C++ Programming Language
Table of content:
- A Brief Intro To C++
- The Timeline Of C++
- Importance Of C++
- Versions Of C++ Language
- Comparison With Other Popular Programming Languages
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Variables In C++?
- Declaration & Definition Of Variables In C++
- Variable Initialization In C++
- Rules & Regulations For Naming Variables In C++ Language
- Different Types Of Variables In C++
- Different Types of Variable Initialization In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Primitive Data Types In C++?
- Derived Data Types In C++
- User-Defined Data Types In C++
- Abstract Data Types In C++
- Data Type Modifiers In C++
- Declaring Variables With Auto Keyword
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Structure Of C++ Program: Components
- Compilation & Execution Of C++ Programs | Step-by-Step Explanation
- Structure Of C++ Program With Example
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What is Typedef in C++?
- The Role & Applications of Typedef in C++
- Basic Syntax for typedef in C++
- How Does typedef Work in C++?
- How to Use Typedef in C++ With Examples? (Multiple Data Types)
- The Difference Between #define & Typedef in C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Strings In C++?
- Types Of Strings In C++
- How To Declare & Initialize C-Style Strings In C++ Programs?
- How To Declare & Initialize Strings In C++ Using String Keyword?
- List Of String Functions In C++
- Operations On Strings Using String Functions In C++
- Concatenation Of Strings In C++
- How To Convert Int To Strings In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is String Concatenation In C++?
- How To Concatenate Two Strings In C++ Using The ‘+' Operator?
- String Concatenation Using The strcat( ) Function
- Concatenation Of Two Strings In C++ Using Loops
- String Concatenation Using The append() Function
- C++ String Concatenation Using The Inheritance Of Class
- Concatenate Two Strings In C++ With The Friend and strcat() Functions
- Why Do We Need To Concatenate Two Strings?
- How To Reverse Concatenation Of Strings In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Find In String C++?
- What Is A Substring?
- How To Find A Substring In A String In C++?
- How To Find A Character In String C++?
- Find All Substrings From A Given String In C++
- Index Substring In String In C++ From A Specific Start To A Specific Length
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Pointers In C++?
- Pointer Declaration In C++
- How To Initialize And Use Pointers In C++?
- Different Types Of Pointers In C++
- References & Pointers In C++
- Arrays And Pointers In C++
- String Literals & Pointers In C++
- Pointers To Pointers In C++ (Double Pointers)
- Arithmetic Operation On Pointers In C++
- Advantages Of Pointers In C++
- Some Common Mistakes To Avoid With Pointers In Cpp
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Understanding Pointers In C++
- What Is Pointer To Object In C++?
- Declaration And Use Of Object Pointers In C++
- Advantages Of Pointer To Object In C++
- Pointer To Objects In C++ With Arrow Operator
- An Array Of Objects Using Pointers In C++
- Base Class Pointer For Derived Class Object In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is 'This' Pointer In C++?
- Defining ‘this’ Pointer In C++
- Example Of 'this' Pointer In C++
- Describing The Constness Of 'this' Pointer In C++
- Important Uses Of 'this' Pointer In C++
- Method Chaining Using 'this' Pointer In C++
- C++ Programs To Show Application Of 'This' Pointer
- How To Delete The ‘this’ Pointer In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What is Reference?
- What is Pointer?
- Comparison Table Of C++ Pointer Vs. Reference
- Differences Between Reference And Pointer: A Detailed Explanation
- Why Are References Less Powerful Than Pointers?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- How To Declare A 2D Array In C++?
- C++ Multi-Dimensional Arrays
- Ways To Initialize A 2D Array In C++
- Methods To Dynamically Allocate A 2D Array In C++
- Accessing/ Referencing Two-Dimensional Array Elements
- How To Initialize A Two-Dimensional Integer Array In C++?
- How To Initialize A Two-Dimensional Character Array?
- How To Enter Data In Two-Dimensional Array In C++?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Arrays Of Strings In C++?
- Different Ways To Create String Arrays In C++
- How To Access The Elements Of A String Array In C++?
- How To Convert Char Array To String?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Memory Allocation in C++?
- The “new" Operator In C++
- The "delete" Operator In C++
- Dynamic Memory Allocation In C++ | Arrays
- Dynamic Memory Allocation In C++ | Objects
- Deallocation Of Dynamic Memory
- Dynamic Memory Allocation In C++ | Uses
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A Substring In C++ (Substr C++)?
- Example For Substr In C++
- Points To Remember For Substr In C++
- Important Applications Of substr() Function
- How to Get a Substring Before a Character?
- Print All Substrings Of A Given String
- Print Sum Of All Substrings Of A String Representing A Number
- Print Minimum Value Of All Substrings Of A String Representing A Number
- Print Maximum Value Of All Substrings Of A String Representing A Number
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Operator In C++?
- Types Of Operators In C++ With Examples
- What Are Arithmetic Operators In C++?
- What Are Assignment Operators In C++?
- What Are Relational Operators In C++?
- What Are Logical Operators In C++?
- What Are Bitwise Operators In C++?
- What Is Ternary/ Conditional Operator In C++?
- Miscellaneous Operators In C++
- Precedence & Associativity Of Operators In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is The New Operator In C++?
- Example To Understand New Operator In C++
- The Grammar Elements Of The New Operator In C++
- Storage Space Allocation
- How Does The C++ New Operator Works?
- What Happens When Enough Memory In The Program Is Not Available?
- Initializing Objects Allocated With New Operator In C++
- Lifetime Of Objects Allocated With The New Operator In C++
- What Is The Delete Operator In C++?
- Difference Between New And Delete Operator In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Types Of Overloading In C++
- What Is Operator Overloading In C++?
- How To Overload An Operator In C++?
- Overloadable & Non-overloadable Operators In C++
- Unary Operator Overloading In C++
- Binary Operator Overloading In C++
- Special Operator Overloading In C++
- Rules For Operator Overloading In C++
- Advantages And Disadvantages Of Operator Overloading In C++
- Function Overloading In C++
- What Is the Difference Between Operator Functions and Normal Functions?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Operators In C++?
- Introduction To Logical Operators In C++
- Types Of Logical Operators In C++ With Example Program
- Logical AND (&&) Operator In C++
- Logical NOT(!) Operator In C++
- Logical Operator Precedence And Associativity In C++
- Relation Between Conditional Statements And Logical Operators In C++
- C++ Relational Operators
- Conclusion
- Frequently Asked Important Interview Questions:
- Test Your Skills: Quiz Time
Table of content:
- Different Type Of C++ Bitwise Operators
- C++ Bitwise AND Operator
- C++ Bitwise OR Operator
- C++ Bitwise XOR Operator
- Bitwise Left Shift Operator In C++
- Bitwise Right Shift Operator In C++
- Bitwise NOT Operator
- What Is The Meaning Of Set Bit In C++?
