Difference Between Structure And Class In C++ Programming Decoded
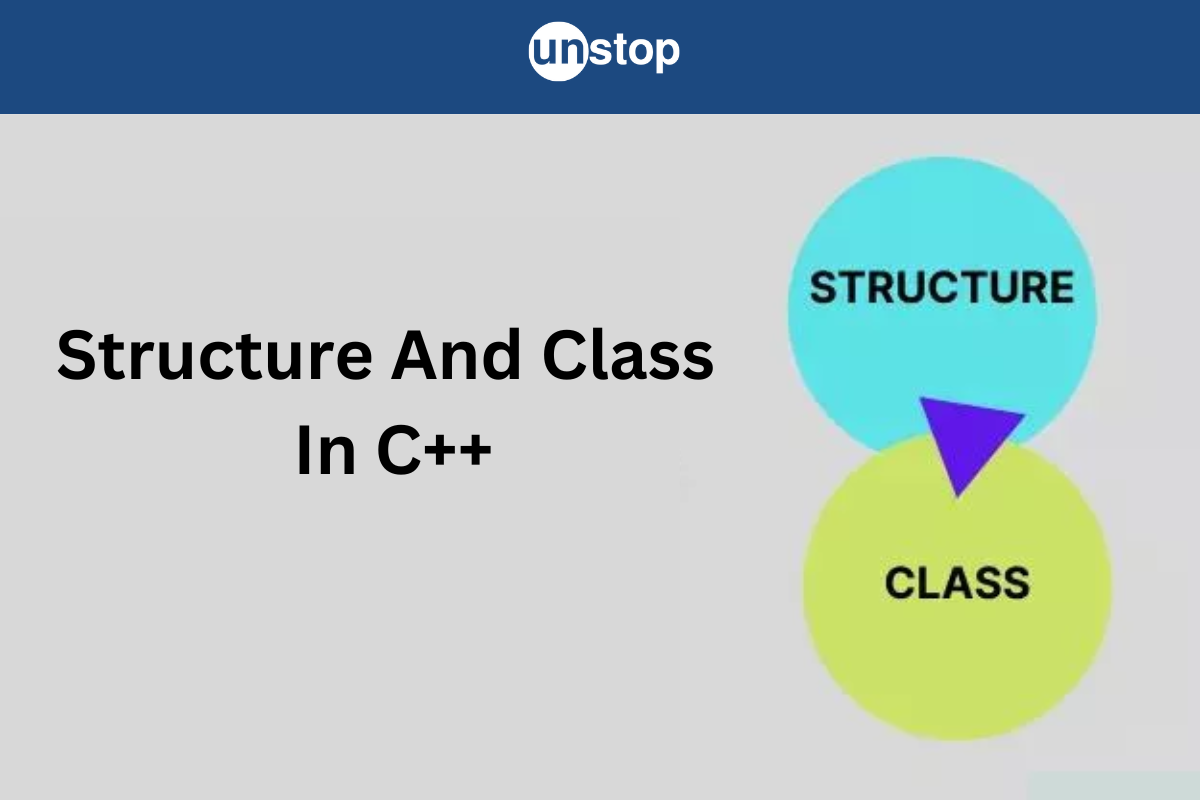
C++ is an object-oriented programming language and it places a greater emphasis on data and its management. It is a technique for modularizing programs by generating a partitioned memory space for both data and functions. A structure or a class can both be used to construct a customized data type that can then be used to create instances. In C++, structure and class are very similar. The difference between a structure and a class in C++ will be discussed in this article. But, before we get into the differences, let's review the structure and classes in C++.
Structure
A structure is a grouping of values that may or may not be of the same kind. It is a user-defined data type. A structure object is similar to a record in many aspects. It keeps track of an entity's related data. It combines numerous data types and creates a single data type from them.
The most notable difference between a structure and an array is that an array can only store data of the same data type. As a result, a structure is a collection of variables with a single name. A structure's variables are of various data types, and each has a name that is used to choose it from the structure.
Until a structure type variable is formed, the structure member variables do not occupy memory. All of the structure instances are stored at a contiguous memory location.
Structure Declaration
The term struct is followed by the structure name to define a structure. The structure's variables are all specified within the structure. The following syntax is typically used to declare a structure type. It is declared using the struct keyword,
struct struct_name {
structure element 1;
structure element 2;
. . .
structure element n;
};Example:
struct student {
int rno;
char nm[20];
float fees;
};
struct student s1;
A member of a structure is a variable declared within the structure. The structure declaration, on the other hand, does not occupy any memory or storage space. When you define a structure, you're creating a template for building structure objects with the same data members and member functions.
Let's look at another way of declaring the structure
struct student {
int rno;
char nm[20];
float fees;
} std1, std2;
If you wish to specify more than one structural variable, use a comma to separate the variables. As a result, each variable has its own memory allocation.
