity: Hybrid inheritance can create complex class hierarchies, making the code harder to understand and maintain. Tracking relationships between classes may become cumbersome.
- Ambiguity Issues: When multiple base classes define the same member, ambiguity arises when derived classes try to access that member. This can lead to confusion and errors in the code.
- Diamond Problem: In scenarios where a class inherits from two classes that share a common base class, the "diamond problem" occurs. This can create ambiguity in method resolution and lead to redundant instances of the base class.
- Higher Coupling: Classes in hybrid inheritance may become tightly coupled, meaning changes in one class can significantly affect others. This can complicate code updates and refactoring.
- Difficulty in Debugging: The complex relationships in hybrid inheritance can make debugging more challenging. Identifying the source of an issue may require navigating through multiple layers of inheritance.
- Performance Overhead: The added complexity of hybrid inheritance may lead to performance issues, as more classes and methods may need to be processed during runtime.
- Maintenance Challenges: Maintaining hybrid inheritance structures can be more difficult due to the potential for inherited members to change or become obsolete, necessitating careful updates across multiple classes.
Q. Is hybrid inheritance supported in all programming languages?
Hybrid inheritance in C++ is not universally supported in all programming languages. While languages like C++ and Python allow for hybrid inheritance by combining different inheritance types (such as single, multiple, and hierarchical inheritance), others may have limitations.
For instance, Java supports single and multilevel inheritance but does not allow multiple inheritance to avoid ambiguity, utilizing interfaces instead to achieve similar functionality. Similarly, some languages like C# also restrict multiple inheritance but allow for a form of hybrid inheritance through interfaces.
Here are a few other interesting C++ topics you must explore:
- C++ Type Conversion & Type Casting Demystified (With Examples)
- C++ If-Else | All Conditional Statements Explained With Examples
- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
- Friend Function In C++ | Class, Types, Uses & More (+Examples)
- Logical Operators In C++ | Use, Precedence & More (With Examples)
- C++ 2D Array & Multi-Dimensional Arrays Explained (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment