What Are Storage Classes In C++? A Detailed Guide With Examples
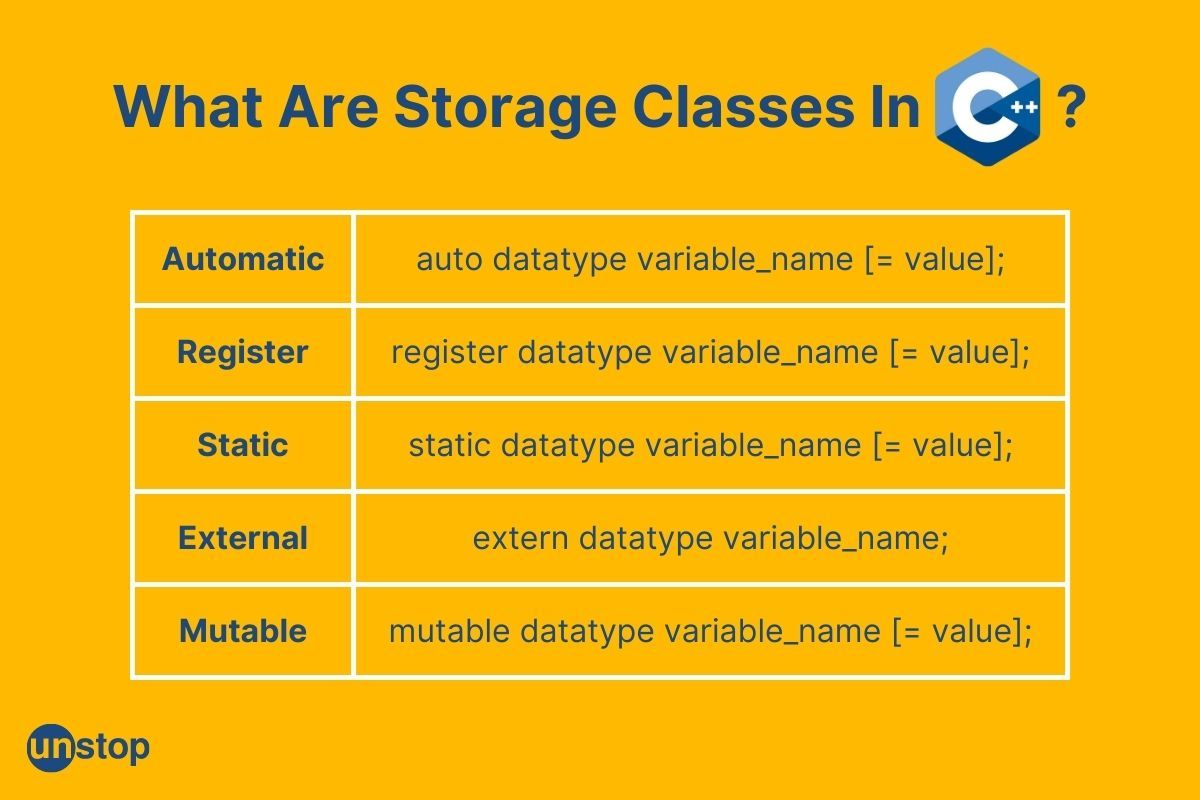
Table of content:
- What Are Storage Classes In Cpp?
- What Is The Scope Of Variables?
- What Are Lifetime And Visibility Of Variables In C++?
- Types of Storage Classes in C++
- Automatic Storage Class In C++
- Register Storage Class In C++
- Static Storage Class In C++
- External Storage Class In C++
- Mutable Storage Class In C++
- Conclusion
- Frequently Asked Questions
Storage classes tell us about the scope of variables as well as their lifespan and visibility. These functionalities help us to find the existence of a particular variable/function during the execution of a program. In C++, there are five storage classes, namely automatic, register, static, extern, and mutable.
In this article, we will look at storage classes, lifetime, visibility, and scope of variables, and different types of storage classes in C++ with their syntax and uses. We will see various examples along with the explanation to have a better understanding of the topic. So, let’s get started!
What Are Storage Classes In Cpp?
Storage classes in C++ programming language are used for variables or functions in a program to know about their scope, lifetime, visibility, and initial values. These functionalities help us to find the existence of a particular variable/function during the execution of a program.
The general syntax for defining C++ storage classes for a variable is as follows:
storage_class variable_data_type variable_name;
Here,
- storage_class: denotes the type of storage class used.
- variable_data_type: denotes the data type of a variable.
- variable_name: denotes the name of the variable.
Below are some examples of these storage classes:
auto int a = 5;
register char c = 'A';
static int count = 0;
As we have mentioned before, there are five types of storage classes in C++, and we will discuss each of these in the sections ahead.
What Is The Scope Of Variables?
The scope of a variable refers to a particular area in the program where the respective variable is declared, used, and can be modified. A variable can have different scopes ranging from a function to the entire program depending upon its type. The concept of storage classes is what defines the scope of a variable, and it also determines the lifetime and visibility of a variable.
Variables can be divided into various types depending on the storage class in C++. They are:
- Global Variables- They are declared at the beginning of the program before all other functions. Their scope is the entire program length and can be accessed from any function.
- Local variables- Their scope is limited to the function in which they are declared. It cannot be accessed outside that function or in any other part of the program.
- Static Variables- The scope of a static variable is the same as that of a local variable. Although the memory is allocated for the variable during the whole program until the program ends and memory deallocates.
- Register Variables- The scope of a register variable is only in the parent block in which it is declared. As soon as the function ends, the lifetime of the register variable in the current code block also comes to an end.
- Thread local variables- The scope of these variables depends on the creation of the thread. Whenever a thread is created, variables are also created, and similarly, the variable destroys as soon as the threads are destroyed.
What Are Lifetime And Visibility Of Variables In C++?
The lifetime of a variable in C++ refers to the time period during which the variable occupies some space in the memory and can be accessed in a function or anywhere else in the program. Some variables' lifetime is limited to a function, whereas others have a lifetime in the whole of the program.
The visibility of a variable in C++ refers to a region where a variable is accessible (or not). For example, a variable could be declared in a method, and hence it will be visible only in that variable and not anywhere else in the program. Although, a variable declared as global has its visibility in the entire program.
Types of Storage Classes in C++
In this section, we will have a look at the five major types of storage classes in C++ with the help of examples.
Automatic Storage Class In C++
The automatic storage class is the default storage class for all local variables. It is declared and can only be accessed within a particular function/method.
- In case there's no keyword specified to an automatic storage variable, it automatically gets assigned with the auto keyword.
- As per the C++11 standards, the auto keyword is used for the automatic deduction of datatype in C++.
- In this way, the compiler saves running time as it automatically predicts the data type of a variable. Even though the compilation time increases but the access time of the program does not get affected.
- The lifetime of an auto variable is limited to a function, and it cannot be accessed outside it. As soon as the function completes its execution, the automatic variables are destroyed because of its limited scope.
- We have to make use of pointer variables to access it faster outside a function in which it is not declared. The pointer points to that area in memory where the variables are stored.
- Its visibility is similar to that of a local variable. And the initial values of automatic storage classes are always garbage values.
Syntax:
auto datatype variable_name [= value];
Here,
- auto: is the storage class.
- datatype: denotes the data type of a variable.
- variable_name: denotes the name of the variable.
- value: initial garbage value.
Code Example:
Output:
Automatic Storage Class
Marks=495
gpa=9.5
Remark=You are Unstoppable
Explanation:
The above code snippets begins with the inclusion of iostream file and namespace std. Then,
- We define an automatic storage class with three different variables- marks, gpa, and remark as input. The data type for these variables is from the automatic storage class.
- The values assigned to the three variables are 495, 9.5, and You are Unstoppable, respectively.
- We then use the cout statement to print the values of three variables.
- In the main function, we again use the cout statement to print the phrase 'Automatic Storage Class' along with the new line specifier to move to the nest line in the output window.
Note: The program demonstrates how automatic storage classes work and the way in which they are declared inside a function. We can notice here that the scope of the variables is limited to that function, and we need to invoke the function to print its actual value.
Register Storage Class In C++
The register storage class is used for register variables when we want them to be stored in a free register instead of being stored in memory. Unlike the automatic storage class, these variables get stored in a free register, and in case no register is free at some moment, the variables get stored in the memory itself.
