Company Interview Questions for Freshers
Table of content:
- List of TCS Interview Questions - Technical
- TCS HR Interview Questions
Table of content:
- Overview of Cognizant Recruitment Process
- Cognizant Interview Questions: Technical
- Cognizant Interview Questions: HR Round
Table of content:
- Overview of Wipro Technologies Recruitment Rounds
- Wipro Interview Questions
- Technical Round
- HR Round
- Online Assessment Sample Questions
- Frequently Asked Questions
Table of content:
- Overview of Google Recruitment Process
- Google Interview Questions: Technical
- Google Interview Questions: HR round
- Interview Preparation Tips
- About Google
Table of content:
- Deloitte Technical Interview Questions
- Deloitte HR Interview Questions
- Deloitte Recruitment Process
Table of content:
- Technical Interview Questions and Answers
- Level 1 difficulty
- Level 2 difficulty
- Level 3 difficulty
- Behavioral Questions
Table of content:
- Eligibility Criteria for Mindtree Recruitment
- Mindtree Recruitment Process: Rounds Overview
- Skills required to crack Mindtree interview rounds
- Mindtree Recruitment Rounds: Sample Questions
- About Mindtree
Table of content:
- Preparing for Microsoft interview questions
- Microsoft technical interview questions
- Microsoft behavioural interview questions
Table of content:
- Aptitude Interview Questions
- Technical Interview Questions
- Easy
- Intermediate
- Hard
- HR Interview Questions
Table of content:
- Eligibility criteria
- Recruitment rounds & assessments
- Tech Mahindra interview questions - Technical round
- Tech Mahindra interview questions - HR round
Table of content:
- Hiring process at Mphasis
- Mphasis technical interview questions
- Mphasis HR interview questions
- About Mphasis
Table of content:
- Technical interview questions
- HR interview questions
- Recruitment process
- About Virtusa
Table of content:
- Goldman Sachs Interview Process
- Technical Questions for Goldman Sachs Interview
- Sample HR Question for Goldman Sachs Interview
- About Goldman Sachs
Table of content:
- Nagarro Recruitment Process
- Nagarro HR Interview Questions
- Nagarro Aptitude Test Questions
- Nagarro Technical Test Questions
- About Nagarro
Table of content:
- PwC Recruitment Process
- PwC Technical Interview Questions: Freshers and Experienced
- PwC Interview Questions for HR Rounds
- PwC Interview Preparation
- Frequently Asked Questions
Table of content:
- EY Technical Interview Questions (2023)
- EY Interview Questions for HR Round
- About EY
Table of content:
- Morgan Stanley Recruitment Process
- HR Questions for Morgan Stanley Interview
- HR Questions for Morgan Stanley Interview
- About Morgan Stanley
Table of content:
- Recruitment Process at Flipkart
- Technical Flipkart Interview Questions
- Code-Based Flipkart Interview Questions
- Sample Flipkart Interview Questions- HR Round
- Conclusion
- FAQs
Table of content:
- Recruitment Process at Paytm
- Technical Interview Questions for Paytm Interview
- HR Sample Questions for Paytm Interview
- About Paytm
Table of content:
- Most Probable Accenture Interview Questions
- Accenture Technical Interview Questions
- Accenture HR Interview Questions
Table of content:
- Amazon Recruitment Process
- Amazon Interview Rounds
- Common Amazon Interview Questions
- Amazon Interview Questions: Behavioral-based Questions
- Amazon Interview Questions: Leadership Principles
- Company-specific Amazon Interview Questions
- 43 Top Technical/ Coding Amazon Interview Questions
Table of content:
- Juspay Recruitment: Stages and Timeline
- Juspay Interview Questions and Answers
- How to prepare for Juspay interview questions
- Prepare for the Juspay Interview: Stages and Timeline
- Frequently Asked Questions
Table of content:
- Adobe Interview Questions - Technical
- Adobe Interview Questions - HR
- Recruitment Process at Adobe
- About Adobe
Table of content:
- Cisco technical interview questions
- Sample HR interview questions
- The recruitment process at Cisco
- About Cisco
Table of content:
- JP Morgan interview questions (Technical round)
- JP Morgan interview questions HR round)
- Recruitment process at JP Morgan
- About JP Morgan
Table of content:
- Wipro Elite NTH: Selection Process
- Wipro Elite NTH Technical Interview Questions
- Wipro Elite NTH Interview Round- HR Questions
Table of content:
- BYJU's BDA Interview Questions
- BYJU's SDE Interview Questions
- BYJU's HR Round Interview Questions
Table of content:
- A Quick Overview of the KPMG Recruitment Process
- Technical Questions for KPMG Interview
- HR Questions for KPMG Interview
- About KPMG
Table of content:
- DXC Technology Interview Process
- DCX Technical Interview Questions
- Sample HR Questions for DXC Technology
- About DXC Technology
Table of content:
- Recruitment Process at PayPal
- Technical Questions for PayPal Interview
- HR Sample Questions for PayPal Interview
- About PayPal
Table of content:
- Capgemini Recruitment Rounds
- Capgemini Interview Questions: Technical round
- Capgemini Interview Questions: HR round
- Preparation tips
- FAQs
Table of content:
- Technical interview questions for Siemens
- Sample HR questions for Siemens
- The recruitment process at Siemens
- About Siemens
Table of content:
- HCL Technical Interview Questions
- HR Interview Questions
- HCL Technologies Recruitment Process
Table of content:
- List of EPAM Interview Questions for Technical Interviews
- About EPAM
Table of content:
- Atlassian Interview Process
- Top Skills for Different Roles at Atlassian
- Atlassian Interview Questions: Technical Knowledge
- Atlassian Interview Questions: Behavioral Skills
- Atlassian Interview Questions: Tips for Effective Preparation
Table of content:
- Walmart Recruitment Process
- Walmart Interview Questions and Sample Answers (HR Round)
- Walmart Interview Questions and Sample Answers (Technical Round)
- Tips for Interviewing at Walmart and Interview Preparation Tips
- Frequently Asked Questions
Table of content:
- Uber Interview Questions For Engineering Profiles: Coding
- Technical Uber Interview Questions: Theoretical
- Uber Interview Question: HR Round
- Uber Recruitment Procedure
- About Uber Technologies Ltd.
Table of content:
- Intel Technical Interview Questions
- Computer Architecture Intel Interview Questions
- Intel DFT Interview Questions
- Intel Interview Questions for Verification Engineer Role
Table of content:
- Recruitment Process Overview
- Important Accenture HR Interview Questions
- Points to remember
Table of content:
- What is Selenium?
- What are the components of the Selenium suite?
- Why is it important to use Selenium?
- What's the major difference between Selenium 3.0 & Selenium 2.0?
- What is Automation testing and what are its benefits?
- What are the benefits of Selenium as an Automation Tool?
- What are the drawbacks to using Selenium for testing?
- Why should Selenium not be used as a web application or system testing tool?
- Is it possible to use selenium to launch web browsers?
- What does Selenese mean?
- What does it mean to be a locator?
- Identify the main difference between "assert", and "verify" commands within Selenium
- What does an exception test in Selenium mean?
- What does XPath mean in Selenium? Describe XPath Absolute & XPath Relation
- What is the difference in Xpath between "//"? and "/"?
- What is the difference between "type" and the "typeAndWait" commands within Selenium?
- Distinguish between findElement() & findElements() in context of Selenium
- How long will Selenium wait before a website is loaded fully?
- What is the difference between the driver.close() and driver.quit() commands in Selenium?
- Describe the different navigation commands that Selenium supports
- What is Selenium's approach to the same-origin policy?
- Explain the difference between findElement() in Selenium and findElements()
- Explain the pause function in SeleniumIDE
- Explain the differences between different frameworks and how they are connected to Selenium's Robot Framework
- What are your thoughts on the Page Object Model within the context of Selenium
- What are your thoughts on Jenkins?
- What are the parameters that selenium commands come with a minimum?
- How can you tell the differences in the Absolute pathway as well as Relative Path?
- What's the distinction in Assert or Verify declarations within Selenium?
- What are the points of verification that are in Selenium?
- Define Implicit wait, Explicit wait, and Fluent
- Can Selenium manage windows-based pop-ups?
- What's the definition of an Object Repository?
- What is the main difference between obtainwindowhandle() as well as the getwindowhandles ()?
- What are the various types of Annotations that are used in Selenium?
- What is the main difference in the setsSpeed() or sleep() methods?
- What is the way to retrieve the alert message?
- How do you determine the exact location of an element on the web?
- Why do we use Selenium RC?
- What are the benefits or advantages of Selenium RC?
- Do you have a list of the technical limitations when making use of Selenium RC? Selenium RC?
- What's the reason to utilize the TestNG together with Selenium?
- What Language do you prefer to use to build test case sets in Selenium?
- What are Start and Breakpoints?
- What is the purpose of this capability relevant in relation to Selenium?
- When do you use AutoIT?
- Do you have a reason why you require Session management in Selenium?
- Are you able to automatize CAPTCHA?
- How can we launch various browsers on Selenium?
- Why should you select Selenium rather than QTP (Quick Test Professional)?
Table of content:
- Airbus Interview Questions and Answers: HR/ Behavioral
- Industry/ Company-Specific Airbus Interview Questions
- Airbus Interview Questions and Answers: Aptitude
- Airbus Software Engineer Interview Questions and Answers: Technical
Table of content:
- Importance of Spring Framework
- Spring Interview Questions (Basic)
- Advanced Spring Interview Questions
Table of content:
- C++ Interview Questions and Answers: The Basics
- C++ Interview Questions: Intermediate
- C++ Interview Questions And Answers With Code Examples
- C++ Interview Questions and Answers: Advanced
- Test Your Skills: Quiz Time
Table of content:
- MBA Interview Questions: B.Com Economics
- B.Com Marketing
- B.Com Finance
- B.Com Accounting and Finance
- Business Studies
- Chartered Accountant
Table of content:
- Q1. Please tell us something about yourself/ Introduce yourself to us.
- Q2. Describe yourself in one word.
- Q3. Tell us about your strengths and weaknesses.
- Q4. Why did you apply for this job/ What attracted you to this role?
- Q5. What are your hobbies?
- Q6. Where do you see yourself in five years OR What are your long-term goals?
- Q7. Why do you want to work with this company?
- Q8. Tell us what you know about our organization
- Q9. Do you have any idea about our biggest competitors?
- Q10. What motivates you to do a good job?
- Q11. What is an ideal job for you?
- Q12. What is the difference between a group and a team?
- Q13. Are you a team player/ Do you like to work in teams?
- Q14. Are you good at handling pressure/ deadlines?
- Q15. When can you start?
- Q16. How flexible are you regarding overtime?
- Q17. Are you willing to relocate for work?
- Q18. Why do you think you are the right candidate for this job?
- Q19. How can you be an asset to the organization?
- Q20. What is your salary expectation?
- Q21. How long do you plan to remain with this company?
- Q22. What is your objective in life?
- Q23. Would you like to pursue your Master's degree anytime soon?
- Q24. How have you planned to achieve your career goal?
- Q25. Can you tell us about your biggest achievement in life?
- Q26. What was the most challenging decision you ever made?
- Q27. What kind of work environment do you prefer to work in?
- Q28. What is the difference between a smart worker and a hard worker?
- Q29. What will you do if you don't get hired?
- Q30. Tell us three things that are most important for you in a job.
- Q31. Who is your role model and what have you learned from him/her?
- Q32. In case of a disagreement, how do you handle the situation?
- Q33. What is the difference between confidence and overconfidence?
- Q34. If you have more than enough money in hand right now, would you still want to work?
- Q35. Do you have any questions for us?
- Interview Tips for Freshers
Table of content:
- Tell me about yourself
- What are your greatest strengths?
- What are your greatest weaknesses?
- Tell me about something you did that you now feel a little ashamed of
- Why are you leaving (or did you leave) this position??
- 15+ resources for preparing most-asked interview questions
Table of content:
- CoCubes Interview Process Overview
- Common CoCubes Interview Questions
- Key Areas to Focus on for CoCubes Interview Preparation
- Conclusion
- Frequently Asked Questions (FAQs)
Table of content:
- Data Analyst Interview Questions With Answers
- About Data Analyst
Bookmark These Morgan Stanley Interview Questions With Answers (2025)
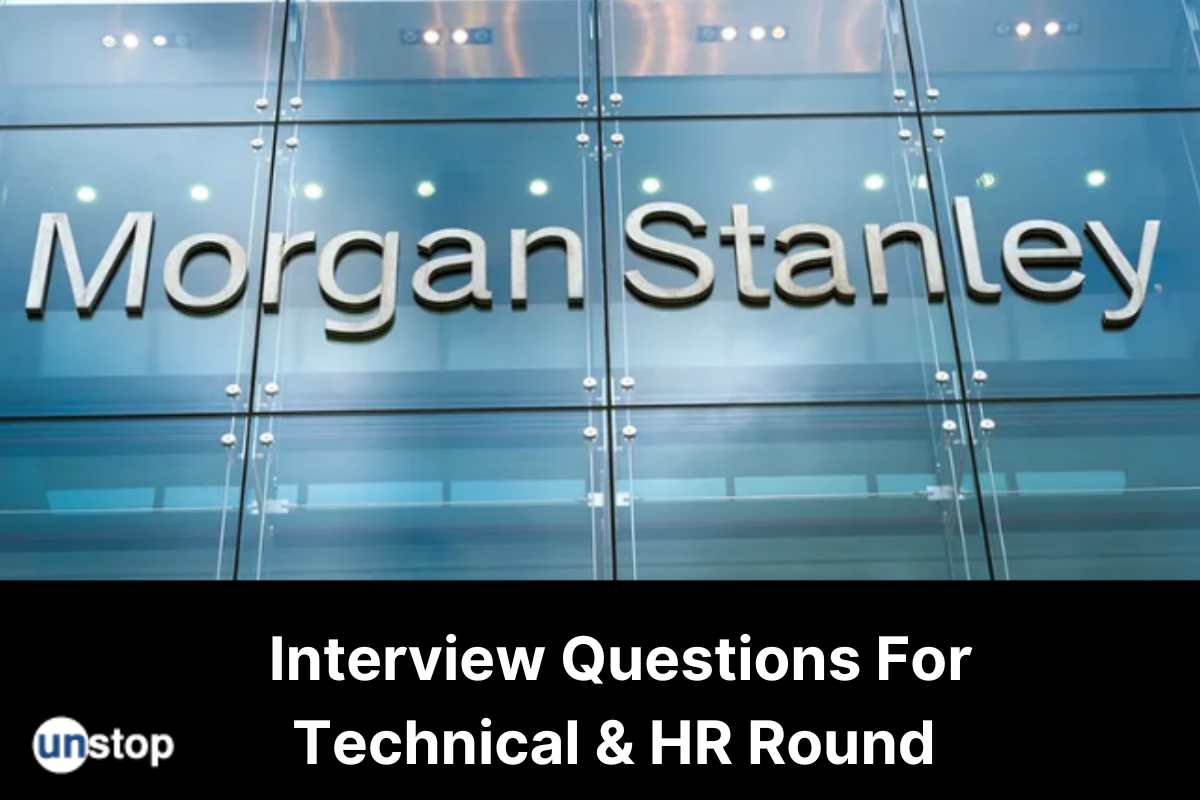
Morgan Stanley, one of the biggest banks and wealth management firms, is a brilliant organization to work for any qualified candidates with high career ambitions. You can land a job role of your choice at this renowned organization in the finance industry after clearing some challenging rounds of interviews with their recruitment team.
Here we are sharing some technical and HR questions that are frequently asked during Morgan Stanley interviews to help with your preparation.
Morgan Stanley's Recruitment Process
Morgan Stanley seeks bright, focused, analytical, hardworking, and intellectually curious individuals who have good interpersonal abilities for graduate roles. Apart from technical roles for engineering graduates, the company can be a great place for individuals wanting to make a career in investment banking, trading, or taking up various managerial roles.
Morgan Stanley also provides students with a wonderful internship program, in which interns are provided with outstanding training, incredible exposure to the senior leaders of the company, and many opportunities to network with other professionals in the field.
The Morgan Stanley recruitment process comprises a total of four rounds, which every single candidate has to go through. These are:
- Screening round: Tests the analytical and problem-solving skills of the candidates.
- Writing/coding test: To assess the coding skills of the candidates. The level of difficulty of this round can be from moderate to hard.
- Technical interview: Tests the candidates' breadth of knowledge.
- HR round: To assess interpersonal and communication skills.
Below are some sample questions for technical and HR rounds that will help you in your interview prep.
Morgan Stanley Interview Questions for Technical Round
Here is a list of the common interview questions that you must prepare before appearing for the Morgan Stanley interview:
Q1. What is the use of abstract class?
In Java, an abstract class is a class that cannot be instantiated directly but can be used as a base class for other classes. Abstract classes are used to provide a common interface and shared functionality for a group of related classes.
Here are some of the main uses of abstract classes in Java:
-
Providing a common interface: An abstract class can define a set of methods that must be implemented by any concrete subclass. This allows the abstract class to provide a common interface that all subclasses can use, while allowing each subclass to implement its own behavior.
-
Sharing code: An abstract class can define common methods and fields that are shared by all subclasses. This can help to reduce code duplication and make the code easier to maintain.
-
Encapsulating implementation details: An abstract class can provide a level of abstraction that hides the implementation details of the class from the user. This can make the code easier to use and reduce the risk of errors.
-
Enforcing a contract: An abstract class can define a set of rules or constraints that must be followed by any concrete subclass. This can help to ensure that the subclasses behave correctly and consistently.
Here's an example of an abstract class in Java:
abstract class Shape {
protected int x;
protected int y;
public Shape(int x, int y) {
this.x = x;
this.y = y;
}
public abstract void draw();
}
class Circle extends Shape {
private int radius;
public Circle(int x, int y, int radius) {
super(x, y);
this.radius = radius;
}
public void draw() {
System.out.println("Drawing a circle at (" + x + "," + y + ") with radius " + radius);
}
}
class Square extends Shape {
private int side;
public Square(int x, int y, int side) {
super(x, y);
this.side = side;
}
public void draw() {
System.out.println("Drawing a square at (" + x + "," + y + ") with side " + side);
}
}
public class Example {
public static void main(String[] args) {
Shape[] shapes = new Shape[2];
shapes[0] = new Circle(10, 10, 5);
shapes[1] = new Square(20, 20, 10);
for (Shape shape : shapes) {
shape.draw();
}
}
}
In this example, we define an abstract class called that has two fields ( and ) and an abstract method . We then define two concrete subclasses of ( and ) that implement the method in their own way.
We can then create an array of objects and loop through them, calling the method on each one. This allows us to treat the different shapes as a single type () even though they have different behavior.
Q2. What is an action class?
In Java, an action class is a type of class that encapsulates an action that can be performed in a GUI application. An action can be anything from clicking a button to selecting a menu item or pressing a keyboard shortcut.
The javax.swing.Action interface defines the standard contract for an action, which includes methods for getting and setting the name, description, and icon of the action, as well as methods for enabling or disabling the action and for performing the action itself.
To create an action in a Java GUI application, you typically create a class that implements the Action interface and provides an implementation of the actionPerformed() method. This method is called when the action is triggered, and it typically performs some action such as displaying a dialog box or updating the contents of a text field.
Here's an example of how to create and use an action class in a Java Swing application:
import javax.swing.AbstractAction;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import java.awt.event.ActionEvent;
public class ExampleAction extends AbstractAction {
public ExampleAction() {
super("Example Action");
}
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("Example Action performed");
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("Example Action");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
JButton button = new JButton(new ExampleAction());
panel.add(button);
frame.getContentPane().add(panel);
frame.pack();
frame.setVisible(true);
});
}
}
Q3. What is the StringBuilder class in Java?
The class in Java is a mutable sequence of characters. It is part of the package and was introduced in Java 1.5 as a replacement for the earlier class.
The class allows you to create and manipulate strings dynamically, by appending, inserting, or deleting characters from the string. Unlike the class, which creates a new string object every time you modify it, the class modifies the same string object, which can be more efficient if you are working with large strings.
Here are some of the methods provided by the class:
- : adds the specified string to the end of the current string
- : inserts the specified string at the specified position in the current string
- : deletes the characters between the specified start and end indexes in the current string
- : replaces the characters between the specified start and end indexes in the current string with the specified string
- : reverses the order of the characters in the current string
Here's an example of how to use the class to create and manipulate a string:
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append(" ");
sb.append("world");
sb.insert(5, ",");
sb.deleteCharAt(11);
System.out.println(sb.toString()); // prints "Hello, world"
In this example, we create a new object and use the method to add the words "Hello" and "world" to it. We then use the method to insert a comma after the fifth character and the method to remove the twelfth character (the space between "Hello" and "world"). Finally, we use the method to convert the object to a object, which we print to the console. The output of this program is "Hello, world".
Q4. How do I make a thread-safe class?
Making a class thread-safe means ensuring that it can be safely accessed and modified by multiple threads at the same time, without causing any unexpected behavior or data corruption. Here are some common techniques for making a class thread-safe:
-
Synchronization: You can use the keyword to ensure that only one thread at a time can execute a particular block of code or method. For example, you can synchronize access to critical sections of your class's code by using the keyword on methods that modify shared data.
-
Locking: You can use the interface and its implementations, such as , to create explicit locks that can be used to synchronize access to shared data.
-
Atomic variables: You can use classes like , , and to perform atomic operations on shared variables, without the need for synchronization.
-
Immutable data: You can design your class so that its data is immutable, meaning that once it is created, it cannot be modified. Immutable data is inherently thread-safe, as there is no need to synchronize access to it.
-
Thread-local storage: You can use the class to create variables that are unique to each thread, avoiding the need for synchronization altogether.
Q5. Define array elements.
A sequence of values is what we refer to as an array and the individual values in an array are referred to as its elements. You are free to create an array out of integers, doubles, or any other kind, but all of the values contained in an array must be of the same type. Declaring a variable with an array type is the first step in the process of creating an array; the next step is to create the array itself.
Q6. What is the sorted array in C?
In C, a sorted array is an array where the elements are arranged in ascending or descending order. Sorting is the process of arranging the elements of an array in a specific order.
There are different sorting algorithms that can be used to sort an array in C, such as bubble sort, selection sort, insertion sort, merge sort, quicksort, and others.
For example, to sort an array in ascending order using the bubble sort algorithm, you can use the following code:
#include <stdio.h>
void bubbleSort(int arr[], int n) {
int i, j, temp;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// swap arr[j] and arr[j+1]
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
int arr[] = {5, 2, 8, 12, 1};
int n = sizeof(arr) / sizeof(arr[0]);
bubbleSort(arr, n);
printf("Sorted array in ascending order: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
In this example, we define a function called that implements the bubble sort algorithm to sort the given array in ascending order. We then call this function from the function, passing the array and its size as arguments. Finally, we print the sorted array using a loop.
Also Read: Coding Algorithms Will Help You Crack Any Interview!
Q7. What is selenium scenario-based testing?
Selenium is a popular tool for automating web browser interactions and is often used for functional and regression testing of web applications. Scenario-based testing is a type of testing where test scenarios are derived from real-world usage of the application.
Selenium scenario-based testing involves creating and running automated tests that simulate real-world scenarios of how users interact with the web application. This approach can help ensure that the application is working as expected and meets the user's needs.
To perform scenario-based testing with Selenium, you would typically follow these steps:
-
Identify user scenarios: Identify the scenarios that users are likely to perform on the web application. These scenarios can be based on user stories, use cases, or other requirements documents.
-
Create test cases: Create automated test cases using Selenium that simulate the user scenarios. Each test case should cover a specific scenario, and should be designed to test a specific feature or functionality of the application.
-
Run the tests: Run the automated tests to verify that the application behaves as expected. Use the test results to identify any defects or issues that need to be addressed.
-
Refine the tests: Refine the automated tests as necessary to improve their accuracy and effectiveness. This may involve modifying the test cases, adjusting the test data, or changing the test environment.
-
Repeat the process: Continue to repeat the process of scenario-based testing, refining the tests as necessary, until the application is stable and meets the desired quality standards.
Scenario-based testing with Selenium can be an effective way to ensure that web applications meet the needs of users and perform as expected in a variety of real-world scenarios. It can also help identify and address defects early in the development cycle, saving time and resources in the long run.
Q8. What are the types of indexes available?
In database systems, indexes are used to improve the performance of queries by allowing faster access to data. There are several types of indexes available, including:
-
Primary index: A primary index is used to uniquely identify records in a table. It is typically created on the primary key of the table, which is a column or set of columns that uniquely identify each record. Primary indexes are usually implemented as a clustered index, which means that the data is physically stored on disk in the order of the index.
-
Secondary index: A secondary index is used to speed up access to data in a table based on columns other than the primary key. It can be created on one or more columns, and allows for faster retrieval of data based on the values in those columns. Secondary indexes are usually implemented as non-clustered indexes, which means that they are stored separately from the data and provide a reference to the data.
-
Clustered index: A clustered index is a type of index that determines the physical order of data in a table. It is typically created on the primary key of the table, and is used to speed up access to data based on that key. Because the data is physically stored in the order of the index, clustered indexes can improve the performance of range queries.
-
Non-clustered index: A non-clustered index is a type of index that is used to speed up access to data based on columns other than the primary key. Unlike clustered indexes, non-clustered indexes do not affect the physical order of the data in the table. They are stored separately from the data and provide a reference to the data.
-
Unique index: A unique index is a type of index that ensures that the values in a column or set of columns are unique. It is similar to a primary index, but can be created on non-primary key columns.
-
Full-text index: A full-text index is a type of index that allows for faster searching of text-based data, such as documents or web pages. It allows for more sophisticated searches than traditional indexes, such as partial matches or fuzzy matching.
These are some of the commonly used types of indexes available in database systems. The choice of index type will depend on the specific needs of the application and the characteristics of the data being stored.
Q9. What is the difference between a list and a tuple?
In Python, both lists and tuples are used to store a collection of items, but there are some differences between the two:
-
Mutability: Lists are mutable, which means that you can add, remove, or modify items in a list after it has been created. Tuples, on the other hand, are immutable, which means that once a tuple has been created, you cannot add, remove, or modify items in it.
-
Syntax: Lists are enclosed in square brackets [] and each item is separated by a comma. Tuples, on the other hand, are enclosed in parentheses () and each item is also separated by a comma.
-
Use case: Lists are typically used to store collections of items that may change over time, such as a list of shopping items. Tuples, on the other hand, are typically used to store collections of items that should not change over time, such as a pair of coordinates representing a location.
-
Performance: Tuples are generally faster than lists because they are immutable, which means that they take up less memory and can be accessed more quickly. However, if you need to add or remove items frequently, or if you need to modify the items in the collection, then a list would be a better choice.
In summary, the main difference between lists and tuples in Python is that lists are mutable and tuples are immutable. Lists are typically used to store collections of items that may change over time, while tuples are typically used to store collections of items that should not change over time.
Also Read: Everything You Need To Know About Programming In Python!
Q10. What is the difference between severity and priority?
In software testing, severity and priority are both used to indicate the level of impact of a defect or issue, but they refer to different aspects of the defect:
-
Severity: Severity refers to the degree of impact that a defect has on the system or application being tested. It is usually categorized as critical, high, medium, or low. A defect that is classified as critical has the highest severity and represents a complete failure of the system, while a defect that is classified as low represents a minor issue that does not affect the overall functionality of the system.
-
Priority: Priority refers to the urgency with which a defect needs to be fixed. It is usually categorized as high, medium, or low. A defect that is classified as high priority needs to be fixed as soon as possible, while a defect that is classified as low priority can be fixed later, after more important defects have been resolved.
In summary, severity and priority are both used to classify defects in software testing, but they represent different aspects of the defect. Severity refers to the impact of the defect on the system, while priority refers to the urgency with which the defect needs to be fixed.
Q11. What is the difference between sleep and yield in Java?
In Java, both sleep and yield are methods that are used to pause the execution of a thread, but there are some differences between the two:
-
Functionality: The sleep method is used to suspend the execution of the current thread for a specified period of time, while the yield method is used to temporarily pause the execution of the current thread and allow other threads to execute.
-
Time: The sleep method requires a specific amount of time to be specified, and the thread will be suspended for that exact amount of time. The yield method does not require a specific time to be specified, and the thread may only be paused for a short period of time before it resumes execution.
-
Usage: The sleep method is used when you want to pause a thread for a specific amount of time, such as when you need to wait for a resource to become available. The yield method is typically used when you want to give other threads a chance to execute, but you don't need to pause the current thread for a specific amount of time.
In summary, sleep is used to pause the execution of a thread for a specific amount of time, while yield is used to temporarily pause the execution of a thread and allow other threads to execute. Sleep requires a specific time to be specified, while yield does not.
Q12. What is limited memory?
Limited memory refers to the finite amount of memory available to a computer system or electronic device for storing and processing data. This can include physical memory such as RAM (Random Access Memory) or virtual memory managed by the operating system.
When a system runs out of memory, it can experience performance issues such as slowing down, freezing, or crashing. This can be especially problematic for resource-intensive applications such as video editing or gaming.
To optimize memory usage, operating systems and applications often employ various techniques such as caching, compression, and memory swapping to move data between physical and virtual memory. However, these techniques may also have their own limitations and trade-offs in terms of performance and reliability.
Q13. What are memory constraints?
Memory constraints refer to limitations on the amount of memory that can be allocated or used by a program, application, or system. These constraints can be imposed by the hardware or software environment and can have a significant impact on system performance and functionality.
Hardware memory constraints are typically determined by the amount of physical memory available on the system. This can be influenced by factors such as the number of memory slots, the type of memory modules supported, and the maximum amount of memory that can be installed. For example, a computer with only 4GB of RAM may struggle to run memory-intensive applications such as virtual machines or large databases.
Software memory constraints can be more complex and are often determined by the programming language, operating system, or application framework being used. Some programming languages and frameworks have more efficient memory management techniques than others, which can help reduce memory usage and improve performance. Operating systems and applications may also have memory usage limits or quotas, which can prevent programs from using too much memory and causing system instability.
In general, memory constraints can be a significant challenge for developers and system administrators, as they must balance performance requirements with available resources and technical limitations. Effective memory management techniques such as memory profiling, garbage collection, and memory pooling can help optimize memory usage and improve system performance.
Q14. What is meant by typecasting?
In Java, typecasting is the process of converting a variable of one data type to another. This can be useful in situations where you need to perform operations on data that may be stored in different formats.
Java provides two types of typecasting: explicit and implicit. Explicit typecasting requires you to specify the target data type using a casting operator, whereas implicit typecasting is done automatically by the Java runtime.
Here is an example of explicit typecasting in Java:
int num1 = 10;
double num2 = 2.5;
double result = num1 * (double) num2;
In this example, we are explicitly casting the variable to a data type, so that we can multiply it with the variable, which is already a .
Here is an example of implicit typecasting in Java:
int num1 = 10;
double num2 = 2.5;
double result = num1 * num2;
In this example, Java automatically converts the variable from an to a in order to perform the multiplication with .
It's important to note that typecasting can result in data loss or unexpected results if not used carefully. For example, casting a floating-point number to an integer will truncate the decimal portion of the number. Therefore, it's important to test your code thoroughly to ensure that typecasting is being used correctly and does not introduce any errors.
Q15. What is garbage collection in Java?
In Java, garbage collection is the process of automatically freeing up memory that is no longer being used by a program. When an object is created in Java, it is stored in memory allocated by the JVM (Java Virtual Machine). As the program runs, objects may become obsolete or unreachable, meaning they are no longer being used or referenced by the program.
Garbage collection is the process by which the JVM identifies and removes these unused objects from memory, freeing up space that can be used for other objects. This process is automatic and transparent to the developer, meaning that you do not have to manually manage memory allocation and deallocation in Java as you would in languages like C or C++.
The garbage collection process in Java works by periodically scanning memory to identify objects that are no longer being used. Once these objects are identified, the JVM marks them as "garbage" and frees up the memory they were occupying. This process is efficient and optimized for performance so that it does not impact the overall speed of the program.
There are different algorithms that can be used for garbage collection in Java, such as the mark-and-sweep algorithm and the copying algorithm. The algorithm used depends on the JVM implementation and the specific needs of the program.
Overall, garbage collection in Java is an important feature that makes it easier to manage memory allocation and helps to prevent memory leaks and other issues that can arise from manual memory management.
Unlock endless job & internship opportunities!
Q16. What is the condition of starvation?
In computer science, starvation is a condition where a process is unable to make progress because it is not receiving the resources it needs to continue executing. This can occur when resources such as CPU time, memory, or I/O operations are monopolized by other processes or threads, leaving insufficient resources for the starved process to make progress.
Starvation can cause significant problems in a system, as it can lead to reduced performance and even system crashes. For example, if a process is waiting for a critical resource such as a database connection or network access and is unable to obtain it due to contention from other processes, it may become unresponsive or crash.
Starvation can be mitigated through various techniques, such as resource allocation policies that ensure fair access to resources among competing processes or threads. One such technique is to implement priority scheduling, where higher-priority processes are given precedence over lower-priority ones, ensuring that critical processes are able to obtain the resources they need to make progress.
It's important for developers and system administrators to be aware of the potential for starvation in their systems and to implement appropriate measures to prevent it from occurring. This can help to ensure that their systems operate reliably and efficiently, even under high loads and resource contention.
Q17. What are some of the more practical applications of volatile modifiers?
In Java, the modifier is used to indicate that a variable's value may be modified by multiple threads simultaneously. When a variable is marked as , the JVM ensures that any changes to the variable's value are immediately visible to all other threads that access the variable, preventing the visibility and consistency issues that can arise with multithreaded access to non-volatile variables.
Some practical applications of the modifier in Java include:
-
Thread synchronization: Volatile variables can be used as a lightweight alternative to locking mechanisms such as blocks or objects. By marking a shared variable as , multiple threads can safely read and write to the variable without the need for explicit synchronization.
-
Caching optimization: Volatile variables can be used to ensure that the most up-to-date value of a variable is always used, even if the variable is cached locally by a thread. This can be useful for optimizing performance in cases where a variable's value is frequently accessed but changes infrequently.
-
Event signaling: Volatile variables can be used to signal events between threads. For example, a thread can wait for a volatile variable to be set by another thread to indicate that some work has been completed.
-
Cross-thread communication: Volatile variables can be used as a communication mechanism between threads. For example, one thread can set a volatile variable to indicate that it has completed some work, while another thread can read the variable to determine when the work is complete.
Overall, the modifier can be a useful tool for managing shared state in multithreaded Java applications, helping to prevent visibility and consistency issues that can arise with concurrent access to non-volatile variables.
Q18. What exactly is involved in a busy spin?
In computer science, a busy spin (also known as busy waiting or spinning) is a programming technique used to wait for a condition to be true by repeatedly checking the condition in a loop. In a busy spin, the loop continuously checks the condition and does not yield the CPU to other threads or processes during the check. This means that the thread executing the busy spin is actively consuming CPU cycles, even when there is no work to be done.
Busy spins can be used in situations where it is expected that the condition will become true quickly, such as waiting for a lock to become available in a multithreaded program. By using a busy spin instead of waiting for a lock, the waiting thread can quickly acquire the lock as soon as it becomes available, avoiding the overhead of suspending and resuming the thread.
However, busy spins can also be problematic if the condition being checked takes a long time to become true or if there are many threads performing busy spins simultaneously. In such cases, the CPU cycles consumed by the busy spins can lead to reduced performance and increased power consumption.
To avoid the problems associated with busy spins, other waiting techniques such as wait-notify mechanisms or blocking methods can be used instead. These techniques allow the waiting thread to yield the CPU to other threads or processes while waiting for the condition to become true, reducing CPU consumption and improving performance.
Q19. Why is it not possible to change a string in Java?
In Java, strings are immutable, which means that once a string object is created, its value cannot be changed. When a string is "modified" in Java, a new string object is created with the modified value instead of modifying the original string object.
There are a few reasons why strings are immutable in Java:
-
Thread safety: Because strings are immutable, they can be shared between threads without the risk of data corruption from multiple threads modifying the same object simultaneously.
-
Caching and optimization: Because strings are immutable, they can be cached and reused by the JVM, which can improve performance in some cases.
-
Security: Because strings are immutable, they cannot be modified by malicious code, which can help prevent security vulnerabilities such as buffer overflow attacks.
While it may seem inconvenient that strings cannot be modified directly in Java, there are several workarounds that can be used to achieve similar effects, such as creating a new string with the modified value or using a or object to build a new string dynamically.
Q20. What is a 'comparator' when it comes to Java?
In Java, a is an interface that defines a way to compare two objects of a certain type. It is often used to sort collections of objects based on certain criteria, such as alphabetical order, numerical value, or some other user-defined order.
The interface has a single method called which takes two objects of the same type as parameters and returns an integer value indicating their relative order. If the first object is less than the second object, the method returns a negative integer. If they are equal, the method returns 0. If the first object is greater than the second object, the method returns a positive integer.
Here's an example of how to use a to sort a collection of objects in reverse alphabetical order:
import java.util.*;
public class Example {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("apple");
list.add("banana");
list.add("orange");
list.add("grape");
Comparator<String> comparator = new Comparator<String>() {
public int compare(String s1, String s2) {
return s2.compareTo(s1);
}
};
Collections.sort(list, comparator);
System.out.println(list);
}
}
Q21. What exactly is this thing called inheritance?
In object-oriented programming, inheritance is a mechanism that allows a new class to be based on an existing class, inheriting its properties and behavior. The existing class is known as the superclass or parent class, while the new class is known as the subclass or child class.
Inheritance is a fundamental concept in object-oriented programming and is used to model relationships between objects. It allows subclasses to reuse the code and behavior of their parent class, while also providing the ability to extend or modify that behavior.
When a subclass inherits from a parent class, it automatically has access to all of the public and protected methods and fields of the parent class, which can be used in the subclass as if they were defined in the subclass itself. The subclass can also add its own methods and fields and can override or modify the behavior of methods inherited from the parent class.
For example, suppose you have a class called that represents a generic vehicle and a class called that represents a car. You could use inheritance to create the class as a subclass of the class as follows:
public class Vehicle {
protected int speed;
public void accelerate() {
speed += 10;
}
public void brake() {
speed -= 10;
}
}
public class Car extends Vehicle {
private boolean isRunning;
public void start() {
isRunning = true;
}
public void stop() {
isRunning = false;
}
public boolean isRunning() {
return isRunning;
}
}
In this example, the class extends the class, inheriting its field and and methods. The class adds its own field and and methods.
Inheritance allows you to create more specialized classes that are based on more generic classes, without having to rewrite the same code multiple times. It also allows you to model complex relationships between objects, and to design your code in a more modular and reusable way.
Q22. Why does Java not support multiple inheritances?
Java does not support multiple inheritances because its developers wanted to minimize Java's linguistic burdens and keep the programming language as straightforward as possible. Take into consideration the possibility that there are three different classes labeled A, B, and C. A and B classes are passed down to the C class by inheritance. If A and B classes both utilize the same method as well as you call it from an object of a child class, then there's going to be uncertainty over which method to call because both the A and B classes will have the same method.
If you inherit two different classes in Java, then you will receive a compile-time error. This is because Java considers compile-time errors to be preferable to runtime errors. Therefore, there will be a compilation time error regardless of whether or not you use the same method or a different one.
Thus we can say that Java was designed without support for multiple inheritances to avoid the problems associated with it, such as the diamond problem.
The diamond problem occurs when a class inherits from two or more classes that have a common superclass. This creates a diamond-shaped inheritance hierarchy, where the subclass inherits the methods and fields of the common superclass multiple times, leading to ambiguity and potential conflicts.
To avoid the diamond problem, Java uses single inheritance and interfaces. Single inheritance allows a class to inherit from one superclass, while interfaces define a set of methods that a class can implement. This approach provides the benefits of inheritance without the potential conflicts that can arise from multiple inheritance.
Another reason why Java does not support multiple inheritances is to maintain the simplicity and readability of the language. Multiple inheritances can lead to complex and hard-to-read code, especially when inheritance hierarchies become deep and complex.
Instead, Java provides other mechanisms to achieve similar functionality as multiple inheritance, such as composition and delegation. With composition, an object can contain other objects as part of its state, while delegation involves forwarding method calls to another object that performs the actual work.
Q23. What does aggregation mean?
In object-oriented programming, aggregation is a way to represent a "has-a" relationship between objects. Aggregation is a form of association, where one object is composed of or contains other objects as part of its state.
Aggregation differs from composition in that the contained objects can exist independently of the containing object, and can be shared between multiple objects. In other words, the contained objects have their own identity and lifecycle, and are not owned by the containing object.
For example, suppose you have a class called that represents a department in a company, and a class called that represents an employee. You could use aggregation to represent the fact that a department has many employees as follows:
public class Department {
private List<Employee> employees;
public Department() {
employees = new ArrayList<>();
}
public void addEmployee(Employee employee) {
employees.add(employee);
}
// Other methods for managing employees in the department
}
In this example, the class has a private field called that contains a list of objects. The class has a method called that adds an object to the list.
Aggregation allows you to represent relationships between objects in a flexible and extensible way. It can make your code more modular and maintainable, since objects can be reused and shared between multiple objects. Aggregation can also make your code more scalable since you can add or remove objects from a collection without affecting the rest of the system.
Q24. What does composition mean?
In object-oriented programming, composition is a way to combine multiple classes or objects to create more complex behavior. Composition is a form of aggregation, where an object contains other objects as part of its state.
In composition, one object owns or contains other objects, and delegates some of its behavior to them. The contained objects can be thought of as parts of the whole, and are responsible for implementing specific aspects of the overall behavior.
For example, suppose you have a class called that represents a car, and a class called that represents an engine. You could use composition to create a object that contains an object as follows:
public class Car {
private Engine engine;
public Car() {
engine = new Engine();
}
public void start() {
engine.start();
}
public void stop() {
engine.stop();
}
}
In this example, the class contains an instance of the class as a private field. The class delegates the behavior of starting and stopping the engine to the class, by calling the and methods on the field.
Composition allows you to create more complex behavior by combining simpler objects or classes. It can make your code more modular, since each object or class can be designed and tested independently. Composition can also make your code more flexible and maintainable, since you can change the behavior of an object by replacing the objects it contains.
Q25. What is the final variable?
In Java programming, a final variable is a variable whose value cannot be changed once it has been assigned.
Declaring a variable as final is done using the "final" keyword. Once a final variable has been assigned a value, it cannot be reassigned to a different value. Attempting to assign a new value to a final variable will result in a compiler error.
Final variables are often used to define constants, which are values that do not change throughout the execution of a program. By declaring a variable as final, you indicate that the value should not be changed, making it easier to reason about the behavior of your program.
Here's an example of how to declare a final variable:
final int MAX_VALUE = 100;
In this example, the variable "MAX_VALUE" is declared as final, and assigned the value 100. Once the value has been assigned, it cannot be changed.
It's important to note that final variables can only be assigned a value once. If you need to initialize a final variable with a value that is not known at compile time, you can use an initializer block to assign the value. For example:
final int MAX_VALUE;
{
// Assign a value to MAX_VALUE at runtime
MAX_VALUE = computeMaxValue();
}
In this example, the value of "MAX_VALUE" is computed at runtime using a method called "computeMaxValue()", and assigned to the final variable using an initializer block. Once the value has been assigned, it cannot be changed.
Q26. What is an anonymous inner class?
In Java programming, an anonymous inner class is a class that is defined and instantiated in a single expression, without giving the class a name.
Anonymous inner classes are typically used as a way to define a class that implements an interface or extends a class, without having to create a separate class file for the implementation. Instead, you can define the class inline, within the context where it is used.
The syntax for creating an anonymous inner class is as follows:
new SuperClassOrInterface() {
// Class body goes here
}
Where is the class or interface that the anonymous inner class is extending or implementing, and the class body contains the implementation of the class or interface.
For example, suppose you have an interface called with a single method . You can create an anonymous inner class that implements the interface as follows:
Runnable r = new Runnable() {
public void run() {
System.out.println("Hello, world!");
}
};
In this example, the anonymous inner class is implementing the interface and providing an implementation for the method. The anonymous inner class is assigned to a variable of type , and can be used just like any other object of that type.
Anonymous inner classes are often used in event handling, where you want to define a class that implements an event listener interface, but you don't need to reuse the class in other contexts. Anonymous inner classes can also be used to create simple adapters or decorators on-the-fly, without having to create a separate class for each adaptation.
Q27. What are Java's wrapper classes and why are they necessary?
In Java programming, wrapper classes are classes that provide an object-oriented representation of primitive data types, such as int, float, boolean, etc. The wrapper classes are:
- Integer
- Float
- Double
- Boolean
- Byte
- Short
- Long
- Character
Wrapper classes are necessary in Java because primitive data types are not objects and do not have methods or other object-oriented features. For example, you cannot call methods on an int variable or pass an int variable as an argument to a method that expects an object.
Wrapper classes provide a way to work with primitive data types as objects, by providing methods for performing common operations on the data, such as converting between data types, comparing values, and performing arithmetic operations. For example, the Integer class provides methods for parsing strings into integers, converting integers to strings, and performing arithmetic operations on integers.
Wrapper classes are also used in Java collections and other APIs that require objects rather than primitive data types. For example, you cannot use an int as a key in a HashMap, but you can use an Integer object.
In addition, wrapper classes provide a way to work with nullable values, by allowing null values to be assigned to the wrapper object. For example, an Integer variable can have a value of null, whereas an int variable cannot.
Finally, autoboxing and unboxing are features in Java that allow automatic conversion between primitive data types and their corresponding wrapper classes. For example, you can assign an int to an Integer variable, and Java will automatically convert the int to an Integer object (autoboxing). Similarly, you can assign an Integer object to an int variable, and Java will automatically extract the int value from the Integer object (unboxing). This makes it easier to work with both primitive data types and objects in Java.
Q28. What is meant by the term 'string pool' in Java?
In Java programming, the string pool is a special memory area in the heap that stores a pool of string literals, which are shared by multiple string variables.
When you create a string literal in Java, such as "Hello, world!", the Java compiler automatically stores the string in the string pool, rather than creating a new object in the heap for each occurrence of the same string literal. This means that if you create multiple string variables that contain the same string literal, they will all reference the same object in the string pool, rather than creating separate objects in the heap for each variable.
Using the string pool can improve the performance and memory usage of your Java program, as it reduces the number of objects that need to be created and stored in the heap. Additionally, it can simplify your code, as you can use the "==" operator to compare string literals, since they are stored in the same location in memory.
It's important to note that not all strings are stored in the string pool. Strings that are created using the "new" keyword, such as String str = new String("Hello, world!"), are stored in the heap and not in the string pool, even if they contain the same text as a string literal. To add a string to the string pool, you can use the "intern()" method, which returns a canonical representation of the string. For example, String str = "Hello, world!".intern() adds the string "Hello, world!" to the string pool and returns a reference to the shared object.
Q29. What is a servlet?
In Java programming, a servlet is a Java class that runs on a web server and handles HTTP requests and responses. A servlet can dynamically generate web pages, receive and process user input, interact with databases and other resources, and perform other web-related tasks.
Servlets are typically used to build web applications that follow the Model-View-Controller (MVC) architecture, where the servlet acts as the controller that receives user requests, performs business logic, and updates the model or interacts with other resources, and then generates the appropriate response to send back to the user.
Servlets are part of the Java Servlet API, which is a standard Java interface for developing web applications. The API provides a set of classes and interfaces that define the contract between the web server and the servlet, such as the Servlet interface, the HttpServletRequest and HttpServletResponse interfaces, and other utility classes for handling cookies, sessions, and other web-related tasks.
To use servlets, you typically need to deploy them on a web server that supports the Java Servlet API, such as Apache Tomcat, Jetty, or JBoss. The servlet container (or web container) in the web server is responsible for managing the lifecycle of the servlets, handling incoming requests, and sending responses back to the client.
Q30. What's the difference between the words final, finalize, and finally?
The words "final", "finalize", and "finally" are related to different concepts in Java:
-
"final" is a keyword in Java that is used to declare a variable, a method, or a class as unmodifiable, meaning that their value or implementation cannot be changed once they are initialized or defined. When applied to a variable, the "final" keyword makes the variable a constant, which cannot be reassigned. When applied to a method, the "final" keyword makes the method un-overridable, meaning that it cannot be overridden by a subclass. When applied to a class, the "final" keyword makes the class un-inheritable, meaning that it cannot be subclassed.
-
"finalize" is a method in Java's Object class that is called by the garbage collector when it is about to reclaim an object's memory. The purpose of the "finalize" method is to provide a way for an object to perform some cleanup tasks or release resources before it is destroyed. The "finalize" method is called only once for each object, and its execution is not guaranteed.
-
"finally" is a keyword in Java that is used in a try-catch-finally block to specify a block of code that will always be executed, regardless of whether an exception is thrown or not. The "finally" block is typically used to release resources or perform cleanup tasks that should be executed even if an exception is thrown in the try block.
In summary, "final" is used to declare unmodifiable variables, methods, or classes, "finalize" is a method used for object cleanup before destruction, and "finally" is a keyword used to specify a block of code that will always be executed in a try-catch-finally block.
Q31. What is the compile-time constant when referring to Java?
In Java, a compile-time constant is a constant value that is evaluated at compile time and cannot be changed during the runtime of the program.
A constant in Java is a variable that is declared with the keyword "final" and whose value cannot be changed once it has been assigned. A compile-time constant is a special type of constant that can be evaluated by the compiler at compile-time and can be used in expressions and annotations.
Some examples of compile-time constants in Java include:
- Numeric literals, such as 42, 3.14, or 0b1101
- String literals, such as "Hello, world!"
- Class literals, such as String.class or Integer.class
- Enum constants, such as Color.RED or Direction.NORTH
- Annotation constants, such as @SuppressWarnings("unchecked")
Using compile-time constants can improve the performance of your Java program, as the compiler can optimize the code by replacing references to constants with their values at compile time. Additionally, using compile-time constants can make your code easier to read and maintain, as it helps to make the intent of the code clearer.
Q32. What is meant by the adapter pattern?
The Adapter Pattern is a design pattern that allows incompatible interfaces of two or more classes to work together by creating a bridge between them.
In software engineering, interfaces are used to define the methods and properties of classes that can be accessed by other classes. However, when two classes have incompatible interfaces, it becomes difficult to use them together. The Adapter Pattern solves this problem by creating an adapter class that acts as a bridge between the two incompatible classes, allowing them to work together seamlessly.
The adapter class implements the interface of one of the incompatible classes, while internally using the interface of the other incompatible class to translate the method calls and parameters from one interface to another. This way, the client code that uses the adapter class can interact with both classes without having to know about their incompatible interfaces.
The Adapter Pattern is a commonly used pattern in object-oriented programming and is useful in situations where you need to integrate two systems that have incompatible interfaces, or when you need to reuse existing code that has a different interface than what you require.
Q33. What is a template method pattern?
The Template Method pattern is a behavioral design pattern in which an abstract base class defines the skeleton of an algorithm but delegates some steps to its subclasses. In other words, it provides a framework for defining algorithms and lets subclasses implement certain steps of the algorithm without changing the overall structure.
The Template Method pattern consists of the following components:
-
Abstract class: The abstract class defines the overall algorithm and provides a template method that consists of a series of steps that are executed in a specific order. The template method calls other methods that are either abstract or have default implementations.
-
Concrete classes: The concrete classes are subclasses of the abstract class and provide implementations for the abstract methods or override the default implementations.
Here is an example of the Template Method pattern in Java:
public abstract class AbstractClass {
public void templateMethod() {
// Do step 1
doStep1();
// Do step 2
doStep2();
// Do step 3
doStep3();
}
public abstract void doStep1();
public void doStep2() {
// Default implementation
}
public abstract void doStep3();
}
public class ConcreteClass extends AbstractClass {
@Override
public void doStep1() {
// Implementation for step 1
}
@Override
public void doStep3() {
// Implementation for step 3
}
}
public class Main {
public static void main(String[] args) {
AbstractClass abstractClass = new ConcreteClass();
abstractClass.templateMethod();
}
}
Q34. What is the JDBC connection interface?
In Java, the JDBC (Java Database Connectivity) API provides a standard interface for connecting to and interacting with databases. The JDBC Connection interface is a core part of the JDBC API, and is used to establish a connection to a database and perform various database operations.
The Connection interface is defined in the package, and provides methods for:
-
Establishing a connection to a database: The method is used to establish a connection to a database, and takes a URL, username, and password as arguments.
-
Creating statements: The and methods are used to create Statement and PreparedStatement objects for executing SQL statements against the database.
-
Controlling transactions: The method is used to enable or disable automatic transaction commits, and the and methods are used to manually commit or rollback a transaction.
-
Managing database metadata: The method is used to retrieve information about the database, such as the available tables and columns.
-
Closing the connection: The method is used to close the connection and release any associated resources.
Here is an example code snippet that demonstrates how to use the Connection interface to establish a connection to a MySQL database:
import java.sql.*;
public class JdbcExample {
public static void main(String[] args) {
try {
// Load the MySQL JDBC driver
Class.forName("com.mysql.jdbc.Driver");
// Establish a connection to the database
String url = "jdbc:mysql://localhost:3306/mydb";
String username = "user";
String password = "password";
Connection conn = DriverManager.getConnection(url, username, password);
// Create a Statement object and execute a query
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
// Process the result set
while (rs.next()) {
// Do something with each row of data
}
// Close the connection
conn.close();
}
catch (Exception e) {
e.printStackTrace();
}
}
}
Q35. What are Java packages?
In Java, a package is a mechanism for organizing classes and interfaces into namespaces. Packages provide a way to group related classes together and avoid naming conflicts with classes in other packages.
A package is defined using the keyword at the beginning of a Java source file, followed by the package name, which is a unique identifier separated by periods. For example, defines a package named .
Classes and interfaces within a package are accessed using the fully qualified class name, which includes the package name as a prefix. For example, if the package contains a class named , it can be accessed from another class using the fully qualified name .
Java provides several built-in packages, such as , which contains fundamental classes and interfaces that are automatically imported into every Java program, and , which contains utility classes for working with collections, dates, and other common tasks.
Packages can also be organized into a hierarchical structure, with sub-packages nested within parent packages. For example, a package named would be a sub-package of the package.
Using packages in Java provides several benefits, including:
-
Organizing code: Packages allow code to be organized into logical groups, making it easier to navigate and maintain.
-
Avoiding naming conflicts: Packages help avoid naming conflicts between classes with the same name that exist in different packages.
-
Encapsulation: Packages can be used to control the visibility of classes and interfaces, allowing certain classes to be hidden from outside code.
-
Modularity: Packages allow code to be modularized, making it easier to reuse and combine with other code.
In summary, a package in Java is a mechanism for organizing classes and interfaces into namespaces. Packages provide a way to group related classes together, avoid naming conflicts, control visibility, and improve modularity.
Q36. What is super in Java?
In Java, is a keyword that is used to refer to the parent class of a subclass. It is often used in inheritance, where a subclass inherits properties and methods from its parent class.
The keyword can be used in several ways:
-
Accessing parent class members: When a subclass overrides a method or property of its parent class, the keyword can be used to access the parent class version of the method or property. For example, can be used to call the parent class version of a method that has been overridden in the subclass.
-
Calling parent class constructors: When a subclass is instantiated, its constructor must call the constructor of its parent class using the keyword. This ensures that the parent class constructor is executed before the subclass constructor. For example, can be used to call the default constructor of the parent class, or can be used to call a specific constructor of the parent class with the specified arguments.
-
Referring to parent class type: The keyword can also be used to refer to the parent class type, which can be useful for type casting or method parameter declarations. For example, can be used to get the class object of the parent class, or can be used to declare a method parameter that can accept objects of both the subclass and parent class types.
In summary, is a keyword in Java that is used to refer to the parent class of a subclass. It can be used to access parent class members, call parent class constructors, or refer to the parent class type.
Q37. What is polymorphism and why is it important?
Polymorphism is a fundamental concept in object-oriented programming that allows objects of different classes to be treated as if they were of the same class. It allows different objects to be used interchangeably, as long as they implement a common interface or have a common base class.
Polymorphism is important for several reasons:
-
Code reuse: Polymorphism allows you to write code that can work with objects of different types, which can reduce code duplication and make your code more modular and reusable.
-
Flexibility: Polymorphism allows you to write more flexible and adaptable code, since you can work with objects of different types without having to know their specific implementation details.
-
Extensibility: Polymorphism makes it easier to extend and modify your code, since you can add new classes that implement the same interface or inherit from the same base class, without having to modify existing code.