- What Does Clear Bit Mean?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Types of Comments in C++
- Single Line Comment In C++
- Multi-Line Comment In C++
- How Do Compilers Process Comments In C++?
- C- Style Comments In C++
- How To Use Comment In C++ For Debugging Purposes?
- When To Use Comments While Writing Codes?
- Why Do We Use Comments In Codes?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Storage Classes In Cpp?
- What Is The Scope Of Variables?
- What Are Lifetime And Visibility Of Variables In C++?
- Types of Storage Classes in C++
- Automatic Storage Class In C++
- Register Storage Class In C++
- Static Storage Class In C++
- External Storage Class In C++
- Mutable Storage Class In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Decision Making Statements In C++
- Types Of Conditional Statements In C++
- If-Else Statement In C++
- If-Else-If Ladder Statement In C++
- Nested If Statements In C++
- Alternatives To Conditional If-Else In C++
- Switch Case Statement In C++
- Jump Statements & If-Else In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A Switch Statement/ Switch Case In C++?
- Rules Of Switch Case In C++
- How Does Switch Case In C++ Work?
- The break Keyword In Switch Case C++
- The default Keyword In C++ Switch Case
- Switch Case Without Break And Default
- Advantages & Disadvantages of C++ Switch Case
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A For Loop In C++?
- Syntax Of For Loop In C++
- How Does A For Loop In C++ Work?
- Examples Of For Loop Program In C++
- Ranged Based For Loop In C++
- Nested For Loop In C++
- Infinite For Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A While Loop In C++?
- Parts Of The While Loop In C++
- C++ While Loop Program Example
- How Does A While Loop In C++ Work?
- What Is Pre-checking Process Or Entry-controlled Loop?
- When Are While Loops In C++ Useful?
- Example C++ While Loop Program
- What Are Nested While Loops In C++?
- Infinite While Loop In C++
- Alternatives To While Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Loops & Its Types In C++?
- What Is A Do-While Loop In C++?
- Do-While Loop Example In C++ To Print Numbers
- How Does A Do-While Loop In C++ Work?
- Various Components Of The Do-While Loop In C++
- Example 2: Adding User-Input Positive Numbers With Do-While Loop
- C++ Nested Do-While Loop
- C++ Infinitive Do-while Loop
- What is the Difference Between While Loop and Do While Loop in C++?
- When To Use A Do-While Loop?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are 2D Vectors In C++?
- How To Declare 2D Vector In C++?
- How To Initialize 2D Vector In C++?
- C++ Program Examples For 2D Vectors
- How To Access & Modify 2D Vector Elements In C++?
- Methods To Traverse, Manipulate & Print 2D Vectors In C++
- Adding Elements To 2-D Vector Using push_back() Function
- Removing Elements From Vector In C++ Using pop_back() Function
- Creating 2D Vector In C++ With User Input For Size Of Column & Row
- Advantages of 2D Vectors Over Traditional Arrays
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- How To Print A Vector In C++ By Overloading Left Shift (<<) Operator?
- How To Print Vector In C++ Using Range-Based For-Loop?
- Print Vector In C++ With Comma Separator
- Printing Vector In C++ Using Indices (Square Brackets/ Double Brackets & at() Function)
- How To Print A Vector In C++ Using std::copy?
- How To Print A Vector In C++ Using for_each() Function?
- Printing C++ Vector Using The Lambda Function
- How To Print Vector In C++ Using Iterators?
- Conclusion
- Frequently Asked Questions
Table of content:
- Definition Of C++ Find In Vector
- Using The std::find() Function
- How Does find() In Vector C++ Function Work?
- Finding An Element By Custom Comparator Using std::find_if() Function
- Use std::find_if() With std::distance()
- Element Find In Vector C++ Using For Loop
- Using The find_if_not Function
- Find Elements With The Linear Search Approach
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Sort() Function In C++?
- Sort() Function In C++ From Standard Template Library
- Exceptions Of Sort() Function/ Algorithm In C++
- The Stable Sort() Function In C++
- Partial Sort() Function In C++
- Sorting In Ascending Order With Sort() Function In C++
- Sorting In Descending Order With Sort Function In C++
- Sorting In Desired Order With Custom Comparator Function & Sort Function In C++
- Sorting Elements In Desired Order Using Lambda Expression & Sort Function In C++
- Types of Sorting Algorithms In C++
- Advanced Sorting Algorithms In C++
- How Does the Sort() Function Algorithm Work In C++?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Function Overloading In C++?
- Ways Of Function Overloading In C++
- Function Overloading In C++ Using Different Types Of Parameters
- Function Overloading In C++ With Different Number Of Parameters
- Function Overloading In C++ Using Different Sequence Of Parameters
- How Does Function Overloading In C++ Work?
- Rules Of Function Overloading In C++
- Why Is Function Overloading Used?
- Types Of Function Overloading Based On Time Of Resolution
- Causes Of Function Overloading In C++
- Ambiguity & Function Overloading In C++
- Advantages Of Function Overloading In C++
- Disadvantages Of Function Overloading In C++
- Operator Overloading In C++
- Function Overriding In C++
- Difference Between Function Overriding & Function Overloading In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is An Inline Function In C++?
- How To Define The Inline Function In C++?
- How Does Inline Function In C++ Work?
- The Need For An Inline Function In C++
- Can The Compiler Ignore/ Reject Inline Function In C++ Programs?
- Normal Function Vs. Inline Function In C++
- Classes & Inline Function In C++
- Understanding Inline, __inline, And __forceinline Functions In C++
- When To Use An Inline Function In C++?
- Advantages Of Inline Function In C++
- Disadvantages Of Inline Function In C++
- Why Not Use Macros Instead Of An Inline Function In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Static Data Member In C++?
- How To Declare Static Data Members In C++?
- How To Initialize/ Define Static Data Member In C++?
- Ways To Access A Static Data Member In C++
- What Are Static Member Functions In C++?
- Example Of Member Function & Static Data Member In C++
- Practical Applications Of Static Data Member In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constant In C++?
- Ways To Define Constant In C++
- What Are Literals In C++?
