Table of content:
- C++ Interview Questions and Answers: The Basics
- C++ Interview Questions: Intermediate
- C++ Interview Questions And Answers With Code Examples
- C++ Interview Questions and Answers: Advanced
51 C++ Interview Questions For Freshers & Experienced (With Answers)
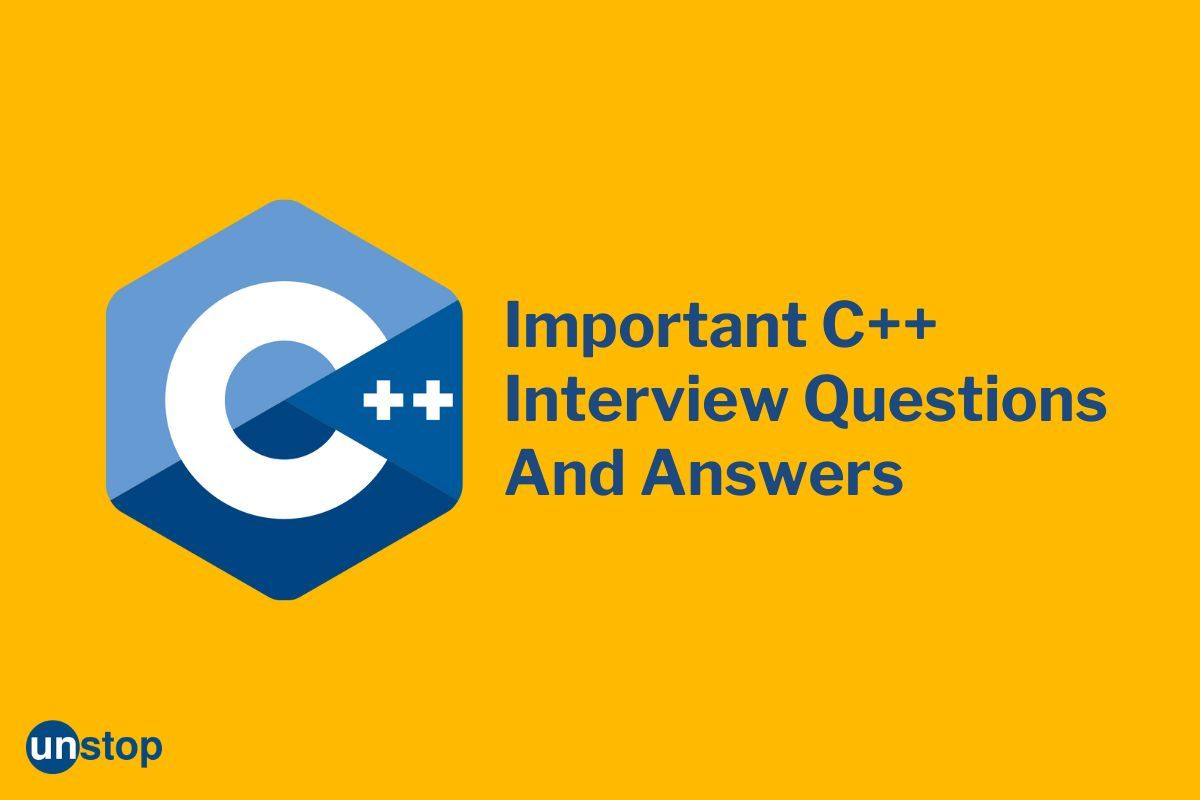
The C++ language, also called C with Classes, is a general-purpose language. Bjarne Stroustrup, a Danish computer scientist, created C++ in 1985 as an extension of the C language. Even after 37 years since it first appeared, the language is one of the most popular cross-platform languages used in high-performance application creation. Naturally, the demand for developers/ programmers skilled in C++ is high. And with this cut-throat competition, your answers to C++ interview questions must show expertise. To help you prepare for your interview, here is a list of questions that will test your knowledge of basic, intermediate, and advanced topics. Irrespective of whether you are a fresher or an expert, you will benefit from this question bank for the C++ language. So let's get started!
C++ Interview Questions and Answers: The Basics
In this section, we will look at some C++ interview questions that will test your understanding of some basic concepts in the language. Note that these might seem like simple questions for freshers, but they are not. Since the theme for these is the basics, everyone, including experienced programmers, must be able to answer them quickly.
Q1. What is C++ programming language?
C++ is a versatile coding language used to develop applications and systems. In 1983, Bjarne Stroustrup created a programming language that was an offspring of the C programming language at Bell Labs. C++ is also a portable language that consists of various programming paradigms, including procedural, functional, and generic. It allows for an object-oriented approach to coding with multiple ways to solve problems.
It is used in operating systems, web browsers, game development, banking applications, graphic applications, compilers, cloud systems, embedded systems, and as the core language for libraries.
You might also be interested in reading- History Of C++ | Detailed Explanation (With Timeline Infographic)
Q2. How do C and C++ vary from one another?
Even though C++ is an extension of C, there are some important differences between the two, as given in the table below:
C Programming Language | C++ Programming Language |
C is a procedural programming language. | C++ is an object-oriented programming language. |
The C language is the Subset of C++ | The C++ language is the Superset of C |
It does not support any object-oriented programming (OOPs) concepts like polymorphism, data abstraction, encapsulation, classes, and objects. | C++ supports all object-oriented programming (OOPs) concepts. |
No namespace feature is available. | The namespace feature is available in C++. |
It is function-driven | It is object-driven |
Supports only built-in data types. | Support built-in datatypes as well as user-defined data types. |
Q3. Mention the different data types that are there in C++.
There are 4 data types in C++, which are as follows:
- Primary data type- character, short, integer, float, long double, void, Boolean, etc.
- Derived data type- arrays, pointers, functions, references, etc.
- User-defined data type- class, structure, union, etc.
- Enumeration data type – enum.
For more information, read- Data Types In C++ | A Detailed Explanation With Examples
Q4. Explain the difference between struct and a class.
STRUCT | CLASS |
Struct members are always public. | Class members can be in public, private, or protected mode. |
Inheritance is not possible in struct. | The class supports inheritance property. |
It has stack memory storage. | It has heap memory storage. |
Also, read- Decoding The Difference Between Structure And Class In C++
Q5. What are the different types of tokens in C++?
The different types of tokens in C++ are-
Variables | They refer to the name given to a data storage location. |
Constants | The value of these tokens never changes |
Keywords | These tokens have a meaning. For example, auto, break, double, etc |
Identifiers | These are case-sensitive tokens, and hence their names are unique. |
Operators | This includes operators used to perform various operations, like +,-,*,++,--,% ,etc |
Q6. What is a string in C++?
A collection (or series) of various characters is represented by an object type called a string in C++. The string class records track of a string's characters as a collection of bytes, and memory is dynamic size.
Syntax:
string str_name = "Be Unstoppable";
Source code example:
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8c3RyaW5nPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewpzdHJpbmcgczEgPSAiSGVsbG8sICI7CnN0cmluZyBzMiA9ICJ3b3JsZCEiOwpzdHJpbmcgczMgPSBzMSArIHMyOwoKY291dCA8PCBzMyA8PCBlbmRsOyAvLyBPdXRwdXQ6ICJIZWxsbywgd29ybGQhIgpjb3V0IDw8ICJUaGUgbGVuZ3RoIG9mIHMzIGlzICIgPDwgczMubGVuZ3RoKCkgPDwgIiBjaGFyYWN0ZXJzLiIgPDwgZW5kbDsgLy8gT3V0cHV0OiAiVGhlIGxlbmd0aCBvZiBzMyBpcyAxMyBjaGFyYWN0ZXJzLiIKCnJldHVybiAwOwp9
Source Output:
Hello, world!
The length of s3 is 13 characters.
Q7. Explain storage class in C++.
In C++, storage classes are type specifiers that enable programmers to define the visibility and lifetime of variables and functions. When a program is running, C++ storage classes can be used to check whether a specific variable or function is present. They let us know where in a program a variable can be accessed.
Some storage classes in C++ are:
- Register
- Static
- Automatic
- Mutable
- External
Q8. Tell the name of the 4 pillars of OOPs in C++.
OOPs stands for object-oriented programming, and the four pillars of OOPs are-
- Inheritance
- Encapsulation
- Polymorphism
- Abstraction
Q9. Explain reference variables in C++.
A reference variable in C++ is a variable that points to another variable's address. In other words, the reference variable stands in for the name of another variable, location, or value and is produced using the ‘&’ operator. A reference cannot be changed to refer to a different object once it has been initialized. Different address values cannot be reassigned to reference variables in C++.
Syntax:
Data_type Variable_name =&existing_variable;
int x = 7;
int& ref = x;
Q10. What does the term polymorphism mean in C++?
In simple terms, polymorphism is when something has multiple forms, i.e., it morphs into many forms. In C++, polymorphism can be simply defined as the capability of a message to be displayed in more than one form. There are 2 types of polymorphism, that is, runtime polymorphism and compile time polymorphism.
Let's look at an example to better understand this. Take a woman who, at the same time, can be a mother, a daughter, or a wife. Now this woman is the same person but with different characteristics in different situations.
Q11. Define object and class in C++.
Object: An object is an instance of a class and an entity with value and state. It serves as a catalyst or a representation for a class member. The parameter list for an object could be varied or empty.
Class: This is an integral part of object-oriented programming. Class is a user-defined data type, and it consists of member functions and data members. Here, data members refer to the data variables in a class, and the procedures used to manipulate these variables can be identified as the member functions.
C++ Interview Questions: Intermediate
The questions in this question will further explore your foundational understanding of the language.
Q12. How many types of C++ access specifiers are there?
There are 3 types of access specifiers available in C++, which are-
- Public access specifier: It allows the members to be accessed from anywhere, including outside the class.
- Private specifier: It allows the members to be accessed only from within the class.
- Protected specifier: It allows the members to be accessed from within the class and from a derived class.
Q13. Discuss the difference between compile-time polymorphism and runtime polymorphism
Compile time polymorphism | Run-time polymorphism |
The execution of compile time polymorphism is fast because the execution is known at the compile time. | Rum time polymorphism has a comparatively slow execution rate because the execution is known at the run time. |
It is also known as static binding. | It is also known as dynamic binding. |
Operator and function overloading are used to achieve it. | Virtual functions and function overriding are used to achieve it. |
Q14. What do you mean by a virtual function?
A virtual function is a member function that is declared in a base class and redefined (overridden) by a derived class. It is possible to access a virtual function for an object of the derived class by invoking its version when you refer to this particular kind of object through a pointer or reference belonging to the base class.
Q15. What do you understand by inline function?
When a function is inline, the compiler inserts a duplicate of that function's code at each location where it is called during compilation. The elimination of function calling overhead compared to utilizing a standard function is one of the key benefits of employing an inline function, and they are faster than normal functions in C++.
Syntax:
inline data_type function_name()
{
Body;
}
Q16. Why do we use the delete operator in C++?
The 'delete' operator is generally used to deallocate one chunk of memory in C++ language. The pointer variable that is formed with the use of this delete operator can be deallocated by the user.
Syntax:
delete pointer_variable;
Q17. Tell me the difference between new and malloc().
Both the 'new' operator and the malloc() fn are used to allocate memory, but there are some key differences in how they do so.
New | Malloc() |
The new operator creates an object calling on the constructor, and there is no need to specify the memory size. | The malloc() function does not call the constructor, and memory size specification is necessary. |
This can be overloaded. | Malloc() function cannot be overloaded. |
New is an operator, so it is faster than malloc(). | Malloc() is slower than new because it is a function. |
The delete operator is used to deallocate the dynamic memory. | The free () function is used to deallocate the memory. |
Q18. What is abstraction in C++, explain in your own words.
Abstraction is the technique of only displaying information that is necessary to the user while suppressing information that is unnecessary or irrelevant to that user. There are two types of abstractions in C++, that is- data abstraction and control abstraction.
Q19. What is the difference between call by value and call by reference in C++?
Call by Value | Call by reference |
Call by value method is comparatively slower. | Call by reference method is comparatively faster. |
The value of the variable is passed as an argument in this method. | The address of the variable is passed as an argument in this method. |
The same memory location will not be used to create actual and formal arguments. | The same memory location is used to create actual and formal arguments. |
The copy of the value is passed to the function. | The address of the value is passed to the function. |
Q20. What distinguishes delete [] from delete?
Delete[] | Delete |
It is utilized to delete an entire array. | It is utilized to delete just one pointer. |
It is used to delete the new[] objects. | It is utilized for erasing newly created objects. |
Delete[] can call a destructor any number of times. | Delete can call the destructor only once. |
Q21. What is the difference between arrays and linked lists in C++
Arrays | Linked Lists |
An array in C++ is a group of identical data type objects that are kept in adjacent memory locations, and each may be accessed independently using the index. | Lists have a fixed size, and they are connected to the elements with the help of a pointer. |
The size of the arrays is fixed. | The size of lists is dynamic. |
In arrays, you can access the elements easily. | To access the elements in the list, you have to traverse the whole linked list. |
Arrays use less memory than a linked list. | The linked list uses more memory in comparison to arrays. |
C++ Interview Questions And Answers With Code Examples
Q22. What do you understand by operator overloading?
Operator overloading, in simple terms, is a polymorphism at compile time, which is a feature of object-oriented programming. The idea behind it is to provide an operator in C++ with a special meaning without altering its default meaning is the rationale behind this concept. Operational overloading is a feature of C++ that allows the operators to be given special meaning for a certain data type. We can overload the operator "+" in a class like "String" such that we can concatenate two strings with simply the letter "+." An example of the same is given ahead.
Example of operator overloading:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIENvbXBsZXggewoKcHJpdmF0ZToKCmludCByZWFsLCBpbWFnOwoKCgoKcHVibGljOgoKQ29tcGxleChpbnQgciA9IDAsIGludCBpID0gMCkgewoKcmVhbCA9IHI7CgppbWFnID0gaTsKCn0KCgoKCkNvbXBsZXggb3BlcmF0b3IrKENvbXBsZXggY29uc3QgJm9iaikgewoKQ29tcGxleCByZXM7CgpyZXMucmVhbCA9IHJlYWwgKyBvYmoucmVhbDsKCnJlcy5pbWFnID0gaW1hZyArIG9iai5pbWFnOwoKcmV0dXJuIHJlczsKCn0KCgoKCnZvaWQgcHJpbnQoKSB7Cgpjb3V0IDw8IHJlYWwgPDwgIiArIGkiIDw8IGltYWcgPDwgZW5kbDsKCn0KCn07CgoKCgppbnQgbWFpbigpIHsKCkNvbXBsZXggYzEoMywgNCksIGMyKDUsIDYpOwoKQ29tcGxleCBjMyA9IGMxICsgYzI7CgpjMy5wcmludCgpOwoKcmV0dXJuIDA7Cgp9
Output:
8 + i10
Q23. What is a constructor in C++?
A constructor is a special kind of member function in C++, and its name is the same as the class it belongs to. Upon execution, a class member function known as the constructor initializes an object within its respective class with predefined values. There are 3 types of constructors in C++, which are:
Default constructor: Once an object is created without any arguments, it is referred to as the default constructor. The compiler calls the default constructor automatically when an object is created.
Example :
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIE15Q2xhc3MgewoKcHVibGljOgoKaW50IHg7CgpzdHJpbmcgeTsKCgoKCk15Q2xhc3MoKSB7Cgp4ID0gMDsKCnkgPSAiZGVmYXVsdCI7Cgp9Cgp9OwoKCgoKaW50IG1haW4oKSB7CgpNeUNsYXNzIG9iajsKCmNvdXQgPDwgInggPSAiIDw8IG9iai54IDw8IGVuZGw7Cgpjb3V0IDw8ICJ5ID0gIiA8PCBvYmoueSA8PCBlbmRsOwoKcmV0dXJuIDA7Cgp9
Output:
y = default
Parameterized constructor: The parameterized constructor accepts arguments. And when an object is formed, these arguments assist in initializing it. One simply has to add parameters to it as you would to any other function to create a parameterized constructor. An example of the same is given below:
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIE15Q2xhc3MgewoKcHVibGljOgoKaW50IHg7CgpzdHJpbmcgeTsKCgoKCk15Q2xhc3MoaW50IGEsIHN0cmluZyBiKSB7Cgp4ID0gYTsKCnkgPSBiOwoKfQoKfTsKCgoKCmludCBtYWluKCkgewoKTXlDbGFzcyBvYmooMTAsICJoZWxsbyIpOwoKY291dCA8PCAieCA9ICIgPDwgb2JqLnggPDwgZW5kbDsKCmNvdXQgPDwgInkgPSAiIDw8IG9iai55IDw8IGVuZGw7CgpyZXR1cm4gMDsKCn0=
Output:
y = hello
Copy constructor: By employing a copy constructor member function, you can initialize an object using another within its own class. This involves duplicating all of the data from one instance and transferring it over to construct a new one that matches it.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIE15Q2xhc3MgewoKcHVibGljOgoKaW50IHg7CgpzdHJpbmcgeTsKCgoKCk15Q2xhc3MoaW50IGEsIHN0cmluZyBiKSB7Cgp4ID0gYTsKCnkgPSBiOwoKfQoKCgoKTXlDbGFzcyhjb25zdCBNeUNsYXNzJiBvYmopIHsKCnggPSBvYmoueDsKCnkgPSBvYmoueTsKCn0KCn07CgoKCgppbnQgbWFpbigpIHsKCk15Q2xhc3Mgb2JqMSgxMCwgImhlbGxvIik7CgpNeUNsYXNzIG9iajIgPSBvYmoxOwoKY291dCA8PCAieCA9ICIgPDwgb2JqMi54IDw8IGVuZGw7Cgpjb3V0IDw8ICJ5ID0gIiA8PCBvYmoyLnkgPDwgZW5kbDsKCnJldHVybiAwOwoKfQ==
Output:
y = hello
Q24. What is your understanding of the friend function and friend class in C++? Differentiate between them with the help of an example.
A friend class refers to the class that has been designated as a friend and can access the private and protected members of other classes. This class is declared with the friend keyword. The idea is that sometimes it is advantageous to give certain class access to the private and protected members of other classes.
Source Code:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIE15Q2xhc3MgewoKcHJpdmF0ZToKCmludCB4OwoKCgoKcHVibGljOgoKTXlDbGFzcyhpbnQgYSkgewoKeCA9IGE7Cgp9CgoKCgpmcmllbmQgY2xhc3MgRnJpZW5kQ2xhc3M7Cgp9OwoKCgoKY2xhc3MgRnJpZW5kQ2xhc3MgewoKcHVibGljOgoKdm9pZCBwcmludFgoTXlDbGFzcyBvYmopIHsKCmNvdXQgPDwgIlRoZSB2YWx1ZSBvZiB4IGlzOiAiIDw8IG9iai54IDw8IGVuZGw7Cgp9Cgp9OwoKCgoKaW50IG1haW4oKSB7CgpNeUNsYXNzIG9iaigxMCk7CgpGcmllbmRDbGFzcyBmYzsKCmZjLnByaW50WChvYmopOwoKcmV0dXJuIDA7Cgp9
Output:
The value of x is: 10
The friend function is used to access the private, protected, and public data of members or member functions of other classes. It has the keyword "friend" stated. The advantage of a friend function is that when it is declared in a class, it is not bound to the scope of the class, which means it can be called by other functions as well. In this sense, one can say that a friend function is a global function.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIE15Q2xhc3MgewoKcHJpdmF0ZToKCmludCB4OwoKCgoKcHVibGljOgoKTXlDbGFzcyhpbnQgYSkgewoKeCA9IGE7Cgp9CgoKCgpmcmllbmQgdm9pZCBwcmludFgoTXlDbGFzcyBvYmopOwoKfTsKCgoKCnZvaWQgcHJpbnRYKE15Q2xhc3Mgb2JqKSB7Cgpjb3V0IDw8ICJUaGUgdmFsdWUgb2YgeCBpczogIiA8PCBvYmoueCA8PCBlbmRsOwoKfQoKCgoKaW50IG1haW4oKSB7CgpNeUNsYXNzIG9iaigxMCk7CgpwcmludFgob2JqKTsKCnJldHVybiAwOwoKfQ==
Output:
The value of x is: 10
Q25. Explain virtual destructor in C++.
When an object from a child class or derived class is deleted from memory using the parent class pointer object, we need to free up the memory space it vacates. Here, the virtual destructor member function in C++ is used to free up the memory space allocated and consumed by the object, and it hence prevents memory leaks. Virtual destructor uses the ~ tilde operator and is declared with a virtual keyword.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIEJhc2UgewoKcHVibGljOgoKdmlydHVhbCB+QmFzZSgpIHsKCmNvdXQgPDwgIkJhc2UgZGVzdHJ1Y3RvciBjYWxsZWQiIDw8IGVuZGw7Cgp9Cgp9OwoKCgoKY2xhc3MgRGVyaXZlZCA6IHB1YmxpYyBCYXNlIHsKCnB1YmxpYzoKCn5EZXJpdmVkKCkgewoKY291dCA8PCAiRGVyaXZlZCBkZXN0cnVjdG9yIGNhbGxlZCIgPDwgZW5kbDsKCn0KCn07CgoKCgppbnQgbWFpbigpIHsKCkJhc2UqIGIgPSBuZXcgRGVyaXZlZCgpOwoKZGVsZXRlIGI7CgpyZXR1cm4gMDsKCn0=
Output:
Derived destructor called
Base destructor called
Q26. What do you understand by the static member and static member functions?