-
Abstraction: Polymorphism allows you to work with objects at a higher level of abstraction, since you can treat them as if they were all of the same type, regardless of their specific implementation.
There are two main types of polymorphism in object-oriented programming: compile-time (or static) polymorphism, which is implemented through function overloading or operator overloading, and runtime (or dynamic) polymorphism, which is implemented through inheritance and virtual functions.
In summary, polymorphism is a fundamental concept in object-oriented programming that allows objects of different classes to be treated as if they were of the same class. It is important for code reuse, flexibility, extensibility, and abstraction, and is implemented through function overloading, operator overloading, inheritance, and virtual functions.
38. What is meant by the term 'encapsulation'?
Encapsulation is a fundamental concept in object-oriented programming that refers to bundling data and methods together within a single unit, called a class. It is one of the four fundamental principles of object-oriented programming: inheritance, polymorphism, and abstraction.
The main purpose of encapsulation is to hide the internal details of an object from the outside world and to provide a well-defined interface for interacting with the object. This interface consists of a set of public methods and properties that can be accessed and manipulated from outside the class, while the internal data and implementation details are hidden and protected from direct access.
Encapsulation provides several benefits, including:
-
Improved security: By hiding the internal details of an object, encapsulation helps prevent unauthorized access or modification of sensitive data.
-
Easier maintenance: Encapsulation makes it easier to modify the internal implementation of an object without affecting the external interface, which can help reduce the risk of introducing bugs or breaking existing code.
-
Improved code organization: Encapsulation allows code to be organized into modular, reusable components that can be easily combined and extended.
-
Reduced complexity: By limiting the amount of information that needs to be considered at any given time, encapsulation can help reduce the overall complexity of a system.
In summary, encapsulation is a fundamental concept in object-oriented programming that involves bundling data and methods together within a class, and providing a well-defined interface for interacting with the object while hiding its internal implementation details.
Q39. What is meant by the 'function Object() { [native code] } chaining'?
The phrase "function Object() { [native code] } chaining" typically refers to the process of linking together multiple function calls in JavaScript using the dot notation (e.g., ). This is sometimes referred to as method chaining or function chaining.
When a method is called on an object, it typically returns a reference to that object, which allows additional methods to be called on the same object using the dot notation. This can be done repeatedly, creating a chain of method calls that operate on the same object.
For example, suppose you have an object with three methods: , , and . You could call these methods sequentially using dot notation, like this:
myObject.method1().method2().method3();
In this case, the call returns a reference to , which is then used to call , which in turn returns another reference to , allowing to be called on the same object.
Function chaining can be used to write more concise and readable code, as it allows complex operations to be expressed in a single line of code. It is a common technique in many JavaScript libraries and frameworks, such as jQuery and AngularJS, and is widely used in modern web development.
Q40. Can you explain what the chained exception is?
In Java, a chained exception is an exception that is caused by another exception. It is a way of indicating that an error occurred, but that the underlying cause of the error was itself caused by some other error.
When a method throws an exception, it can optionally include another exception as its cause, using the constructor of the new exception that takes a throwable parameter. This creates a chain of exceptions, where each exception in the chain represents a different level of abstraction for the error that occurred.
Chained exceptions are useful because they provide more detailed information about the cause of an error. By following the chain of exceptions, it is possible to trace the root cause of the error back to its original source. This can be very helpful in debugging complex systems, where errors can occur at multiple levels of abstraction.
For example, suppose method A throws an exception because it received invalid input from method B. Instead of just throwing an exception, method A can wrap the original exception from method B in a new exception that provides additional context about what went wrong. This new exception can then be thrown, with the original exception passed as its cause. This creates a chain of exceptions that provides a more detailed picture of what happened and makes it easier to diagnose and fix the problem.
In summary, a chained exception is an exception that is caused by another exception. It is a way of providing more detailed information about the cause of an error and can be very helpful in debugging complex systems.
Q41. Explain what the term 'Java Applet' means.
A Java Applet is a small program written in the Java programming language that can be executed within a web browser. Applets are designed to be embedded within HTML pages and can provide interactive features such as animations, user input forms, and graphical user interfaces.
When a user visits a web page that contains a Java Applet, the applet is downloaded and executed within the user's web browser. This allows the applet to run on multiple platforms without requiring any modifications to the code. Applets are platform-independent because they run within the Java Virtual Machine (JVM), which provides a consistent runtime environment across different operating systems and hardware configurations.
Java Applets were a popular technology in the 1990s and early 2000s for creating interactive web content, but their usage has declined in recent years due to security concerns and the increasing popularity of alternative web technologies such as JavaScript and HTML5.
Q42. Walk us through the final block's significance.
In Java, a final block is a section of code that can be included at the end of a try-catch block. The final block is executed regardless of whether an exception was thrown or not. It is typically used to clean up resources or perform final actions that should be executed no matter what happens in the try-catch block.
The significance of the final block is that it provides a way to ensure that important cleanup or finalization tasks are always executed, even if an exception occurs. For example, if a try block includes code that opens a file, the final block can be used to ensure that the file is always closed, even if an exception is thrown. This is important because leaving files or other resources open can lead to problems such as memory leaks or file corruption.
Another use of the final block is to release resources such as database connections or network sockets. By including this code in the final block, it can be guaranteed that these resources will be properly released, even if an exception occurs that prevents the normal flow of execution.
In summary, the final block is significant because it provides a way to execute important cleanup or finalization tasks that should always be performed, even if an exception occurs. It can help prevent resource leaks and other problems that can arise from leaving resources open or unreleased.
Morgan Stanley Interview Questions for HR Round
Check out some of the frequently asked HR questions and behavioral interview questions.
1. Are you a team player?
2. Do you have any experience in working on any OOPs projects?
3. As a manager, have you ever handled a difficult team or faced a difficult challenge?
4. How do you assess yourself on your leadership skills or leadership potential?
5. Are you a people person?
6. How do you deal with difficult times or stressful situations at work?
7. What has been your biggest challenge so far?
8. How do you deal with criticism or bad feedback?
9. What are the corporate values of Morgan Stanley?
Also Read: 20 Popular Behavioral Interview Questions With Answers 2024
Tips to answer HR or Behavioral questions
- Always keep a positive outlook while framing responses to the questions asked during the HR round. Also, positive body language helps to lay a good impression too.
- It is advisable that you state examples when answering scenario-based questions rather than providing a generic response. So, you may keep a bank of stories ready to be used for various situations. For example, a story about the time you led a difficult team or when you handled a stressful situation.
- You have limited time during the face-to-face interview. Hence, keep your answers short, crisp, and effective. Mock interviews can help you in polishing this skill and train you in handling any tricky interview question.
- Go through the company's website thoroughly to understand its aim, objectives, core values, etc. Also, keep yourself updated about the financial markets, tech trends, etc.
- Be ready with witty responses for ice-breaker questions and brace yourself for potential follow-up questions during the HR interview round.
About Morgan Stanley
Morgan Stanley is among the most prominent investment banks and wealth management companies in the global financial services industry. The American multinational investment bank and financial services company is headquartered in New York City and has offices in 41 countries. The company has more than 75,000 employees with corporations, governments, institutions, and individuals as its clients. Because of the professional exposure and potential for growth that Morgan Stanley offers, the company can be a great workplace for professionals to achieve their career goals.
Suggested Reads:
I am a biotechnologist-turned-content writer and try to add an element of science in my writings wherever possible. Apart from writing, I like to cook, read and travel.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
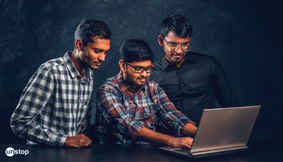
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
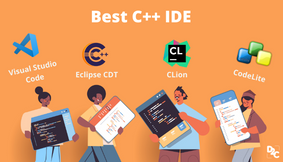
10 Best C++ IDEs That Developers Mention The Most!
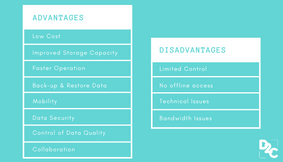
Advantages and Disadvantages of Cloud Computing that you should know!
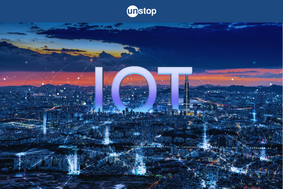
Comments
Add comment