The typedef keyword allows you to use an existing data type to define a new data type name. Instead of creating new data, an alternative name for an existing data type is given.
struct student {
int rno;
char nm[20];
float fees;
};
typedef struct student std;
Initialization of Structure
The initializers are separated by commas and are contained in braces.
struct student std1 = {18, "Saurav", 125000};
The element declared inside the body of the structure can also be initialized or accessed using the dot operator (.).
struct rectangle {
int l;
int b;
};
r.l = 5;
r.b = 10;
Copying and Comparing Structures
A structure can be assigned to another structure of the same type.
Example
struct student s2 = {15, "Saurav", 12500};
struct student s1;
s1 = s2;
We can't do mathematical operations like +, -, *, /, and other comparable ones. Relational and equality operators are also incompatible with structure variables. Individual members of one structure, on the other hand, can be compared to members of another structure.
Array of Structures
An array of structure can also be created in the same way that we can make an array of integers or a character array. Multiple data types of the same kind can also be stored in an array of structures. So here we can have a common structure definition for all the employees.
struct employee {
int code;
char name[20];
char gender;
float sal;
};
struct employee emp1[10];
To allow the array of structural variables to be initialised at the time of declaration. We can write as follows:
struct employee emp1[3] = {{10, "Saurav", 'M', 1200}, {14, "Rani", 'F', 1100}, {15, "Rahul", 'M', 1400} };
Nested Structures
A structure can be contained within another structure, i.e. a structure can be a member of another structure.
struct employee {
int code;
float salary;
struct name {
char first_name[10];
char last_name[10];
}nm;
struct date_of_join {
int day;
int month;
int year;
}doj;
}e1;
name and date_of_join are both included in the employee structure in this case. Each of these structures has its own set of fields. We can access the members of the inner structure as well as other members by using the dot operator (.).
Pointer to a Structure
When you have a variable that contains a struct, you can use the dot operator to access its fields (.). If you have a pointer to a struct, however, this will not work. A pointer to a structure is never a structure itself, but rather a variable that retains the structure's address. You must use the arrow operator (->) to access its fields.
#include <iostream>
using namespace std;
struct student {
int rno;
char name [25];
float marks;
};
int main(){
struct student *s;
struct student st;
s = &st;
cout << "Enter Roll No Name Marks: ";
cin >> st.rno >> st.name >> st.marks;
cout << "Roll No is: " << s->rno;
cout << "\nName is: " << s->name;
cout << "\nMarks is: " << s->marks;
}
Structure as Function Argument
The call by value approach is inefficient for passing huge structures to functions. The parameters of each argument must match those of the function parameter. When there are a significant number of structure members, passing them is inefficient.
As a result, passing structures using pointers is recommended. A structure pointer could be passed to a function. The structure's address is passed as an actual argument in this situation.
#include <iostream>
using namespace std;
struct student {
int rno;
char name [25];
float marks;
};
void display(struct student *);
int main(){
struct student *s;
struct student st;
s = &st;
cout << "Enter Roll No Name Marks: ";
cin >> st.rno >> st.name >> st.marks;
display(s);
}
void display(struct student *p){
cout << "Roll No is: " << p->rno;
cout << "\nName is: " << p->name;
cout << "\nMarks is: " << p->marks;
}
Self Referential Structures
Structures that hold a reference to data of the same kind are known as self-referential structures. Self-referential structures are those that have one or more pointers that point to the same type of structure as its member.
struct node {
int val;
struct node *next;
}
Class
A class is a type that has been defined by the user. A class is a collection of class members, which can include variables, functions, types, and typedefs, as well as member class templates. It is the basic building block of an object-oriented programming language(OOP). A class is a set of objects that have common properties for data and certain programming parts.
The role of structure in C++ is expanded to form a class. Class instances are referred to as "objects," and each has the same organization as a class. An object has a physical reality, but a class is a logical abstraction. The class was created to support the object-oriented design paradigm while also providing far more capability than structures. The object is used to get access to the class's member functions and variables. A class contains:
- Data members: The data members are variables declared both inside and outside of the class declaration and functions.
- Member functions: A member function is a function that is declared within a class declaration.
The general syntax of the class keyword is
class classIdentifier{
class members list
};
Variable declarations and/or functions are included in the class members list. A semicolon is part of the syntax.
In C++, class is a reserved word that simply specifies a data type and does not allocate memory. It notifies that a class has been declared.
- If a class member is a variable, you define it the same way you would any other variable. Additionally, you cannot declare a variable and then initialize it in the class definition.
- If a class member is a function, the function prototype is often used to define that member.
- If a class member is a function, it has access to all members of the class, including data and function members.
Access Specifiers:
Member access specifiers divide a class's members into three categories: private, public, and protected.
- Public Access Specifier: Everyone has access
- Protected Access Specifier: Only the class itself, derived classes and friends have access
- Private Access Specifier: Only the class itself and friends have access.
The default access specifier of a class is all private by default. If a class member is private, you won't be able to see them outside of the class. Outside of the class, a public member can be accessed.
Constructors:
Because there is no assurance that the instance variables of a class will be populated when an object is instantiated, we use constructors. The constructor without the parameters is called the default constructor.
It has the following characteristics:
- The constructor's name is the same as the class's name. There can be several constructors in a class. All class constructors, however, have the same time.
- Constructor executes automatically when a class object enters its scope.
- If a class has many constructors, each one must have its own formal parameter list.
#include <iostream>
using namespace std;
class Employee {
public:
Employee() {
cout<<"Default Constructor Invoked"<<endl;
}
};
int main() {
Employee e1; //creating an object of Employee
Employee e2;
return 0;
}
Object Declaration
Once a class has been created, variables of that type can be declared. A class object, also known as a class instance, is a variable in a class. Objects are instances of classes. Constructors with arguments and default constructors are two sorts of constructors that may be found in a class. Therefore when you declare a class object either the default constructor executes or constructor with parameter executes.
The following is the standard syntax for defining a class object that calls the default constructor is
className classObjectName;
#include <iostream>
using namespace std;
class Student {
public:
string course;
int rno;
string name;
void studentDetails(){
cout << "Details are: \n";
cout << "Student Name is " << name;
cout << "\nRoll No is " << rno;
cout << "\nCourse Name is " << course;
}
};
int main()
{
Student std;
std.course = "Maths";
std.rno = 15;
std.name = "Rahul";
std.studentDetails();
return 0;
}
Accessing Class Members
Once a class object is declared, it has access to all of the class's members. To access a member of a class, an object uses the following syntax:
classObjectName.memberName
The class members that a class object can access are determined by the object's declaration location.
The object can access both public and private members if it is declared in the definition of a member function of the class.
If the object is declared elsewhere, it can only access the class's public members.
class Student
{
private:
int rno;
public:
int getRno()
{
return rno;
}
void setRollno(int i)
{
rollno=i;
}
};
int main()
{
Student A;
A.rollono=1; //Compile time error
cout << A.rollno; //Compile time error
A.setRollno(1); //Rollno initialized to 1
cout<< A.getRollno(); //Output will be 1
}
Similarities between Structure and Class
- In C++, structures and classes have the same syntactic meaning.
- The name of a class or structure can be used as a stand-alone type.
- Any of the members of a class or structure can be declared private.
- Inheritance procedures are supported by both class and structure.
- Constructors are allowed in both structure and class.
Which One Should You Choose?
Use structure if you're sure it actually logically represents a single value, like primitive types (int, double, etc.). When a small memory access is required to establish default values, the structure should be employed. If you want reference types, use classes.
You can select a class if you have a huge memory usage. If any of the members aren't public, use class instead of struct. If you want value types, use structure.
Key Difference Between a Structure and Class
Structure | Class |
A structure is a collection of variables of different data types with the same name. | A class in C++ is a single structure that contains a collection of related variables and functions. |
The struct keyword can be used to declare a structure. | The keyword class can be used to declare a class. |
Structure Declaration :struct struct_name { structure element 1; structure element 2; . . . structure element n; }; |
Class Declaration:class classIdentifier{ class members list}; |
Stack memory is used to store structures | Heap memory is used to hold classes. |
The structure variable is the name for a structure instance | The term 'object' refers to a class instance. |
In small programs, it's used. | It's employed in large programs. |
A destructor is not allowed in a structure. | A destructor can be added to a class. |
A structure is a value type. | A class is a reference type |
Null values are not permitted in structures | Null values are allowed in classes. |
All member variables are public in structure by default | By default, member variables and functions are private in classes. |
The members of the structure will be automatically initialized. | Constructors and destructors, on the other hand, are used to initialize class members. |
Summing Up
To conclude, you can choose a class if you have a huge memory usage. The structure, on the other hand, can be used to initialize default values when you have a short memory field or size. A structure is a value type whereas A class is a reference type. The structure in C++ has some drawbacks, such as the inability to hide data, which can be overcome through classes.
You may also like to read:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
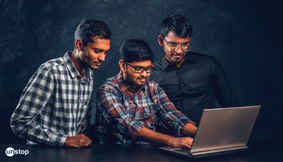
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
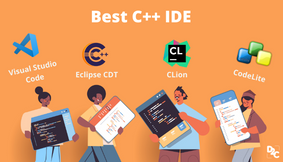
10 Best C++ IDEs That Developers Mention The Most!
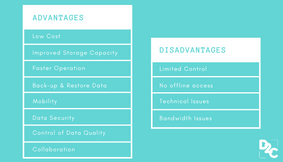
Advantages and Disadvantages of Cloud Computing that you should know!
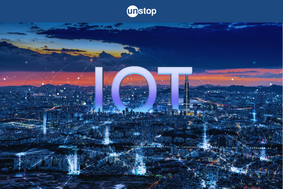
Comments
Add comment