- Pointer To A Constant In C++
- Constant Function Arguments In C++
- Constant Member Function Of Class In C++
- Constant Data Members In C++
- Object Constant In C++
- Conclusion
- Frequently Asked Questions(FAQ)
Table of content:
- What Is Friend Function In C++?
- Declaration Of Friend Function In C++ With Example
- Characteristics Of Friend Function In C++
- Global Friend Function In C++ (Global Function As Friend Function )
- Member Function Of Another Class As Friend Function In C++
- Function Overloading Using Friend Function In C++
- Advantages & Disadvantages Of Friend Function in C++
- What Is A C++ Friend Class?
- A Function Friendly To Multiple Classes
- C++ Friend Class Vs. Friend Function In C++
- Some Important Points About Friend Functions And Classes In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Function Overriding In C++?
- The Working Mechanism Of Function Overriding In C++
- Real-Life Example Of Function Overriding In C++
- Accessing Overriding Function In C++
- Accessing Overridden Function In C++
- Function Call Binding With Class Objects | Function Overriding In C++
- Function Call Binding With Base Class Pointers | Function Overriding In C++
- Advantages Of Function Overriding In C++
- Variations In Function Overriding In C++
- Function Overloading In C++
- Function Overloading Vs Function Overriding In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Errors In C++
- What Is Exception Handling In C++?
- Exception Handling In C++ Program Example
- C++ Exception Handling: Basic Keywords
- The Need For C++ Exception Handling
- C++ Standard Exceptions
- C++ Exception Classes
- User-Defined Exceptions In C++
- Advantages & Disadvantages Of C++ Exception Handling
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Templates In C++ & How Do They Work?
- Types Of Templates In C++
- What Are Function Templates In C++?
- C++ Template Functions With Multiple Parameters
- C++ Template Function Overloading
- What Are Class Templates In C++?
- Defining A Class Member Outside C++ Template Class
- C++ Template Class With Multiple Parameters
- What Is C++ Template Specialization?
- How To Specify Default Arguments For Templates In C++?
- Advantages Of C++ Templates
- Disadvantages Of C++ Templates
- Difference Between Function Overloading And Templates In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Structure
- Structure Declaration
- Initialization of Structure
- Copying and Comparing Structures
- Array of Structures
- Nested Structures
- Pointer to a Structure
- Structure as Function Argument
- Self Referential Structures
- Class
- Object Declaration
- Accessing Class Members
- Similarities between Structure and Class
- Which One Should You Choose?
- Key Difference Between a Structure and Class
- Summing Up
- Test Your Skills: Quiz Time
Table of content:
- What Is A Class And Object In C++?
- What Is An Object In C++?
- How To Create A Class & Object In C++? With Example
- Access Modifiers & Class/ Object In C++
- Member Functions Of A Class In C++
- How To Access Data Members And Member Functions?
- Significance Of Class & Object In C++
- What Are Constructors In C++ & Its Types?
- What Is A Destructor Of Class In C++?
- An Array Of Objects In C++
- Object In C++ As Function Arguments
- The this (->) Pointer & Classes In C++
- The Need For Semicolons At The End Of A Class In C++
- Difference Between Structure & Class In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Static Members In C++?
- Static Member Functions in C++
- Ways To Call Static Member Function In C++
- Properties Of Static Member Function In C++
- Need Of Static Member Functions In C++
- Regular Member Function Vs. Static Member Function In C++
- Limitations Of Static Member Functions In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Constructor In C++?
- Characteristics Of A Constructor In C++
- Types Of Constructors In C++
- Default Constructor In C++
- Parameterized Constructor In C++
- Copy Constructor In C++
- Dynamic Constructor In C++
- Benefits Of Using Constructor In C++
- How Does Constructor In C++ Differ From Normal Member Function?
- Constructor Overloading In C++
- Constructor For Array Of Objects In C++
- Constructor In C++ With Default Arguments
- Initializer List For Constructor In C++
- Dynamic Initialization Using Constructor In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constructor In C++?
- What Is Constructor Overloading In C++?
- Dеclaration Of Constructor Ovеrloading In C++
- Condition For Constructor Overloading In C++
- How Constructor Ovеrloading In C++ Works?
- Examples Of Constructor Overloading In C++
- Lеgal & Illеgal Constructor Ovеrloading In C++
- Types Of Constructors In C++
- Characteristics Of Constructors In C++
- Advantage Of Constructor Overloading In C++
- Disadvantage Of Constructor Overloading In C++
- Conclusion
- Frеquеntly Askеd Quеstions
Table of content:
- What Is A Destructor In C++?
- Rules For Defining A Destructor In C++
- When Is A Destructor in C++ Called?
- Order Of Destruction In C++
- Default Destructor & User-Defined Destructor In C++
- Virtual Destructor In C++
- Pure Virtual Destructor In C++
- Key Properties Of Destructor In C++ You Must Know
- Explicit Destructor Calls In C++
- Destructor Overloading In C++
- Difference Between Normal Member Function & Destructor In C++
- Important Uses Of Destructor In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constructor In C++?
- What Is A Destructor In C++?
- Difference Between Constructor And Destructor In C++
- Constructor In C++ | A Brief Explanation
- Destructor In C++ | A Brief Explanation
- Difference Between Constructor And Destructor In C++ Explained
- Order Of Calling Constructor And Destructor In C++ Classes
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Type Conversion In C++?
- What Is Type Casting In C++?
- Types Of Type Conversion In C++
- Implicit Type Conversion (Coercion) In C++
- Explicit Type Conversion (Casting) In C++
- Advantages Of Type Conversion In C++
- Disadvantages Of Type Conversion In C++
- Difference Between Type Casting & Type Conversion In C++
- Application Of Type Casting In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Copy Constructor In C++?
- Characteristics Of Copy Constructors In C++
- Types Of Copy Constructors In C++
- When Do We Call The Copy Constructor In C++?
- When Is A User-Defined Copy Constructor Needed In C++?
- Types Of Constructor Copies In C++
- Can We Make The Copy Constructor In C++ Private?
- Assignment Operator Vs Copy Constructor In C++
- Example Of Class Where A Copy Constructor Is Essential
- Uses Of Copy Constructors In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do You Need Object-Oriented Programming (OOP) In C++?
- OOPs Concepts In C++ With Examples
- The Class OOPs Concept In C++
- The Object OOPs Concept In C++
- The Inheritance OOPs Concept In C++
- Polymorphism OOPs Concept In C++
- Abstraction OOPs Concept In C++
- Encapsulation OOPs Concept In C++
- Other Features Of OOPs In C++
- Benefits Of OOP In C++ Over Procedural-Oriented Programming
- Disadvantages Of OOPS Concept In C++
- Why Is C++ A Partial OOP Language?