A class's static data member is a regular data member that is preceded by the term static. It runs in a program before main() and is initialized to 0 when the class's first object is created. It has a lifetime scope but is only visible to a specific class.
Syntax:
static Data_Type Data_Member;
Functions that can only access members of static data are known as static member functions in C++. With the various objects belonging to the same class, these static data members share a single copy of themselves. The static keyword should be used before the function name when defining a class to make a function static.
Syntax:
static returntype function_name()
The code snippet below contains an example of this concept-
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIEVtcGxveWVlIHsKCnByaXZhdGU6CgpzdHJpbmcgbmFtZTsKCmludCBhZ2U7CgpzdGF0aWMgaW50IGNvdW50OyAvLyBzdGF0aWMgZGF0YSBtZW1iZXIgZGVjbGFyYXRpb24KCgoKCnB1YmxpYzoKCkVtcGxveWVlKHN0cmluZyBuLCBpbnQgYSkgewoKbmFtZSA9IG47CgphZ2UgPSBhOwoKY291bnQrKzsgLy8gaW5jcmVtZW50IHRoZSBjb3VudCBvZiBvYmplY3RzCgp9CgoKCgp2b2lkIGRpc3BsYXkoKSB7Cgpjb3V0IDw8ICJOYW1lOiAiIDw8IG5hbWUgPDwgIiwgQWdlOiAiIDw8IGFnZSA8PCBlbmRsOwoKfQoKCgoKc3RhdGljIHZvaWQgZ2V0Q291bnQoKSB7IC8vIHN0YXRpYyBtZW1iZXIgZnVuY3Rpb24gZGVjbGFyYXRpb24KCmNvdXQgPDwgIlRvdGFsIGVtcGxveWVlczogIiA8PCBjb3VudCA8PCBlbmRsOwoKfQoKfTsKCgoKCmludCBFbXBsb3llZTo6Y291bnQgPSAwOyAvLyBpbml0aWFsaXplIHN0YXRpYyBkYXRhIG1lbWJlcgoKCgoKaW50IG1haW4oKSB7CgpFbXBsb3llZSBlMSgiSm9obiIsIDMwKTsKCkVtcGxveWVlIGUyKCJKYW5lIiwgMjUpOwoKRW1wbG95ZWUgZTMoIkFkYW0iLCAzNSk7CgoKCgplMS5kaXNwbGF5KCk7CgplMi5kaXNwbGF5KCk7CgplMy5kaXNwbGF5KCk7CgoKCgpFbXBsb3llZTo6Z2V0Q291bnQoKTsgLy8gY2FsbCB0aGUgc3RhdGljIG1lbWJlciBmdW5jdGlvbgoKCgoKcmV0dXJuIDA7Cgp9
Output:
Name: John, Age: 30
Name: Jane, Age: 25
Name: Adam, Age: 35
Total employees: 3
Q27. What do you understand by an abstract class?
In C++, an abstract class is one that contains at least one pure virtual function, i.e., a function without a definition. Note that abstract class objects cannot be made. In addition to serving as base classes, abstract classes also give derived classes a means to create an interface.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIFNoYXBlIHsKCnB1YmxpYzoKCnZpcnR1YWwgdm9pZCBkcmF3KCkgPSAwOwoKfTsKCgoKCmNsYXNzIENpcmNsZSA6IHB1YmxpYyBTaGFwZSB7CgpwdWJsaWM6Cgp2b2lkIGRyYXcoKSB7Cgpjb3V0IDw8ICJEcmF3aW5nIGEgY2lyY2xlIiA8PCBlbmRsOwoKfQoKfTsKCgoKCmNsYXNzIFJlY3RhbmdsZSA6IHB1YmxpYyBTaGFwZSB7CgpwdWJsaWM6Cgp2b2lkIGRyYXcoKSB7Cgpjb3V0IDw8ICJEcmF3aW5nIGEgcmVjdGFuZ2xlIiA8PCBlbmRsOwoKfQoKfTsKCgoKCmludCBtYWluKCkgewoKU2hhcGUqIHMxID0gbmV3IENpcmNsZSgpOwoKU2hhcGUqIHMyID0gbmV3IFJlY3RhbmdsZSgpOwoKczEtPmRyYXcoKTsKCnMyLT5kcmF3KCk7CgpyZXR1cm4gMDsKCn0=
Output:
Drawing a circle
Drawing a rectangle
Q28. Can you explain the destructors in C++?
A constructor is what is immediately called into action when an object is created. Similarly, when an object is destroyed, another function called 'destructor' comes into action. This destructor is preceded by the tilda (~) sign whenever it is invoked, and a similar name is shared with the class name and constructor.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIFBlcnNvbiB7Cgpwcml2YXRlOgoKc3RyaW5nIG5hbWU7CgppbnQgYWdlOwoKCgoKcHVibGljOgoKUGVyc29uKHN0cmluZyBuLCBpbnQgYSkgewoKbmFtZSA9IG47CgphZ2UgPSBhOwoKY291dCA8PCAiUGVyc29uIGNvbnN0cnVjdG9yIGNhbGxlZCBmb3IgIiA8PCBuYW1lIDw8IGVuZGw7Cgp9CgoKCgpQZXJzb24oKSB7Cgpjb3V0IDw8ICJEZWZhdWx0IFBlcnNvbiBjb25zdHJ1Y3RvciBjYWxsZWQiIDw8IGVuZGw7Cgp9CgoKCgp+UGVyc29uKCkgewoKY291dCA8PCAiUGVyc29uIGRlc3RydWN0b3IgY2FsbGVkIGZvciAiIDw8IG5hbWUgPDwgZW5kbDsKCn0KCn07CgoKCgppbnQgbWFpbigpIHsKClBlcnNvbiBwMSgiSm9obiIsIDMwKTsKClBlcnNvbiBwMjsKCnJldHVybiAwOwoKfQ==
Output:
Person constructor called for John
Default Person constructor called
Person destructor called for
Person destructor called for John
Q29. Can you tell the difference between assignment operator (=) and equal to operator(==)?
The assignment operator (=) is used to give a value to a variable, whereas the equal to operator (==) is used to determine whether two values are equal or not. Let's look at the code snippet below, which showcases this difference with the help of an example.