- We use the keyword register for storing variables under this storage class.
- As the variables are stored in a register, the operation is much faster as compared to the variables stored in memory as they utilize CPU registers.
- Register storage class is usually used for variables that need to be accessed regularly in a program, thereby increasing the program execution speed.
- The lifetime of a register variable is limited to a function, and it cannot be accessed outside it.
- As soon as the function completes its execution, the automatic variables are destroyed because of its limited scope.
- The addresses of such a register cannot even be obtained using pointers like auto storage class. Its visibility is similar to that of a local variable. The initial values of register storage classes are always garbage values.
Syntax:
register datatype variable_name [= value];
Here,
- register is the keyword for the storage class.
- datatype refers to the type of the variable.
- variable_name denotes the name of the variable.
- value refers to the initial garbage value.
Code Example:
Output:
Register Storage Class
Value of a declared as register storage class: U
Explanation:
In the C++ program above we first include the iostream file and the namespace std.
- Then we define the register storage class and a variable of the character datatype as input from the class.
- We intialise the variable with U and use the out statement to print the 'Value of a declared as register storage class'.
- In the main() function, we once again use the cout statement to print 'Register storage class'.
Note: The program simply demonstrates how register storage classes work and the way in which they are declared inside a function. We can notice here that the scope of the variables is limited to that function, and we need to invoke the function to print its actual value.
Static Storage Class In C++
The static storage class is used to declare static storage class variables, which retain their value even outside the function in which it is declared. We use the static keyword to declare variables in this class.
- The memory location for such variables remains until the end of the program. They are hence initialized only once, and no new memory is allocated.
- Global static storage class variables have their block scope as the entire program.
- In C++, whenever we use a static variable on a class data member, only a single copy of that data member is shared by all objects in the entire class.
- These static variables are most popularly used while writing programs in C++.
- The lifetime of a variable's static is the whole program, and it can be accessed anywhere in the program.
- The variable is destroyed only after the completion of the program in the case of the static storage classes.
- Its visibility is similar to that of a local variable. And the initial values of static storage classes are set to be 0.
Syntax:
static datatype variable_name [= value];
Here,
- static is the keyword for the storage class.
- datatype denotes the type of a variable.
- variable_name refers to the name you want to give to the respective variable.
- value refers to the initial value, which here is 0.
Code Example:
Output-
Static Storage Class
Static Variable: 1
Static Variable: 2
Non-Static Variable: 1
Non-Static Variable: 1
Explanation:
In the code example,
- We make use of two types of variables that are static variables (i.e., they retain their value) and non-static variables (that don't retain value).
- The program simply demonstrates how static variables retain their value even outside the function in which they are declared.
- We can notice here that the scope of the static variables are limited to that function, but the memory location remains until the end of the program.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
External Storage Class In C++
The extern storage class is used to declare external variables, which are defined somewhere else and not in the function where it is used. We use the extern keyword for variable declaration.
- The external storage class variables are like global variables that can be accessed anywhere in the program.
- A global variable can be made an external variable by using the extern keyword before its declaration in a separate file.
- The main objective of using extern variables is that no new variable needs to be initialized. This is because the global variable itself can be accessed across several different program files in the case of large executable programs with external linkage.
- The lifetime of an external variable is the whole program, as it can be accessed anywhere in the program.
- This means that the variable is destroyed only after the completion/ execution of the program.
- The visibility of external variables is similar to that of a global variable and can be accessed throughout the file. The initial values of extern storage classes are set to 0.
Syntax:
extern datatype variable_name;
Here,
- extern is the storage location class.
- datatype denotes the data type of a variable.
- variable_name denotes the name of the variable.
C++ Program Example:
Output:
External Storage class
Value of 'a' declared as extern: 0
Modified value of 'a' declared as extern:7.5
Explanation:
The above code snippet depicts the use of an external storage class.
- In this program, we first declare a float variable 'a' outside of the external storage class.
- Then inside the class, we use the extern keyword to declare 'a' as external. Next, we print its default value 0 using the cout statement.
- Later on, we alter the value of ‘a’ to 7.5 and print it using the cout statement. This depicts how we can make use of external storage classes.
Mutable Storage Class In C++
The mutable storage class is used only for those class objects that have mutable specifiers.