- Conclusion
- Frequently Asked Questions
Table of content:
- Introduction To Abstraction In C++
- Types Of Abstraction In C++
- What Is Data Abstraction In C++?
- Understanding Data Abstraction In C++ Using Real Life Example
- Ways Of Achieving Data Abstraction In C++
- What Is An Abstract Class?
- Advantages Of Data Abstraction In C++
- Use Cases Of Data Abstraction In C++
- Encapsulation Vs. Abstraction In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Encapsulation In C++?
- How Does Encapsulation Work In C++?
- Types Of Encapsulation In C++
- Why Do We Need Encapsulation In C++?
- Implementation Of Encapsulation In C++
- Access Specifiers & Encapsulation In C++
- Role Of Access Specifiers In Encapsulation In C++
- Member Functions & Encapsulation In C++
- Data Hiding & Encapsulation In C++
- Features Of Encapsulation In C++
- Advantages & Disadvantages Of Encapsulation In C++
- Difference Between Abstraction and Encapsulation In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Inheritance In C++?
- What Are Child And Parent Classes?
- Syntax And Structure Of Inheritance In C++
- Implementing Inheritance In C++
- Importance Of Inheritance In C++
- Types Of Inheritance In C++
- Visibility Modes Of Inheritance In C++
- Access Modifiers & Inheritance In C++
- How To Make A Private Member Inheritable?
- Member Function Overriding In Inheritance In C++
- The Diamond Problem | Inheritance In C++ & Ambiguity
- Ways To Avoid Ambiguity Inheritance In C++
- Why & When To Use Inheritance In C++?
- Advantages Of Inheritance In C++
- The Disadvantages Of Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Hybrid Inheritance In C++?
- Importance Of Hybrid Inheritance In Object Oriented Programming
- Example Of Hybrid Inheritance In C++: Using Single and Multiple Inheritance
- Example Of Hybrid Inheritance In C++: Using Multilevel and Hierarchical Inheritance
- Real-World Applications Of Hybrid Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Multiple Inheritance In C++?
- Examples Of Multiple Inheritance In C++
- Ambiguity Problem In Multiple Inheritance In C++
- Ambiguity Resolution In Multiple Inheritance In C++
- The Diamond Problem In Multiple Inheritance In C++
- Visibility Modes In Multiple Inheritance In C++
- Advantages & Disadvantages Of Multiple Inheritance In C++
- Multiple Inheritance Vs. Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Multilevel Inheritance In C++?
- Block Diagram For Multilevel Inheritance In C++
- Multilevel Inheritance In C++ Example
- Constructor & Multilevel Inheritance In C++
- Use Cases Of Multilevel Inheritance In C++
- Multiple Vs Multilevel Inheritance In C++
- Advantages & Disadvantages Of Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Hierarchical Inheritance In C++?
- Example 1: Hierarchical Inheritance In C++
- Example 2: Hierarchical Inheritance In C++
- Impact of Visibility Modes In Hierarchical Inheritance In C++
- Advantages And Disadvantages Of Hierarchical Inheritance In C++
- Use Cases Of Hierarchical Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Access Specifiers In C++?
- Types Of Access Specifiers In C++
- Public Access Specifiers In C++
- Private Access Specifier In C++
- Protected Access Specifier In C++
- The Need For Access Specifiers In C++
- Combined Example For All Access Specifiers In C++
- Best Practices For Using Access Specifiers In C++
- Why Can't Private Members Be Accessed From Outside A Class?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Diamond Problem In C++?
- Example Of The Diamond Problem In C++
- Resolution Of The Diamond Problem In C++
- Virtual Inheritance To Resolve Diamond Problem In C++
- Scope Resolution Operator To Resolve Diamond Problem In C++
- Conclusion
- Frequently Asked Questions
C++ If-Else Statement | Syntax, Types & More (+Code Examples)
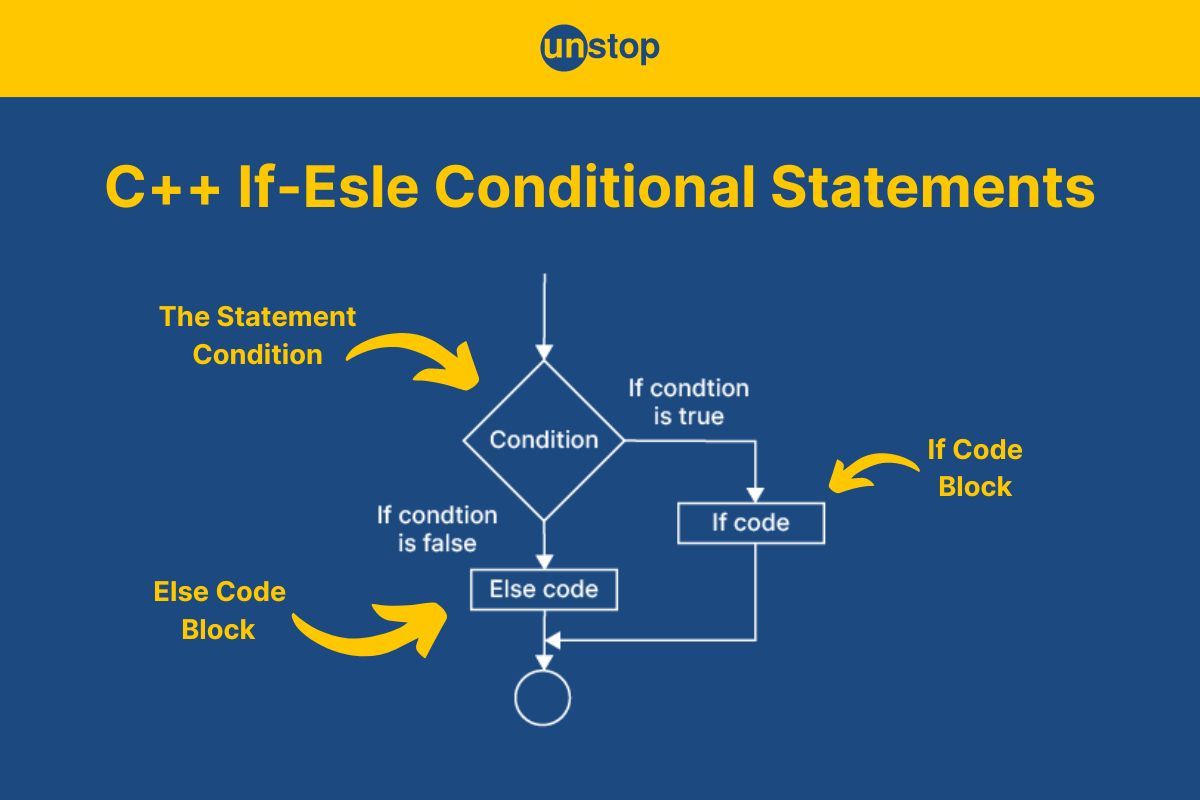
When incorporating logic into our code, we often need to make decisions based on certain conditions being fulfilled. The results of these logical conditions determine which particular block of code will be executed to get the desired result. We use specific statements for this decision-making process that ultimately control program flow. They are referred to as if-else conditional statements or if-else C++ for short.
In this article, we will examine the different types of decision-making statements in C++ programming. These include simple if statements, if-else statements, nested if, if else, if ladder, and jump statements. So, let’s get started exploring the if-else statement in C++.
Decision Making Statements In C++
In C++ programming language, we have to make decisions on program flow (regarding, say, what to do next) based on the conditions in our problem statement. These decisions help determine the flow and even change the sequential order of execution of a program.
Often, we need to pit possibilities against each other and provide probable control flow options when either of the possibilities comes true. This is where the decision-making and, hence, the conditional/ decision-driven statements come into play in programming.
Take, for example, a simple scenario where a program prints the results for students.
- The students whose marks are 40 or more pass, and those below fail.
- So if the condition of marks greater than or equal to 40 is met, the program will print Pass. Otherwise, it will print fail.
This shows how a simple decision affects the further course of action of a C++ program. In general, using decision-making statements in codes helps the compiler decide the direction or flow of program execution. Let's examine the general syntax followed by most of these if-else C++ statements.
Syntax Of If-Else C++:
if (condition){
// Code to be executed if the condition yields true
}else {
// Code to be executed if the condition yields false
}
Here,
- The if and else keywords mark the two possibilities in our decision-making structure.
- The condition inside braces refers to the conditional logic/ target statement whose outcome will determine which block of code is implemented.
- The curly brackets {} contain the blocks of code to be executed depending upon the result of the logical condition.
Note: The simple if statement does not have the else keyword. We will discuss this in the sections ahead.
The above flowchart gives a general overview of how the decision-making and execution process works when if/ if-else C++ conditional statements are applied.
Types Of Conditional Statements In C++
In C++, several variations and types of if statements can be used to handle different scenarios and conditions. The decision-making statements available in C++ are:
- If statement
- If-else statement
- Nested if statement
- If-else-if ladder
- Condition Statement
- Switch case
- Jump statements (break, continue, goto, return)
We will discuss each of these types of loop control statements in detail, with the help of code examples, in the section ahead.
If Statement In C++
In C++, the if statement is the simplest form of decision-making statement. It is used to determine whether a block of statements will be executed based on a conditional statement. The condition has only two boolean values/results, i.e., either true or false.
- If the condition in the if statement is true, the code block inside the curly brackets is executed.
- In case the condition evaluates to false, we move to the line of code after the if-block.
- If there are no curly braces {} after the if statement, only the first statement inside the if block is considered.
Syntax-
if (condition){
// conditional code body
}
Here,
- The if keyword marks the beginning/ initiation of the if statement.
- The (condition) refers to the condition we are verifying.
- The curly braces {} contain the conditional code that will be executed if the condition is fulfilled.
How Do If Statements In C++ Work?
The general working mechanism behind the if statement is mentioned below and is also represented in the flow diagram.
A simple if statement in C++ is used to check whether a condition is true or false. Then-
- If the condition is true, the statements inside the if block are executed.
- In case the condition is false, the control goes to the first statement after the if block, and the normal execution of the program continues.
For example, let's say the condition we check in the if statement is a>b for variables a and b.
- If the expression (a>b) is true, then the statements/ code block inside the curly bracket is executed. After the execution of the code block is done, the control is transferred to the statements outside the body of the if statement.
- On the other hand, if the expression (a>b) is false, then the statements/ code inside the curly brackets are ignored. The control is directly passed to the statements outside the body of the if statement.
Now, let's look at a code example that showcases the implementation of an if statement in C++.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewppbnQgbWFya3MgPSA2MDsKCmlmIChtYXJrcyA+IDQwKSB7CmNvdXQgPDwgIlBhc3NlZCI7Cn0KCmNvdXQgPDwgIlxuSGFwcHkiOwoKcmV0dXJuIDA7Cn0=
Output:
Passed
Happy
Explanation:
In the simple C++ program, we begin by including the essential header file <iostream> and the namespace std.
- Inside the main() function, which is the entry point of program execution, we declare an integer data type variable marks and assign it a value of 60.
- Then, we define an if statement to find whether a student has passed or not. The condition uses the greater than relational operator to determine if the marks are greater than 40.
- If the condition is true, the cout statements inside the code block are executed and the statement is printed to the console.
- If the condition is false, the code block inside the if-statement will be skipped.
- After that, we move to the cout statement outside of the statement. The newline escape sequence in the second count statement shifts the cursor to the next line and prints the string- Happy.
- This clearly shows that if the condition is fulfilled, which it is in this example, then the conditions code is executed.
- Finally, the program terminates with a return 0 statement indicating successful execution to the operating system.
Here, the if condition is true; hence, the output has both strings, i.e., Passed and Happy. Had the condition not been met, the output would have been the single string Happy. Try to run this C++ program with an integer value of less than 40.
If-Else Statement In C++
The if-else in C++ is the most commonly used decision-making statement. It is used to decide which block of statements will be executed based on the result of the conditional statement. Here also, the condition has only two boolean values, i.e., either true or false.
If the condition is true, the statements inside the if block are executed, and if the state is false, the statements inside the else block are executed.
Syntax For If-Else C++:
if (condition){
// Executed if the condition is true
}else{
// Executed if the condition is false
}
The syntax is the same as the one given at the beginning of the article.
How Does If-Else In C++ Work?
The working mechanism of the if-else condition in C++ can be seen in the flowchart shared at the beginning of the article. In the if-else statement, we have two code blocks, either of which is implemented depending on whether the condition is true or false.
- We begin by checking whether the condition is true or false.