Sample code:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKaW50IG1haW4oKSB7CgppbnQgeCA9IDU7CgppbnQgeSA9IDEwOwoKYm9vbCByZXN1bHQ7CgpyZXN1bHQgPSAoeCA9PSB5KTsgLy8gZmFsc2UKCnN0ZDo6Y291dCA8PCAieCA9PSB5OiAiIDw8IHJlc3VsdCA8PCBzdGQ6OmVuZGw7CgpyZXN1bHQgPSAoeCA9IHkpOyAvLyAxMAoKc3RkOjpjb3V0IDw8ICJ4ID0geTogIiA8PCByZXN1bHQgPDwgc3RkOjplbmRsOwoKcmVzdWx0ID0gKHggPT0geSk7IC8vIHRydWUKCnN0ZDo6Y291dCA8PCAieCA9PSB5OiAiIDw8IHJlc3VsdCA8PCBzdGQ6OmVuZGw7CgpyZXR1cm4gMDsKCn0=
Output:
x == y: 0
x = y: 1
x == y: 1
Q30. Distinguish between shallow copy and deep copy.
In programming, object copying is the act of copying an object. The main purpose of creating a copy is to move the copied object without affecting the original. There are two types of copying- shallow and deep. The difference between the two are-
Shallow copy | Deep copy |
As the name suggests, this entails copying the object by copying data for its member variable only. | This entails copying the data for members contained in the object, as well as any other object that may be handled by the initial object. |
Shallow copy is always faster than deep copy. | Deep copy is slower as compared to shallow copy. |
References of objects are stored in shallow copies with their original memory addresses | Deep copy creates a fresh, independent copy of an entire object. |
The original object modifications to the new/copied item are reflected in the shallow copy. | Deep copies don't update the original object to reflect changes made to the new or duplicated object. |
Q31. Explain the term encapsulation in the context of C++.
Encapsulation is an object-oriented programming concept that unites the data and the functions that alter the data and protects both from outside influence and misuse. Data encapsulation gave rise to the crucial OOP idea of data hiding.
The block of code given below shows an implementation of encapsulation.
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIFBlcnNvbiB7Cgpwcml2YXRlOgoKc3RyaW5nIG5hbWU7CgppbnQgYWdlOwoKCgoKcHVibGljOgoKdm9pZCBzZXROYW1lKHN0cmluZyBuKSB7CgpuYW1lID0gbjsKCn0KCgoKCnZvaWQgc2V0QWdlKGludCBhKSB7CgphZ2UgPSBhOwoKfQoKCgoKc3RyaW5nIGdldE5hbWUoKSB7CgpyZXR1cm4gbmFtZTsKCn0KCgoKCmludCBnZXRBZ2UoKSB7CgpyZXR1cm4gYWdlOwoKfQoKfTsKCgoKCmludCBtYWluKCkgewoKUGVyc29uIHAxOwoKcDEuc2V0TmFtZSgiSm9obiBEb2UiKTsKCnAxLnNldEFnZSgzMCk7Cgpjb3V0IDw8ICJOYW1lOiAiIDw8IHAxLmdldE5hbWUoKSA8PCBlbmRsOwoKY291dCA8PCAiQWdlOiAiIDw8IHAxLmdldEFnZSgpIDw8IGVuZGw7CgpyZXR1cm4gMDsKCn0=
Output:
Name: John Doe
Age: 30
Q32. What do you mean by inheritance in C++?
The process of deriving new classes from pre-existing classes is known as inheritance. Base classes are referred to as pre-existing classes. Although the derived classes can add new features and enhancements of their own, they inherit all the abilities of the base class. There are mainly 5 types of inheritances, that is, single, multiple, multilevel, hierarchical, and hybrid inheritances.
An example showing the implementation of inheritance in C++ is:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCgoKCmNsYXNzIFNoYXBlIHsKCnByb3RlY3RlZDoKCmludCB3aWR0aDsKCmludCBoZWlnaHQ7CgoKCgpwdWJsaWM6Cgp2b2lkIHNldFdpZHRoKGludCB3KSB7Cgp3aWR0aCA9IHc7Cgp9CgoKCgp2b2lkIHNldEhlaWdodChpbnQgaCkgewoKaGVpZ2h0ID0gaDsKCn0KCn07CgoKCgpjbGFzcyBSZWN0YW5nbGUgOiBwdWJsaWMgU2hhcGUgewoKcHVibGljOgoKaW50IGdldEFyZWEoKSB7CgpyZXR1cm4gKHdpZHRoICogaGVpZ2h0KTsKCn0KCn07CgoKCgppbnQgbWFpbigpIHsKClJlY3RhbmdsZSByZWN0OwoKcmVjdC5zZXRXaWR0aCg1KTsKCnJlY3Quc2V0SGVpZ2h0KDcpOwoKY291dCA8PCAiQXJlYSBvZiB0aGUgcmVjdGFuZ2xlIGlzICIgPDwgcmVjdC5nZXRBcmVhKCkgPDwgZW5kbDsKCnJldHVybiAwOwoKfQ==
Output:
Area of the rectangle is 35
Q33. Can we use a constructor to call a virtual function?
Yes, technically, we can use a constructor to call a virtual function. However, one must avoid doing this because it is only possible to call a virtual function from the base class and not a derived class.
C++ Interview Questions and Answers: Advanced
Q34. Explain the concept of exception handling in C++.
A program can manage anomalies or abnormal conditions that arise during runtime using the exception handling mechanism in C++. Exceptions are used to transfer control from one section of the program to another and to signal an error or unanticipated event. 'Try', 'Catch', and 'Throw' are the three keywords that make up the exception-handling system in C++. An example implementation program is given below
Source code:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKaW50IG1haW4oKSB7Cgp0cnkgewoKaW50IHggPSAxMDsKCmludCB5ID0gMDsKCmlmICh5ID09IDApIHsKCnRocm93ICJEaXZpc2lvbiBieSB6ZXJvIjsKCn0KCmludCB6ID0geCAvIHk7CgpzdGQ6OmNvdXQgPDwgInogPSAiIDw8IHogPDwgc3RkOjplbmRsOwoKfQoKY2F0Y2ggKGNvbnN0IGNoYXIqIG1zZykgewoKc3RkOjpjZXJyIDw8ICJFcnJvcjogIiA8PCBtc2cgPDwgc3RkOjplbmRsOwoKfQoKcmV0dXJuIDA7Cgp9
Output:
Error: Division by zero
Q35. Explain the term upcasting in C++.
Upcasting in C++ is the process of changing a pointer or reference from a derived class to the base class. Through the use of its common interface, upcasting enables one to treat a derived class as a base class. This cast is secure and doesn't need a cast notation explicitly. In other words, using the derived class object reference or pointer to refer to the base class object is known as upcasting. It is helpful when passing a derived class object to a function that anticipates a base class object.
Sample code:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgQW5pbWFsIHsKCnB1YmxpYzoKCnZpcnR1YWwgdm9pZCBtYWtlU291bmQoKSB7CgpzdGQ6OmNvdXQgPDwgIkFuaW1hbCBzb3VuZCIgPDwgc3RkOjplbmRsOwoKfQoKfTsKCgoKCmNsYXNzIERvZyA6IHB1YmxpYyBBbmltYWwgewoKcHVibGljOgoKdm9pZCBtYWtlU291bmQoKSB7CgpzdGQ6OmNvdXQgPDwgIldvb2YhIiA8PCBzdGQ6OmVuZGw7Cgp9Cgp9OwoKCgoKaW50IG1haW4oKSB7CgpEb2cgZG9nOwoKQW5pbWFsJiBhbmltYWwgPSBkb2c7IC8vIHVwY2FzdGluZwoKYW5pbWFsLm1ha2VTb3VuZCgpOyAvLyBjYWxscyBEb2c6Om1ha2VTb3VuZCgpCgpyZXR1cm4gMDsKCn0=
Output:
Woof!