- Data members of a constant object can be modified using the keyword mutable.
- A constant member function can be overridden by a member of a class object using mutable specifiers.
- At times, we might need to change some data members without updating other members of the class, which can be done using the const function.
- In this case, the mutable keyword makes this task much easier to complete, as it allows only a particular data member of the const object to be altered.
- The lifetime of a mutable variable is in the class in which it is declared. It helps a member of a class object to override a const member function.
- The visibility of mutable variables is similar to that of a local variable and has ease of access as it can be accessed anywhere in the entire program. The initial value of mutable storage is a garbage value.
Syntax:
mutable datatype variable_name [= value];
Here,
- mutable is the keyword for the storage default class.
- datatype refers to the type of data in a single variable.
- variable_name denotes the name of the respective variable
- value refers to the initial garbage value of the variable
C++ Program Example:
Output:
Mutable Storage Class
b= 15
Explanation:
The above code snippet depicts the use of a mutable storage class.
- We begin by defining a class Mutable after including the iostream file and namespace std.
- We then define two public variables- the first is int a and the second variable b, which is mutable (which can be modified later on).
- Next, we intialise a and b with the values of 5 and 10, respectively.
- Now, we create an object of the class mutable and try to change the value of b.
- We will notice that only the value of b can be changed as it is of the type mutable storage class.
- We use the cout statement to print the results on the output window.
Conclusion
In this article, we discussed storage classes in C++ and their different types.
- We discussed the scope of variables, lifetime, and visibility of other storage classes.
- It is understood by now that there are five storage classes, namely automatic, register, static, extern, and mutable.
- We have covered all the topics related to storage classes in C++ with syntax, as well as source code examples and uses of it.
Also read- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
Frequently Asked Questions
Q. What is the storage class of functions?
Storage classes in C++ are used for variables or functions in a program to know about their scope, lifetime, visibility, and initial values. These functionalities help us to find the existence of a particular variable/function during the execution of a program.
The general syntax for defining storage class for a variable is as follows:
storage_class variable_data_type variable_name;
Here,
- storage_class refers to the specific type of storage class we are using.
- variable_data_type refers to the type of data
- variable_name refers to the name you give to the variable.
Q. What are the types of storage classes in C++?
Storage classes tell us about the scope of variables as well as their lifespan and visibility. In the C++ programming language, there are five storage classes. These are:
-
Automatic Storage Class
-
Register Storage Class
-
Static Storage Class
-
The extern Storage Class
-
The mutable Storage Class
Q. What is the static storage class?
Static storage class is used to declare static variables, which retain their value even outside the function in which it is declared. The memory allocation for such variables remains until the end of the program. Global static variables have their scope as the entire program.
Syntax:
static datatype variable_name [= value];
Q. What are static variables and static functions in C++?
Static variables are variables whose scope is the same as that of a local variable. Although the memory is allocated for the variable during the whole program until the program ends and memory deallocates.
Static data members are accessed by special functions in C++, which are known as static functions. Any function can be called a static function if we add the keyword static before the function name.
Q. What are member functions in C++?
Memory functions are functions in C++ that are defined in the class itself. These functions work on objects of the class and can have quick access to all the members of that class. Member functions can be written anywhere inside or outside of their class definition.
Q. What is an auto-storage class in C++?
Automatic Storage Class is the default storage class for all local variables, and it is declared as well as accessed only within a particular function/method. In case there's no keyword specified to a variable, it automatically gets assigned with the keyword auto
Syntax:
auto datatype variable_name [= value];
Q. What is the function of the storage system in computers?
The function of a storage system in computers is to store important info/data, modify it, and transfer it from one system to the other. There are various computer storage devices that allow users to save and manipulate data and applications securely on their computers. We all are familiar with some basic examples of storage systems that include hard disks, disk drives, tape systems, and other media types.
This compiles our discussion on storage classes in C++. You might also be interested in reading the following:
- Operators In C++ | Types & Precedence Explained (With Examples)
- Comment In C++ | Types, Usage, C-Style Comments & More (+Examples)
- Strings In C++ | Functions, How To Convert & More (With Examples)
- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
- Pointer To Object In C++ | Simplified Explanation & Examples!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment