C++ Programming Language
Table of content:
- A Brief Intro To C++
- The Timeline Of C++
- Importance Of C++
- Versions Of C++ Language
- Comparison With Other Popular Programming Languages
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Variables In C++?
- Declaration & Definition Of Variables In C++
- Variable Initialization In C++
- Rules & Regulations For Naming Variables In C++ Language
- Different Types Of Variables In C++
- Different Types of Variable Initialization In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Primitive Data Types In C++?
- Derived Data Types In C++
- User-Defined Data Types In C++
- Abstract Data Types In C++
- Data Type Modifiers In C++
- Declaring Variables With Auto Keyword
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Structure Of C++ Program: Components
- Compilation & Execution Of C++ Programs | Step-by-Step Explanation
- Structure Of C++ Program With Example
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What is Typedef in C++?
- The Role & Applications of Typedef in C++
- Basic Syntax for typedef in C++
- How Does typedef Work in C++?
- How to Use Typedef in C++ With Examples? (Multiple Data Types)
- The Difference Between #define & Typedef in C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Strings In C++?
- Types Of Strings In C++
- How To Declare & Initialize C-Style Strings In C++ Programs?
- How To Declare & Initialize Strings In C++ Using String Keyword?
- List Of String Functions In C++
- Operations On Strings Using String Functions In C++
- Concatenation Of Strings In C++
- How To Convert Int To Strings In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is String Concatenation In C++?
- How To Concatenate Two Strings In C++ Using The ‘+' Operator?
- String Concatenation Using The strcat( ) Function
- Concatenation Of Two Strings In C++ Using Loops
- String Concatenation Using The append() Function
- C++ String Concatenation Using The Inheritance Of Class
- Concatenate Two Strings In C++ With The Friend and strcat() Functions
- Why Do We Need To Concatenate Two Strings?
- How To Reverse Concatenation Of Strings In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Find In String C++?
- What Is A Substring?
- How To Find A Substring In A String In C++?
- How To Find A Character In String C++?
- Find All Substrings From A Given String In C++
- Index Substring In String In C++ From A Specific Start To A Specific Length
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Pointers In C++?
- Pointer Declaration In C++
- How To Initialize And Use Pointers In C++?
- Different Types Of Pointers In C++
- References & Pointers In C++
- Arrays And Pointers In C++
- String Literals & Pointers In C++
- Pointers To Pointers In C++ (Double Pointers)
- Arithmetic Operation On Pointers In C++
- Advantages Of Pointers In C++
- Some Common Mistakes To Avoid With Pointers In Cpp
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Understanding Pointers In C++
- What Is Pointer To Object In C++?
- Declaration And Use Of Object Pointers In C++
- Advantages Of Pointer To Object In C++
- Pointer To Objects In C++ With Arrow Operator
- An Array Of Objects Using Pointers In C++
- Base Class Pointer For Derived Class Object In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is 'This' Pointer In C++?
- Defining ‘this’ Pointer In C++
- Example Of 'this' Pointer In C++
- Describing The Constness Of 'this' Pointer In C++
- Important Uses Of 'this' Pointer In C++
- Method Chaining Using 'this' Pointer In C++
- C++ Programs To Show Application Of 'This' Pointer
- How To Delete The ‘this’ Pointer In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What is Reference?
- What is Pointer?
- Comparison Table Of C++ Pointer Vs. Reference
- Differences Between Reference And Pointer: A Detailed Explanation
- Why Are References Less Powerful Than Pointers?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- How To Declare A 2D Array In C++?
- C++ Multi-Dimensional Arrays
- Ways To Initialize A 2D Array In C++
- Methods To Dynamically Allocate A 2D Array In C++
- Accessing/ Referencing Two-Dimensional Array Elements
- How To Initialize A Two-Dimensional Integer Array In C++?
- How To Initialize A Two-Dimensional Character Array?
- How To Enter Data In Two-Dimensional Array In C++?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Arrays Of Strings In C++?
- Different Ways To Create String Arrays In C++
- How To Access The Elements Of A String Array In C++?
- How To Convert Char Array To String?
- Conclusion
- Frequently Asked Questions
Table of content:
- What is Memory Allocation in C++?
- The “new" Operator In C++
- The "delete" Operator In C++
- Dynamic Memory Allocation In C++ | Arrays
- Dynamic Memory Allocation In C++ | Objects
- Deallocation Of Dynamic Memory
- Dynamic Memory Allocation In C++ | Uses
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A Substring In C++ (Substr C++)?
- Example For Substr In C++
- Points To Remember For Substr In C++
- Important Applications Of substr() Function
- How to Get a Substring Before a Character?
- Print All Substrings Of A Given String
- Print Sum Of All Substrings Of A String Representing A Number
- Print Minimum Value Of All Substrings Of A String Representing A Number
- Print Maximum Value Of All Substrings Of A String Representing A Number
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Operator In C++?
- Types Of Operators In C++ With Examples
- What Are Arithmetic Operators In C++?