Q36. What is the void function in C++?
The C++ term void is utilized to indicate that a function does not yield any results. If the return type of a function has been designated as void, then it clarifies that said function will not return anything, even without expecting a return value from invoking the respective call on this particular function, and the normal code execution continues.
Sample code:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKCgoKdm9pZCBwcmludE1lc3NhZ2Uoc3RkOjpzdHJpbmcgbWVzc2FnZSkgewoKc3RkOjpjb3V0IDw8IG1lc3NhZ2UgPDwgc3RkOjplbmRsOwoKfQoKCgoKaW50IG1haW4oKSB7CgpwcmludE1lc3NhZ2UoIkhlbGxvISIpOwoKcmV0dXJuIDA7Cgp9
Output:
Hello
Q37. Explain the scope of the resolution operator in C++.
The scope of the resolution operator is represented by (::) in C++. It is used to access out-of-scope global variables or member functions. To distinguish between the same variable name in several scopes, we use the scope resolution operator, which is related to the function definition of a specific class.
Implementation of scope resolution operator
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCB2YWwgPSAyMDsKCm5hbWVzcGFjZSBmaXJzdCB7CgppbnQgdmFsID0gNjA7Cgp9CgppbnQgbWFpbigpIHsKCmludCB2YWwgPSAyOyAvLyBWYWx1ZSBvZiB0aGUgdmFyaWFibGUgdmFsIGluIHRoZSBsb2NhbCBzY29wZS4KCmNvdXQgPDwgIlZhbHVlIG9mIHZhbCBpbiBsb2NhbCBzY29wZTogIiA8PCB2YWwgPDwgIlxuIjsKCmNvdXQgPDwgIlZhbHVlIG9mIHZhbCBpbiBnbG9iYWwgc2NvcGU6ICIgPDwgOjp2YWwgPDwgIlxuIjsKCmNvdXQgPDwgIlZhbHVlIG9mIHZhbCBpbiBmaXJzdCBuYW1lc3BhY2U6ICIgPDwgZmlyc3Q6OnZhbCA8PCAiXG4iOwoKcmV0dXJuIDA7Cgp9
Output:
Value of val in local scope: 2
Value of val in global scope: 20
Value of val in first namespace: 60
Q38. How does C++ handle memory allocation and deallocation?
In C++, memory can be allocated using either the malloc() function or the new operator, which allocates memory on the heap. The free() function or the delete operator can be used to deallocate memory. Both of these operations release memory that was previously allocated with the new operator.
Source code:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKaW50IG1haW4oKSB7CgppbnQqIHB0ciA9IG5ldyBpbnQ7IC8vIGFsbG9jYXRlIG1lbW9yeSBvbiB0aGUgaGVhcAoKKnB0ciA9IDEwOyAvLyBhc3NpZ24gYSB2YWx1ZSB0byB0aGUgbWVtb3J5CgpzdGQ6OmNvdXQgPDwgIlZhbHVlIG9mIHB0cjogIiA8PCAqcHRyIDw8IHN0ZDo6ZW5kbDsKCmRlbGV0ZSBwdHI7IC8vIGZyZWUgbWVtb3J5IGFsbG9jYXRlZCB1c2luZyBuZXcKCmludCogYXJyID0gbmV3IGludFs1XTsgLy8gYWxsb2NhdGUgYW4gYXJyYXkgb2YgaW50ZWdlcnMgb24gdGhlIGhlYXAKCmZvciAoaW50IGkgPSAwOyBpIDwgNTsgaSsrKSB7CgphcnJbaV0gPSBpOwoKfQoKc3RkOjpjb3V0IDw8ICJWYWx1ZXMgb2YgYXJyOiAiOwoKZm9yIChpbnQgaSA9IDA7IGkgPCA1OyBpKyspIHsKCnN0ZDo6Y291dCA8PCBhcnJbaV0gPDwgIiAiOwoKfQoKc3RkOjpjb3V0IDw8IHN0ZDo6ZW5kbDsKCmRlbGV0ZVtdIGFycjsgLy8gZnJlZSBtZW1vcnkgYWxsb2NhdGVkIHVzaW5nIG5ld1tdCgpyZXR1cm4gMDsKCn0=
Output:
Value of ptr: 10
Values of arr: 0 1 2 3 4
Q39. Explain “this” pointer in C++.
In C++, every object has access to its own address via a crucial pointer known as this pointer. All member functions take the 'this pointer' as an implicit parameter. This pointer holds the memory address of the current variable. Consequently, this may be used to refer to invoking an object inside a member function.
Also note that friends are not members of a class, which is why friend functions do not have a 'this pointer'. So the 'this pointers' are exclusive to member functions.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmNsYXNzIFJlY3RhbmdsZSB7Cgpwcml2YXRlOgoKaW50IGxlbmd0aDsKCmludCB3aWR0aDsKCnB1YmxpYzoKClJlY3RhbmdsZShpbnQgbGVuZ3RoLCBpbnQgd2lkdGgpIHsKCnRoaXMtPmxlbmd0aCA9IGxlbmd0aDsKCnRoaXMtPndpZHRoID0gd2lkdGg7Cgp9CgppbnQgZ2V0TGVuZ3RoKCkgewoKcmV0dXJuIHRoaXMtPmxlbmd0aDsKCn0KCmludCBnZXRXaWR0aCgpIHsKCnJldHVybiB0aGlzLT53aWR0aDsKCn0KClJlY3RhbmdsZSYgc2V0TGVuZ3RoKGludCBsZW5ndGgpIHsKCnRoaXMtPmxlbmd0aCA9IGxlbmd0aDsKCnJldHVybiAqdGhpczsKCn0KClJlY3RhbmdsZSYgc2V0V2lkdGgoaW50IHdpZHRoKSB7Cgp0aGlzLT53aWR0aCA9IHdpZHRoOwoKcmV0dXJuICp0aGlzOwoKfQoKfTsKCmludCBtYWluKCkgewoKUmVjdGFuZ2xlIHIoNSwgMTApOwoKY291dCA8PCAiTGVuZ3RoOiAiIDw8IHIuZ2V0TGVuZ3RoKCkgPDwgZW5kbDsKCmNvdXQgPDwgIldpZHRoOiAiIDw8IHIuZ2V0V2lkdGgoKSA8PCBlbmRsOwoKci5zZXRMZW5ndGgoNykuc2V0V2lkdGgoMTIpOwoKY291dCA8PCAiTGVuZ3RoOiAiIDw8IHIuZ2V0TGVuZ3RoKCkgPDwgZW5kbDsKCmNvdXQgPDwgIldpZHRoOiAiIDw8IHIuZ2V0V2lkdGgoKSA8PCBlbmRsOwoKcmV0dXJuIDA7Cgp9
Output:
Length: 5
Width: 10
Length: 7
Width: 12
Q40. What do you understand by void pointers in C++?
A pointer with no related data type is referred to as a void pointer. Any type of address can be stored in.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCkgewoKdm9pZCogcHRyOwoKaW50IGkgPSA1OwoKZmxvYXQgZiA9IDMuMTRmOwoKcHRyID0gJmk7Cgpjb3V0IDw8ICJWYWx1ZSBvZiBpOiAiIDw8ICooaW50KilwdHIgPDwgZW5kbDsKCnB0ciA9ICZmOwoKY291dCA8PCAiVmFsdWUgb2YgZjogIiA8PCAqKGZsb2F0KilwdHIgPDwgZW5kbDsKCnJldHVybiAwOwoKfQ==
Output:
Value of i: 5
Value of f: 3.14
Q41. Is a virtual constructor possible in C++?
It is not possible to have virtual constructors in C++ since there isn't a virtual table in memory when the class's constructor is called. This means there isn't a virtual pointer yet. As a result, the constructor can never be virtual.
Q42. What do you understand by method overriding?
A function is said to be overridden if we inherit a class into a derived class and include a description for one of the base class's functions once more inside the derived class. This mechanism is known as function overriding.
The code block below gives an implementation of the overriding method-
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgQW5pbWFsIHsKcHVibGljOgp2aXJ0dWFsIHZvaWQgZWF0KCkgewpzdGQ6OmNvdXQgPDwgIkVhdGluZyBmb29kIiA8PCBzdGQ6OmVuZGw7Cn0KfTsKCmNsYXNzIERvZyA6IHB1YmxpYyBBbmltYWwgewpwdWJsaWM6CnZvaWQgZWF0KCkgewpzdGQ6OmNvdXQgPDwgIkVhdGluZyBib25lcyIgPDwgc3RkOjplbmRsOwp9Cn07CgppbnQgbWFpbigpIHsKQW5pbWFsKiBhbmltYWwxID0gbmV3IEFuaW1hbCgpOwpBbmltYWwqIGFuaW1hbDIgPSBuZXcgRG9nKCk7CgphbmltYWwxLT5lYXQoKTsKYW5pbWFsMi0+ZWF0KCk7CgpkZWxldGUgYW5pbWFsMTsKZGVsZXRlIGFuaW1hbDI7CgpyZXR1cm4gMDsKfQ==
Output:
Eating food
Eating bones
Q43. What do you understand by the auto keyword in C++?
The auto keyword in C++ allows the compiler to determine a variable's type based on its initializer automatically.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKaW50IG1haW4oKSB7CmF1dG8gaSA9IDQyOwphdXRvIGQgPSAzLjE0MTU5OwphdXRvIHMgPSAiSGVsbG8sIHdvcmxkISI7CgpzdGQ6OmNvdXQgPDwgImkgPSAiIDw8IGkgPDwgc3RkOjplbmRsOyAvLyBPdXRwdXQ6ICJpID0gNDIiCnN0ZDo6Y291dCA8PCAiZCA9ICIgPDwgZCA8PCBzdGQ6OmVuZGw7IC8vIE91dHB1dDogImQgPSAzLjE0MTU5IgpzdGQ6OmNvdXQgPDwgInMgPSAiIDw8IHMgPDwgc3RkOjplbmRsOyAvLyBPdXRwdXQ6ICJzID0gSGVsbG8sIHdvcmxkISIKCnJldHVybiAwOwp9
Output:
i = 42
d = 3.14159
s = Hello, world!
Q44. What do you understand by static variable?
In C++, a static variable refers to one that has static storage duration meaning it will exist throughout the time the program is running. Static variables are allocated only once and remain in the memory for the duration of the program.
Example of static variable:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdm9pZCBmb28oKSB7CnN0YXRpYyBpbnQgY291bnQgPSAwOwpjb3VudCsrOwpzdGQ6OmNvdXQgPDwgImZvbygpIGhhcyBiZWVuIGNhbGxlZCAiIDw8IGNvdW50IDw8ICIgdGltZXMuIiA8PCBzdGQ6OmVuZGw7Cn0KCmludCBtYWluKCkgewpmb28oKTsKZm9vKCk7CmZvbygpOwpyZXR1cm4gMDsKfQ==
Output:
foo() has been called 1 time.
foo() has been called 2 times.
foo() has been called 3 times.
Q45. Tell me the difference between late binding and early binding.
Early binding occurs when the compiler selects a function declaration from a large pool of functions with various parameters. As the name implies, binding occurs early in the program's execution. An example for this is given by the code block below:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdm9pZCBkaXNwbGF5KGludCB4KSB7CnN0ZDo6Y291dCA8PCB4IDw8IHN0ZDo6ZW5kbDsKfQoKaW50IG1haW4oKSB7CmRpc3BsYXkoNSk7CnJldHVybiAwOwp9
Output:
5
Dynamic binding is also known as runtime polymorphism, and it involves the addition of code by the compiler at the run time. It then matches the call to the function. The virtual function helps in achieving this.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgU2hhcGUgewpwdWJsaWM6CnZpcnR1YWwgdm9pZCBkcmF3KCkgewpzdGQ6OmNvdXQgPDwgIkRyYXdpbmcgYSBzaGFwZS4iIDw8IHN0ZDo6ZW5kbDsKfQp9OwoKY2xhc3MgQ2lyY2xlIDogcHVibGljIFNoYXBlIHsKcHVibGljOgp2b2lkIGRyYXcoKSB7CnN0ZDo6Y291dCA8PCAiRHJhd2luZyBhIGNpcmNsZS4iIDw8IHN0ZDo6ZW5kbDsKfQp9OwoKaW50IG1haW4oKSB7ClNoYXBlKiBzaGFwZSA9IG5ldyBDaXJjbGUoKTsKc2hhcGUtPmRyYXcoKTsKZGVsZXRlIHNoYXBlOwpyZXR1cm4gMDsKfQ==
Output:
Drawing a circle.
Q46. What is a volatile keyword in C++? Give a code example for the same.
The volatile keyword is a type qualifier used to indicate that an object within a program may be altered by the operating system, hardware, or another thread that is currently running.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKaW50IG1haW4oKSB7CmludCB4ID0gNTsKdm9sYXRpbGUgaW50IHkgPSAxMDsKCnN0ZDo6Y291dCA8PCAieCA9ICIgPDwgeCA8PCBzdGQ6OmVuZGw7CnN0ZDo6Y291dCA8PCAieSA9ICIgPDwgeSA8PCBzdGQ6OmVuZGw7Cgp4ID0gNzsKeSA9IDE1OwoKc3RkOjpjb3V0IDw8ICJ4ID0gIiA8PCB4IDw8IHN0ZDo6ZW5kbDsKc3RkOjpjb3V0IDw8ICJ5ID0gIiA8PCB5IDw8IHN0ZDo6ZW5kbDsKCnJldHVybiAwOwp9
Output:
x = 5
y = 10
x = 7
y = 15
Q47. Tell the difference between method overloading and method overriding.
Method overloading |
Method overriding |
Method overloading happens at compile time. |
Method overriding happens at the run time. |
No keyword is used in the main class. |
Virtual keyword is used in the main() class. |
It is also known as early binding. |
It is also known as late binding. |
In method overloading, multiple functions can be defined with the same name but different numbers and parameters. |
In method overriding, we can express a function with the same return type and parameters in both the parent class and the child class. |
Q48. What do you understand by the term conversion constructor? How will you implement this in a code?
A conversion constructor is one that can be called with a single parameter but is not explicitly specified as a function that defines a conversion from the type of its first parameter to the type of its class.
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgTXlJbnQgewpwdWJsaWM6Ck15SW50KGRvdWJsZSBkKSB7CnZhbHVlID0gc3RhdGljX2Nhc3Q8aW50PihkKTsKfQoKaW50IGdldFZhbHVlKCkgewpyZXR1cm4gdmFsdWU7Cn0KCnByaXZhdGU6CmludCB2YWx1ZTsKfTsKCmludCBtYWluKCkgewpNeUludCBteUludCA9IDMuMTQ7CnN0ZDo6Y291dCA8PCAiVmFsdWUgb2YgbXlJbnQ6ICIgPDwgbXlJbnQuZ2V0VmFsdWUoKSA8PCBzdGQ6OmVuZGw7CnJldHVybiAwOwp9
Output:
Value of myInt: 3
Q49. What does an array of objects in C++ mean?
An array of objects in C++ is a group of identical objects that are kept in the same contiguous memory locations.
An example of an array of objects is:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgUmVjdGFuZ2xlIHsKcHVibGljOgppbnQgd2lkdGg7CmludCBoZWlnaHQ7CgppbnQgYXJlYSgpIHsKcmV0dXJuIHdpZHRoICogaGVpZ2h0Owp9Cn07CgppbnQgbWFpbigpIHsKUmVjdGFuZ2xlIHJlY3RhbmdsZXNbMl07CgpyZWN0YW5nbGVzWzBdLndpZHRoID0gNTsKcmVjdGFuZ2xlc1swXS5oZWlnaHQgPSAxMDsKCnJlY3RhbmdsZXNbMV0ud2lkdGggPSAzOwpyZWN0YW5nbGVzWzFdLmhlaWdodCA9IDc7CgovLyBsb29wIG92ZXIgdGhlIHZhbGlkIGVsZW1lbnRzIGluIHRoZSBhcnJheQpmb3IgKGludCBpID0gMDsgaSA8IDI7IGkrKykgewpzdGQ6OmNvdXQgPDwgIlJlY3RhbmdsZSAiIDw8IGkgKyAxIDw8ICIgYXJlYTogIiA8PCByZWN0YW5nbGVzW2ldLmFyZWEoKSA8PCBzdGQ6OmVuZGw7Cn0KCnJldHVybiAwOwp9
Output:
Rectangle 1 area: 50
Rectangle 2 area: 21
Q50. Can you explain the term mutable storage class specifier in C++?
A mutable storage class specifier helps in editing one or more data members through the const functions when you don't want the function to update the other members of the class or struct. The mutable keyword simplifies this task.
Example of a program using a mutable storage class specifier:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgTXlDbGFzcyB7CnB1YmxpYzoKaW50IHg7Cm11dGFibGUgaW50IHk7Cgp2b2lkIHByaW50KCkgY29uc3QgewpzdGQ6OmNvdXQgPDwgInggPSAiIDw8IHggPDwgc3RkOjplbmRsOwpzdGQ6OmNvdXQgPDwgInkgPSAiIDw8IHkgPDwgc3RkOjplbmRsOwoKLy8gQXR0ZW1wdCB0byBtb2RpZnkgeCBhbmQgeQovLyBUaGlzIHdpbGwgcmVzdWx0IGluIGEgY29tcGlsZXIgZXJyb3IKLy8geCA9IDU7Ci8vIHkgPSAxMDsKfQp9OwoKaW50IG1haW4oKSB7CmNvbnN0IE15Q2xhc3MgbXlPYmogPSB7MywgN307Cm15T2JqLnByaW50KCk7CgpyZXR1cm4gMDsKfQ==
Output:
x = 3
y = 7
Q51. Explain how is a temporary object generated.
A temporary object in C++ is an unnamed object that the compiler creates to hold a temporary value. A C++ program's execution frequently results in the creation of temporary objects. They are always created as a result of return-by-value functions and C++ operators like (unary, binary, logical, etc.).
Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgojaW5jbHVkZSA8c3RyaW5nPgoKc3RkOjpzdHJpbmcgY29uY2F0ZW5hdGUoc3RkOjpzdHJpbmcgc3RyMSwgc3RkOjpzdHJpbmcgc3RyMikgewpyZXR1cm4gc3RyMSArIHN0cjI7Cn0KCmludCBtYWluKCkgewpzdGQ6OnN0cmluZyBuYW1lID0gIkpvaG4iOwpzdGQ6OnN0cmluZyBncmVldGluZyA9ICJIZWxsbywgIiArIG5hbWU7IC8vIHRlbXBvcmFyeSBvYmplY3QgY3JlYXRlZCBoZXJlCnN0ZDo6c3RyaW5nIG1lc3NhZ2UgPSBjb25jYXRlbmF0ZShncmVldGluZywgIiEiKTsgLy8gdGVtcG9yYXJ5IG9iamVjdCBjcmVhdGVkIGhlcmUKc3RkOjpjb3V0IDw8IG1lc3NhZ2UgPDwgc3RkOjplbmRsOwpyZXR1cm4gMDsKfQ==
Output:
Hello, John!
Q52. What do you understand by internal iterators in C++?
An iterator in C++ is a term for an object that points to an element inside a container that allows access to the element it points to. It also allows movement across the container's content. A member function of the class whose elements need to be iterated is executed through an internal iterator. Internal and external iterators are the two different categories of iterators.
Q53. Explain the term virtual member function.
A member function that is declared in a base class and redefined (overridden) by a derived class is known as a virtual member function. You can call a virtual function for an object of a derived class and run the derived class's version using a pointer or a reference to the base class. The primary purpose of a virtual member function is to implement runtime polymorphism. In the base class, functions are declared using the virtual keyword.
This brings us to the end of our question bank on C++ interview question. You might also be interested in reading the following:
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
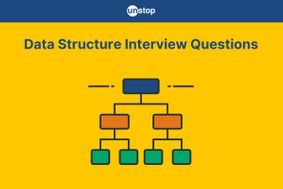
55+ Data Structure Interview Questions For 2025 (Detailed Answers)
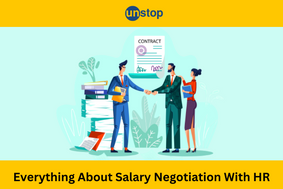
How To Negotiate Salary With HR: Tips And Insider Advice
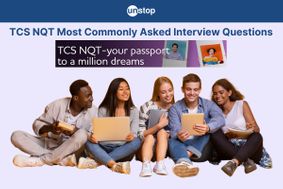
Best 80+ TCS NQT Most Commonly Asked Interview Questions for 2025
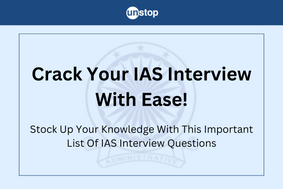
Comments
Add comment