Copy Constructor In C++ | Syntax, Types, Uses & More (+Examples)
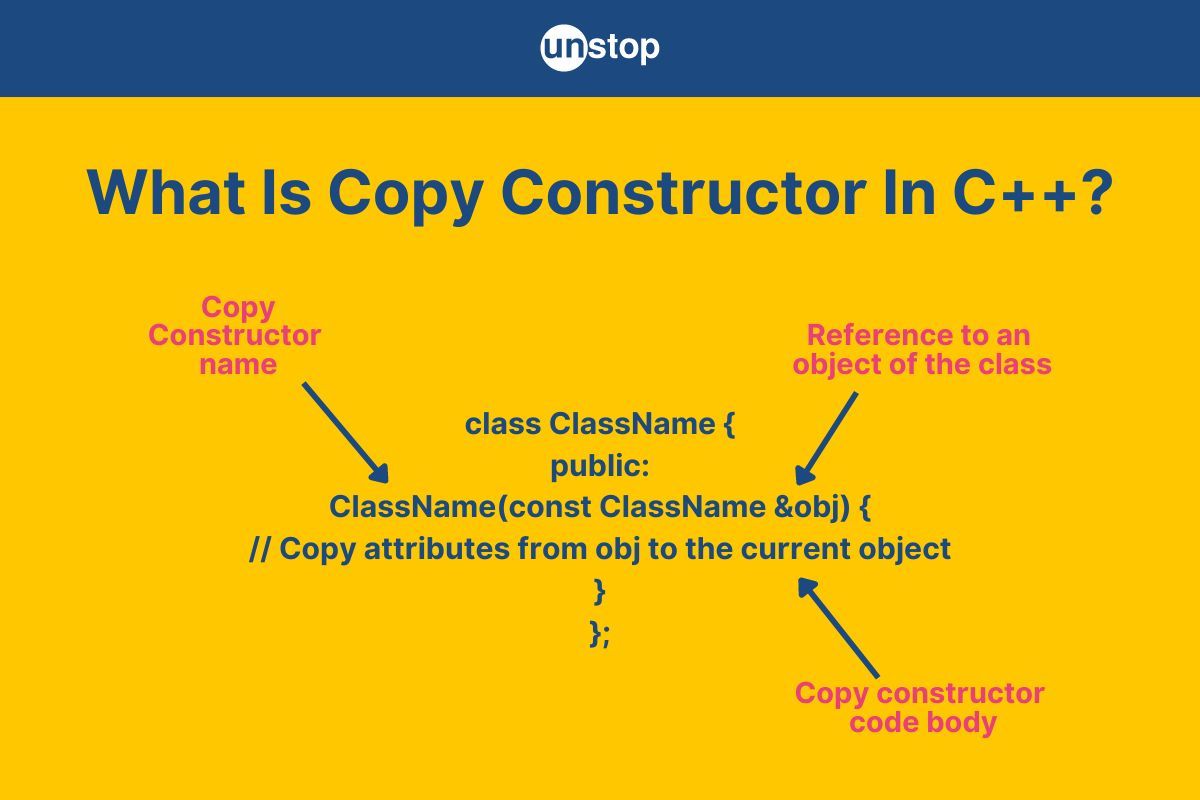
A constructor is a special member function that is called automatically when a class object is created. It initializes the respective object's data members, prepping it for use. There are multiple types of constructors, each with its own characteristics and use cases. In this article, we will focus on one of these types, the copy constructor in C++ programming.
We will discuss what it is, syntax, the use of copy constructor, its need, and more with examples.
What Is A Copy Constructor In C++?
A copy constructor in C++ language is a unique constructor that initializes a new class object by copying an existing object. It is automatically invoked when a new object is created from an existing one, whether through copying or passing by value of that object.
Imagine you have set up a magical workshop to create unique items. Every object has unique qualities, such as a name and a power level. Now, say you wish to make an item exactly like an already-existing object. Normally, it might be difficult to create an exact copy of something hand-made. But not in programming because the copy constructor in C++ provides the perfect way to get the job done. It's like making a magical copy of anything that has the same characteristics and abilities as that of the original one.
The use of a copy constructor in C++ resembles casting a spell to copy one object's characteristics into another one. They play a crucial role in ensuring that the copy is made correctly and that the new object is an independent instance with its own memory space/ distinct memory location. Let's look at the syntax of copy constructor followed by an example.