- What Are Assignment Operators In C++?
- What Are Relational Operators In C++?
- What Are Logical Operators In C++?
- What Are Bitwise Operators In C++?
- What Is Ternary/ Conditional Operator In C++?
- Miscellaneous Operators In C++
- Precedence & Associativity Of Operators In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is The New Operator In C++?
- Example To Understand New Operator In C++
- The Grammar Elements Of The New Operator In C++
- Storage Space Allocation
- How Does The C++ New Operator Works?
- What Happens When Enough Memory In The Program Is Not Available?
- Initializing Objects Allocated With New Operator In C++
- Lifetime Of Objects Allocated With The New Operator In C++
- What Is The Delete Operator In C++?
- Difference Between New And Delete Operator In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Types Of Overloading In C++
- What Is Operator Overloading In C++?
- How To Overload An Operator In C++?
- Overloadable & Non-overloadable Operators In C++
- Unary Operator Overloading In C++
- Binary Operator Overloading In C++
- Special Operator Overloading In C++
- Rules For Operator Overloading In C++
- Advantages And Disadvantages Of Operator Overloading In C++
- Function Overloading In C++
- What Is the Difference Between Operator Functions and Normal Functions?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Operators In C++?
- Introduction To Logical Operators In C++
- Types Of Logical Operators In C++ With Example Program
- Logical AND (&&) Operator In C++
- Logical NOT(!) Operator In C++
- Logical Operator Precedence And Associativity In C++
- Relation Between Conditional Statements And Logical Operators In C++
- C++ Relational Operators
- Conclusion
- Frequently Asked Important Interview Questions:
- Test Your Skills: Quiz Time
Table of content:
- Different Type Of C++ Bitwise Operators
- C++ Bitwise AND Operator
- C++ Bitwise OR Operator
- C++ Bitwise XOR Operator
- Bitwise Left Shift Operator In C++
- Bitwise Right Shift Operator In C++
- Bitwise NOT Operator
- What Is The Meaning Of Set Bit In C++?
- What Does Clear Bit Mean?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Types of Comments in C++
- Single Line Comment In C++
- Multi-Line Comment In C++
- How Do Compilers Process Comments In C++?
- C- Style Comments In C++
- How To Use Comment In C++ For Debugging Purposes?
- When To Use Comments While Writing Codes?
- Why Do We Use Comments In Codes?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Storage Classes In Cpp?
- What Is The Scope Of Variables?
- What Are Lifetime And Visibility Of Variables In C++?
- Types of Storage Classes in C++
- Automatic Storage Class In C++
- Register Storage Class In C++
- Static Storage Class In C++
- External Storage Class In C++
- Mutable Storage Class In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- Decision Making Statements In C++
- Types Of Conditional Statements In C++
- If-Else Statement In C++
- If-Else-If Ladder Statement In C++
- Nested If Statements In C++
- Alternatives To Conditional If-Else In C++
- Switch Case Statement In C++
- Jump Statements & If-Else In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A Switch Statement/ Switch Case In C++?
- Rules Of Switch Case In C++
- How Does Switch Case In C++ Work?
- The break Keyword In Switch Case C++
- The default Keyword In C++ Switch Case
- Switch Case Without Break And Default
- Advantages & Disadvantages of C++ Switch Case
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A For Loop In C++?
- Syntax Of For Loop In C++
- How Does A For Loop In C++ Work?
- Examples Of For Loop Program In C++
- Ranged Based For Loop In C++
- Nested For Loop In C++
- Infinite For Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is A While Loop In C++?
- Parts Of The While Loop In C++
- C++ While Loop Program Example
- How Does A While Loop In C++ Work?
- What Is Pre-checking Process Or Entry-controlled Loop?
- When Are While Loops In C++ Useful?
- Example C++ While Loop Program
- What Are Nested While Loops In C++?
- Infinite While Loop In C++
- Alternatives To While Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are Loops & Its Types In C++?
- What Is A Do-While Loop In C++?
- Do-While Loop Example In C++ To Print Numbers
- How Does A Do-While Loop In C++ Work?
- Various Components Of The Do-While Loop In C++
- Example 2: Adding User-Input Positive Numbers With Do-While Loop
- C++ Nested Do-While Loop
- C++ Infinitive Do-while Loop
- What is the Difference Between While Loop and Do While Loop in C++?
- When To Use A Do-While Loop?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Are 2D Vectors In C++?
- How To Declare 2D Vector In C++?
- How To Initialize 2D Vector In C++?
- C++ Program Examples For 2D Vectors
- How To Access & Modify 2D Vector Elements In C++?
- Methods To Traverse, Manipulate & Print 2D Vectors In C++
- Adding Elements To 2-D Vector Using push_back() Function
- Removing Elements From Vector In C++ Using pop_back() Function
- Creating 2D Vector In C++ With User Input For Size Of Column & Row
- Advantages of 2D Vectors Over Traditional Arrays
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- How To Print A Vector In C++ By Overloading Left Shift (<<) Operator?
- How To Print Vector In C++ Using Range-Based For-Loop?
- Print Vector In C++ With Comma Separator
- Printing Vector In C++ Using Indices (Square Brackets/ Double Brackets & at() Function)
- How To Print A Vector In C++ Using std::copy?
- How To Print A Vector In C++ Using for_each() Function?
- Printing C++ Vector Using The Lambda Function
- How To Print Vector In C++ Using Iterators?
- Conclusion
- Frequently Asked Questions
Table of content:
- Definition Of C++ Find In Vector
- Using The std::find() Function
- How Does find() In Vector C++ Function Work?
- Finding An Element By Custom Comparator Using std::find_if() Function
- Use std::find_if() With std::distance()
- Element Find In Vector C++ Using For Loop
- Using The find_if_not Function
- Find Elements With The Linear Search Approach
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Sort() Function In C++?
- Sort() Function In C++ From Standard Template Library
- Exceptions Of Sort() Function/ Algorithm In C++
- The Stable Sort() Function In C++
- Partial Sort() Function In C++
- Sorting In Ascending Order With Sort() Function In C++
- Sorting In Descending Order With Sort Function In C++
- Sorting In Desired Order With Custom Comparator Function & Sort Function In C++
- Sorting Elements In Desired Order Using Lambda Expression & Sort Function In C++
- Types of Sorting Algorithms In C++
- Advanced Sorting Algorithms In C++
- How Does the Sort() Function Algorithm Work In C++?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Function Overloading In C++?
- Ways Of Function Overloading In C++
- Function Overloading In C++ Using Different Types Of Parameters
- Function Overloading In C++ With Different Number Of Parameters
- Function Overloading In C++ Using Different Sequence Of Parameters
- How Does Function Overloading In C++ Work?
- Rules Of Function Overloading In C++
- Why Is Function Overloading Used?
- Types Of Function Overloading Based On Time Of Resolution
- Causes Of Function Overloading In C++
- Ambiguity & Function Overloading In C++
- Advantages Of Function Overloading In C++
- Disadvantages Of Function Overloading In C++
- Operator Overloading In C++
- Function Overriding In C++
- Difference Between Function Overriding & Function Overloading In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is An Inline Function In C++?
- How To Define The Inline Function In C++?
- How Does Inline Function In C++ Work?
- The Need For An Inline Function In C++
- Can The Compiler Ignore/ Reject Inline Function In C++ Programs?
- Normal Function Vs. Inline Function In C++
- Classes & Inline Function In C++
- Understanding Inline, __inline, And __forceinline Functions In C++
- When To Use An Inline Function In C++?
- Advantages Of Inline Function In C++
- Disadvantages Of Inline Function In C++
- Why Not Use Macros Instead Of An Inline Function In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Static Data Member In C++?
- How To Declare Static Data Members In C++?
- How To Initialize/ Define Static Data Member In C++?
- Ways To Access A Static Data Member In C++
- What Are Static Member Functions In C++?
- Example Of Member Function & Static Data Member In C++
- Practical Applications Of Static Data Member In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constant In C++?
- Ways To Define Constant In C++
- What Are Literals In C++?
- Pointer To A Constant In C++
- Constant Function Arguments In C++
- Constant Member Function Of Class In C++
- Constant Data Members In C++
- Object Constant In C++
- Conclusion
- Frequently Asked Questions(FAQ)
Table of content:
- What Is Friend Function In C++?
- Declaration Of Friend Function In C++ With Example
- Characteristics Of Friend Function In C++
- Global Friend Function In C++ (Global Function As Friend Function )
- Member Function Of Another Class As Friend Function In C++
- Function Overloading Using Friend Function In C++
- Advantages & Disadvantages Of Friend Function in C++
- What Is A C++ Friend Class?
- A Function Friendly To Multiple Classes
- C++ Friend Class Vs. Friend Function In C++
- Some Important Points About Friend Functions And Classes In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Function Overriding In C++?
- The Working Mechanism Of Function Overriding In C++
- Real-Life Example Of Function Overriding In C++
- Accessing Overriding Function In C++
- Accessing Overridden Function In C++
- Function Call Binding With Class Objects | Function Overriding In C++
- Function Call Binding With Base Class Pointers | Function Overriding In C++
- Advantages Of Function Overriding In C++
- Variations In Function Overriding In C++
- Function Overloading In C++
- Function Overloading Vs Function Overriding In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Errors In C++
- What Is Exception Handling In C++?
- Exception Handling In C++ Program Example
- C++ Exception Handling: Basic Keywords
- The Need For C++ Exception Handling
- C++ Standard Exceptions
- C++ Exception Classes
- User-Defined Exceptions In C++
- Advantages & Disadvantages Of C++ Exception Handling
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Templates In C++ & How Do They Work?
- Types Of Templates In C++
- What Are Function Templates In C++?
- C++ Template Functions With Multiple Parameters
- C++ Template Function Overloading
- What Are Class Templates In C++?
- Defining A Class Member Outside C++ Template Class
- C++ Template Class With Multiple Parameters
- What Is C++ Template Specialization?
- How To Specify Default Arguments For Templates In C++?
- Advantages Of C++ Templates
- Disadvantages Of C++ Templates
- Difference Between Function Overloading And Templates In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Structure
- Structure Declaration
- Initialization of Structure
- Copying and Comparing Structures
- Array of Structures
- Nested Structures
- Pointer to a Structure
- Structure as Function Argument
- Self Referential Structures
- Class
- Object Declaration
- Accessing Class Members
- Similarities between Structure and Class
- Which One Should You Choose?
- Key Difference Between a Structure and Class
- Summing Up
- Test Your Skills: Quiz Time
Table of content:
- What Is A Class And Object In C++?
- What Is An Object In C++?
- How To Create A Class & Object In C++? With Example
- Access Modifiers & Class/ Object In C++
- Member Functions Of A Class In C++
- How To Access Data Members And Member Functions?
- Significance Of Class & Object In C++
- What Are Constructors In C++ & Its Types?
- What Is A Destructor Of Class In C++?
- An Array Of Objects In C++
- Object In C++ As Function Arguments
- The this (->) Pointer & Classes In C++
- The Need For Semicolons At The End Of A Class In C++
- Difference Between Structure & Class In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Static Members In C++?
- Static Member Functions in C++
- Ways To Call Static Member Function In C++
- Properties Of Static Member Function In C++
- Need Of Static Member Functions In C++
- Regular Member Function Vs. Static Member Function In C++
- Limitations Of Static Member Functions In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Constructor In C++?
- Characteristics Of A Constructor In C++
- Types Of Constructors In C++
- Default Constructor In C++
- Parameterized Constructor In C++
- Copy Constructor In C++
- Dynamic Constructor In C++
- Benefits Of Using Constructor In C++
- How Does Constructor In C++ Differ From Normal Member Function?
- Constructor Overloading In C++
- Constructor For Array Of Objects In C++
- Constructor In C++ With Default Arguments
- Initializer List For Constructor In C++
- Dynamic Initialization Using Constructor In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constructor In C++?
- What Is Constructor Overloading In C++?
- Dеclaration Of Constructor Ovеrloading In C++
- Condition For Constructor Overloading In C++
- How Constructor Ovеrloading In C++ Works?
- Examples Of Constructor Overloading In C++
- Lеgal & Illеgal Constructor Ovеrloading In C++
- Types Of Constructors In C++
- Characteristics Of Constructors In C++
- Advantage Of Constructor Overloading In C++
- Disadvantage Of Constructor Overloading In C++
- Conclusion
- Frеquеntly Askеd Quеstions
Table of content:
- What Is A Destructor In C++?
- Rules For Defining A Destructor In C++
- When Is A Destructor in C++ Called?
- Order Of Destruction In C++
- Default Destructor & User-Defined Destructor In C++
- Virtual Destructor In C++
- Pure Virtual Destructor In C++
- Key Properties Of Destructor In C++ You Must Know
- Explicit Destructor Calls In C++
- Destructor Overloading In C++
- Difference Between Normal Member Function & Destructor In C++
- Important Uses Of Destructor In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Constructor In C++?
- What Is A Destructor In C++?
- Difference Between Constructor And Destructor In C++
- Constructor In C++ | A Brief Explanation
- Destructor In C++ | A Brief Explanation
- Difference Between Constructor And Destructor In C++ Explained
- Order Of Calling Constructor And Destructor In C++ Classes
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
Table of content:
- What Is Type Conversion In C++?
- What Is Type Casting In C++?
- Types Of Type Conversion In C++
- Implicit Type Conversion (Coercion) In C++
- Explicit Type Conversion (Casting) In C++
- Advantages Of Type Conversion In C++
- Disadvantages Of Type Conversion In C++
- Difference Between Type Casting & Type Conversion In C++
- Application Of Type Casting In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Copy Constructor In C++?
- Characteristics Of Copy Constructors In C++
- Types Of Copy Constructors In C++
- When Do We Call The Copy Constructor In C++?
- When Is A User-Defined Copy Constructor Needed In C++?
- Types Of Constructor Copies In C++
- Can We Make The Copy Constructor In C++ Private?
- Assignment Operator Vs Copy Constructor In C++
- Example Of Class Where A Copy Constructor Is Essential
- Uses Of Copy Constructors In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Do You Need Object-Oriented Programming (OOP) In C++?
- OOPs Concepts In C++ With Examples
- The Class OOPs Concept In C++
- The Object OOPs Concept In C++
- The Inheritance OOPs Concept In C++
- Polymorphism OOPs Concept In C++
- Abstraction OOPs Concept In C++
- Encapsulation OOPs Concept In C++
- Other Features Of OOPs In C++
- Benefits Of OOP In C++ Over Procedural-Oriented Programming
- Disadvantages Of OOPS Concept In C++
- Why Is C++ A Partial OOP Language?
- Conclusion
- Frequently Asked Questions
Table of content:
- Introduction To Abstraction In C++
- Types Of Abstraction In C++
- What Is Data Abstraction In C++?
- Understanding Data Abstraction In C++ Using Real Life Example
- Ways Of Achieving Data Abstraction In C++
- What Is An Abstract Class?
- Advantages Of Data Abstraction In C++
- Use Cases Of Data Abstraction In C++
- Encapsulation Vs. Abstraction In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Encapsulation In C++?
- How Does Encapsulation Work In C++?
- Types Of Encapsulation In C++
- Why Do We Need Encapsulation In C++?
- Implementation Of Encapsulation In C++
- Access Specifiers & Encapsulation In C++
- Role Of Access Specifiers In Encapsulation In C++
- Member Functions & Encapsulation In C++
- Data Hiding & Encapsulation In C++
- Features Of Encapsulation In C++
- Advantages & Disadvantages Of Encapsulation In C++
- Difference Between Abstraction and Encapsulation In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Inheritance In C++?
- What Are Child And Parent Classes?
- Syntax And Structure Of Inheritance In C++
- Implementing Inheritance In C++
- Importance Of Inheritance In C++
- Types Of Inheritance In C++
- Visibility Modes Of Inheritance In C++
- Access Modifiers & Inheritance In C++
- How To Make A Private Member Inheritable?
- Member Function Overriding In Inheritance In C++
- The Diamond Problem | Inheritance In C++ & Ambiguity
- Ways To Avoid Ambiguity Inheritance In C++
- Why & When To Use Inheritance In C++?
- Advantages Of Inheritance In C++
- The Disadvantages Of Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Hybrid Inheritance In C++?
- Importance Of Hybrid Inheritance In Object Oriented Programming
- Example Of Hybrid Inheritance In C++: Using Single and Multiple Inheritance
- Example Of Hybrid Inheritance In C++: Using Multilevel and Hierarchical Inheritance
- Real-World Applications Of Hybrid Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Multiple Inheritance In C++?
- Examples Of Multiple Inheritance In C++
- Ambiguity Problem In Multiple Inheritance In C++
- Ambiguity Resolution In Multiple Inheritance In C++
- The Diamond Problem In Multiple Inheritance In C++
- Visibility Modes In Multiple Inheritance In C++
- Advantages & Disadvantages Of Multiple Inheritance In C++
- Multiple Inheritance Vs. Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Multilevel Inheritance In C++?
- Block Diagram For Multilevel Inheritance In C++
- Multilevel Inheritance In C++ Example
- Constructor & Multilevel Inheritance In C++
- Use Cases Of Multilevel Inheritance In C++
- Multiple Vs Multilevel Inheritance In C++
- Advantages & Disadvantages Of Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Hierarchical Inheritance In C++?
- Example 1: Hierarchical Inheritance In C++
- Example 2: Hierarchical Inheritance In C++
- Impact of Visibility Modes In Hierarchical Inheritance In C++
- Advantages And Disadvantages Of Hierarchical Inheritance In C++
- Use Cases Of Hierarchical Inheritance In C++
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Access Specifiers In C++?
- Types Of Access Specifiers In C++
- Public Access Specifiers In C++
- Private Access Specifier In C++
- Protected Access Specifier In C++
- The Need For Access Specifiers In C++
- Combined Example For All Access Specifiers In C++
- Best Practices For Using Access Specifiers In C++
- Why Can't Private Members Be Accessed From Outside A Class?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Diamond Problem In C++?
- Example Of The Diamond Problem In C++
- Resolution Of The Diamond Problem In C++
- Virtual Inheritance To Resolve Diamond Problem In C++
- Scope Resolution Operator To Resolve Diamond Problem In C++
- Conclusion
- Frequently Asked Questions
Comment In C++ | Types, Usage, C-Style Comments & More (+Examples)
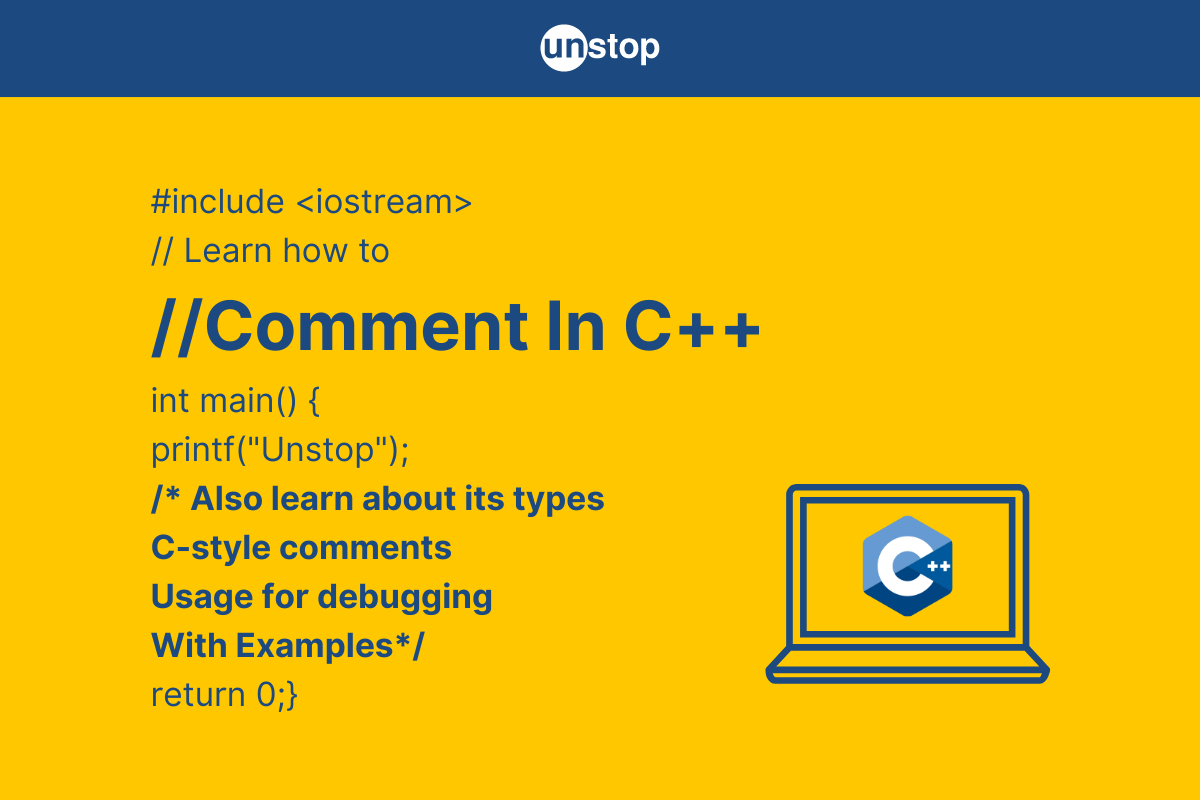
Comments are texts written in a program along with the code to provide descriptions and key points about what’s happening in the code. These comments are mostly supported in all programming languages to make the code readable. Comments in C++ are mostly of two types- single-line comments and multi-line comments in C++. It helps programmers to understand the code better and check execution for alternative codes as well.
In this article, we will have a look at different types of comments in C++, how to comment in C++, c style comments in C++ programming, the use of comments for debugging, and how the compiler processes C++ comments. We will see various examples along with the explanation to better understand the topic.
Types of Comments in C++
As mentioned before, we mostly use two types of comments to make our code more readable and understandable in C++ language. They are:
- Single Line Comments
- Multi-Line Comments
We will discuss both of them one by one in this article. So, let’s get started.
Single Line Comment In C++
As the name suggests, a C++ single-line comment is a comment that consists of one line only. That is, it can occupy only a single line of the code. These comments are represented by two forward slashes(//).
Anything written after // until the end of the first line is a single-line comment. These comments are only for the programmers to understand the code better. The compiler ignores everything written after //, and hence the comments do not affect the program directly in any way.
Syntax of Single line comment in C++
// declaring a string s
string s;// initializing the string 's' as “unstop”
s= “unstop”;
Here, the single-line comments are used to mention what we are doing with strings. That is,
- // declaring a string s
- // initializing the string 's' as “unstop”
Let's take a look at a code example to see single-line comments in action.
Code Example:
#include <iostream>
using namespace std;
int main () {
// This single-line comment is skipped while compiling.
cout<<âsingle-line comment in C++â;
return0;
}
Output:
Single-line comment in C++
Explanation:
In the example here,
- We begin by including the iostream file.
- Next, in the main function, we add a single-line comment beginning with //.
- And then, we use the cout command to print a statement.
- The output shows that only the cout command is implemented while the content written after // goes unseen.
The above code snippet demonstrates the use of single-line comments in C++. These comments are normally unseen/ ignored by the compiler while the rest of the code gets executed. In the above example, the program simply prints a single-line comment in C++ as output.
Multi-Line Comment In C++
C++ also supports multi-line comments, which are spread over many lines to provide a description of the code. That is, these comments can span multiple lines in a code. They are enclosed in the delimiters /*.....*/.
That is, anything written between /* until the end of the */ is a multi-line comment. Just like single-line comments, these comments are also only for the programmers to understand the code better. And again, the compiler ignores everything written between /*..*/, and hence the comments do not affect the program directly in any way.
Syntax of multi-line comment in C++
/*
C++
Multi
Line
Comment
*/
Code Example:
#include <iostream>
using namespacestd;
int main()
{
/* Demonstrate
Multi Line comment
In C++
*/
cout << "Multi Line Comment in C++";
return 0;
}
Output:
Multi Line Comment in C++
Explanation:
In the code example, we begin by-
- The iostream file and also use the namespace std.
- We then leave a comment enclosed in /*...*/ in the main int function.
- After that, we use the cout command to print output and close the program with return 0.
- As seen in the output window, only the cout command is executed, and the content within the /*...*/ delimiters is unprocessed.
The code above demonstrates the use of multi-line comments in C++. Again the comments go unseen by the compiler while it executes the rest of the code. In the above example, the program simply prints multi-line comment in C++ as output.
Also read: Pointers in C++ | A Roadmap To All Types Of Pointers With Examples
How Do Compilers Process Comments In C++?
The compilation process consists of four primary stages, i.e., the pre-processor stage, compiling, assembling, and linking. The compiler carries out lexical analysis, syntax analysis, and cod generations within the compiling stage.
The lexical analysis stage is also known as the scanning or tokenization phase, during which the compiler identifies the different elements/ tokens of the code, such as identifiers, operators, literals, etc. During this stage, the compiler would ideally decide what to do with the comments. But it instead treats them as whitespace and skips them. In other words, the compiler ignores the comments during the compilation process.
In other words, the comments are not a part of the compiled output, and the only primary function they serve is to provide an explanation of the code without affecting its functionality. So, at the end of the C++ program, there are no comments left, and the program is compiled successfully. This proves that comments are only meant for programmers to understand things and not for the machine itself.
Code Example-
//showcasing how a compiler processes C++ comments
#include <iostream>
using namespacestd;
int main()
{
/* Demonstrate
Multi-Line comment
In C++
*/
cout << "Demonstrating compiler process";
return 0;
}
Output:
Demonstrating compiler process.
Explanation:
As shown in the code example above,
- Beginning with the first line, the compiler ignores the first single-line comment during the compilation process and moves ahead to include the iostream file and the namespace std.
- Within the main function, it ignores the multi-line comment and goes directly to the cout command.
- It hence prints “Demonstrating compiler process”.
- Finally, the function then returns 0, and the compilation finishes.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
C- Style Comments In C++
C-style comment methods are for large blocks of text or code and are popularly known as multi-line comments. However, they can be used to comment on single lines as well. We can simply write texts between /* … */ to indicate it as a C-style comment. The compiler ignores all the contents between /* … */during compilation.
In the case of C++-style comments, they are usually used to comment on single lines of text or code and are popularly known as single-line comments. However, they can be merged together to form multi-line comments. We can simply write texts starting with // to indicate it as C++-style comments. Everything written between // until the start of the new line is ignored by the compiler during compilation.
The syntax for writing C-style comments within C++-style comments:
// int a=5; /*declare a integer variable a as 5 */
Here,
// int a=5; is a C++ style comment and,
/*declare a integer variable a as 5 */ is a C style comment.
Code Example:
/* C-style
comment acting as
Multi-line comment */
#include <iostream>
using namespace std;
int main()
{
//C++ style comment as single line comment
cout << "C style comments in C++";
return 0;
}
Output:
C style comments in C++
Explanation:
The above code snippets explain the difference between C-style comments and C++-style comments. It denotes the syntax in which it can be written in a code segment. During compilation, the compiler ignores both of them, and the program simply prints “C style comments in C++” as output.
How To Use Comment In C++ For Debugging Purposes?
One of the most important uses of program comments is to debug programs in code editors. Whenever we come across errors in a code, we start the debugging process by commenting out some segments of error-prone code. For this, we can use the delimiters /*..*/ to check whether the respective part of the code is causing an error. This helps us save time and helps avoid deleting/ rewriting the entire code.
Also, even if the code turns out to be right, we can move ahead with some other segment of code and continue until we find the error-causing code. This is an efficient way to figure out errors and help in debugging the code. The syntax for this is the same as a multiline comment in C++.
Syntax:
/* int x=5, y=6, mult=0;
mult= x*y;
cout << “multiply=” << mult; */
Code Example (Without Comments):
#include <iostream>
using namespace std;
int main() {
int a=6, b=5, sum=0, diff=0;
sum = a+b;
cout << âsum=â << sum;
diff = a-b
cout<< âdifference=â << diff;
return 0;
}
Output:
Compilation error
missing ; before cout.
Here, we get a compilation error because a (;) is missing from one of the lines. While this is a short code and one can browse through it. The same might not be feasible in the case of comparatively complex codes.
Debugging the code using comment-
#include <iostream>
using namespace std;
int main()
{
int a=6, b=5, sum=0, diff=0;
sum = a+b;
cout << âsum=â << sum;
/* diff = a-b
cout<< âdifference=â << diff; */
return 0;
}
Output:
Sum=11
Explanation:
In the above code example, the program first displays an error while compiling it for the first time. To figure out the error, we can convert the second half of the code into a comment and see if the rest of the code works.
So, we comment out the later section of the code and then compile it again. This time the code executes and gives the output as Sum = 11. It helps us understand that the problem is with that latter part where a ; missing. With the scope of error narrowed down, we can easily rectify our code and compile it again to get the output.