- If the condition is true, the code block following the if statement is executed. Once the execution of this block is done, the code block following else is ignored, and we move to the next line.
- If the condition is false, the else-block code is executed, and the if block is ignored. The flow of control then moves to the line after the else code block.
If-else in C++ is also referred to as the control flow statement since it controls the flow of a program and is the most commonly used statement. Let’s take the example where we are testing the condition (a>b).
- Here if a>b holds true, then the code after the if statement is executed. The code in the else-block is ignored. After this, the program moves to the next line.
- Contrarily, if a>b is false, then the code in the if-block is ignored, and the else-block is executed. After that, the program execution moves to the next line after that.
Below is a code implementation showcasing the if-else statement in C++.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewppbnQgbWFya3MgPSAyODsKCi8vIEluaXRpYXRpbmcgaWYtZWxzZSBzdGF0ZW1lbnQKaWYgKG1hcmtzID4gNDApIHsKY291dCA8PCAiU3R1ZGVudCBoYXMgcGFzc2VkIjsKfSBlbHNlIHsKY291dCA8PCAiU3R1ZGVudCBoYXMgZmFpbGVkIjsKfQoKcmV0dXJuIDA7Cn0=
Output:
Student has failed
Explanation:
In the sample C++ program-
- We first declare an integer variable marks inside the main() function and initialize it with the value of 28.
- As mentioned in code comments, we then employ an if-else statement to check if the student has passed or failed.
- We check the condition to see if the value of marks variable is greater than 40. If the condition marks>40 is true, then we use the cout statement to print Student has passed to the console.
- If the condition marks<40 is false, then we use the cout statement to print Student has failed.
- Since here, marks=28<40, the cout command after the else statement is executed, and we get the output- Student has failed.
If-Else-If Ladder Statement In C++
The if-else-if ladder is similar to the switch statement, where we get multiple options, and one among them is selected. As soon as the condition matches, the statement inside that respective block is executed, and the rest of the block is skipped. This continues until none of the statements matches, and the control is transferred to the last/ default else-block.
Syntax:
if (boolean expression 1){
// Execute this if expression1 is true
}
else if (boolean expression 2){
//Execute this if expression2 is true
}else{
//Execute this if none of the above expressions are true
}
How Does If-Else-If Ladder Statement In C++ Work?
The working of the ladder statement is depicted in the flow diagram below and explained ahead.
The if-else-if ladder in C++ is used to execute a code block/ statement conditional upon certain expressions being met. Note that only one code block gets executed according to the matching condition.
Like other flow control methods/ statements, the ladder statement begins by checking whether a condition/ expression has been met.
- We check if the boolean expression 1 in the first if-block is true. If it is true, then the code inside the if-block is executed, and all the else-blocks are ignored.
- If boolean expression 1 is false, the control flow goes to the else-if condition (here, boolean expression 2).
- If expression 2 is true, then the code inside the respective else-if-block is executed, and all other else-blocks are ignored.
- If both expressions 1 and 2 are false, the control goes to else-block 2, and the program verifies condition/ boolean expression 3. If it is true, the code inside else-block two is executed, and all other else-blocks are ignored. If it is false, the control goes to the next else condition/ expression.
- This is continued until none of the conditions/ expressions match. The control transfers to the last else-block, and the program's normal execution continues.
To better understand the concept, look at the code example below, which implements the if-else-if ladder statement in C++.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewppbnQgbWFya3MgPSA4MDsKCmlmIChtYXJrcyA+PSA5MCkgewpjb3V0IDw8ICJHcmFkZSBBIjsKfSBlbHNlIGlmIChtYXJrcyA+PSA4MCkgewpjb3V0IDw8ICJHcmFkZSBCIjsKfSBlbHNlIGlmIChtYXJrcyA+PSA2MCkgewpjb3V0IDw8ICJHcmFkZSBDIjsKfSBlbHNlIGlmIChtYXJrcyA+PSA0MCkgewpjb3V0IDw8ICJHcmFkZSBEIjsKfSBlbHNlIHsKY291dCA8PCAiR3JhZGUgRiI7Cn0KCnJldHVybiAwOwp9
Output:
Grade B
Explanation:
The C++ code above illustrates the functioning of an if-else-if ladder statement. The program takes marks of a student as input and prints the grade based on various conditions.
- We begin by initializing an integer variable marks with the value 80 inside the main() function.
- Then, we create an if-else-if ladder statement where the first condition/ expression checks if marks exceed 90. If the condition marks>90 is true, the cout statement in corresponding if-block prints- Grade A.
- If marks<90 is false, the execution moves to the else-block to check expression/ condition 2, i.e., marks>80. If this condition is true, the cout statement in corresponding else-block prints- Grade B.
- If condition marks<80 is false, then the program proceeds to check condition 3, and so on.
- Since condition 1 is false here, but condition 2 is met, the output displayed is Grade B.
Nested If Statements In C++
Nested if statements in C++ refer to a conditional statement where one if-statement is placed inside another if-statement. This is used when we want to add one condition inside another condition. We can have any number of nested if statements in C++.
Syntax Of Nested If-Statement In C++:
if (condition1){
// Executes this if condition1 is true
if (condition2){
// Executes this if condition2 is true
}
}
We can similarly construct a nested if-else statement in C++. Its syntax is discussed below.
Syntax Of Nested If-Else In C++:
if (condition1) {
// Executes this if condition1 is true
if (condition2) {
// Executes this if condition2 is true
} else {
// Executes this if condition2 is false
}
} else {
// Executes this if condition1 is false
}
How Do Nested If Statements Work In C++?
The nested-if statement in C++ simplifies the decision-making process in complex situations where multiple conditions must be met simultaneously. Here is how these work:
- At first, the condition (i.e., condition 1) in the first if-block is checked. If condition 1 is true, the program enters the if-block.
- Inside this if block, there is another if-block, and the second condition (i.e., condition 2) is checked.
- If condition 2 is true, the code inside the nested if-block is executed.
- If both the if-conditions are false, the program flow moves outside the statement, and the next line of code is executed as normal.
- It can be concluded that for nested-if statements in C++, only a particular block is executed based on a condition.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewppbnQgbjEgPSAxMjsKaW50IG4yID0gMTY7CmludCBuMyA9IDIwOwoKaWYgKG4xID4gbjIpIHsKaWYgKG4xID4gbjMpIHsKY291dCA8PCAibjEgaXMgdGhlIGxhcmdlc3QgbnVtYmVyIjsKfSBlbHNlIHsKY291dCA8PCAibjMgaXMgdGhlIGxhcmdlc3QgbnVtYmVyIjsKfQp9IGVsc2UgewppZiAobjIgPiBuMykgewpjb3V0IDw8ICJuMiBpcyB0aGUgbGFyZ2VzdCBudW1iZXIiOwp9IGVsc2Ugewpjb3V0IDw8ICJuMyBpcyB0aGUgbGFyZ2VzdCBudW1iZXIiOwp9Cn0KCnJldHVybiAwOwp9
Output:
n3 is the largest number
Explanation:
In the example above-
- We declare and initialize three integer variables, n1, n2, and n3, with the values 12, 16, and 20, respectively.
- Then, we create a nested if-else statement to find the largest number among three numbers.
- The nested statement starts with condition 1, which is n1>n2.
- If condition 1 is true, we move to the if statement nested inside the first if-else statement. Here, condition 2 is n1>n3, which checks if n1 is greater than n3.
- If both if-conditions are true, then the cout statement in the second if-block is executed. It prints the string message- n1 is the largest number.
- If condition 1 is true, but condition 2 is false, then the cout statement inside the nested else block is executed to print n3 is the largest number.
- If condition 1 is false, we move to the corresponding else block, which contains another if-else statement.
- Inside this, the program verifies condition 3, i.e. n2>n3. If condition 3 is true, the cout statement in the corresponding if-block is executed, and it prints- n2 as the largest number.
- If both conditions 1 and 3 are false, we move to the else-block after condition 3. The cout statement then prints n3 is the largest number.
- Since, in our example, none of the conditions is true, the last else-block is executed, and the corresponding message is printed.
Alternatives To Conditional If-Else In C++
While the if-statements are some of the most commonly used decision-making/ condition statements, there are some alternatives that can be used. These include the switch case and jump statements. Let's discuss these alternatives and see how they are implemented.
Switch Case Statement In C++
In C++, the switch case statement provides a convenient way to execute different blocks of code based on the value of a single expression. It is similar to the if-else-if ladder, where we have multiple choices, and a block of statements is executed based on which choice we make. It's particularly useful when dealing with multiple possible values for a variable and simplifies the code compared to using multiple nested if-else in C++.
Syntax:
switch(expression){
case 1: //statement;
break;
case 2: //statement;
break;
......
default: //statement if none of the case match;
break;
}
How Does Switch Case Work?
The switch case in C++ evaluates an expression provided within parentheses after the switch keyword.
- It compares the value of the expression sequentially with constant values specified in each case label within the switch block.
- If a match is found, the corresponding block of code following that case label is executed.
- After executing the code within a matching case block, the program encounters a break statement, which exits the switch block, skipping any subsequent case blocks.
- If none of the case values match the expression value and a default case is provided, the code within the default case block is executed.
- Once the code within a case block (or default block) is executed, the control exits the switch statement and continues with the code following the switch block.
- The default case is optional; if omitted and no case labels match the expression value, the switch statement does nothing, and control continues with the code following the switch block.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewppbnQgeCA9IDI7Cgpzd2l0Y2ggKHgpIHsKY2FzZSAxOgpjb3V0IDw8ICJPcHRpb24gMSI7CmJyZWFrOwoKY2FzZSAyOgpjb3V0IDw8ICJPcHRpb24gMiI7CmJyZWFrOwoKZGVmYXVsdDoKY291dCA8PCAiTm8gb3B0aW9ucyI7CmJyZWFrOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Option 2
Explanation:
In the above code snippet, we take an integer variable x as input whose value is 2. Using the switch case statement, the value of x is matched with the expression of the switch case. Since here, case 2 matches with the value 2, the statement Option 2 gets printed as output.
Jump Statements & If-Else In C++
Jump statements in C++ are used to change the conditional flow of a program. There are four types of jump statements in C++, namely, break, continue, goto, and return. Each of these is explained ahead.
Break Statements In C++
The break statement is used primarily inside loops (like for loop, while loop, or do-while loop) and switch statements. When encountered, it causes immediate termination of the loop or switch block in which it appears. Control then passes to the statement following the terminated loop or switch block.
Syntax:
break ;
Working Of Break Statement In C++:
The break statement is primarily used to exit from loops, switch-case and even if-else in C++. Its working mechanism is as follows:
- The break statement is placed within the body of a loop or any other control statement.
- When encountered, the break statement immediately terminates the execution of the loop or statement that encloses it.
- Control then passes to the line of code following the terminated loop or control statement, effectively ending further iterations of the loop or control statement.
- It's important to note that the break statement does not affect the conditions of the loop or control statement itself; it simply provides a means to exit prematurely based on certain conditions.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCnZvaWQgZmluZE51bWJlcihpbnQgYVtdLCBpbnQgbiwgaW50IGtleSkgewpmb3IgKGludCBpID0gMDsgaSA8IG47IGkrKykgewppZiAoYVtpXSA9PSBrZXkpIHsKY291dCA8PCAiTnVtYmVyIGZvdW5kIGluIGFuIGFycmF5IjsKYnJlYWs7Cn0KfQp9CgppbnQgbWFpbigpIHsKaW50IGFbXSA9IHs1LCA0LCAzLCAyLCAxfTsKaW50IG4gPSA1OwppbnQga2V5ID0gNDsKCmZpbmROdW1iZXIoYSwgbiwga2V5KTsKCnJldHVybiAwOwp9
Output:
Number found in an array
Explanation:
In the above code snippet, we take an array as input and check if a number is present in the array or not. As soon as the number is found, we terminate the loop using the break statement.
Continue Statement In C++
The continue statement is also used within loops. When encountered, it causes the current iteration of the loop to end prematurely, and the control jumps to the next iteration, bypassing any remaining code within the loop block for the current iteration.
Syntax:
continue;
Working of Continue Statement:
- The continue statement is placed within the body of a loop, such as a for loop, while loop, or do-while loop. This can be executed with the help of if-else in C++.
- When encountered, the continue statement immediately stops the current iteration of the loop.
- Control then jumps to the next iteration of the loop, bypassing any remaining code within the loop body for the current iteration.
- The loop's condition (if it's a while or a do-while loop) or the increment portion (if it's a for loop) is then evaluated, and the loop continues with the next iteration accordingly.
- It's important to note that the continue statement does not terminate the loop itself; instead, it simply skips the remaining code for the current iteration and proceeds with the next iteration.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewpmb3IgKGludCBpID0gMTsgaSA8PSA1OyBpKyspIHsKaWYgKGkgPT0gMykgewpjb250aW51ZTsKfSBlbHNlIHsKY291dCA8PCBpIDw8ICIgIjsKfQp9CgpyZXR1cm4gMDsKfQ==
Output:
1 2 4 5
Explanation:
In the above code snippet, we run a loop from 1 to 5. As the value of i becomes 3, we continue to the next iteration without printing the value 3. After that, the normal execution of the program takes place, and we get 1 2 4 5 as output.
Goto Statement In C++
The goto statement in C++ is also known as the unconditional jump statement, which is used to jump from one part of the function to the other. A target statement is specified with the help of a user-defined label. The control always goes to the statement after the specified label.
Syntax:
label:
.....
.....
goto label;
Working of Goto Statement
- The goto statement is followed by a label identifier, which marks a specific location in the code.
- When encountered, the goto statement transfers control to the labelled statement elsewhere in the code.
- The labelled statement is typically identified by a unique label followed by a colon (:), and it can be placed anywhere within the same function or code block as the goto statement.
- Upon reaching the labelled statement, execution continues from that point onwards, irrespective of the normal control flow.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCnZvaWQgcHJpbnQoKSB7CmludCBuID0gMTsKCmxhYmVsMToKY291dCA8PCBuOwpuKys7CgppZiAobiA8PSA1KSB7CmdvdG8gbGFiZWwxOwp9Cn0KCmludCBtYWluKCkgewpwcmludCgpOwpyZXR1cm4gMDsKfQ==
Output:
1 2 3 4 5
Explanation:
In the above code snippet, we make use of the goto statement to print numbers from 1-5. We take an integer variable n as 1 and print the value of n until the value is less than or equal to 5. In this case, we get 1 2 3 4 5 as output.
Return Statement In C++
The return statement is used within functions to exit the function and return a value to the caller. It can also be used to exit early from a function without returning a value in case of functions with a void return type. When encountered, it immediately terminates the function execution and returns control to the calling function along with the specified return value, if any.
Syntax:
return[expression];
Working of Return Statement:
- The return statement is followed by an optional expression, which represents the value to be returned to the caller.
- When encountered, the return statement immediately terminates the function execution and returns control to the calling function.
- If a return value is specified, it is evaluated, and control, along with the return value, is passed back to the calling function.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBkaWZmKGludCBhLCBpbnQgYikgewppbnQgZDEgPSBhIC0gYjsKcmV0dXJuIGQxOwp9CgppbnQgbWFpbigpIHsKaW50IG4xID0gMjA7CmludCBuMiA9IDEwOwoKaW50IGRpZmZlcmVuY2UgPSBkaWZmKG4xLCBuMik7Cgpjb3V0IDw8ICJEaWZmZXJlbmNlIGlzICIgPDwgZGlmZmVyZW5jZTsKCnJldHVybiAwOwp9
Output:
Difference is 10
Explanation:
In the above code snippet, we write two functions to find the difference between 2 numbers. The diff() function calculates the difference and returns it. The main() function uses this to find the difference, which is 10 in this case, and print it as the output.
Conclusion
The if-else statement is a fundamental tool in C++ programming for implementing decision-making logic. It allows developers to control the flow of their programs based on specific conditions. In this article, we discussed if-else in C++ and how they facilitate the decision-making process in programs. We have discussed the syntax and implementation of the various types of statements, i.e., nested if-else statements, simple if, if-else, and if-else-if ladder. We also know that there are alternatives to conditional statements, including switch and jump statements.
Understanding how to use if-else in C++ effectively is essential for writing clear, concise, and efficient code. Whether it's simple conditions or complex nested scenarios, mastering if-else statements is a key step towards becoming proficient in C++ programming.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. Can I use two if statements in C++?
Yes, you can use multiple if statements in C++. There is no restriction on the number of if statements you can use within a C++ program or within a function. Each if statement is evaluated independently based on its condition, and the corresponding code block is executed if the condition is true.
Here's an example of using multiple if statements in C++:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKaW50IG1haW4oKSB7CmludCB4ID0gMTA7CgppZiAoeCA+IDUpIHsKc3RkOjpjb3V0IDw8ICJ4IGlzIGdyZWF0ZXIgdGhhbiA1IiA8PCBzdGQ6OmVuZGw7Cn0KCmlmICh4IDwgMTUpIHsKc3RkOjpjb3V0IDw8ICJ4IGlzIGxlc3MgdGhhbiAxNSIgPDwgc3RkOjplbmRsOwp9CgpyZXR1cm4gMDsKfQ==
Output:
x is greater than 5
x is less than 15
Explanation:
In this example, there are two if statements, each with its own condition. Both conditions are evaluated independently, and if they are true, the corresponding message is printed to the console.
Q. What is the basic syntax of if-else C++ statements?
In C++, the if-else statement is the most used form of decision-making statement. It is used to decide which block of statement will be executed based on the conditional statement. The condition has boolean return values, i.e., true or false.
If the condition is true, the statements inside the if block are executed, and if the state is false, the statements inside the else block are executed.
Syntax:
if (condition){
// Executes this if the condition is true
statements;
}else{
// Executes this if the condition is false
statements;}
Q. Can I use multiple else if in C++?
Yes, we can do that using an if-else-if ladder. It is similar to the switch variable statement, where we get multiple options, and one among them is selected. As soon as the condition matches, the statement inside that block is executed, and the rest of the block is skipped. This continues until none of the single statements matches, and the control is transferred to the corresponding else block.
Q. What are the four types of if statements?
At times, there are situations where the program needs to make a decision, and based on that, the flow of the program is decided. In general, decision-making statements in programming help the compiler to decide the direction of the flow of program execution. The different types of if statements available in C++ are:
- Simple if statement
- Simple If-else statement
- Nested if statement
- If-else-if ladder statement
Q. How many conditions do we have in if-else statements?
In if-else statements, there is typically a single condition specified in the if-block.
- If the statement condition is true, then the code block associated with the if statement is executed.
- If the condition is false, the code block associated with the else statement (if present) is executed.
So, even though there is a single condition, there are two possible ways for control to flow. This way, the if-else C++ statements help in decision-making.
Test Your Skills: Quiz Time
You might also be interested in reading the following:
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Structure of C++ Programs Explained With Examples
- 2D Vector In C++ | Declare, Initialize & Operations (+ Examples)
- New Operator In C++ | Syntax, Usage, Working & More (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Divyansh Shrivastava 1 day ago