Syntax Of Copy Constructor In C++
class ClassName {
public:
ClassName(const ClassName &obj) {
// Copy attributes from obj to the current object
}
};
Here,
- ClassName: is the name of the class being defined, which will be the same as its constructors.
- ClassName() refers to the constructor where the braces() contain the argument.
- Expression const ClassName &obj is the constructor argument, where &obj is a reference to the object we want to copy (from the same class).
- The object argument is marked constant (const) because the source object is not being modified in copying.
Example Of Copy Constructor In C++
In the example below, we will define a class and then showcase the implementation of a copy constructor in C++ programming.
Code Example:
Output:
Person 1: Alia, 25 years
Person 2: Alia, 25 years
Explanation:
We begin the C++ program example by including the necessary header file to use input/output stream functionalities.
- We then define a class named Person with two public member variables, name of string data type and age of integer data type. These are used to store the person's name and age.
- As mentioned in code comments, the class also has a parameterised constructor, which initializes the variables name and age using the constructor's parameters.
- We then define a copy constructor, which takes a reference to another Person object and copies the values of name and age to the new object.
- Inside the main() function, we first create an instance of Person class named person1 and initialize its data member name with the string value- "Alia" and age 25 using parameterized constructor.
- Next, we create another Person object called person2 and initialize it with person1. This invokes the copy constructor, which copies the name and age values from person1 to person2.
- Following this, we use the cout commands to print the attributes, name, and age of both person1 and person2.
- Finally, the main function returns 0, indicating successful execution without any errors.
Characteristics Of Copy Constructors In C++
Copy constructors play a crucial role in ensuring proper copying of data members and resources between objects. Here are the key characteristics of copy constructors in C++:
- Name and Signature: The copy constructor in C++ has the same name as the class and takes a single argument of the same class type (by reference or by value). Its primary purpose is to create a new object by copying the contents of another object.
- Usage: A copy constructor in C++ can be invoked in several scenarios, including when an object is being passed by value as a function argument, when an object is being returned by value from a C++ function, and when an object is being explicitly or implicitly copied.
- Default Copy Constructor: If you do not provide a copy constructor in your C++ class, the compiler generates a default copy constructor which performs a shallow copy. That is, this compiler-created copy constructor in C++ copies the values of the data members without considering any dynamically allocated resources.
- Custom Copy Constructor: If your class involves dynamically allocated memory, file handles, or other resources, you should define a custom copy constructor to ensure proper copying and management of these resources. This copy constructor in C++ should allocate new resources and copy the contents of the source object appropriately.
- Reference Parameter: The copy constructor in C++ usually takes its argument by reference (const reference if the source object is not being modified) to avoid unnecessary copying and improve performance. This way, the constructor works with the actual object, and no additional copy is made during the invocation.
- Deep vs. Shallow Copy: The copy constructor in C++ is responsible for deciding whether to perform a shallow copy or a deep copy. A shallow copy simply copies the values of data members, while a deep copy involves creating new copies of dynamically allocated resources.
- Initialization Lists: You should use an initializer list inside the copy constructor in C++ to initialize the data members of the new object. This helps to avoid unnecessary default construction initializations and improve efficiency.
- Const-Correctness: If you're copying from a const object, your copy constructor should also be able to accept the const reference type variables as its parameter to ensure const-correctness.
- Explicitly Deleted Copy Constructor: You can explicitly delete the copy constructor in C++ by using the delete operator. This prevents instances of your class from being copied.
Types Of Copy Constructors In C++
Just as artisans can craft replicas in various styles, copy constructors in C++ also come in different forms to suit specific copying needs. While the classic copy constructor performs a direct replica, some scenarios demand a more intricate approach.
This is where the concept of types of copy constructors comes into play. From deep copies that meticulously clone every detail to the default copy constructor that works silently in the background, each type has its purpose and intricacies.
Default Copy Constructor In C++
In C++, the default copy constructor is a built-in feature provided by the compiler. It's automatically generated when a class doesn't have a user-defined copy constructor. The default copy constructor in C++ performs a member-wise copy of the attributes from the source object to the newly created object. This process involves copying each attribute's value directly, which can lead to shallow copies if the attributes involve pointer variables or dynamically allocated memory.
Rules/ Points To Note When Working With Default Copy Constructor In C++
- When is it Generated: The default copy constructor in C++ is generated by the compiler when a class doesn't have any user-defined copy constructor.
- Member-Wise Copy: The default copy constructor in C++ copies each attribute from the source object to the new object in a sequential manner.
- Shallow Copy: For attributes involving pointers or dynamically allocated memory, the default copy constructor in C++ performs a shallow copy. Meaning it copies the memory addresses, not the actual data. This can sometimes lead to multiple objects pointing to the same memory location, potentially causing unintended side effects.
- Automatic Initialization: You don't need to invoke the default copy constructor explicitly. It is automatically invoked when a new object is created using an existing object.
- Accessibility: The default copy constructor in C++ has the same accessibility as the class itself. If the class is defined as public, the copy constructor is accessible from outside the class.
- User-Defined Constructors: If a class has any user-defined constructors, the default copy constructor won't be generated. This emphasizes the need to explicitly define a copy constructor in C++ whenever specific copying behaviour is required.
- Overriding: You can provide your own copy constructor by defining it explicitly in the class. This will override the default behaviour.
- Default Copy Constructor Limitations: While suitable for simple classes with basic attributes, the default copy constructor in C++ may not be sufficient for classes with dynamic memory management or complex relationships between attributes.
Syntax Of Default Copy Constructor In C++
The syntax of the default copy constructor is not explicitly written in the code, as it is automatically generated by the compiler when a user-defined copy constructor is not provided. However, here is a conceptual idea of how the default copy constructor works:
ClassName::ClassName(const ClassName &source) {
// Perform member-wise copy of attributes from source object to this object
}
Here,
- ClassName::ClassName: This is the constructor declaration, where ClassName is the name of the class, as well as the constructor.
- (const ClassName &source): This is the parameter list of the constructor, which takes a const reference of an object of the same class. The ampersand (&) is used to pass the object by reference, and the const keyword is used to prevent the object from being modified.
Please note that you don't typically write or see the default copy constructor in code. It is generated implicitly by the compiler when needed. If you want to provide specific copying behavior or handle dynamic memory, it's recommended to define your own copy constructor explicitly.
Code Example:
Output:
Person 1: Alice, 25 years
Person 2: Alice, 25 years
Explanation:
This example is a continuation of the first example above, which has a Person class with two private data members: name (of type string) and age (of type int).
- The class also has a constructor that initializes the data members, i.e., name with value n, and age with value a (i.e., Person(string n, int a)).
- Note that since there is no user-defined copy constructor within the class, the compiler generates a default copy constructor.
- Then, inside the main() function, we create an object of class Person, called person1, with values Alia and 25, assigned to variables name and age, respectively.
- After that, we create another object, person2, as a copy of the person1 object. This invokes the default copy constructor, which duplicates the name and age values from person1 into person2.
- Next, we use the cout commands to print the attributes of both person1 and person2.
- Finally, the main() function returns 0 to indicate successful program completion.
User-Defined Copy Constructors In C++
In order to conduct a particular type of copying when creating a new object from an existing one, you must declare a user-defined copy constructor within a class. This will give you greater control over how attributes and resources are replicated in contrast to the default copy constructor in C++ programs, which executes a member-wise shallow copy.
Rules For Creating User-defined Copy Constructor In C++
- Constructor Declaration: The user-defined copy constructor in C++ has the same name as the class, and its parameter is a reference to an object of the same class type, typically marked as const.
- Explicit Definition: You need to define the user-defined copy constructor within the class definition explicitly.
- Customized Copying: You have the freedom to decide how attributes and resources are copied from the source object to the new object. This is especially useful for classes involving dynamic memory or complex copying requirements.
- Deep Copying: User-defined copy constructors in C++ are often used to achieve deep copying, where dynamically allocated memory is duplicated to ensure independent instances.
- Shallow vs. Deep Copy: Depending on the scenario, you can implement either a shallow copy (copying memory addresses) or a deep copy (copying actual data) as needed.
Syntax Of User-Defined Copy Constructor In C++:
class ClassName {
public:
// Attributes and other member functions
// User-defined copy constructor
ClassName(const ClassName &source) {
// Copy attributes and resources from the source to this object
}
};
Here,
- The class keyword is used to define a class, while ClassName refers to the name of this class and its constructor.
- ClassName(const ClassName &source) is the signature of the user-defined copy constructor. It takes a constant reference to another object of the same class type as its parameter. The parameter name source is just a placeholder; you can use any valid name.
- And the curly brackets {} contain the body of the copy constructor.
Code Example:
Output:
Number 1: 42
Number 2: 42
Explanation:
In the example C++ program-
- We first define a class named Number with a public data member, value of int data type. Inside the class-
- We have a constructor that initializes the variable value with the integer n provided as an argument.
- Next, we also define a user-defined copy constructor that takes a constant reference to other object of Number class as a parameter (i.e., cont Number &other).
- It copies the value variable from the other object to the current object.
- In the main() function, we create an object of the Number class called num1 and initialize it with the value 42 using the constructor.
- Then, we create another object num2, as a copy of the object num1. This invokes the user-defined copy constructor, which copies the value from num1 to num2.
- Finally, we print the values for both num1 and num2 using cout commands.
When Do We Call The Copy Constructor In C++?
A copy constructor in C++ is typically used when you want to initialize a new object using the values of an existing object of the same class. They are particularly useful when you want to create a deep copy of an object to avoid issues with shared references. Some common situations where we use a copy constructor in C++ are explained ahead.
To Create A Copy Of An Existing Object
This happens when you initialize a new object using an existing object's data. This can occur during object declaration, passing objects by value or returning objects by value from functions.
Code Example:
Output:
obj2.value: 10
Explanation:
In the above code example-
- We define a class called MyClass with a public data member variable value of type int. The class also contains-
- A parameterized constructor that takes an integer val as an argument and assigns it to the value member variable.
- A copy constructor that takes a constant reference to other object of MyClass (i.e., MyClass& other) as a parameter. This copies the value from the other object to the current object.
- In the main() function, we create an object of MyClass called obj1 and initialize it with the value 10 using the parameterized constructor.
- Next, we create another object, obj2, and initialize it with the value of obj1 using the copy constructor.
- Then, we print the value of obj2 using the cout command and the dot operator.
When Objects Are Passed By Value As Function Arguments
When you pass an object by value to a function, a copy of the object is made and sent to the function. This copy is created using the copy constructor in C++.
Code Example:
Output:
Inside function: 10
Explanation:
In the above code example-
- We define a class MyClass, that contains a public member variable, value, of type int. The class also contains-
- A parameterized constructor, which initializes val to the given parameter value.
- A copy constructor that takes a reference to other object of MyClass as parameter. It copies the value from the other object to the current object.
- A member function called displayObject() takes an object of MyClass as a parameter. It prints the value of the argument passed to it using the cout command.
- Inside the main() function, we create an object of the MyClass called obj1 and initialize it with the value 10 using the parameterized constructor.
- Next, we call the displayObject() function on the class object obj1. When this happens a copy of obj1 is passed to the function, which is created by the copy constructor.
- Effectively, the function prints the value of the object's data members to the console.
When Objects Are Returned By Value From Functions
When a function returns an object by value, a copy of the object is created using the copy constructor in C++ programs and returned to the caller.
Code Example:
Output:
obj2.value: 20
Explanation:
In the above code example-
- A class called MyClass is defined in the code above, which contains a public member variable called value (type integer). The class includes-
- A parameterized constructor which initializes the given parameter value with val.
- A copy constructor that takes 'const MyClass& other' reference as its parameter. It copies the value from the other object to the current object.
- The createObject() function returns a MyClass object initialized with the value 20. It invokes the copy constructor when returning the object.
- In the main() function, we create a temporary object of MyClass, obj2, using the createObject() function.
- This invokes the copy constructor is invoked as the temporary object is returned and assigned to obj2.
- The cout command is then used to print the value of obj2 to the console
- The program returns 0 to indicate successful execution.
When Is A User-Defined Copy Constructor Needed In C++?
A user-defined copy constructor in C++ is needed when the default copy constructor provided by the compiler does not fulfill the requirements for copying objects properly.
- The default copy constructor performs a shallow copy, which means it copies the values of member variables directly, including any pointer variables.
- This can lead to issues if the class contains dynamically allocated memory, pointers, or other resources that must be managed properly.
- In such cases, a user-defined copy constructor can be used to ensure a deep copy or to properly manage resources during copying.
Here are some scenarios when a user-defined copy constructor in C++ is needed:
- Dynamic Memory Allocation: If your class contains dynamically allocated memory (e.g., using the new operator to allocate memory during the time of object creation), a shallow copy performed by the default copy constructor will result in multiple objects sharing the same memory, leading to potential memory leaks or double deletion. In this case, a user-defined copy constructor in C++ can properly manage the memory by creating a new copy of the dynamically allocated memory for the new object.
- Resource Management: If your class manages external resources such as file handles, network connections, or other external entities, the default copy constructor in C++ might not handle these resources correctly. A user-defined copy constructor can ensure that each copy of the object maintains its own set of resources and properly handles their lifecycle.
- Non-shareable References: If your class contains pointers to other objects, a shallow copy will result in multiple objects pointing to the same memory locations. If one object modifies the pointed-to data, it could affect other objects unintentionally. A user-defined copy constructor in C++ can create a new set of pointers and copy the pointed-to data, resulting in a more independent copy.
- Customized Behavior: In some cases, you might want to implement specific behavior during the copy operation, such as logging, validation, or additional initialization steps. A user-defined copy constructor allows you to implement such custom behavior.
- Inheritance and Polymorphism: If your class is part of an inheritance hierarchy or participates in polymorphism, a proper copy constructor can help maintain the correct behavior of derived classes when copied.
Check out this amazing C++ course and participate in a coding sprint to supercharge your C++ programming skills!
Types Of Constructor Copies In C++
In C++, constructors can be classified into two main types based on how they perform copying, i.e., shallow copy and deep copy.
Shallow Copy Using Copy Constructor In C++
A shallow copy is a special type of copy where the values of the member variables of an object are copied directly to another object. If the class contains pointers or references to external resources, the copy process merely duplicates the memory addresses, leading to multiple objects sharing the same resources. The downside of a shallow copy is that changes made to the shared resources in one object can affect other objects as well.
Code Example:
Output:
obj1.data: 5
obj2.data: 5
After modifying obj1.data...
obj1.data: 10
obj2.data: 10
Explanation:
In the above code example-
- We define a class named ShallowCopyExample, which has a public member variable data, which points to an integer stored on the heap.
- The class includes a constructor which initializes the data pointer with a dynamically allocated integer having the value val.
- We also define a shallow copy constructor which takes a reference to other class objects as a parameter and copies the value of the data pointer from that to the object.
- This results in the data member of both objects pointing to the same memory address and sharing the same location for the integer value.
- In the main() function, we create two objects of the ShallowCopyExample class, called obj1 and obj2. Here, we initialize obj1 with the value 5 using the simple constructor.
- Next, we initialize obj2 by creating a shallow copy of obj1, using the assignment operator, i.e., ShallowCopyExample obj2 = obj1). This results in obj2 sharing the same data pointer as obj1.
- We then use the pointer to obj1 and obj2 with cout commands to display the initial data pointed to by both objects.
- After that, we modify the value pointed to by obj1 to 10, using the pointer, i.e., *obj1.data=10.
- We once again use the cout command to print the modified data pointer to by obj1.data and obj2.data.
- The output demonstrates that since both objects share the same memory address for their data pointers, modifying obj1.data also affects obj2.data.
Deep Copy Using Copy Constructor In C++
Deep copy is also known as deep clone or deep duplication. It relates to recursively creating new copies of all the objects that an object references. To put it another way, a deep copy is when an entirely separate copy of the original object is created, replete with all of its nested objects and their data.
Changes made to the copied item or its nested objects will not affect the original object or its nested objects when you conduct a deep copy, and vice versa. The deep copy of each thing completely differs from its original object counterpart in every way.
Code Example:
Output:
obj1.data: 5
obj2.data: 5
After modifying obj1.data...
obj1.data: 10
obj2.data: 5
Explanation:
In this example, we define a DeepCopyExample class to demonstrate the deep copy behavior.
- The class has a public integer pointer called data and a constructor that initializes the data pointer member with a dynamically allocated integer, using the value passed as an argument.
- It also has a deep copy constructor that takes a reference to other class objects as a parameter and creates an independent copy of it.
- Inside this constructor, we use the new operator to create a new interger whose value is copied from the other object's data member. (Comparing this with the previous example shows the difference between the copy creation methods)
- Next, we define a destructor to properly deallocate the dynamically allocated memory for data pointer members using the delete operator.
- In the main() function, we create an instance of DeepCopyExample named obj1 and initial it with the value of 5.
- Next, we initialize the object obj2 by equating it to obj1. This invokes the copy constructor to make a deep copy of obj1. It ensures that the integer values are copied from obj1, while creating a new integer variable with separate memory space.
- Then, we print the value pointed to by the data pointer member of obj1 and obj2 to the console using std::cout.
- After that, we modify the value pointed to by obj1 data pointer member to 10, accessing it using a dot pointer.
- We once again, print the values pointed to by both object members to the console, using std::cout.
- The output shows that the value pointed to by obj2 remains unchanged. This is because we created a deep copy and any change in obj1 will not affect obj2, and vice-versa.
Can We Make The Copy Constructor In C++ Private?
Yes, you can make the copy constructor in C++ private to prevent instances of a class from being copied. This is a technique often used to enforce a design pattern called the Singleton pattern, where you want to ensure that only one instance of a class can exist during the lifetime of the program.
Use Cases for Private Copy Constructor in C++ are:
- Singleton Pattern: In the Singleton design pattern, where you want to ensure that only one instance of a class can exist, making the copy constructor private prevents the creation of additional instances through copying.
- Immutable Classes: If you're designing an immutable class where instances cannot be modified after creation, a private copy constructor in C++ prevents the creation of mutable copies of the object.
- Preventing Direct Copying: In cases where you want to enforce certain rules or restrictions when copying objects, making the copy constructor in C++ private gives you control over how objects are copied.
Let's look at an example showcasing its implementation for a better understanding.
Code Example:
Output:
obj1.getData(): 10
Explanation:
In the above code example,
- We include the namespace to use names from the standard library without the std:: prefix.
- Then, we define a class named Singleton, containing a private integer member variable data and a private constructor Singleton(int val). The constructor takes the integer argument val and initializes the private member data with the provided access value.
- Here, the private access specifier indicates that the respective class members will only be accessible from within the class itself.
- Next, we define a public static member function getInstance() which creates an instance of the class and returns a reference to the object (i.e., static Singleton& getInstance()).
- This function is the only way to access the instance of Singleton class (which is private).
- The static keyword ensures that the instance is created only once on the first call and all other subsequent calls to the function return the same object.
- Then, we have a public member function getData() which provides access to the private data member data. The const keyword ensures that the function doesn't modify any member variables.
- The public access modifier in the above two methods, indicates that they are accessible from outside the class.
- Next, we begin the main() function, which serves as the starting point of the program's execution.
- Inside main(), as mentioned in the comment, we attempt create an instance of Singleton using the copy constructor. But this is not possible since the copy constructor is private and cannot be accessed here.
- So, we call the getInstance() method to create an instance of the Singleton class using the scope resolution operator and initialize its data member with value 10.
- We assign the object created to obj1 using reference/ address of operator.
- Following this, we call the getData() method to access the object obj1 and print the same to the console using cout statement.
Assignment Operator Vs Copy Constructor In C++
Although both the copy constructor and assignment operator deal with copying data between objects, the copy constructor is typically used when creating new class objects, while the assignment operator is used to update an already existing object. Here's a table outlining the difference between assignment operator and copy constructor in C++:
Aspect |
Copy Constructor |
Assignment Operator |
Purpose |
Copies the data from the source object to the new object, creating a new memory location if needed. |
Copies data from the source object to an existing target object, potentially reusing existing memory. |
Syntax |
ClassName newObj = existingObj; |
existingObj1 = existingObj2; |
Memory Allocation |
Can allocate new memory for the new object if necessary. |
Reuses the memory of the target object, which should already be allocated. |
Copy Control |
Often used when objects are passed by value or returned by value from functions. |
Used to assign the values of one object to another, particularly after both are already initialized. |
Example |
MyClass newObj = existingObj; |
existingObj1 = existingObj2; |
Example Of Class Where A Copy Constructor Is Essential
In general, if your class involves any of the following scenarios, you should provide your own copy constructor to ensure correct behavior:
- Dynamic memory allocation: If your class allocates memory dynamically (using new or malloc), the default copy constructor will only copy the memory address, resulting in shallow copies and memory leaks or double deallocations.
- Pointers to external resources: If your class holds pointers to external resources (files, databases, network connections), a proper copy constructor should handle these resources appropriately.
- Complex interactions: If your class has complex internal interactions that need to be duplicated properly when making a copy, the default copy constructor might not suffice.
Code Example:
Output:
Original Data: 5
Copied Data: 5
Explanation:
In the above code example-
- We define a class named DynamicResource to manage a dynamically allocated integer resource.
- The class has a private member, data, which is a pointer to an integer. This pointer will be used to store and manipulate the integer value dynamically allocated on the heap.
- Then, we define a public constructor, which allocates memory for an integer variable on the heap (using new operator), initializes it with a value val, and assigns its address to data pointer.
- We also define a custom copy constructor, i.e., DynamicResource(), which takes reference to other object and creates a deep copy of the resource by allocating new memory for an integer.
- It then copies the value from data pointer of the other object into this newly allocated memory.
- The class also contains a member function getData() that returns the value pointed to by the data pointer. This function is marked as const because it does not modify any member variables.
- Next, we have a destructor ~DynamicResource(), which deallocates the dynamically allocated memory to the data member using the delete operator.
- In the main() function-
- We first create an instance of DynamicResource named originalResource, initializing it with the value 5.
- We then create another instance, copiedResource, by copying originalResource. This invokes the custom copy constructor, which creates a new DynamicResource object with its own separate copy of the integer value.
- The data stored in both objects is displayed using getData() function.
- Finally, the main() function returns 0 to indicate successful execution.
What Happens When We Remove Copy Constructor From The Above Code?
If we remove the custom copy constructor from the provided code, the default copy constructor generated by the compiler will be used instead. However, the default copy constructor performs a shallow copy, which can lead to significant problems due to the presence of dynamically allocated memory in the DynamicResoruce class.
Here's what would happen if you remove the custom copy constructor:
- Default Copy Constructor (Shallow Copy): The compiler-generated default copy constructor would copy the value of the data pointer from the source object to the new object. This would result in two objects sharing the same dynamically allocated memory.
- Memory Issues: Since both the original object and the copied object would point to the exact memory location, the following problems could arise:
- If one of the objects is destructed or its DynamicResource destructor is called, it would delete the shared memory, leaving the other object with a dangling pointer.
- If one of the objects modifies the shared memory, it will affect the other object, leading to unintended behaviour and crashes.
- Resource Leaks: When both the original object and copied object are destructed, they would both attempt to delete the same memory location. This would result in a double deletion, leading to undefined behaviour.
Uses Of Copy Constructors In C++
Copy constructors in C++ play a crucial role in managing resources and ensuring proper object copying. Here are some important uses of the copy constructor:
-
Copying Objects: The primary use of a copy constructor is to create a new object as a copy of an existing object. This is essential when an object is passed by value to a function or returned by value from a function.
-
Deep Copying: In cases where an object contains pointers to dynamically allocated memory, a copy constructor is used to perform a deep copy, ensuring that the new object gets its own copy of the resources rather than just copying the pointer. This prevents issues like double deletion.
-
Object Initialization: Copy constructors are invoked when an object is initialized using another object of the same type, either during declaration or assignment. This is important for initializing objects in classes that manage resources like memory, file handles, or network connections.
-
Passing Objects by Value: When an object is passed by value to a function, the copy constructor is used to create a copy of the object. This ensures that the original object remains unchanged, and any modifications are made to the copy.
-
Returning Objects by Value: When a function returns an object by value, the copy constructor is called to create a temporary copy of the object being returned. This ensures that the object is properly transferred to the caller without altering the original object within the function.
-
Storing Objects in Containers: When storing objects in containers like std::vector or std::list, the copy constructor is often used to copy elements as the container resizes or reorders its elements. This ensures that the objects are properly managed and remain consistent.
Conclusion
The copy constructor in C++ is like a special recipe for making copies of objects. Just like when you want to duplicate a drawing, you have to follow a specific set of steps. Similarly, the copy constructor outlines how to create a new object that's an exact copy of another.
By learning about shallow and deep copying, we understand how to copy not only the surface details but also any hidden parts. Copy constructors in C++ help prevent messes, like forgetting to clean up after yourself or losing important information when copying. This knowledge helps us build software that's not only reliable but also efficient and well-organized. All in all, just like a recipe, a copy constructor in C++ lets us create duplicate objects with the right ingredients and steps.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. Why do we use const in the copy constructor in C++?
In C++, using const (constant) in the copy constructor's parameter serves multiple important purposes. Let's explore why const is used in the copy constructor:
-
Preventing Modification of Source Object: By using const in the copy constructor's parameter, you are indicating that the source object being copied should not be modified within the copy constructor. This helps ensure that the copy constructor does not unintentionally modify the source object's state, maintaining the object's integrity.
class MyClass {
public:
// Copy constructor with const parameter
MyClass(const MyClass &other) {
// 'other' is treated as read-only
// ...
}
};
- Allowing Const and Non-Const Source Objects: Using const in the copy constructor's parameter allows you to copy both constant (const MyClass obj;) and non-constant source objects (MyClass obj;). If the object with parameter were not const, you would only be able to copy non-const source objects.
- Supporting Temporary Objects: When using the copy constructor in C++ to copy temporary objects (created during expressions), a const reference in the copy constructor allows you to handle these temporary objects effectively.