Final Code after debugging-
#include <iostream>
using namespace std;
int main()
{
int a=6, b=5, sum=0, diff=0;
sum = a+b;
cout << âsum=â << sum;
diff = a-b;
cout<< " difference=" << diff;
return 0;
}
Output:
Sum=11
Difference=1
Note: After successfully debugging the code, we can rectify the errors and arrive at the final solution. Now, the code snippets evaluate the sum and difference of 2 numbers and print it. The function then returns 0, and the compilation ends.
When To Use Comments While Writing Codes?
There are three primary cases in which we can use comments in codes. They are:
Case 1: At the beginning of the program, to mention the aim of the program and what the program does.
Case 2: At the beginning of each function, to write the description of the function and what the function returns.
Case 3: In case of complex lines of code, to understand the logic and help debug the code.
Why Do We Use Comments In Codes?
Comments in programming are used to provide well-structured and self-explanatory code. They are written in human-readable language and are ignored by the compiler or interpreter, meaning they don't affect the execution of the program. Comments are primarily used for the following reasons:
- It makes the code more readable.
- It can help summarize the entire code and what the program does in explanatory statements.
- While working in a group, individuals' commenting functions help fellow programmers understand what's happening.
- It helps in understanding the difficult segments of a code by providing a brief description.
- It makes finding bugs easier by debugging the program step-by-step.
- It makes commenting on alternative approaches to the solution much easier and more understandable.
- It helps save time by identifying important blocks of code easily.
- It makes the task of going through past codes much easier, and the maintenance of code is also done nicely.
Conclusion
In this article, we discussed comments in C++ and its different types with the help of examples. We also discussed how it is a part of good programming practices and helps one write more readable code. We now know that the compiler simply ignores the comment and focuses only on the code. It is also evident that comments can play a crucial role in debugging and making a program functional. As discussed in the article, there are multiple reasons for using comments, which is why everyone must know how to use them properly.
Frequently Asked Questions
Q. What are the comments in C++?
Comments are texts written in a program along with the code to provide a description and key points about what’s happening in the code. These comments are mostly supported in all programming languages to make the code readable. Comments in C++ have mainly two types, i.e., single-line and multi-line comments in C++. It helps programmers to understand the code better and check execution for alternative codes as well.
Q. Does C++ compile comments?
The answer to this is no. The compiler skips over the comments and does not include them as a part of the lexical analysis in the tokenization phase. Since the comments do not add any functionality or affect the execution of the code in any way, they are simply ignored in the compilation process. This is also because comments are only for programmers to either understand the code better, for future references, or for debugging, etc.
Q. What types of comments are supported by C++?
Comments are mostly supported in all programming languages to make the code readable. Comments in C++ are mostly of two types:
1. Single-line comments in C++, i.e., comments that span only one code line. These comments begin with double slashes (//), and the syntax for these is-
//C++ single-line comment
2. Multi-line comments in C++, i.e., comments that can span multiple lines of code. These comments are enclosed in /*...*/ delimiters. The syntax hence is:
/*
C++
Multi
Line
Comment
*/
These comments help programmers understand the code, debug it, make it more readable, and help check execution for alternative codes.
Q. How do you comment on multiple lines?
To begin with, note that a multi-line comment is a single comment which spans multiple lines of code. So we can directly use these types of comments to comment on multiple lines. But this is not the only way to do so. In fact, we can also use single-line comments to comment on multiple lines. Let's see how that is done.
Using single-line comments:
When using single-line comments, we have to comment on every line we want individually. And as usual, the comment needs to start with a double forward slash (//).
Example:
#include <iostream>
using namespace std;
int main(){
// cout<<âlearning comments in C++â;
cout<<"Commenting on line1";
// cout<< âThis block will not be printedâ;
cout << "\nUsing single-line comment";
return 0;
}
Output:
Using single-line comment
Using multi-line comments:
Multi-line comments in C++, by definition, allow us to comment on multiple lines. So all we have to do is enclose our comments in the /*..*/ delimiters. An example of the same is given below:
Example:
#include <iostream>
using namespace std;
int main(){
/*cout<<âlearning comments in C++â;
cout<< âThis block will not be printedâ; */
cout << "\nUsing multi-line comment";
return 0;
}
Output:
Commenting on line1
Using single-line comment
Q. What is the use of /* */?
Multi-line comments are used in C++ to write large text descriptions and explain the code to make it more user-friendly. It can also be used to mark different alternative approaches to code as comments, which can be useful to fellow programmers in the future. All of these blocks of comments will be simply ignored by the compiler while compilation of the program.
Test Your Skills: Quiz Time
We have reached the end of the article on comments in C++. You might also be interested in reading:
- References In C++ | Declare, Types, Properties & More (+Examples)
- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- Difference Between C And C++| Features | Application & More!
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Kartik Deshmukh 2 days ago
YASH SRIVASTAVA 1 month ago
Sanchit Dhale 1 month ago
Divyansh Shrivastava 1 month ago