- Consistency with Immutable Objects: If your class or its members are designed to be immutable (cannot be modified after creation), using const in the copy constructor in C++ reinforces this immutability concept by indicating that the source object won't be modified.
Q. Is the copy constructor in C++ automatically generated?
Yes, if you don't explicitly define a copy constructor in your C++ class, the compiler will automatically generate a default copy constructor for you.
- This default copy constructor in C++ performs a member-wise copy of the class's data members.
- It essentially creates a shallow copy, which might not be suitable for classes that manage dynamic runtime resource allocation or require special copying logic.
- The automatically generated copy constructor has the following signature:
ClassName(const ClassName &other);
Here, ClassName represents the name of your class.
It's important to note that while the automatically generated copy constructor in C++ is convenient, it might not work correctly for classes that involve deep copying or resource management. If your class contains pointers or dynamically allocated memory, you might need to define a custom copy constructor to ensure proper copying and resource handling.
Q. What are the two features of a copy constructor in C++?
The two primary features of a copy constructor in C++ are:
- Creation of a New Object: The primary purpose of a copy constructor in C++ is to create a copy of an existing object. It initializes the new object's member variables with the values of the corresponding member variables of the source object.
- Passive Invocation: The copy constructor in C++ is invoked automatically by the C++ compiler when a new object is being created as a copy of an existing object. It is called implicitly and is not directly invoked in the code sample.
Q. Does the copy constructor in C++ return a value?
No, a copy constructor in C++ does not return a value because the purpose of a copy constructor is to create a new object by copying the content of an existing object. It is responsible for initializing the new object's member variables with the values of the corresponding member variables of the source object. The copy constructor in C++ is invoked when an object is being created as a copy of another object, typically during initialization or when objects are passed by value.
Q. What is the difference between Copy Constructor and Cloning?
Aspect |
Copy Constructor |
Cloning |
Purpose |
Creates a new object as a copy of an existing object. |
Creates a new object as an independent copy of another object. |
Implementation |
Part of the class definition, invoked when a new object is created from an existing object. |
Typically implemented using a special member function or a specialized interface. |
Deep Copy VS Shallow Copy |
The copy constructor may create either a shallow copy (copying references) or a deep copy (copying the actual data). |
The cloning process can be designed to ensure deep copying, creating separate copies of the data. |
Use Cases |
Used when you want to create a copy of an object within the same class. |
Useful when you want to create a new object that's similar to the source object but possibly modified in some way. |
Flexibility |
Limited to copying objects of the same class. |
Can be implemented to clone objects across different classes through common interfaces. |
Q. What happens if there is no copy constructor in C++?
If you don't provide a copy constructor for a class, C++ will automatically generate a default copy constructor for you. This default constructor performs a member-wise copy of the class's data members. However, this default copy constructor in C++ might not work correctly for classes that contain dynamically allocated resources (like pointers), leading to unexpected behavior and sharing of memory issues.
Here's what happens if there is no copy constructor or if the default copy constructor in C++ is used:
- Shallow Copy: The default copy constructor in C++ performs a shallow copy of the object's data members. This means that it simply copies the default values of the member variables. If your class contains pointers or dynamically allocated memory, the copy will copy the pointers, not the actual data they point to.
- Shared Resources: If the original object (let's call it objectA) and the copied object (objectB) both contain pointers pointing to the same dynamically allocated memory (like an array or another object), modifying the data through one object will affect the data accessed through the other object. This can lead to unintended side effects and bugs.
- Memory Leaks and Double Deletion: If your class involves dynamically allocated memory and the default copy constructor in C++ is used, there can be memory leaks or double deletion issues. When the copied object goes out of scope, it might delete the same memory that the original object is still using.
- Inadequate Resource Management: The default copy constructor in C++ doesn't account for proper resource management. If your class manages resources that need cleanup (e.g., file handles, network connections), the copied object might not handle these resources correctly.
To address these issues, in a class with separate memory allocations or other resources, it's important to define a custom copy constructor, which creates new copies of dynamically allocated data to handle resource management properly. This way, a custom copy constructor in C++ can help ensure that each object operates independently without causing memory leaks or unintended sharing of resources.
You might also be interested in reading the following:
- What is Function Prototype In C++ (Definition, Purpose, Examples)
- Structure of C++ Programs Explained With Examples
- C++ Templates | Class, Function, & Specialization (With Examples)
- C++ If-Else & Other Decision-Making Statements (+Examples)
- OOPs Concept In C++ | A Detailed Guide With Codes & Explanations
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment